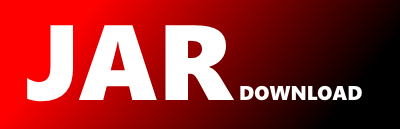
com.hcl.domino.naming.UserDirectoryQuery Maven / Gradle / Ivy
/*
* ==========================================================================
* Copyright (C) 2019-2022 HCL America, Inc. ( http://www.hcl.com/ )
* All rights reserved.
* ==========================================================================
* Licensed under the Apache License, Version 2.0 (the "License"). You may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at .
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
* ==========================================================================
*/
package com.hcl.domino.naming;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.stream.Stream;
import com.hcl.domino.data.SearchQuery;
/**
* Represents a query into the names directory system of the local runtime or
* a remote server.
*
* @author Jesse Gallagher
* @since 1.0.2
*/
public interface UserDirectoryQuery extends SearchQuery {
/**
* Sets the query to search through all available directories,
* rather than stopping with the first directory to contain a match.
*
* @return this query builder
*/
UserDirectoryQuery exhaustive();
/**
* Instructs the query to update the back-end views before performing
* the search.
*
* @return this query builder
*/
UserDirectoryQuery forceUpdate();
/**
* Specifies the items to extract from found directory entries.
*
* This must be specified before a call to {@link #stream()}
*
*
* @param items a {@link Collection} of item names
* @return this query builder
*/
UserDirectoryQuery items(Collection items);
/**
* Specifies the items to extract from found directory entries.
*
* This must be specified before a call to {@link #stream()}
*
*
* @param items a {@link Collection} of item names
* @return this query builder
* @since 1.0.17
*/
default UserDirectoryQuery items(final String... items) {
return this.items(Arrays.asList(items));
}
/**
* Specifies the names to look up. If unspecified, the query will return all
* entries in the specified namespaces.
*
* @param names a {@link Collection} of string names to look up
* @return this query builder
*/
UserDirectoryQuery names(Collection names);
/**
* Specifies the names to look up. If unspecified, the query will return all
* entries in the specified namespaces.
*
* @param names the names to look up
* @return this query builder
* @since 1.0.17
*/
default UserDirectoryQuery names(final String... names) {
return this.names(Arrays.asList(names));
}
/**
* Instructs the query to search the provided namespaces, which in practice
* correspond to Domino view names. The default for this setting is to
* search "$Users".
*
* If specified, this must be non-null and non-empty.
*
*
* @param namespaces a {@link Collection} of namespace names to search
* @return this query builder
*/
UserDirectoryQuery namespaces(Collection namespaces);
/**
* Instructs the query to search the provided namespaces, which in practice
* correspond to Domino view names. The default for this setting is to
* search "$Users".
*
* @param namespaces the namespaces names to search
* @return this query builder
* @since 1.0.17
*/
default UserDirectoryQuery namespaces(final String... namespaces) {
return this.namespaces(Arrays.asList(namespaces));
}
/**
* Executes and retrieves a stream of results from the query.
*
* The result stream will contain one entry per queried name per queried
* namespace. Each of
* those entries will contain a list of matches for the name+namespace pair.
*
*
* @return a {@link Stream} of {@code List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy