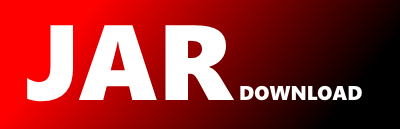
com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: CryptoUpdate.proto
package com.hederahashgraph.api.proto.java;
/**
*
*
*Change properties for the given account. Any null field is ignored (left unchanged). This transaction must be signed by the existing key for this account. If the transaction is changing the key field, then the transaction must be signed by both the old key (from before the change) and the new key. The old key must sign for security. The new key must sign as a safeguard to avoid accidentally changing to an invalid key, and then having no way to recover.
*
*
* Protobuf type {@code proto.CryptoUpdateTransactionBody}
*/
public final class CryptoUpdateTransactionBody extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:proto.CryptoUpdateTransactionBody)
CryptoUpdateTransactionBodyOrBuilder {
private static final long serialVersionUID = 0L;
// Use CryptoUpdateTransactionBody.newBuilder() to construct.
private CryptoUpdateTransactionBody(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CryptoUpdateTransactionBody() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CryptoUpdateTransactionBody();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CryptoUpdateTransactionBody(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 18: {
com.hederahashgraph.api.proto.java.AccountID.Builder subBuilder = null;
if (accountIDToUpdate_ != null) {
subBuilder = accountIDToUpdate_.toBuilder();
}
accountIDToUpdate_ = input.readMessage(com.hederahashgraph.api.proto.java.AccountID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(accountIDToUpdate_);
accountIDToUpdate_ = subBuilder.buildPartial();
}
break;
}
case 26: {
com.hederahashgraph.api.proto.java.Key.Builder subBuilder = null;
if (key_ != null) {
subBuilder = key_.toBuilder();
}
key_ = input.readMessage(com.hederahashgraph.api.proto.java.Key.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(key_);
key_ = subBuilder.buildPartial();
}
break;
}
case 34: {
com.hederahashgraph.api.proto.java.AccountID.Builder subBuilder = null;
if (proxyAccountID_ != null) {
subBuilder = proxyAccountID_.toBuilder();
}
proxyAccountID_ = input.readMessage(com.hederahashgraph.api.proto.java.AccountID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(proxyAccountID_);
proxyAccountID_ = subBuilder.buildPartial();
}
break;
}
case 40: {
proxyFraction_ = input.readInt32();
break;
}
case 48: {
sendRecordThresholdFieldCase_ = 6;
sendRecordThresholdField_ = input.readUInt64();
break;
}
case 56: {
receiveRecordThresholdFieldCase_ = 7;
receiveRecordThresholdField_ = input.readUInt64();
break;
}
case 66: {
com.hederahashgraph.api.proto.java.Duration.Builder subBuilder = null;
if (autoRenewPeriod_ != null) {
subBuilder = autoRenewPeriod_.toBuilder();
}
autoRenewPeriod_ = input.readMessage(com.hederahashgraph.api.proto.java.Duration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(autoRenewPeriod_);
autoRenewPeriod_ = subBuilder.buildPartial();
}
break;
}
case 74: {
com.hederahashgraph.api.proto.java.Timestamp.Builder subBuilder = null;
if (expirationTime_ != null) {
subBuilder = expirationTime_.toBuilder();
}
expirationTime_ = input.readMessage(com.hederahashgraph.api.proto.java.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(expirationTime_);
expirationTime_ = subBuilder.buildPartial();
}
break;
}
case 80: {
receiverSigRequiredFieldCase_ = 10;
receiverSigRequiredField_ = input.readBool();
break;
}
case 90: {
com.google.protobuf.UInt64Value.Builder subBuilder = null;
if (sendRecordThresholdFieldCase_ == 11) {
subBuilder = ((com.google.protobuf.UInt64Value) sendRecordThresholdField_).toBuilder();
}
sendRecordThresholdField_ =
input.readMessage(com.google.protobuf.UInt64Value.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.google.protobuf.UInt64Value) sendRecordThresholdField_);
sendRecordThresholdField_ = subBuilder.buildPartial();
}
sendRecordThresholdFieldCase_ = 11;
break;
}
case 98: {
com.google.protobuf.UInt64Value.Builder subBuilder = null;
if (receiveRecordThresholdFieldCase_ == 12) {
subBuilder = ((com.google.protobuf.UInt64Value) receiveRecordThresholdField_).toBuilder();
}
receiveRecordThresholdField_ =
input.readMessage(com.google.protobuf.UInt64Value.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.google.protobuf.UInt64Value) receiveRecordThresholdField_);
receiveRecordThresholdField_ = subBuilder.buildPartial();
}
receiveRecordThresholdFieldCase_ = 12;
break;
}
case 106: {
com.google.protobuf.BoolValue.Builder subBuilder = null;
if (receiverSigRequiredFieldCase_ == 13) {
subBuilder = ((com.google.protobuf.BoolValue) receiverSigRequiredField_).toBuilder();
}
receiverSigRequiredField_ =
input.readMessage(com.google.protobuf.BoolValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.google.protobuf.BoolValue) receiverSigRequiredField_);
receiverSigRequiredField_ = subBuilder.buildPartial();
}
receiverSigRequiredFieldCase_ = 13;
break;
}
case 114: {
com.google.protobuf.StringValue.Builder subBuilder = null;
if (memo_ != null) {
subBuilder = memo_.toBuilder();
}
memo_ = input.readMessage(com.google.protobuf.StringValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(memo_);
memo_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.hederahashgraph.api.proto.java.CryptoUpdate.internal_static_proto_CryptoUpdateTransactionBody_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.hederahashgraph.api.proto.java.CryptoUpdate.internal_static_proto_CryptoUpdateTransactionBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody.class, com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody.Builder.class);
}
private int sendRecordThresholdFieldCase_ = 0;
private java.lang.Object sendRecordThresholdField_;
public enum SendRecordThresholdFieldCase
implements com.google.protobuf.Internal.EnumLite {
@java.lang.Deprecated SENDRECORDTHRESHOLD(6),
@java.lang.Deprecated SENDRECORDTHRESHOLDWRAPPER(11),
SENDRECORDTHRESHOLDFIELD_NOT_SET(0);
private final int value;
private SendRecordThresholdFieldCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static SendRecordThresholdFieldCase valueOf(int value) {
return forNumber(value);
}
public static SendRecordThresholdFieldCase forNumber(int value) {
switch (value) {
case 6: return SENDRECORDTHRESHOLD;
case 11: return SENDRECORDTHRESHOLDWRAPPER;
case 0: return SENDRECORDTHRESHOLDFIELD_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public SendRecordThresholdFieldCase
getSendRecordThresholdFieldCase() {
return SendRecordThresholdFieldCase.forNumber(
sendRecordThresholdFieldCase_);
}
private int receiveRecordThresholdFieldCase_ = 0;
private java.lang.Object receiveRecordThresholdField_;
public enum ReceiveRecordThresholdFieldCase
implements com.google.protobuf.Internal.EnumLite {
@java.lang.Deprecated RECEIVERECORDTHRESHOLD(7),
@java.lang.Deprecated RECEIVERECORDTHRESHOLDWRAPPER(12),
RECEIVERECORDTHRESHOLDFIELD_NOT_SET(0);
private final int value;
private ReceiveRecordThresholdFieldCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ReceiveRecordThresholdFieldCase valueOf(int value) {
return forNumber(value);
}
public static ReceiveRecordThresholdFieldCase forNumber(int value) {
switch (value) {
case 7: return RECEIVERECORDTHRESHOLD;
case 12: return RECEIVERECORDTHRESHOLDWRAPPER;
case 0: return RECEIVERECORDTHRESHOLDFIELD_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ReceiveRecordThresholdFieldCase
getReceiveRecordThresholdFieldCase() {
return ReceiveRecordThresholdFieldCase.forNumber(
receiveRecordThresholdFieldCase_);
}
private int receiverSigRequiredFieldCase_ = 0;
private java.lang.Object receiverSigRequiredField_;
public enum ReceiverSigRequiredFieldCase
implements com.google.protobuf.Internal.EnumLite {
@java.lang.Deprecated RECEIVERSIGREQUIRED(10),
RECEIVERSIGREQUIREDWRAPPER(13),
RECEIVERSIGREQUIREDFIELD_NOT_SET(0);
private final int value;
private ReceiverSigRequiredFieldCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ReceiverSigRequiredFieldCase valueOf(int value) {
return forNumber(value);
}
public static ReceiverSigRequiredFieldCase forNumber(int value) {
switch (value) {
case 10: return RECEIVERSIGREQUIRED;
case 13: return RECEIVERSIGREQUIREDWRAPPER;
case 0: return RECEIVERSIGREQUIREDFIELD_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ReceiverSigRequiredFieldCase
getReceiverSigRequiredFieldCase() {
return ReceiverSigRequiredFieldCase.forNumber(
receiverSigRequiredFieldCase_);
}
public static final int ACCOUNTIDTOUPDATE_FIELD_NUMBER = 2;
private com.hederahashgraph.api.proto.java.AccountID accountIDToUpdate_;
/**
*
* The account ID which is being updated in this transaction
*
*
* .proto.AccountID accountIDToUpdate = 2;
*/
public boolean hasAccountIDToUpdate() {
return accountIDToUpdate_ != null;
}
/**
*
* The account ID which is being updated in this transaction
*
*
* .proto.AccountID accountIDToUpdate = 2;
*/
public com.hederahashgraph.api.proto.java.AccountID getAccountIDToUpdate() {
return accountIDToUpdate_ == null ? com.hederahashgraph.api.proto.java.AccountID.getDefaultInstance() : accountIDToUpdate_;
}
/**
*
* The account ID which is being updated in this transaction
*
*
* .proto.AccountID accountIDToUpdate = 2;
*/
public com.hederahashgraph.api.proto.java.AccountIDOrBuilder getAccountIDToUpdateOrBuilder() {
return getAccountIDToUpdate();
}
public static final int KEY_FIELD_NUMBER = 3;
private com.hederahashgraph.api.proto.java.Key key_;
/**
*
* The new key
*
*
* .proto.Key key = 3;
*/
public boolean hasKey() {
return key_ != null;
}
/**
*
* The new key
*
*
* .proto.Key key = 3;
*/
public com.hederahashgraph.api.proto.java.Key getKey() {
return key_ == null ? com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : key_;
}
/**
*
* The new key
*
*
* .proto.Key key = 3;
*/
public com.hederahashgraph.api.proto.java.KeyOrBuilder getKeyOrBuilder() {
return getKey();
}
public static final int PROXYACCOUNTID_FIELD_NUMBER = 4;
private com.hederahashgraph.api.proto.java.AccountID proxyAccountID_;
/**
*
* ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an invalid account, or is an account that isn't a node, then this account is automatically proxy staked to a node chosen by the network, but without earning payments. If the proxyAccountID account refuses to accept proxy staking , or if it is not currently running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 4;
*/
public boolean hasProxyAccountID() {
return proxyAccountID_ != null;
}
/**
*
* ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an invalid account, or is an account that isn't a node, then this account is automatically proxy staked to a node chosen by the network, but without earning payments. If the proxyAccountID account refuses to accept proxy staking , or if it is not currently running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 4;
*/
public com.hederahashgraph.api.proto.java.AccountID getProxyAccountID() {
return proxyAccountID_ == null ? com.hederahashgraph.api.proto.java.AccountID.getDefaultInstance() : proxyAccountID_;
}
/**
*
* ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an invalid account, or is an account that isn't a node, then this account is automatically proxy staked to a node chosen by the network, but without earning payments. If the proxyAccountID account refuses to accept proxy staking , or if it is not currently running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 4;
*/
public com.hederahashgraph.api.proto.java.AccountIDOrBuilder getProxyAccountIDOrBuilder() {
return getProxyAccountID();
}
public static final int PROXYFRACTION_FIELD_NUMBER = 5;
private int proxyFraction_;
/**
*
* [Deprecated]. Payments earned from proxy staking are shared between the node and this account, with proxyFraction / 10000 going to this account
*
*
* int32 proxyFraction = 5 [deprecated = true];
*/
@java.lang.Deprecated public int getProxyFraction() {
return proxyFraction_;
}
public static final int SENDRECORDTHRESHOLD_FIELD_NUMBER = 6;
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* uint64 sendRecordThreshold = 6 [deprecated = true];
*/
@java.lang.Deprecated public long getSendRecordThreshold() {
if (sendRecordThresholdFieldCase_ == 6) {
return (java.lang.Long) sendRecordThresholdField_;
}
return 0L;
}
public static final int SENDRECORDTHRESHOLDWRAPPER_FIELD_NUMBER = 11;
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* .google.protobuf.UInt64Value sendRecordThresholdWrapper = 11 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasSendRecordThresholdWrapper() {
return sendRecordThresholdFieldCase_ == 11;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* .google.protobuf.UInt64Value sendRecordThresholdWrapper = 11 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.UInt64Value getSendRecordThresholdWrapper() {
if (sendRecordThresholdFieldCase_ == 11) {
return (com.google.protobuf.UInt64Value) sendRecordThresholdField_;
}
return com.google.protobuf.UInt64Value.getDefaultInstance();
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* .google.protobuf.UInt64Value sendRecordThresholdWrapper = 11 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.UInt64ValueOrBuilder getSendRecordThresholdWrapperOrBuilder() {
if (sendRecordThresholdFieldCase_ == 11) {
return (com.google.protobuf.UInt64Value) sendRecordThresholdField_;
}
return com.google.protobuf.UInt64Value.getDefaultInstance();
}
public static final int RECEIVERECORDTHRESHOLD_FIELD_NUMBER = 7;
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* uint64 receiveRecordThreshold = 7 [deprecated = true];
*/
@java.lang.Deprecated public long getReceiveRecordThreshold() {
if (receiveRecordThresholdFieldCase_ == 7) {
return (java.lang.Long) receiveRecordThresholdField_;
}
return 0L;
}
public static final int RECEIVERECORDTHRESHOLDWRAPPER_FIELD_NUMBER = 12;
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* .google.protobuf.UInt64Value receiveRecordThresholdWrapper = 12 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasReceiveRecordThresholdWrapper() {
return receiveRecordThresholdFieldCase_ == 12;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* .google.protobuf.UInt64Value receiveRecordThresholdWrapper = 12 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.UInt64Value getReceiveRecordThresholdWrapper() {
if (receiveRecordThresholdFieldCase_ == 12) {
return (com.google.protobuf.UInt64Value) receiveRecordThresholdField_;
}
return com.google.protobuf.UInt64Value.getDefaultInstance();
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* .google.protobuf.UInt64Value receiveRecordThresholdWrapper = 12 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.UInt64ValueOrBuilder getReceiveRecordThresholdWrapperOrBuilder() {
if (receiveRecordThresholdFieldCase_ == 12) {
return (com.google.protobuf.UInt64Value) receiveRecordThresholdField_;
}
return com.google.protobuf.UInt64Value.getDefaultInstance();
}
public static final int AUTORENEWPERIOD_FIELD_NUMBER = 8;
private com.hederahashgraph.api.proto.java.Duration autoRenewPeriod_;
/**
*
* The duration in which it will automatically extend the expiration period. If it doesn't have enough balance, it extends as long as possible. If it is empty when it expires, then it is deleted.
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
public boolean hasAutoRenewPeriod() {
return autoRenewPeriod_ != null;
}
/**
*
* The duration in which it will automatically extend the expiration period. If it doesn't have enough balance, it extends as long as possible. If it is empty when it expires, then it is deleted.
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
public com.hederahashgraph.api.proto.java.Duration getAutoRenewPeriod() {
return autoRenewPeriod_ == null ? com.hederahashgraph.api.proto.java.Duration.getDefaultInstance() : autoRenewPeriod_;
}
/**
*
* The duration in which it will automatically extend the expiration period. If it doesn't have enough balance, it extends as long as possible. If it is empty when it expires, then it is deleted.
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
public com.hederahashgraph.api.proto.java.DurationOrBuilder getAutoRenewPeriodOrBuilder() {
return getAutoRenewPeriod();
}
public static final int EXPIRATIONTIME_FIELD_NUMBER = 9;
private com.hederahashgraph.api.proto.java.Timestamp expirationTime_;
/**
*
* The new expiration time to extend to (ignored if equal to or before the current one)
*
*
* .proto.Timestamp expirationTime = 9;
*/
public boolean hasExpirationTime() {
return expirationTime_ != null;
}
/**
*
* The new expiration time to extend to (ignored if equal to or before the current one)
*
*
* .proto.Timestamp expirationTime = 9;
*/
public com.hederahashgraph.api.proto.java.Timestamp getExpirationTime() {
return expirationTime_ == null ? com.hederahashgraph.api.proto.java.Timestamp.getDefaultInstance() : expirationTime_;
}
/**
*
* The new expiration time to extend to (ignored if equal to or before the current one)
*
*
* .proto.Timestamp expirationTime = 9;
*/
public com.hederahashgraph.api.proto.java.TimestampOrBuilder getExpirationTimeOrBuilder() {
return getExpirationTime();
}
public static final int RECEIVERSIGREQUIRED_FIELD_NUMBER = 10;
/**
*
* [Deprecated] Do NOT use this field to set a false value because the server cannot distinguish from the default value. Use receiverSigRequiredWrapper field for this purpose.
*
*
* bool receiverSigRequired = 10 [deprecated = true];
*/
@java.lang.Deprecated public boolean getReceiverSigRequired() {
if (receiverSigRequiredFieldCase_ == 10) {
return (java.lang.Boolean) receiverSigRequiredField_;
}
return false;
}
public static final int RECEIVERSIGREQUIREDWRAPPER_FIELD_NUMBER = 13;
/**
*
* If true, this account's key must sign any transaction depositing into this account (in addition to all withdrawals)
*
*
* .google.protobuf.BoolValue receiverSigRequiredWrapper = 13;
*/
public boolean hasReceiverSigRequiredWrapper() {
return receiverSigRequiredFieldCase_ == 13;
}
/**
*
* If true, this account's key must sign any transaction depositing into this account (in addition to all withdrawals)
*
*
* .google.protobuf.BoolValue receiverSigRequiredWrapper = 13;
*/
public com.google.protobuf.BoolValue getReceiverSigRequiredWrapper() {
if (receiverSigRequiredFieldCase_ == 13) {
return (com.google.protobuf.BoolValue) receiverSigRequiredField_;
}
return com.google.protobuf.BoolValue.getDefaultInstance();
}
/**
*
* If true, this account's key must sign any transaction depositing into this account (in addition to all withdrawals)
*
*
* .google.protobuf.BoolValue receiverSigRequiredWrapper = 13;
*/
public com.google.protobuf.BoolValueOrBuilder getReceiverSigRequiredWrapperOrBuilder() {
if (receiverSigRequiredFieldCase_ == 13) {
return (com.google.protobuf.BoolValue) receiverSigRequiredField_;
}
return com.google.protobuf.BoolValue.getDefaultInstance();
}
public static final int MEMO_FIELD_NUMBER = 14;
private com.google.protobuf.StringValue memo_;
/**
*
* If set, the new memo to be associated with the account (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 14;
*/
public boolean hasMemo() {
return memo_ != null;
}
/**
*
* If set, the new memo to be associated with the account (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 14;
*/
public com.google.protobuf.StringValue getMemo() {
return memo_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : memo_;
}
/**
*
* If set, the new memo to be associated with the account (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 14;
*/
public com.google.protobuf.StringValueOrBuilder getMemoOrBuilder() {
return getMemo();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (accountIDToUpdate_ != null) {
output.writeMessage(2, getAccountIDToUpdate());
}
if (key_ != null) {
output.writeMessage(3, getKey());
}
if (proxyAccountID_ != null) {
output.writeMessage(4, getProxyAccountID());
}
if (proxyFraction_ != 0) {
output.writeInt32(5, proxyFraction_);
}
if (sendRecordThresholdFieldCase_ == 6) {
output.writeUInt64(
6, (long)((java.lang.Long) sendRecordThresholdField_));
}
if (receiveRecordThresholdFieldCase_ == 7) {
output.writeUInt64(
7, (long)((java.lang.Long) receiveRecordThresholdField_));
}
if (autoRenewPeriod_ != null) {
output.writeMessage(8, getAutoRenewPeriod());
}
if (expirationTime_ != null) {
output.writeMessage(9, getExpirationTime());
}
if (receiverSigRequiredFieldCase_ == 10) {
output.writeBool(
10, (boolean)((java.lang.Boolean) receiverSigRequiredField_));
}
if (sendRecordThresholdFieldCase_ == 11) {
output.writeMessage(11, (com.google.protobuf.UInt64Value) sendRecordThresholdField_);
}
if (receiveRecordThresholdFieldCase_ == 12) {
output.writeMessage(12, (com.google.protobuf.UInt64Value) receiveRecordThresholdField_);
}
if (receiverSigRequiredFieldCase_ == 13) {
output.writeMessage(13, (com.google.protobuf.BoolValue) receiverSigRequiredField_);
}
if (memo_ != null) {
output.writeMessage(14, getMemo());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (accountIDToUpdate_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getAccountIDToUpdate());
}
if (key_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getKey());
}
if (proxyAccountID_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getProxyAccountID());
}
if (proxyFraction_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, proxyFraction_);
}
if (sendRecordThresholdFieldCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(
6, (long)((java.lang.Long) sendRecordThresholdField_));
}
if (receiveRecordThresholdFieldCase_ == 7) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(
7, (long)((java.lang.Long) receiveRecordThresholdField_));
}
if (autoRenewPeriod_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getAutoRenewPeriod());
}
if (expirationTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getExpirationTime());
}
if (receiverSigRequiredFieldCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(
10, (boolean)((java.lang.Boolean) receiverSigRequiredField_));
}
if (sendRecordThresholdFieldCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, (com.google.protobuf.UInt64Value) sendRecordThresholdField_);
}
if (receiveRecordThresholdFieldCase_ == 12) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, (com.google.protobuf.UInt64Value) receiveRecordThresholdField_);
}
if (receiverSigRequiredFieldCase_ == 13) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, (com.google.protobuf.BoolValue) receiverSigRequiredField_);
}
if (memo_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, getMemo());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody)) {
return super.equals(obj);
}
com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody other = (com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody) obj;
if (hasAccountIDToUpdate() != other.hasAccountIDToUpdate()) return false;
if (hasAccountIDToUpdate()) {
if (!getAccountIDToUpdate()
.equals(other.getAccountIDToUpdate())) return false;
}
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (hasProxyAccountID() != other.hasProxyAccountID()) return false;
if (hasProxyAccountID()) {
if (!getProxyAccountID()
.equals(other.getProxyAccountID())) return false;
}
if (getProxyFraction()
!= other.getProxyFraction()) return false;
if (hasAutoRenewPeriod() != other.hasAutoRenewPeriod()) return false;
if (hasAutoRenewPeriod()) {
if (!getAutoRenewPeriod()
.equals(other.getAutoRenewPeriod())) return false;
}
if (hasExpirationTime() != other.hasExpirationTime()) return false;
if (hasExpirationTime()) {
if (!getExpirationTime()
.equals(other.getExpirationTime())) return false;
}
if (hasMemo() != other.hasMemo()) return false;
if (hasMemo()) {
if (!getMemo()
.equals(other.getMemo())) return false;
}
if (!getSendRecordThresholdFieldCase().equals(other.getSendRecordThresholdFieldCase())) return false;
switch (sendRecordThresholdFieldCase_) {
case 6:
if (getSendRecordThreshold()
!= other.getSendRecordThreshold()) return false;
break;
case 11:
if (!getSendRecordThresholdWrapper()
.equals(other.getSendRecordThresholdWrapper())) return false;
break;
case 0:
default:
}
if (!getReceiveRecordThresholdFieldCase().equals(other.getReceiveRecordThresholdFieldCase())) return false;
switch (receiveRecordThresholdFieldCase_) {
case 7:
if (getReceiveRecordThreshold()
!= other.getReceiveRecordThreshold()) return false;
break;
case 12:
if (!getReceiveRecordThresholdWrapper()
.equals(other.getReceiveRecordThresholdWrapper())) return false;
break;
case 0:
default:
}
if (!getReceiverSigRequiredFieldCase().equals(other.getReceiverSigRequiredFieldCase())) return false;
switch (receiverSigRequiredFieldCase_) {
case 10:
if (getReceiverSigRequired()
!= other.getReceiverSigRequired()) return false;
break;
case 13:
if (!getReceiverSigRequiredWrapper()
.equals(other.getReceiverSigRequiredWrapper())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccountIDToUpdate()) {
hash = (37 * hash) + ACCOUNTIDTOUPDATE_FIELD_NUMBER;
hash = (53 * hash) + getAccountIDToUpdate().hashCode();
}
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (hasProxyAccountID()) {
hash = (37 * hash) + PROXYACCOUNTID_FIELD_NUMBER;
hash = (53 * hash) + getProxyAccountID().hashCode();
}
hash = (37 * hash) + PROXYFRACTION_FIELD_NUMBER;
hash = (53 * hash) + getProxyFraction();
if (hasAutoRenewPeriod()) {
hash = (37 * hash) + AUTORENEWPERIOD_FIELD_NUMBER;
hash = (53 * hash) + getAutoRenewPeriod().hashCode();
}
if (hasExpirationTime()) {
hash = (37 * hash) + EXPIRATIONTIME_FIELD_NUMBER;
hash = (53 * hash) + getExpirationTime().hashCode();
}
if (hasMemo()) {
hash = (37 * hash) + MEMO_FIELD_NUMBER;
hash = (53 * hash) + getMemo().hashCode();
}
switch (sendRecordThresholdFieldCase_) {
case 6:
hash = (37 * hash) + SENDRECORDTHRESHOLD_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSendRecordThreshold());
break;
case 11:
hash = (37 * hash) + SENDRECORDTHRESHOLDWRAPPER_FIELD_NUMBER;
hash = (53 * hash) + getSendRecordThresholdWrapper().hashCode();
break;
case 0:
default:
}
switch (receiveRecordThresholdFieldCase_) {
case 7:
hash = (37 * hash) + RECEIVERECORDTHRESHOLD_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getReceiveRecordThreshold());
break;
case 12:
hash = (37 * hash) + RECEIVERECORDTHRESHOLDWRAPPER_FIELD_NUMBER;
hash = (53 * hash) + getReceiveRecordThresholdWrapper().hashCode();
break;
case 0:
default:
}
switch (receiverSigRequiredFieldCase_) {
case 10:
hash = (37 * hash) + RECEIVERSIGREQUIRED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getReceiverSigRequired());
break;
case 13:
hash = (37 * hash) + RECEIVERSIGREQUIREDWRAPPER_FIELD_NUMBER;
hash = (53 * hash) + getReceiverSigRequiredWrapper().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*Change properties for the given account. Any null field is ignored (left unchanged). This transaction must be signed by the existing key for this account. If the transaction is changing the key field, then the transaction must be signed by both the old key (from before the change) and the new key. The old key must sign for security. The new key must sign as a safeguard to avoid accidentally changing to an invalid key, and then having no way to recover.
*
*
* Protobuf type {@code proto.CryptoUpdateTransactionBody}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:proto.CryptoUpdateTransactionBody)
com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBodyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.hederahashgraph.api.proto.java.CryptoUpdate.internal_static_proto_CryptoUpdateTransactionBody_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.hederahashgraph.api.proto.java.CryptoUpdate.internal_static_proto_CryptoUpdateTransactionBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody.class, com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody.Builder.class);
}
// Construct using com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountIDToUpdateBuilder_ == null) {
accountIDToUpdate_ = null;
} else {
accountIDToUpdate_ = null;
accountIDToUpdateBuilder_ = null;
}
if (keyBuilder_ == null) {
key_ = null;
} else {
key_ = null;
keyBuilder_ = null;
}
if (proxyAccountIDBuilder_ == null) {
proxyAccountID_ = null;
} else {
proxyAccountID_ = null;
proxyAccountIDBuilder_ = null;
}
proxyFraction_ = 0;
if (autoRenewPeriodBuilder_ == null) {
autoRenewPeriod_ = null;
} else {
autoRenewPeriod_ = null;
autoRenewPeriodBuilder_ = null;
}
if (expirationTimeBuilder_ == null) {
expirationTime_ = null;
} else {
expirationTime_ = null;
expirationTimeBuilder_ = null;
}
if (memoBuilder_ == null) {
memo_ = null;
} else {
memo_ = null;
memoBuilder_ = null;
}
sendRecordThresholdFieldCase_ = 0;
sendRecordThresholdField_ = null;
receiveRecordThresholdFieldCase_ = 0;
receiveRecordThresholdField_ = null;
receiverSigRequiredFieldCase_ = 0;
receiverSigRequiredField_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.hederahashgraph.api.proto.java.CryptoUpdate.internal_static_proto_CryptoUpdateTransactionBody_descriptor;
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody getDefaultInstanceForType() {
return com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody.getDefaultInstance();
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody build() {
com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody buildPartial() {
com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody result = new com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody(this);
if (accountIDToUpdateBuilder_ == null) {
result.accountIDToUpdate_ = accountIDToUpdate_;
} else {
result.accountIDToUpdate_ = accountIDToUpdateBuilder_.build();
}
if (keyBuilder_ == null) {
result.key_ = key_;
} else {
result.key_ = keyBuilder_.build();
}
if (proxyAccountIDBuilder_ == null) {
result.proxyAccountID_ = proxyAccountID_;
} else {
result.proxyAccountID_ = proxyAccountIDBuilder_.build();
}
result.proxyFraction_ = proxyFraction_;
if (sendRecordThresholdFieldCase_ == 6) {
result.sendRecordThresholdField_ = sendRecordThresholdField_;
}
if (sendRecordThresholdFieldCase_ == 11) {
if (sendRecordThresholdWrapperBuilder_ == null) {
result.sendRecordThresholdField_ = sendRecordThresholdField_;
} else {
result.sendRecordThresholdField_ = sendRecordThresholdWrapperBuilder_.build();
}
}
if (receiveRecordThresholdFieldCase_ == 7) {
result.receiveRecordThresholdField_ = receiveRecordThresholdField_;
}
if (receiveRecordThresholdFieldCase_ == 12) {
if (receiveRecordThresholdWrapperBuilder_ == null) {
result.receiveRecordThresholdField_ = receiveRecordThresholdField_;
} else {
result.receiveRecordThresholdField_ = receiveRecordThresholdWrapperBuilder_.build();
}
}
if (autoRenewPeriodBuilder_ == null) {
result.autoRenewPeriod_ = autoRenewPeriod_;
} else {
result.autoRenewPeriod_ = autoRenewPeriodBuilder_.build();
}
if (expirationTimeBuilder_ == null) {
result.expirationTime_ = expirationTime_;
} else {
result.expirationTime_ = expirationTimeBuilder_.build();
}
if (receiverSigRequiredFieldCase_ == 10) {
result.receiverSigRequiredField_ = receiverSigRequiredField_;
}
if (receiverSigRequiredFieldCase_ == 13) {
if (receiverSigRequiredWrapperBuilder_ == null) {
result.receiverSigRequiredField_ = receiverSigRequiredField_;
} else {
result.receiverSigRequiredField_ = receiverSigRequiredWrapperBuilder_.build();
}
}
if (memoBuilder_ == null) {
result.memo_ = memo_;
} else {
result.memo_ = memoBuilder_.build();
}
result.sendRecordThresholdFieldCase_ = sendRecordThresholdFieldCase_;
result.receiveRecordThresholdFieldCase_ = receiveRecordThresholdFieldCase_;
result.receiverSigRequiredFieldCase_ = receiverSigRequiredFieldCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody) {
return mergeFrom((com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody other) {
if (other == com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody.getDefaultInstance()) return this;
if (other.hasAccountIDToUpdate()) {
mergeAccountIDToUpdate(other.getAccountIDToUpdate());
}
if (other.hasKey()) {
mergeKey(other.getKey());
}
if (other.hasProxyAccountID()) {
mergeProxyAccountID(other.getProxyAccountID());
}
if (other.getProxyFraction() != 0) {
setProxyFraction(other.getProxyFraction());
}
if (other.hasAutoRenewPeriod()) {
mergeAutoRenewPeriod(other.getAutoRenewPeriod());
}
if (other.hasExpirationTime()) {
mergeExpirationTime(other.getExpirationTime());
}
if (other.hasMemo()) {
mergeMemo(other.getMemo());
}
switch (other.getSendRecordThresholdFieldCase()) {
case SENDRECORDTHRESHOLD: {
setSendRecordThreshold(other.getSendRecordThreshold());
break;
}
case SENDRECORDTHRESHOLDWRAPPER: {
mergeSendRecordThresholdWrapper(other.getSendRecordThresholdWrapper());
break;
}
case SENDRECORDTHRESHOLDFIELD_NOT_SET: {
break;
}
}
switch (other.getReceiveRecordThresholdFieldCase()) {
case RECEIVERECORDTHRESHOLD: {
setReceiveRecordThreshold(other.getReceiveRecordThreshold());
break;
}
case RECEIVERECORDTHRESHOLDWRAPPER: {
mergeReceiveRecordThresholdWrapper(other.getReceiveRecordThresholdWrapper());
break;
}
case RECEIVERECORDTHRESHOLDFIELD_NOT_SET: {
break;
}
}
switch (other.getReceiverSigRequiredFieldCase()) {
case RECEIVERSIGREQUIRED: {
setReceiverSigRequired(other.getReceiverSigRequired());
break;
}
case RECEIVERSIGREQUIREDWRAPPER: {
mergeReceiverSigRequiredWrapper(other.getReceiverSigRequiredWrapper());
break;
}
case RECEIVERSIGREQUIREDFIELD_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int sendRecordThresholdFieldCase_ = 0;
private java.lang.Object sendRecordThresholdField_;
public SendRecordThresholdFieldCase
getSendRecordThresholdFieldCase() {
return SendRecordThresholdFieldCase.forNumber(
sendRecordThresholdFieldCase_);
}
public Builder clearSendRecordThresholdField() {
sendRecordThresholdFieldCase_ = 0;
sendRecordThresholdField_ = null;
onChanged();
return this;
}
private int receiveRecordThresholdFieldCase_ = 0;
private java.lang.Object receiveRecordThresholdField_;
public ReceiveRecordThresholdFieldCase
getReceiveRecordThresholdFieldCase() {
return ReceiveRecordThresholdFieldCase.forNumber(
receiveRecordThresholdFieldCase_);
}
public Builder clearReceiveRecordThresholdField() {
receiveRecordThresholdFieldCase_ = 0;
receiveRecordThresholdField_ = null;
onChanged();
return this;
}
private int receiverSigRequiredFieldCase_ = 0;
private java.lang.Object receiverSigRequiredField_;
public ReceiverSigRequiredFieldCase
getReceiverSigRequiredFieldCase() {
return ReceiverSigRequiredFieldCase.forNumber(
receiverSigRequiredFieldCase_);
}
public Builder clearReceiverSigRequiredField() {
receiverSigRequiredFieldCase_ = 0;
receiverSigRequiredField_ = null;
onChanged();
return this;
}
private com.hederahashgraph.api.proto.java.AccountID accountIDToUpdate_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.AccountID, com.hederahashgraph.api.proto.java.AccountID.Builder, com.hederahashgraph.api.proto.java.AccountIDOrBuilder> accountIDToUpdateBuilder_;
/**
*
* The account ID which is being updated in this transaction
*
*
* .proto.AccountID accountIDToUpdate = 2;
*/
public boolean hasAccountIDToUpdate() {
return accountIDToUpdateBuilder_ != null || accountIDToUpdate_ != null;
}
/**
*
* The account ID which is being updated in this transaction
*
*
* .proto.AccountID accountIDToUpdate = 2;
*/
public com.hederahashgraph.api.proto.java.AccountID getAccountIDToUpdate() {
if (accountIDToUpdateBuilder_ == null) {
return accountIDToUpdate_ == null ? com.hederahashgraph.api.proto.java.AccountID.getDefaultInstance() : accountIDToUpdate_;
} else {
return accountIDToUpdateBuilder_.getMessage();
}
}
/**
*
* The account ID which is being updated in this transaction
*
*
* .proto.AccountID accountIDToUpdate = 2;
*/
public Builder setAccountIDToUpdate(com.hederahashgraph.api.proto.java.AccountID value) {
if (accountIDToUpdateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
accountIDToUpdate_ = value;
onChanged();
} else {
accountIDToUpdateBuilder_.setMessage(value);
}
return this;
}
/**
*
* The account ID which is being updated in this transaction
*
*
* .proto.AccountID accountIDToUpdate = 2;
*/
public Builder setAccountIDToUpdate(
com.hederahashgraph.api.proto.java.AccountID.Builder builderForValue) {
if (accountIDToUpdateBuilder_ == null) {
accountIDToUpdate_ = builderForValue.build();
onChanged();
} else {
accountIDToUpdateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The account ID which is being updated in this transaction
*
*
* .proto.AccountID accountIDToUpdate = 2;
*/
public Builder mergeAccountIDToUpdate(com.hederahashgraph.api.proto.java.AccountID value) {
if (accountIDToUpdateBuilder_ == null) {
if (accountIDToUpdate_ != null) {
accountIDToUpdate_ =
com.hederahashgraph.api.proto.java.AccountID.newBuilder(accountIDToUpdate_).mergeFrom(value).buildPartial();
} else {
accountIDToUpdate_ = value;
}
onChanged();
} else {
accountIDToUpdateBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The account ID which is being updated in this transaction
*
*
* .proto.AccountID accountIDToUpdate = 2;
*/
public Builder clearAccountIDToUpdate() {
if (accountIDToUpdateBuilder_ == null) {
accountIDToUpdate_ = null;
onChanged();
} else {
accountIDToUpdate_ = null;
accountIDToUpdateBuilder_ = null;
}
return this;
}
/**
*
* The account ID which is being updated in this transaction
*
*
* .proto.AccountID accountIDToUpdate = 2;
*/
public com.hederahashgraph.api.proto.java.AccountID.Builder getAccountIDToUpdateBuilder() {
onChanged();
return getAccountIDToUpdateFieldBuilder().getBuilder();
}
/**
*
* The account ID which is being updated in this transaction
*
*
* .proto.AccountID accountIDToUpdate = 2;
*/
public com.hederahashgraph.api.proto.java.AccountIDOrBuilder getAccountIDToUpdateOrBuilder() {
if (accountIDToUpdateBuilder_ != null) {
return accountIDToUpdateBuilder_.getMessageOrBuilder();
} else {
return accountIDToUpdate_ == null ?
com.hederahashgraph.api.proto.java.AccountID.getDefaultInstance() : accountIDToUpdate_;
}
}
/**
*
* The account ID which is being updated in this transaction
*
*
* .proto.AccountID accountIDToUpdate = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.AccountID, com.hederahashgraph.api.proto.java.AccountID.Builder, com.hederahashgraph.api.proto.java.AccountIDOrBuilder>
getAccountIDToUpdateFieldBuilder() {
if (accountIDToUpdateBuilder_ == null) {
accountIDToUpdateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.AccountID, com.hederahashgraph.api.proto.java.AccountID.Builder, com.hederahashgraph.api.proto.java.AccountIDOrBuilder>(
getAccountIDToUpdate(),
getParentForChildren(),
isClean());
accountIDToUpdate_ = null;
}
return accountIDToUpdateBuilder_;
}
private com.hederahashgraph.api.proto.java.Key key_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder> keyBuilder_;
/**
*
* The new key
*
*
* .proto.Key key = 3;
*/
public boolean hasKey() {
return keyBuilder_ != null || key_ != null;
}
/**
*
* The new key
*
*
* .proto.Key key = 3;
*/
public com.hederahashgraph.api.proto.java.Key getKey() {
if (keyBuilder_ == null) {
return key_ == null ? com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : key_;
} else {
return keyBuilder_.getMessage();
}
}
/**
*
* The new key
*
*
* .proto.Key key = 3;
*/
public Builder setKey(com.hederahashgraph.api.proto.java.Key value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
} else {
keyBuilder_.setMessage(value);
}
return this;
}
/**
*
* The new key
*
*
* .proto.Key key = 3;
*/
public Builder setKey(
com.hederahashgraph.api.proto.java.Key.Builder builderForValue) {
if (keyBuilder_ == null) {
key_ = builderForValue.build();
onChanged();
} else {
keyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The new key
*
*
* .proto.Key key = 3;
*/
public Builder mergeKey(com.hederahashgraph.api.proto.java.Key value) {
if (keyBuilder_ == null) {
if (key_ != null) {
key_ =
com.hederahashgraph.api.proto.java.Key.newBuilder(key_).mergeFrom(value).buildPartial();
} else {
key_ = value;
}
onChanged();
} else {
keyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The new key
*
*
* .proto.Key key = 3;
*/
public Builder clearKey() {
if (keyBuilder_ == null) {
key_ = null;
onChanged();
} else {
key_ = null;
keyBuilder_ = null;
}
return this;
}
/**
*
* The new key
*
*
* .proto.Key key = 3;
*/
public com.hederahashgraph.api.proto.java.Key.Builder getKeyBuilder() {
onChanged();
return getKeyFieldBuilder().getBuilder();
}
/**
*
* The new key
*
*
* .proto.Key key = 3;
*/
public com.hederahashgraph.api.proto.java.KeyOrBuilder getKeyOrBuilder() {
if (keyBuilder_ != null) {
return keyBuilder_.getMessageOrBuilder();
} else {
return key_ == null ?
com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : key_;
}
}
/**
*
* The new key
*
*
* .proto.Key key = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder>
getKeyFieldBuilder() {
if (keyBuilder_ == null) {
keyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder>(
getKey(),
getParentForChildren(),
isClean());
key_ = null;
}
return keyBuilder_;
}
private com.hederahashgraph.api.proto.java.AccountID proxyAccountID_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.AccountID, com.hederahashgraph.api.proto.java.AccountID.Builder, com.hederahashgraph.api.proto.java.AccountIDOrBuilder> proxyAccountIDBuilder_;
/**
*
* ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an invalid account, or is an account that isn't a node, then this account is automatically proxy staked to a node chosen by the network, but without earning payments. If the proxyAccountID account refuses to accept proxy staking , or if it is not currently running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 4;
*/
public boolean hasProxyAccountID() {
return proxyAccountIDBuilder_ != null || proxyAccountID_ != null;
}
/**
*
* ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an invalid account, or is an account that isn't a node, then this account is automatically proxy staked to a node chosen by the network, but without earning payments. If the proxyAccountID account refuses to accept proxy staking , or if it is not currently running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 4;
*/
public com.hederahashgraph.api.proto.java.AccountID getProxyAccountID() {
if (proxyAccountIDBuilder_ == null) {
return proxyAccountID_ == null ? com.hederahashgraph.api.proto.java.AccountID.getDefaultInstance() : proxyAccountID_;
} else {
return proxyAccountIDBuilder_.getMessage();
}
}
/**
*
* ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an invalid account, or is an account that isn't a node, then this account is automatically proxy staked to a node chosen by the network, but without earning payments. If the proxyAccountID account refuses to accept proxy staking , or if it is not currently running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 4;
*/
public Builder setProxyAccountID(com.hederahashgraph.api.proto.java.AccountID value) {
if (proxyAccountIDBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
proxyAccountID_ = value;
onChanged();
} else {
proxyAccountIDBuilder_.setMessage(value);
}
return this;
}
/**
*
* ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an invalid account, or is an account that isn't a node, then this account is automatically proxy staked to a node chosen by the network, but without earning payments. If the proxyAccountID account refuses to accept proxy staking , or if it is not currently running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 4;
*/
public Builder setProxyAccountID(
com.hederahashgraph.api.proto.java.AccountID.Builder builderForValue) {
if (proxyAccountIDBuilder_ == null) {
proxyAccountID_ = builderForValue.build();
onChanged();
} else {
proxyAccountIDBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an invalid account, or is an account that isn't a node, then this account is automatically proxy staked to a node chosen by the network, but without earning payments. If the proxyAccountID account refuses to accept proxy staking , or if it is not currently running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 4;
*/
public Builder mergeProxyAccountID(com.hederahashgraph.api.proto.java.AccountID value) {
if (proxyAccountIDBuilder_ == null) {
if (proxyAccountID_ != null) {
proxyAccountID_ =
com.hederahashgraph.api.proto.java.AccountID.newBuilder(proxyAccountID_).mergeFrom(value).buildPartial();
} else {
proxyAccountID_ = value;
}
onChanged();
} else {
proxyAccountIDBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an invalid account, or is an account that isn't a node, then this account is automatically proxy staked to a node chosen by the network, but without earning payments. If the proxyAccountID account refuses to accept proxy staking , or if it is not currently running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 4;
*/
public Builder clearProxyAccountID() {
if (proxyAccountIDBuilder_ == null) {
proxyAccountID_ = null;
onChanged();
} else {
proxyAccountID_ = null;
proxyAccountIDBuilder_ = null;
}
return this;
}
/**
*
* ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an invalid account, or is an account that isn't a node, then this account is automatically proxy staked to a node chosen by the network, but without earning payments. If the proxyAccountID account refuses to accept proxy staking , or if it is not currently running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 4;
*/
public com.hederahashgraph.api.proto.java.AccountID.Builder getProxyAccountIDBuilder() {
onChanged();
return getProxyAccountIDFieldBuilder().getBuilder();
}
/**
*
* ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an invalid account, or is an account that isn't a node, then this account is automatically proxy staked to a node chosen by the network, but without earning payments. If the proxyAccountID account refuses to accept proxy staking , or if it is not currently running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 4;
*/
public com.hederahashgraph.api.proto.java.AccountIDOrBuilder getProxyAccountIDOrBuilder() {
if (proxyAccountIDBuilder_ != null) {
return proxyAccountIDBuilder_.getMessageOrBuilder();
} else {
return proxyAccountID_ == null ?
com.hederahashgraph.api.proto.java.AccountID.getDefaultInstance() : proxyAccountID_;
}
}
/**
*
* ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an invalid account, or is an account that isn't a node, then this account is automatically proxy staked to a node chosen by the network, but without earning payments. If the proxyAccountID account refuses to accept proxy staking , or if it is not currently running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.AccountID, com.hederahashgraph.api.proto.java.AccountID.Builder, com.hederahashgraph.api.proto.java.AccountIDOrBuilder>
getProxyAccountIDFieldBuilder() {
if (proxyAccountIDBuilder_ == null) {
proxyAccountIDBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.AccountID, com.hederahashgraph.api.proto.java.AccountID.Builder, com.hederahashgraph.api.proto.java.AccountIDOrBuilder>(
getProxyAccountID(),
getParentForChildren(),
isClean());
proxyAccountID_ = null;
}
return proxyAccountIDBuilder_;
}
private int proxyFraction_ ;
/**
*
* [Deprecated]. Payments earned from proxy staking are shared between the node and this account, with proxyFraction / 10000 going to this account
*
*
* int32 proxyFraction = 5 [deprecated = true];
*/
@java.lang.Deprecated public int getProxyFraction() {
return proxyFraction_;
}
/**
*
* [Deprecated]. Payments earned from proxy staking are shared between the node and this account, with proxyFraction / 10000 going to this account
*
*
* int32 proxyFraction = 5 [deprecated = true];
*/
@java.lang.Deprecated public Builder setProxyFraction(int value) {
proxyFraction_ = value;
onChanged();
return this;
}
/**
*
* [Deprecated]. Payments earned from proxy staking are shared between the node and this account, with proxyFraction / 10000 going to this account
*
*
* int32 proxyFraction = 5 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearProxyFraction() {
proxyFraction_ = 0;
onChanged();
return this;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* uint64 sendRecordThreshold = 6 [deprecated = true];
*/
@java.lang.Deprecated public long getSendRecordThreshold() {
if (sendRecordThresholdFieldCase_ == 6) {
return (java.lang.Long) sendRecordThresholdField_;
}
return 0L;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* uint64 sendRecordThreshold = 6 [deprecated = true];
*/
@java.lang.Deprecated public Builder setSendRecordThreshold(long value) {
sendRecordThresholdFieldCase_ = 6;
sendRecordThresholdField_ = value;
onChanged();
return this;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* uint64 sendRecordThreshold = 6 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearSendRecordThreshold() {
if (sendRecordThresholdFieldCase_ == 6) {
sendRecordThresholdFieldCase_ = 0;
sendRecordThresholdField_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt64Value, com.google.protobuf.UInt64Value.Builder, com.google.protobuf.UInt64ValueOrBuilder> sendRecordThresholdWrapperBuilder_;
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* .google.protobuf.UInt64Value sendRecordThresholdWrapper = 11 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasSendRecordThresholdWrapper() {
return sendRecordThresholdFieldCase_ == 11;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* .google.protobuf.UInt64Value sendRecordThresholdWrapper = 11 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.UInt64Value getSendRecordThresholdWrapper() {
if (sendRecordThresholdWrapperBuilder_ == null) {
if (sendRecordThresholdFieldCase_ == 11) {
return (com.google.protobuf.UInt64Value) sendRecordThresholdField_;
}
return com.google.protobuf.UInt64Value.getDefaultInstance();
} else {
if (sendRecordThresholdFieldCase_ == 11) {
return sendRecordThresholdWrapperBuilder_.getMessage();
}
return com.google.protobuf.UInt64Value.getDefaultInstance();
}
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* .google.protobuf.UInt64Value sendRecordThresholdWrapper = 11 [deprecated = true];
*/
@java.lang.Deprecated public Builder setSendRecordThresholdWrapper(com.google.protobuf.UInt64Value value) {
if (sendRecordThresholdWrapperBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
sendRecordThresholdField_ = value;
onChanged();
} else {
sendRecordThresholdWrapperBuilder_.setMessage(value);
}
sendRecordThresholdFieldCase_ = 11;
return this;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* .google.protobuf.UInt64Value sendRecordThresholdWrapper = 11 [deprecated = true];
*/
@java.lang.Deprecated public Builder setSendRecordThresholdWrapper(
com.google.protobuf.UInt64Value.Builder builderForValue) {
if (sendRecordThresholdWrapperBuilder_ == null) {
sendRecordThresholdField_ = builderForValue.build();
onChanged();
} else {
sendRecordThresholdWrapperBuilder_.setMessage(builderForValue.build());
}
sendRecordThresholdFieldCase_ = 11;
return this;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* .google.protobuf.UInt64Value sendRecordThresholdWrapper = 11 [deprecated = true];
*/
@java.lang.Deprecated public Builder mergeSendRecordThresholdWrapper(com.google.protobuf.UInt64Value value) {
if (sendRecordThresholdWrapperBuilder_ == null) {
if (sendRecordThresholdFieldCase_ == 11 &&
sendRecordThresholdField_ != com.google.protobuf.UInt64Value.getDefaultInstance()) {
sendRecordThresholdField_ = com.google.protobuf.UInt64Value.newBuilder((com.google.protobuf.UInt64Value) sendRecordThresholdField_)
.mergeFrom(value).buildPartial();
} else {
sendRecordThresholdField_ = value;
}
onChanged();
} else {
if (sendRecordThresholdFieldCase_ == 11) {
sendRecordThresholdWrapperBuilder_.mergeFrom(value);
}
sendRecordThresholdWrapperBuilder_.setMessage(value);
}
sendRecordThresholdFieldCase_ = 11;
return this;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* .google.protobuf.UInt64Value sendRecordThresholdWrapper = 11 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearSendRecordThresholdWrapper() {
if (sendRecordThresholdWrapperBuilder_ == null) {
if (sendRecordThresholdFieldCase_ == 11) {
sendRecordThresholdFieldCase_ = 0;
sendRecordThresholdField_ = null;
onChanged();
}
} else {
if (sendRecordThresholdFieldCase_ == 11) {
sendRecordThresholdFieldCase_ = 0;
sendRecordThresholdField_ = null;
}
sendRecordThresholdWrapperBuilder_.clear();
}
return this;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* .google.protobuf.UInt64Value sendRecordThresholdWrapper = 11 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.UInt64Value.Builder getSendRecordThresholdWrapperBuilder() {
return getSendRecordThresholdWrapperFieldBuilder().getBuilder();
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* .google.protobuf.UInt64Value sendRecordThresholdWrapper = 11 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.UInt64ValueOrBuilder getSendRecordThresholdWrapperOrBuilder() {
if ((sendRecordThresholdFieldCase_ == 11) && (sendRecordThresholdWrapperBuilder_ != null)) {
return sendRecordThresholdWrapperBuilder_.getMessageOrBuilder();
} else {
if (sendRecordThresholdFieldCase_ == 11) {
return (com.google.protobuf.UInt64Value) sendRecordThresholdField_;
}
return com.google.protobuf.UInt64Value.getDefaultInstance();
}
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any send/withdraw transaction
*
*
* .google.protobuf.UInt64Value sendRecordThresholdWrapper = 11 [deprecated = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt64Value, com.google.protobuf.UInt64Value.Builder, com.google.protobuf.UInt64ValueOrBuilder>
getSendRecordThresholdWrapperFieldBuilder() {
if (sendRecordThresholdWrapperBuilder_ == null) {
if (!(sendRecordThresholdFieldCase_ == 11)) {
sendRecordThresholdField_ = com.google.protobuf.UInt64Value.getDefaultInstance();
}
sendRecordThresholdWrapperBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt64Value, com.google.protobuf.UInt64Value.Builder, com.google.protobuf.UInt64ValueOrBuilder>(
(com.google.protobuf.UInt64Value) sendRecordThresholdField_,
getParentForChildren(),
isClean());
sendRecordThresholdField_ = null;
}
sendRecordThresholdFieldCase_ = 11;
onChanged();;
return sendRecordThresholdWrapperBuilder_;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* uint64 receiveRecordThreshold = 7 [deprecated = true];
*/
@java.lang.Deprecated public long getReceiveRecordThreshold() {
if (receiveRecordThresholdFieldCase_ == 7) {
return (java.lang.Long) receiveRecordThresholdField_;
}
return 0L;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* uint64 receiveRecordThreshold = 7 [deprecated = true];
*/
@java.lang.Deprecated public Builder setReceiveRecordThreshold(long value) {
receiveRecordThresholdFieldCase_ = 7;
receiveRecordThresholdField_ = value;
onChanged();
return this;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* uint64 receiveRecordThreshold = 7 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearReceiveRecordThreshold() {
if (receiveRecordThresholdFieldCase_ == 7) {
receiveRecordThresholdFieldCase_ = 0;
receiveRecordThresholdField_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt64Value, com.google.protobuf.UInt64Value.Builder, com.google.protobuf.UInt64ValueOrBuilder> receiveRecordThresholdWrapperBuilder_;
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* .google.protobuf.UInt64Value receiveRecordThresholdWrapper = 12 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasReceiveRecordThresholdWrapper() {
return receiveRecordThresholdFieldCase_ == 12;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* .google.protobuf.UInt64Value receiveRecordThresholdWrapper = 12 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.UInt64Value getReceiveRecordThresholdWrapper() {
if (receiveRecordThresholdWrapperBuilder_ == null) {
if (receiveRecordThresholdFieldCase_ == 12) {
return (com.google.protobuf.UInt64Value) receiveRecordThresholdField_;
}
return com.google.protobuf.UInt64Value.getDefaultInstance();
} else {
if (receiveRecordThresholdFieldCase_ == 12) {
return receiveRecordThresholdWrapperBuilder_.getMessage();
}
return com.google.protobuf.UInt64Value.getDefaultInstance();
}
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* .google.protobuf.UInt64Value receiveRecordThresholdWrapper = 12 [deprecated = true];
*/
@java.lang.Deprecated public Builder setReceiveRecordThresholdWrapper(com.google.protobuf.UInt64Value value) {
if (receiveRecordThresholdWrapperBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
receiveRecordThresholdField_ = value;
onChanged();
} else {
receiveRecordThresholdWrapperBuilder_.setMessage(value);
}
receiveRecordThresholdFieldCase_ = 12;
return this;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* .google.protobuf.UInt64Value receiveRecordThresholdWrapper = 12 [deprecated = true];
*/
@java.lang.Deprecated public Builder setReceiveRecordThresholdWrapper(
com.google.protobuf.UInt64Value.Builder builderForValue) {
if (receiveRecordThresholdWrapperBuilder_ == null) {
receiveRecordThresholdField_ = builderForValue.build();
onChanged();
} else {
receiveRecordThresholdWrapperBuilder_.setMessage(builderForValue.build());
}
receiveRecordThresholdFieldCase_ = 12;
return this;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* .google.protobuf.UInt64Value receiveRecordThresholdWrapper = 12 [deprecated = true];
*/
@java.lang.Deprecated public Builder mergeReceiveRecordThresholdWrapper(com.google.protobuf.UInt64Value value) {
if (receiveRecordThresholdWrapperBuilder_ == null) {
if (receiveRecordThresholdFieldCase_ == 12 &&
receiveRecordThresholdField_ != com.google.protobuf.UInt64Value.getDefaultInstance()) {
receiveRecordThresholdField_ = com.google.protobuf.UInt64Value.newBuilder((com.google.protobuf.UInt64Value) receiveRecordThresholdField_)
.mergeFrom(value).buildPartial();
} else {
receiveRecordThresholdField_ = value;
}
onChanged();
} else {
if (receiveRecordThresholdFieldCase_ == 12) {
receiveRecordThresholdWrapperBuilder_.mergeFrom(value);
}
receiveRecordThresholdWrapperBuilder_.setMessage(value);
}
receiveRecordThresholdFieldCase_ = 12;
return this;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* .google.protobuf.UInt64Value receiveRecordThresholdWrapper = 12 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearReceiveRecordThresholdWrapper() {
if (receiveRecordThresholdWrapperBuilder_ == null) {
if (receiveRecordThresholdFieldCase_ == 12) {
receiveRecordThresholdFieldCase_ = 0;
receiveRecordThresholdField_ = null;
onChanged();
}
} else {
if (receiveRecordThresholdFieldCase_ == 12) {
receiveRecordThresholdFieldCase_ = 0;
receiveRecordThresholdField_ = null;
}
receiveRecordThresholdWrapperBuilder_.clear();
}
return this;
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* .google.protobuf.UInt64Value receiveRecordThresholdWrapper = 12 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.UInt64Value.Builder getReceiveRecordThresholdWrapperBuilder() {
return getReceiveRecordThresholdWrapperFieldBuilder().getBuilder();
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* .google.protobuf.UInt64Value receiveRecordThresholdWrapper = 12 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.UInt64ValueOrBuilder getReceiveRecordThresholdWrapperOrBuilder() {
if ((receiveRecordThresholdFieldCase_ == 12) && (receiveRecordThresholdWrapperBuilder_ != null)) {
return receiveRecordThresholdWrapperBuilder_.getMessageOrBuilder();
} else {
if (receiveRecordThresholdFieldCase_ == 12) {
return (com.google.protobuf.UInt64Value) receiveRecordThresholdField_;
}
return com.google.protobuf.UInt64Value.getDefaultInstance();
}
}
/**
*
* [Deprecated]. The new threshold amount (in tinybars) for which an account record is created for any receive/deposit transaction.
*
*
* .google.protobuf.UInt64Value receiveRecordThresholdWrapper = 12 [deprecated = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt64Value, com.google.protobuf.UInt64Value.Builder, com.google.protobuf.UInt64ValueOrBuilder>
getReceiveRecordThresholdWrapperFieldBuilder() {
if (receiveRecordThresholdWrapperBuilder_ == null) {
if (!(receiveRecordThresholdFieldCase_ == 12)) {
receiveRecordThresholdField_ = com.google.protobuf.UInt64Value.getDefaultInstance();
}
receiveRecordThresholdWrapperBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt64Value, com.google.protobuf.UInt64Value.Builder, com.google.protobuf.UInt64ValueOrBuilder>(
(com.google.protobuf.UInt64Value) receiveRecordThresholdField_,
getParentForChildren(),
isClean());
receiveRecordThresholdField_ = null;
}
receiveRecordThresholdFieldCase_ = 12;
onChanged();;
return receiveRecordThresholdWrapperBuilder_;
}
private com.hederahashgraph.api.proto.java.Duration autoRenewPeriod_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Duration, com.hederahashgraph.api.proto.java.Duration.Builder, com.hederahashgraph.api.proto.java.DurationOrBuilder> autoRenewPeriodBuilder_;
/**
*
* The duration in which it will automatically extend the expiration period. If it doesn't have enough balance, it extends as long as possible. If it is empty when it expires, then it is deleted.
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
public boolean hasAutoRenewPeriod() {
return autoRenewPeriodBuilder_ != null || autoRenewPeriod_ != null;
}
/**
*
* The duration in which it will automatically extend the expiration period. If it doesn't have enough balance, it extends as long as possible. If it is empty when it expires, then it is deleted.
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
public com.hederahashgraph.api.proto.java.Duration getAutoRenewPeriod() {
if (autoRenewPeriodBuilder_ == null) {
return autoRenewPeriod_ == null ? com.hederahashgraph.api.proto.java.Duration.getDefaultInstance() : autoRenewPeriod_;
} else {
return autoRenewPeriodBuilder_.getMessage();
}
}
/**
*
* The duration in which it will automatically extend the expiration period. If it doesn't have enough balance, it extends as long as possible. If it is empty when it expires, then it is deleted.
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
public Builder setAutoRenewPeriod(com.hederahashgraph.api.proto.java.Duration value) {
if (autoRenewPeriodBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
autoRenewPeriod_ = value;
onChanged();
} else {
autoRenewPeriodBuilder_.setMessage(value);
}
return this;
}
/**
*
* The duration in which it will automatically extend the expiration period. If it doesn't have enough balance, it extends as long as possible. If it is empty when it expires, then it is deleted.
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
public Builder setAutoRenewPeriod(
com.hederahashgraph.api.proto.java.Duration.Builder builderForValue) {
if (autoRenewPeriodBuilder_ == null) {
autoRenewPeriod_ = builderForValue.build();
onChanged();
} else {
autoRenewPeriodBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The duration in which it will automatically extend the expiration period. If it doesn't have enough balance, it extends as long as possible. If it is empty when it expires, then it is deleted.
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
public Builder mergeAutoRenewPeriod(com.hederahashgraph.api.proto.java.Duration value) {
if (autoRenewPeriodBuilder_ == null) {
if (autoRenewPeriod_ != null) {
autoRenewPeriod_ =
com.hederahashgraph.api.proto.java.Duration.newBuilder(autoRenewPeriod_).mergeFrom(value).buildPartial();
} else {
autoRenewPeriod_ = value;
}
onChanged();
} else {
autoRenewPeriodBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The duration in which it will automatically extend the expiration period. If it doesn't have enough balance, it extends as long as possible. If it is empty when it expires, then it is deleted.
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
public Builder clearAutoRenewPeriod() {
if (autoRenewPeriodBuilder_ == null) {
autoRenewPeriod_ = null;
onChanged();
} else {
autoRenewPeriod_ = null;
autoRenewPeriodBuilder_ = null;
}
return this;
}
/**
*
* The duration in which it will automatically extend the expiration period. If it doesn't have enough balance, it extends as long as possible. If it is empty when it expires, then it is deleted.
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
public com.hederahashgraph.api.proto.java.Duration.Builder getAutoRenewPeriodBuilder() {
onChanged();
return getAutoRenewPeriodFieldBuilder().getBuilder();
}
/**
*
* The duration in which it will automatically extend the expiration period. If it doesn't have enough balance, it extends as long as possible. If it is empty when it expires, then it is deleted.
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
public com.hederahashgraph.api.proto.java.DurationOrBuilder getAutoRenewPeriodOrBuilder() {
if (autoRenewPeriodBuilder_ != null) {
return autoRenewPeriodBuilder_.getMessageOrBuilder();
} else {
return autoRenewPeriod_ == null ?
com.hederahashgraph.api.proto.java.Duration.getDefaultInstance() : autoRenewPeriod_;
}
}
/**
*
* The duration in which it will automatically extend the expiration period. If it doesn't have enough balance, it extends as long as possible. If it is empty when it expires, then it is deleted.
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Duration, com.hederahashgraph.api.proto.java.Duration.Builder, com.hederahashgraph.api.proto.java.DurationOrBuilder>
getAutoRenewPeriodFieldBuilder() {
if (autoRenewPeriodBuilder_ == null) {
autoRenewPeriodBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Duration, com.hederahashgraph.api.proto.java.Duration.Builder, com.hederahashgraph.api.proto.java.DurationOrBuilder>(
getAutoRenewPeriod(),
getParentForChildren(),
isClean());
autoRenewPeriod_ = null;
}
return autoRenewPeriodBuilder_;
}
private com.hederahashgraph.api.proto.java.Timestamp expirationTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Timestamp, com.hederahashgraph.api.proto.java.Timestamp.Builder, com.hederahashgraph.api.proto.java.TimestampOrBuilder> expirationTimeBuilder_;
/**
*
* The new expiration time to extend to (ignored if equal to or before the current one)
*
*
* .proto.Timestamp expirationTime = 9;
*/
public boolean hasExpirationTime() {
return expirationTimeBuilder_ != null || expirationTime_ != null;
}
/**
*
* The new expiration time to extend to (ignored if equal to or before the current one)
*
*
* .proto.Timestamp expirationTime = 9;
*/
public com.hederahashgraph.api.proto.java.Timestamp getExpirationTime() {
if (expirationTimeBuilder_ == null) {
return expirationTime_ == null ? com.hederahashgraph.api.proto.java.Timestamp.getDefaultInstance() : expirationTime_;
} else {
return expirationTimeBuilder_.getMessage();
}
}
/**
*
* The new expiration time to extend to (ignored if equal to or before the current one)
*
*
* .proto.Timestamp expirationTime = 9;
*/
public Builder setExpirationTime(com.hederahashgraph.api.proto.java.Timestamp value) {
if (expirationTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
expirationTime_ = value;
onChanged();
} else {
expirationTimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* The new expiration time to extend to (ignored if equal to or before the current one)
*
*
* .proto.Timestamp expirationTime = 9;
*/
public Builder setExpirationTime(
com.hederahashgraph.api.proto.java.Timestamp.Builder builderForValue) {
if (expirationTimeBuilder_ == null) {
expirationTime_ = builderForValue.build();
onChanged();
} else {
expirationTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The new expiration time to extend to (ignored if equal to or before the current one)
*
*
* .proto.Timestamp expirationTime = 9;
*/
public Builder mergeExpirationTime(com.hederahashgraph.api.proto.java.Timestamp value) {
if (expirationTimeBuilder_ == null) {
if (expirationTime_ != null) {
expirationTime_ =
com.hederahashgraph.api.proto.java.Timestamp.newBuilder(expirationTime_).mergeFrom(value).buildPartial();
} else {
expirationTime_ = value;
}
onChanged();
} else {
expirationTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The new expiration time to extend to (ignored if equal to or before the current one)
*
*
* .proto.Timestamp expirationTime = 9;
*/
public Builder clearExpirationTime() {
if (expirationTimeBuilder_ == null) {
expirationTime_ = null;
onChanged();
} else {
expirationTime_ = null;
expirationTimeBuilder_ = null;
}
return this;
}
/**
*
* The new expiration time to extend to (ignored if equal to or before the current one)
*
*
* .proto.Timestamp expirationTime = 9;
*/
public com.hederahashgraph.api.proto.java.Timestamp.Builder getExpirationTimeBuilder() {
onChanged();
return getExpirationTimeFieldBuilder().getBuilder();
}
/**
*
* The new expiration time to extend to (ignored if equal to or before the current one)
*
*
* .proto.Timestamp expirationTime = 9;
*/
public com.hederahashgraph.api.proto.java.TimestampOrBuilder getExpirationTimeOrBuilder() {
if (expirationTimeBuilder_ != null) {
return expirationTimeBuilder_.getMessageOrBuilder();
} else {
return expirationTime_ == null ?
com.hederahashgraph.api.proto.java.Timestamp.getDefaultInstance() : expirationTime_;
}
}
/**
*
* The new expiration time to extend to (ignored if equal to or before the current one)
*
*
* .proto.Timestamp expirationTime = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Timestamp, com.hederahashgraph.api.proto.java.Timestamp.Builder, com.hederahashgraph.api.proto.java.TimestampOrBuilder>
getExpirationTimeFieldBuilder() {
if (expirationTimeBuilder_ == null) {
expirationTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Timestamp, com.hederahashgraph.api.proto.java.Timestamp.Builder, com.hederahashgraph.api.proto.java.TimestampOrBuilder>(
getExpirationTime(),
getParentForChildren(),
isClean());
expirationTime_ = null;
}
return expirationTimeBuilder_;
}
/**
*
* [Deprecated] Do NOT use this field to set a false value because the server cannot distinguish from the default value. Use receiverSigRequiredWrapper field for this purpose.
*
*
* bool receiverSigRequired = 10 [deprecated = true];
*/
@java.lang.Deprecated public boolean getReceiverSigRequired() {
if (receiverSigRequiredFieldCase_ == 10) {
return (java.lang.Boolean) receiverSigRequiredField_;
}
return false;
}
/**
*
* [Deprecated] Do NOT use this field to set a false value because the server cannot distinguish from the default value. Use receiverSigRequiredWrapper field for this purpose.
*
*
* bool receiverSigRequired = 10 [deprecated = true];
*/
@java.lang.Deprecated public Builder setReceiverSigRequired(boolean value) {
receiverSigRequiredFieldCase_ = 10;
receiverSigRequiredField_ = value;
onChanged();
return this;
}
/**
*
* [Deprecated] Do NOT use this field to set a false value because the server cannot distinguish from the default value. Use receiverSigRequiredWrapper field for this purpose.
*
*
* bool receiverSigRequired = 10 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearReceiverSigRequired() {
if (receiverSigRequiredFieldCase_ == 10) {
receiverSigRequiredFieldCase_ = 0;
receiverSigRequiredField_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder> receiverSigRequiredWrapperBuilder_;
/**
*
* If true, this account's key must sign any transaction depositing into this account (in addition to all withdrawals)
*
*
* .google.protobuf.BoolValue receiverSigRequiredWrapper = 13;
*/
public boolean hasReceiverSigRequiredWrapper() {
return receiverSigRequiredFieldCase_ == 13;
}
/**
*
* If true, this account's key must sign any transaction depositing into this account (in addition to all withdrawals)
*
*
* .google.protobuf.BoolValue receiverSigRequiredWrapper = 13;
*/
public com.google.protobuf.BoolValue getReceiverSigRequiredWrapper() {
if (receiverSigRequiredWrapperBuilder_ == null) {
if (receiverSigRequiredFieldCase_ == 13) {
return (com.google.protobuf.BoolValue) receiverSigRequiredField_;
}
return com.google.protobuf.BoolValue.getDefaultInstance();
} else {
if (receiverSigRequiredFieldCase_ == 13) {
return receiverSigRequiredWrapperBuilder_.getMessage();
}
return com.google.protobuf.BoolValue.getDefaultInstance();
}
}
/**
*
* If true, this account's key must sign any transaction depositing into this account (in addition to all withdrawals)
*
*
* .google.protobuf.BoolValue receiverSigRequiredWrapper = 13;
*/
public Builder setReceiverSigRequiredWrapper(com.google.protobuf.BoolValue value) {
if (receiverSigRequiredWrapperBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
receiverSigRequiredField_ = value;
onChanged();
} else {
receiverSigRequiredWrapperBuilder_.setMessage(value);
}
receiverSigRequiredFieldCase_ = 13;
return this;
}
/**
*
* If true, this account's key must sign any transaction depositing into this account (in addition to all withdrawals)
*
*
* .google.protobuf.BoolValue receiverSigRequiredWrapper = 13;
*/
public Builder setReceiverSigRequiredWrapper(
com.google.protobuf.BoolValue.Builder builderForValue) {
if (receiverSigRequiredWrapperBuilder_ == null) {
receiverSigRequiredField_ = builderForValue.build();
onChanged();
} else {
receiverSigRequiredWrapperBuilder_.setMessage(builderForValue.build());
}
receiverSigRequiredFieldCase_ = 13;
return this;
}
/**
*
* If true, this account's key must sign any transaction depositing into this account (in addition to all withdrawals)
*
*
* .google.protobuf.BoolValue receiverSigRequiredWrapper = 13;
*/
public Builder mergeReceiverSigRequiredWrapper(com.google.protobuf.BoolValue value) {
if (receiverSigRequiredWrapperBuilder_ == null) {
if (receiverSigRequiredFieldCase_ == 13 &&
receiverSigRequiredField_ != com.google.protobuf.BoolValue.getDefaultInstance()) {
receiverSigRequiredField_ = com.google.protobuf.BoolValue.newBuilder((com.google.protobuf.BoolValue) receiverSigRequiredField_)
.mergeFrom(value).buildPartial();
} else {
receiverSigRequiredField_ = value;
}
onChanged();
} else {
if (receiverSigRequiredFieldCase_ == 13) {
receiverSigRequiredWrapperBuilder_.mergeFrom(value);
}
receiverSigRequiredWrapperBuilder_.setMessage(value);
}
receiverSigRequiredFieldCase_ = 13;
return this;
}
/**
*
* If true, this account's key must sign any transaction depositing into this account (in addition to all withdrawals)
*
*
* .google.protobuf.BoolValue receiverSigRequiredWrapper = 13;
*/
public Builder clearReceiverSigRequiredWrapper() {
if (receiverSigRequiredWrapperBuilder_ == null) {
if (receiverSigRequiredFieldCase_ == 13) {
receiverSigRequiredFieldCase_ = 0;
receiverSigRequiredField_ = null;
onChanged();
}
} else {
if (receiverSigRequiredFieldCase_ == 13) {
receiverSigRequiredFieldCase_ = 0;
receiverSigRequiredField_ = null;
}
receiverSigRequiredWrapperBuilder_.clear();
}
return this;
}
/**
*
* If true, this account's key must sign any transaction depositing into this account (in addition to all withdrawals)
*
*
* .google.protobuf.BoolValue receiverSigRequiredWrapper = 13;
*/
public com.google.protobuf.BoolValue.Builder getReceiverSigRequiredWrapperBuilder() {
return getReceiverSigRequiredWrapperFieldBuilder().getBuilder();
}
/**
*
* If true, this account's key must sign any transaction depositing into this account (in addition to all withdrawals)
*
*
* .google.protobuf.BoolValue receiverSigRequiredWrapper = 13;
*/
public com.google.protobuf.BoolValueOrBuilder getReceiverSigRequiredWrapperOrBuilder() {
if ((receiverSigRequiredFieldCase_ == 13) && (receiverSigRequiredWrapperBuilder_ != null)) {
return receiverSigRequiredWrapperBuilder_.getMessageOrBuilder();
} else {
if (receiverSigRequiredFieldCase_ == 13) {
return (com.google.protobuf.BoolValue) receiverSigRequiredField_;
}
return com.google.protobuf.BoolValue.getDefaultInstance();
}
}
/**
*
* If true, this account's key must sign any transaction depositing into this account (in addition to all withdrawals)
*
*
* .google.protobuf.BoolValue receiverSigRequiredWrapper = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>
getReceiverSigRequiredWrapperFieldBuilder() {
if (receiverSigRequiredWrapperBuilder_ == null) {
if (!(receiverSigRequiredFieldCase_ == 13)) {
receiverSigRequiredField_ = com.google.protobuf.BoolValue.getDefaultInstance();
}
receiverSigRequiredWrapperBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>(
(com.google.protobuf.BoolValue) receiverSigRequiredField_,
getParentForChildren(),
isClean());
receiverSigRequiredField_ = null;
}
receiverSigRequiredFieldCase_ = 13;
onChanged();;
return receiverSigRequiredWrapperBuilder_;
}
private com.google.protobuf.StringValue memo_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> memoBuilder_;
/**
*
* If set, the new memo to be associated with the account (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 14;
*/
public boolean hasMemo() {
return memoBuilder_ != null || memo_ != null;
}
/**
*
* If set, the new memo to be associated with the account (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 14;
*/
public com.google.protobuf.StringValue getMemo() {
if (memoBuilder_ == null) {
return memo_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : memo_;
} else {
return memoBuilder_.getMessage();
}
}
/**
*
* If set, the new memo to be associated with the account (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 14;
*/
public Builder setMemo(com.google.protobuf.StringValue value) {
if (memoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
memo_ = value;
onChanged();
} else {
memoBuilder_.setMessage(value);
}
return this;
}
/**
*
* If set, the new memo to be associated with the account (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 14;
*/
public Builder setMemo(
com.google.protobuf.StringValue.Builder builderForValue) {
if (memoBuilder_ == null) {
memo_ = builderForValue.build();
onChanged();
} else {
memoBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* If set, the new memo to be associated with the account (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 14;
*/
public Builder mergeMemo(com.google.protobuf.StringValue value) {
if (memoBuilder_ == null) {
if (memo_ != null) {
memo_ =
com.google.protobuf.StringValue.newBuilder(memo_).mergeFrom(value).buildPartial();
} else {
memo_ = value;
}
onChanged();
} else {
memoBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* If set, the new memo to be associated with the account (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 14;
*/
public Builder clearMemo() {
if (memoBuilder_ == null) {
memo_ = null;
onChanged();
} else {
memo_ = null;
memoBuilder_ = null;
}
return this;
}
/**
*
* If set, the new memo to be associated with the account (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 14;
*/
public com.google.protobuf.StringValue.Builder getMemoBuilder() {
onChanged();
return getMemoFieldBuilder().getBuilder();
}
/**
*
* If set, the new memo to be associated with the account (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 14;
*/
public com.google.protobuf.StringValueOrBuilder getMemoOrBuilder() {
if (memoBuilder_ != null) {
return memoBuilder_.getMessageOrBuilder();
} else {
return memo_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : memo_;
}
}
/**
*
* If set, the new memo to be associated with the account (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 14;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getMemoFieldBuilder() {
if (memoBuilder_ == null) {
memoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getMemo(),
getParentForChildren(),
isClean());
memo_ = null;
}
return memoBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:proto.CryptoUpdateTransactionBody)
}
// @@protoc_insertion_point(class_scope:proto.CryptoUpdateTransactionBody)
private static final com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody();
}
public static com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CryptoUpdateTransactionBody parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CryptoUpdateTransactionBody(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.CryptoUpdateTransactionBody getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy