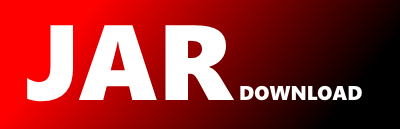
com.healthy.common.security.jackson2.UserDeserializer Maven / Gradle / Ivy
The newest version!
package com.healthy.common.security.jackson2;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.DeserializationContext;
import com.fasterxml.jackson.databind.JsonDeserializer;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.MissingNode;
import com.healthy.common.security.userdetails.User;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.authority.SimpleGrantedAuthority;
import java.io.IOException;
import java.util.Collection;
import java.util.Map;
/**
* 自定义的 User jackson 反序列化器
*
* 参考 {@link UserDeserializer}
*
* @author xm.z
*/
public class UserDeserializer extends JsonDeserializer {
private static final TypeReference> SIMPLE_GRANTED_AUTHORITY_SET = new TypeReference>() {
};
private static final TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy