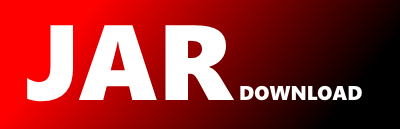
com.healthy.security.app.authentication.HealthyAuthenticationSuccessHandler Maven / Gradle / Ivy
package com.healthy.security.app.authentication;
import cn.hutool.core.map.MapUtil;
import cn.hutool.extra.spring.SpringUtil;
import com.healthy.common.core.support.Message;
import com.healthy.common.core.util.WebUtils;
import com.healthy.common.security.authentication.event.AuthenticationFailureLoggerEvent;
import com.healthy.common.security.authentication.event.AuthenticationSuccessLoggerEvent;
import lombok.extern.slf4j.Slf4j;
import org.springframework.security.authentication.BadCredentialsException;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.AuthenticationException;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.security.oauth2.common.OAuth2AccessToken;
import org.springframework.security.oauth2.common.exceptions.UnapprovedClientAuthenticationException;
import org.springframework.security.oauth2.provider.*;
import org.springframework.security.oauth2.provider.token.AuthorizationServerTokenServices;
import org.springframework.security.web.authentication.SavedRequestAwareAuthenticationSuccessHandler;
import org.springframework.security.web.authentication.www.BasicAuthenticationConverter;
import org.springframework.stereotype.Component;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* AuthenticationSuccessHandler After successful authentication, the
* authentication information is returned to the user
*
* @author xiaomingzhang
*/
@Slf4j
@Component("healthyAuthenticationSuccessHandler")
public class HealthyAuthenticationSuccessHandler extends SavedRequestAwareAuthenticationSuccessHandler {
private final BasicAuthenticationConverter authenticationConverter = new BasicAuthenticationConverter();
@Resource
private PasswordEncoder passwordEncoder;
@Resource
private ClientDetailsService clientDetailsService;
@Resource
private AuthorizationServerTokenServices authorizationServerTokenServices;
@Override
public void onAuthenticationSuccess(HttpServletRequest request, HttpServletResponse response,
Authentication authentication) {
log.info("认证成功: [{}]", authentication.getName());
try {
UsernamePasswordAuthenticationToken authRequest = authenticationConverter.convert(request);
if (authRequest == null) {
throw new BadCredentialsException("Empty basic authentication token");
}
String clientId = authRequest.getName();
String clientSecret = authRequest.getCredentials().toString();
if (log.isDebugEnabled()) {
log.debug("Basic Authentication Authorization header found for user '" + clientId + "'");
}
ClientDetails clientDetails = clientDetailsService.loadClientByClientId(clientId);
if (!passwordEncoder.matches(clientSecret, clientDetails.getClientSecret())) {
throw new UnapprovedClientAuthenticationException("client secret is incorrect");
}
TokenRequest tokenRequest = new TokenRequest(MapUtil.newHashMap(), clientDetails.getClientId(),
clientDetails.getScope(), "custom");
OAuth2Request oAuth2Request = tokenRequest.createOAuth2Request(clientDetails);
OAuth2Authentication oAuth2Authentication = new OAuth2Authentication(oAuth2Request, authentication);
OAuth2AccessToken token = authorizationServerTokenServices.createAccessToken(oAuth2Authentication);
SpringUtil.publishEvent(new AuthenticationSuccessLoggerEvent(authentication));
WebUtils.renderJson(response, Message.ok(token));
}
catch (ClientRegistrationException e) {
log.error(e.getMessage(), e);
WebUtils.renderJson(response, Message.failed(e.getMessage()));
}
catch (AuthenticationException e) {
log.error(e.getMessage(), e);
SpringUtil.publishEvent(new AuthenticationFailureLoggerEvent(e));
response.setStatus(HttpServletResponse.SC_UNAUTHORIZED);
WebUtils.renderJson(response, Message.failed(e.getMessage()));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy