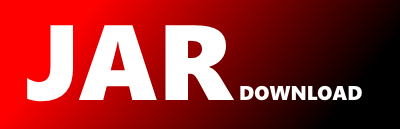
com.hcl.domino.html.HtmlConvertOption Maven / Gradle / Ivy
/*
* ==========================================================================
* Copyright (C) 2019-2022 HCL America, Inc. ( http://www.hcl.com/ )
* All rights reserved.
* ==========================================================================
* Licensed under the Apache License, Version 2.0 (the "License"). You may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at .
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
* ==========================================================================
*/
package com.hcl.domino.html;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import com.hcl.domino.html.RichTextHTMLConverter.Builder;
/**
* An enum with existing HTML conversion options to be set via
* {@link Builder#option(HtmlConvertOption, String)}.
*
* Please note that we provide an additional option method to set further
* undocumented options
* via {@link Builder#options(Collection, String)}.
*
* See these blog articles to get an idea what can be done with these
* options:
* What
* if.....we didn’t use <font> tags in our HTML output anymore?
* Domino
* HTML generation and FontConversion=1
*
* Possible values for the "spec" and "tag" attributes to control HTML output
* (e.g. {@link #FontSizeSpec}):
*
* - 0 (none) - do not generate anything for the spec
* - 1 (native) - use the default HTML generation for the spec
* - 2 (outer span tag with style attribute) - surround any native tag with a
* <span> tag that specifies a style attribute, e.g. <span
* style="font-family: sans-serif ; ">........</span>
* - 4 (inner font tag with style attribute) - use a <font> tag with a
* style attribute inside any native tag, e.g. == <font style="font-family:
* sans-serif ; ">........</font>
* - 8 (native tag with style attribute - only applicable if there is a native
* tag) - use the native tag with a style attribute, e.g. <tt
* style="font-family: monospace">........</tt>
* - 16 (outer span tag with class attribute) - surround any native tag with a
* <span> tag that specifies a class attribute, e.g. <span
* class="domino-font-sansserif ">........</span>
* - 32 (native tag with class attribute - only applicable if there is a
* native tag) - use the native tag with a class attribute, e.g. <tt
* class="domino-font-monospace">........</tt>
*
*/
public enum HtmlConvertOption {
/**
* Forces all sections to be expanded, regardless of their expansion in the
* Notes® rich text fields.
*/
ForceSectionExpand,
/**
* Forces alternate formatting of tables with tabbed sections.
* All of the tabs are displayed at the same time, one below the other, with the
* tab labels included as headers.
*/
RowAtATimeTableAlt,
/**
* Forces all outlines to be expanded, regardless of their expansion in the
* Notes rich text.
*/
ForceOutlineExpand,
/** Disables passthru HTML, treating the HTML as plain text. */
DisablePassThruHTML,
/** Preserves Notes intraline whitespace (spaces between characters). */
TextExactSpacing,
/** enable new code for better representation of indented lists */
ListFidelity,
/**
* allow multiple embedded view if: 1) page is in raw mode; 2) view is marked
* render as html
*/
MultiEmbeddedHTMLView,
/** If set, converts tabs to the number of spaces specified. */
TextConvertTabsToSpaces,
/** run the ActiveContentfilter on open item (useful for HAPI) */
ACFOnItem,
/** automatically add class names. */
AutoClass,
/** add classes to field names - experimental */
AutoClassField,
/** add field name as inner span */
FieldName,
/** use xpages style theme files (reduced features from xpages) */
Themes,
/** generate <label> around input tags for checkboxes and radio buttons */
FieldChoiceLabel,
/** use the TITLE attribute of the TABLE as the <caption> */
TableCaptionFromTitle,
/** alternate Section representation (no dynamics) */
SectionAlt,
/**
* CD->HTML attachments don't have <a><img>, just text saying
* "see attachment..."
*/
AttachmentLink,
/** alternate reps for table style ; 1 = dropm ec blank */
TableStyle,
/** include a notes:// url when generating doclink urls */
OfferNotesURLInLink,
/**
* similar to AttachmentLink, but for when the Item is type MIME separate option
* just to not
* interfere with already released behavior of the other option.
* This option only has effect if the internal logic of the mime processor would
* have
* otherwise produced attachment links.
* For example, explicitly setting this to 0 will NOT cause attachment links to
* appear
* if the internal logic has determined that attachment links will not appear.
*/
MIMEAttachmentLink,
/** Add bits of the link object to the link url. */
LinkArg,
/**
* Force using redirect URLs regardless of NAB setting. This is just to create
* the
* URLs not to process them.
*/
ForceRedirectURL,
/** html option version of the HAPI property */
LinkHandling,
/** alternate html for links */
LinkHTMLAlt,
MIMEItemFrame,
/** for FontConverstion = 0, render the notes highlight text */
RenderHighlight,
/** 0 will use <ul> for indenting, 1 will use <div> */
ParagraphIndent,
/** flags (see below) to control trimming of keyword values on fields */
FieldKeywordTrim,
/**
* Enable XML-compatible HTML output
*/
XMLCompatibleHTML,
/**
* FontConversion - the master option
*
* - 0 (classic) - the original web server handling
* - 1 (style) - use combinations of span and other new tags, as controlled by
* the remaining options.
*
*/
FontConversion,
FontSizeBase,
// set these to "2" to get modern inline CSS attributes
FontColorSpec,
FontFaceSerifSpec,
FontFaceSansserifSpec,
FontFaceMonospaceSpec,
FontFaceSpecificSpec,
FontSizeSpec,
FontStyleBoldSpec,
FontStyleItalicSpec,
FontStyleUnderlineSpec,
FontStyleStrikethroughSpec,
// set these to "0" to remove old font tags from the output
FontFaceMonospaceTag,
FontStyleBoldTag,
FontStyleItalicTag,
FontStyleUnderlineTag,
FontStyleStrikethroughTag;
/**
* Returns all "spec" options, e.g. {@link #FontColorSpec}
*
* @return spec options
*/
public static Set allSpecs() {
final HashSet specs = new HashSet<>();
specs.add(FontColorSpec);
specs.add(FontFaceSerifSpec);
specs.add(FontFaceSansserifSpec);
specs.add(FontFaceMonospaceSpec);
specs.add(FontFaceSpecificSpec);
specs.add(FontSizeSpec);
specs.add(FontStyleBoldSpec);
specs.add(FontStyleItalicSpec);
specs.add(FontStyleUnderlineSpec);
specs.add(FontStyleStrikethroughSpec);
return specs;
}
/**
* Returns all "tag" options, e.g. {@link #FontStyleBoldTag}
*
* @return tag options
*/
public static Set allTags() {
final HashSet tags = new HashSet<>();
tags.add(FontFaceMonospaceTag);
tags.add(FontStyleBoldTag);
tags.add(FontStyleItalicTag);
tags.add(FontStyleUnderlineTag);
tags.add(FontStyleStrikethroughTag);
return tags;
}
public static String[] toStringArray(final Collection options) {
final List optionsAsStrList = new ArrayList<>(options.size());
for (final HtmlConvertOption currOption : options) {
optionsAsStrList.add(currOption.toString() + "=1"); //$NON-NLS-1$
}
return optionsAsStrList.toArray(new String[optionsAsStrList.size()]);
}
/**
* Converts this enumerated option to a string value suitable for passing to an
* HTML converter.
*
* @param value the value of the option. This is usually {@code "1"}.
* @return a string version of this option with the provided value
* @since 1.0.3
*/
public String toOption(final String value) {
final String val = value == null ? "" : value; //$NON-NLS-1$
return this.name() + "=" + val; //$NON-NLS-1$
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy