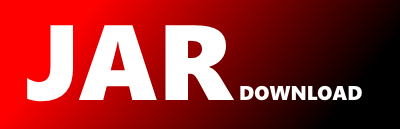
com.hcl.domino.jna.internal.search.ItemTableDataDocAdapter Maven / Gradle / Ivy
The newest version!
/*
* ==========================================================================
* Copyright (C) 2019-2022 HCL America, Inc. ( http://www.hcl.com/ )
* All rights reserved.
* ==========================================================================
* Licensed under the Apache License, Version 2.0 (the "License"). You may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at .
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
* ==========================================================================
*/
package com.hcl.domino.jna.internal.search;
import java.time.temporal.Temporal;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.TreeMap;
import com.hcl.domino.DominoException;
import com.hcl.domino.commons.data.AbstractTypedAccess;
import com.hcl.domino.commons.util.NotesDateTimeUtils;
import com.hcl.domino.commons.util.StringUtil;
import com.hcl.domino.commons.views.IItemTableData;
import com.hcl.domino.data.Formula;
import com.hcl.domino.data.Item;
import com.hcl.domino.data.ItemDataType;
import com.hcl.domino.data.TypedAccess;
import com.hcl.domino.jna.data.JNADocument;
import com.hcl.domino.jna.data.JNADominoDateTime;
import com.hcl.domino.jna.internal.LMBCSString;
/**
* Adapter that maps the {@link IItemTableData} interface onto a docunent
*
* @author Karsten Lehmann
*/
public class ItemTableDataDocAdapter implements IItemTableData {
private JNADocument m_doc;
private LinkedHashMap m_columnValues;
private boolean m_preferTimeDate = true;
private TypedAccess m_typedItems;
private Map m_compiledFormulas;
private Map> m_compiledFormulaValues;
private List m_docItemNames;
public ItemTableDataDocAdapter(JNADocument doc, LinkedHashMap columnValues) {
m_doc = doc;
m_columnValues = columnValues;
m_compiledFormulas = new TreeMap<>(String.CASE_INSENSITIVE_ORDER);
m_compiledFormulaValues = new TreeMap<>(String.CASE_INSENSITIVE_ORDER);
m_typedItems = new AbstractTypedAccess() {
@Override
public boolean hasItem(String itemName) {
if (m_columnValues!=null) {
return m_columnValues.containsKey(itemName);
}
else {
return m_doc.hasItem(itemName);
}
}
@Override
public List getItemNames() {
if (m_docItemNames==null) {
if (m_columnValues!=null) {
m_docItemNames = new ArrayList<>(m_columnValues.keySet());
}
else {
m_docItemNames = m_doc.getItemNames();
}
}
return m_docItemNames;
}
@Override
protected List> getItemValue(String itemName) {
checkDisposed();
if (m_columnValues!=null) {
String formulaStr = m_columnValues.get(itemName);
if (StringUtil.isEmpty(formulaStr)) {
return m_doc.getItemValue(itemName);
}
else {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy