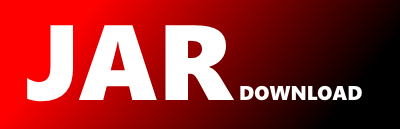
com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: SystemDelete.proto
package com.hederahashgraph.api.proto.java;
/**
*
*Delete a file or smart contract - can only be done with a Hedera administrative multisignature. When it is deleted, it immediately disappears from the system as seen by the user, but is still stored internally until the expiration time, at which time it is truly and permanently deleted. Until that time, it can be undeleted by the Hedera administrative multisignature. When a smart contract is deleted, the cryptocurrency account within it continues to exist, and is not affected by the expiration time here.
*
*
* Protobuf type {@code proto.SystemDeleteTransactionBody}
*/
public final class SystemDeleteTransactionBody extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:proto.SystemDeleteTransactionBody)
SystemDeleteTransactionBodyOrBuilder {
private static final long serialVersionUID = 0L;
// Use SystemDeleteTransactionBody.newBuilder() to construct.
private SystemDeleteTransactionBody(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SystemDeleteTransactionBody() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SystemDeleteTransactionBody();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SystemDeleteTransactionBody(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.hederahashgraph.api.proto.java.FileID.Builder subBuilder = null;
if (idCase_ == 1) {
subBuilder = ((com.hederahashgraph.api.proto.java.FileID) id_).toBuilder();
}
id_ =
input.readMessage(com.hederahashgraph.api.proto.java.FileID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.hederahashgraph.api.proto.java.FileID) id_);
id_ = subBuilder.buildPartial();
}
idCase_ = 1;
break;
}
case 18: {
com.hederahashgraph.api.proto.java.ContractID.Builder subBuilder = null;
if (idCase_ == 2) {
subBuilder = ((com.hederahashgraph.api.proto.java.ContractID) id_).toBuilder();
}
id_ =
input.readMessage(com.hederahashgraph.api.proto.java.ContractID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.hederahashgraph.api.proto.java.ContractID) id_);
id_ = subBuilder.buildPartial();
}
idCase_ = 2;
break;
}
case 26: {
com.hederahashgraph.api.proto.java.TimestampSeconds.Builder subBuilder = null;
if (expirationTime_ != null) {
subBuilder = expirationTime_.toBuilder();
}
expirationTime_ = input.readMessage(com.hederahashgraph.api.proto.java.TimestampSeconds.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(expirationTime_);
expirationTime_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.hederahashgraph.api.proto.java.SystemDelete.internal_static_proto_SystemDeleteTransactionBody_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.hederahashgraph.api.proto.java.SystemDelete.internal_static_proto_SystemDeleteTransactionBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody.class, com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody.Builder.class);
}
private int idCase_ = 0;
private java.lang.Object id_;
public enum IdCase
implements com.google.protobuf.Internal.EnumLite {
FILEID(1),
CONTRACTID(2),
ID_NOT_SET(0);
private final int value;
private IdCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static IdCase valueOf(int value) {
return forNumber(value);
}
public static IdCase forNumber(int value) {
switch (value) {
case 1: return FILEID;
case 2: return CONTRACTID;
case 0: return ID_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public IdCase
getIdCase() {
return IdCase.forNumber(
idCase_);
}
public static final int FILEID_FIELD_NUMBER = 1;
/**
*
* The file ID of the file to delete, in the format used in transactions
*
*
* .proto.FileID fileID = 1;
*/
public boolean hasFileID() {
return idCase_ == 1;
}
/**
*
* The file ID of the file to delete, in the format used in transactions
*
*
* .proto.FileID fileID = 1;
*/
public com.hederahashgraph.api.proto.java.FileID getFileID() {
if (idCase_ == 1) {
return (com.hederahashgraph.api.proto.java.FileID) id_;
}
return com.hederahashgraph.api.proto.java.FileID.getDefaultInstance();
}
/**
*
* The file ID of the file to delete, in the format used in transactions
*
*
* .proto.FileID fileID = 1;
*/
public com.hederahashgraph.api.proto.java.FileIDOrBuilder getFileIDOrBuilder() {
if (idCase_ == 1) {
return (com.hederahashgraph.api.proto.java.FileID) id_;
}
return com.hederahashgraph.api.proto.java.FileID.getDefaultInstance();
}
public static final int CONTRACTID_FIELD_NUMBER = 2;
/**
*
* The contract ID instance to delete, in the format used in transactions
*
*
* .proto.ContractID contractID = 2;
*/
public boolean hasContractID() {
return idCase_ == 2;
}
/**
*
* The contract ID instance to delete, in the format used in transactions
*
*
* .proto.ContractID contractID = 2;
*/
public com.hederahashgraph.api.proto.java.ContractID getContractID() {
if (idCase_ == 2) {
return (com.hederahashgraph.api.proto.java.ContractID) id_;
}
return com.hederahashgraph.api.proto.java.ContractID.getDefaultInstance();
}
/**
*
* The contract ID instance to delete, in the format used in transactions
*
*
* .proto.ContractID contractID = 2;
*/
public com.hederahashgraph.api.proto.java.ContractIDOrBuilder getContractIDOrBuilder() {
if (idCase_ == 2) {
return (com.hederahashgraph.api.proto.java.ContractID) id_;
}
return com.hederahashgraph.api.proto.java.ContractID.getDefaultInstance();
}
public static final int EXPIRATIONTIME_FIELD_NUMBER = 3;
private com.hederahashgraph.api.proto.java.TimestampSeconds expirationTime_;
/**
*
* The timestamp in seconds at which the "deleted" file should truly be permanently deleted
*
*
* .proto.TimestampSeconds expirationTime = 3;
*/
public boolean hasExpirationTime() {
return expirationTime_ != null;
}
/**
*
* The timestamp in seconds at which the "deleted" file should truly be permanently deleted
*
*
* .proto.TimestampSeconds expirationTime = 3;
*/
public com.hederahashgraph.api.proto.java.TimestampSeconds getExpirationTime() {
return expirationTime_ == null ? com.hederahashgraph.api.proto.java.TimestampSeconds.getDefaultInstance() : expirationTime_;
}
/**
*
* The timestamp in seconds at which the "deleted" file should truly be permanently deleted
*
*
* .proto.TimestampSeconds expirationTime = 3;
*/
public com.hederahashgraph.api.proto.java.TimestampSecondsOrBuilder getExpirationTimeOrBuilder() {
return getExpirationTime();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (idCase_ == 1) {
output.writeMessage(1, (com.hederahashgraph.api.proto.java.FileID) id_);
}
if (idCase_ == 2) {
output.writeMessage(2, (com.hederahashgraph.api.proto.java.ContractID) id_);
}
if (expirationTime_ != null) {
output.writeMessage(3, getExpirationTime());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (idCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, (com.hederahashgraph.api.proto.java.FileID) id_);
}
if (idCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (com.hederahashgraph.api.proto.java.ContractID) id_);
}
if (expirationTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getExpirationTime());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody)) {
return super.equals(obj);
}
com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody other = (com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody) obj;
if (hasExpirationTime() != other.hasExpirationTime()) return false;
if (hasExpirationTime()) {
if (!getExpirationTime()
.equals(other.getExpirationTime())) return false;
}
if (!getIdCase().equals(other.getIdCase())) return false;
switch (idCase_) {
case 1:
if (!getFileID()
.equals(other.getFileID())) return false;
break;
case 2:
if (!getContractID()
.equals(other.getContractID())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasExpirationTime()) {
hash = (37 * hash) + EXPIRATIONTIME_FIELD_NUMBER;
hash = (53 * hash) + getExpirationTime().hashCode();
}
switch (idCase_) {
case 1:
hash = (37 * hash) + FILEID_FIELD_NUMBER;
hash = (53 * hash) + getFileID().hashCode();
break;
case 2:
hash = (37 * hash) + CONTRACTID_FIELD_NUMBER;
hash = (53 * hash) + getContractID().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*Delete a file or smart contract - can only be done with a Hedera administrative multisignature. When it is deleted, it immediately disappears from the system as seen by the user, but is still stored internally until the expiration time, at which time it is truly and permanently deleted. Until that time, it can be undeleted by the Hedera administrative multisignature. When a smart contract is deleted, the cryptocurrency account within it continues to exist, and is not affected by the expiration time here.
*
*
* Protobuf type {@code proto.SystemDeleteTransactionBody}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:proto.SystemDeleteTransactionBody)
com.hederahashgraph.api.proto.java.SystemDeleteTransactionBodyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.hederahashgraph.api.proto.java.SystemDelete.internal_static_proto_SystemDeleteTransactionBody_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.hederahashgraph.api.proto.java.SystemDelete.internal_static_proto_SystemDeleteTransactionBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody.class, com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody.Builder.class);
}
// Construct using com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (expirationTimeBuilder_ == null) {
expirationTime_ = null;
} else {
expirationTime_ = null;
expirationTimeBuilder_ = null;
}
idCase_ = 0;
id_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.hederahashgraph.api.proto.java.SystemDelete.internal_static_proto_SystemDeleteTransactionBody_descriptor;
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody getDefaultInstanceForType() {
return com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody.getDefaultInstance();
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody build() {
com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody buildPartial() {
com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody result = new com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody(this);
if (idCase_ == 1) {
if (fileIDBuilder_ == null) {
result.id_ = id_;
} else {
result.id_ = fileIDBuilder_.build();
}
}
if (idCase_ == 2) {
if (contractIDBuilder_ == null) {
result.id_ = id_;
} else {
result.id_ = contractIDBuilder_.build();
}
}
if (expirationTimeBuilder_ == null) {
result.expirationTime_ = expirationTime_;
} else {
result.expirationTime_ = expirationTimeBuilder_.build();
}
result.idCase_ = idCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody) {
return mergeFrom((com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody other) {
if (other == com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody.getDefaultInstance()) return this;
if (other.hasExpirationTime()) {
mergeExpirationTime(other.getExpirationTime());
}
switch (other.getIdCase()) {
case FILEID: {
mergeFileID(other.getFileID());
break;
}
case CONTRACTID: {
mergeContractID(other.getContractID());
break;
}
case ID_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int idCase_ = 0;
private java.lang.Object id_;
public IdCase
getIdCase() {
return IdCase.forNumber(
idCase_);
}
public Builder clearId() {
idCase_ = 0;
id_ = null;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.FileID, com.hederahashgraph.api.proto.java.FileID.Builder, com.hederahashgraph.api.proto.java.FileIDOrBuilder> fileIDBuilder_;
/**
*
* The file ID of the file to delete, in the format used in transactions
*
*
* .proto.FileID fileID = 1;
*/
public boolean hasFileID() {
return idCase_ == 1;
}
/**
*
* The file ID of the file to delete, in the format used in transactions
*
*
* .proto.FileID fileID = 1;
*/
public com.hederahashgraph.api.proto.java.FileID getFileID() {
if (fileIDBuilder_ == null) {
if (idCase_ == 1) {
return (com.hederahashgraph.api.proto.java.FileID) id_;
}
return com.hederahashgraph.api.proto.java.FileID.getDefaultInstance();
} else {
if (idCase_ == 1) {
return fileIDBuilder_.getMessage();
}
return com.hederahashgraph.api.proto.java.FileID.getDefaultInstance();
}
}
/**
*
* The file ID of the file to delete, in the format used in transactions
*
*
* .proto.FileID fileID = 1;
*/
public Builder setFileID(com.hederahashgraph.api.proto.java.FileID value) {
if (fileIDBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
} else {
fileIDBuilder_.setMessage(value);
}
idCase_ = 1;
return this;
}
/**
*
* The file ID of the file to delete, in the format used in transactions
*
*
* .proto.FileID fileID = 1;
*/
public Builder setFileID(
com.hederahashgraph.api.proto.java.FileID.Builder builderForValue) {
if (fileIDBuilder_ == null) {
id_ = builderForValue.build();
onChanged();
} else {
fileIDBuilder_.setMessage(builderForValue.build());
}
idCase_ = 1;
return this;
}
/**
*
* The file ID of the file to delete, in the format used in transactions
*
*
* .proto.FileID fileID = 1;
*/
public Builder mergeFileID(com.hederahashgraph.api.proto.java.FileID value) {
if (fileIDBuilder_ == null) {
if (idCase_ == 1 &&
id_ != com.hederahashgraph.api.proto.java.FileID.getDefaultInstance()) {
id_ = com.hederahashgraph.api.proto.java.FileID.newBuilder((com.hederahashgraph.api.proto.java.FileID) id_)
.mergeFrom(value).buildPartial();
} else {
id_ = value;
}
onChanged();
} else {
if (idCase_ == 1) {
fileIDBuilder_.mergeFrom(value);
}
fileIDBuilder_.setMessage(value);
}
idCase_ = 1;
return this;
}
/**
*
* The file ID of the file to delete, in the format used in transactions
*
*
* .proto.FileID fileID = 1;
*/
public Builder clearFileID() {
if (fileIDBuilder_ == null) {
if (idCase_ == 1) {
idCase_ = 0;
id_ = null;
onChanged();
}
} else {
if (idCase_ == 1) {
idCase_ = 0;
id_ = null;
}
fileIDBuilder_.clear();
}
return this;
}
/**
*
* The file ID of the file to delete, in the format used in transactions
*
*
* .proto.FileID fileID = 1;
*/
public com.hederahashgraph.api.proto.java.FileID.Builder getFileIDBuilder() {
return getFileIDFieldBuilder().getBuilder();
}
/**
*
* The file ID of the file to delete, in the format used in transactions
*
*
* .proto.FileID fileID = 1;
*/
public com.hederahashgraph.api.proto.java.FileIDOrBuilder getFileIDOrBuilder() {
if ((idCase_ == 1) && (fileIDBuilder_ != null)) {
return fileIDBuilder_.getMessageOrBuilder();
} else {
if (idCase_ == 1) {
return (com.hederahashgraph.api.proto.java.FileID) id_;
}
return com.hederahashgraph.api.proto.java.FileID.getDefaultInstance();
}
}
/**
*
* The file ID of the file to delete, in the format used in transactions
*
*
* .proto.FileID fileID = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.FileID, com.hederahashgraph.api.proto.java.FileID.Builder, com.hederahashgraph.api.proto.java.FileIDOrBuilder>
getFileIDFieldBuilder() {
if (fileIDBuilder_ == null) {
if (!(idCase_ == 1)) {
id_ = com.hederahashgraph.api.proto.java.FileID.getDefaultInstance();
}
fileIDBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.FileID, com.hederahashgraph.api.proto.java.FileID.Builder, com.hederahashgraph.api.proto.java.FileIDOrBuilder>(
(com.hederahashgraph.api.proto.java.FileID) id_,
getParentForChildren(),
isClean());
id_ = null;
}
idCase_ = 1;
onChanged();;
return fileIDBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.ContractID, com.hederahashgraph.api.proto.java.ContractID.Builder, com.hederahashgraph.api.proto.java.ContractIDOrBuilder> contractIDBuilder_;
/**
*
* The contract ID instance to delete, in the format used in transactions
*
*
* .proto.ContractID contractID = 2;
*/
public boolean hasContractID() {
return idCase_ == 2;
}
/**
*
* The contract ID instance to delete, in the format used in transactions
*
*
* .proto.ContractID contractID = 2;
*/
public com.hederahashgraph.api.proto.java.ContractID getContractID() {
if (contractIDBuilder_ == null) {
if (idCase_ == 2) {
return (com.hederahashgraph.api.proto.java.ContractID) id_;
}
return com.hederahashgraph.api.proto.java.ContractID.getDefaultInstance();
} else {
if (idCase_ == 2) {
return contractIDBuilder_.getMessage();
}
return com.hederahashgraph.api.proto.java.ContractID.getDefaultInstance();
}
}
/**
*
* The contract ID instance to delete, in the format used in transactions
*
*
* .proto.ContractID contractID = 2;
*/
public Builder setContractID(com.hederahashgraph.api.proto.java.ContractID value) {
if (contractIDBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
} else {
contractIDBuilder_.setMessage(value);
}
idCase_ = 2;
return this;
}
/**
*
* The contract ID instance to delete, in the format used in transactions
*
*
* .proto.ContractID contractID = 2;
*/
public Builder setContractID(
com.hederahashgraph.api.proto.java.ContractID.Builder builderForValue) {
if (contractIDBuilder_ == null) {
id_ = builderForValue.build();
onChanged();
} else {
contractIDBuilder_.setMessage(builderForValue.build());
}
idCase_ = 2;
return this;
}
/**
*
* The contract ID instance to delete, in the format used in transactions
*
*
* .proto.ContractID contractID = 2;
*/
public Builder mergeContractID(com.hederahashgraph.api.proto.java.ContractID value) {
if (contractIDBuilder_ == null) {
if (idCase_ == 2 &&
id_ != com.hederahashgraph.api.proto.java.ContractID.getDefaultInstance()) {
id_ = com.hederahashgraph.api.proto.java.ContractID.newBuilder((com.hederahashgraph.api.proto.java.ContractID) id_)
.mergeFrom(value).buildPartial();
} else {
id_ = value;
}
onChanged();
} else {
if (idCase_ == 2) {
contractIDBuilder_.mergeFrom(value);
}
contractIDBuilder_.setMessage(value);
}
idCase_ = 2;
return this;
}
/**
*
* The contract ID instance to delete, in the format used in transactions
*
*
* .proto.ContractID contractID = 2;
*/
public Builder clearContractID() {
if (contractIDBuilder_ == null) {
if (idCase_ == 2) {
idCase_ = 0;
id_ = null;
onChanged();
}
} else {
if (idCase_ == 2) {
idCase_ = 0;
id_ = null;
}
contractIDBuilder_.clear();
}
return this;
}
/**
*
* The contract ID instance to delete, in the format used in transactions
*
*
* .proto.ContractID contractID = 2;
*/
public com.hederahashgraph.api.proto.java.ContractID.Builder getContractIDBuilder() {
return getContractIDFieldBuilder().getBuilder();
}
/**
*
* The contract ID instance to delete, in the format used in transactions
*
*
* .proto.ContractID contractID = 2;
*/
public com.hederahashgraph.api.proto.java.ContractIDOrBuilder getContractIDOrBuilder() {
if ((idCase_ == 2) && (contractIDBuilder_ != null)) {
return contractIDBuilder_.getMessageOrBuilder();
} else {
if (idCase_ == 2) {
return (com.hederahashgraph.api.proto.java.ContractID) id_;
}
return com.hederahashgraph.api.proto.java.ContractID.getDefaultInstance();
}
}
/**
*
* The contract ID instance to delete, in the format used in transactions
*
*
* .proto.ContractID contractID = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.ContractID, com.hederahashgraph.api.proto.java.ContractID.Builder, com.hederahashgraph.api.proto.java.ContractIDOrBuilder>
getContractIDFieldBuilder() {
if (contractIDBuilder_ == null) {
if (!(idCase_ == 2)) {
id_ = com.hederahashgraph.api.proto.java.ContractID.getDefaultInstance();
}
contractIDBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.ContractID, com.hederahashgraph.api.proto.java.ContractID.Builder, com.hederahashgraph.api.proto.java.ContractIDOrBuilder>(
(com.hederahashgraph.api.proto.java.ContractID) id_,
getParentForChildren(),
isClean());
id_ = null;
}
idCase_ = 2;
onChanged();;
return contractIDBuilder_;
}
private com.hederahashgraph.api.proto.java.TimestampSeconds expirationTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.TimestampSeconds, com.hederahashgraph.api.proto.java.TimestampSeconds.Builder, com.hederahashgraph.api.proto.java.TimestampSecondsOrBuilder> expirationTimeBuilder_;
/**
*
* The timestamp in seconds at which the "deleted" file should truly be permanently deleted
*
*
* .proto.TimestampSeconds expirationTime = 3;
*/
public boolean hasExpirationTime() {
return expirationTimeBuilder_ != null || expirationTime_ != null;
}
/**
*
* The timestamp in seconds at which the "deleted" file should truly be permanently deleted
*
*
* .proto.TimestampSeconds expirationTime = 3;
*/
public com.hederahashgraph.api.proto.java.TimestampSeconds getExpirationTime() {
if (expirationTimeBuilder_ == null) {
return expirationTime_ == null ? com.hederahashgraph.api.proto.java.TimestampSeconds.getDefaultInstance() : expirationTime_;
} else {
return expirationTimeBuilder_.getMessage();
}
}
/**
*
* The timestamp in seconds at which the "deleted" file should truly be permanently deleted
*
*
* .proto.TimestampSeconds expirationTime = 3;
*/
public Builder setExpirationTime(com.hederahashgraph.api.proto.java.TimestampSeconds value) {
if (expirationTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
expirationTime_ = value;
onChanged();
} else {
expirationTimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* The timestamp in seconds at which the "deleted" file should truly be permanently deleted
*
*
* .proto.TimestampSeconds expirationTime = 3;
*/
public Builder setExpirationTime(
com.hederahashgraph.api.proto.java.TimestampSeconds.Builder builderForValue) {
if (expirationTimeBuilder_ == null) {
expirationTime_ = builderForValue.build();
onChanged();
} else {
expirationTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The timestamp in seconds at which the "deleted" file should truly be permanently deleted
*
*
* .proto.TimestampSeconds expirationTime = 3;
*/
public Builder mergeExpirationTime(com.hederahashgraph.api.proto.java.TimestampSeconds value) {
if (expirationTimeBuilder_ == null) {
if (expirationTime_ != null) {
expirationTime_ =
com.hederahashgraph.api.proto.java.TimestampSeconds.newBuilder(expirationTime_).mergeFrom(value).buildPartial();
} else {
expirationTime_ = value;
}
onChanged();
} else {
expirationTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The timestamp in seconds at which the "deleted" file should truly be permanently deleted
*
*
* .proto.TimestampSeconds expirationTime = 3;
*/
public Builder clearExpirationTime() {
if (expirationTimeBuilder_ == null) {
expirationTime_ = null;
onChanged();
} else {
expirationTime_ = null;
expirationTimeBuilder_ = null;
}
return this;
}
/**
*
* The timestamp in seconds at which the "deleted" file should truly be permanently deleted
*
*
* .proto.TimestampSeconds expirationTime = 3;
*/
public com.hederahashgraph.api.proto.java.TimestampSeconds.Builder getExpirationTimeBuilder() {
onChanged();
return getExpirationTimeFieldBuilder().getBuilder();
}
/**
*
* The timestamp in seconds at which the "deleted" file should truly be permanently deleted
*
*
* .proto.TimestampSeconds expirationTime = 3;
*/
public com.hederahashgraph.api.proto.java.TimestampSecondsOrBuilder getExpirationTimeOrBuilder() {
if (expirationTimeBuilder_ != null) {
return expirationTimeBuilder_.getMessageOrBuilder();
} else {
return expirationTime_ == null ?
com.hederahashgraph.api.proto.java.TimestampSeconds.getDefaultInstance() : expirationTime_;
}
}
/**
*
* The timestamp in seconds at which the "deleted" file should truly be permanently deleted
*
*
* .proto.TimestampSeconds expirationTime = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.TimestampSeconds, com.hederahashgraph.api.proto.java.TimestampSeconds.Builder, com.hederahashgraph.api.proto.java.TimestampSecondsOrBuilder>
getExpirationTimeFieldBuilder() {
if (expirationTimeBuilder_ == null) {
expirationTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.TimestampSeconds, com.hederahashgraph.api.proto.java.TimestampSeconds.Builder, com.hederahashgraph.api.proto.java.TimestampSecondsOrBuilder>(
getExpirationTime(),
getParentForChildren(),
isClean());
expirationTime_ = null;
}
return expirationTimeBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:proto.SystemDeleteTransactionBody)
}
// @@protoc_insertion_point(class_scope:proto.SystemDeleteTransactionBody)
private static final com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody();
}
public static com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SystemDeleteTransactionBody parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SystemDeleteTransactionBody(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.SystemDeleteTransactionBody getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy