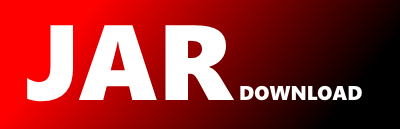
com.healthy.security.browser.authentication.HealthyAuthenticationFailureHandler Maven / Gradle / Ivy
package com.healthy.security.browser.authentication;
import cn.hutool.json.JSONUtil;
import com.healthy.common.core.support.Message;
import com.healthy.common.security.properties.LoginResponseType;
import com.healthy.common.security.properties.SecurityProperties;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.security.core.AuthenticationException;
import org.springframework.security.web.authentication.SimpleUrlAuthenticationFailureHandler;
import org.springframework.stereotype.Component;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import static com.healthy.common.core.constant.CommonConstants.APPLICATION_JSON_UTF8_VALUE;
/**
* AuthenticationFailureHandler which performs a redirect to the value of the
* onAuthenticationFailure method is called.
* it will send a 401 response to the client, with the error message from the
* AuthenticationException which caused the failure.
*
*
* @author xiaomingzhang
*/
@Slf4j
@Component("healthyAuthenticationFailureHandler")
public class HealthyAuthenticationFailureHandler extends SimpleUrlAuthenticationFailureHandler {
@Autowired
private SecurityProperties securityProperties;
@Override
public void onAuthenticationFailure(HttpServletRequest request, HttpServletResponse response, AuthenticationException exception) throws IOException, ServletException {
log.info("登录失败, 原因: {}", exception.getMessage());
if (LoginResponseType.JSON.equals(securityProperties.getBrowser().getSignInResponseType())) {
response.setStatus(HttpStatus.UNAUTHORIZED.value());
response.setContentType(APPLICATION_JSON_UTF8_VALUE);
response.getWriter().write(JSONUtil.toJsonStr(Message.failed(exception.getMessage())));
} else {
setDefaultFailureUrl(securityProperties.getBrowser().getSignInPage());
super.onAuthenticationFailure(request, response, exception);
}
}
}