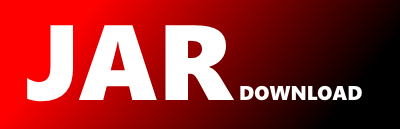
com.hedera.hapi.block.stream.output.protoc.StateChange Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: stream/output/state_changes.proto
package com.hedera.hapi.block.stream.output.protoc;
/**
*
**
* A change to any item in the merkle tree.
* A State change SHALL represent one mutation of the network state merkle
* tree. The state changes published in the block stream MAY be combined
* into an ordered set of state mutations that transform the tree from any
* initial state to a destination state.<br/>
* When the full set of state change items from the block stream for a round
* is applied to the network state at the start of that round the result
* SHALL match the network state at the end of the round.
* TODO: Need documentation for how the merkle tree is constructed.
* Need to reference that document, stored in platform docs?, here.
*
*
* Protobuf type {@code com.hedera.hapi.block.stream.output.StateChange}
*/
public final class StateChange extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.hedera.hapi.block.stream.output.StateChange)
StateChangeOrBuilder {
private static final long serialVersionUID = 0L;
// Use StateChange.newBuilder() to construct.
private StateChange(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StateChange() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StateChange();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StateChange(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
stateId_ = input.readUInt32();
break;
}
case 18: {
com.hedera.hapi.block.stream.output.protoc.NewStateChange.Builder subBuilder = null;
if (changeOperationCase_ == 2) {
subBuilder = ((com.hedera.hapi.block.stream.output.protoc.NewStateChange) changeOperation_).toBuilder();
}
changeOperation_ =
input.readMessage(com.hedera.hapi.block.stream.output.protoc.NewStateChange.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.hedera.hapi.block.stream.output.protoc.NewStateChange) changeOperation_);
changeOperation_ = subBuilder.buildPartial();
}
changeOperationCase_ = 2;
break;
}
case 26: {
com.hedera.hapi.block.stream.output.protoc.RemovedStateChange.Builder subBuilder = null;
if (changeOperationCase_ == 3) {
subBuilder = ((com.hedera.hapi.block.stream.output.protoc.RemovedStateChange) changeOperation_).toBuilder();
}
changeOperation_ =
input.readMessage(com.hedera.hapi.block.stream.output.protoc.RemovedStateChange.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.hedera.hapi.block.stream.output.protoc.RemovedStateChange) changeOperation_);
changeOperation_ = subBuilder.buildPartial();
}
changeOperationCase_ = 3;
break;
}
case 34: {
com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange.Builder subBuilder = null;
if (changeOperationCase_ == 4) {
subBuilder = ((com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange) changeOperation_).toBuilder();
}
changeOperation_ =
input.readMessage(com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange) changeOperation_);
changeOperation_ = subBuilder.buildPartial();
}
changeOperationCase_ = 4;
break;
}
case 42: {
com.hedera.hapi.block.stream.output.protoc.MapUpdateChange.Builder subBuilder = null;
if (changeOperationCase_ == 5) {
subBuilder = ((com.hedera.hapi.block.stream.output.protoc.MapUpdateChange) changeOperation_).toBuilder();
}
changeOperation_ =
input.readMessage(com.hedera.hapi.block.stream.output.protoc.MapUpdateChange.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.hedera.hapi.block.stream.output.protoc.MapUpdateChange) changeOperation_);
changeOperation_ = subBuilder.buildPartial();
}
changeOperationCase_ = 5;
break;
}
case 50: {
com.hedera.hapi.block.stream.output.protoc.MapDeleteChange.Builder subBuilder = null;
if (changeOperationCase_ == 6) {
subBuilder = ((com.hedera.hapi.block.stream.output.protoc.MapDeleteChange) changeOperation_).toBuilder();
}
changeOperation_ =
input.readMessage(com.hedera.hapi.block.stream.output.protoc.MapDeleteChange.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.hedera.hapi.block.stream.output.protoc.MapDeleteChange) changeOperation_);
changeOperation_ = subBuilder.buildPartial();
}
changeOperationCase_ = 6;
break;
}
case 58: {
com.hedera.hapi.block.stream.output.protoc.QueuePushChange.Builder subBuilder = null;
if (changeOperationCase_ == 7) {
subBuilder = ((com.hedera.hapi.block.stream.output.protoc.QueuePushChange) changeOperation_).toBuilder();
}
changeOperation_ =
input.readMessage(com.hedera.hapi.block.stream.output.protoc.QueuePushChange.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.hedera.hapi.block.stream.output.protoc.QueuePushChange) changeOperation_);
changeOperation_ = subBuilder.buildPartial();
}
changeOperationCase_ = 7;
break;
}
case 66: {
com.hedera.hapi.block.stream.output.protoc.QueuePopChange.Builder subBuilder = null;
if (changeOperationCase_ == 8) {
subBuilder = ((com.hedera.hapi.block.stream.output.protoc.QueuePopChange) changeOperation_).toBuilder();
}
changeOperation_ =
input.readMessage(com.hedera.hapi.block.stream.output.protoc.QueuePopChange.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.hedera.hapi.block.stream.output.protoc.QueuePopChange) changeOperation_);
changeOperation_ = subBuilder.buildPartial();
}
changeOperationCase_ = 8;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.hedera.hapi.block.stream.output.protoc.StateChangesOuterClass.internal_static_com_hedera_hapi_block_stream_output_StateChange_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.hedera.hapi.block.stream.output.protoc.StateChangesOuterClass.internal_static_com_hedera_hapi_block_stream_output_StateChange_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.hedera.hapi.block.stream.output.protoc.StateChange.class, com.hedera.hapi.block.stream.output.protoc.StateChange.Builder.class);
}
private int changeOperationCase_ = 0;
private java.lang.Object changeOperation_;
public enum ChangeOperationCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
STATE_ADD(2),
STATE_REMOVE(3),
SINGLETON_UPDATE(4),
MAP_UPDATE(5),
MAP_DELETE(6),
QUEUE_PUSH(7),
QUEUE_POP(8),
CHANGEOPERATION_NOT_SET(0);
private final int value;
private ChangeOperationCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ChangeOperationCase valueOf(int value) {
return forNumber(value);
}
public static ChangeOperationCase forNumber(int value) {
switch (value) {
case 2: return STATE_ADD;
case 3: return STATE_REMOVE;
case 4: return SINGLETON_UPDATE;
case 5: return MAP_UPDATE;
case 6: return MAP_DELETE;
case 7: return QUEUE_PUSH;
case 8: return QUEUE_POP;
case 0: return CHANGEOPERATION_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ChangeOperationCase
getChangeOperationCase() {
return ChangeOperationCase.forNumber(
changeOperationCase_);
}
public static final int STATE_ID_FIELD_NUMBER = 1;
private int stateId_;
/**
*
**
* A state identifier.<br/>
* The reason we use an integer field here, and not an enum field is to
* better support forward compatibility. There will be many cases when a
* block node or other client (such as a dApp) with HAPI version N wants
* to process blocks from HAPI version N+1, for example. If we use a
* protobuf enum then when that is mapped Java or Rust it would not be
* parsed as an enum value because those languages do not support unknown
* enum values. ProtoC has a workaround for this but it is complex and
* requires non-deterministic parsing. Our solution to create an integer
* field and provide an enumeration for mapping that integer is intended
* as an acceptable compromise solution.
* <p>
* This number SHALL identify the merkle subtree "state" to be modified and
* often corresponds to a `VirtualMap` identifier.
* This number SHALL be a valid value for the `StateIdentifier` enum.
*
*
* uint32 state_id = 1;
* @return The stateId.
*/
@java.lang.Override
public int getStateId() {
return stateId_;
}
public static final int STATE_ADD_FIELD_NUMBER = 2;
/**
*
**
* Addition of a new state.<br/>
* This may be a singleton, virtual map, or queue state.
*
*
* .com.hedera.hapi.block.stream.output.NewStateChange state_add = 2;
* @return Whether the stateAdd field is set.
*/
@java.lang.Override
public boolean hasStateAdd() {
return changeOperationCase_ == 2;
}
/**
*
**
* Addition of a new state.<br/>
* This may be a singleton, virtual map, or queue state.
*
*
* .com.hedera.hapi.block.stream.output.NewStateChange state_add = 2;
* @return The stateAdd.
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.NewStateChange getStateAdd() {
if (changeOperationCase_ == 2) {
return (com.hedera.hapi.block.stream.output.protoc.NewStateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.NewStateChange.getDefaultInstance();
}
/**
*
**
* Addition of a new state.<br/>
* This may be a singleton, virtual map, or queue state.
*
*
* .com.hedera.hapi.block.stream.output.NewStateChange state_add = 2;
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.NewStateChangeOrBuilder getStateAddOrBuilder() {
if (changeOperationCase_ == 2) {
return (com.hedera.hapi.block.stream.output.protoc.NewStateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.NewStateChange.getDefaultInstance();
}
public static final int STATE_REMOVE_FIELD_NUMBER = 3;
/**
*
**
* Removal of an existing state.<br/>
* The entire singleton, virtual map, or queue state is removed,
* and not just the contents.
*
*
* .com.hedera.hapi.block.stream.output.RemovedStateChange state_remove = 3;
* @return Whether the stateRemove field is set.
*/
@java.lang.Override
public boolean hasStateRemove() {
return changeOperationCase_ == 3;
}
/**
*
**
* Removal of an existing state.<br/>
* The entire singleton, virtual map, or queue state is removed,
* and not just the contents.
*
*
* .com.hedera.hapi.block.stream.output.RemovedStateChange state_remove = 3;
* @return The stateRemove.
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.RemovedStateChange getStateRemove() {
if (changeOperationCase_ == 3) {
return (com.hedera.hapi.block.stream.output.protoc.RemovedStateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.RemovedStateChange.getDefaultInstance();
}
/**
*
**
* Removal of an existing state.<br/>
* The entire singleton, virtual map, or queue state is removed,
* and not just the contents.
*
*
* .com.hedera.hapi.block.stream.output.RemovedStateChange state_remove = 3;
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.RemovedStateChangeOrBuilder getStateRemoveOrBuilder() {
if (changeOperationCase_ == 3) {
return (com.hedera.hapi.block.stream.output.protoc.RemovedStateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.RemovedStateChange.getDefaultInstance();
}
public static final int SINGLETON_UPDATE_FIELD_NUMBER = 4;
/**
*
**
* An add or update to a `Singleton` state.
*
*
* .com.hedera.hapi.block.stream.output.SingletonUpdateChange singleton_update = 4;
* @return Whether the singletonUpdate field is set.
*/
@java.lang.Override
public boolean hasSingletonUpdate() {
return changeOperationCase_ == 4;
}
/**
*
**
* An add or update to a `Singleton` state.
*
*
* .com.hedera.hapi.block.stream.output.SingletonUpdateChange singleton_update = 4;
* @return The singletonUpdate.
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange getSingletonUpdate() {
if (changeOperationCase_ == 4) {
return (com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange.getDefaultInstance();
}
/**
*
**
* An add or update to a `Singleton` state.
*
*
* .com.hedera.hapi.block.stream.output.SingletonUpdateChange singleton_update = 4;
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChangeOrBuilder getSingletonUpdateOrBuilder() {
if (changeOperationCase_ == 4) {
return (com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange.getDefaultInstance();
}
public static final int MAP_UPDATE_FIELD_NUMBER = 5;
/**
*
**
* An add or update to a single item in a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapUpdateChange map_update = 5;
* @return Whether the mapUpdate field is set.
*/
@java.lang.Override
public boolean hasMapUpdate() {
return changeOperationCase_ == 5;
}
/**
*
**
* An add or update to a single item in a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapUpdateChange map_update = 5;
* @return The mapUpdate.
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.MapUpdateChange getMapUpdate() {
if (changeOperationCase_ == 5) {
return (com.hedera.hapi.block.stream.output.protoc.MapUpdateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.MapUpdateChange.getDefaultInstance();
}
/**
*
**
* An add or update to a single item in a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapUpdateChange map_update = 5;
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.MapUpdateChangeOrBuilder getMapUpdateOrBuilder() {
if (changeOperationCase_ == 5) {
return (com.hedera.hapi.block.stream.output.protoc.MapUpdateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.MapUpdateChange.getDefaultInstance();
}
public static final int MAP_DELETE_FIELD_NUMBER = 6;
/**
*
**
* A removal of a single item from a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapDeleteChange map_delete = 6;
* @return Whether the mapDelete field is set.
*/
@java.lang.Override
public boolean hasMapDelete() {
return changeOperationCase_ == 6;
}
/**
*
**
* A removal of a single item from a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapDeleteChange map_delete = 6;
* @return The mapDelete.
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.MapDeleteChange getMapDelete() {
if (changeOperationCase_ == 6) {
return (com.hedera.hapi.block.stream.output.protoc.MapDeleteChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.MapDeleteChange.getDefaultInstance();
}
/**
*
**
* A removal of a single item from a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapDeleteChange map_delete = 6;
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.MapDeleteChangeOrBuilder getMapDeleteOrBuilder() {
if (changeOperationCase_ == 6) {
return (com.hedera.hapi.block.stream.output.protoc.MapDeleteChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.MapDeleteChange.getDefaultInstance();
}
public static final int QUEUE_PUSH_FIELD_NUMBER = 7;
/**
*
**
* Addition of an item to a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePushChange queue_push = 7;
* @return Whether the queuePush field is set.
*/
@java.lang.Override
public boolean hasQueuePush() {
return changeOperationCase_ == 7;
}
/**
*
**
* Addition of an item to a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePushChange queue_push = 7;
* @return The queuePush.
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.QueuePushChange getQueuePush() {
if (changeOperationCase_ == 7) {
return (com.hedera.hapi.block.stream.output.protoc.QueuePushChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.QueuePushChange.getDefaultInstance();
}
/**
*
**
* Addition of an item to a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePushChange queue_push = 7;
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.QueuePushChangeOrBuilder getQueuePushOrBuilder() {
if (changeOperationCase_ == 7) {
return (com.hedera.hapi.block.stream.output.protoc.QueuePushChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.QueuePushChange.getDefaultInstance();
}
public static final int QUEUE_POP_FIELD_NUMBER = 8;
/**
*
**
* Removal of an item from a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePopChange queue_pop = 8;
* @return Whether the queuePop field is set.
*/
@java.lang.Override
public boolean hasQueuePop() {
return changeOperationCase_ == 8;
}
/**
*
**
* Removal of an item from a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePopChange queue_pop = 8;
* @return The queuePop.
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.QueuePopChange getQueuePop() {
if (changeOperationCase_ == 8) {
return (com.hedera.hapi.block.stream.output.protoc.QueuePopChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.QueuePopChange.getDefaultInstance();
}
/**
*
**
* Removal of an item from a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePopChange queue_pop = 8;
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.QueuePopChangeOrBuilder getQueuePopOrBuilder() {
if (changeOperationCase_ == 8) {
return (com.hedera.hapi.block.stream.output.protoc.QueuePopChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.QueuePopChange.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (stateId_ != 0) {
output.writeUInt32(1, stateId_);
}
if (changeOperationCase_ == 2) {
output.writeMessage(2, (com.hedera.hapi.block.stream.output.protoc.NewStateChange) changeOperation_);
}
if (changeOperationCase_ == 3) {
output.writeMessage(3, (com.hedera.hapi.block.stream.output.protoc.RemovedStateChange) changeOperation_);
}
if (changeOperationCase_ == 4) {
output.writeMessage(4, (com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange) changeOperation_);
}
if (changeOperationCase_ == 5) {
output.writeMessage(5, (com.hedera.hapi.block.stream.output.protoc.MapUpdateChange) changeOperation_);
}
if (changeOperationCase_ == 6) {
output.writeMessage(6, (com.hedera.hapi.block.stream.output.protoc.MapDeleteChange) changeOperation_);
}
if (changeOperationCase_ == 7) {
output.writeMessage(7, (com.hedera.hapi.block.stream.output.protoc.QueuePushChange) changeOperation_);
}
if (changeOperationCase_ == 8) {
output.writeMessage(8, (com.hedera.hapi.block.stream.output.protoc.QueuePopChange) changeOperation_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (stateId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, stateId_);
}
if (changeOperationCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (com.hedera.hapi.block.stream.output.protoc.NewStateChange) changeOperation_);
}
if (changeOperationCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (com.hedera.hapi.block.stream.output.protoc.RemovedStateChange) changeOperation_);
}
if (changeOperationCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange) changeOperation_);
}
if (changeOperationCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (com.hedera.hapi.block.stream.output.protoc.MapUpdateChange) changeOperation_);
}
if (changeOperationCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, (com.hedera.hapi.block.stream.output.protoc.MapDeleteChange) changeOperation_);
}
if (changeOperationCase_ == 7) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, (com.hedera.hapi.block.stream.output.protoc.QueuePushChange) changeOperation_);
}
if (changeOperationCase_ == 8) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, (com.hedera.hapi.block.stream.output.protoc.QueuePopChange) changeOperation_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.hedera.hapi.block.stream.output.protoc.StateChange)) {
return super.equals(obj);
}
com.hedera.hapi.block.stream.output.protoc.StateChange other = (com.hedera.hapi.block.stream.output.protoc.StateChange) obj;
if (getStateId()
!= other.getStateId()) return false;
if (!getChangeOperationCase().equals(other.getChangeOperationCase())) return false;
switch (changeOperationCase_) {
case 2:
if (!getStateAdd()
.equals(other.getStateAdd())) return false;
break;
case 3:
if (!getStateRemove()
.equals(other.getStateRemove())) return false;
break;
case 4:
if (!getSingletonUpdate()
.equals(other.getSingletonUpdate())) return false;
break;
case 5:
if (!getMapUpdate()
.equals(other.getMapUpdate())) return false;
break;
case 6:
if (!getMapDelete()
.equals(other.getMapDelete())) return false;
break;
case 7:
if (!getQueuePush()
.equals(other.getQueuePush())) return false;
break;
case 8:
if (!getQueuePop()
.equals(other.getQueuePop())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + STATE_ID_FIELD_NUMBER;
hash = (53 * hash) + getStateId();
switch (changeOperationCase_) {
case 2:
hash = (37 * hash) + STATE_ADD_FIELD_NUMBER;
hash = (53 * hash) + getStateAdd().hashCode();
break;
case 3:
hash = (37 * hash) + STATE_REMOVE_FIELD_NUMBER;
hash = (53 * hash) + getStateRemove().hashCode();
break;
case 4:
hash = (37 * hash) + SINGLETON_UPDATE_FIELD_NUMBER;
hash = (53 * hash) + getSingletonUpdate().hashCode();
break;
case 5:
hash = (37 * hash) + MAP_UPDATE_FIELD_NUMBER;
hash = (53 * hash) + getMapUpdate().hashCode();
break;
case 6:
hash = (37 * hash) + MAP_DELETE_FIELD_NUMBER;
hash = (53 * hash) + getMapDelete().hashCode();
break;
case 7:
hash = (37 * hash) + QUEUE_PUSH_FIELD_NUMBER;
hash = (53 * hash) + getQueuePush().hashCode();
break;
case 8:
hash = (37 * hash) + QUEUE_POP_FIELD_NUMBER;
hash = (53 * hash) + getQueuePop().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.hedera.hapi.block.stream.output.protoc.StateChange parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hedera.hapi.block.stream.output.protoc.StateChange parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hedera.hapi.block.stream.output.protoc.StateChange parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hedera.hapi.block.stream.output.protoc.StateChange parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hedera.hapi.block.stream.output.protoc.StateChange parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hedera.hapi.block.stream.output.protoc.StateChange parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hedera.hapi.block.stream.output.protoc.StateChange parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.hedera.hapi.block.stream.output.protoc.StateChange parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.hedera.hapi.block.stream.output.protoc.StateChange parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.hedera.hapi.block.stream.output.protoc.StateChange parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.hedera.hapi.block.stream.output.protoc.StateChange parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.hedera.hapi.block.stream.output.protoc.StateChange parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.hedera.hapi.block.stream.output.protoc.StateChange prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* A change to any item in the merkle tree.
* A State change SHALL represent one mutation of the network state merkle
* tree. The state changes published in the block stream MAY be combined
* into an ordered set of state mutations that transform the tree from any
* initial state to a destination state.<br/>
* When the full set of state change items from the block stream for a round
* is applied to the network state at the start of that round the result
* SHALL match the network state at the end of the round.
* TODO: Need documentation for how the merkle tree is constructed.
* Need to reference that document, stored in platform docs?, here.
*
*
* Protobuf type {@code com.hedera.hapi.block.stream.output.StateChange}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.hedera.hapi.block.stream.output.StateChange)
com.hedera.hapi.block.stream.output.protoc.StateChangeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.hedera.hapi.block.stream.output.protoc.StateChangesOuterClass.internal_static_com_hedera_hapi_block_stream_output_StateChange_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.hedera.hapi.block.stream.output.protoc.StateChangesOuterClass.internal_static_com_hedera_hapi_block_stream_output_StateChange_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.hedera.hapi.block.stream.output.protoc.StateChange.class, com.hedera.hapi.block.stream.output.protoc.StateChange.Builder.class);
}
// Construct using com.hedera.hapi.block.stream.output.protoc.StateChange.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
stateId_ = 0;
changeOperationCase_ = 0;
changeOperation_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.hedera.hapi.block.stream.output.protoc.StateChangesOuterClass.internal_static_com_hedera_hapi_block_stream_output_StateChange_descriptor;
}
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.StateChange getDefaultInstanceForType() {
return com.hedera.hapi.block.stream.output.protoc.StateChange.getDefaultInstance();
}
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.StateChange build() {
com.hedera.hapi.block.stream.output.protoc.StateChange result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.StateChange buildPartial() {
com.hedera.hapi.block.stream.output.protoc.StateChange result = new com.hedera.hapi.block.stream.output.protoc.StateChange(this);
result.stateId_ = stateId_;
if (changeOperationCase_ == 2) {
if (stateAddBuilder_ == null) {
result.changeOperation_ = changeOperation_;
} else {
result.changeOperation_ = stateAddBuilder_.build();
}
}
if (changeOperationCase_ == 3) {
if (stateRemoveBuilder_ == null) {
result.changeOperation_ = changeOperation_;
} else {
result.changeOperation_ = stateRemoveBuilder_.build();
}
}
if (changeOperationCase_ == 4) {
if (singletonUpdateBuilder_ == null) {
result.changeOperation_ = changeOperation_;
} else {
result.changeOperation_ = singletonUpdateBuilder_.build();
}
}
if (changeOperationCase_ == 5) {
if (mapUpdateBuilder_ == null) {
result.changeOperation_ = changeOperation_;
} else {
result.changeOperation_ = mapUpdateBuilder_.build();
}
}
if (changeOperationCase_ == 6) {
if (mapDeleteBuilder_ == null) {
result.changeOperation_ = changeOperation_;
} else {
result.changeOperation_ = mapDeleteBuilder_.build();
}
}
if (changeOperationCase_ == 7) {
if (queuePushBuilder_ == null) {
result.changeOperation_ = changeOperation_;
} else {
result.changeOperation_ = queuePushBuilder_.build();
}
}
if (changeOperationCase_ == 8) {
if (queuePopBuilder_ == null) {
result.changeOperation_ = changeOperation_;
} else {
result.changeOperation_ = queuePopBuilder_.build();
}
}
result.changeOperationCase_ = changeOperationCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.hedera.hapi.block.stream.output.protoc.StateChange) {
return mergeFrom((com.hedera.hapi.block.stream.output.protoc.StateChange)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.hedera.hapi.block.stream.output.protoc.StateChange other) {
if (other == com.hedera.hapi.block.stream.output.protoc.StateChange.getDefaultInstance()) return this;
if (other.getStateId() != 0) {
setStateId(other.getStateId());
}
switch (other.getChangeOperationCase()) {
case STATE_ADD: {
mergeStateAdd(other.getStateAdd());
break;
}
case STATE_REMOVE: {
mergeStateRemove(other.getStateRemove());
break;
}
case SINGLETON_UPDATE: {
mergeSingletonUpdate(other.getSingletonUpdate());
break;
}
case MAP_UPDATE: {
mergeMapUpdate(other.getMapUpdate());
break;
}
case MAP_DELETE: {
mergeMapDelete(other.getMapDelete());
break;
}
case QUEUE_PUSH: {
mergeQueuePush(other.getQueuePush());
break;
}
case QUEUE_POP: {
mergeQueuePop(other.getQueuePop());
break;
}
case CHANGEOPERATION_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.hedera.hapi.block.stream.output.protoc.StateChange parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.hedera.hapi.block.stream.output.protoc.StateChange) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int changeOperationCase_ = 0;
private java.lang.Object changeOperation_;
public ChangeOperationCase
getChangeOperationCase() {
return ChangeOperationCase.forNumber(
changeOperationCase_);
}
public Builder clearChangeOperation() {
changeOperationCase_ = 0;
changeOperation_ = null;
onChanged();
return this;
}
private int stateId_ ;
/**
*
**
* A state identifier.<br/>
* The reason we use an integer field here, and not an enum field is to
* better support forward compatibility. There will be many cases when a
* block node or other client (such as a dApp) with HAPI version N wants
* to process blocks from HAPI version N+1, for example. If we use a
* protobuf enum then when that is mapped Java or Rust it would not be
* parsed as an enum value because those languages do not support unknown
* enum values. ProtoC has a workaround for this but it is complex and
* requires non-deterministic parsing. Our solution to create an integer
* field and provide an enumeration for mapping that integer is intended
* as an acceptable compromise solution.
* <p>
* This number SHALL identify the merkle subtree "state" to be modified and
* often corresponds to a `VirtualMap` identifier.
* This number SHALL be a valid value for the `StateIdentifier` enum.
*
*
* uint32 state_id = 1;
* @return The stateId.
*/
@java.lang.Override
public int getStateId() {
return stateId_;
}
/**
*
**
* A state identifier.<br/>
* The reason we use an integer field here, and not an enum field is to
* better support forward compatibility. There will be many cases when a
* block node or other client (such as a dApp) with HAPI version N wants
* to process blocks from HAPI version N+1, for example. If we use a
* protobuf enum then when that is mapped Java or Rust it would not be
* parsed as an enum value because those languages do not support unknown
* enum values. ProtoC has a workaround for this but it is complex and
* requires non-deterministic parsing. Our solution to create an integer
* field and provide an enumeration for mapping that integer is intended
* as an acceptable compromise solution.
* <p>
* This number SHALL identify the merkle subtree "state" to be modified and
* often corresponds to a `VirtualMap` identifier.
* This number SHALL be a valid value for the `StateIdentifier` enum.
*
*
* uint32 state_id = 1;
* @param value The stateId to set.
* @return This builder for chaining.
*/
public Builder setStateId(int value) {
stateId_ = value;
onChanged();
return this;
}
/**
*
**
* A state identifier.<br/>
* The reason we use an integer field here, and not an enum field is to
* better support forward compatibility. There will be many cases when a
* block node or other client (such as a dApp) with HAPI version N wants
* to process blocks from HAPI version N+1, for example. If we use a
* protobuf enum then when that is mapped Java or Rust it would not be
* parsed as an enum value because those languages do not support unknown
* enum values. ProtoC has a workaround for this but it is complex and
* requires non-deterministic parsing. Our solution to create an integer
* field and provide an enumeration for mapping that integer is intended
* as an acceptable compromise solution.
* <p>
* This number SHALL identify the merkle subtree "state" to be modified and
* often corresponds to a `VirtualMap` identifier.
* This number SHALL be a valid value for the `StateIdentifier` enum.
*
*
* uint32 state_id = 1;
* @return This builder for chaining.
*/
public Builder clearStateId() {
stateId_ = 0;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.NewStateChange, com.hedera.hapi.block.stream.output.protoc.NewStateChange.Builder, com.hedera.hapi.block.stream.output.protoc.NewStateChangeOrBuilder> stateAddBuilder_;
/**
*
**
* Addition of a new state.<br/>
* This may be a singleton, virtual map, or queue state.
*
*
* .com.hedera.hapi.block.stream.output.NewStateChange state_add = 2;
* @return Whether the stateAdd field is set.
*/
@java.lang.Override
public boolean hasStateAdd() {
return changeOperationCase_ == 2;
}
/**
*
**
* Addition of a new state.<br/>
* This may be a singleton, virtual map, or queue state.
*
*
* .com.hedera.hapi.block.stream.output.NewStateChange state_add = 2;
* @return The stateAdd.
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.NewStateChange getStateAdd() {
if (stateAddBuilder_ == null) {
if (changeOperationCase_ == 2) {
return (com.hedera.hapi.block.stream.output.protoc.NewStateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.NewStateChange.getDefaultInstance();
} else {
if (changeOperationCase_ == 2) {
return stateAddBuilder_.getMessage();
}
return com.hedera.hapi.block.stream.output.protoc.NewStateChange.getDefaultInstance();
}
}
/**
*
**
* Addition of a new state.<br/>
* This may be a singleton, virtual map, or queue state.
*
*
* .com.hedera.hapi.block.stream.output.NewStateChange state_add = 2;
*/
public Builder setStateAdd(com.hedera.hapi.block.stream.output.protoc.NewStateChange value) {
if (stateAddBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
changeOperation_ = value;
onChanged();
} else {
stateAddBuilder_.setMessage(value);
}
changeOperationCase_ = 2;
return this;
}
/**
*
**
* Addition of a new state.<br/>
* This may be a singleton, virtual map, or queue state.
*
*
* .com.hedera.hapi.block.stream.output.NewStateChange state_add = 2;
*/
public Builder setStateAdd(
com.hedera.hapi.block.stream.output.protoc.NewStateChange.Builder builderForValue) {
if (stateAddBuilder_ == null) {
changeOperation_ = builderForValue.build();
onChanged();
} else {
stateAddBuilder_.setMessage(builderForValue.build());
}
changeOperationCase_ = 2;
return this;
}
/**
*
**
* Addition of a new state.<br/>
* This may be a singleton, virtual map, or queue state.
*
*
* .com.hedera.hapi.block.stream.output.NewStateChange state_add = 2;
*/
public Builder mergeStateAdd(com.hedera.hapi.block.stream.output.protoc.NewStateChange value) {
if (stateAddBuilder_ == null) {
if (changeOperationCase_ == 2 &&
changeOperation_ != com.hedera.hapi.block.stream.output.protoc.NewStateChange.getDefaultInstance()) {
changeOperation_ = com.hedera.hapi.block.stream.output.protoc.NewStateChange.newBuilder((com.hedera.hapi.block.stream.output.protoc.NewStateChange) changeOperation_)
.mergeFrom(value).buildPartial();
} else {
changeOperation_ = value;
}
onChanged();
} else {
if (changeOperationCase_ == 2) {
stateAddBuilder_.mergeFrom(value);
}
stateAddBuilder_.setMessage(value);
}
changeOperationCase_ = 2;
return this;
}
/**
*
**
* Addition of a new state.<br/>
* This may be a singleton, virtual map, or queue state.
*
*
* .com.hedera.hapi.block.stream.output.NewStateChange state_add = 2;
*/
public Builder clearStateAdd() {
if (stateAddBuilder_ == null) {
if (changeOperationCase_ == 2) {
changeOperationCase_ = 0;
changeOperation_ = null;
onChanged();
}
} else {
if (changeOperationCase_ == 2) {
changeOperationCase_ = 0;
changeOperation_ = null;
}
stateAddBuilder_.clear();
}
return this;
}
/**
*
**
* Addition of a new state.<br/>
* This may be a singleton, virtual map, or queue state.
*
*
* .com.hedera.hapi.block.stream.output.NewStateChange state_add = 2;
*/
public com.hedera.hapi.block.stream.output.protoc.NewStateChange.Builder getStateAddBuilder() {
return getStateAddFieldBuilder().getBuilder();
}
/**
*
**
* Addition of a new state.<br/>
* This may be a singleton, virtual map, or queue state.
*
*
* .com.hedera.hapi.block.stream.output.NewStateChange state_add = 2;
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.NewStateChangeOrBuilder getStateAddOrBuilder() {
if ((changeOperationCase_ == 2) && (stateAddBuilder_ != null)) {
return stateAddBuilder_.getMessageOrBuilder();
} else {
if (changeOperationCase_ == 2) {
return (com.hedera.hapi.block.stream.output.protoc.NewStateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.NewStateChange.getDefaultInstance();
}
}
/**
*
**
* Addition of a new state.<br/>
* This may be a singleton, virtual map, or queue state.
*
*
* .com.hedera.hapi.block.stream.output.NewStateChange state_add = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.NewStateChange, com.hedera.hapi.block.stream.output.protoc.NewStateChange.Builder, com.hedera.hapi.block.stream.output.protoc.NewStateChangeOrBuilder>
getStateAddFieldBuilder() {
if (stateAddBuilder_ == null) {
if (!(changeOperationCase_ == 2)) {
changeOperation_ = com.hedera.hapi.block.stream.output.protoc.NewStateChange.getDefaultInstance();
}
stateAddBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.NewStateChange, com.hedera.hapi.block.stream.output.protoc.NewStateChange.Builder, com.hedera.hapi.block.stream.output.protoc.NewStateChangeOrBuilder>(
(com.hedera.hapi.block.stream.output.protoc.NewStateChange) changeOperation_,
getParentForChildren(),
isClean());
changeOperation_ = null;
}
changeOperationCase_ = 2;
onChanged();;
return stateAddBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.RemovedStateChange, com.hedera.hapi.block.stream.output.protoc.RemovedStateChange.Builder, com.hedera.hapi.block.stream.output.protoc.RemovedStateChangeOrBuilder> stateRemoveBuilder_;
/**
*
**
* Removal of an existing state.<br/>
* The entire singleton, virtual map, or queue state is removed,
* and not just the contents.
*
*
* .com.hedera.hapi.block.stream.output.RemovedStateChange state_remove = 3;
* @return Whether the stateRemove field is set.
*/
@java.lang.Override
public boolean hasStateRemove() {
return changeOperationCase_ == 3;
}
/**
*
**
* Removal of an existing state.<br/>
* The entire singleton, virtual map, or queue state is removed,
* and not just the contents.
*
*
* .com.hedera.hapi.block.stream.output.RemovedStateChange state_remove = 3;
* @return The stateRemove.
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.RemovedStateChange getStateRemove() {
if (stateRemoveBuilder_ == null) {
if (changeOperationCase_ == 3) {
return (com.hedera.hapi.block.stream.output.protoc.RemovedStateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.RemovedStateChange.getDefaultInstance();
} else {
if (changeOperationCase_ == 3) {
return stateRemoveBuilder_.getMessage();
}
return com.hedera.hapi.block.stream.output.protoc.RemovedStateChange.getDefaultInstance();
}
}
/**
*
**
* Removal of an existing state.<br/>
* The entire singleton, virtual map, or queue state is removed,
* and not just the contents.
*
*
* .com.hedera.hapi.block.stream.output.RemovedStateChange state_remove = 3;
*/
public Builder setStateRemove(com.hedera.hapi.block.stream.output.protoc.RemovedStateChange value) {
if (stateRemoveBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
changeOperation_ = value;
onChanged();
} else {
stateRemoveBuilder_.setMessage(value);
}
changeOperationCase_ = 3;
return this;
}
/**
*
**
* Removal of an existing state.<br/>
* The entire singleton, virtual map, or queue state is removed,
* and not just the contents.
*
*
* .com.hedera.hapi.block.stream.output.RemovedStateChange state_remove = 3;
*/
public Builder setStateRemove(
com.hedera.hapi.block.stream.output.protoc.RemovedStateChange.Builder builderForValue) {
if (stateRemoveBuilder_ == null) {
changeOperation_ = builderForValue.build();
onChanged();
} else {
stateRemoveBuilder_.setMessage(builderForValue.build());
}
changeOperationCase_ = 3;
return this;
}
/**
*
**
* Removal of an existing state.<br/>
* The entire singleton, virtual map, or queue state is removed,
* and not just the contents.
*
*
* .com.hedera.hapi.block.stream.output.RemovedStateChange state_remove = 3;
*/
public Builder mergeStateRemove(com.hedera.hapi.block.stream.output.protoc.RemovedStateChange value) {
if (stateRemoveBuilder_ == null) {
if (changeOperationCase_ == 3 &&
changeOperation_ != com.hedera.hapi.block.stream.output.protoc.RemovedStateChange.getDefaultInstance()) {
changeOperation_ = com.hedera.hapi.block.stream.output.protoc.RemovedStateChange.newBuilder((com.hedera.hapi.block.stream.output.protoc.RemovedStateChange) changeOperation_)
.mergeFrom(value).buildPartial();
} else {
changeOperation_ = value;
}
onChanged();
} else {
if (changeOperationCase_ == 3) {
stateRemoveBuilder_.mergeFrom(value);
}
stateRemoveBuilder_.setMessage(value);
}
changeOperationCase_ = 3;
return this;
}
/**
*
**
* Removal of an existing state.<br/>
* The entire singleton, virtual map, or queue state is removed,
* and not just the contents.
*
*
* .com.hedera.hapi.block.stream.output.RemovedStateChange state_remove = 3;
*/
public Builder clearStateRemove() {
if (stateRemoveBuilder_ == null) {
if (changeOperationCase_ == 3) {
changeOperationCase_ = 0;
changeOperation_ = null;
onChanged();
}
} else {
if (changeOperationCase_ == 3) {
changeOperationCase_ = 0;
changeOperation_ = null;
}
stateRemoveBuilder_.clear();
}
return this;
}
/**
*
**
* Removal of an existing state.<br/>
* The entire singleton, virtual map, or queue state is removed,
* and not just the contents.
*
*
* .com.hedera.hapi.block.stream.output.RemovedStateChange state_remove = 3;
*/
public com.hedera.hapi.block.stream.output.protoc.RemovedStateChange.Builder getStateRemoveBuilder() {
return getStateRemoveFieldBuilder().getBuilder();
}
/**
*
**
* Removal of an existing state.<br/>
* The entire singleton, virtual map, or queue state is removed,
* and not just the contents.
*
*
* .com.hedera.hapi.block.stream.output.RemovedStateChange state_remove = 3;
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.RemovedStateChangeOrBuilder getStateRemoveOrBuilder() {
if ((changeOperationCase_ == 3) && (stateRemoveBuilder_ != null)) {
return stateRemoveBuilder_.getMessageOrBuilder();
} else {
if (changeOperationCase_ == 3) {
return (com.hedera.hapi.block.stream.output.protoc.RemovedStateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.RemovedStateChange.getDefaultInstance();
}
}
/**
*
**
* Removal of an existing state.<br/>
* The entire singleton, virtual map, or queue state is removed,
* and not just the contents.
*
*
* .com.hedera.hapi.block.stream.output.RemovedStateChange state_remove = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.RemovedStateChange, com.hedera.hapi.block.stream.output.protoc.RemovedStateChange.Builder, com.hedera.hapi.block.stream.output.protoc.RemovedStateChangeOrBuilder>
getStateRemoveFieldBuilder() {
if (stateRemoveBuilder_ == null) {
if (!(changeOperationCase_ == 3)) {
changeOperation_ = com.hedera.hapi.block.stream.output.protoc.RemovedStateChange.getDefaultInstance();
}
stateRemoveBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.RemovedStateChange, com.hedera.hapi.block.stream.output.protoc.RemovedStateChange.Builder, com.hedera.hapi.block.stream.output.protoc.RemovedStateChangeOrBuilder>(
(com.hedera.hapi.block.stream.output.protoc.RemovedStateChange) changeOperation_,
getParentForChildren(),
isClean());
changeOperation_ = null;
}
changeOperationCase_ = 3;
onChanged();;
return stateRemoveBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange, com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange.Builder, com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChangeOrBuilder> singletonUpdateBuilder_;
/**
*
**
* An add or update to a `Singleton` state.
*
*
* .com.hedera.hapi.block.stream.output.SingletonUpdateChange singleton_update = 4;
* @return Whether the singletonUpdate field is set.
*/
@java.lang.Override
public boolean hasSingletonUpdate() {
return changeOperationCase_ == 4;
}
/**
*
**
* An add or update to a `Singleton` state.
*
*
* .com.hedera.hapi.block.stream.output.SingletonUpdateChange singleton_update = 4;
* @return The singletonUpdate.
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange getSingletonUpdate() {
if (singletonUpdateBuilder_ == null) {
if (changeOperationCase_ == 4) {
return (com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange.getDefaultInstance();
} else {
if (changeOperationCase_ == 4) {
return singletonUpdateBuilder_.getMessage();
}
return com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange.getDefaultInstance();
}
}
/**
*
**
* An add or update to a `Singleton` state.
*
*
* .com.hedera.hapi.block.stream.output.SingletonUpdateChange singleton_update = 4;
*/
public Builder setSingletonUpdate(com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange value) {
if (singletonUpdateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
changeOperation_ = value;
onChanged();
} else {
singletonUpdateBuilder_.setMessage(value);
}
changeOperationCase_ = 4;
return this;
}
/**
*
**
* An add or update to a `Singleton` state.
*
*
* .com.hedera.hapi.block.stream.output.SingletonUpdateChange singleton_update = 4;
*/
public Builder setSingletonUpdate(
com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange.Builder builderForValue) {
if (singletonUpdateBuilder_ == null) {
changeOperation_ = builderForValue.build();
onChanged();
} else {
singletonUpdateBuilder_.setMessage(builderForValue.build());
}
changeOperationCase_ = 4;
return this;
}
/**
*
**
* An add or update to a `Singleton` state.
*
*
* .com.hedera.hapi.block.stream.output.SingletonUpdateChange singleton_update = 4;
*/
public Builder mergeSingletonUpdate(com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange value) {
if (singletonUpdateBuilder_ == null) {
if (changeOperationCase_ == 4 &&
changeOperation_ != com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange.getDefaultInstance()) {
changeOperation_ = com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange.newBuilder((com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange) changeOperation_)
.mergeFrom(value).buildPartial();
} else {
changeOperation_ = value;
}
onChanged();
} else {
if (changeOperationCase_ == 4) {
singletonUpdateBuilder_.mergeFrom(value);
}
singletonUpdateBuilder_.setMessage(value);
}
changeOperationCase_ = 4;
return this;
}
/**
*
**
* An add or update to a `Singleton` state.
*
*
* .com.hedera.hapi.block.stream.output.SingletonUpdateChange singleton_update = 4;
*/
public Builder clearSingletonUpdate() {
if (singletonUpdateBuilder_ == null) {
if (changeOperationCase_ == 4) {
changeOperationCase_ = 0;
changeOperation_ = null;
onChanged();
}
} else {
if (changeOperationCase_ == 4) {
changeOperationCase_ = 0;
changeOperation_ = null;
}
singletonUpdateBuilder_.clear();
}
return this;
}
/**
*
**
* An add or update to a `Singleton` state.
*
*
* .com.hedera.hapi.block.stream.output.SingletonUpdateChange singleton_update = 4;
*/
public com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange.Builder getSingletonUpdateBuilder() {
return getSingletonUpdateFieldBuilder().getBuilder();
}
/**
*
**
* An add or update to a `Singleton` state.
*
*
* .com.hedera.hapi.block.stream.output.SingletonUpdateChange singleton_update = 4;
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChangeOrBuilder getSingletonUpdateOrBuilder() {
if ((changeOperationCase_ == 4) && (singletonUpdateBuilder_ != null)) {
return singletonUpdateBuilder_.getMessageOrBuilder();
} else {
if (changeOperationCase_ == 4) {
return (com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange.getDefaultInstance();
}
}
/**
*
**
* An add or update to a `Singleton` state.
*
*
* .com.hedera.hapi.block.stream.output.SingletonUpdateChange singleton_update = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange, com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange.Builder, com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChangeOrBuilder>
getSingletonUpdateFieldBuilder() {
if (singletonUpdateBuilder_ == null) {
if (!(changeOperationCase_ == 4)) {
changeOperation_ = com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange.getDefaultInstance();
}
singletonUpdateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange, com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange.Builder, com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChangeOrBuilder>(
(com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange) changeOperation_,
getParentForChildren(),
isClean());
changeOperation_ = null;
}
changeOperationCase_ = 4;
onChanged();;
return singletonUpdateBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.MapUpdateChange, com.hedera.hapi.block.stream.output.protoc.MapUpdateChange.Builder, com.hedera.hapi.block.stream.output.protoc.MapUpdateChangeOrBuilder> mapUpdateBuilder_;
/**
*
**
* An add or update to a single item in a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapUpdateChange map_update = 5;
* @return Whether the mapUpdate field is set.
*/
@java.lang.Override
public boolean hasMapUpdate() {
return changeOperationCase_ == 5;
}
/**
*
**
* An add or update to a single item in a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapUpdateChange map_update = 5;
* @return The mapUpdate.
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.MapUpdateChange getMapUpdate() {
if (mapUpdateBuilder_ == null) {
if (changeOperationCase_ == 5) {
return (com.hedera.hapi.block.stream.output.protoc.MapUpdateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.MapUpdateChange.getDefaultInstance();
} else {
if (changeOperationCase_ == 5) {
return mapUpdateBuilder_.getMessage();
}
return com.hedera.hapi.block.stream.output.protoc.MapUpdateChange.getDefaultInstance();
}
}
/**
*
**
* An add or update to a single item in a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapUpdateChange map_update = 5;
*/
public Builder setMapUpdate(com.hedera.hapi.block.stream.output.protoc.MapUpdateChange value) {
if (mapUpdateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
changeOperation_ = value;
onChanged();
} else {
mapUpdateBuilder_.setMessage(value);
}
changeOperationCase_ = 5;
return this;
}
/**
*
**
* An add or update to a single item in a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapUpdateChange map_update = 5;
*/
public Builder setMapUpdate(
com.hedera.hapi.block.stream.output.protoc.MapUpdateChange.Builder builderForValue) {
if (mapUpdateBuilder_ == null) {
changeOperation_ = builderForValue.build();
onChanged();
} else {
mapUpdateBuilder_.setMessage(builderForValue.build());
}
changeOperationCase_ = 5;
return this;
}
/**
*
**
* An add or update to a single item in a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapUpdateChange map_update = 5;
*/
public Builder mergeMapUpdate(com.hedera.hapi.block.stream.output.protoc.MapUpdateChange value) {
if (mapUpdateBuilder_ == null) {
if (changeOperationCase_ == 5 &&
changeOperation_ != com.hedera.hapi.block.stream.output.protoc.MapUpdateChange.getDefaultInstance()) {
changeOperation_ = com.hedera.hapi.block.stream.output.protoc.MapUpdateChange.newBuilder((com.hedera.hapi.block.stream.output.protoc.MapUpdateChange) changeOperation_)
.mergeFrom(value).buildPartial();
} else {
changeOperation_ = value;
}
onChanged();
} else {
if (changeOperationCase_ == 5) {
mapUpdateBuilder_.mergeFrom(value);
}
mapUpdateBuilder_.setMessage(value);
}
changeOperationCase_ = 5;
return this;
}
/**
*
**
* An add or update to a single item in a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapUpdateChange map_update = 5;
*/
public Builder clearMapUpdate() {
if (mapUpdateBuilder_ == null) {
if (changeOperationCase_ == 5) {
changeOperationCase_ = 0;
changeOperation_ = null;
onChanged();
}
} else {
if (changeOperationCase_ == 5) {
changeOperationCase_ = 0;
changeOperation_ = null;
}
mapUpdateBuilder_.clear();
}
return this;
}
/**
*
**
* An add or update to a single item in a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapUpdateChange map_update = 5;
*/
public com.hedera.hapi.block.stream.output.protoc.MapUpdateChange.Builder getMapUpdateBuilder() {
return getMapUpdateFieldBuilder().getBuilder();
}
/**
*
**
* An add or update to a single item in a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapUpdateChange map_update = 5;
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.MapUpdateChangeOrBuilder getMapUpdateOrBuilder() {
if ((changeOperationCase_ == 5) && (mapUpdateBuilder_ != null)) {
return mapUpdateBuilder_.getMessageOrBuilder();
} else {
if (changeOperationCase_ == 5) {
return (com.hedera.hapi.block.stream.output.protoc.MapUpdateChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.MapUpdateChange.getDefaultInstance();
}
}
/**
*
**
* An add or update to a single item in a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapUpdateChange map_update = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.MapUpdateChange, com.hedera.hapi.block.stream.output.protoc.MapUpdateChange.Builder, com.hedera.hapi.block.stream.output.protoc.MapUpdateChangeOrBuilder>
getMapUpdateFieldBuilder() {
if (mapUpdateBuilder_ == null) {
if (!(changeOperationCase_ == 5)) {
changeOperation_ = com.hedera.hapi.block.stream.output.protoc.MapUpdateChange.getDefaultInstance();
}
mapUpdateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.MapUpdateChange, com.hedera.hapi.block.stream.output.protoc.MapUpdateChange.Builder, com.hedera.hapi.block.stream.output.protoc.MapUpdateChangeOrBuilder>(
(com.hedera.hapi.block.stream.output.protoc.MapUpdateChange) changeOperation_,
getParentForChildren(),
isClean());
changeOperation_ = null;
}
changeOperationCase_ = 5;
onChanged();;
return mapUpdateBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.MapDeleteChange, com.hedera.hapi.block.stream.output.protoc.MapDeleteChange.Builder, com.hedera.hapi.block.stream.output.protoc.MapDeleteChangeOrBuilder> mapDeleteBuilder_;
/**
*
**
* A removal of a single item from a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapDeleteChange map_delete = 6;
* @return Whether the mapDelete field is set.
*/
@java.lang.Override
public boolean hasMapDelete() {
return changeOperationCase_ == 6;
}
/**
*
**
* A removal of a single item from a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapDeleteChange map_delete = 6;
* @return The mapDelete.
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.MapDeleteChange getMapDelete() {
if (mapDeleteBuilder_ == null) {
if (changeOperationCase_ == 6) {
return (com.hedera.hapi.block.stream.output.protoc.MapDeleteChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.MapDeleteChange.getDefaultInstance();
} else {
if (changeOperationCase_ == 6) {
return mapDeleteBuilder_.getMessage();
}
return com.hedera.hapi.block.stream.output.protoc.MapDeleteChange.getDefaultInstance();
}
}
/**
*
**
* A removal of a single item from a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapDeleteChange map_delete = 6;
*/
public Builder setMapDelete(com.hedera.hapi.block.stream.output.protoc.MapDeleteChange value) {
if (mapDeleteBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
changeOperation_ = value;
onChanged();
} else {
mapDeleteBuilder_.setMessage(value);
}
changeOperationCase_ = 6;
return this;
}
/**
*
**
* A removal of a single item from a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapDeleteChange map_delete = 6;
*/
public Builder setMapDelete(
com.hedera.hapi.block.stream.output.protoc.MapDeleteChange.Builder builderForValue) {
if (mapDeleteBuilder_ == null) {
changeOperation_ = builderForValue.build();
onChanged();
} else {
mapDeleteBuilder_.setMessage(builderForValue.build());
}
changeOperationCase_ = 6;
return this;
}
/**
*
**
* A removal of a single item from a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapDeleteChange map_delete = 6;
*/
public Builder mergeMapDelete(com.hedera.hapi.block.stream.output.protoc.MapDeleteChange value) {
if (mapDeleteBuilder_ == null) {
if (changeOperationCase_ == 6 &&
changeOperation_ != com.hedera.hapi.block.stream.output.protoc.MapDeleteChange.getDefaultInstance()) {
changeOperation_ = com.hedera.hapi.block.stream.output.protoc.MapDeleteChange.newBuilder((com.hedera.hapi.block.stream.output.protoc.MapDeleteChange) changeOperation_)
.mergeFrom(value).buildPartial();
} else {
changeOperation_ = value;
}
onChanged();
} else {
if (changeOperationCase_ == 6) {
mapDeleteBuilder_.mergeFrom(value);
}
mapDeleteBuilder_.setMessage(value);
}
changeOperationCase_ = 6;
return this;
}
/**
*
**
* A removal of a single item from a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapDeleteChange map_delete = 6;
*/
public Builder clearMapDelete() {
if (mapDeleteBuilder_ == null) {
if (changeOperationCase_ == 6) {
changeOperationCase_ = 0;
changeOperation_ = null;
onChanged();
}
} else {
if (changeOperationCase_ == 6) {
changeOperationCase_ = 0;
changeOperation_ = null;
}
mapDeleteBuilder_.clear();
}
return this;
}
/**
*
**
* A removal of a single item from a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapDeleteChange map_delete = 6;
*/
public com.hedera.hapi.block.stream.output.protoc.MapDeleteChange.Builder getMapDeleteBuilder() {
return getMapDeleteFieldBuilder().getBuilder();
}
/**
*
**
* A removal of a single item from a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapDeleteChange map_delete = 6;
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.MapDeleteChangeOrBuilder getMapDeleteOrBuilder() {
if ((changeOperationCase_ == 6) && (mapDeleteBuilder_ != null)) {
return mapDeleteBuilder_.getMessageOrBuilder();
} else {
if (changeOperationCase_ == 6) {
return (com.hedera.hapi.block.stream.output.protoc.MapDeleteChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.MapDeleteChange.getDefaultInstance();
}
}
/**
*
**
* A removal of a single item from a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapDeleteChange map_delete = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.MapDeleteChange, com.hedera.hapi.block.stream.output.protoc.MapDeleteChange.Builder, com.hedera.hapi.block.stream.output.protoc.MapDeleteChangeOrBuilder>
getMapDeleteFieldBuilder() {
if (mapDeleteBuilder_ == null) {
if (!(changeOperationCase_ == 6)) {
changeOperation_ = com.hedera.hapi.block.stream.output.protoc.MapDeleteChange.getDefaultInstance();
}
mapDeleteBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.MapDeleteChange, com.hedera.hapi.block.stream.output.protoc.MapDeleteChange.Builder, com.hedera.hapi.block.stream.output.protoc.MapDeleteChangeOrBuilder>(
(com.hedera.hapi.block.stream.output.protoc.MapDeleteChange) changeOperation_,
getParentForChildren(),
isClean());
changeOperation_ = null;
}
changeOperationCase_ = 6;
onChanged();;
return mapDeleteBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.QueuePushChange, com.hedera.hapi.block.stream.output.protoc.QueuePushChange.Builder, com.hedera.hapi.block.stream.output.protoc.QueuePushChangeOrBuilder> queuePushBuilder_;
/**
*
**
* Addition of an item to a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePushChange queue_push = 7;
* @return Whether the queuePush field is set.
*/
@java.lang.Override
public boolean hasQueuePush() {
return changeOperationCase_ == 7;
}
/**
*
**
* Addition of an item to a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePushChange queue_push = 7;
* @return The queuePush.
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.QueuePushChange getQueuePush() {
if (queuePushBuilder_ == null) {
if (changeOperationCase_ == 7) {
return (com.hedera.hapi.block.stream.output.protoc.QueuePushChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.QueuePushChange.getDefaultInstance();
} else {
if (changeOperationCase_ == 7) {
return queuePushBuilder_.getMessage();
}
return com.hedera.hapi.block.stream.output.protoc.QueuePushChange.getDefaultInstance();
}
}
/**
*
**
* Addition of an item to a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePushChange queue_push = 7;
*/
public Builder setQueuePush(com.hedera.hapi.block.stream.output.protoc.QueuePushChange value) {
if (queuePushBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
changeOperation_ = value;
onChanged();
} else {
queuePushBuilder_.setMessage(value);
}
changeOperationCase_ = 7;
return this;
}
/**
*
**
* Addition of an item to a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePushChange queue_push = 7;
*/
public Builder setQueuePush(
com.hedera.hapi.block.stream.output.protoc.QueuePushChange.Builder builderForValue) {
if (queuePushBuilder_ == null) {
changeOperation_ = builderForValue.build();
onChanged();
} else {
queuePushBuilder_.setMessage(builderForValue.build());
}
changeOperationCase_ = 7;
return this;
}
/**
*
**
* Addition of an item to a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePushChange queue_push = 7;
*/
public Builder mergeQueuePush(com.hedera.hapi.block.stream.output.protoc.QueuePushChange value) {
if (queuePushBuilder_ == null) {
if (changeOperationCase_ == 7 &&
changeOperation_ != com.hedera.hapi.block.stream.output.protoc.QueuePushChange.getDefaultInstance()) {
changeOperation_ = com.hedera.hapi.block.stream.output.protoc.QueuePushChange.newBuilder((com.hedera.hapi.block.stream.output.protoc.QueuePushChange) changeOperation_)
.mergeFrom(value).buildPartial();
} else {
changeOperation_ = value;
}
onChanged();
} else {
if (changeOperationCase_ == 7) {
queuePushBuilder_.mergeFrom(value);
}
queuePushBuilder_.setMessage(value);
}
changeOperationCase_ = 7;
return this;
}
/**
*
**
* Addition of an item to a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePushChange queue_push = 7;
*/
public Builder clearQueuePush() {
if (queuePushBuilder_ == null) {
if (changeOperationCase_ == 7) {
changeOperationCase_ = 0;
changeOperation_ = null;
onChanged();
}
} else {
if (changeOperationCase_ == 7) {
changeOperationCase_ = 0;
changeOperation_ = null;
}
queuePushBuilder_.clear();
}
return this;
}
/**
*
**
* Addition of an item to a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePushChange queue_push = 7;
*/
public com.hedera.hapi.block.stream.output.protoc.QueuePushChange.Builder getQueuePushBuilder() {
return getQueuePushFieldBuilder().getBuilder();
}
/**
*
**
* Addition of an item to a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePushChange queue_push = 7;
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.QueuePushChangeOrBuilder getQueuePushOrBuilder() {
if ((changeOperationCase_ == 7) && (queuePushBuilder_ != null)) {
return queuePushBuilder_.getMessageOrBuilder();
} else {
if (changeOperationCase_ == 7) {
return (com.hedera.hapi.block.stream.output.protoc.QueuePushChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.QueuePushChange.getDefaultInstance();
}
}
/**
*
**
* Addition of an item to a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePushChange queue_push = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.QueuePushChange, com.hedera.hapi.block.stream.output.protoc.QueuePushChange.Builder, com.hedera.hapi.block.stream.output.protoc.QueuePushChangeOrBuilder>
getQueuePushFieldBuilder() {
if (queuePushBuilder_ == null) {
if (!(changeOperationCase_ == 7)) {
changeOperation_ = com.hedera.hapi.block.stream.output.protoc.QueuePushChange.getDefaultInstance();
}
queuePushBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.QueuePushChange, com.hedera.hapi.block.stream.output.protoc.QueuePushChange.Builder, com.hedera.hapi.block.stream.output.protoc.QueuePushChangeOrBuilder>(
(com.hedera.hapi.block.stream.output.protoc.QueuePushChange) changeOperation_,
getParentForChildren(),
isClean());
changeOperation_ = null;
}
changeOperationCase_ = 7;
onChanged();;
return queuePushBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.QueuePopChange, com.hedera.hapi.block.stream.output.protoc.QueuePopChange.Builder, com.hedera.hapi.block.stream.output.protoc.QueuePopChangeOrBuilder> queuePopBuilder_;
/**
*
**
* Removal of an item from a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePopChange queue_pop = 8;
* @return Whether the queuePop field is set.
*/
@java.lang.Override
public boolean hasQueuePop() {
return changeOperationCase_ == 8;
}
/**
*
**
* Removal of an item from a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePopChange queue_pop = 8;
* @return The queuePop.
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.QueuePopChange getQueuePop() {
if (queuePopBuilder_ == null) {
if (changeOperationCase_ == 8) {
return (com.hedera.hapi.block.stream.output.protoc.QueuePopChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.QueuePopChange.getDefaultInstance();
} else {
if (changeOperationCase_ == 8) {
return queuePopBuilder_.getMessage();
}
return com.hedera.hapi.block.stream.output.protoc.QueuePopChange.getDefaultInstance();
}
}
/**
*
**
* Removal of an item from a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePopChange queue_pop = 8;
*/
public Builder setQueuePop(com.hedera.hapi.block.stream.output.protoc.QueuePopChange value) {
if (queuePopBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
changeOperation_ = value;
onChanged();
} else {
queuePopBuilder_.setMessage(value);
}
changeOperationCase_ = 8;
return this;
}
/**
*
**
* Removal of an item from a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePopChange queue_pop = 8;
*/
public Builder setQueuePop(
com.hedera.hapi.block.stream.output.protoc.QueuePopChange.Builder builderForValue) {
if (queuePopBuilder_ == null) {
changeOperation_ = builderForValue.build();
onChanged();
} else {
queuePopBuilder_.setMessage(builderForValue.build());
}
changeOperationCase_ = 8;
return this;
}
/**
*
**
* Removal of an item from a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePopChange queue_pop = 8;
*/
public Builder mergeQueuePop(com.hedera.hapi.block.stream.output.protoc.QueuePopChange value) {
if (queuePopBuilder_ == null) {
if (changeOperationCase_ == 8 &&
changeOperation_ != com.hedera.hapi.block.stream.output.protoc.QueuePopChange.getDefaultInstance()) {
changeOperation_ = com.hedera.hapi.block.stream.output.protoc.QueuePopChange.newBuilder((com.hedera.hapi.block.stream.output.protoc.QueuePopChange) changeOperation_)
.mergeFrom(value).buildPartial();
} else {
changeOperation_ = value;
}
onChanged();
} else {
if (changeOperationCase_ == 8) {
queuePopBuilder_.mergeFrom(value);
}
queuePopBuilder_.setMessage(value);
}
changeOperationCase_ = 8;
return this;
}
/**
*
**
* Removal of an item from a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePopChange queue_pop = 8;
*/
public Builder clearQueuePop() {
if (queuePopBuilder_ == null) {
if (changeOperationCase_ == 8) {
changeOperationCase_ = 0;
changeOperation_ = null;
onChanged();
}
} else {
if (changeOperationCase_ == 8) {
changeOperationCase_ = 0;
changeOperation_ = null;
}
queuePopBuilder_.clear();
}
return this;
}
/**
*
**
* Removal of an item from a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePopChange queue_pop = 8;
*/
public com.hedera.hapi.block.stream.output.protoc.QueuePopChange.Builder getQueuePopBuilder() {
return getQueuePopFieldBuilder().getBuilder();
}
/**
*
**
* Removal of an item from a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePopChange queue_pop = 8;
*/
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.QueuePopChangeOrBuilder getQueuePopOrBuilder() {
if ((changeOperationCase_ == 8) && (queuePopBuilder_ != null)) {
return queuePopBuilder_.getMessageOrBuilder();
} else {
if (changeOperationCase_ == 8) {
return (com.hedera.hapi.block.stream.output.protoc.QueuePopChange) changeOperation_;
}
return com.hedera.hapi.block.stream.output.protoc.QueuePopChange.getDefaultInstance();
}
}
/**
*
**
* Removal of an item from a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePopChange queue_pop = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.QueuePopChange, com.hedera.hapi.block.stream.output.protoc.QueuePopChange.Builder, com.hedera.hapi.block.stream.output.protoc.QueuePopChangeOrBuilder>
getQueuePopFieldBuilder() {
if (queuePopBuilder_ == null) {
if (!(changeOperationCase_ == 8)) {
changeOperation_ = com.hedera.hapi.block.stream.output.protoc.QueuePopChange.getDefaultInstance();
}
queuePopBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hedera.hapi.block.stream.output.protoc.QueuePopChange, com.hedera.hapi.block.stream.output.protoc.QueuePopChange.Builder, com.hedera.hapi.block.stream.output.protoc.QueuePopChangeOrBuilder>(
(com.hedera.hapi.block.stream.output.protoc.QueuePopChange) changeOperation_,
getParentForChildren(),
isClean());
changeOperation_ = null;
}
changeOperationCase_ = 8;
onChanged();;
return queuePopBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.hedera.hapi.block.stream.output.StateChange)
}
// @@protoc_insertion_point(class_scope:com.hedera.hapi.block.stream.output.StateChange)
private static final com.hedera.hapi.block.stream.output.protoc.StateChange DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.hedera.hapi.block.stream.output.protoc.StateChange();
}
public static com.hedera.hapi.block.stream.output.protoc.StateChange getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StateChange parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StateChange(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.hedera.hapi.block.stream.output.protoc.StateChange getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy