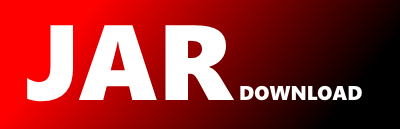
com.hcl.domino.design.DbDesign Maven / Gradle / Ivy
/*
* ==========================================================================
* Copyright (C) 2019-2022 HCL America, Inc. ( http://www.hcl.com/ )
* All rights reserved.
* ==========================================================================
* Licensed under the Apache License, Version 2.0 (the "License"). You may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at .
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
* ==========================================================================
*/
package com.hcl.domino.design;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.Collection;
import java.util.Optional;
import java.util.OptionalInt;
import java.util.Set;
import java.util.function.Consumer;
import java.util.stream.Stream;
import com.hcl.domino.admin.idvault.UserId;
import com.hcl.domino.data.Database;
import com.hcl.domino.data.Database.Action;
import com.hcl.domino.data.DocumentClass;
import com.hcl.domino.design.agent.DesignLotusScriptAgent;
import com.hcl.domino.misc.NotesConstants;
/**
* Provides access to a database's design elements, allowing querying,
* selection,
* and creation.
*/
public interface DbDesign {
@Deprecated
public enum FormOrSubform {
FORM, SUBFORM, ANY
}
/**
* Callback interface to get notified about progress when signing
*
* @author Karsten Lehmann
*/
public interface SignCallback {
/**
* Method is called after signing a note
*
* @param designElement design element
* @return return value to stop signing
*/
Action noteSigned(DesignElement designElement);
/**
* Method to skip signing for specific notes
*
* @param designElement design element
* @param currentSigner current design element signer
* @return true to sign
*/
boolean shouldSign(DesignElement designElement, String currentSigner);
}
/**
* Creates a new, unsaved agent design element.
*
* @param agent subtype
* @param agentType type of agent, e.g. {@link DesignLotusScriptAgent}
* @param agentName the name of the agent to create
* @return the newly-created in-memory {@link DesignAgent}
*/
T createAgent(Class agentType, String agentName);
/**
* Creates a new, unsaved script library design element.
*
* @param library subtype
* @param libraryType type of library, e.g. {@link LotusScriptLibrary}
* @param libName the name of the library to create
* @return the newly-created in-memory {@link ScriptLibrary}
*/
T createScriptLibrary(Class libraryType, String libName);
/**
* Create a new unsaved database script library if it does not exist yet or the
* one already available in the database
*
* @return database script library
*/
DatabaseScriptLibrary createDatabaseScriptLibrary();
/**
* Creates a new, unsaved File Resource design element.
*
* @param filePath the name of the file resource element
* @return the newly-created in-memory {@link FileResource}
* @since 1.1.2
*/
FileResource createFileResource(String filePath);
/**
* Creates a new, unsaved folder design element.
*
* @param folderName the name of the folder to create
* @return the newly-created in-memory {@link Folder}
*/
Folder createFolder(String folderName);
/**
* Creates a new, unsaved form design element.
*
* @param formName the name of the form to create
* @return the newly-created in-memory {@link Form}
*/
Form createForm(String formName);
/**
* Creates a new, unsaved subform design element.
*
* @param subformName the name of the subform to create
* @return the newly-created in-memory {@link Subform}
*/
Subform createSubform(String subformName);
/**
* Creates a new, unsaved page design element
*
* @param pageName the name of the page to create
* @return the newly-created in-memory {@link Page}
* @since 1.7.8
*/
Page createPage(String pageName);
/**
* Creates a new, unsaved view design element.
*
* @param viewName the name of the view to create
* @return the newly-created in-memory {@link View}
*/
View createView(String viewName);
/**
* Creates a new, unsaved shared column design element
*
* @param columnName the name of the shared column to create
* @return the newly-created in-memory {@link SharedColumn}
* @since 1.5.7
*/
SharedColumn createSharedColumn(String columnName);
/**
* Creates a new, unsaved shared field design element
*
* @param fieldName the name of the shared field to create
* @return the newly-created in-memory {@link SharedField}
* @since 1.27.0
*/
SharedField createSharedField(String fieldName);
/**
* Creates a new, unsaved frameset design element.
*
* @param framesetName the name of the frameset to create
* @return the newly-created in-memory {@link Frameset}
*/
Frameset createFrameset(String framesetName);
/**
* Queries the design collection for a single design note.
*
* @param the type of design element to find
* @param type a {@link Class} object representing {@code }
* @param name the name or alias of the design note
* @param partialMatch whether partial matches are allowed
* @return an {@link OptionalInt} describing the note ID of the specified
* design note, or an empty one if the note was not found
* @impl.Spec It is expected that implementations will use {@code NIFFindDesignNoteExt}
* if available
* @since 1.40.0
*/
OptionalInt findDesignNote(Class type, String name,
boolean partialMatch);
/**
* Queries the design collection for a single design note.
*
* @param noteClass the class of note to query (see NOTE_CLASS_*
* in {@link NotesConstants})
* @param pattern the note flag pattern to query (see
* DFLAGPAT_*
in {@link NotesConstants})
* @param name the name or alias of the design note
* @param partialMatch whether partial matches are allowed
* @return an {@link OptionalInt} describing the note ID of the specified
* design note, or an empty one if the note was not found
* @impl.Spec It is expected that implementations will use {@code NIFFindDesignNoteExt}
* if available
* @since 1.40.0
*/
OptionalInt findDesignNote(DocumentClass noteClass, String pattern, String name,
boolean partialMatch);
/**
* Retrieves the named agent.
*
* @param name the element name to restrict to
* @return an {@link Optional} describing the {@link DesignAgent},
* or an empty {@code Optional} if no such element exists
*/
Optional getAgent(String name);
/**
* Retrieves all agents in the database.
*
* @return a {@link Stream} of {@link DesignAgent}s
*/
Stream getAgents();
/**
* Retrieves the named folder or view.
*
* @param name the element name to restrict to
* @return an {@link Optional} describing the {@link Folder} or {@link View},
* or an empty {@code Optional} if no such element exists
* @param collection type
*/
> Optional> getCollection(
String name);
/**
* Retrieves all folders and views in the database.
*
* @return a {@link Stream} of {@link Folder}s and/or {@link View}s
*/
Stream> getCollections();
/**
* Retrieves the database properties. This is, for implementation reasons, also
* known
* as the icon note.
*
* @return the DB properties element for this database
*/
DbProperties getDatabaseProperties();
/**
* Retrieves a single design element in the database of the provided type
* matching the provided
* title.
*
* @param the type of design element to retrieve
* @param type a {@link Class} object representing {@code }
* @param name the element name to restrict to
* @return an {@link Optional} describing the requested design element,
* or an empty {@code Optional} if no such element exists
*/
Optional getDesignElementByName(Class type, String name);
/**
* Retrieves all design elements in the database of the provided type.
*
* @param the type of design element to retrieve
* @param type a {@link Class} object representing {@code }
* @return a {@link Stream} of matching {@link DesignElement}s
*/
Stream getDesignElements(Class type);
/**
* Retrieves summary information about all design elements in the database of
* the provided type.
*
* Unlike {@link #getDesignElements(Class)}, this method does not actually
* load the underlying design-element notes, and can be used to more-efficiently
* retrieve information about large numbers of design elements at a time.
*
*
* @param the type of design element to retrieve
* @param type a {@link Class} object representing {@code }
* @return a {@link Stream} of {@link DesignEntry} objects matching the
* element type
* @since 1.21.0
*/
Stream> getDesignEntries(Class type);
/**
* Retrieves summary information about all design elements in the database
* matching a {@code $FLAGS} pattern.
*
* Unlike {@link #getDesignElements(Class)}, this method does not actually
* load the underlying design-element notes, and can be used to more-efficiently
* retrieve information about large numbers of design elements at a time.
*
*
* If your patterns matche several design element types, it is safest to use
* {@link DesignElement} directly as the return component type.
*
*
* @param the type of design element to retrieve
* @param noteClasses the {@link DocumentClass}es of the elements to limit to
* @param patterns the flags patterns to match to the elements
* @return a {@link Stream} of {@link DesignEntry} objects matching the
* flags pattern
* @since 1.39.0
*/
Stream> getDesignEntries(
Collection noteClasses, Collection patterns);
/**
* Retrieves all design elements in the database of the provided type matching
* the provided
* title.
*
* @param the type of design element to retrieve
* @param type a {@link Class} object representing {@code }
* @param name the element name to restrict to
* @return a {@link Stream} of matching {@link DesignElement}s
*/
Stream getDesignElementsByName(Class type, String name);
/**
* Retrieves the named file resource.
*
* Note: this method uses the value of the "$TITLE" field of the design element,
* not the "$FileNames" of the file resource. These may diverge, such as when a
* file resource has an alias assigned to it.
*
*
* Moreover, this method finds only elements listed in the "File Resources"
* list in Designer, not elements like "loose" resources in the WebContent
* directory.
*
*
* @param name the name of the resource to restrict to
* @return an {@link Optional} describing the {@link FileResource}, or an empty
* one if no such element exists
* @since 1.0.24
*/
default Optional getFileResource(String name) {
return getFileResource(name, false);
}
/**
* Retrieves the named file resource, optionally including the pool of "XPages-side"
* resources, such as files placed in the "WebContent" directory.
*
* Note: this method uses the value of the "$TITLE" field of the design element,
* not the "$FileNames" of the file resource. These may diverge, such as when a
* file resource has an alias assigned to it.
*
*
* @param name the name of the resource to restrict to
* @param includeXsp whether to include XPages-side file resources
* @return an {@link Optional} describing the {@link FileResource}, or an empty
* one if no such element exists
* @since 1.0.38
*/
Optional getFileResource(String name, boolean includeXsp);
/**
* Retrieves all file resource design elements in the database.
*
* This method finds only elements listed in the "File Resources"
* list in Designer, not elements like "loose" resources in the WebContent
* directory.
*
*
* @return a {@link Stream} of {@link FileResource}s
* @since 1.0.24
*/
default Stream getFileResources() {
return getFileResources(false);
}
/**
* Retrieves all file resource design elements in the database, optionally
* including the pool of "XPages-side" resources, such as files placed in the
* "WebContent" directory.
*
* @param includeXsp whether to include XPages-side file resources
* @return a {@link Stream} of {@link FileResource}s
* @since 1.0.38
*/
Stream getFileResources(boolean includeXsp);
/**
* Retrieves the named folder.
*
* @param name the element name to restrict to
* @return an {@link Optional} describing the {@link Folder}, or an empty one if
* no such view
* exists
* @since 1.0.27
*/
Optional getFolder(String name);
/**
* Retrieves all folders in the database
*
* @return a {@link Stream} of {@link Folder}s
* @since 1.0.27
*/
Stream getFolders();
/**
* Retrieves the named form.
*
* @param name the element name to restrict to
* @return an {@link Optional} describing the {@link Form},
* or an empty {@code Optional} if no such element exists
*/
Optional