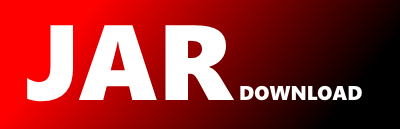
com.hcl.domino.jna.internal.structs.HtmlAPIReference32Struct Maven / Gradle / Ivy
The newest version!
/*
* ==========================================================================
* Copyright (C) 2019-2022 HCL America, Inc. ( http://www.hcl.com/ )
* All rights reserved.
* ==========================================================================
* Licensed under the Apache License, Version 2.0 (the "License"). You may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at .
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
* ==========================================================================
*/
package com.hcl.domino.jna.internal.structs;
import java.security.AccessController;
import java.security.PrivilegedAction;
import java.util.Arrays;
import java.util.List;
import com.hcl.domino.commons.structs.WrongArraySizeException;
import com.sun.jna.Pointer;
import com.sun.jna.Structure;
/**
* This file was autogenerated by JNAerator,
* a tool written by Olivier Chafik that uses a few opensource projects..
* For help, please visit NativeLibs4Java , Rococoa, or JNA.
*/
public class HtmlAPIReference32Struct extends BaseStructure {
/**
* How ref is used in the HTML text (HTMLAPI_REF_xxx - see list above)
* C type : HTMLAPI_REF_TYPE
*/
public int RefType;
/**
* Reference's NULL-terminated text string
* C type : char*
*/
public Pointer pRefText;
/**
* A web server command associated with the target URL
* C type : CmdId
*/
public int CommandId;
/**
* Reference's target components
* C type : HTMLAPI_URLComponent*
*/
public Pointer pTargets;
/**
* Reference's arguments (eg, &Start=xxx, &Count=xxx, etc).
* C type : HTMLAPI_URLComponent*
*/
public Pointer pArgs;
/** Number of components in the target part of the reference (dbname, unid, etc.) */
public int NumTargets;
/** Number of components in the args part of the reference (&Start, etc.) */
public int NumArgs;
/**
* NULL-terminated LMBCS text string -- the fragment part of URL
* if there is no fragment, pointer points to "" (i.e. '\0')
* C type : char*
*/
public Pointer pFragment;
/**
* C trick to align first member of an array of target and arg values
* C type : HTMLAPI_URLComponent[1]
*/
public HtmlApi_UrlComponentStruct[] URLParts = new HtmlApi_UrlComponentStruct[1];
public HtmlAPIReference32Struct() {
super();
}
public static HtmlAPIReference32Struct newInstance() {
return AccessController.doPrivileged((PrivilegedAction) () -> new HtmlAPIReference32Struct());
}
@Override
protected List getFieldOrder() {
return Arrays.asList(
"RefType", //$NON-NLS-1$
"pRefText", //$NON-NLS-1$
"CommandId", //$NON-NLS-1$
"pTargets", //$NON-NLS-1$
"pArgs", //$NON-NLS-1$
"NumTargets", //$NON-NLS-1$
"NumArgs", //$NON-NLS-1$
"pFragment", //$NON-NLS-1$
"URLParts" //$NON-NLS-1$
);
}
/**
* @param RefType How ref is used in the HTML text (HTMLAPI_REF_xxx - see list above)
* C type : HTMLAPI_REF_TYPE
* @param pRefText Reference's NULL-terminated text string
* C type : char*
* @param CommandId A web server command associated with the target URL
* C type : CmdId
* @param pTargets Reference's target components
* C type : HTMLAPI_URLComponent*
* @param pArgs Reference's arguments (eg, &Start=xxx, &Count=xxx, etc).
* C type : HTMLAPI_URLComponent*
* @param NumTargets Number of components in the target part of the reference (dbname, unid, etc.)
* @param NumArgs Number of components in the args part of the reference (&Start, etc.)
* @param pFragment NULL-terminated LMBCS text string -- the fragment part of URL
* if there is no fragment, pointer points to "" (i.e. '\0')
* C type : char*
* @param URLParts C trick to align first member of an array of target and arg values
* C type : HTMLAPI_URLComponent[1]
*/
public HtmlAPIReference32Struct(int RefType, Pointer pRefText, int CommandId, Pointer pTargets, Pointer pArgs, int NumTargets, int NumArgs, Pointer pFragment, HtmlApi_UrlComponentStruct URLParts[]) {
super();
this.RefType = RefType;
this.pRefText = pRefText;
this.CommandId = CommandId;
this.pTargets = pTargets;
this.pArgs = pArgs;
this.NumTargets = NumTargets;
this.NumArgs = NumArgs;
this.pFragment = pFragment;
if ((URLParts.length != this.URLParts.length)) {
throw new WrongArraySizeException("URLParts"); //$NON-NLS-1$
}
this.URLParts = URLParts;
}
public static HtmlAPIReference32Struct newInstance(final int RefType, final Pointer pRefText, final int CommandId, final Pointer pTargets, final Pointer pArgs, final int NumTargets, final int NumArgs, final Pointer pFragment, final HtmlApi_UrlComponentStruct URLParts[]) {
return AccessController.doPrivileged((PrivilegedAction) () -> new HtmlAPIReference32Struct(RefType, pRefText, CommandId, pTargets, pArgs, NumTargets, NumArgs, pFragment, URLParts));
}
public HtmlAPIReference32Struct(Pointer peer) {
super(peer);
}
public static HtmlAPIReference32Struct newInstance(final Pointer peer) {
return AccessController.doPrivileged((PrivilegedAction) () -> new HtmlAPIReference32Struct(peer));
}
public static class ByReference extends HtmlAPIReference32Struct implements Structure.ByReference {
};
public static class ByValue extends HtmlAPIReference32Struct implements Structure.ByValue {
};
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy