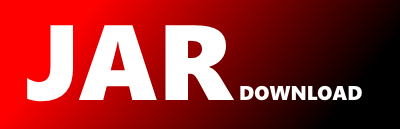
com.hcl.domino.person.OutOfOffice Maven / Gradle / Ivy
/*
* ==========================================================================
* Copyright (C) 2019-2022 HCL America, Inc. ( http://www.hcl.com/ )
* All rights reserved.
* ==========================================================================
* Licensed under the Apache License, Version 2.0 (the "License"). You may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at .
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
* ==========================================================================
*/
package com.hcl.domino.person;
import java.time.temporal.TemporalAccessor;
import com.hcl.domino.data.Database;
import com.hcl.domino.data.DominoDateRange;
import com.hcl.domino.misc.Ref;
/**
* Out of Office information for a single user
*/
public interface OutOfOffice {
public enum OOOType {
AGENT, SERVICE
}
/**
* This function returns time parameters that control OOO.
*
* @return away period
*/
DominoDateRange getAwayPeriod();
/**
* OOO supports two sets of messages. They are called General message/subject
* and
* Special message/subject.
* This function returns the text of the general message.
*
* @return message
*/
String getGeneralMessage();
/**
* OOO supports two sets of messages.
*
* They are called General message/subject and Special message/subject.
* This function gets the general subject.
* This is string that will appear as the subject line of the OOO notification.
*
* @return subject
*/
String getGeneralSubject();
Person getParentPerson();
/**
* This function returns the version (agent, service) and the state (disabled,
* enabled)
* of the out of office functionality.
* The version information can be used to show or hide UI elements that might
* not be
* supported for a given version.
* For example, the agent does not support durations of less than 1 day and some
* clients might choose not to show the hours in the user interface.
* When you need to make {@link #getState(Ref, Ref)} as efficient as possible,
* call
* {@link Person#openOutOfOffice(String, boolean, Database)}
* with the home mail server and the opened mail database.
* This function is read only and does not return an error if user ACL rights
* are below Editor (which are required to turn on/off the Out of office
* functionality).
* If {@link #getState(Ref, Ref)} is called immediately following
* {@link #setEnabled(boolean)} it will
* not reflect the state set by the {@link #setEnabled(boolean)}.
* To see the current state, start a new operation using
* {@link Person#openOutOfOffice(String, boolean, Database)},
* {@link OutOfOffice#getState(Ref, Ref)}.
*
* @param retType returns the type of the OOO system (agent or service)
* @param retIsEnabled returns whether the service is enabled for the user
*/
void getState(Ref retType, Ref retIsEnabled);
/**
* Convenience method to read which kind of OOO system is used (agent or
* service).
* Calls {@link #getState(Ref, Ref)} internally.
*
* @return type
*/
OOOType getType();
/**
* Convenience method to check whether the OOO functionality is enabled. Calls
* {@link #getState(Ref, Ref)} internally.
*
* @return true if enabled
*/
boolean isEnabled();
/**
* This function returns a flag which defines how to treat internet emails.
* This functional call is optional.
* If this flag is set to {@code true} OOO notifications will not be generated
* for
* email originating from the internet. The default for this flag is
* {@code true}.
*
* @return true if excluded
*/
boolean isExcludeInternet();
/**
* This function validates and sets the time parameters that control OOO.
*
* This information is required for enabling the OOO.
* If you want turn on OOO functionality for a given period of time the
* sequence of calls needed is:
* {@link Person#openOutOfOffice(String, boolean, Database)},
* {@link #setAwayPeriod(TemporalAccessor, TemporalAccessor)}, and
* {@link #setEnabled(boolean)}.
*
* When you need to enable OOO (i.e. call it with enabled
flag set
* to {@code true})
* you should call {@link #setAwayPeriod(TemporalAccessor, TemporalAccessor)}
* prior to calling
* {@link #setEnabled(boolean)}.
*
* If you need to change the length of the away period after OOO has already
* been
* enabled, the sequence of calls needed to perform this action is
* {@link Person#openOutOfOffice(String, boolean, Database)},
* {@link #setAwayPeriod(TemporalAccessor, TemporalAccessor)}.
*
* If the Domino server is configured to run an OOO agent, it can only be turned
* on
* for full days, the time portion of the date parameter will not be used.
*
* @param tdStartAway This is date and time when Out of office will begin.
* @param tdEndAway This is date and time when Out of office will end.
*/
void setAwayPeriod(TemporalAccessor tdStartAway, TemporalAccessor tdEndAway);
/**
* This function changes the state of the OOO functionality as indicated by
* the enabled
variable.
* If the OOO functionality is already in the state indicated by the
* enabled
flag, this function does nothing.
*
* When you need to enable OOO (i.e. call it with enabled
flag set
* to {@code true}) you should call
* {@link #setAwayPeriod(TemporalAccessor, TemporalAccessor)}
* prior to calling {@link #setEnabled(boolean)}.
*
* If {@link #setAwayPeriod(TemporalAccessor, TemporalAccessor)} is not called,
* {@link #setEnabled(boolean)} will use the previous value for start and
* end.
*
* If they are in the past then the OOO functionality will not be enabled.
* When you need to disable OOO (i.e. call it with enabled
set to
* {@code false})
* {@link #setAwayPeriod(TemporalAccessor, TemporalAccessor)} does not need to
* be called.
*
* When {@link #setEnabled(boolean)} is called with the enabled
set
* to {@code false} it means you want to disable OOO immediately.
* If you don’t want to disable OOO functionality immediately, but rather you
* just want to change the time when OOO should stop operating, the sequence
* of calls is: {@link Person#openOutOfOffice(String, boolean, Database)},
* {@link #setAwayPeriod(TemporalAccessor, TemporalAccessor)}.
* If OOO is configured to run as a service and {@link #setEnabled(boolean)} is
* used to disable, the OOO service will be auto-disabled immediately.
*
* The summary report will be generated on the first email received after the
* disable has been requested, or if no messages are received it will
* generated during the nightly router maintenance.
*
* If OOO is configured as an agent, the user will receive a summary report
* and a request to disable the agent on the next scheduled run of the agent
* will occur.
*
* @param enabled true to enable
*/
void setEnabled(boolean enabled);
/**
* This function sets a flag which defines how to treat internet emails.
*
* This functional call is optional.
* If this flag is set to {@code true} OOO notifications will not be generated
* for
* email originating from the internet.
* The default for this flag is {@code true}.
*
* @param exclude true to exclude
*/
void setExcludeInternet(boolean exclude);
/**
* OOO supports two sets of notification messages.
* They are called General message/subject and Special message/subject.
* The following text is always appended to the body of the message, where
* the "Message subject" is obtained from the message which caused the
* notification to be generated.
* "Note: This is an automated response to your message "Message subject"
* sent on 2/12/2009 10:12:17 AM. This is the only notification you will receive
* while this person is away."
*
* @param msg message, max 65535 bytes LMBCS encoded (WORD datatype for length)
*/
void setGeneralMessage(String msg);
/**
* OOO supports two sets of notification messages.
*
* They are called General message/subject and Special message/subject.
* The rest of the people will receive the general message/subject message.
* This function sets the general subject.
* If this field is not specified in by this API call, the value defined
* using Notes Client will be used, otherwise the default for this field
* is the following text AUTO: Katherine Smith is out of the office
* (returning 02/23/2009 10:12:17 AM).
*
* @param subject string that will appear as the subject line of the
* OOO notification
* @param displayReturnDate Boolean which controls whether (“returning
* <date>”) appears on the subject line
*/
void setGeneralSubject(String subject, boolean displayReturnDate);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy