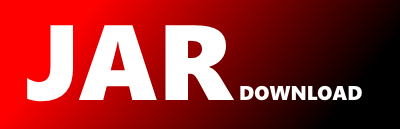
com.hederahashgraph.api.proto.java.TransactionID Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: BasicTypes.proto
package com.hederahashgraph.api.proto.java;
/**
*
* The ID for a transaction. This is used for retrieving receipts and records for a transaction, for appending to a file right after creating it, for instantiating a smart contract with bytecode in a file just created, and internally by the network for detecting when duplicate transactions are submitted. A user might get a transaction processed faster by submitting it to N nodes, each with a different node account, but all with the same TransactionID. Then, the transaction will take effect when the first of all those nodes submits the transaction and it reaches consensus. The other transactions will not take effect. So this could make the transaction take effect faster, if any given node might be slow. However, the full transaction fee is charged for each transaction, so the total fee is N times as much if the transaction is sent to N nodes.
*
*
* Protobuf type {@code proto.TransactionID}
*/
public final class TransactionID extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:proto.TransactionID)
TransactionIDOrBuilder {
private static final long serialVersionUID = 0L;
// Use TransactionID.newBuilder() to construct.
private TransactionID(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TransactionID() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TransactionID();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TransactionID(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.hederahashgraph.api.proto.java.Timestamp.Builder subBuilder = null;
if (transactionValidStart_ != null) {
subBuilder = transactionValidStart_.toBuilder();
}
transactionValidStart_ = input.readMessage(com.hederahashgraph.api.proto.java.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(transactionValidStart_);
transactionValidStart_ = subBuilder.buildPartial();
}
break;
}
case 18: {
com.hederahashgraph.api.proto.java.AccountID.Builder subBuilder = null;
if (accountID_ != null) {
subBuilder = accountID_.toBuilder();
}
accountID_ = input.readMessage(com.hederahashgraph.api.proto.java.AccountID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(accountID_);
accountID_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.hederahashgraph.api.proto.java.BasicTypes.internal_static_proto_TransactionID_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.hederahashgraph.api.proto.java.BasicTypes.internal_static_proto_TransactionID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.hederahashgraph.api.proto.java.TransactionID.class, com.hederahashgraph.api.proto.java.TransactionID.Builder.class);
}
public static final int TRANSACTIONVALIDSTART_FIELD_NUMBER = 1;
private com.hederahashgraph.api.proto.java.Timestamp transactionValidStart_;
/**
*
* The transaction is invalid if consensusTimestamp < transactionID.transactionStartValid
*
*
* .proto.Timestamp transactionValidStart = 1;
*/
public boolean hasTransactionValidStart() {
return transactionValidStart_ != null;
}
/**
*
* The transaction is invalid if consensusTimestamp < transactionID.transactionStartValid
*
*
* .proto.Timestamp transactionValidStart = 1;
*/
public com.hederahashgraph.api.proto.java.Timestamp getTransactionValidStart() {
return transactionValidStart_ == null ? com.hederahashgraph.api.proto.java.Timestamp.getDefaultInstance() : transactionValidStart_;
}
/**
*
* The transaction is invalid if consensusTimestamp < transactionID.transactionStartValid
*
*
* .proto.Timestamp transactionValidStart = 1;
*/
public com.hederahashgraph.api.proto.java.TimestampOrBuilder getTransactionValidStartOrBuilder() {
return getTransactionValidStart();
}
public static final int ACCOUNTID_FIELD_NUMBER = 2;
private com.hederahashgraph.api.proto.java.AccountID accountID_;
/**
*
*The Account ID that paid for this transaction
*
*
* .proto.AccountID accountID = 2;
*/
public boolean hasAccountID() {
return accountID_ != null;
}
/**
*
*The Account ID that paid for this transaction
*
*
* .proto.AccountID accountID = 2;
*/
public com.hederahashgraph.api.proto.java.AccountID getAccountID() {
return accountID_ == null ? com.hederahashgraph.api.proto.java.AccountID.getDefaultInstance() : accountID_;
}
/**
*
*The Account ID that paid for this transaction
*
*
* .proto.AccountID accountID = 2;
*/
public com.hederahashgraph.api.proto.java.AccountIDOrBuilder getAccountIDOrBuilder() {
return getAccountID();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (transactionValidStart_ != null) {
output.writeMessage(1, getTransactionValidStart());
}
if (accountID_ != null) {
output.writeMessage(2, getAccountID());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (transactionValidStart_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getTransactionValidStart());
}
if (accountID_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getAccountID());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.hederahashgraph.api.proto.java.TransactionID)) {
return super.equals(obj);
}
com.hederahashgraph.api.proto.java.TransactionID other = (com.hederahashgraph.api.proto.java.TransactionID) obj;
if (hasTransactionValidStart() != other.hasTransactionValidStart()) return false;
if (hasTransactionValidStart()) {
if (!getTransactionValidStart()
.equals(other.getTransactionValidStart())) return false;
}
if (hasAccountID() != other.hasAccountID()) return false;
if (hasAccountID()) {
if (!getAccountID()
.equals(other.getAccountID())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTransactionValidStart()) {
hash = (37 * hash) + TRANSACTIONVALIDSTART_FIELD_NUMBER;
hash = (53 * hash) + getTransactionValidStart().hashCode();
}
if (hasAccountID()) {
hash = (37 * hash) + ACCOUNTID_FIELD_NUMBER;
hash = (53 * hash) + getAccountID().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.hederahashgraph.api.proto.java.TransactionID parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.TransactionID parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.TransactionID parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.TransactionID parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.TransactionID parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.TransactionID parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.TransactionID parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.TransactionID parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.TransactionID parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.TransactionID parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.TransactionID parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.TransactionID parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.hederahashgraph.api.proto.java.TransactionID prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The ID for a transaction. This is used for retrieving receipts and records for a transaction, for appending to a file right after creating it, for instantiating a smart contract with bytecode in a file just created, and internally by the network for detecting when duplicate transactions are submitted. A user might get a transaction processed faster by submitting it to N nodes, each with a different node account, but all with the same TransactionID. Then, the transaction will take effect when the first of all those nodes submits the transaction and it reaches consensus. The other transactions will not take effect. So this could make the transaction take effect faster, if any given node might be slow. However, the full transaction fee is charged for each transaction, so the total fee is N times as much if the transaction is sent to N nodes.
*
*
* Protobuf type {@code proto.TransactionID}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:proto.TransactionID)
com.hederahashgraph.api.proto.java.TransactionIDOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.hederahashgraph.api.proto.java.BasicTypes.internal_static_proto_TransactionID_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.hederahashgraph.api.proto.java.BasicTypes.internal_static_proto_TransactionID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.hederahashgraph.api.proto.java.TransactionID.class, com.hederahashgraph.api.proto.java.TransactionID.Builder.class);
}
// Construct using com.hederahashgraph.api.proto.java.TransactionID.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (transactionValidStartBuilder_ == null) {
transactionValidStart_ = null;
} else {
transactionValidStart_ = null;
transactionValidStartBuilder_ = null;
}
if (accountIDBuilder_ == null) {
accountID_ = null;
} else {
accountID_ = null;
accountIDBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.hederahashgraph.api.proto.java.BasicTypes.internal_static_proto_TransactionID_descriptor;
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.TransactionID getDefaultInstanceForType() {
return com.hederahashgraph.api.proto.java.TransactionID.getDefaultInstance();
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.TransactionID build() {
com.hederahashgraph.api.proto.java.TransactionID result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.TransactionID buildPartial() {
com.hederahashgraph.api.proto.java.TransactionID result = new com.hederahashgraph.api.proto.java.TransactionID(this);
if (transactionValidStartBuilder_ == null) {
result.transactionValidStart_ = transactionValidStart_;
} else {
result.transactionValidStart_ = transactionValidStartBuilder_.build();
}
if (accountIDBuilder_ == null) {
result.accountID_ = accountID_;
} else {
result.accountID_ = accountIDBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.hederahashgraph.api.proto.java.TransactionID) {
return mergeFrom((com.hederahashgraph.api.proto.java.TransactionID)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.hederahashgraph.api.proto.java.TransactionID other) {
if (other == com.hederahashgraph.api.proto.java.TransactionID.getDefaultInstance()) return this;
if (other.hasTransactionValidStart()) {
mergeTransactionValidStart(other.getTransactionValidStart());
}
if (other.hasAccountID()) {
mergeAccountID(other.getAccountID());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.hederahashgraph.api.proto.java.TransactionID parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.hederahashgraph.api.proto.java.TransactionID) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.hederahashgraph.api.proto.java.Timestamp transactionValidStart_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Timestamp, com.hederahashgraph.api.proto.java.Timestamp.Builder, com.hederahashgraph.api.proto.java.TimestampOrBuilder> transactionValidStartBuilder_;
/**
*
* The transaction is invalid if consensusTimestamp < transactionID.transactionStartValid
*
*
* .proto.Timestamp transactionValidStart = 1;
*/
public boolean hasTransactionValidStart() {
return transactionValidStartBuilder_ != null || transactionValidStart_ != null;
}
/**
*
* The transaction is invalid if consensusTimestamp < transactionID.transactionStartValid
*
*
* .proto.Timestamp transactionValidStart = 1;
*/
public com.hederahashgraph.api.proto.java.Timestamp getTransactionValidStart() {
if (transactionValidStartBuilder_ == null) {
return transactionValidStart_ == null ? com.hederahashgraph.api.proto.java.Timestamp.getDefaultInstance() : transactionValidStart_;
} else {
return transactionValidStartBuilder_.getMessage();
}
}
/**
*
* The transaction is invalid if consensusTimestamp < transactionID.transactionStartValid
*
*
* .proto.Timestamp transactionValidStart = 1;
*/
public Builder setTransactionValidStart(com.hederahashgraph.api.proto.java.Timestamp value) {
if (transactionValidStartBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
transactionValidStart_ = value;
onChanged();
} else {
transactionValidStartBuilder_.setMessage(value);
}
return this;
}
/**
*
* The transaction is invalid if consensusTimestamp < transactionID.transactionStartValid
*
*
* .proto.Timestamp transactionValidStart = 1;
*/
public Builder setTransactionValidStart(
com.hederahashgraph.api.proto.java.Timestamp.Builder builderForValue) {
if (transactionValidStartBuilder_ == null) {
transactionValidStart_ = builderForValue.build();
onChanged();
} else {
transactionValidStartBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The transaction is invalid if consensusTimestamp < transactionID.transactionStartValid
*
*
* .proto.Timestamp transactionValidStart = 1;
*/
public Builder mergeTransactionValidStart(com.hederahashgraph.api.proto.java.Timestamp value) {
if (transactionValidStartBuilder_ == null) {
if (transactionValidStart_ != null) {
transactionValidStart_ =
com.hederahashgraph.api.proto.java.Timestamp.newBuilder(transactionValidStart_).mergeFrom(value).buildPartial();
} else {
transactionValidStart_ = value;
}
onChanged();
} else {
transactionValidStartBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The transaction is invalid if consensusTimestamp < transactionID.transactionStartValid
*
*
* .proto.Timestamp transactionValidStart = 1;
*/
public Builder clearTransactionValidStart() {
if (transactionValidStartBuilder_ == null) {
transactionValidStart_ = null;
onChanged();
} else {
transactionValidStart_ = null;
transactionValidStartBuilder_ = null;
}
return this;
}
/**
*
* The transaction is invalid if consensusTimestamp < transactionID.transactionStartValid
*
*
* .proto.Timestamp transactionValidStart = 1;
*/
public com.hederahashgraph.api.proto.java.Timestamp.Builder getTransactionValidStartBuilder() {
onChanged();
return getTransactionValidStartFieldBuilder().getBuilder();
}
/**
*
* The transaction is invalid if consensusTimestamp < transactionID.transactionStartValid
*
*
* .proto.Timestamp transactionValidStart = 1;
*/
public com.hederahashgraph.api.proto.java.TimestampOrBuilder getTransactionValidStartOrBuilder() {
if (transactionValidStartBuilder_ != null) {
return transactionValidStartBuilder_.getMessageOrBuilder();
} else {
return transactionValidStart_ == null ?
com.hederahashgraph.api.proto.java.Timestamp.getDefaultInstance() : transactionValidStart_;
}
}
/**
*
* The transaction is invalid if consensusTimestamp < transactionID.transactionStartValid
*
*
* .proto.Timestamp transactionValidStart = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Timestamp, com.hederahashgraph.api.proto.java.Timestamp.Builder, com.hederahashgraph.api.proto.java.TimestampOrBuilder>
getTransactionValidStartFieldBuilder() {
if (transactionValidStartBuilder_ == null) {
transactionValidStartBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Timestamp, com.hederahashgraph.api.proto.java.Timestamp.Builder, com.hederahashgraph.api.proto.java.TimestampOrBuilder>(
getTransactionValidStart(),
getParentForChildren(),
isClean());
transactionValidStart_ = null;
}
return transactionValidStartBuilder_;
}
private com.hederahashgraph.api.proto.java.AccountID accountID_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.AccountID, com.hederahashgraph.api.proto.java.AccountID.Builder, com.hederahashgraph.api.proto.java.AccountIDOrBuilder> accountIDBuilder_;
/**
*
*The Account ID that paid for this transaction
*
*
* .proto.AccountID accountID = 2;
*/
public boolean hasAccountID() {
return accountIDBuilder_ != null || accountID_ != null;
}
/**
*
*The Account ID that paid for this transaction
*
*
* .proto.AccountID accountID = 2;
*/
public com.hederahashgraph.api.proto.java.AccountID getAccountID() {
if (accountIDBuilder_ == null) {
return accountID_ == null ? com.hederahashgraph.api.proto.java.AccountID.getDefaultInstance() : accountID_;
} else {
return accountIDBuilder_.getMessage();
}
}
/**
*
*The Account ID that paid for this transaction
*
*
* .proto.AccountID accountID = 2;
*/
public Builder setAccountID(com.hederahashgraph.api.proto.java.AccountID value) {
if (accountIDBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
accountID_ = value;
onChanged();
} else {
accountIDBuilder_.setMessage(value);
}
return this;
}
/**
*
*The Account ID that paid for this transaction
*
*
* .proto.AccountID accountID = 2;
*/
public Builder setAccountID(
com.hederahashgraph.api.proto.java.AccountID.Builder builderForValue) {
if (accountIDBuilder_ == null) {
accountID_ = builderForValue.build();
onChanged();
} else {
accountIDBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
*The Account ID that paid for this transaction
*
*
* .proto.AccountID accountID = 2;
*/
public Builder mergeAccountID(com.hederahashgraph.api.proto.java.AccountID value) {
if (accountIDBuilder_ == null) {
if (accountID_ != null) {
accountID_ =
com.hederahashgraph.api.proto.java.AccountID.newBuilder(accountID_).mergeFrom(value).buildPartial();
} else {
accountID_ = value;
}
onChanged();
} else {
accountIDBuilder_.mergeFrom(value);
}
return this;
}
/**
*
*The Account ID that paid for this transaction
*
*
* .proto.AccountID accountID = 2;
*/
public Builder clearAccountID() {
if (accountIDBuilder_ == null) {
accountID_ = null;
onChanged();
} else {
accountID_ = null;
accountIDBuilder_ = null;
}
return this;
}
/**
*
*The Account ID that paid for this transaction
*
*
* .proto.AccountID accountID = 2;
*/
public com.hederahashgraph.api.proto.java.AccountID.Builder getAccountIDBuilder() {
onChanged();
return getAccountIDFieldBuilder().getBuilder();
}
/**
*
*The Account ID that paid for this transaction
*
*
* .proto.AccountID accountID = 2;
*/
public com.hederahashgraph.api.proto.java.AccountIDOrBuilder getAccountIDOrBuilder() {
if (accountIDBuilder_ != null) {
return accountIDBuilder_.getMessageOrBuilder();
} else {
return accountID_ == null ?
com.hederahashgraph.api.proto.java.AccountID.getDefaultInstance() : accountID_;
}
}
/**
*
*The Account ID that paid for this transaction
*
*
* .proto.AccountID accountID = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.AccountID, com.hederahashgraph.api.proto.java.AccountID.Builder, com.hederahashgraph.api.proto.java.AccountIDOrBuilder>
getAccountIDFieldBuilder() {
if (accountIDBuilder_ == null) {
accountIDBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.AccountID, com.hederahashgraph.api.proto.java.AccountID.Builder, com.hederahashgraph.api.proto.java.AccountIDOrBuilder>(
getAccountID(),
getParentForChildren(),
isClean());
accountID_ = null;
}
return accountIDBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:proto.TransactionID)
}
// @@protoc_insertion_point(class_scope:proto.TransactionID)
private static final com.hederahashgraph.api.proto.java.TransactionID DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.hederahashgraph.api.proto.java.TransactionID();
}
public static com.hederahashgraph.api.proto.java.TransactionID getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TransactionID parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TransactionID(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.TransactionID getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy