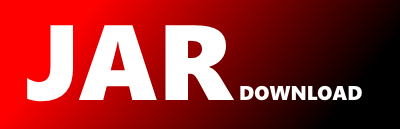
com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: TokenUpdate.proto
package com.hederahashgraph.api.proto.java;
/**
*
* At consensus, updates an already created token to the given values.
*If no value is given for a field, that field is left unchanged. For an immutable tokens (that is, a token without an admin key), only the expiry may be updated. Setting any other field in that case will cause the transaction status to resolve to TOKEN_IS_IMMUTABLE.
*--- Signing Requirements ---
*1. Whether or not a token has an admin key, its expiry can be extended with only the transaction payer's signature.
*2. Updating any other field of a mutable token requires the admin key's signature.
*3. If a new admin key is set, this new key must sign <b>unless</b> it is exactly an empty <tt>KeyList</tt>. This special sentinel key removes the existing admin key and causes the token to become immutable. (Other <tt>Key</tt> structures without a constituent <tt>Ed25519</tt> key will be rejected with <tt>INVALID_ADMIN_KEY</tt>.)
*4. If a new treasury is set, the new treasury account's key must sign the transaction.
*
*
* Protobuf type {@code proto.TokenUpdateTransactionBody}
*/
public final class TokenUpdateTransactionBody extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:proto.TokenUpdateTransactionBody)
TokenUpdateTransactionBodyOrBuilder {
private static final long serialVersionUID = 0L;
// Use TokenUpdateTransactionBody.newBuilder() to construct.
private TokenUpdateTransactionBody(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TokenUpdateTransactionBody() {
symbol_ = "";
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TokenUpdateTransactionBody();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TokenUpdateTransactionBody(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.hederahashgraph.api.proto.java.TokenID.Builder subBuilder = null;
if (token_ != null) {
subBuilder = token_.toBuilder();
}
token_ = input.readMessage(com.hederahashgraph.api.proto.java.TokenID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(token_);
token_ = subBuilder.buildPartial();
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
symbol_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 34: {
com.hederahashgraph.api.proto.java.AccountID.Builder subBuilder = null;
if (treasury_ != null) {
subBuilder = treasury_.toBuilder();
}
treasury_ = input.readMessage(com.hederahashgraph.api.proto.java.AccountID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(treasury_);
treasury_ = subBuilder.buildPartial();
}
break;
}
case 42: {
com.hederahashgraph.api.proto.java.Key.Builder subBuilder = null;
if (adminKey_ != null) {
subBuilder = adminKey_.toBuilder();
}
adminKey_ = input.readMessage(com.hederahashgraph.api.proto.java.Key.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(adminKey_);
adminKey_ = subBuilder.buildPartial();
}
break;
}
case 50: {
com.hederahashgraph.api.proto.java.Key.Builder subBuilder = null;
if (kycKey_ != null) {
subBuilder = kycKey_.toBuilder();
}
kycKey_ = input.readMessage(com.hederahashgraph.api.proto.java.Key.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(kycKey_);
kycKey_ = subBuilder.buildPartial();
}
break;
}
case 58: {
com.hederahashgraph.api.proto.java.Key.Builder subBuilder = null;
if (freezeKey_ != null) {
subBuilder = freezeKey_.toBuilder();
}
freezeKey_ = input.readMessage(com.hederahashgraph.api.proto.java.Key.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(freezeKey_);
freezeKey_ = subBuilder.buildPartial();
}
break;
}
case 66: {
com.hederahashgraph.api.proto.java.Key.Builder subBuilder = null;
if (wipeKey_ != null) {
subBuilder = wipeKey_.toBuilder();
}
wipeKey_ = input.readMessage(com.hederahashgraph.api.proto.java.Key.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(wipeKey_);
wipeKey_ = subBuilder.buildPartial();
}
break;
}
case 74: {
com.hederahashgraph.api.proto.java.Key.Builder subBuilder = null;
if (supplyKey_ != null) {
subBuilder = supplyKey_.toBuilder();
}
supplyKey_ = input.readMessage(com.hederahashgraph.api.proto.java.Key.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(supplyKey_);
supplyKey_ = subBuilder.buildPartial();
}
break;
}
case 82: {
com.hederahashgraph.api.proto.java.AccountID.Builder subBuilder = null;
if (autoRenewAccount_ != null) {
subBuilder = autoRenewAccount_.toBuilder();
}
autoRenewAccount_ = input.readMessage(com.hederahashgraph.api.proto.java.AccountID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(autoRenewAccount_);
autoRenewAccount_ = subBuilder.buildPartial();
}
break;
}
case 90: {
com.hederahashgraph.api.proto.java.Duration.Builder subBuilder = null;
if (autoRenewPeriod_ != null) {
subBuilder = autoRenewPeriod_.toBuilder();
}
autoRenewPeriod_ = input.readMessage(com.hederahashgraph.api.proto.java.Duration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(autoRenewPeriod_);
autoRenewPeriod_ = subBuilder.buildPartial();
}
break;
}
case 98: {
com.hederahashgraph.api.proto.java.Timestamp.Builder subBuilder = null;
if (expiry_ != null) {
subBuilder = expiry_.toBuilder();
}
expiry_ = input.readMessage(com.hederahashgraph.api.proto.java.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(expiry_);
expiry_ = subBuilder.buildPartial();
}
break;
}
case 106: {
com.google.protobuf.StringValue.Builder subBuilder = null;
if (memo_ != null) {
subBuilder = memo_.toBuilder();
}
memo_ = input.readMessage(com.google.protobuf.StringValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(memo_);
memo_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.hederahashgraph.api.proto.java.TokenUpdate.internal_static_proto_TokenUpdateTransactionBody_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.hederahashgraph.api.proto.java.TokenUpdate.internal_static_proto_TokenUpdateTransactionBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody.class, com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody.Builder.class);
}
public static final int TOKEN_FIELD_NUMBER = 1;
private com.hederahashgraph.api.proto.java.TokenID token_;
/**
*
* The Token to be updated
*
*
* .proto.TokenID token = 1;
*/
public boolean hasToken() {
return token_ != null;
}
/**
*
* The Token to be updated
*
*
* .proto.TokenID token = 1;
*/
public com.hederahashgraph.api.proto.java.TokenID getToken() {
return token_ == null ? com.hederahashgraph.api.proto.java.TokenID.getDefaultInstance() : token_;
}
/**
*
* The Token to be updated
*
*
* .proto.TokenID token = 1;
*/
public com.hederahashgraph.api.proto.java.TokenIDOrBuilder getTokenOrBuilder() {
return getToken();
}
public static final int SYMBOL_FIELD_NUMBER = 2;
private volatile java.lang.Object symbol_;
/**
*
* The new publicly visible Token symbol, limited to a UTF-8 encoding of length <tt>tokens.maxTokenNameUtf8Bytes</tt>.
*
*
* string symbol = 2;
*/
public java.lang.String getSymbol() {
java.lang.Object ref = symbol_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
symbol_ = s;
return s;
}
}
/**
*
* The new publicly visible Token symbol, limited to a UTF-8 encoding of length <tt>tokens.maxTokenNameUtf8Bytes</tt>.
*
*
* string symbol = 2;
*/
public com.google.protobuf.ByteString
getSymbolBytes() {
java.lang.Object ref = symbol_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
symbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object name_;
/**
*
* The new publicly visible name of the Token, limited to a UTF-8 encoding of length <tt>tokens.maxSymbolUtf8Bytes</tt>.
*
*
* string name = 3;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* The new publicly visible name of the Token, limited to a UTF-8 encoding of length <tt>tokens.maxSymbolUtf8Bytes</tt>.
*
*
* string name = 3;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TREASURY_FIELD_NUMBER = 4;
private com.hederahashgraph.api.proto.java.AccountID treasury_;
/**
*
* The new Treasury account of the Token. If the provided treasury account is not existing or deleted, the response will be INVALID_TREASURY_ACCOUNT_FOR_TOKEN. If successful, the Token balance held in the previous Treasury Account is transferred to the new one.
*
*
* .proto.AccountID treasury = 4;
*/
public boolean hasTreasury() {
return treasury_ != null;
}
/**
*
* The new Treasury account of the Token. If the provided treasury account is not existing or deleted, the response will be INVALID_TREASURY_ACCOUNT_FOR_TOKEN. If successful, the Token balance held in the previous Treasury Account is transferred to the new one.
*
*
* .proto.AccountID treasury = 4;
*/
public com.hederahashgraph.api.proto.java.AccountID getTreasury() {
return treasury_ == null ? com.hederahashgraph.api.proto.java.AccountID.getDefaultInstance() : treasury_;
}
/**
*
* The new Treasury account of the Token. If the provided treasury account is not existing or deleted, the response will be INVALID_TREASURY_ACCOUNT_FOR_TOKEN. If successful, the Token balance held in the previous Treasury Account is transferred to the new one.
*
*
* .proto.AccountID treasury = 4;
*/
public com.hederahashgraph.api.proto.java.AccountIDOrBuilder getTreasuryOrBuilder() {
return getTreasury();
}
public static final int ADMINKEY_FIELD_NUMBER = 5;
private com.hederahashgraph.api.proto.java.Key adminKey_;
/**
*
* The new admin key of the Token. If Token is immutable, transaction will resolve to TOKEN_IS_IMMUTABlE.
*
*
* .proto.Key adminKey = 5;
*/
public boolean hasAdminKey() {
return adminKey_ != null;
}
/**
*
* The new admin key of the Token. If Token is immutable, transaction will resolve to TOKEN_IS_IMMUTABlE.
*
*
* .proto.Key adminKey = 5;
*/
public com.hederahashgraph.api.proto.java.Key getAdminKey() {
return adminKey_ == null ? com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : adminKey_;
}
/**
*
* The new admin key of the Token. If Token is immutable, transaction will resolve to TOKEN_IS_IMMUTABlE.
*
*
* .proto.Key adminKey = 5;
*/
public com.hederahashgraph.api.proto.java.KeyOrBuilder getAdminKeyOrBuilder() {
return getAdminKey();
}
public static final int KYCKEY_FIELD_NUMBER = 6;
private com.hederahashgraph.api.proto.java.Key kycKey_;
/**
*
* The new KYC key of the Token. If Token does not have currently a KYC key, transaction will resolve to TOKEN_HAS_NO_KYC_KEY.
*
*
* .proto.Key kycKey = 6;
*/
public boolean hasKycKey() {
return kycKey_ != null;
}
/**
*
* The new KYC key of the Token. If Token does not have currently a KYC key, transaction will resolve to TOKEN_HAS_NO_KYC_KEY.
*
*
* .proto.Key kycKey = 6;
*/
public com.hederahashgraph.api.proto.java.Key getKycKey() {
return kycKey_ == null ? com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : kycKey_;
}
/**
*
* The new KYC key of the Token. If Token does not have currently a KYC key, transaction will resolve to TOKEN_HAS_NO_KYC_KEY.
*
*
* .proto.Key kycKey = 6;
*/
public com.hederahashgraph.api.proto.java.KeyOrBuilder getKycKeyOrBuilder() {
return getKycKey();
}
public static final int FREEZEKEY_FIELD_NUMBER = 7;
private com.hederahashgraph.api.proto.java.Key freezeKey_;
/**
*
* The new Freeze key of the Token. If the Token does not have currently a Freeze key, transaction will resolve to TOKEN_HAS_NO_FREEZE_KEY.
*
*
* .proto.Key freezeKey = 7;
*/
public boolean hasFreezeKey() {
return freezeKey_ != null;
}
/**
*
* The new Freeze key of the Token. If the Token does not have currently a Freeze key, transaction will resolve to TOKEN_HAS_NO_FREEZE_KEY.
*
*
* .proto.Key freezeKey = 7;
*/
public com.hederahashgraph.api.proto.java.Key getFreezeKey() {
return freezeKey_ == null ? com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : freezeKey_;
}
/**
*
* The new Freeze key of the Token. If the Token does not have currently a Freeze key, transaction will resolve to TOKEN_HAS_NO_FREEZE_KEY.
*
*
* .proto.Key freezeKey = 7;
*/
public com.hederahashgraph.api.proto.java.KeyOrBuilder getFreezeKeyOrBuilder() {
return getFreezeKey();
}
public static final int WIPEKEY_FIELD_NUMBER = 8;
private com.hederahashgraph.api.proto.java.Key wipeKey_;
/**
*
* The new Wipe key of the Token. If the Token does not have currently a Wipe key, transaction will resolve to TOKEN_HAS_NO_WIPE_KEY.
*
*
* .proto.Key wipeKey = 8;
*/
public boolean hasWipeKey() {
return wipeKey_ != null;
}
/**
*
* The new Wipe key of the Token. If the Token does not have currently a Wipe key, transaction will resolve to TOKEN_HAS_NO_WIPE_KEY.
*
*
* .proto.Key wipeKey = 8;
*/
public com.hederahashgraph.api.proto.java.Key getWipeKey() {
return wipeKey_ == null ? com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : wipeKey_;
}
/**
*
* The new Wipe key of the Token. If the Token does not have currently a Wipe key, transaction will resolve to TOKEN_HAS_NO_WIPE_KEY.
*
*
* .proto.Key wipeKey = 8;
*/
public com.hederahashgraph.api.proto.java.KeyOrBuilder getWipeKeyOrBuilder() {
return getWipeKey();
}
public static final int SUPPLYKEY_FIELD_NUMBER = 9;
private com.hederahashgraph.api.proto.java.Key supplyKey_;
/**
*
* The new Supply key of the Token. If the Token does not have currently a Supply key, transaction will resolve to TOKEN_HAS_NO_SUPPLY_KEY.
*
*
* .proto.Key supplyKey = 9;
*/
public boolean hasSupplyKey() {
return supplyKey_ != null;
}
/**
*
* The new Supply key of the Token. If the Token does not have currently a Supply key, transaction will resolve to TOKEN_HAS_NO_SUPPLY_KEY.
*
*
* .proto.Key supplyKey = 9;
*/
public com.hederahashgraph.api.proto.java.Key getSupplyKey() {
return supplyKey_ == null ? com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : supplyKey_;
}
/**
*
* The new Supply key of the Token. If the Token does not have currently a Supply key, transaction will resolve to TOKEN_HAS_NO_SUPPLY_KEY.
*
*
* .proto.Key supplyKey = 9;
*/
public com.hederahashgraph.api.proto.java.KeyOrBuilder getSupplyKeyOrBuilder() {
return getSupplyKey();
}
public static final int AUTORENEWACCOUNT_FIELD_NUMBER = 10;
private com.hederahashgraph.api.proto.java.AccountID autoRenewAccount_;
/**
*
* The new account which will be automatically charged to renew the token's expiration, at autoRenewPeriod interval.
*
*
* .proto.AccountID autoRenewAccount = 10;
*/
public boolean hasAutoRenewAccount() {
return autoRenewAccount_ != null;
}
/**
*
* The new account which will be automatically charged to renew the token's expiration, at autoRenewPeriod interval.
*
*
* .proto.AccountID autoRenewAccount = 10;
*/
public com.hederahashgraph.api.proto.java.AccountID getAutoRenewAccount() {
return autoRenewAccount_ == null ? com.hederahashgraph.api.proto.java.AccountID.getDefaultInstance() : autoRenewAccount_;
}
/**
*
* The new account which will be automatically charged to renew the token's expiration, at autoRenewPeriod interval.
*
*
* .proto.AccountID autoRenewAccount = 10;
*/
public com.hederahashgraph.api.proto.java.AccountIDOrBuilder getAutoRenewAccountOrBuilder() {
return getAutoRenewAccount();
}
public static final int AUTORENEWPERIOD_FIELD_NUMBER = 11;
private com.hederahashgraph.api.proto.java.Duration autoRenewPeriod_;
/**
*
* The new interval at which the auto-renew account will be charged to extend the token's expiry.
*
*
* .proto.Duration autoRenewPeriod = 11;
*/
public boolean hasAutoRenewPeriod() {
return autoRenewPeriod_ != null;
}
/**
*
* The new interval at which the auto-renew account will be charged to extend the token's expiry.
*
*
* .proto.Duration autoRenewPeriod = 11;
*/
public com.hederahashgraph.api.proto.java.Duration getAutoRenewPeriod() {
return autoRenewPeriod_ == null ? com.hederahashgraph.api.proto.java.Duration.getDefaultInstance() : autoRenewPeriod_;
}
/**
*
* The new interval at which the auto-renew account will be charged to extend the token's expiry.
*
*
* .proto.Duration autoRenewPeriod = 11;
*/
public com.hederahashgraph.api.proto.java.DurationOrBuilder getAutoRenewPeriodOrBuilder() {
return getAutoRenewPeriod();
}
public static final int EXPIRY_FIELD_NUMBER = 12;
private com.hederahashgraph.api.proto.java.Timestamp expiry_;
/**
*
* The new expiry time of the token. Expiry can be updated even if admin key is not set. If the provided expiry is earlier than the current token expiry, transaction wil resolve to INVALID_EXPIRATION_TIME
*
*
* .proto.Timestamp expiry = 12;
*/
public boolean hasExpiry() {
return expiry_ != null;
}
/**
*
* The new expiry time of the token. Expiry can be updated even if admin key is not set. If the provided expiry is earlier than the current token expiry, transaction wil resolve to INVALID_EXPIRATION_TIME
*
*
* .proto.Timestamp expiry = 12;
*/
public com.hederahashgraph.api.proto.java.Timestamp getExpiry() {
return expiry_ == null ? com.hederahashgraph.api.proto.java.Timestamp.getDefaultInstance() : expiry_;
}
/**
*
* The new expiry time of the token. Expiry can be updated even if admin key is not set. If the provided expiry is earlier than the current token expiry, transaction wil resolve to INVALID_EXPIRATION_TIME
*
*
* .proto.Timestamp expiry = 12;
*/
public com.hederahashgraph.api.proto.java.TimestampOrBuilder getExpiryOrBuilder() {
return getExpiry();
}
public static final int MEMO_FIELD_NUMBER = 13;
private com.google.protobuf.StringValue memo_;
/**
*
* If set, the new memo to be associated with the token (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 13;
*/
public boolean hasMemo() {
return memo_ != null;
}
/**
*
* If set, the new memo to be associated with the token (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 13;
*/
public com.google.protobuf.StringValue getMemo() {
return memo_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : memo_;
}
/**
*
* If set, the new memo to be associated with the token (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 13;
*/
public com.google.protobuf.StringValueOrBuilder getMemoOrBuilder() {
return getMemo();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (token_ != null) {
output.writeMessage(1, getToken());
}
if (!getSymbolBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, symbol_);
}
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, name_);
}
if (treasury_ != null) {
output.writeMessage(4, getTreasury());
}
if (adminKey_ != null) {
output.writeMessage(5, getAdminKey());
}
if (kycKey_ != null) {
output.writeMessage(6, getKycKey());
}
if (freezeKey_ != null) {
output.writeMessage(7, getFreezeKey());
}
if (wipeKey_ != null) {
output.writeMessage(8, getWipeKey());
}
if (supplyKey_ != null) {
output.writeMessage(9, getSupplyKey());
}
if (autoRenewAccount_ != null) {
output.writeMessage(10, getAutoRenewAccount());
}
if (autoRenewPeriod_ != null) {
output.writeMessage(11, getAutoRenewPeriod());
}
if (expiry_ != null) {
output.writeMessage(12, getExpiry());
}
if (memo_ != null) {
output.writeMessage(13, getMemo());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (token_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getToken());
}
if (!getSymbolBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, symbol_);
}
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, name_);
}
if (treasury_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getTreasury());
}
if (adminKey_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getAdminKey());
}
if (kycKey_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getKycKey());
}
if (freezeKey_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getFreezeKey());
}
if (wipeKey_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getWipeKey());
}
if (supplyKey_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getSupplyKey());
}
if (autoRenewAccount_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getAutoRenewAccount());
}
if (autoRenewPeriod_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getAutoRenewPeriod());
}
if (expiry_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getExpiry());
}
if (memo_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, getMemo());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody)) {
return super.equals(obj);
}
com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody other = (com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody) obj;
if (hasToken() != other.hasToken()) return false;
if (hasToken()) {
if (!getToken()
.equals(other.getToken())) return false;
}
if (!getSymbol()
.equals(other.getSymbol())) return false;
if (!getName()
.equals(other.getName())) return false;
if (hasTreasury() != other.hasTreasury()) return false;
if (hasTreasury()) {
if (!getTreasury()
.equals(other.getTreasury())) return false;
}
if (hasAdminKey() != other.hasAdminKey()) return false;
if (hasAdminKey()) {
if (!getAdminKey()
.equals(other.getAdminKey())) return false;
}
if (hasKycKey() != other.hasKycKey()) return false;
if (hasKycKey()) {
if (!getKycKey()
.equals(other.getKycKey())) return false;
}
if (hasFreezeKey() != other.hasFreezeKey()) return false;
if (hasFreezeKey()) {
if (!getFreezeKey()
.equals(other.getFreezeKey())) return false;
}
if (hasWipeKey() != other.hasWipeKey()) return false;
if (hasWipeKey()) {
if (!getWipeKey()
.equals(other.getWipeKey())) return false;
}
if (hasSupplyKey() != other.hasSupplyKey()) return false;
if (hasSupplyKey()) {
if (!getSupplyKey()
.equals(other.getSupplyKey())) return false;
}
if (hasAutoRenewAccount() != other.hasAutoRenewAccount()) return false;
if (hasAutoRenewAccount()) {
if (!getAutoRenewAccount()
.equals(other.getAutoRenewAccount())) return false;
}
if (hasAutoRenewPeriod() != other.hasAutoRenewPeriod()) return false;
if (hasAutoRenewPeriod()) {
if (!getAutoRenewPeriod()
.equals(other.getAutoRenewPeriod())) return false;
}
if (hasExpiry() != other.hasExpiry()) return false;
if (hasExpiry()) {
if (!getExpiry()
.equals(other.getExpiry())) return false;
}
if (hasMemo() != other.hasMemo()) return false;
if (hasMemo()) {
if (!getMemo()
.equals(other.getMemo())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasToken()) {
hash = (37 * hash) + TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getToken().hashCode();
}
hash = (37 * hash) + SYMBOL_FIELD_NUMBER;
hash = (53 * hash) + getSymbol().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
if (hasTreasury()) {
hash = (37 * hash) + TREASURY_FIELD_NUMBER;
hash = (53 * hash) + getTreasury().hashCode();
}
if (hasAdminKey()) {
hash = (37 * hash) + ADMINKEY_FIELD_NUMBER;
hash = (53 * hash) + getAdminKey().hashCode();
}
if (hasKycKey()) {
hash = (37 * hash) + KYCKEY_FIELD_NUMBER;
hash = (53 * hash) + getKycKey().hashCode();
}
if (hasFreezeKey()) {
hash = (37 * hash) + FREEZEKEY_FIELD_NUMBER;
hash = (53 * hash) + getFreezeKey().hashCode();
}
if (hasWipeKey()) {
hash = (37 * hash) + WIPEKEY_FIELD_NUMBER;
hash = (53 * hash) + getWipeKey().hashCode();
}
if (hasSupplyKey()) {
hash = (37 * hash) + SUPPLYKEY_FIELD_NUMBER;
hash = (53 * hash) + getSupplyKey().hashCode();
}
if (hasAutoRenewAccount()) {
hash = (37 * hash) + AUTORENEWACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAutoRenewAccount().hashCode();
}
if (hasAutoRenewPeriod()) {
hash = (37 * hash) + AUTORENEWPERIOD_FIELD_NUMBER;
hash = (53 * hash) + getAutoRenewPeriod().hashCode();
}
if (hasExpiry()) {
hash = (37 * hash) + EXPIRY_FIELD_NUMBER;
hash = (53 * hash) + getExpiry().hashCode();
}
if (hasMemo()) {
hash = (37 * hash) + MEMO_FIELD_NUMBER;
hash = (53 * hash) + getMemo().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* At consensus, updates an already created token to the given values.
*If no value is given for a field, that field is left unchanged. For an immutable tokens (that is, a token without an admin key), only the expiry may be updated. Setting any other field in that case will cause the transaction status to resolve to TOKEN_IS_IMMUTABLE.
*--- Signing Requirements ---
*1. Whether or not a token has an admin key, its expiry can be extended with only the transaction payer's signature.
*2. Updating any other field of a mutable token requires the admin key's signature.
*3. If a new admin key is set, this new key must sign <b>unless</b> it is exactly an empty <tt>KeyList</tt>. This special sentinel key removes the existing admin key and causes the token to become immutable. (Other <tt>Key</tt> structures without a constituent <tt>Ed25519</tt> key will be rejected with <tt>INVALID_ADMIN_KEY</tt>.)
*4. If a new treasury is set, the new treasury account's key must sign the transaction.
*
*
* Protobuf type {@code proto.TokenUpdateTransactionBody}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:proto.TokenUpdateTransactionBody)
com.hederahashgraph.api.proto.java.TokenUpdateTransactionBodyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.hederahashgraph.api.proto.java.TokenUpdate.internal_static_proto_TokenUpdateTransactionBody_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.hederahashgraph.api.proto.java.TokenUpdate.internal_static_proto_TokenUpdateTransactionBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody.class, com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody.Builder.class);
}
// Construct using com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (tokenBuilder_ == null) {
token_ = null;
} else {
token_ = null;
tokenBuilder_ = null;
}
symbol_ = "";
name_ = "";
if (treasuryBuilder_ == null) {
treasury_ = null;
} else {
treasury_ = null;
treasuryBuilder_ = null;
}
if (adminKeyBuilder_ == null) {
adminKey_ = null;
} else {
adminKey_ = null;
adminKeyBuilder_ = null;
}
if (kycKeyBuilder_ == null) {
kycKey_ = null;
} else {
kycKey_ = null;
kycKeyBuilder_ = null;
}
if (freezeKeyBuilder_ == null) {
freezeKey_ = null;
} else {
freezeKey_ = null;
freezeKeyBuilder_ = null;
}
if (wipeKeyBuilder_ == null) {
wipeKey_ = null;
} else {
wipeKey_ = null;
wipeKeyBuilder_ = null;
}
if (supplyKeyBuilder_ == null) {
supplyKey_ = null;
} else {
supplyKey_ = null;
supplyKeyBuilder_ = null;
}
if (autoRenewAccountBuilder_ == null) {
autoRenewAccount_ = null;
} else {
autoRenewAccount_ = null;
autoRenewAccountBuilder_ = null;
}
if (autoRenewPeriodBuilder_ == null) {
autoRenewPeriod_ = null;
} else {
autoRenewPeriod_ = null;
autoRenewPeriodBuilder_ = null;
}
if (expiryBuilder_ == null) {
expiry_ = null;
} else {
expiry_ = null;
expiryBuilder_ = null;
}
if (memoBuilder_ == null) {
memo_ = null;
} else {
memo_ = null;
memoBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.hederahashgraph.api.proto.java.TokenUpdate.internal_static_proto_TokenUpdateTransactionBody_descriptor;
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody getDefaultInstanceForType() {
return com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody.getDefaultInstance();
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody build() {
com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody buildPartial() {
com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody result = new com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody(this);
if (tokenBuilder_ == null) {
result.token_ = token_;
} else {
result.token_ = tokenBuilder_.build();
}
result.symbol_ = symbol_;
result.name_ = name_;
if (treasuryBuilder_ == null) {
result.treasury_ = treasury_;
} else {
result.treasury_ = treasuryBuilder_.build();
}
if (adminKeyBuilder_ == null) {
result.adminKey_ = adminKey_;
} else {
result.adminKey_ = adminKeyBuilder_.build();
}
if (kycKeyBuilder_ == null) {
result.kycKey_ = kycKey_;
} else {
result.kycKey_ = kycKeyBuilder_.build();
}
if (freezeKeyBuilder_ == null) {
result.freezeKey_ = freezeKey_;
} else {
result.freezeKey_ = freezeKeyBuilder_.build();
}
if (wipeKeyBuilder_ == null) {
result.wipeKey_ = wipeKey_;
} else {
result.wipeKey_ = wipeKeyBuilder_.build();
}
if (supplyKeyBuilder_ == null) {
result.supplyKey_ = supplyKey_;
} else {
result.supplyKey_ = supplyKeyBuilder_.build();
}
if (autoRenewAccountBuilder_ == null) {
result.autoRenewAccount_ = autoRenewAccount_;
} else {
result.autoRenewAccount_ = autoRenewAccountBuilder_.build();
}
if (autoRenewPeriodBuilder_ == null) {
result.autoRenewPeriod_ = autoRenewPeriod_;
} else {
result.autoRenewPeriod_ = autoRenewPeriodBuilder_.build();
}
if (expiryBuilder_ == null) {
result.expiry_ = expiry_;
} else {
result.expiry_ = expiryBuilder_.build();
}
if (memoBuilder_ == null) {
result.memo_ = memo_;
} else {
result.memo_ = memoBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody) {
return mergeFrom((com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody other) {
if (other == com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody.getDefaultInstance()) return this;
if (other.hasToken()) {
mergeToken(other.getToken());
}
if (!other.getSymbol().isEmpty()) {
symbol_ = other.symbol_;
onChanged();
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (other.hasTreasury()) {
mergeTreasury(other.getTreasury());
}
if (other.hasAdminKey()) {
mergeAdminKey(other.getAdminKey());
}
if (other.hasKycKey()) {
mergeKycKey(other.getKycKey());
}
if (other.hasFreezeKey()) {
mergeFreezeKey(other.getFreezeKey());
}
if (other.hasWipeKey()) {
mergeWipeKey(other.getWipeKey());
}
if (other.hasSupplyKey()) {
mergeSupplyKey(other.getSupplyKey());
}
if (other.hasAutoRenewAccount()) {
mergeAutoRenewAccount(other.getAutoRenewAccount());
}
if (other.hasAutoRenewPeriod()) {
mergeAutoRenewPeriod(other.getAutoRenewPeriod());
}
if (other.hasExpiry()) {
mergeExpiry(other.getExpiry());
}
if (other.hasMemo()) {
mergeMemo(other.getMemo());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.hederahashgraph.api.proto.java.TokenID token_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.TokenID, com.hederahashgraph.api.proto.java.TokenID.Builder, com.hederahashgraph.api.proto.java.TokenIDOrBuilder> tokenBuilder_;
/**
*
* The Token to be updated
*
*
* .proto.TokenID token = 1;
*/
public boolean hasToken() {
return tokenBuilder_ != null || token_ != null;
}
/**
*
* The Token to be updated
*
*
* .proto.TokenID token = 1;
*/
public com.hederahashgraph.api.proto.java.TokenID getToken() {
if (tokenBuilder_ == null) {
return token_ == null ? com.hederahashgraph.api.proto.java.TokenID.getDefaultInstance() : token_;
} else {
return tokenBuilder_.getMessage();
}
}
/**
*
* The Token to be updated
*
*
* .proto.TokenID token = 1;
*/
public Builder setToken(com.hederahashgraph.api.proto.java.TokenID value) {
if (tokenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
token_ = value;
onChanged();
} else {
tokenBuilder_.setMessage(value);
}
return this;
}
/**
*
* The Token to be updated
*
*
* .proto.TokenID token = 1;
*/
public Builder setToken(
com.hederahashgraph.api.proto.java.TokenID.Builder builderForValue) {
if (tokenBuilder_ == null) {
token_ = builderForValue.build();
onChanged();
} else {
tokenBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The Token to be updated
*
*
* .proto.TokenID token = 1;
*/
public Builder mergeToken(com.hederahashgraph.api.proto.java.TokenID value) {
if (tokenBuilder_ == null) {
if (token_ != null) {
token_ =
com.hederahashgraph.api.proto.java.TokenID.newBuilder(token_).mergeFrom(value).buildPartial();
} else {
token_ = value;
}
onChanged();
} else {
tokenBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The Token to be updated
*
*
* .proto.TokenID token = 1;
*/
public Builder clearToken() {
if (tokenBuilder_ == null) {
token_ = null;
onChanged();
} else {
token_ = null;
tokenBuilder_ = null;
}
return this;
}
/**
*
* The Token to be updated
*
*
* .proto.TokenID token = 1;
*/
public com.hederahashgraph.api.proto.java.TokenID.Builder getTokenBuilder() {
onChanged();
return getTokenFieldBuilder().getBuilder();
}
/**
*
* The Token to be updated
*
*
* .proto.TokenID token = 1;
*/
public com.hederahashgraph.api.proto.java.TokenIDOrBuilder getTokenOrBuilder() {
if (tokenBuilder_ != null) {
return tokenBuilder_.getMessageOrBuilder();
} else {
return token_ == null ?
com.hederahashgraph.api.proto.java.TokenID.getDefaultInstance() : token_;
}
}
/**
*
* The Token to be updated
*
*
* .proto.TokenID token = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.TokenID, com.hederahashgraph.api.proto.java.TokenID.Builder, com.hederahashgraph.api.proto.java.TokenIDOrBuilder>
getTokenFieldBuilder() {
if (tokenBuilder_ == null) {
tokenBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.TokenID, com.hederahashgraph.api.proto.java.TokenID.Builder, com.hederahashgraph.api.proto.java.TokenIDOrBuilder>(
getToken(),
getParentForChildren(),
isClean());
token_ = null;
}
return tokenBuilder_;
}
private java.lang.Object symbol_ = "";
/**
*
* The new publicly visible Token symbol, limited to a UTF-8 encoding of length <tt>tokens.maxTokenNameUtf8Bytes</tt>.
*
*
* string symbol = 2;
*/
public java.lang.String getSymbol() {
java.lang.Object ref = symbol_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
symbol_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The new publicly visible Token symbol, limited to a UTF-8 encoding of length <tt>tokens.maxTokenNameUtf8Bytes</tt>.
*
*
* string symbol = 2;
*/
public com.google.protobuf.ByteString
getSymbolBytes() {
java.lang.Object ref = symbol_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
symbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The new publicly visible Token symbol, limited to a UTF-8 encoding of length <tt>tokens.maxTokenNameUtf8Bytes</tt>.
*
*
* string symbol = 2;
*/
public Builder setSymbol(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
symbol_ = value;
onChanged();
return this;
}
/**
*
* The new publicly visible Token symbol, limited to a UTF-8 encoding of length <tt>tokens.maxTokenNameUtf8Bytes</tt>.
*
*
* string symbol = 2;
*/
public Builder clearSymbol() {
symbol_ = getDefaultInstance().getSymbol();
onChanged();
return this;
}
/**
*
* The new publicly visible Token symbol, limited to a UTF-8 encoding of length <tt>tokens.maxTokenNameUtf8Bytes</tt>.
*
*
* string symbol = 2;
*/
public Builder setSymbolBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
symbol_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* The new publicly visible name of the Token, limited to a UTF-8 encoding of length <tt>tokens.maxSymbolUtf8Bytes</tt>.
*
*
* string name = 3;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The new publicly visible name of the Token, limited to a UTF-8 encoding of length <tt>tokens.maxSymbolUtf8Bytes</tt>.
*
*
* string name = 3;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The new publicly visible name of the Token, limited to a UTF-8 encoding of length <tt>tokens.maxSymbolUtf8Bytes</tt>.
*
*
* string name = 3;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
*
* The new publicly visible name of the Token, limited to a UTF-8 encoding of length <tt>tokens.maxSymbolUtf8Bytes</tt>.
*
*
* string name = 3;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* The new publicly visible name of the Token, limited to a UTF-8 encoding of length <tt>tokens.maxSymbolUtf8Bytes</tt>.
*
*
* string name = 3;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private com.hederahashgraph.api.proto.java.AccountID treasury_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.AccountID, com.hederahashgraph.api.proto.java.AccountID.Builder, com.hederahashgraph.api.proto.java.AccountIDOrBuilder> treasuryBuilder_;
/**
*
* The new Treasury account of the Token. If the provided treasury account is not existing or deleted, the response will be INVALID_TREASURY_ACCOUNT_FOR_TOKEN. If successful, the Token balance held in the previous Treasury Account is transferred to the new one.
*
*
* .proto.AccountID treasury = 4;
*/
public boolean hasTreasury() {
return treasuryBuilder_ != null || treasury_ != null;
}
/**
*
* The new Treasury account of the Token. If the provided treasury account is not existing or deleted, the response will be INVALID_TREASURY_ACCOUNT_FOR_TOKEN. If successful, the Token balance held in the previous Treasury Account is transferred to the new one.
*
*
* .proto.AccountID treasury = 4;
*/
public com.hederahashgraph.api.proto.java.AccountID getTreasury() {
if (treasuryBuilder_ == null) {
return treasury_ == null ? com.hederahashgraph.api.proto.java.AccountID.getDefaultInstance() : treasury_;
} else {
return treasuryBuilder_.getMessage();
}
}
/**
*
* The new Treasury account of the Token. If the provided treasury account is not existing or deleted, the response will be INVALID_TREASURY_ACCOUNT_FOR_TOKEN. If successful, the Token balance held in the previous Treasury Account is transferred to the new one.
*
*
* .proto.AccountID treasury = 4;
*/
public Builder setTreasury(com.hederahashgraph.api.proto.java.AccountID value) {
if (treasuryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
treasury_ = value;
onChanged();
} else {
treasuryBuilder_.setMessage(value);
}
return this;
}
/**
*
* The new Treasury account of the Token. If the provided treasury account is not existing or deleted, the response will be INVALID_TREASURY_ACCOUNT_FOR_TOKEN. If successful, the Token balance held in the previous Treasury Account is transferred to the new one.
*
*
* .proto.AccountID treasury = 4;
*/
public Builder setTreasury(
com.hederahashgraph.api.proto.java.AccountID.Builder builderForValue) {
if (treasuryBuilder_ == null) {
treasury_ = builderForValue.build();
onChanged();
} else {
treasuryBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The new Treasury account of the Token. If the provided treasury account is not existing or deleted, the response will be INVALID_TREASURY_ACCOUNT_FOR_TOKEN. If successful, the Token balance held in the previous Treasury Account is transferred to the new one.
*
*
* .proto.AccountID treasury = 4;
*/
public Builder mergeTreasury(com.hederahashgraph.api.proto.java.AccountID value) {
if (treasuryBuilder_ == null) {
if (treasury_ != null) {
treasury_ =
com.hederahashgraph.api.proto.java.AccountID.newBuilder(treasury_).mergeFrom(value).buildPartial();
} else {
treasury_ = value;
}
onChanged();
} else {
treasuryBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The new Treasury account of the Token. If the provided treasury account is not existing or deleted, the response will be INVALID_TREASURY_ACCOUNT_FOR_TOKEN. If successful, the Token balance held in the previous Treasury Account is transferred to the new one.
*
*
* .proto.AccountID treasury = 4;
*/
public Builder clearTreasury() {
if (treasuryBuilder_ == null) {
treasury_ = null;
onChanged();
} else {
treasury_ = null;
treasuryBuilder_ = null;
}
return this;
}
/**
*
* The new Treasury account of the Token. If the provided treasury account is not existing or deleted, the response will be INVALID_TREASURY_ACCOUNT_FOR_TOKEN. If successful, the Token balance held in the previous Treasury Account is transferred to the new one.
*
*
* .proto.AccountID treasury = 4;
*/
public com.hederahashgraph.api.proto.java.AccountID.Builder getTreasuryBuilder() {
onChanged();
return getTreasuryFieldBuilder().getBuilder();
}
/**
*
* The new Treasury account of the Token. If the provided treasury account is not existing or deleted, the response will be INVALID_TREASURY_ACCOUNT_FOR_TOKEN. If successful, the Token balance held in the previous Treasury Account is transferred to the new one.
*
*
* .proto.AccountID treasury = 4;
*/
public com.hederahashgraph.api.proto.java.AccountIDOrBuilder getTreasuryOrBuilder() {
if (treasuryBuilder_ != null) {
return treasuryBuilder_.getMessageOrBuilder();
} else {
return treasury_ == null ?
com.hederahashgraph.api.proto.java.AccountID.getDefaultInstance() : treasury_;
}
}
/**
*
* The new Treasury account of the Token. If the provided treasury account is not existing or deleted, the response will be INVALID_TREASURY_ACCOUNT_FOR_TOKEN. If successful, the Token balance held in the previous Treasury Account is transferred to the new one.
*
*
* .proto.AccountID treasury = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.AccountID, com.hederahashgraph.api.proto.java.AccountID.Builder, com.hederahashgraph.api.proto.java.AccountIDOrBuilder>
getTreasuryFieldBuilder() {
if (treasuryBuilder_ == null) {
treasuryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.AccountID, com.hederahashgraph.api.proto.java.AccountID.Builder, com.hederahashgraph.api.proto.java.AccountIDOrBuilder>(
getTreasury(),
getParentForChildren(),
isClean());
treasury_ = null;
}
return treasuryBuilder_;
}
private com.hederahashgraph.api.proto.java.Key adminKey_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder> adminKeyBuilder_;
/**
*
* The new admin key of the Token. If Token is immutable, transaction will resolve to TOKEN_IS_IMMUTABlE.
*
*
* .proto.Key adminKey = 5;
*/
public boolean hasAdminKey() {
return adminKeyBuilder_ != null || adminKey_ != null;
}
/**
*
* The new admin key of the Token. If Token is immutable, transaction will resolve to TOKEN_IS_IMMUTABlE.
*
*
* .proto.Key adminKey = 5;
*/
public com.hederahashgraph.api.proto.java.Key getAdminKey() {
if (adminKeyBuilder_ == null) {
return adminKey_ == null ? com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : adminKey_;
} else {
return adminKeyBuilder_.getMessage();
}
}
/**
*
* The new admin key of the Token. If Token is immutable, transaction will resolve to TOKEN_IS_IMMUTABlE.
*
*
* .proto.Key adminKey = 5;
*/
public Builder setAdminKey(com.hederahashgraph.api.proto.java.Key value) {
if (adminKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
adminKey_ = value;
onChanged();
} else {
adminKeyBuilder_.setMessage(value);
}
return this;
}
/**
*
* The new admin key of the Token. If Token is immutable, transaction will resolve to TOKEN_IS_IMMUTABlE.
*
*
* .proto.Key adminKey = 5;
*/
public Builder setAdminKey(
com.hederahashgraph.api.proto.java.Key.Builder builderForValue) {
if (adminKeyBuilder_ == null) {
adminKey_ = builderForValue.build();
onChanged();
} else {
adminKeyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The new admin key of the Token. If Token is immutable, transaction will resolve to TOKEN_IS_IMMUTABlE.
*
*
* .proto.Key adminKey = 5;
*/
public Builder mergeAdminKey(com.hederahashgraph.api.proto.java.Key value) {
if (adminKeyBuilder_ == null) {
if (adminKey_ != null) {
adminKey_ =
com.hederahashgraph.api.proto.java.Key.newBuilder(adminKey_).mergeFrom(value).buildPartial();
} else {
adminKey_ = value;
}
onChanged();
} else {
adminKeyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The new admin key of the Token. If Token is immutable, transaction will resolve to TOKEN_IS_IMMUTABlE.
*
*
* .proto.Key adminKey = 5;
*/
public Builder clearAdminKey() {
if (adminKeyBuilder_ == null) {
adminKey_ = null;
onChanged();
} else {
adminKey_ = null;
adminKeyBuilder_ = null;
}
return this;
}
/**
*
* The new admin key of the Token. If Token is immutable, transaction will resolve to TOKEN_IS_IMMUTABlE.
*
*
* .proto.Key adminKey = 5;
*/
public com.hederahashgraph.api.proto.java.Key.Builder getAdminKeyBuilder() {
onChanged();
return getAdminKeyFieldBuilder().getBuilder();
}
/**
*
* The new admin key of the Token. If Token is immutable, transaction will resolve to TOKEN_IS_IMMUTABlE.
*
*
* .proto.Key adminKey = 5;
*/
public com.hederahashgraph.api.proto.java.KeyOrBuilder getAdminKeyOrBuilder() {
if (adminKeyBuilder_ != null) {
return adminKeyBuilder_.getMessageOrBuilder();
} else {
return adminKey_ == null ?
com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : adminKey_;
}
}
/**
*
* The new admin key of the Token. If Token is immutable, transaction will resolve to TOKEN_IS_IMMUTABlE.
*
*
* .proto.Key adminKey = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder>
getAdminKeyFieldBuilder() {
if (adminKeyBuilder_ == null) {
adminKeyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder>(
getAdminKey(),
getParentForChildren(),
isClean());
adminKey_ = null;
}
return adminKeyBuilder_;
}
private com.hederahashgraph.api.proto.java.Key kycKey_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder> kycKeyBuilder_;
/**
*
* The new KYC key of the Token. If Token does not have currently a KYC key, transaction will resolve to TOKEN_HAS_NO_KYC_KEY.
*
*
* .proto.Key kycKey = 6;
*/
public boolean hasKycKey() {
return kycKeyBuilder_ != null || kycKey_ != null;
}
/**
*
* The new KYC key of the Token. If Token does not have currently a KYC key, transaction will resolve to TOKEN_HAS_NO_KYC_KEY.
*
*
* .proto.Key kycKey = 6;
*/
public com.hederahashgraph.api.proto.java.Key getKycKey() {
if (kycKeyBuilder_ == null) {
return kycKey_ == null ? com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : kycKey_;
} else {
return kycKeyBuilder_.getMessage();
}
}
/**
*
* The new KYC key of the Token. If Token does not have currently a KYC key, transaction will resolve to TOKEN_HAS_NO_KYC_KEY.
*
*
* .proto.Key kycKey = 6;
*/
public Builder setKycKey(com.hederahashgraph.api.proto.java.Key value) {
if (kycKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
kycKey_ = value;
onChanged();
} else {
kycKeyBuilder_.setMessage(value);
}
return this;
}
/**
*
* The new KYC key of the Token. If Token does not have currently a KYC key, transaction will resolve to TOKEN_HAS_NO_KYC_KEY.
*
*
* .proto.Key kycKey = 6;
*/
public Builder setKycKey(
com.hederahashgraph.api.proto.java.Key.Builder builderForValue) {
if (kycKeyBuilder_ == null) {
kycKey_ = builderForValue.build();
onChanged();
} else {
kycKeyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The new KYC key of the Token. If Token does not have currently a KYC key, transaction will resolve to TOKEN_HAS_NO_KYC_KEY.
*
*
* .proto.Key kycKey = 6;
*/
public Builder mergeKycKey(com.hederahashgraph.api.proto.java.Key value) {
if (kycKeyBuilder_ == null) {
if (kycKey_ != null) {
kycKey_ =
com.hederahashgraph.api.proto.java.Key.newBuilder(kycKey_).mergeFrom(value).buildPartial();
} else {
kycKey_ = value;
}
onChanged();
} else {
kycKeyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The new KYC key of the Token. If Token does not have currently a KYC key, transaction will resolve to TOKEN_HAS_NO_KYC_KEY.
*
*
* .proto.Key kycKey = 6;
*/
public Builder clearKycKey() {
if (kycKeyBuilder_ == null) {
kycKey_ = null;
onChanged();
} else {
kycKey_ = null;
kycKeyBuilder_ = null;
}
return this;
}
/**
*
* The new KYC key of the Token. If Token does not have currently a KYC key, transaction will resolve to TOKEN_HAS_NO_KYC_KEY.
*
*
* .proto.Key kycKey = 6;
*/
public com.hederahashgraph.api.proto.java.Key.Builder getKycKeyBuilder() {
onChanged();
return getKycKeyFieldBuilder().getBuilder();
}
/**
*
* The new KYC key of the Token. If Token does not have currently a KYC key, transaction will resolve to TOKEN_HAS_NO_KYC_KEY.
*
*
* .proto.Key kycKey = 6;
*/
public com.hederahashgraph.api.proto.java.KeyOrBuilder getKycKeyOrBuilder() {
if (kycKeyBuilder_ != null) {
return kycKeyBuilder_.getMessageOrBuilder();
} else {
return kycKey_ == null ?
com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : kycKey_;
}
}
/**
*
* The new KYC key of the Token. If Token does not have currently a KYC key, transaction will resolve to TOKEN_HAS_NO_KYC_KEY.
*
*
* .proto.Key kycKey = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder>
getKycKeyFieldBuilder() {
if (kycKeyBuilder_ == null) {
kycKeyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder>(
getKycKey(),
getParentForChildren(),
isClean());
kycKey_ = null;
}
return kycKeyBuilder_;
}
private com.hederahashgraph.api.proto.java.Key freezeKey_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder> freezeKeyBuilder_;
/**
*
* The new Freeze key of the Token. If the Token does not have currently a Freeze key, transaction will resolve to TOKEN_HAS_NO_FREEZE_KEY.
*
*
* .proto.Key freezeKey = 7;
*/
public boolean hasFreezeKey() {
return freezeKeyBuilder_ != null || freezeKey_ != null;
}
/**
*
* The new Freeze key of the Token. If the Token does not have currently a Freeze key, transaction will resolve to TOKEN_HAS_NO_FREEZE_KEY.
*
*
* .proto.Key freezeKey = 7;
*/
public com.hederahashgraph.api.proto.java.Key getFreezeKey() {
if (freezeKeyBuilder_ == null) {
return freezeKey_ == null ? com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : freezeKey_;
} else {
return freezeKeyBuilder_.getMessage();
}
}
/**
*
* The new Freeze key of the Token. If the Token does not have currently a Freeze key, transaction will resolve to TOKEN_HAS_NO_FREEZE_KEY.
*
*
* .proto.Key freezeKey = 7;
*/
public Builder setFreezeKey(com.hederahashgraph.api.proto.java.Key value) {
if (freezeKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
freezeKey_ = value;
onChanged();
} else {
freezeKeyBuilder_.setMessage(value);
}
return this;
}
/**
*
* The new Freeze key of the Token. If the Token does not have currently a Freeze key, transaction will resolve to TOKEN_HAS_NO_FREEZE_KEY.
*
*
* .proto.Key freezeKey = 7;
*/
public Builder setFreezeKey(
com.hederahashgraph.api.proto.java.Key.Builder builderForValue) {
if (freezeKeyBuilder_ == null) {
freezeKey_ = builderForValue.build();
onChanged();
} else {
freezeKeyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The new Freeze key of the Token. If the Token does not have currently a Freeze key, transaction will resolve to TOKEN_HAS_NO_FREEZE_KEY.
*
*
* .proto.Key freezeKey = 7;
*/
public Builder mergeFreezeKey(com.hederahashgraph.api.proto.java.Key value) {
if (freezeKeyBuilder_ == null) {
if (freezeKey_ != null) {
freezeKey_ =
com.hederahashgraph.api.proto.java.Key.newBuilder(freezeKey_).mergeFrom(value).buildPartial();
} else {
freezeKey_ = value;
}
onChanged();
} else {
freezeKeyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The new Freeze key of the Token. If the Token does not have currently a Freeze key, transaction will resolve to TOKEN_HAS_NO_FREEZE_KEY.
*
*
* .proto.Key freezeKey = 7;
*/
public Builder clearFreezeKey() {
if (freezeKeyBuilder_ == null) {
freezeKey_ = null;
onChanged();
} else {
freezeKey_ = null;
freezeKeyBuilder_ = null;
}
return this;
}
/**
*
* The new Freeze key of the Token. If the Token does not have currently a Freeze key, transaction will resolve to TOKEN_HAS_NO_FREEZE_KEY.
*
*
* .proto.Key freezeKey = 7;
*/
public com.hederahashgraph.api.proto.java.Key.Builder getFreezeKeyBuilder() {
onChanged();
return getFreezeKeyFieldBuilder().getBuilder();
}
/**
*
* The new Freeze key of the Token. If the Token does not have currently a Freeze key, transaction will resolve to TOKEN_HAS_NO_FREEZE_KEY.
*
*
* .proto.Key freezeKey = 7;
*/
public com.hederahashgraph.api.proto.java.KeyOrBuilder getFreezeKeyOrBuilder() {
if (freezeKeyBuilder_ != null) {
return freezeKeyBuilder_.getMessageOrBuilder();
} else {
return freezeKey_ == null ?
com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : freezeKey_;
}
}
/**
*
* The new Freeze key of the Token. If the Token does not have currently a Freeze key, transaction will resolve to TOKEN_HAS_NO_FREEZE_KEY.
*
*
* .proto.Key freezeKey = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder>
getFreezeKeyFieldBuilder() {
if (freezeKeyBuilder_ == null) {
freezeKeyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder>(
getFreezeKey(),
getParentForChildren(),
isClean());
freezeKey_ = null;
}
return freezeKeyBuilder_;
}
private com.hederahashgraph.api.proto.java.Key wipeKey_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder> wipeKeyBuilder_;
/**
*
* The new Wipe key of the Token. If the Token does not have currently a Wipe key, transaction will resolve to TOKEN_HAS_NO_WIPE_KEY.
*
*
* .proto.Key wipeKey = 8;
*/
public boolean hasWipeKey() {
return wipeKeyBuilder_ != null || wipeKey_ != null;
}
/**
*
* The new Wipe key of the Token. If the Token does not have currently a Wipe key, transaction will resolve to TOKEN_HAS_NO_WIPE_KEY.
*
*
* .proto.Key wipeKey = 8;
*/
public com.hederahashgraph.api.proto.java.Key getWipeKey() {
if (wipeKeyBuilder_ == null) {
return wipeKey_ == null ? com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : wipeKey_;
} else {
return wipeKeyBuilder_.getMessage();
}
}
/**
*
* The new Wipe key of the Token. If the Token does not have currently a Wipe key, transaction will resolve to TOKEN_HAS_NO_WIPE_KEY.
*
*
* .proto.Key wipeKey = 8;
*/
public Builder setWipeKey(com.hederahashgraph.api.proto.java.Key value) {
if (wipeKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
wipeKey_ = value;
onChanged();
} else {
wipeKeyBuilder_.setMessage(value);
}
return this;
}
/**
*
* The new Wipe key of the Token. If the Token does not have currently a Wipe key, transaction will resolve to TOKEN_HAS_NO_WIPE_KEY.
*
*
* .proto.Key wipeKey = 8;
*/
public Builder setWipeKey(
com.hederahashgraph.api.proto.java.Key.Builder builderForValue) {
if (wipeKeyBuilder_ == null) {
wipeKey_ = builderForValue.build();
onChanged();
} else {
wipeKeyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The new Wipe key of the Token. If the Token does not have currently a Wipe key, transaction will resolve to TOKEN_HAS_NO_WIPE_KEY.
*
*
* .proto.Key wipeKey = 8;
*/
public Builder mergeWipeKey(com.hederahashgraph.api.proto.java.Key value) {
if (wipeKeyBuilder_ == null) {
if (wipeKey_ != null) {
wipeKey_ =
com.hederahashgraph.api.proto.java.Key.newBuilder(wipeKey_).mergeFrom(value).buildPartial();
} else {
wipeKey_ = value;
}
onChanged();
} else {
wipeKeyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The new Wipe key of the Token. If the Token does not have currently a Wipe key, transaction will resolve to TOKEN_HAS_NO_WIPE_KEY.
*
*
* .proto.Key wipeKey = 8;
*/
public Builder clearWipeKey() {
if (wipeKeyBuilder_ == null) {
wipeKey_ = null;
onChanged();
} else {
wipeKey_ = null;
wipeKeyBuilder_ = null;
}
return this;
}
/**
*
* The new Wipe key of the Token. If the Token does not have currently a Wipe key, transaction will resolve to TOKEN_HAS_NO_WIPE_KEY.
*
*
* .proto.Key wipeKey = 8;
*/
public com.hederahashgraph.api.proto.java.Key.Builder getWipeKeyBuilder() {
onChanged();
return getWipeKeyFieldBuilder().getBuilder();
}
/**
*
* The new Wipe key of the Token. If the Token does not have currently a Wipe key, transaction will resolve to TOKEN_HAS_NO_WIPE_KEY.
*
*
* .proto.Key wipeKey = 8;
*/
public com.hederahashgraph.api.proto.java.KeyOrBuilder getWipeKeyOrBuilder() {
if (wipeKeyBuilder_ != null) {
return wipeKeyBuilder_.getMessageOrBuilder();
} else {
return wipeKey_ == null ?
com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : wipeKey_;
}
}
/**
*
* The new Wipe key of the Token. If the Token does not have currently a Wipe key, transaction will resolve to TOKEN_HAS_NO_WIPE_KEY.
*
*
* .proto.Key wipeKey = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder>
getWipeKeyFieldBuilder() {
if (wipeKeyBuilder_ == null) {
wipeKeyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder>(
getWipeKey(),
getParentForChildren(),
isClean());
wipeKey_ = null;
}
return wipeKeyBuilder_;
}
private com.hederahashgraph.api.proto.java.Key supplyKey_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder> supplyKeyBuilder_;
/**
*
* The new Supply key of the Token. If the Token does not have currently a Supply key, transaction will resolve to TOKEN_HAS_NO_SUPPLY_KEY.
*
*
* .proto.Key supplyKey = 9;
*/
public boolean hasSupplyKey() {
return supplyKeyBuilder_ != null || supplyKey_ != null;
}
/**
*
* The new Supply key of the Token. If the Token does not have currently a Supply key, transaction will resolve to TOKEN_HAS_NO_SUPPLY_KEY.
*
*
* .proto.Key supplyKey = 9;
*/
public com.hederahashgraph.api.proto.java.Key getSupplyKey() {
if (supplyKeyBuilder_ == null) {
return supplyKey_ == null ? com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : supplyKey_;
} else {
return supplyKeyBuilder_.getMessage();
}
}
/**
*
* The new Supply key of the Token. If the Token does not have currently a Supply key, transaction will resolve to TOKEN_HAS_NO_SUPPLY_KEY.
*
*
* .proto.Key supplyKey = 9;
*/
public Builder setSupplyKey(com.hederahashgraph.api.proto.java.Key value) {
if (supplyKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
supplyKey_ = value;
onChanged();
} else {
supplyKeyBuilder_.setMessage(value);
}
return this;
}
/**
*
* The new Supply key of the Token. If the Token does not have currently a Supply key, transaction will resolve to TOKEN_HAS_NO_SUPPLY_KEY.
*
*
* .proto.Key supplyKey = 9;
*/
public Builder setSupplyKey(
com.hederahashgraph.api.proto.java.Key.Builder builderForValue) {
if (supplyKeyBuilder_ == null) {
supplyKey_ = builderForValue.build();
onChanged();
} else {
supplyKeyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The new Supply key of the Token. If the Token does not have currently a Supply key, transaction will resolve to TOKEN_HAS_NO_SUPPLY_KEY.
*
*
* .proto.Key supplyKey = 9;
*/
public Builder mergeSupplyKey(com.hederahashgraph.api.proto.java.Key value) {
if (supplyKeyBuilder_ == null) {
if (supplyKey_ != null) {
supplyKey_ =
com.hederahashgraph.api.proto.java.Key.newBuilder(supplyKey_).mergeFrom(value).buildPartial();
} else {
supplyKey_ = value;
}
onChanged();
} else {
supplyKeyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The new Supply key of the Token. If the Token does not have currently a Supply key, transaction will resolve to TOKEN_HAS_NO_SUPPLY_KEY.
*
*
* .proto.Key supplyKey = 9;
*/
public Builder clearSupplyKey() {
if (supplyKeyBuilder_ == null) {
supplyKey_ = null;
onChanged();
} else {
supplyKey_ = null;
supplyKeyBuilder_ = null;
}
return this;
}
/**
*
* The new Supply key of the Token. If the Token does not have currently a Supply key, transaction will resolve to TOKEN_HAS_NO_SUPPLY_KEY.
*
*
* .proto.Key supplyKey = 9;
*/
public com.hederahashgraph.api.proto.java.Key.Builder getSupplyKeyBuilder() {
onChanged();
return getSupplyKeyFieldBuilder().getBuilder();
}
/**
*
* The new Supply key of the Token. If the Token does not have currently a Supply key, transaction will resolve to TOKEN_HAS_NO_SUPPLY_KEY.
*
*
* .proto.Key supplyKey = 9;
*/
public com.hederahashgraph.api.proto.java.KeyOrBuilder getSupplyKeyOrBuilder() {
if (supplyKeyBuilder_ != null) {
return supplyKeyBuilder_.getMessageOrBuilder();
} else {
return supplyKey_ == null ?
com.hederahashgraph.api.proto.java.Key.getDefaultInstance() : supplyKey_;
}
}
/**
*
* The new Supply key of the Token. If the Token does not have currently a Supply key, transaction will resolve to TOKEN_HAS_NO_SUPPLY_KEY.
*
*
* .proto.Key supplyKey = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder>
getSupplyKeyFieldBuilder() {
if (supplyKeyBuilder_ == null) {
supplyKeyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Key, com.hederahashgraph.api.proto.java.Key.Builder, com.hederahashgraph.api.proto.java.KeyOrBuilder>(
getSupplyKey(),
getParentForChildren(),
isClean());
supplyKey_ = null;
}
return supplyKeyBuilder_;
}
private com.hederahashgraph.api.proto.java.AccountID autoRenewAccount_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.AccountID, com.hederahashgraph.api.proto.java.AccountID.Builder, com.hederahashgraph.api.proto.java.AccountIDOrBuilder> autoRenewAccountBuilder_;
/**
*
* The new account which will be automatically charged to renew the token's expiration, at autoRenewPeriod interval.
*
*
* .proto.AccountID autoRenewAccount = 10;
*/
public boolean hasAutoRenewAccount() {
return autoRenewAccountBuilder_ != null || autoRenewAccount_ != null;
}
/**
*
* The new account which will be automatically charged to renew the token's expiration, at autoRenewPeriod interval.
*
*
* .proto.AccountID autoRenewAccount = 10;
*/
public com.hederahashgraph.api.proto.java.AccountID getAutoRenewAccount() {
if (autoRenewAccountBuilder_ == null) {
return autoRenewAccount_ == null ? com.hederahashgraph.api.proto.java.AccountID.getDefaultInstance() : autoRenewAccount_;
} else {
return autoRenewAccountBuilder_.getMessage();
}
}
/**
*
* The new account which will be automatically charged to renew the token's expiration, at autoRenewPeriod interval.
*
*
* .proto.AccountID autoRenewAccount = 10;
*/
public Builder setAutoRenewAccount(com.hederahashgraph.api.proto.java.AccountID value) {
if (autoRenewAccountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
autoRenewAccount_ = value;
onChanged();
} else {
autoRenewAccountBuilder_.setMessage(value);
}
return this;
}
/**
*
* The new account which will be automatically charged to renew the token's expiration, at autoRenewPeriod interval.
*
*
* .proto.AccountID autoRenewAccount = 10;
*/
public Builder setAutoRenewAccount(
com.hederahashgraph.api.proto.java.AccountID.Builder builderForValue) {
if (autoRenewAccountBuilder_ == null) {
autoRenewAccount_ = builderForValue.build();
onChanged();
} else {
autoRenewAccountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The new account which will be automatically charged to renew the token's expiration, at autoRenewPeriod interval.
*
*
* .proto.AccountID autoRenewAccount = 10;
*/
public Builder mergeAutoRenewAccount(com.hederahashgraph.api.proto.java.AccountID value) {
if (autoRenewAccountBuilder_ == null) {
if (autoRenewAccount_ != null) {
autoRenewAccount_ =
com.hederahashgraph.api.proto.java.AccountID.newBuilder(autoRenewAccount_).mergeFrom(value).buildPartial();
} else {
autoRenewAccount_ = value;
}
onChanged();
} else {
autoRenewAccountBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The new account which will be automatically charged to renew the token's expiration, at autoRenewPeriod interval.
*
*
* .proto.AccountID autoRenewAccount = 10;
*/
public Builder clearAutoRenewAccount() {
if (autoRenewAccountBuilder_ == null) {
autoRenewAccount_ = null;
onChanged();
} else {
autoRenewAccount_ = null;
autoRenewAccountBuilder_ = null;
}
return this;
}
/**
*
* The new account which will be automatically charged to renew the token's expiration, at autoRenewPeriod interval.
*
*
* .proto.AccountID autoRenewAccount = 10;
*/
public com.hederahashgraph.api.proto.java.AccountID.Builder getAutoRenewAccountBuilder() {
onChanged();
return getAutoRenewAccountFieldBuilder().getBuilder();
}
/**
*
* The new account which will be automatically charged to renew the token's expiration, at autoRenewPeriod interval.
*
*
* .proto.AccountID autoRenewAccount = 10;
*/
public com.hederahashgraph.api.proto.java.AccountIDOrBuilder getAutoRenewAccountOrBuilder() {
if (autoRenewAccountBuilder_ != null) {
return autoRenewAccountBuilder_.getMessageOrBuilder();
} else {
return autoRenewAccount_ == null ?
com.hederahashgraph.api.proto.java.AccountID.getDefaultInstance() : autoRenewAccount_;
}
}
/**
*
* The new account which will be automatically charged to renew the token's expiration, at autoRenewPeriod interval.
*
*
* .proto.AccountID autoRenewAccount = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.AccountID, com.hederahashgraph.api.proto.java.AccountID.Builder, com.hederahashgraph.api.proto.java.AccountIDOrBuilder>
getAutoRenewAccountFieldBuilder() {
if (autoRenewAccountBuilder_ == null) {
autoRenewAccountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.AccountID, com.hederahashgraph.api.proto.java.AccountID.Builder, com.hederahashgraph.api.proto.java.AccountIDOrBuilder>(
getAutoRenewAccount(),
getParentForChildren(),
isClean());
autoRenewAccount_ = null;
}
return autoRenewAccountBuilder_;
}
private com.hederahashgraph.api.proto.java.Duration autoRenewPeriod_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Duration, com.hederahashgraph.api.proto.java.Duration.Builder, com.hederahashgraph.api.proto.java.DurationOrBuilder> autoRenewPeriodBuilder_;
/**
*
* The new interval at which the auto-renew account will be charged to extend the token's expiry.
*
*
* .proto.Duration autoRenewPeriod = 11;
*/
public boolean hasAutoRenewPeriod() {
return autoRenewPeriodBuilder_ != null || autoRenewPeriod_ != null;
}
/**
*
* The new interval at which the auto-renew account will be charged to extend the token's expiry.
*
*
* .proto.Duration autoRenewPeriod = 11;
*/
public com.hederahashgraph.api.proto.java.Duration getAutoRenewPeriod() {
if (autoRenewPeriodBuilder_ == null) {
return autoRenewPeriod_ == null ? com.hederahashgraph.api.proto.java.Duration.getDefaultInstance() : autoRenewPeriod_;
} else {
return autoRenewPeriodBuilder_.getMessage();
}
}
/**
*
* The new interval at which the auto-renew account will be charged to extend the token's expiry.
*
*
* .proto.Duration autoRenewPeriod = 11;
*/
public Builder setAutoRenewPeriod(com.hederahashgraph.api.proto.java.Duration value) {
if (autoRenewPeriodBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
autoRenewPeriod_ = value;
onChanged();
} else {
autoRenewPeriodBuilder_.setMessage(value);
}
return this;
}
/**
*
* The new interval at which the auto-renew account will be charged to extend the token's expiry.
*
*
* .proto.Duration autoRenewPeriod = 11;
*/
public Builder setAutoRenewPeriod(
com.hederahashgraph.api.proto.java.Duration.Builder builderForValue) {
if (autoRenewPeriodBuilder_ == null) {
autoRenewPeriod_ = builderForValue.build();
onChanged();
} else {
autoRenewPeriodBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The new interval at which the auto-renew account will be charged to extend the token's expiry.
*
*
* .proto.Duration autoRenewPeriod = 11;
*/
public Builder mergeAutoRenewPeriod(com.hederahashgraph.api.proto.java.Duration value) {
if (autoRenewPeriodBuilder_ == null) {
if (autoRenewPeriod_ != null) {
autoRenewPeriod_ =
com.hederahashgraph.api.proto.java.Duration.newBuilder(autoRenewPeriod_).mergeFrom(value).buildPartial();
} else {
autoRenewPeriod_ = value;
}
onChanged();
} else {
autoRenewPeriodBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The new interval at which the auto-renew account will be charged to extend the token's expiry.
*
*
* .proto.Duration autoRenewPeriod = 11;
*/
public Builder clearAutoRenewPeriod() {
if (autoRenewPeriodBuilder_ == null) {
autoRenewPeriod_ = null;
onChanged();
} else {
autoRenewPeriod_ = null;
autoRenewPeriodBuilder_ = null;
}
return this;
}
/**
*
* The new interval at which the auto-renew account will be charged to extend the token's expiry.
*
*
* .proto.Duration autoRenewPeriod = 11;
*/
public com.hederahashgraph.api.proto.java.Duration.Builder getAutoRenewPeriodBuilder() {
onChanged();
return getAutoRenewPeriodFieldBuilder().getBuilder();
}
/**
*
* The new interval at which the auto-renew account will be charged to extend the token's expiry.
*
*
* .proto.Duration autoRenewPeriod = 11;
*/
public com.hederahashgraph.api.proto.java.DurationOrBuilder getAutoRenewPeriodOrBuilder() {
if (autoRenewPeriodBuilder_ != null) {
return autoRenewPeriodBuilder_.getMessageOrBuilder();
} else {
return autoRenewPeriod_ == null ?
com.hederahashgraph.api.proto.java.Duration.getDefaultInstance() : autoRenewPeriod_;
}
}
/**
*
* The new interval at which the auto-renew account will be charged to extend the token's expiry.
*
*
* .proto.Duration autoRenewPeriod = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Duration, com.hederahashgraph.api.proto.java.Duration.Builder, com.hederahashgraph.api.proto.java.DurationOrBuilder>
getAutoRenewPeriodFieldBuilder() {
if (autoRenewPeriodBuilder_ == null) {
autoRenewPeriodBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Duration, com.hederahashgraph.api.proto.java.Duration.Builder, com.hederahashgraph.api.proto.java.DurationOrBuilder>(
getAutoRenewPeriod(),
getParentForChildren(),
isClean());
autoRenewPeriod_ = null;
}
return autoRenewPeriodBuilder_;
}
private com.hederahashgraph.api.proto.java.Timestamp expiry_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Timestamp, com.hederahashgraph.api.proto.java.Timestamp.Builder, com.hederahashgraph.api.proto.java.TimestampOrBuilder> expiryBuilder_;
/**
*
* The new expiry time of the token. Expiry can be updated even if admin key is not set. If the provided expiry is earlier than the current token expiry, transaction wil resolve to INVALID_EXPIRATION_TIME
*
*
* .proto.Timestamp expiry = 12;
*/
public boolean hasExpiry() {
return expiryBuilder_ != null || expiry_ != null;
}
/**
*
* The new expiry time of the token. Expiry can be updated even if admin key is not set. If the provided expiry is earlier than the current token expiry, transaction wil resolve to INVALID_EXPIRATION_TIME
*
*
* .proto.Timestamp expiry = 12;
*/
public com.hederahashgraph.api.proto.java.Timestamp getExpiry() {
if (expiryBuilder_ == null) {
return expiry_ == null ? com.hederahashgraph.api.proto.java.Timestamp.getDefaultInstance() : expiry_;
} else {
return expiryBuilder_.getMessage();
}
}
/**
*
* The new expiry time of the token. Expiry can be updated even if admin key is not set. If the provided expiry is earlier than the current token expiry, transaction wil resolve to INVALID_EXPIRATION_TIME
*
*
* .proto.Timestamp expiry = 12;
*/
public Builder setExpiry(com.hederahashgraph.api.proto.java.Timestamp value) {
if (expiryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
expiry_ = value;
onChanged();
} else {
expiryBuilder_.setMessage(value);
}
return this;
}
/**
*
* The new expiry time of the token. Expiry can be updated even if admin key is not set. If the provided expiry is earlier than the current token expiry, transaction wil resolve to INVALID_EXPIRATION_TIME
*
*
* .proto.Timestamp expiry = 12;
*/
public Builder setExpiry(
com.hederahashgraph.api.proto.java.Timestamp.Builder builderForValue) {
if (expiryBuilder_ == null) {
expiry_ = builderForValue.build();
onChanged();
} else {
expiryBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The new expiry time of the token. Expiry can be updated even if admin key is not set. If the provided expiry is earlier than the current token expiry, transaction wil resolve to INVALID_EXPIRATION_TIME
*
*
* .proto.Timestamp expiry = 12;
*/
public Builder mergeExpiry(com.hederahashgraph.api.proto.java.Timestamp value) {
if (expiryBuilder_ == null) {
if (expiry_ != null) {
expiry_ =
com.hederahashgraph.api.proto.java.Timestamp.newBuilder(expiry_).mergeFrom(value).buildPartial();
} else {
expiry_ = value;
}
onChanged();
} else {
expiryBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The new expiry time of the token. Expiry can be updated even if admin key is not set. If the provided expiry is earlier than the current token expiry, transaction wil resolve to INVALID_EXPIRATION_TIME
*
*
* .proto.Timestamp expiry = 12;
*/
public Builder clearExpiry() {
if (expiryBuilder_ == null) {
expiry_ = null;
onChanged();
} else {
expiry_ = null;
expiryBuilder_ = null;
}
return this;
}
/**
*
* The new expiry time of the token. Expiry can be updated even if admin key is not set. If the provided expiry is earlier than the current token expiry, transaction wil resolve to INVALID_EXPIRATION_TIME
*
*
* .proto.Timestamp expiry = 12;
*/
public com.hederahashgraph.api.proto.java.Timestamp.Builder getExpiryBuilder() {
onChanged();
return getExpiryFieldBuilder().getBuilder();
}
/**
*
* The new expiry time of the token. Expiry can be updated even if admin key is not set. If the provided expiry is earlier than the current token expiry, transaction wil resolve to INVALID_EXPIRATION_TIME
*
*
* .proto.Timestamp expiry = 12;
*/
public com.hederahashgraph.api.proto.java.TimestampOrBuilder getExpiryOrBuilder() {
if (expiryBuilder_ != null) {
return expiryBuilder_.getMessageOrBuilder();
} else {
return expiry_ == null ?
com.hederahashgraph.api.proto.java.Timestamp.getDefaultInstance() : expiry_;
}
}
/**
*
* The new expiry time of the token. Expiry can be updated even if admin key is not set. If the provided expiry is earlier than the current token expiry, transaction wil resolve to INVALID_EXPIRATION_TIME
*
*
* .proto.Timestamp expiry = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Timestamp, com.hederahashgraph.api.proto.java.Timestamp.Builder, com.hederahashgraph.api.proto.java.TimestampOrBuilder>
getExpiryFieldBuilder() {
if (expiryBuilder_ == null) {
expiryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.Timestamp, com.hederahashgraph.api.proto.java.Timestamp.Builder, com.hederahashgraph.api.proto.java.TimestampOrBuilder>(
getExpiry(),
getParentForChildren(),
isClean());
expiry_ = null;
}
return expiryBuilder_;
}
private com.google.protobuf.StringValue memo_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> memoBuilder_;
/**
*
* If set, the new memo to be associated with the token (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 13;
*/
public boolean hasMemo() {
return memoBuilder_ != null || memo_ != null;
}
/**
*
* If set, the new memo to be associated with the token (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 13;
*/
public com.google.protobuf.StringValue getMemo() {
if (memoBuilder_ == null) {
return memo_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : memo_;
} else {
return memoBuilder_.getMessage();
}
}
/**
*
* If set, the new memo to be associated with the token (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 13;
*/
public Builder setMemo(com.google.protobuf.StringValue value) {
if (memoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
memo_ = value;
onChanged();
} else {
memoBuilder_.setMessage(value);
}
return this;
}
/**
*
* If set, the new memo to be associated with the token (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 13;
*/
public Builder setMemo(
com.google.protobuf.StringValue.Builder builderForValue) {
if (memoBuilder_ == null) {
memo_ = builderForValue.build();
onChanged();
} else {
memoBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* If set, the new memo to be associated with the token (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 13;
*/
public Builder mergeMemo(com.google.protobuf.StringValue value) {
if (memoBuilder_ == null) {
if (memo_ != null) {
memo_ =
com.google.protobuf.StringValue.newBuilder(memo_).mergeFrom(value).buildPartial();
} else {
memo_ = value;
}
onChanged();
} else {
memoBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* If set, the new memo to be associated with the token (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 13;
*/
public Builder clearMemo() {
if (memoBuilder_ == null) {
memo_ = null;
onChanged();
} else {
memo_ = null;
memoBuilder_ = null;
}
return this;
}
/**
*
* If set, the new memo to be associated with the token (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 13;
*/
public com.google.protobuf.StringValue.Builder getMemoBuilder() {
onChanged();
return getMemoFieldBuilder().getBuilder();
}
/**
*
* If set, the new memo to be associated with the token (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 13;
*/
public com.google.protobuf.StringValueOrBuilder getMemoOrBuilder() {
if (memoBuilder_ != null) {
return memoBuilder_.getMessageOrBuilder();
} else {
return memo_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : memo_;
}
}
/**
*
* If set, the new memo to be associated with the token (UTF-8 encoding max 100 bytes)
*
*
* .google.protobuf.StringValue memo = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getMemoFieldBuilder() {
if (memoBuilder_ == null) {
memoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getMemo(),
getParentForChildren(),
isClean());
memo_ = null;
}
return memoBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:proto.TokenUpdateTransactionBody)
}
// @@protoc_insertion_point(class_scope:proto.TokenUpdateTransactionBody)
private static final com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody();
}
public static com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TokenUpdateTransactionBody parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TokenUpdateTransactionBody(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.TokenUpdateTransactionBody getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy