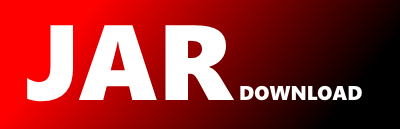
com.hcl.domino.jna.data.JNADocSummaryCollectionEntry Maven / Gradle / Ivy
/*
* ==========================================================================
* Copyright (C) 2019-2022 HCL America, Inc. ( http://www.hcl.com/ )
* All rights reserved.
* ==========================================================================
* Licensed under the Apache License, Version 2.0 (the "License"). You may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at .
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
* ==========================================================================
*/
package com.hcl.domino.jna.data;
import java.text.MessageFormat;
import java.time.OffsetDateTime;
import java.time.temporal.Temporal;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.TreeMap;
import com.hcl.domino.commons.data.AbstractCollectionEntry;
import com.hcl.domino.commons.data.AbstractTypedAccess;
import com.hcl.domino.data.CollectionEntry;
import com.hcl.domino.data.Document;
import com.hcl.domino.data.DocumentClass;
import com.hcl.domino.data.DominoDateTime;
import com.hcl.domino.data.TypedAccess;
/**
* Implementation of {@link CollectionEntry} that has been read
* from the summary buffer data of a document
*
* @author Karsten Lehmann
*/
public class JNADocSummaryCollectionEntry extends AbstractCollectionEntry {
private JNADatabase m_parentDb;
private JNADominoCollection m_parentCollection;
private int m_noteId;
private String m_unid;
private int m_sequenceNumber;
private DominoDateTime m_sequenceTime;
private TreeMap m_values;
private TypedAccess m_typedAccess;
private JNADocument m_doc;
public JNADocSummaryCollectionEntry(JNADatabase parentDb, JNADominoCollection parentCollection,
int noteId, String unid, int seq, DominoDateTime seqTime, TreeMap values) {
m_parentDb = parentDb;
m_parentCollection = parentCollection;
m_noteId = noteId;
m_unid = unid;
m_sequenceNumber = seq;
m_sequenceTime = seqTime;
m_values = values;
m_typedAccess = new AbstractTypedAccess() {
@Override
public boolean hasItem(String itemName) {
return JNADocSummaryCollectionEntry.this.hasItem(itemName);
}
@Override
public List getItemNames() {
return JNADocSummaryCollectionEntry.this.getItemNames();
}
@Override
protected List> getItemValue(String itemName) {
Object obj = m_values.get(itemName);
if (obj==null) {
return null;
}
else if (obj instanceof List) {
return (List>) obj;
}
else {
return Arrays.asList(obj);
}
}
};
}
@Override
public boolean hasItem(String itemName) {
return m_values.containsKey(itemName);
}
@Override
public List getItemNames() {
return new ArrayList<>(m_values.keySet());
}
@Override
public T get(String itemName, Class valueType, T defaultValue) {
return m_typedAccess.get(itemName, valueType, defaultValue);
}
@Override
public List getAsList(String itemName, Class valueType, List defaultValue) {
return m_typedAccess.getAsList(itemName, valueType, defaultValue);
}
@Override
public int size() {
return m_values.size();
}
@Override
public boolean isEmpty() {
return m_values.isEmpty();
}
@Override
public boolean containsKey(Object key) {
return m_values.containsKey(key);
}
@Override
public boolean containsValue(Object value) {
return m_values.containsValue(value);
}
@Override
public Object get(Object key) {
return m_values.get(key);
}
@Override
public Object put(String key, Object value) {
return m_values.put(key, value);
}
@Override
public Object remove(Object key) {
return m_values.remove(key);
}
@Override
public void putAll(Map extends String, ? extends Object> m) {
m_values.putAll(m);
}
@Override
public void clear() {
m_values.clear();
}
@Override
public Set keySet() {
return m_values.keySet();
}
@Override
public Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy