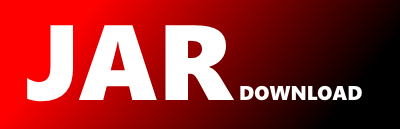
com.hedera.services.stream.proto.ContractActionOrBuilder Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: contract_action.proto
package com.hedera.services.stream.proto;
public interface ContractActionOrBuilder extends
// @@protoc_insertion_point(interface_extends:proto.ContractAction)
com.google.protobuf.MessageOrBuilder {
/**
*
**
* The type of this action.
*
*
* .proto.ContractActionType call_type = 1;
* @return The enum numeric value on the wire for callType.
*/
int getCallTypeValue();
/**
*
**
* The type of this action.
*
*
* .proto.ContractActionType call_type = 1;
* @return The callType.
*/
com.hedera.services.stream.proto.ContractActionType getCallType();
/**
*
**
* If the caller was a regular account, the AccountID.
*
*
* .proto.AccountID calling_account = 2;
* @return Whether the callingAccount field is set.
*/
boolean hasCallingAccount();
/**
*
**
* If the caller was a regular account, the AccountID.
*
*
* .proto.AccountID calling_account = 2;
* @return The callingAccount.
*/
com.hederahashgraph.api.proto.java.AccountID getCallingAccount();
/**
*
**
* If the caller was a regular account, the AccountID.
*
*
* .proto.AccountID calling_account = 2;
*/
com.hederahashgraph.api.proto.java.AccountIDOrBuilder getCallingAccountOrBuilder();
/**
*
**
* If the caller was a smart contract account, the ContractID.
*
*
* .proto.ContractID calling_contract = 3;
* @return Whether the callingContract field is set.
*/
boolean hasCallingContract();
/**
*
**
* If the caller was a smart contract account, the ContractID.
*
*
* .proto.ContractID calling_contract = 3;
* @return The callingContract.
*/
com.hederahashgraph.api.proto.java.ContractID getCallingContract();
/**
*
**
* If the caller was a smart contract account, the ContractID.
*
*
* .proto.ContractID calling_contract = 3;
*/
com.hederahashgraph.api.proto.java.ContractIDOrBuilder getCallingContractOrBuilder();
/**
*
**
* The upper limit of gas this action can spend.
*
*
* int64 gas = 4;
* @return The gas.
*/
long getGas();
/**
*
**
* Bytes passed in as input data to this action.
*
*
* bytes input = 5;
* @return The input.
*/
com.google.protobuf.ByteString getInput();
/**
*
**
* The AccountID of the recipient if the recipient is an account. Only HBars will be transferred, no other side
* effects should be expected.
*
*
* .proto.AccountID recipient_account = 6;
* @return Whether the recipientAccount field is set.
*/
boolean hasRecipientAccount();
/**
*
**
* The AccountID of the recipient if the recipient is an account. Only HBars will be transferred, no other side
* effects should be expected.
*
*
* .proto.AccountID recipient_account = 6;
* @return The recipientAccount.
*/
com.hederahashgraph.api.proto.java.AccountID getRecipientAccount();
/**
*
**
* The AccountID of the recipient if the recipient is an account. Only HBars will be transferred, no other side
* effects should be expected.
*
*
* .proto.AccountID recipient_account = 6;
*/
com.hederahashgraph.api.proto.java.AccountIDOrBuilder getRecipientAccountOrBuilder();
/**
*
**
* The ContractID of the recipient if the recipient is a smart contract.
*
*
* .proto.ContractID recipient_contract = 7;
* @return Whether the recipientContract field is set.
*/
boolean hasRecipientContract();
/**
*
**
* The ContractID of the recipient if the recipient is a smart contract.
*
*
* .proto.ContractID recipient_contract = 7;
* @return The recipientContract.
*/
com.hederahashgraph.api.proto.java.ContractID getRecipientContract();
/**
*
**
* The ContractID of the recipient if the recipient is a smart contract.
*
*
* .proto.ContractID recipient_contract = 7;
*/
com.hederahashgraph.api.proto.java.ContractIDOrBuilder getRecipientContractOrBuilder();
/**
*
**
* The bytes of the targeted by the action address.
* Only set on failed executions. If set, denotes that the address did not
* correspond to any account or contract at the time of finalization of
* this action.
* An example would be a failed lazy create as per HIP-583.
*
*
* bytes targeted_address = 8;
* @return Whether the targetedAddress field is set.
*/
boolean hasTargetedAddress();
/**
*
**
* The bytes of the targeted by the action address.
* Only set on failed executions. If set, denotes that the address did not
* correspond to any account or contract at the time of finalization of
* this action.
* An example would be a failed lazy create as per HIP-583.
*
*
* bytes targeted_address = 8;
* @return The targetedAddress.
*/
com.google.protobuf.ByteString getTargetedAddress();
/**
*
**
* The value (in tinybars) that is associated with this action.
*
*
* int64 value = 9;
* @return The value.
*/
long getValue();
/**
*
**
* The actual gas spent by this action.
*
*
* int64 gas_used = 10;
* @return The gasUsed.
*/
long getGasUsed();
/**
*
**
* If successful, the output bytes of the action.
*
*
* bytes output = 11;
* @return Whether the output field is set.
*/
boolean hasOutput();
/**
*
**
* If successful, the output bytes of the action.
*
*
* bytes output = 11;
* @return The output.
*/
com.google.protobuf.ByteString getOutput();
/**
*
**
* The contract itself caused the transaction to fail via the `REVERT` operation
*
*
* bytes revert_reason = 12;
* @return Whether the revertReason field is set.
*/
boolean hasRevertReason();
/**
*
**
* The contract itself caused the transaction to fail via the `REVERT` operation
*
*
* bytes revert_reason = 12;
* @return The revertReason.
*/
com.google.protobuf.ByteString getRevertReason();
/**
*
**
* The transaction itself failed without an explicit `REVERT`
*
*
* bytes error = 13;
* @return Whether the error field is set.
*/
boolean hasError();
/**
*
**
* The transaction itself failed without an explicit `REVERT`
*
*
* bytes error = 13;
* @return The error.
*/
com.google.protobuf.ByteString getError();
/**
*
**
* The nesting depth of this call. The original action is at depth=0.
*
*
* int32 call_depth = 14;
* @return The callDepth.
*/
int getCallDepth();
/**
*
**
* The call operation type
*
*
* .proto.CallOperationType call_operation_type = 15;
* @return The enum numeric value on the wire for callOperationType.
*/
int getCallOperationTypeValue();
/**
*
**
* The call operation type
*
*
* .proto.CallOperationType call_operation_type = 15;
* @return The callOperationType.
*/
com.hedera.services.stream.proto.CallOperationType getCallOperationType();
public com.hedera.services.stream.proto.ContractAction.CallerCase getCallerCase();
public com.hedera.services.stream.proto.ContractAction.RecipientCase getRecipientCase();
public com.hedera.services.stream.proto.ContractAction.ResultDataCase getResultDataCase();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy