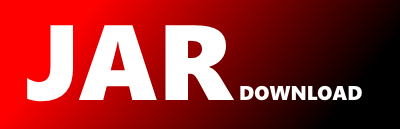
com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: CryptoTransfer.proto
package com.hederahashgraph.api.proto.java;
/**
*
* Transfer cryptocurrency from some accounts to other accounts. The accounts list can contain up to 10 accounts. The amounts list must be the same length as the accounts list. Each negative amount is withdrawn from the corresponding account (a sender), and each positive one is added to the corresponding account (a receiver). The amounts list must sum to zero. Each amount is a number of tinyBars (there are 100,000,000 tinyBars in one Hbar).
*If any sender account fails to have sufficient hbars, then the entire transaction fails, and none of those transfers occur, though the transaction fee is still charged. This transaction must be signed by the keys for all the sending accounts, and for any receiving accounts that have receiverSigRequired == true. The signatures are in the same order as the accounts, skipping those accounts that don't need a signature.
*
*
* Protobuf type {@code proto.CryptoTransferTransactionBody}
*/
public final class CryptoTransferTransactionBody extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:proto.CryptoTransferTransactionBody)
CryptoTransferTransactionBodyOrBuilder {
private static final long serialVersionUID = 0L;
// Use CryptoTransferTransactionBody.newBuilder() to construct.
private CryptoTransferTransactionBody(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CryptoTransferTransactionBody() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CryptoTransferTransactionBody(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.hederahashgraph.api.proto.java.TransferList.Builder subBuilder = null;
if (transfers_ != null) {
subBuilder = transfers_.toBuilder();
}
transfers_ = input.readMessage(com.hederahashgraph.api.proto.java.TransferList.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(transfers_);
transfers_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.hederahashgraph.api.proto.java.CryptoTransfer.internal_static_proto_CryptoTransferTransactionBody_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.hederahashgraph.api.proto.java.CryptoTransfer.internal_static_proto_CryptoTransferTransactionBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody.class, com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody.Builder.class);
}
public static final int TRANSFERS_FIELD_NUMBER = 1;
private com.hederahashgraph.api.proto.java.TransferList transfers_;
/**
*
* Accounts and amounts to transfer
*
*
* .proto.TransferList transfers = 1;
*/
public boolean hasTransfers() {
return transfers_ != null;
}
/**
*
* Accounts and amounts to transfer
*
*
* .proto.TransferList transfers = 1;
*/
public com.hederahashgraph.api.proto.java.TransferList getTransfers() {
return transfers_ == null ? com.hederahashgraph.api.proto.java.TransferList.getDefaultInstance() : transfers_;
}
/**
*
* Accounts and amounts to transfer
*
*
* .proto.TransferList transfers = 1;
*/
public com.hederahashgraph.api.proto.java.TransferListOrBuilder getTransfersOrBuilder() {
return getTransfers();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (transfers_ != null) {
output.writeMessage(1, getTransfers());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (transfers_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getTransfers());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody)) {
return super.equals(obj);
}
com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody other = (com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody) obj;
boolean result = true;
result = result && (hasTransfers() == other.hasTransfers());
if (hasTransfers()) {
result = result && getTransfers()
.equals(other.getTransfers());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTransfers()) {
hash = (37 * hash) + TRANSFERS_FIELD_NUMBER;
hash = (53 * hash) + getTransfers().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Transfer cryptocurrency from some accounts to other accounts. The accounts list can contain up to 10 accounts. The amounts list must be the same length as the accounts list. Each negative amount is withdrawn from the corresponding account (a sender), and each positive one is added to the corresponding account (a receiver). The amounts list must sum to zero. Each amount is a number of tinyBars (there are 100,000,000 tinyBars in one Hbar).
*If any sender account fails to have sufficient hbars, then the entire transaction fails, and none of those transfers occur, though the transaction fee is still charged. This transaction must be signed by the keys for all the sending accounts, and for any receiving accounts that have receiverSigRequired == true. The signatures are in the same order as the accounts, skipping those accounts that don't need a signature.
*
*
* Protobuf type {@code proto.CryptoTransferTransactionBody}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:proto.CryptoTransferTransactionBody)
com.hederahashgraph.api.proto.java.CryptoTransferTransactionBodyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.hederahashgraph.api.proto.java.CryptoTransfer.internal_static_proto_CryptoTransferTransactionBody_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.hederahashgraph.api.proto.java.CryptoTransfer.internal_static_proto_CryptoTransferTransactionBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody.class, com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody.Builder.class);
}
// Construct using com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
if (transfersBuilder_ == null) {
transfers_ = null;
} else {
transfers_ = null;
transfersBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.hederahashgraph.api.proto.java.CryptoTransfer.internal_static_proto_CryptoTransferTransactionBody_descriptor;
}
public com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody getDefaultInstanceForType() {
return com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody.getDefaultInstance();
}
public com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody build() {
com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody buildPartial() {
com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody result = new com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody(this);
if (transfersBuilder_ == null) {
result.transfers_ = transfers_;
} else {
result.transfers_ = transfersBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody) {
return mergeFrom((com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody other) {
if (other == com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody.getDefaultInstance()) return this;
if (other.hasTransfers()) {
mergeTransfers(other.getTransfers());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.hederahashgraph.api.proto.java.TransferList transfers_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.TransferList, com.hederahashgraph.api.proto.java.TransferList.Builder, com.hederahashgraph.api.proto.java.TransferListOrBuilder> transfersBuilder_;
/**
*
* Accounts and amounts to transfer
*
*
* .proto.TransferList transfers = 1;
*/
public boolean hasTransfers() {
return transfersBuilder_ != null || transfers_ != null;
}
/**
*
* Accounts and amounts to transfer
*
*
* .proto.TransferList transfers = 1;
*/
public com.hederahashgraph.api.proto.java.TransferList getTransfers() {
if (transfersBuilder_ == null) {
return transfers_ == null ? com.hederahashgraph.api.proto.java.TransferList.getDefaultInstance() : transfers_;
} else {
return transfersBuilder_.getMessage();
}
}
/**
*
* Accounts and amounts to transfer
*
*
* .proto.TransferList transfers = 1;
*/
public Builder setTransfers(com.hederahashgraph.api.proto.java.TransferList value) {
if (transfersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
transfers_ = value;
onChanged();
} else {
transfersBuilder_.setMessage(value);
}
return this;
}
/**
*
* Accounts and amounts to transfer
*
*
* .proto.TransferList transfers = 1;
*/
public Builder setTransfers(
com.hederahashgraph.api.proto.java.TransferList.Builder builderForValue) {
if (transfersBuilder_ == null) {
transfers_ = builderForValue.build();
onChanged();
} else {
transfersBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Accounts and amounts to transfer
*
*
* .proto.TransferList transfers = 1;
*/
public Builder mergeTransfers(com.hederahashgraph.api.proto.java.TransferList value) {
if (transfersBuilder_ == null) {
if (transfers_ != null) {
transfers_ =
com.hederahashgraph.api.proto.java.TransferList.newBuilder(transfers_).mergeFrom(value).buildPartial();
} else {
transfers_ = value;
}
onChanged();
} else {
transfersBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Accounts and amounts to transfer
*
*
* .proto.TransferList transfers = 1;
*/
public Builder clearTransfers() {
if (transfersBuilder_ == null) {
transfers_ = null;
onChanged();
} else {
transfers_ = null;
transfersBuilder_ = null;
}
return this;
}
/**
*
* Accounts and amounts to transfer
*
*
* .proto.TransferList transfers = 1;
*/
public com.hederahashgraph.api.proto.java.TransferList.Builder getTransfersBuilder() {
onChanged();
return getTransfersFieldBuilder().getBuilder();
}
/**
*
* Accounts and amounts to transfer
*
*
* .proto.TransferList transfers = 1;
*/
public com.hederahashgraph.api.proto.java.TransferListOrBuilder getTransfersOrBuilder() {
if (transfersBuilder_ != null) {
return transfersBuilder_.getMessageOrBuilder();
} else {
return transfers_ == null ?
com.hederahashgraph.api.proto.java.TransferList.getDefaultInstance() : transfers_;
}
}
/**
*
* Accounts and amounts to transfer
*
*
* .proto.TransferList transfers = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.TransferList, com.hederahashgraph.api.proto.java.TransferList.Builder, com.hederahashgraph.api.proto.java.TransferListOrBuilder>
getTransfersFieldBuilder() {
if (transfersBuilder_ == null) {
transfersBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.hederahashgraph.api.proto.java.TransferList, com.hederahashgraph.api.proto.java.TransferList.Builder, com.hederahashgraph.api.proto.java.TransferListOrBuilder>(
getTransfers(),
getParentForChildren(),
isClean());
transfers_ = null;
}
return transfersBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:proto.CryptoTransferTransactionBody)
}
// @@protoc_insertion_point(class_scope:proto.CryptoTransferTransactionBody)
private static final com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody();
}
public static com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public CryptoTransferTransactionBody parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CryptoTransferTransactionBody(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.hederahashgraph.api.proto.java.CryptoTransferTransactionBody getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy