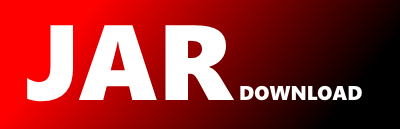
com.hederahashgraph.api.proto.java.TransactionGetRecordQuery Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: TransactionGetRecord.proto
package com.hederahashgraph.api.proto.java;
/**
*
* Get the record for a transaction. If the transaction requested a record, then the record lasts for one hour, and a state proof is available for it. If the transaction created an account, file, or smart contract instance, then the record will contain the ID for what it created. If the transaction called a smart contract function, then the record contains the result of that call. If the transaction was a cryptocurrency transfer, then the record includes the TransferList which gives the details of that transfer. If the transaction didn't return anything that should be in the record, then the results field will be set to nothing.
*
*
* Protobuf type {@code proto.TransactionGetRecordQuery}
*/
public final class TransactionGetRecordQuery extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:proto.TransactionGetRecordQuery)
TransactionGetRecordQueryOrBuilder {
private static final long serialVersionUID = 0L;
// Use TransactionGetRecordQuery.newBuilder() to construct.
private TransactionGetRecordQuery(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TransactionGetRecordQuery() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TransactionGetRecordQuery();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TransactionGetRecordQuery(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.hederahashgraph.api.proto.java.QueryHeader.Builder subBuilder = null;
if (header_ != null) {
subBuilder = header_.toBuilder();
}
header_ = input.readMessage(com.hederahashgraph.api.proto.java.QueryHeader.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(header_);
header_ = subBuilder.buildPartial();
}
break;
}
case 18: {
com.hederahashgraph.api.proto.java.TransactionID.Builder subBuilder = null;
if (transactionID_ != null) {
subBuilder = transactionID_.toBuilder();
}
transactionID_ = input.readMessage(com.hederahashgraph.api.proto.java.TransactionID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(transactionID_);
transactionID_ = subBuilder.buildPartial();
}
break;
}
case 24: {
includeDuplicates_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.hederahashgraph.api.proto.java.TransactionGetRecord.internal_static_proto_TransactionGetRecordQuery_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.hederahashgraph.api.proto.java.TransactionGetRecord.internal_static_proto_TransactionGetRecordQuery_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.hederahashgraph.api.proto.java.TransactionGetRecordQuery.class, com.hederahashgraph.api.proto.java.TransactionGetRecordQuery.Builder.class);
}
public static final int HEADER_FIELD_NUMBER = 1;
private com.hederahashgraph.api.proto.java.QueryHeader header_;
/**
*
* Standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither).
*
*
* .proto.QueryHeader header = 1;
*/
public boolean hasHeader() {
return header_ != null;
}
/**
*
* Standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither).
*
*
* .proto.QueryHeader header = 1;
*/
public com.hederahashgraph.api.proto.java.QueryHeader getHeader() {
return header_ == null ? com.hederahashgraph.api.proto.java.QueryHeader.getDefaultInstance() : header_;
}
/**
*
* Standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither).
*
*
* .proto.QueryHeader header = 1;
*/
public com.hederahashgraph.api.proto.java.QueryHeaderOrBuilder getHeaderOrBuilder() {
return getHeader();
}
public static final int TRANSACTIONID_FIELD_NUMBER = 2;
private com.hederahashgraph.api.proto.java.TransactionID transactionID_;
/**
*
* The ID of the transaction for which the record is requested.
*
*
* .proto.TransactionID transactionID = 2;
*/
public boolean hasTransactionID() {
return transactionID_ != null;
}
/**
*
* The ID of the transaction for which the record is requested.
*
*
* .proto.TransactionID transactionID = 2;
*/
public com.hederahashgraph.api.proto.java.TransactionID getTransactionID() {
return transactionID_ == null ? com.hederahashgraph.api.proto.java.TransactionID.getDefaultInstance() : transactionID_;
}
/**
*
* The ID of the transaction for which the record is requested.
*
*
* .proto.TransactionID transactionID = 2;
*/
public com.hederahashgraph.api.proto.java.TransactionIDOrBuilder getTransactionIDOrBuilder() {
return getTransactionID();
}
public static final int INCLUDEDUPLICATES_FIELD_NUMBER = 3;
private boolean includeDuplicates_;
/**
*
* Whether records of processing duplicate transactions should be returned along with the record of processing the first consensus transaction with the given id whose status was neither <tt>INVALID_NODE_ACCOUNT</tt> nor <tt>INVALID_PAYER_SIGNATURE</tt>; <b>or</b>, if no such record exists, the record of processing the first transaction to reach consensus with the given transaction id..
*
*
* bool includeDuplicates = 3;
*/
public boolean getIncludeDuplicates() {
return includeDuplicates_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (header_ != null) {
output.writeMessage(1, getHeader());
}
if (transactionID_ != null) {
output.writeMessage(2, getTransactionID());
}
if (includeDuplicates_ != false) {
output.writeBool(3, includeDuplicates_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (header_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getHeader());
}
if (transactionID_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getTransactionID());
}
if (includeDuplicates_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, includeDuplicates_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.hederahashgraph.api.proto.java.TransactionGetRecordQuery)) {
return super.equals(obj);
}
com.hederahashgraph.api.proto.java.TransactionGetRecordQuery other = (com.hederahashgraph.api.proto.java.TransactionGetRecordQuery) obj;
if (hasHeader() != other.hasHeader()) return false;
if (hasHeader()) {
if (!getHeader()
.equals(other.getHeader())) return false;
}
if (hasTransactionID() != other.hasTransactionID()) return false;
if (hasTransactionID()) {
if (!getTransactionID()
.equals(other.getTransactionID())) return false;
}
if (getIncludeDuplicates()
!= other.getIncludeDuplicates()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasHeader()) {
hash = (37 * hash) + HEADER_FIELD_NUMBER;
hash = (53 * hash) + getHeader().hashCode();
}
if (hasTransactionID()) {
hash = (37 * hash) + TRANSACTIONID_FIELD_NUMBER;
hash = (53 * hash) + getTransactionID().hashCode();
}
hash = (37 * hash) + INCLUDEDUPLICATES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIncludeDuplicates());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.hederahashgraph.api.proto.java.TransactionGetRecordQuery parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.TransactionGetRecordQuery parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.TransactionGetRecordQuery parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.TransactionGetRecordQuery parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.TransactionGetRecordQuery parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.TransactionGetRecordQuery parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.TransactionGetRecordQuery parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.TransactionGetRecordQuery parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.TransactionGetRecordQuery parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.TransactionGetRecordQuery parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.TransactionGetRecordQuery parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.TransactionGetRecordQuery parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.hederahashgraph.api.proto.java.TransactionGetRecordQuery prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Get the record for a transaction. If the transaction requested a record, then the record lasts for one hour, and a state proof is available for it. If the transaction created an account, file, or smart contract instance, then the record will contain the ID for what it created. If the transaction called a smart contract function, then the record contains the result of that call. If the transaction was a cryptocurrency transfer, then the record includes the TransferList which gives the details of that transfer. If the transaction didn't return anything that should be in the record, then the results field will be set to nothing.
*
*
* Protobuf type {@code proto.TransactionGetRecordQuery}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:proto.TransactionGetRecordQuery)
com.hederahashgraph.api.proto.java.TransactionGetRecordQueryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.hederahashgraph.api.proto.java.TransactionGetRecord.internal_static_proto_TransactionGetRecordQuery_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.hederahashgraph.api.proto.java.TransactionGetRecord.internal_static_proto_TransactionGetRecordQuery_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.hederahashgraph.api.proto.java.TransactionGetRecordQuery.class, com.hederahashgraph.api.proto.java.TransactionGetRecordQuery.Builder.class);
}
// Construct using com.hederahashgraph.api.proto.java.TransactionGetRecordQuery.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (headerBuilder_ == null) {
header_ = null;
} else {
header_ = null;
headerBuilder_ = null;
}
if (transactionIDBuilder_ == null) {
transactionID_ = null;
} else {
transactionID_ = null;
transactionIDBuilder_ = null;
}
includeDuplicates_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.hederahashgraph.api.proto.java.TransactionGetRecord.internal_static_proto_TransactionGetRecordQuery_descriptor;
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.TransactionGetRecordQuery getDefaultInstanceForType() {
return com.hederahashgraph.api.proto.java.TransactionGetRecordQuery.getDefaultInstance();
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.TransactionGetRecordQuery build() {
com.hederahashgraph.api.proto.java.TransactionGetRecordQuery result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.TransactionGetRecordQuery buildPartial() {
com.hederahashgraph.api.proto.java.TransactionGetRecordQuery result = new com.hederahashgraph.api.proto.java.TransactionGetRecordQuery(this);
if (headerBuilder_ == null) {
result.header_ = header_;
} else {
result.header_ = headerBuilder_.build();
}
if (transactionIDBuilder_ == null) {
result.transactionID_ = transactionID_;
} else {
result.transactionID_ = transactionIDBuilder_.build();
}
result.includeDuplicates_ = includeDuplicates_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.hederahashgraph.api.proto.java.TransactionGetRecordQuery) {
return mergeFrom((com.hederahashgraph.api.proto.java.TransactionGetRecordQuery)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.hederahashgraph.api.proto.java.TransactionGetRecordQuery other) {
if (other == com.hederahashgraph.api.proto.java.TransactionGetRecordQuery.getDefaultInstance()) return this;
if (other.hasHeader()) {
mergeHeader(other.getHeader());
}
if (other.hasTransactionID()) {
mergeTransactionID(other.getTransactionID());
}
if (other.getIncludeDuplicates() != false) {
setIncludeDuplicates(other.getIncludeDuplicates());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.hederahashgraph.api.proto.java.TransactionGetRecordQuery parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.hederahashgraph.api.proto.java.TransactionGetRecordQuery) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.hederahashgraph.api.proto.java.QueryHeader header_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.QueryHeader, com.hederahashgraph.api.proto.java.QueryHeader.Builder, com.hederahashgraph.api.proto.java.QueryHeaderOrBuilder> headerBuilder_;
/**
*
* Standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither).
*
*
* .proto.QueryHeader header = 1;
*/
public boolean hasHeader() {
return headerBuilder_ != null || header_ != null;
}
/**
*
* Standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither).
*
*
* .proto.QueryHeader header = 1;
*/
public com.hederahashgraph.api.proto.java.QueryHeader getHeader() {
if (headerBuilder_ == null) {
return header_ == null ? com.hederahashgraph.api.proto.java.QueryHeader.getDefaultInstance() : header_;
} else {
return headerBuilder_.getMessage();
}
}
/**
*
* Standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither).
*
*
* .proto.QueryHeader header = 1;
*/
public Builder setHeader(com.hederahashgraph.api.proto.java.QueryHeader value) {
if (headerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
header_ = value;
onChanged();
} else {
headerBuilder_.setMessage(value);
}
return this;
}
/**
*
* Standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither).
*
*
* .proto.QueryHeader header = 1;
*/
public Builder setHeader(
com.hederahashgraph.api.proto.java.QueryHeader.Builder builderForValue) {
if (headerBuilder_ == null) {
header_ = builderForValue.build();
onChanged();
} else {
headerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither).
*
*
* .proto.QueryHeader header = 1;
*/
public Builder mergeHeader(com.hederahashgraph.api.proto.java.QueryHeader value) {
if (headerBuilder_ == null) {
if (header_ != null) {
header_ =
com.hederahashgraph.api.proto.java.QueryHeader.newBuilder(header_).mergeFrom(value).buildPartial();
} else {
header_ = value;
}
onChanged();
} else {
headerBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither).
*
*
* .proto.QueryHeader header = 1;
*/
public Builder clearHeader() {
if (headerBuilder_ == null) {
header_ = null;
onChanged();
} else {
header_ = null;
headerBuilder_ = null;
}
return this;
}
/**
*
* Standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither).
*
*
* .proto.QueryHeader header = 1;
*/
public com.hederahashgraph.api.proto.java.QueryHeader.Builder getHeaderBuilder() {
onChanged();
return getHeaderFieldBuilder().getBuilder();
}
/**
*
* Standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither).
*
*
* .proto.QueryHeader header = 1;
*/
public com.hederahashgraph.api.proto.java.QueryHeaderOrBuilder getHeaderOrBuilder() {
if (headerBuilder_ != null) {
return headerBuilder_.getMessageOrBuilder();
} else {
return header_ == null ?
com.hederahashgraph.api.proto.java.QueryHeader.getDefaultInstance() : header_;
}
}
/**
*
* Standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither).
*
*
* .proto.QueryHeader header = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.QueryHeader, com.hederahashgraph.api.proto.java.QueryHeader.Builder, com.hederahashgraph.api.proto.java.QueryHeaderOrBuilder>
getHeaderFieldBuilder() {
if (headerBuilder_ == null) {
headerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.QueryHeader, com.hederahashgraph.api.proto.java.QueryHeader.Builder, com.hederahashgraph.api.proto.java.QueryHeaderOrBuilder>(
getHeader(),
getParentForChildren(),
isClean());
header_ = null;
}
return headerBuilder_;
}
private com.hederahashgraph.api.proto.java.TransactionID transactionID_;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.TransactionID, com.hederahashgraph.api.proto.java.TransactionID.Builder, com.hederahashgraph.api.proto.java.TransactionIDOrBuilder> transactionIDBuilder_;
/**
*
* The ID of the transaction for which the record is requested.
*
*
* .proto.TransactionID transactionID = 2;
*/
public boolean hasTransactionID() {
return transactionIDBuilder_ != null || transactionID_ != null;
}
/**
*
* The ID of the transaction for which the record is requested.
*
*
* .proto.TransactionID transactionID = 2;
*/
public com.hederahashgraph.api.proto.java.TransactionID getTransactionID() {
if (transactionIDBuilder_ == null) {
return transactionID_ == null ? com.hederahashgraph.api.proto.java.TransactionID.getDefaultInstance() : transactionID_;
} else {
return transactionIDBuilder_.getMessage();
}
}
/**
*
* The ID of the transaction for which the record is requested.
*
*
* .proto.TransactionID transactionID = 2;
*/
public Builder setTransactionID(com.hederahashgraph.api.proto.java.TransactionID value) {
if (transactionIDBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
transactionID_ = value;
onChanged();
} else {
transactionIDBuilder_.setMessage(value);
}
return this;
}
/**
*
* The ID of the transaction for which the record is requested.
*
*
* .proto.TransactionID transactionID = 2;
*/
public Builder setTransactionID(
com.hederahashgraph.api.proto.java.TransactionID.Builder builderForValue) {
if (transactionIDBuilder_ == null) {
transactionID_ = builderForValue.build();
onChanged();
} else {
transactionIDBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The ID of the transaction for which the record is requested.
*
*
* .proto.TransactionID transactionID = 2;
*/
public Builder mergeTransactionID(com.hederahashgraph.api.proto.java.TransactionID value) {
if (transactionIDBuilder_ == null) {
if (transactionID_ != null) {
transactionID_ =
com.hederahashgraph.api.proto.java.TransactionID.newBuilder(transactionID_).mergeFrom(value).buildPartial();
} else {
transactionID_ = value;
}
onChanged();
} else {
transactionIDBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The ID of the transaction for which the record is requested.
*
*
* .proto.TransactionID transactionID = 2;
*/
public Builder clearTransactionID() {
if (transactionIDBuilder_ == null) {
transactionID_ = null;
onChanged();
} else {
transactionID_ = null;
transactionIDBuilder_ = null;
}
return this;
}
/**
*
* The ID of the transaction for which the record is requested.
*
*
* .proto.TransactionID transactionID = 2;
*/
public com.hederahashgraph.api.proto.java.TransactionID.Builder getTransactionIDBuilder() {
onChanged();
return getTransactionIDFieldBuilder().getBuilder();
}
/**
*
* The ID of the transaction for which the record is requested.
*
*
* .proto.TransactionID transactionID = 2;
*/
public com.hederahashgraph.api.proto.java.TransactionIDOrBuilder getTransactionIDOrBuilder() {
if (transactionIDBuilder_ != null) {
return transactionIDBuilder_.getMessageOrBuilder();
} else {
return transactionID_ == null ?
com.hederahashgraph.api.proto.java.TransactionID.getDefaultInstance() : transactionID_;
}
}
/**
*
* The ID of the transaction for which the record is requested.
*
*
* .proto.TransactionID transactionID = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.TransactionID, com.hederahashgraph.api.proto.java.TransactionID.Builder, com.hederahashgraph.api.proto.java.TransactionIDOrBuilder>
getTransactionIDFieldBuilder() {
if (transactionIDBuilder_ == null) {
transactionIDBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.TransactionID, com.hederahashgraph.api.proto.java.TransactionID.Builder, com.hederahashgraph.api.proto.java.TransactionIDOrBuilder>(
getTransactionID(),
getParentForChildren(),
isClean());
transactionID_ = null;
}
return transactionIDBuilder_;
}
private boolean includeDuplicates_ ;
/**
*
* Whether records of processing duplicate transactions should be returned along with the record of processing the first consensus transaction with the given id whose status was neither <tt>INVALID_NODE_ACCOUNT</tt> nor <tt>INVALID_PAYER_SIGNATURE</tt>; <b>or</b>, if no such record exists, the record of processing the first transaction to reach consensus with the given transaction id..
*
*
* bool includeDuplicates = 3;
*/
public boolean getIncludeDuplicates() {
return includeDuplicates_;
}
/**
*
* Whether records of processing duplicate transactions should be returned along with the record of processing the first consensus transaction with the given id whose status was neither <tt>INVALID_NODE_ACCOUNT</tt> nor <tt>INVALID_PAYER_SIGNATURE</tt>; <b>or</b>, if no such record exists, the record of processing the first transaction to reach consensus with the given transaction id..
*
*
* bool includeDuplicates = 3;
*/
public Builder setIncludeDuplicates(boolean value) {
includeDuplicates_ = value;
onChanged();
return this;
}
/**
*
* Whether records of processing duplicate transactions should be returned along with the record of processing the first consensus transaction with the given id whose status was neither <tt>INVALID_NODE_ACCOUNT</tt> nor <tt>INVALID_PAYER_SIGNATURE</tt>; <b>or</b>, if no such record exists, the record of processing the first transaction to reach consensus with the given transaction id..
*
*
* bool includeDuplicates = 3;
*/
public Builder clearIncludeDuplicates() {
includeDuplicates_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:proto.TransactionGetRecordQuery)
}
// @@protoc_insertion_point(class_scope:proto.TransactionGetRecordQuery)
private static final com.hederahashgraph.api.proto.java.TransactionGetRecordQuery DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.hederahashgraph.api.proto.java.TransactionGetRecordQuery();
}
public static com.hederahashgraph.api.proto.java.TransactionGetRecordQuery getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TransactionGetRecordQuery parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TransactionGetRecordQuery(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.hederahashgraph.api.proto.java.TransactionGetRecordQuery getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy