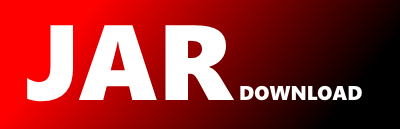
com.hedera.hapi.block.stream.output.protoc.StateChangeOrBuilder Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: stream/output/state_changes.proto
package com.hedera.hapi.block.stream.output.protoc;
public interface StateChangeOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.hedera.hapi.block.stream.output.StateChange)
com.google.protobuf.MessageOrBuilder {
/**
*
**
* A state identifier.<br/>
* The reason we use an integer field here, and not an enum field is to
* better support forward compatibility. There will be many cases when a
* block node or other client (such as a dApp) with HAPI version N wants
* to process blocks from HAPI version N+1, for example. If we use a
* protobuf enum then when that is mapped Java or Rust it would not be
* parsed as an enum value because those languages do not support unknown
* enum values. ProtoC has a workaround for this but it is complex and
* requires non-deterministic parsing. Our solution to create an integer
* field and provide an enumeration for mapping that integer is intended
* as an acceptable compromise solution.
* <p>
* This number SHALL identify the merkle subtree "state" to be modified and
* often corresponds to a `VirtualMap` identifier.
* This number SHALL be a valid value for the `StateIdentifier` enum.
*
*
* uint32 state_id = 1;
* @return The stateId.
*/
int getStateId();
/**
*
**
* Addition of a new state.<br/>
* This may be a singleton, virtual map, or queue state.
*
*
* .com.hedera.hapi.block.stream.output.NewStateChange state_add = 2;
* @return Whether the stateAdd field is set.
*/
boolean hasStateAdd();
/**
*
**
* Addition of a new state.<br/>
* This may be a singleton, virtual map, or queue state.
*
*
* .com.hedera.hapi.block.stream.output.NewStateChange state_add = 2;
* @return The stateAdd.
*/
com.hedera.hapi.block.stream.output.protoc.NewStateChange getStateAdd();
/**
*
**
* Addition of a new state.<br/>
* This may be a singleton, virtual map, or queue state.
*
*
* .com.hedera.hapi.block.stream.output.NewStateChange state_add = 2;
*/
com.hedera.hapi.block.stream.output.protoc.NewStateChangeOrBuilder getStateAddOrBuilder();
/**
*
**
* Removal of an existing state.<br/>
* The entire singleton, virtual map, or queue state is removed,
* and not just the contents.
*
*
* .com.hedera.hapi.block.stream.output.RemovedStateChange state_remove = 3;
* @return Whether the stateRemove field is set.
*/
boolean hasStateRemove();
/**
*
**
* Removal of an existing state.<br/>
* The entire singleton, virtual map, or queue state is removed,
* and not just the contents.
*
*
* .com.hedera.hapi.block.stream.output.RemovedStateChange state_remove = 3;
* @return The stateRemove.
*/
com.hedera.hapi.block.stream.output.protoc.RemovedStateChange getStateRemove();
/**
*
**
* Removal of an existing state.<br/>
* The entire singleton, virtual map, or queue state is removed,
* and not just the contents.
*
*
* .com.hedera.hapi.block.stream.output.RemovedStateChange state_remove = 3;
*/
com.hedera.hapi.block.stream.output.protoc.RemovedStateChangeOrBuilder getStateRemoveOrBuilder();
/**
*
**
* An add or update to a `Singleton` state.
*
*
* .com.hedera.hapi.block.stream.output.SingletonUpdateChange singleton_update = 4;
* @return Whether the singletonUpdate field is set.
*/
boolean hasSingletonUpdate();
/**
*
**
* An add or update to a `Singleton` state.
*
*
* .com.hedera.hapi.block.stream.output.SingletonUpdateChange singleton_update = 4;
* @return The singletonUpdate.
*/
com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChange getSingletonUpdate();
/**
*
**
* An add or update to a `Singleton` state.
*
*
* .com.hedera.hapi.block.stream.output.SingletonUpdateChange singleton_update = 4;
*/
com.hedera.hapi.block.stream.output.protoc.SingletonUpdateChangeOrBuilder getSingletonUpdateOrBuilder();
/**
*
**
* An add or update to a single item in a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapUpdateChange map_update = 5;
* @return Whether the mapUpdate field is set.
*/
boolean hasMapUpdate();
/**
*
**
* An add or update to a single item in a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapUpdateChange map_update = 5;
* @return The mapUpdate.
*/
com.hedera.hapi.block.stream.output.protoc.MapUpdateChange getMapUpdate();
/**
*
**
* An add or update to a single item in a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapUpdateChange map_update = 5;
*/
com.hedera.hapi.block.stream.output.protoc.MapUpdateChangeOrBuilder getMapUpdateOrBuilder();
/**
*
**
* A removal of a single item from a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapDeleteChange map_delete = 6;
* @return Whether the mapDelete field is set.
*/
boolean hasMapDelete();
/**
*
**
* A removal of a single item from a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapDeleteChange map_delete = 6;
* @return The mapDelete.
*/
com.hedera.hapi.block.stream.output.protoc.MapDeleteChange getMapDelete();
/**
*
**
* A removal of a single item from a `VirtualMap`.
*
*
* .com.hedera.hapi.block.stream.output.MapDeleteChange map_delete = 6;
*/
com.hedera.hapi.block.stream.output.protoc.MapDeleteChangeOrBuilder getMapDeleteOrBuilder();
/**
*
**
* Addition of an item to a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePushChange queue_push = 7;
* @return Whether the queuePush field is set.
*/
boolean hasQueuePush();
/**
*
**
* Addition of an item to a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePushChange queue_push = 7;
* @return The queuePush.
*/
com.hedera.hapi.block.stream.output.protoc.QueuePushChange getQueuePush();
/**
*
**
* Addition of an item to a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePushChange queue_push = 7;
*/
com.hedera.hapi.block.stream.output.protoc.QueuePushChangeOrBuilder getQueuePushOrBuilder();
/**
*
**
* Removal of an item from a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePopChange queue_pop = 8;
* @return Whether the queuePop field is set.
*/
boolean hasQueuePop();
/**
*
**
* Removal of an item from a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePopChange queue_pop = 8;
* @return The queuePop.
*/
com.hedera.hapi.block.stream.output.protoc.QueuePopChange getQueuePop();
/**
*
**
* Removal of an item from a `Queue` state.
*
*
* .com.hedera.hapi.block.stream.output.QueuePopChange queue_pop = 8;
*/
com.hedera.hapi.block.stream.output.protoc.QueuePopChangeOrBuilder getQueuePopOrBuilder();
public com.hedera.hapi.block.stream.output.protoc.StateChange.ChangeOperationCase getChangeOperationCase();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy