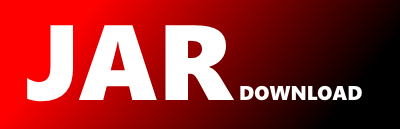
com.hcl.domino.json.JsonSerializer Maven / Gradle / Ivy
/*
* ==========================================================================
* Copyright (C) 2019-2022 HCL America, Inc. ( http://www.hcl.com/ )
* All rights reserved.
* ==========================================================================
* Licensed under the Apache License, Version 2.0 (the "License"). You may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at .
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
* ==========================================================================
*/
package com.hcl.domino.json;
import java.text.MessageFormat;
import java.util.Collection;
import java.util.Collections;
import java.util.TreeSet;
import java.util.function.BiFunction;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import com.hcl.domino.data.Document;
import com.hcl.domino.data.ItemDataType;
import com.hcl.domino.html.HtmlConvertOption;
import com.hcl.domino.misc.JNXServiceFinder;
/**
* This interface allows for serialization of documents into JSON, both as
* strings
* and as implementation-specific Java object representations.
*
* Implementations of this service are expected to be provided by additional
* integration modules, such as {@code domino-jnx-jsonp}.
*
*
* @author Jesse Gallagher
* @since 1.0.7
*/
public interface JsonSerializer {
Collection DEFAULT_EXCLUDED_ITEMS = Collections.unmodifiableSet(Stream.of(
"$Fonts" //$NON-NLS-1$
).collect(Collectors.toCollection(() -> new TreeSet<>(String.CASE_INSENSITIVE_ORDER))));
String PROP_METADATA = "@meta"; //$NON-NLS-1$
String PROP_META_NOTEID = "noteid"; //$NON-NLS-1$
String PROP_META_UNID = "unid"; //$NON-NLS-1$
String PROP_META_CREATED = "created"; //$NON-NLS-1$
String PROP_META_LASTMODIFIED = "lastmodified"; //$NON-NLS-1$
String PROP_META_LASTMODIFIEDINFILE = "lastmodifiedinfile"; //$NON-NLS-1$
String PROP_META_LASTACCESSED = "lastaccessed"; //$NON-NLS-1$
String PROP_META_ADDEDTOFILE = "addedtofile"; //$NON-NLS-1$
String PROP_META_NOTECLASS = "noteclass"; //$NON-NLS-1$
String PROP_META_SEQUENCENUMBER = "seq"; //$NON-NLS-1$
String PROP_META_SEQUENCETIME = "seqtime"; //$NON-NLS-1$
/**
* @since 1.11.0
*/
String PROP_META_PARENTUNID = "parentunid"; //$NON-NLS-1$
/**
* @since 1.11.0
*/
String PROP_META_UNREAD = "unread"; //$NON-NLS-1$
/**
* @since 1.11.0
*/
String PROP_META_THREADID = "threadid"; //$NON-NLS-1$
/**
* @since 1.11.0
*/
String PROP_META_REVISION = "revision"; //$NON-NLS-1$
/**
* @since 1.20.0
*/
String PROP_META_EDITABLE = "editable"; //$NON-NLS-1$
/**
* @since 1.41.0
*/
String PROP_META_SIGNER = "signer"; //$NON-NLS-1$
String PROP_RANGE_FROM = "from"; //$NON-NLS-1$
String PROP_RANGE_TO = "to"; //$NON-NLS-1$
/**
* Constructs a new serializer using the first available JSON service.
*
* @return a new {@link JsonSerializer} implementation
* @throws IllegalStateException if there is no registered serializer
* implementation
*/
static JsonSerializer createSerializer() {
return JNXServiceFinder.findServices(JsonSerializerFactory.class)
.findFirst()
.map(JsonSerializerFactory::newSerializer)
.orElseThrow(() -> new IllegalStateException(
MessageFormat.format("Unable to find a {0} service implementation", JsonDeserializerFactory.class.getName())));
}
/**
* Sets the item names that should be considered boolean values when serialized
* to JSON.
*
* Items of type {@link ItemDataType#TYPE_TEXT TEXT},
* {@link ItemDataType#TYPE_NUMBER NUMBER}, and
* {@link ItemDataType#TYPE_RFC822_TEXT RFC822_TEXT}, as well as
* single-value items of type {@link ItemDataType#TYPE_TEXT_LIST TEXT_LIST} or
* {@link ItemDataType#TYPE_NUMBER_RANGE NUMBER_RANGE} are evaluated for boolean
* conversion.
*
*
* @param booleanItemNames the names of items to serialize as boolean
* @return this serializer
*/
JsonSerializer booleanItemNames(Collection booleanItemNames);
/**
* Sets the values used to determine {@code true} and {@code false} when
* serializing items configured
* via {@link #booleanItemNames(Collection)}.
*
* All values not specified here will be considered {@code false}.
*
*
* @param trueValues a collection of objects to compare to resolve as
* {@code true}
* @return this serializer
*/
JsonSerializer booleanTrueValues(Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy