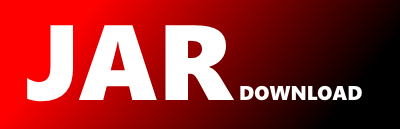
com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk-jdk7 Show documentation
Show all versions of sdk-jdk7 Show documentation
Hedera™ Hashgraph SDK for Java
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: contract_call_local.proto
package com.hedera.hashgraph.sdk.proto;
/**
*
**
* Call a function of the given smart contract instance, giving it functionParameters as its inputs.
* This is performed locally on the particular node that the client is communicating with.
* It cannot change the state of the contract instance (and so, cannot spend anything from the instance's cryptocurrency account).
* It will not have a consensus timestamp. It cannot generate a record or a receipt. The response will contain the output
* returned by the function call. This is useful for calling getter functions, which purely read the state and don't change it.
* It is faster and cheaper than a normal call, because it is purely local to a single node.
*
* Unlike a ContractCall transaction, the node will consume the entire amount of provided gas in determining
* the fee for this query.
*
*
* Protobuf type {@code proto.ContractCallLocalQuery}
*/
public final class ContractCallLocalQuery extends
com.google.protobuf.GeneratedMessageLite<
ContractCallLocalQuery, ContractCallLocalQuery.Builder> implements
// @@protoc_insertion_point(message_implements:proto.ContractCallLocalQuery)
ContractCallLocalQueryOrBuilder {
private ContractCallLocalQuery() {
functionParameters_ = com.google.protobuf.ByteString.EMPTY;
}
public static final int HEADER_FIELD_NUMBER = 1;
private com.hedera.hashgraph.sdk.proto.QueryHeader header_;
/**
*
**
* standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither). The payment must cover the fees and all of the gas offered.
*
*
* .proto.QueryHeader header = 1;
*/
@java.lang.Override
public boolean hasHeader() {
return header_ != null;
}
/**
*
**
* standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither). The payment must cover the fees and all of the gas offered.
*
*
* .proto.QueryHeader header = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.QueryHeader getHeader() {
return header_ == null ? com.hedera.hashgraph.sdk.proto.QueryHeader.getDefaultInstance() : header_;
}
/**
*
**
* standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither). The payment must cover the fees and all of the gas offered.
*
*
* .proto.QueryHeader header = 1;
*/
private void setHeader(com.hedera.hashgraph.sdk.proto.QueryHeader value) {
value.getClass();
header_ = value;
}
/**
*
**
* standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither). The payment must cover the fees and all of the gas offered.
*
*
* .proto.QueryHeader header = 1;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeHeader(com.hedera.hashgraph.sdk.proto.QueryHeader value) {
value.getClass();
if (header_ != null &&
header_ != com.hedera.hashgraph.sdk.proto.QueryHeader.getDefaultInstance()) {
header_ =
com.hedera.hashgraph.sdk.proto.QueryHeader.newBuilder(header_).mergeFrom(value).buildPartial();
} else {
header_ = value;
}
}
/**
*
**
* standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither). The payment must cover the fees and all of the gas offered.
*
*
* .proto.QueryHeader header = 1;
*/
private void clearHeader() { header_ = null;
}
public static final int CONTRACTID_FIELD_NUMBER = 2;
private com.hedera.hashgraph.sdk.proto.ContractID contractID_;
/**
*
**
* The contract to make a static call against
*
*
* .proto.ContractID contractID = 2;
*/
@java.lang.Override
public boolean hasContractID() {
return contractID_ != null;
}
/**
*
**
* The contract to make a static call against
*
*
* .proto.ContractID contractID = 2;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ContractID getContractID() {
return contractID_ == null ? com.hedera.hashgraph.sdk.proto.ContractID.getDefaultInstance() : contractID_;
}
/**
*
**
* The contract to make a static call against
*
*
* .proto.ContractID contractID = 2;
*/
private void setContractID(com.hedera.hashgraph.sdk.proto.ContractID value) {
value.getClass();
contractID_ = value;
}
/**
*
**
* The contract to make a static call against
*
*
* .proto.ContractID contractID = 2;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeContractID(com.hedera.hashgraph.sdk.proto.ContractID value) {
value.getClass();
if (contractID_ != null &&
contractID_ != com.hedera.hashgraph.sdk.proto.ContractID.getDefaultInstance()) {
contractID_ =
com.hedera.hashgraph.sdk.proto.ContractID.newBuilder(contractID_).mergeFrom(value).buildPartial();
} else {
contractID_ = value;
}
}
/**
*
**
* The contract to make a static call against
*
*
* .proto.ContractID contractID = 2;
*/
private void clearContractID() { contractID_ = null;
}
public static final int GAS_FIELD_NUMBER = 3;
private long gas_;
/**
*
**
* The amount of gas to use for the call; all of the gas offered will be used and charged a corresponding fee
*
*
* int64 gas = 3;
* @return The gas.
*/
@java.lang.Override
public long getGas() {
return gas_;
}
/**
*
**
* The amount of gas to use for the call; all of the gas offered will be used and charged a corresponding fee
*
*
* int64 gas = 3;
* @param value The gas to set.
*/
private void setGas(long value) {
gas_ = value;
}
/**
*
**
* The amount of gas to use for the call; all of the gas offered will be used and charged a corresponding fee
*
*
* int64 gas = 3;
*/
private void clearGas() {
gas_ = 0L;
}
public static final int FUNCTIONPARAMETERS_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString functionParameters_;
/**
*
**
* which function to call, and the parameters to pass to the function
*
*
* bytes functionParameters = 4;
* @return The functionParameters.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFunctionParameters() {
return functionParameters_;
}
/**
*
**
* which function to call, and the parameters to pass to the function
*
*
* bytes functionParameters = 4;
* @param value The functionParameters to set.
*/
private void setFunctionParameters(com.google.protobuf.ByteString value) {
java.lang.Class> valueClass = value.getClass();
functionParameters_ = value;
}
/**
*
**
* which function to call, and the parameters to pass to the function
*
*
* bytes functionParameters = 4;
*/
private void clearFunctionParameters() {
functionParameters_ = getDefaultInstance().getFunctionParameters();
}
public static final int MAXRESULTSIZE_FIELD_NUMBER = 5;
private long maxResultSize_;
/**
*
**
* max number of bytes that the result might include. The run will fail if it would have returned more than this number of bytes.
*
*
* int64 maxResultSize = 5 [deprecated = true];
* @deprecated proto.ContractCallLocalQuery.maxResultSize is deprecated.
* See contract_call_local.proto;l=201
* @return The maxResultSize.
*/
@java.lang.Override
@java.lang.Deprecated public long getMaxResultSize() {
return maxResultSize_;
}
/**
*
**
* max number of bytes that the result might include. The run will fail if it would have returned more than this number of bytes.
*
*
* int64 maxResultSize = 5 [deprecated = true];
* @deprecated proto.ContractCallLocalQuery.maxResultSize is deprecated.
* See contract_call_local.proto;l=201
* @param value The maxResultSize to set.
*/
private void setMaxResultSize(long value) {
maxResultSize_ = value;
}
/**
*
**
* max number of bytes that the result might include. The run will fail if it would have returned more than this number of bytes.
*
*
* int64 maxResultSize = 5 [deprecated = true];
* @deprecated proto.ContractCallLocalQuery.maxResultSize is deprecated.
* See contract_call_local.proto;l=201
*/
private void clearMaxResultSize() {
maxResultSize_ = 0L;
}
public static final int SENDER_ID_FIELD_NUMBER = 6;
private com.hedera.hashgraph.sdk.proto.AccountID senderId_;
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
* Typically a different value than specified in the transactionId requires a valid signature
* over either the hedera transaction or foreign transaction data.
*
*
* .proto.AccountID sender_id = 6;
*/
@java.lang.Override
public boolean hasSenderId() {
return senderId_ != null;
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
* Typically a different value than specified in the transactionId requires a valid signature
* over either the hedera transaction or foreign transaction data.
*
*
* .proto.AccountID sender_id = 6;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getSenderId() {
return senderId_ == null ? com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance() : senderId_;
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
* Typically a different value than specified in the transactionId requires a valid signature
* over either the hedera transaction or foreign transaction data.
*
*
* .proto.AccountID sender_id = 6;
*/
private void setSenderId(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
senderId_ = value;
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
* Typically a different value than specified in the transactionId requires a valid signature
* over either the hedera transaction or foreign transaction data.
*
*
* .proto.AccountID sender_id = 6;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeSenderId(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
if (senderId_ != null &&
senderId_ != com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance()) {
senderId_ =
com.hedera.hashgraph.sdk.proto.AccountID.newBuilder(senderId_).mergeFrom(value).buildPartial();
} else {
senderId_ = value;
}
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
* Typically a different value than specified in the transactionId requires a valid signature
* over either the hedera transaction or foreign transaction data.
*
*
* .proto.AccountID sender_id = 6;
*/
private void clearSenderId() { senderId_ = null;
}
public static com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
*
**
* Call a function of the given smart contract instance, giving it functionParameters as its inputs.
* This is performed locally on the particular node that the client is communicating with.
* It cannot change the state of the contract instance (and so, cannot spend anything from the instance's cryptocurrency account).
* It will not have a consensus timestamp. It cannot generate a record or a receipt. The response will contain the output
* returned by the function call. This is useful for calling getter functions, which purely read the state and don't change it.
* It is faster and cheaper than a normal call, because it is purely local to a single node.
*
* Unlike a ContractCall transaction, the node will consume the entire amount of provided gas in determining
* the fee for this query.
*
*
* Protobuf type {@code proto.ContractCallLocalQuery}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery, Builder> implements
// @@protoc_insertion_point(builder_implements:proto.ContractCallLocalQuery)
com.hedera.hashgraph.sdk.proto.ContractCallLocalQueryOrBuilder {
// Construct using com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
*
**
* standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither). The payment must cover the fees and all of the gas offered.
*
*
* .proto.QueryHeader header = 1;
*/
@java.lang.Override
public boolean hasHeader() {
return instance.hasHeader();
}
/**
*
**
* standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither). The payment must cover the fees and all of the gas offered.
*
*
* .proto.QueryHeader header = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.QueryHeader getHeader() {
return instance.getHeader();
}
/**
*
**
* standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither). The payment must cover the fees and all of the gas offered.
*
*
* .proto.QueryHeader header = 1;
*/
public Builder setHeader(com.hedera.hashgraph.sdk.proto.QueryHeader value) {
copyOnWrite();
instance.setHeader(value);
return this;
}
/**
*
**
* standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither). The payment must cover the fees and all of the gas offered.
*
*
* .proto.QueryHeader header = 1;
*/
public Builder setHeader(
com.hedera.hashgraph.sdk.proto.QueryHeader.Builder builderForValue) {
copyOnWrite();
instance.setHeader(builderForValue.build());
return this;
}
/**
*
**
* standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither). The payment must cover the fees and all of the gas offered.
*
*
* .proto.QueryHeader header = 1;
*/
public Builder mergeHeader(com.hedera.hashgraph.sdk.proto.QueryHeader value) {
copyOnWrite();
instance.mergeHeader(value);
return this;
}
/**
*
**
* standard info sent from client to node, including the signed payment, and what kind of response is requested (cost, state proof, both, or neither). The payment must cover the fees and all of the gas offered.
*
*
* .proto.QueryHeader header = 1;
*/
public Builder clearHeader() { copyOnWrite();
instance.clearHeader();
return this;
}
/**
*
**
* The contract to make a static call against
*
*
* .proto.ContractID contractID = 2;
*/
@java.lang.Override
public boolean hasContractID() {
return instance.hasContractID();
}
/**
*
**
* The contract to make a static call against
*
*
* .proto.ContractID contractID = 2;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ContractID getContractID() {
return instance.getContractID();
}
/**
*
**
* The contract to make a static call against
*
*
* .proto.ContractID contractID = 2;
*/
public Builder setContractID(com.hedera.hashgraph.sdk.proto.ContractID value) {
copyOnWrite();
instance.setContractID(value);
return this;
}
/**
*
**
* The contract to make a static call against
*
*
* .proto.ContractID contractID = 2;
*/
public Builder setContractID(
com.hedera.hashgraph.sdk.proto.ContractID.Builder builderForValue) {
copyOnWrite();
instance.setContractID(builderForValue.build());
return this;
}
/**
*
**
* The contract to make a static call against
*
*
* .proto.ContractID contractID = 2;
*/
public Builder mergeContractID(com.hedera.hashgraph.sdk.proto.ContractID value) {
copyOnWrite();
instance.mergeContractID(value);
return this;
}
/**
*
**
* The contract to make a static call against
*
*
* .proto.ContractID contractID = 2;
*/
public Builder clearContractID() { copyOnWrite();
instance.clearContractID();
return this;
}
/**
*
**
* The amount of gas to use for the call; all of the gas offered will be used and charged a corresponding fee
*
*
* int64 gas = 3;
* @return The gas.
*/
@java.lang.Override
public long getGas() {
return instance.getGas();
}
/**
*
**
* The amount of gas to use for the call; all of the gas offered will be used and charged a corresponding fee
*
*
* int64 gas = 3;
* @param value The gas to set.
* @return This builder for chaining.
*/
public Builder setGas(long value) {
copyOnWrite();
instance.setGas(value);
return this;
}
/**
*
**
* The amount of gas to use for the call; all of the gas offered will be used and charged a corresponding fee
*
*
* int64 gas = 3;
* @return This builder for chaining.
*/
public Builder clearGas() {
copyOnWrite();
instance.clearGas();
return this;
}
/**
*
**
* which function to call, and the parameters to pass to the function
*
*
* bytes functionParameters = 4;
* @return The functionParameters.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFunctionParameters() {
return instance.getFunctionParameters();
}
/**
*
**
* which function to call, and the parameters to pass to the function
*
*
* bytes functionParameters = 4;
* @param value The functionParameters to set.
* @return This builder for chaining.
*/
public Builder setFunctionParameters(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setFunctionParameters(value);
return this;
}
/**
*
**
* which function to call, and the parameters to pass to the function
*
*
* bytes functionParameters = 4;
* @return This builder for chaining.
*/
public Builder clearFunctionParameters() {
copyOnWrite();
instance.clearFunctionParameters();
return this;
}
/**
*
**
* max number of bytes that the result might include. The run will fail if it would have returned more than this number of bytes.
*
*
* int64 maxResultSize = 5 [deprecated = true];
* @deprecated proto.ContractCallLocalQuery.maxResultSize is deprecated.
* See contract_call_local.proto;l=201
* @return The maxResultSize.
*/
@java.lang.Override
@java.lang.Deprecated public long getMaxResultSize() {
return instance.getMaxResultSize();
}
/**
*
**
* max number of bytes that the result might include. The run will fail if it would have returned more than this number of bytes.
*
*
* int64 maxResultSize = 5 [deprecated = true];
* @deprecated proto.ContractCallLocalQuery.maxResultSize is deprecated.
* See contract_call_local.proto;l=201
* @param value The maxResultSize to set.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder setMaxResultSize(long value) {
copyOnWrite();
instance.setMaxResultSize(value);
return this;
}
/**
*
**
* max number of bytes that the result might include. The run will fail if it would have returned more than this number of bytes.
*
*
* int64 maxResultSize = 5 [deprecated = true];
* @deprecated proto.ContractCallLocalQuery.maxResultSize is deprecated.
* See contract_call_local.proto;l=201
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder clearMaxResultSize() {
copyOnWrite();
instance.clearMaxResultSize();
return this;
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
* Typically a different value than specified in the transactionId requires a valid signature
* over either the hedera transaction or foreign transaction data.
*
*
* .proto.AccountID sender_id = 6;
*/
@java.lang.Override
public boolean hasSenderId() {
return instance.hasSenderId();
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
* Typically a different value than specified in the transactionId requires a valid signature
* over either the hedera transaction or foreign transaction data.
*
*
* .proto.AccountID sender_id = 6;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getSenderId() {
return instance.getSenderId();
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
* Typically a different value than specified in the transactionId requires a valid signature
* over either the hedera transaction or foreign transaction data.
*
*
* .proto.AccountID sender_id = 6;
*/
public Builder setSenderId(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.setSenderId(value);
return this;
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
* Typically a different value than specified in the transactionId requires a valid signature
* over either the hedera transaction or foreign transaction data.
*
*
* .proto.AccountID sender_id = 6;
*/
public Builder setSenderId(
com.hedera.hashgraph.sdk.proto.AccountID.Builder builderForValue) {
copyOnWrite();
instance.setSenderId(builderForValue.build());
return this;
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
* Typically a different value than specified in the transactionId requires a valid signature
* over either the hedera transaction or foreign transaction data.
*
*
* .proto.AccountID sender_id = 6;
*/
public Builder mergeSenderId(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.mergeSenderId(value);
return this;
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
* Typically a different value than specified in the transactionId requires a valid signature
* over either the hedera transaction or foreign transaction data.
*
*
* .proto.AccountID sender_id = 6;
*/
public Builder clearSenderId() { copyOnWrite();
instance.clearSenderId();
return this;
}
// @@protoc_insertion_point(builder_scope:proto.ContractCallLocalQuery)
}
@java.lang.Override
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery();
}
case NEW_BUILDER: {
return new Builder();
}
case BUILD_MESSAGE_INFO: {
java.lang.Object[] objects = new java.lang.Object[] {
"header_",
"contractID_",
"gas_",
"functionParameters_",
"maxResultSize_",
"senderId_",
};
java.lang.String info =
"\u0000\u0006\u0000\u0000\u0001\u0006\u0006\u0000\u0000\u0000\u0001\t\u0002\t\u0003" +
"\u0002\u0004\n\u0005\u0002\u0006\t";
return newMessageInfo(DEFAULT_INSTANCE, info, objects);
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
com.google.protobuf.Parser parser = PARSER;
if (parser == null) {
synchronized (com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery.class) {
parser = PARSER;
if (parser == null) {
parser =
new DefaultInstanceBasedParser(
DEFAULT_INSTANCE);
PARSER = parser;
}
}
}
return parser;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:proto.ContractCallLocalQuery)
private static final com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery DEFAULT_INSTANCE;
static {
ContractCallLocalQuery defaultInstance = new ContractCallLocalQuery();
// New instances are implicitly immutable so no need to make
// immutable.
DEFAULT_INSTANCE = defaultInstance;
com.google.protobuf.GeneratedMessageLite.registerDefaultInstance(
ContractCallLocalQuery.class, defaultInstance);
}
public static com.hedera.hashgraph.sdk.proto.ContractCallLocalQuery getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy