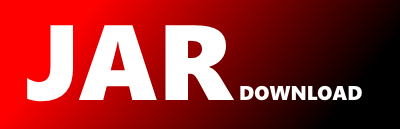
com.hcl.domino.jna.internal.gc.handles.LockUtil Maven / Gradle / Ivy
/*
* ==========================================================================
* Copyright (C) 2019-2022 HCL America, Inc. ( http://www.hcl.com/ )
* All rights reserved.
* ==========================================================================
* Licensed under the Apache License, Version 2.0 (the "License"). You may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at .
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
* ==========================================================================
*/
package com.hcl.domino.jna.internal.gc.handles;
import java.security.AccessController;
import java.security.PrivilegedAction;
public class LockUtil {
/**
* Method to lock one handle
*
* @param type of handle 1
* @param type of handle 1 by val
* @param result type
* @param handle1 handle or null
* @param callback callback
* @return result
*/
public static R lockHandle(IHANDLEBase handle1, HandleAccess callback) {
if (handle1==null || handle1.isNull()) {
handle1 = new NullHandle<>();
}
final IHANDLEBase fHandle1 = handle1;
return fHandle1._lockHandleAccess((handle1ByVal) -> {
return callback.accessLockedHandle(handle1ByVal);
});
}
/**
* Method to lock two handles
*
* @param type of handle 1
* @param type of handle 1 by val
* @param type of handle 2
* @param type of handle 2 by val
* @param result type
* @param handle1 handle 1 or null
* @param handle2 handle 2 or null
* @param callback callback
* @return result
*/
public static R lockHandles(IHANDLEBase handle1, IHANDLEBase handle2,
HandleAccess2 callback) {
if (handle1==null || handle1.isNull()) {
handle1 = new NullHandle<>();
}
if (handle2==null || handle2.isNull()) {
handle2 = new NullHandle<>();
}
final IHANDLEBase fHandle1 = handle1;
final IHANDLEBase fHandle2 = handle2;
return fHandle1._lockHandleAccess((handle1ByVal) -> {
return fHandle2._lockHandleAccess((handle2ByVal) -> {
return callback.accessLockedHandles(handle1ByVal, handle2ByVal);
});
});
}
/**
* Method to lock three handles
*
* @param type of handle 1
* @param type of handle 2 by val
* @param type of handle 2
* @param type of handle 2 by val
* @param type of handle 3
* @param type of handle 3 by val
* @param result type
* @param handle1 handle 1 or null
* @param handle2 handle 2 or null
* @param handle3 handle 3 or null
* @param callback callback
* @return result
*/
public static R lockHandles(IHANDLEBase handle1, IHANDLEBase handle2,
IHANDLEBase handle3, HandleAccess3 callback) {
if (handle1==null || handle1.isNull()) {
handle1 = new NullHandle<>();
}
if (handle2==null || handle2.isNull()) {
handle2 = new NullHandle<>();
}
if (handle3==null || handle3.isNull()) {
handle3 = new NullHandle<>();
}
final IHANDLEBase fHandle1 = handle1;
final IHANDLEBase fHandle2 = handle2;
final IHANDLEBase fHandle3 = handle3;
return fHandle1._lockHandleAccess((handle1ByVal) -> {
return fHandle2._lockHandleAccess((handle2ByVal) -> {
return fHandle3._lockHandleAccess((handle3ByVal) -> {
return callback.accessLockedHandles(handle1ByVal, handle2ByVal, handle3ByVal);
});
});
});
}
/**
* Method to lock four handles
*
* @param type of handle 1
* @param type of handle 2 by val
* @param type of handle 2
* @param type of handle 2 by val
* @param type of handle 3
* @param type of handle 3 by val
* @param type of handle 4
* @param type of handle 4 by val
* @param result type
*
* @param handle1 handle 1 or null
* @param handle2 handle 2 or null
* @param handle3 handle 3 or null
* @param handle4 handle 4 or null
* @param callback callback
* @return result
*/
public static R lockHandles(
IHANDLEBase handle1,
IHANDLEBase handle2,
IHANDLEBase handle3,
IHANDLEBase handle4,
HandleAccess4 callback) {
if (handle1==null || handle1.isNull()) {
handle1 = new NullHandle<>();
}
if (handle2==null || handle2.isNull()) {
handle2 = new NullHandle<>();
}
if (handle3==null || handle3.isNull()) {
handle3 = new NullHandle<>();
}
if (handle4==null || handle4.isNull()) {
handle4 = new NullHandle<>();
}
final IHANDLEBase fHandle1 = handle1;
final IHANDLEBase fHandle2 = handle2;
final IHANDLEBase fHandle3 = handle3;
final IHANDLEBase fHandle4 = handle4;
return fHandle1._lockHandleAccess((handle1ByVal) -> {
return fHandle2._lockHandleAccess((handle2ByVal) -> {
return fHandle3._lockHandleAccess((handle3ByVal) -> {
return fHandle4._lockHandleAccess((handle4ByVal) -> {
return callback.accessLockedHandles(handle1ByVal, handle2ByVal, handle3ByVal, handle4ByVal);
});
});
});
});
}
private static class NullHandle implements IHANDLEBase {
@Override
public R _lockHandleAccess(HandleAccess handleAccess) {
return AccessController.doPrivileged((PrivilegedAction) () -> handleAccess.accessLockedHandle(null));
}
@Override
public void checkDisposed() {
}
@Override
public boolean isNull() {
return true;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy