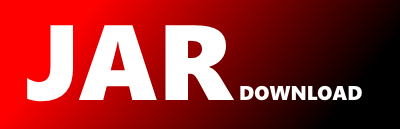
com.healthy.common.qcloud.cos.QcloudCos Maven / Gradle / Ivy
package com.healthy.common.qcloud.cos;
import cn.hutool.core.collection.CollUtil;
import cn.hutool.core.date.DateTime;
import cn.hutool.core.date.DateUtil;
import cn.hutool.core.io.FileTypeUtil;
import cn.hutool.core.io.file.FileNameUtil;
import cn.hutool.core.lang.Validator;
import cn.hutool.core.util.CharUtil;
import cn.hutool.core.util.HexUtil;
import cn.hutool.core.util.IdUtil;
import cn.hutool.core.util.StrUtil;
import com.qcloud.cos.COSClient;
import com.qcloud.cos.ClientConfig;
import com.qcloud.cos.auth.AnonymousCOSCredentials;
import com.qcloud.cos.auth.BasicCOSCredentials;
import com.qcloud.cos.auth.COSCredentials;
import com.qcloud.cos.http.HttpMethodName;
import com.qcloud.cos.http.HttpProtocol;
import com.qcloud.cos.model.GeneratePresignedUrlRequest;
import com.qcloud.cos.model.ObjectMetadata;
import com.qcloud.cos.region.Region;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
/**
* QCloudCos
*
* @author xiaomingzhang
*/
public class QcloudCos {
private static final COSCredentials CRED = new BasicCOSCredentials(QcloudCosConfig.getSecretId(),
QcloudCosConfig.getSecretKey());
public static COSClient getCosClient(String storageName) {
return getCosClient(getStorageConfig(storageName));
}
private static COSClient getCosClient(QcloudStorageConfig qcloudStorageConfig) {
ClientConfig clientConfig = new ClientConfig(new Region(qcloudStorageConfig.getRegion()));
clientConfig.setHttpProtocol(HttpProtocol.https);
return new COSClient(CRED, clientConfig);
}
public static QcloudStorageConfig getStorageConfig(String storageName) {
List storages = QcloudCosConfig.getStorages();
if (CollUtil.isEmpty(storages)) {
throw new RuntimeException("请配置腾讯云存储相关密钥信息.");
}
return storages.stream().filter(v -> v.getName().equals(storageName)).findFirst()
.orElseThrow(() -> new RuntimeException("无效的腾讯云存储配置: " + storageName));
}
/**
* 构建上传文件名称
*
* 示例:{folderName}/{alias}.{suffix}
* @param folderName 文件夹名称
* @param alias 文件别名
* @param suffix 文件扩展名
*/
public static String buildKey(String folderName, String alias, String suffix) {
StringBuilder key = new StringBuilder(folderName + CharUtil.SLASH + alias);
if (StrUtil.isNotBlank(suffix)) {
key.append(CharUtil.DOT).append(suffix);
}
return key.toString();
}
/**
* 文件上传
* @param storageName 存储名称
* @param folderName 文件夹名称
* @param bytes 文件字节数组
*/
public static KeyPath upload(String storageName, String folderName, byte[] bytes) throws IOException {
return upload(getStorageConfig(storageName), folderName, bytes);
}
/**
* 文件上传
* @param storageConfig 存储配置
* @param folderName 文件夹名称
* @param bytes 文件字节数组
*/
public static KeyPath upload(QcloudStorageConfig storageConfig, String folderName, byte[] bytes)
throws IOException {
String suffix = FileTypeUtil.getType(HexUtil.encodeHexStr(bytes, false));
return upload(storageConfig, folderName, suffix, bytes);
}
/**
* 文件上传
* @param storageName 存储名称
* @param folderName 文件夹名称
* @param folderName 文件扩展名
* @param bytes 文件字节数组
*/
public static KeyPath upload(String storageName, String folderName, String suffix, byte[] bytes)
throws IOException {
return upload(getStorageConfig(storageName), folderName, suffix, bytes);
}
/**
* 文件上传
* @param storageConfig 存储配置
* @param folderName 文件夹名称
* @param folderName 文件扩展名
* @param bytes 文件字节数组
*/
public static KeyPath upload(QcloudStorageConfig storageConfig, String folderName, String suffix, byte[] bytes)
throws IOException {
return upload(storageConfig, folderName, IdUtil.fastSimpleUUID(), suffix, bytes);
}
/**
* 文件上传
* @param storageName 存储名称
* @param folderName 文件夹名称
* @param file 上传的文件
*/
public static KeyPath upload(String storageName, String folderName, File file) {
return upload(getStorageConfig(storageName), folderName, file);
}
/**
* 文件上传
* @param storageConfig 存储配置
* @param folderName 文件夹名称
* @param file 上传的文件
*/
public static KeyPath upload(QcloudStorageConfig storageConfig, String folderName, File file) {
String suffix = StrUtil.blankToDefault(FileNameUtil.getSuffix(file), FileTypeUtil.getType(file));
return upload(storageConfig, folderName, suffix, file);
}
/**
* 文件上传
* @param storageName 存储名称
* @param folderName 文件夹名称
* @param file 上传的文件
*/
public static KeyPath upload(String storageName, String folderName, String suffix, File file) {
return upload(getStorageConfig(storageName), folderName, suffix, file);
}
/**
* 文件上传
* @param storageConfig 存储配置
* @param folderName 文件夹名称
* @param file 上传的文件
*/
public static KeyPath upload(QcloudStorageConfig storageConfig, String folderName, String suffix, File file) {
return upload(storageConfig, folderName, IdUtil.fastSimpleUUID(), suffix, file);
}
/**
* 文件上传
* @param storageConfig 存储配置
* @param folderName 文件夹名称
* @param alias 文件别名
* @param bytes 文件字节数组
*/
public static KeyPath upload(QcloudStorageConfig storageConfig, String folderName, String alias, String suffix,
byte[] bytes) throws IOException {
KeyPath keyPath;
try (InputStream inputStream = new ByteArrayInputStream(bytes)) {
String key = buildKey(folderName, alias, suffix);
COSClient cosClient = getCosClient(storageConfig);
ObjectMetadata meta = new ObjectMetadata();
// 必须设置ContentLength
meta.setContentLength(bytes.length);
cosClient.putObject(storageConfig.getBucketName(), key, inputStream, meta);
String url = generatePreSignUrl(cosClient, storageConfig, HttpMethodName.GET, key);
keyPath = new KeyPath(key, url);
}
return keyPath;
}
/**
* 文件上传
* @param storageConfig 存储配置
* @param folderName 文件夹名称
* @param cosFile 上传的文件
* @param alias 文件别名
*/
public static KeyPath upload(QcloudStorageConfig storageConfig, String folderName, String alias, String suffix,
File cosFile) {
String key = buildKey(folderName, alias, suffix);
COSClient cosClient = getCosClient(storageConfig);
String bucketName = storageConfig.getBucketName();
cosClient.putObject(bucketName, key, cosFile);
String url = generatePreSignUrl(cosClient, storageConfig, HttpMethodName.GET, key);
return new KeyPath(key, url);
}
/**
* 文件删除
* @param storageName 存储名称
* @param key 文件名
*/
public static void delKey(String storageName, String key) {
delKey(getStorageConfig(storageName), key);
}
/**
* 文件删除
* @param storageConfig 存储配置
* @param key 文件名
*/
public static void delKey(QcloudStorageConfig storageConfig, String key) {
COSClient cosClient = getCosClient(storageConfig);
cosClient.deleteObject(storageConfig.getBucketName(), key);
cosClient.shutdown();
}
/**
* 生成预签名网址
*
* 默认GET请求方式
* @param storageName 存储名称
* @param key 文件名
*/
public static String generatePreSignUrl(String storageName, String key) {
return generatePreSignUrl(getStorageConfig(storageName), HttpMethodName.GET, key);
}
/**
* 生成预签名网址
*
* 默认GET请求方式
* @param storageConfig 存储配置
* @param key 文件名
*/
public static String generatePreSignUrl(QcloudStorageConfig storageConfig, String key) {
return generatePreSignUrl(storageConfig, HttpMethodName.GET, key);
}
/**
* 生成预签名网址
* @param storageConfig 存储配置
* @param method 请求方式
* @param key 文件名
*/
public static String generatePreSignUrl(QcloudStorageConfig storageConfig, HttpMethodName method, String key) {
return generatePreSignUrl(getCosClient(storageConfig), storageConfig, method, key);
}
/**
* 生成预签名网址
* @param cosClient 存储客户端
* @param storageConfig 存储配置
* @param method 请求方式
* @param key 文件名
*/
public static String generatePreSignUrl(COSClient cosClient, QcloudStorageConfig storageConfig,
HttpMethodName method, String key) {
try {
if (StrUtil.isNotBlank(key) && !Validator.isUrl(key)) {
GeneratePresignedUrlRequest generatePresignedUrlRequest = new GeneratePresignedUrlRequest(
storageConfig.getBucketName(), key, method);
if (storageConfig.isSign()) {
DateTime expiration = DateUtil.offsetMinute(DateUtil.date(), storageConfig.getExpiration());
generatePresignedUrlRequest.setExpiration(expiration);
}
else {
cosClient.setCOSCredentials(new AnonymousCOSCredentials());
}
key = cosClient.generatePresignedUrl(generatePresignedUrlRequest).toString();
generatePresignedUrlRequest.clone();
}
}
finally {
if (cosClient != null) {
cosClient.shutdown();
}
}
return key;
}
}