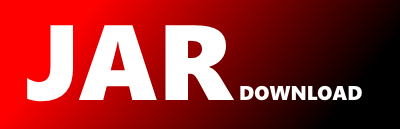
com.hedera.node.app.hapi.utils.sysfiles.serdes.FeesJsonToProtoSerde Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of app-hapi-utils Show documentation
Show all versions of app-hapi-utils Show documentation
Hedera Services API Utilities
/*
* Copyright (C) 2020-2024 Hedera Hashgraph, LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.hedera.node.app.hapi.utils.sysfiles.serdes;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.hederahashgraph.api.proto.java.CurrentAndNextFeeSchedule;
import com.hederahashgraph.api.proto.java.FeeComponents;
import com.hederahashgraph.api.proto.java.FeeData;
import com.hederahashgraph.api.proto.java.FeeSchedule;
import com.hederahashgraph.api.proto.java.HederaFunctionality;
import com.hederahashgraph.api.proto.java.SubType;
import com.hederahashgraph.api.proto.java.TimestampSeconds;
import com.hederahashgraph.api.proto.java.TransactionFeeSchedule;
import java.io.IOException;
import java.io.InputStream;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.List;
import java.util.Map;
/**
* Provides data binding from the fee schedules JSON created by the Hedera product team, to the
* {@link CurrentAndNextFeeSchedule} protobuf type.
*/
@SuppressWarnings("unchecked")
public class FeesJsonToProtoSerde {
private static final String[] FEE_SCHEDULE_KEYS = {"currentFeeSchedule", "nextFeeSchedule"};
private static final String EXPIRY_TIME_KEY = "expiryTime";
private static final String TXN_FEE_SCHEDULE_KEY = "transactionFeeSchedule";
private static final String FEE_DATA_KEY = "fees";
private static final String HEDERA_FUNCTION_KEY = "hederaFunctionality";
private static final String[] FEE_COMPONENT_KEYS = {"nodedata", "networkdata", "servicedata"};
private static final String[] RESOURCE_KEYS = {
"constant", "bpt", "vpt", "rbh", "sbh", "gas", "bpr", "sbpr", "min", "max"
};
private FeesJsonToProtoSerde() {
throw new UnsupportedOperationException("Static utilities class, should not be instantiated");
}
public static CurrentAndNextFeeSchedule loadFeeScheduleFromJson(String jsonResource)
throws IOException, InvocationTargetException, NoSuchMethodException, IllegalAccessException {
return buildFrom(om -> om.readValue(
FeesJsonToProtoSerde.class.getClassLoader().getResourceAsStream(jsonResource), List.class));
}
public static CurrentAndNextFeeSchedule loadFeeScheduleFromStream(InputStream in)
throws IOException, InvocationTargetException, NoSuchMethodException, IllegalAccessException {
return buildFrom(om -> om.readValue(in, List.class));
}
public static CurrentAndNextFeeSchedule parseFeeScheduleFromJson(String literal)
throws IOException, InvocationTargetException, NoSuchMethodException, IllegalAccessException {
return buildFrom(om -> om.readValue(literal, List.class));
}
private static CurrentAndNextFeeSchedule buildFrom(ThrowingReader reader)
throws IOException, InvocationTargetException, NoSuchMethodException, IllegalAccessException {
final var feeSchedules = CurrentAndNextFeeSchedule.newBuilder();
final var om = new ObjectMapper();
final var rawFeeSchedules = reader.readValueWith(om);
constructWithBuilder(feeSchedules, rawFeeSchedules);
return feeSchedules.build();
}
interface ThrowingReader {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy