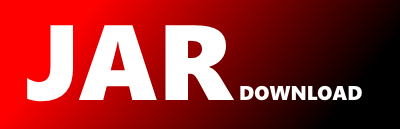
com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk-jdk7 Show documentation
Show all versions of sdk-jdk7 Show documentation
Hedera™ Hashgraph SDK for Java
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: contract_create.proto
package com.hedera.hashgraph.sdk.proto;
/**
*
**
* Start a new smart contract instance. After the instance is created, the ContractID for it is in
* the receipt, and can be retrieved by the Record or with a GetByKey query. The instance will run
* the bytecode, either stored in a previously created file or in the transaction body itself for
* small contracts.
*
*
* The constructor will be executed using the given amount of gas, and any unspent gas will be
* refunded to the paying account. Constructor inputs come from the given constructorParameters.
* - The instance will exist for autoRenewPeriod seconds. When that is reached, it will renew
* itself for another autoRenewPeriod seconds by charging its associated cryptocurrency account
* (which it creates here). If it has insufficient cryptocurrency to extend that long, it will
* extend as long as it can. If its balance is zero, the instance will be deleted.
*
* - A smart contract instance normally enforces rules, so "the code is law". For example, an
* ERC-20 contract prevents a transfer from being undone without a signature by the recipient of
* the transfer. This is always enforced if the contract instance was created with the adminKeys
* being null. But for some uses, it might be desirable to create something like an ERC-20
* contract that has a specific group of trusted individuals who can act as a "supreme court"
* with the ability to override the normal operation, when a sufficient number of them agree to
* do so. If adminKeys is not null, then they can sign a transaction that can change the state of
* the smart contract in arbitrary ways, such as to reverse a transaction that violates some
* standard of behavior that is not covered by the code itself. The admin keys can also be used
* to change the autoRenewPeriod, and change the adminKeys field itself. The API currently does
* not implement this ability. But it does allow the adminKeys field to be set and queried, and
* will in the future implement such admin abilities for any instance that has a non-null
* adminKeys.
*
* - If this constructor stores information, it is charged gas to store it. There is a fee in hbars
* to maintain that storage until the expiration time, and that fee is added as part of the
* transaction fee.
*
* - An entity (account, file, or smart contract instance) must be created in a particular realm.
* If the realmID is left null, then a new realm will be created with the given admin key. If a
* new realm has a null adminKey, then anyone can create/modify/delete entities in that realm.
* But if an admin key is given, then any transaction to create/modify/delete an entity in that
* realm must be signed by that key, though anyone can still call functions on smart contract
* instances that exist in that realm. A realm ceases to exist when everything within it has
* expired and no longer exists.
*
* - The current API ignores shardID, realmID, and newRealmAdminKey, and creates everything in
* shard 0 and realm 0, with a null key. Future versions of the API will support multiple realms
* and multiple shards.
*
* - The optional memo field can contain a string whose length is up to 100 bytes. That is the size
* after Unicode NFD then UTF-8 conversion. This field can be used to describe the smart contract.
* It could also be used for other purposes. One recommended purpose is to hold a hexadecimal
* string that is the SHA-384 hash of a PDF file containing a human-readable legal contract. Then,
* if the admin keys are the public keys of human arbitrators, they can use that legal document to
* guide their decisions during a binding arbitration tribunal, convened to consider any changes
* to the smart contract in the future. The memo field can only be changed using the admin keys.
* If there are no admin keys, then it cannot be changed after the smart contract is created.
*
* <b>Signing requirements:</b> If an admin key is set, it must sign the transaction. If an
* auto-renew account is set, its key must sign the transaction.
*
*
* Protobuf type {@code proto.ContractCreateTransactionBody}
*/
public final class ContractCreateTransactionBody extends
com.google.protobuf.GeneratedMessageLite<
ContractCreateTransactionBody, ContractCreateTransactionBody.Builder> implements
// @@protoc_insertion_point(message_implements:proto.ContractCreateTransactionBody)
ContractCreateTransactionBodyOrBuilder {
private ContractCreateTransactionBody() {
constructorParameters_ = com.google.protobuf.ByteString.EMPTY;
memo_ = "";
}
private int initcodeSourceCase_ = 0;
private java.lang.Object initcodeSource_;
public enum InitcodeSourceCase {
FILEID(1),
INITCODE(16),
INITCODESOURCE_NOT_SET(0);
private final int value;
private InitcodeSourceCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static InitcodeSourceCase valueOf(int value) {
return forNumber(value);
}
public static InitcodeSourceCase forNumber(int value) {
switch (value) {
case 1: return FILEID;
case 16: return INITCODE;
case 0: return INITCODESOURCE_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
@java.lang.Override
public InitcodeSourceCase
getInitcodeSourceCase() {
return InitcodeSourceCase.forNumber(
initcodeSourceCase_);
}
private void clearInitcodeSource() {
initcodeSourceCase_ = 0;
initcodeSource_ = null;
}
private int stakedIdCase_ = 0;
private java.lang.Object stakedId_;
public enum StakedIdCase {
STAKED_ACCOUNT_ID(17),
STAKED_NODE_ID(18),
STAKEDID_NOT_SET(0);
private final int value;
private StakedIdCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static StakedIdCase valueOf(int value) {
return forNumber(value);
}
public static StakedIdCase forNumber(int value) {
switch (value) {
case 17: return STAKED_ACCOUNT_ID;
case 18: return STAKED_NODE_ID;
case 0: return STAKEDID_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
@java.lang.Override
public StakedIdCase
getStakedIdCase() {
return StakedIdCase.forNumber(
stakedIdCase_);
}
private void clearStakedId() {
stakedIdCase_ = 0;
stakedId_ = null;
}
public static final int FILEID_FIELD_NUMBER = 1;
/**
*
**
* The file containing the smart contract initcode. A copy will be made and held by the
* contract instance, and have the same expiration time as the instance.
*
*
* .proto.FileID fileID = 1;
*/
@java.lang.Override
public boolean hasFileID() {
return initcodeSourceCase_ == 1;
}
/**
*
**
* The file containing the smart contract initcode. A copy will be made and held by the
* contract instance, and have the same expiration time as the instance.
*
*
* .proto.FileID fileID = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.FileID getFileID() {
if (initcodeSourceCase_ == 1) {
return (com.hedera.hashgraph.sdk.proto.FileID) initcodeSource_;
}
return com.hedera.hashgraph.sdk.proto.FileID.getDefaultInstance();
}
/**
*
**
* The file containing the smart contract initcode. A copy will be made and held by the
* contract instance, and have the same expiration time as the instance.
*
*
* .proto.FileID fileID = 1;
*/
private void setFileID(com.hedera.hashgraph.sdk.proto.FileID value) {
value.getClass();
initcodeSource_ = value;
initcodeSourceCase_ = 1;
}
/**
*
**
* The file containing the smart contract initcode. A copy will be made and held by the
* contract instance, and have the same expiration time as the instance.
*
*
* .proto.FileID fileID = 1;
*/
private void mergeFileID(com.hedera.hashgraph.sdk.proto.FileID value) {
value.getClass();
if (initcodeSourceCase_ == 1 &&
initcodeSource_ != com.hedera.hashgraph.sdk.proto.FileID.getDefaultInstance()) {
initcodeSource_ = com.hedera.hashgraph.sdk.proto.FileID.newBuilder((com.hedera.hashgraph.sdk.proto.FileID) initcodeSource_)
.mergeFrom(value).buildPartial();
} else {
initcodeSource_ = value;
}
initcodeSourceCase_ = 1;
}
/**
*
**
* The file containing the smart contract initcode. A copy will be made and held by the
* contract instance, and have the same expiration time as the instance.
*
*
* .proto.FileID fileID = 1;
*/
private void clearFileID() {
if (initcodeSourceCase_ == 1) {
initcodeSourceCase_ = 0;
initcodeSource_ = null;
}
}
public static final int INITCODE_FIELD_NUMBER = 16;
/**
*
**
* The bytes of the smart contract initcode. This is only useful if the smart contract init
* is less than the hedera transaction limit. In those cases fileID must be used.
*
*
* bytes initcode = 16;
* @return Whether the initcode field is set.
*/
@java.lang.Override
public boolean hasInitcode() {
return initcodeSourceCase_ == 16;
}
/**
*
**
* The bytes of the smart contract initcode. This is only useful if the smart contract init
* is less than the hedera transaction limit. In those cases fileID must be used.
*
*
* bytes initcode = 16;
* @return The initcode.
*/
@java.lang.Override
public com.google.protobuf.ByteString getInitcode() {
if (initcodeSourceCase_ == 16) {
return (com.google.protobuf.ByteString) initcodeSource_;
}
return com.google.protobuf.ByteString.EMPTY;
}
/**
*
**
* The bytes of the smart contract initcode. This is only useful if the smart contract init
* is less than the hedera transaction limit. In those cases fileID must be used.
*
*
* bytes initcode = 16;
* @param value The initcode to set.
*/
private void setInitcode(com.google.protobuf.ByteString value) {
java.lang.Class> valueClass = value.getClass();
initcodeSourceCase_ = 16;
initcodeSource_ = value;
}
/**
*
**
* The bytes of the smart contract initcode. This is only useful if the smart contract init
* is less than the hedera transaction limit. In those cases fileID must be used.
*
*
* bytes initcode = 16;
*/
private void clearInitcode() {
if (initcodeSourceCase_ == 16) {
initcodeSourceCase_ = 0;
initcodeSource_ = null;
}
}
public static final int ADMINKEY_FIELD_NUMBER = 3;
private com.hedera.hashgraph.sdk.proto.Key adminKey_;
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator to
* authorize changing the admin keys, so there can never be any admin keys for that instance.
*
*
* .proto.Key adminKey = 3;
*/
@java.lang.Override
public boolean hasAdminKey() {
return adminKey_ != null;
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator to
* authorize changing the admin keys, so there can never be any admin keys for that instance.
*
*
* .proto.Key adminKey = 3;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Key getAdminKey() {
return adminKey_ == null ? com.hedera.hashgraph.sdk.proto.Key.getDefaultInstance() : adminKey_;
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator to
* authorize changing the admin keys, so there can never be any admin keys for that instance.
*
*
* .proto.Key adminKey = 3;
*/
private void setAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
value.getClass();
adminKey_ = value;
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator to
* authorize changing the admin keys, so there can never be any admin keys for that instance.
*
*
* .proto.Key adminKey = 3;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
value.getClass();
if (adminKey_ != null &&
adminKey_ != com.hedera.hashgraph.sdk.proto.Key.getDefaultInstance()) {
adminKey_ =
com.hedera.hashgraph.sdk.proto.Key.newBuilder(adminKey_).mergeFrom(value).buildPartial();
} else {
adminKey_ = value;
}
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator to
* authorize changing the admin keys, so there can never be any admin keys for that instance.
*
*
* .proto.Key adminKey = 3;
*/
private void clearAdminKey() { adminKey_ = null;
}
public static final int GAS_FIELD_NUMBER = 4;
private long gas_;
/**
*
**
* gas to run the constructor
*
*
* int64 gas = 4;
* @return The gas.
*/
@java.lang.Override
public long getGas() {
return gas_;
}
/**
*
**
* gas to run the constructor
*
*
* int64 gas = 4;
* @param value The gas to set.
*/
private void setGas(long value) {
gas_ = value;
}
/**
*
**
* gas to run the constructor
*
*
* int64 gas = 4;
*/
private void clearGas() {
gas_ = 0L;
}
public static final int INITIALBALANCE_FIELD_NUMBER = 5;
private long initialBalance_;
/**
*
**
* initial number of tinybars to put into the cryptocurrency account associated with and owned
* by the smart contract
*
*
* int64 initialBalance = 5;
* @return The initialBalance.
*/
@java.lang.Override
public long getInitialBalance() {
return initialBalance_;
}
/**
*
**
* initial number of tinybars to put into the cryptocurrency account associated with and owned
* by the smart contract
*
*
* int64 initialBalance = 5;
* @param value The initialBalance to set.
*/
private void setInitialBalance(long value) {
initialBalance_ = value;
}
/**
*
**
* initial number of tinybars to put into the cryptocurrency account associated with and owned
* by the smart contract
*
*
* int64 initialBalance = 5;
*/
private void clearInitialBalance() {
initialBalance_ = 0L;
}
public static final int PROXYACCOUNTID_FIELD_NUMBER = 6;
private com.hedera.hashgraph.sdk.proto.AccountID proxyAccountID_;
/**
*
**
* [Deprecated] ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an
* invalid account, or is an account that isn't a node, then this account is automatically proxy
* staked to a node chosen by the network, but without earning payments. If the proxyAccountID
* account refuses to accept proxy staking , or if it is not currently running a node, then it
* will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 6 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasProxyAccountID() {
return proxyAccountID_ != null;
}
/**
*
**
* [Deprecated] ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an
* invalid account, or is an account that isn't a node, then this account is automatically proxy
* staked to a node chosen by the network, but without earning payments. If the proxyAccountID
* account refuses to accept proxy staking , or if it is not currently running a node, then it
* will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 6 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.AccountID getProxyAccountID() {
return proxyAccountID_ == null ? com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance() : proxyAccountID_;
}
/**
*
**
* [Deprecated] ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an
* invalid account, or is an account that isn't a node, then this account is automatically proxy
* staked to a node chosen by the network, but without earning payments. If the proxyAccountID
* account refuses to accept proxy staking , or if it is not currently running a node, then it
* will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 6 [deprecated = true];
*/
private void setProxyAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
proxyAccountID_ = value;
}
/**
*
**
* [Deprecated] ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an
* invalid account, or is an account that isn't a node, then this account is automatically proxy
* staked to a node chosen by the network, but without earning payments. If the proxyAccountID
* account refuses to accept proxy staking , or if it is not currently running a node, then it
* will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 6 [deprecated = true];
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeProxyAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
if (proxyAccountID_ != null &&
proxyAccountID_ != com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance()) {
proxyAccountID_ =
com.hedera.hashgraph.sdk.proto.AccountID.newBuilder(proxyAccountID_).mergeFrom(value).buildPartial();
} else {
proxyAccountID_ = value;
}
}
/**
*
**
* [Deprecated] ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an
* invalid account, or is an account that isn't a node, then this account is automatically proxy
* staked to a node chosen by the network, but without earning payments. If the proxyAccountID
* account refuses to accept proxy staking , or if it is not currently running a node, then it
* will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 6 [deprecated = true];
*/
private void clearProxyAccountID() { proxyAccountID_ = null;
}
public static final int AUTORENEWPERIOD_FIELD_NUMBER = 8;
private com.hedera.hashgraph.sdk.proto.Duration autoRenewPeriod_;
/**
*
**
* the instance will charge its account every this many seconds to renew for this long
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
@java.lang.Override
public boolean hasAutoRenewPeriod() {
return autoRenewPeriod_ != null;
}
/**
*
**
* the instance will charge its account every this many seconds to renew for this long
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Duration getAutoRenewPeriod() {
return autoRenewPeriod_ == null ? com.hedera.hashgraph.sdk.proto.Duration.getDefaultInstance() : autoRenewPeriod_;
}
/**
*
**
* the instance will charge its account every this many seconds to renew for this long
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
private void setAutoRenewPeriod(com.hedera.hashgraph.sdk.proto.Duration value) {
value.getClass();
autoRenewPeriod_ = value;
}
/**
*
**
* the instance will charge its account every this many seconds to renew for this long
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeAutoRenewPeriod(com.hedera.hashgraph.sdk.proto.Duration value) {
value.getClass();
if (autoRenewPeriod_ != null &&
autoRenewPeriod_ != com.hedera.hashgraph.sdk.proto.Duration.getDefaultInstance()) {
autoRenewPeriod_ =
com.hedera.hashgraph.sdk.proto.Duration.newBuilder(autoRenewPeriod_).mergeFrom(value).buildPartial();
} else {
autoRenewPeriod_ = value;
}
}
/**
*
**
* the instance will charge its account every this many seconds to renew for this long
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
private void clearAutoRenewPeriod() { autoRenewPeriod_ = null;
}
public static final int CONSTRUCTORPARAMETERS_FIELD_NUMBER = 9;
private com.google.protobuf.ByteString constructorParameters_;
/**
*
**
* parameters to pass to the constructor
*
*
* bytes constructorParameters = 9;
* @return The constructorParameters.
*/
@java.lang.Override
public com.google.protobuf.ByteString getConstructorParameters() {
return constructorParameters_;
}
/**
*
**
* parameters to pass to the constructor
*
*
* bytes constructorParameters = 9;
* @param value The constructorParameters to set.
*/
private void setConstructorParameters(com.google.protobuf.ByteString value) {
java.lang.Class> valueClass = value.getClass();
constructorParameters_ = value;
}
/**
*
**
* parameters to pass to the constructor
*
*
* bytes constructorParameters = 9;
*/
private void clearConstructorParameters() {
constructorParameters_ = getDefaultInstance().getConstructorParameters();
}
public static final int SHARDID_FIELD_NUMBER = 10;
private com.hedera.hashgraph.sdk.proto.ShardID shardID_;
/**
*
**
* shard in which to create this
*
*
* .proto.ShardID shardID = 10;
*/
@java.lang.Override
public boolean hasShardID() {
return shardID_ != null;
}
/**
*
**
* shard in which to create this
*
*
* .proto.ShardID shardID = 10;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ShardID getShardID() {
return shardID_ == null ? com.hedera.hashgraph.sdk.proto.ShardID.getDefaultInstance() : shardID_;
}
/**
*
**
* shard in which to create this
*
*
* .proto.ShardID shardID = 10;
*/
private void setShardID(com.hedera.hashgraph.sdk.proto.ShardID value) {
value.getClass();
shardID_ = value;
}
/**
*
**
* shard in which to create this
*
*
* .proto.ShardID shardID = 10;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeShardID(com.hedera.hashgraph.sdk.proto.ShardID value) {
value.getClass();
if (shardID_ != null &&
shardID_ != com.hedera.hashgraph.sdk.proto.ShardID.getDefaultInstance()) {
shardID_ =
com.hedera.hashgraph.sdk.proto.ShardID.newBuilder(shardID_).mergeFrom(value).buildPartial();
} else {
shardID_ = value;
}
}
/**
*
**
* shard in which to create this
*
*
* .proto.ShardID shardID = 10;
*/
private void clearShardID() { shardID_ = null;
}
public static final int REALMID_FIELD_NUMBER = 11;
private com.hedera.hashgraph.sdk.proto.RealmID realmID_;
/**
*
**
* realm in which to create this (leave this null to create a new realm)
*
*
* .proto.RealmID realmID = 11;
*/
@java.lang.Override
public boolean hasRealmID() {
return realmID_ != null;
}
/**
*
**
* realm in which to create this (leave this null to create a new realm)
*
*
* .proto.RealmID realmID = 11;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.RealmID getRealmID() {
return realmID_ == null ? com.hedera.hashgraph.sdk.proto.RealmID.getDefaultInstance() : realmID_;
}
/**
*
**
* realm in which to create this (leave this null to create a new realm)
*
*
* .proto.RealmID realmID = 11;
*/
private void setRealmID(com.hedera.hashgraph.sdk.proto.RealmID value) {
value.getClass();
realmID_ = value;
}
/**
*
**
* realm in which to create this (leave this null to create a new realm)
*
*
* .proto.RealmID realmID = 11;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeRealmID(com.hedera.hashgraph.sdk.proto.RealmID value) {
value.getClass();
if (realmID_ != null &&
realmID_ != com.hedera.hashgraph.sdk.proto.RealmID.getDefaultInstance()) {
realmID_ =
com.hedera.hashgraph.sdk.proto.RealmID.newBuilder(realmID_).mergeFrom(value).buildPartial();
} else {
realmID_ = value;
}
}
/**
*
**
* realm in which to create this (leave this null to create a new realm)
*
*
* .proto.RealmID realmID = 11;
*/
private void clearRealmID() { realmID_ = null;
}
public static final int NEWREALMADMINKEY_FIELD_NUMBER = 12;
private com.hedera.hashgraph.sdk.proto.Key newRealmAdminKey_;
/**
*
**
* if realmID is null, then this the admin key for the new realm that will be created
*
*
* .proto.Key newRealmAdminKey = 12;
*/
@java.lang.Override
public boolean hasNewRealmAdminKey() {
return newRealmAdminKey_ != null;
}
/**
*
**
* if realmID is null, then this the admin key for the new realm that will be created
*
*
* .proto.Key newRealmAdminKey = 12;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Key getNewRealmAdminKey() {
return newRealmAdminKey_ == null ? com.hedera.hashgraph.sdk.proto.Key.getDefaultInstance() : newRealmAdminKey_;
}
/**
*
**
* if realmID is null, then this the admin key for the new realm that will be created
*
*
* .proto.Key newRealmAdminKey = 12;
*/
private void setNewRealmAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
value.getClass();
newRealmAdminKey_ = value;
}
/**
*
**
* if realmID is null, then this the admin key for the new realm that will be created
*
*
* .proto.Key newRealmAdminKey = 12;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeNewRealmAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
value.getClass();
if (newRealmAdminKey_ != null &&
newRealmAdminKey_ != com.hedera.hashgraph.sdk.proto.Key.getDefaultInstance()) {
newRealmAdminKey_ =
com.hedera.hashgraph.sdk.proto.Key.newBuilder(newRealmAdminKey_).mergeFrom(value).buildPartial();
} else {
newRealmAdminKey_ = value;
}
}
/**
*
**
* if realmID is null, then this the admin key for the new realm that will be created
*
*
* .proto.Key newRealmAdminKey = 12;
*/
private void clearNewRealmAdminKey() { newRealmAdminKey_ = null;
}
public static final int MEMO_FIELD_NUMBER = 13;
private java.lang.String memo_;
/**
*
**
* the memo that was submitted as part of the contract (max 100 bytes)
*
*
* string memo = 13;
* @return The memo.
*/
@java.lang.Override
public java.lang.String getMemo() {
return memo_;
}
/**
*
**
* the memo that was submitted as part of the contract (max 100 bytes)
*
*
* string memo = 13;
* @return The bytes for memo.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMemoBytes() {
return com.google.protobuf.ByteString.copyFromUtf8(memo_);
}
/**
*
**
* the memo that was submitted as part of the contract (max 100 bytes)
*
*
* string memo = 13;
* @param value The memo to set.
*/
private void setMemo(
java.lang.String value) {
java.lang.Class> valueClass = value.getClass();
memo_ = value;
}
/**
*
**
* the memo that was submitted as part of the contract (max 100 bytes)
*
*
* string memo = 13;
*/
private void clearMemo() {
memo_ = getDefaultInstance().getMemo();
}
/**
*
**
* the memo that was submitted as part of the contract (max 100 bytes)
*
*
* string memo = 13;
* @param value The bytes for memo to set.
*/
private void setMemoBytes(
com.google.protobuf.ByteString value) {
checkByteStringIsUtf8(value);
memo_ = value.toStringUtf8();
}
public static final int MAX_AUTOMATIC_TOKEN_ASSOCIATIONS_FIELD_NUMBER = 14;
private int maxAutomaticTokenAssociations_;
/**
*
**
* The maximum number of tokens that this contract can be automatically associated
* with (i.e., receive air-drops from).
*
*
* int32 max_automatic_token_associations = 14;
* @return The maxAutomaticTokenAssociations.
*/
@java.lang.Override
public int getMaxAutomaticTokenAssociations() {
return maxAutomaticTokenAssociations_;
}
/**
*
**
* The maximum number of tokens that this contract can be automatically associated
* with (i.e., receive air-drops from).
*
*
* int32 max_automatic_token_associations = 14;
* @param value The maxAutomaticTokenAssociations to set.
*/
private void setMaxAutomaticTokenAssociations(int value) {
maxAutomaticTokenAssociations_ = value;
}
/**
*
**
* The maximum number of tokens that this contract can be automatically associated
* with (i.e., receive air-drops from).
*
*
* int32 max_automatic_token_associations = 14;
*/
private void clearMaxAutomaticTokenAssociations() {
maxAutomaticTokenAssociations_ = 0;
}
public static final int AUTO_RENEW_ACCOUNT_ID_FIELD_NUMBER = 15;
private com.hedera.hashgraph.sdk.proto.AccountID autoRenewAccountId_;
/**
*
**
* An account to charge for auto-renewal of this contract. If not set, or set to an
* account with zero hbar balance, the contract's own hbar balance will be used to
* cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 15;
*/
@java.lang.Override
public boolean hasAutoRenewAccountId() {
return autoRenewAccountId_ != null;
}
/**
*
**
* An account to charge for auto-renewal of this contract. If not set, or set to an
* account with zero hbar balance, the contract's own hbar balance will be used to
* cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 15;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getAutoRenewAccountId() {
return autoRenewAccountId_ == null ? com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance() : autoRenewAccountId_;
}
/**
*
**
* An account to charge for auto-renewal of this contract. If not set, or set to an
* account with zero hbar balance, the contract's own hbar balance will be used to
* cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 15;
*/
private void setAutoRenewAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
autoRenewAccountId_ = value;
}
/**
*
**
* An account to charge for auto-renewal of this contract. If not set, or set to an
* account with zero hbar balance, the contract's own hbar balance will be used to
* cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 15;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeAutoRenewAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
if (autoRenewAccountId_ != null &&
autoRenewAccountId_ != com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance()) {
autoRenewAccountId_ =
com.hedera.hashgraph.sdk.proto.AccountID.newBuilder(autoRenewAccountId_).mergeFrom(value).buildPartial();
} else {
autoRenewAccountId_ = value;
}
}
/**
*
**
* An account to charge for auto-renewal of this contract. If not set, or set to an
* account with zero hbar balance, the contract's own hbar balance will be used to
* cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 15;
*/
private void clearAutoRenewAccountId() { autoRenewAccountId_ = null;
}
public static final int STAKED_ACCOUNT_ID_FIELD_NUMBER = 17;
/**
*
**
* ID of the account to which this contract is staking.
*
*
* .proto.AccountID staked_account_id = 17;
*/
@java.lang.Override
public boolean hasStakedAccountId() {
return stakedIdCase_ == 17;
}
/**
*
**
* ID of the account to which this contract is staking.
*
*
* .proto.AccountID staked_account_id = 17;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getStakedAccountId() {
if (stakedIdCase_ == 17) {
return (com.hedera.hashgraph.sdk.proto.AccountID) stakedId_;
}
return com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance();
}
/**
*
**
* ID of the account to which this contract is staking.
*
*
* .proto.AccountID staked_account_id = 17;
*/
private void setStakedAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
stakedId_ = value;
stakedIdCase_ = 17;
}
/**
*
**
* ID of the account to which this contract is staking.
*
*
* .proto.AccountID staked_account_id = 17;
*/
private void mergeStakedAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
if (stakedIdCase_ == 17 &&
stakedId_ != com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance()) {
stakedId_ = com.hedera.hashgraph.sdk.proto.AccountID.newBuilder((com.hedera.hashgraph.sdk.proto.AccountID) stakedId_)
.mergeFrom(value).buildPartial();
} else {
stakedId_ = value;
}
stakedIdCase_ = 17;
}
/**
*
**
* ID of the account to which this contract is staking.
*
*
* .proto.AccountID staked_account_id = 17;
*/
private void clearStakedAccountId() {
if (stakedIdCase_ == 17) {
stakedIdCase_ = 0;
stakedId_ = null;
}
}
public static final int STAKED_NODE_ID_FIELD_NUMBER = 18;
/**
*
**
* ID of the node this contract is staked to.
*
*
* int64 staked_node_id = 18;
* @return Whether the stakedNodeId field is set.
*/
@java.lang.Override
public boolean hasStakedNodeId() {
return stakedIdCase_ == 18;
}
/**
*
**
* ID of the node this contract is staked to.
*
*
* int64 staked_node_id = 18;
* @return The stakedNodeId.
*/
@java.lang.Override
public long getStakedNodeId() {
if (stakedIdCase_ == 18) {
return (java.lang.Long) stakedId_;
}
return 0L;
}
/**
*
**
* ID of the node this contract is staked to.
*
*
* int64 staked_node_id = 18;
* @param value The stakedNodeId to set.
*/
private void setStakedNodeId(long value) {
stakedIdCase_ = 18;
stakedId_ = value;
}
/**
*
**
* ID of the node this contract is staked to.
*
*
* int64 staked_node_id = 18;
*/
private void clearStakedNodeId() {
if (stakedIdCase_ == 18) {
stakedIdCase_ = 0;
stakedId_ = null;
}
}
public static final int DECLINE_REWARD_FIELD_NUMBER = 19;
private boolean declineReward_;
/**
*
**
* If true, the contract declines receiving a staking reward. The default value is false.
*
*
* bool decline_reward = 19;
* @return The declineReward.
*/
@java.lang.Override
public boolean getDeclineReward() {
return declineReward_;
}
/**
*
**
* If true, the contract declines receiving a staking reward. The default value is false.
*
*
* bool decline_reward = 19;
* @param value The declineReward to set.
*/
private void setDeclineReward(boolean value) {
declineReward_ = value;
}
/**
*
**
* If true, the contract declines receiving a staking reward. The default value is false.
*
*
* bool decline_reward = 19;
*/
private void clearDeclineReward() {
declineReward_ = false;
}
public static com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
*
**
* Start a new smart contract instance. After the instance is created, the ContractID for it is in
* the receipt, and can be retrieved by the Record or with a GetByKey query. The instance will run
* the bytecode, either stored in a previously created file or in the transaction body itself for
* small contracts.
*
*
* The constructor will be executed using the given amount of gas, and any unspent gas will be
* refunded to the paying account. Constructor inputs come from the given constructorParameters.
* - The instance will exist for autoRenewPeriod seconds. When that is reached, it will renew
* itself for another autoRenewPeriod seconds by charging its associated cryptocurrency account
* (which it creates here). If it has insufficient cryptocurrency to extend that long, it will
* extend as long as it can. If its balance is zero, the instance will be deleted.
*
* - A smart contract instance normally enforces rules, so "the code is law". For example, an
* ERC-20 contract prevents a transfer from being undone without a signature by the recipient of
* the transfer. This is always enforced if the contract instance was created with the adminKeys
* being null. But for some uses, it might be desirable to create something like an ERC-20
* contract that has a specific group of trusted individuals who can act as a "supreme court"
* with the ability to override the normal operation, when a sufficient number of them agree to
* do so. If adminKeys is not null, then they can sign a transaction that can change the state of
* the smart contract in arbitrary ways, such as to reverse a transaction that violates some
* standard of behavior that is not covered by the code itself. The admin keys can also be used
* to change the autoRenewPeriod, and change the adminKeys field itself. The API currently does
* not implement this ability. But it does allow the adminKeys field to be set and queried, and
* will in the future implement such admin abilities for any instance that has a non-null
* adminKeys.
*
* - If this constructor stores information, it is charged gas to store it. There is a fee in hbars
* to maintain that storage until the expiration time, and that fee is added as part of the
* transaction fee.
*
* - An entity (account, file, or smart contract instance) must be created in a particular realm.
* If the realmID is left null, then a new realm will be created with the given admin key. If a
* new realm has a null adminKey, then anyone can create/modify/delete entities in that realm.
* But if an admin key is given, then any transaction to create/modify/delete an entity in that
* realm must be signed by that key, though anyone can still call functions on smart contract
* instances that exist in that realm. A realm ceases to exist when everything within it has
* expired and no longer exists.
*
* - The current API ignores shardID, realmID, and newRealmAdminKey, and creates everything in
* shard 0 and realm 0, with a null key. Future versions of the API will support multiple realms
* and multiple shards.
*
* - The optional memo field can contain a string whose length is up to 100 bytes. That is the size
* after Unicode NFD then UTF-8 conversion. This field can be used to describe the smart contract.
* It could also be used for other purposes. One recommended purpose is to hold a hexadecimal
* string that is the SHA-384 hash of a PDF file containing a human-readable legal contract. Then,
* if the admin keys are the public keys of human arbitrators, they can use that legal document to
* guide their decisions during a binding arbitration tribunal, convened to consider any changes
* to the smart contract in the future. The memo field can only be changed using the admin keys.
* If there are no admin keys, then it cannot be changed after the smart contract is created.
*
* <b>Signing requirements:</b> If an admin key is set, it must sign the transaction. If an
* auto-renew account is set, its key must sign the transaction.
*
*
* Protobuf type {@code proto.ContractCreateTransactionBody}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody, Builder> implements
// @@protoc_insertion_point(builder_implements:proto.ContractCreateTransactionBody)
com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBodyOrBuilder {
// Construct using com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
@java.lang.Override
public InitcodeSourceCase
getInitcodeSourceCase() {
return instance.getInitcodeSourceCase();
}
public Builder clearInitcodeSource() {
copyOnWrite();
instance.clearInitcodeSource();
return this;
}
@java.lang.Override
public StakedIdCase
getStakedIdCase() {
return instance.getStakedIdCase();
}
public Builder clearStakedId() {
copyOnWrite();
instance.clearStakedId();
return this;
}
/**
*
**
* The file containing the smart contract initcode. A copy will be made and held by the
* contract instance, and have the same expiration time as the instance.
*
*
* .proto.FileID fileID = 1;
*/
@java.lang.Override
public boolean hasFileID() {
return instance.hasFileID();
}
/**
*
**
* The file containing the smart contract initcode. A copy will be made and held by the
* contract instance, and have the same expiration time as the instance.
*
*
* .proto.FileID fileID = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.FileID getFileID() {
return instance.getFileID();
}
/**
*
**
* The file containing the smart contract initcode. A copy will be made and held by the
* contract instance, and have the same expiration time as the instance.
*
*
* .proto.FileID fileID = 1;
*/
public Builder setFileID(com.hedera.hashgraph.sdk.proto.FileID value) {
copyOnWrite();
instance.setFileID(value);
return this;
}
/**
*
**
* The file containing the smart contract initcode. A copy will be made and held by the
* contract instance, and have the same expiration time as the instance.
*
*
* .proto.FileID fileID = 1;
*/
public Builder setFileID(
com.hedera.hashgraph.sdk.proto.FileID.Builder builderForValue) {
copyOnWrite();
instance.setFileID(builderForValue.build());
return this;
}
/**
*
**
* The file containing the smart contract initcode. A copy will be made and held by the
* contract instance, and have the same expiration time as the instance.
*
*
* .proto.FileID fileID = 1;
*/
public Builder mergeFileID(com.hedera.hashgraph.sdk.proto.FileID value) {
copyOnWrite();
instance.mergeFileID(value);
return this;
}
/**
*
**
* The file containing the smart contract initcode. A copy will be made and held by the
* contract instance, and have the same expiration time as the instance.
*
*
* .proto.FileID fileID = 1;
*/
public Builder clearFileID() {
copyOnWrite();
instance.clearFileID();
return this;
}
/**
*
**
* The bytes of the smart contract initcode. This is only useful if the smart contract init
* is less than the hedera transaction limit. In those cases fileID must be used.
*
*
* bytes initcode = 16;
* @return Whether the initcode field is set.
*/
@java.lang.Override
public boolean hasInitcode() {
return instance.hasInitcode();
}
/**
*
**
* The bytes of the smart contract initcode. This is only useful if the smart contract init
* is less than the hedera transaction limit. In those cases fileID must be used.
*
*
* bytes initcode = 16;
* @return The initcode.
*/
@java.lang.Override
public com.google.protobuf.ByteString getInitcode() {
return instance.getInitcode();
}
/**
*
**
* The bytes of the smart contract initcode. This is only useful if the smart contract init
* is less than the hedera transaction limit. In those cases fileID must be used.
*
*
* bytes initcode = 16;
* @param value The initcode to set.
* @return This builder for chaining.
*/
public Builder setInitcode(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setInitcode(value);
return this;
}
/**
*
**
* The bytes of the smart contract initcode. This is only useful if the smart contract init
* is less than the hedera transaction limit. In those cases fileID must be used.
*
*
* bytes initcode = 16;
* @return This builder for chaining.
*/
public Builder clearInitcode() {
copyOnWrite();
instance.clearInitcode();
return this;
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator to
* authorize changing the admin keys, so there can never be any admin keys for that instance.
*
*
* .proto.Key adminKey = 3;
*/
@java.lang.Override
public boolean hasAdminKey() {
return instance.hasAdminKey();
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator to
* authorize changing the admin keys, so there can never be any admin keys for that instance.
*
*
* .proto.Key adminKey = 3;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Key getAdminKey() {
return instance.getAdminKey();
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator to
* authorize changing the admin keys, so there can never be any admin keys for that instance.
*
*
* .proto.Key adminKey = 3;
*/
public Builder setAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
copyOnWrite();
instance.setAdminKey(value);
return this;
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator to
* authorize changing the admin keys, so there can never be any admin keys for that instance.
*
*
* .proto.Key adminKey = 3;
*/
public Builder setAdminKey(
com.hedera.hashgraph.sdk.proto.Key.Builder builderForValue) {
copyOnWrite();
instance.setAdminKey(builderForValue.build());
return this;
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator to
* authorize changing the admin keys, so there can never be any admin keys for that instance.
*
*
* .proto.Key adminKey = 3;
*/
public Builder mergeAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
copyOnWrite();
instance.mergeAdminKey(value);
return this;
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator to
* authorize changing the admin keys, so there can never be any admin keys for that instance.
*
*
* .proto.Key adminKey = 3;
*/
public Builder clearAdminKey() { copyOnWrite();
instance.clearAdminKey();
return this;
}
/**
*
**
* gas to run the constructor
*
*
* int64 gas = 4;
* @return The gas.
*/
@java.lang.Override
public long getGas() {
return instance.getGas();
}
/**
*
**
* gas to run the constructor
*
*
* int64 gas = 4;
* @param value The gas to set.
* @return This builder for chaining.
*/
public Builder setGas(long value) {
copyOnWrite();
instance.setGas(value);
return this;
}
/**
*
**
* gas to run the constructor
*
*
* int64 gas = 4;
* @return This builder for chaining.
*/
public Builder clearGas() {
copyOnWrite();
instance.clearGas();
return this;
}
/**
*
**
* initial number of tinybars to put into the cryptocurrency account associated with and owned
* by the smart contract
*
*
* int64 initialBalance = 5;
* @return The initialBalance.
*/
@java.lang.Override
public long getInitialBalance() {
return instance.getInitialBalance();
}
/**
*
**
* initial number of tinybars to put into the cryptocurrency account associated with and owned
* by the smart contract
*
*
* int64 initialBalance = 5;
* @param value The initialBalance to set.
* @return This builder for chaining.
*/
public Builder setInitialBalance(long value) {
copyOnWrite();
instance.setInitialBalance(value);
return this;
}
/**
*
**
* initial number of tinybars to put into the cryptocurrency account associated with and owned
* by the smart contract
*
*
* int64 initialBalance = 5;
* @return This builder for chaining.
*/
public Builder clearInitialBalance() {
copyOnWrite();
instance.clearInitialBalance();
return this;
}
/**
*
**
* [Deprecated] ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an
* invalid account, or is an account that isn't a node, then this account is automatically proxy
* staked to a node chosen by the network, but without earning payments. If the proxyAccountID
* account refuses to accept proxy staking , or if it is not currently running a node, then it
* will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 6 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasProxyAccountID() {
return instance.hasProxyAccountID();
}
/**
*
**
* [Deprecated] ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an
* invalid account, or is an account that isn't a node, then this account is automatically proxy
* staked to a node chosen by the network, but without earning payments. If the proxyAccountID
* account refuses to accept proxy staking , or if it is not currently running a node, then it
* will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 6 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.AccountID getProxyAccountID() {
return instance.getProxyAccountID();
}
/**
*
**
* [Deprecated] ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an
* invalid account, or is an account that isn't a node, then this account is automatically proxy
* staked to a node chosen by the network, but without earning payments. If the proxyAccountID
* account refuses to accept proxy staking , or if it is not currently running a node, then it
* will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 6 [deprecated = true];
*/
@java.lang.Deprecated public Builder setProxyAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.setProxyAccountID(value);
return this;
}
/**
*
**
* [Deprecated] ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an
* invalid account, or is an account that isn't a node, then this account is automatically proxy
* staked to a node chosen by the network, but without earning payments. If the proxyAccountID
* account refuses to accept proxy staking , or if it is not currently running a node, then it
* will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 6 [deprecated = true];
*/
@java.lang.Deprecated public Builder setProxyAccountID(
com.hedera.hashgraph.sdk.proto.AccountID.Builder builderForValue) {
copyOnWrite();
instance.setProxyAccountID(builderForValue.build());
return this;
}
/**
*
**
* [Deprecated] ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an
* invalid account, or is an account that isn't a node, then this account is automatically proxy
* staked to a node chosen by the network, but without earning payments. If the proxyAccountID
* account refuses to accept proxy staking , or if it is not currently running a node, then it
* will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 6 [deprecated = true];
*/
@java.lang.Deprecated public Builder mergeProxyAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.mergeProxyAccountID(value);
return this;
}
/**
*
**
* [Deprecated] ID of the account to which this account is proxy staked. If proxyAccountID is null, or is an
* invalid account, or is an account that isn't a node, then this account is automatically proxy
* staked to a node chosen by the network, but without earning payments. If the proxyAccountID
* account refuses to accept proxy staking , or if it is not currently running a node, then it
* will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxyAccountID = 6 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearProxyAccountID() { copyOnWrite();
instance.clearProxyAccountID();
return this;
}
/**
*
**
* the instance will charge its account every this many seconds to renew for this long
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
@java.lang.Override
public boolean hasAutoRenewPeriod() {
return instance.hasAutoRenewPeriod();
}
/**
*
**
* the instance will charge its account every this many seconds to renew for this long
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Duration getAutoRenewPeriod() {
return instance.getAutoRenewPeriod();
}
/**
*
**
* the instance will charge its account every this many seconds to renew for this long
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
public Builder setAutoRenewPeriod(com.hedera.hashgraph.sdk.proto.Duration value) {
copyOnWrite();
instance.setAutoRenewPeriod(value);
return this;
}
/**
*
**
* the instance will charge its account every this many seconds to renew for this long
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
public Builder setAutoRenewPeriod(
com.hedera.hashgraph.sdk.proto.Duration.Builder builderForValue) {
copyOnWrite();
instance.setAutoRenewPeriod(builderForValue.build());
return this;
}
/**
*
**
* the instance will charge its account every this many seconds to renew for this long
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
public Builder mergeAutoRenewPeriod(com.hedera.hashgraph.sdk.proto.Duration value) {
copyOnWrite();
instance.mergeAutoRenewPeriod(value);
return this;
}
/**
*
**
* the instance will charge its account every this many seconds to renew for this long
*
*
* .proto.Duration autoRenewPeriod = 8;
*/
public Builder clearAutoRenewPeriod() { copyOnWrite();
instance.clearAutoRenewPeriod();
return this;
}
/**
*
**
* parameters to pass to the constructor
*
*
* bytes constructorParameters = 9;
* @return The constructorParameters.
*/
@java.lang.Override
public com.google.protobuf.ByteString getConstructorParameters() {
return instance.getConstructorParameters();
}
/**
*
**
* parameters to pass to the constructor
*
*
* bytes constructorParameters = 9;
* @param value The constructorParameters to set.
* @return This builder for chaining.
*/
public Builder setConstructorParameters(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setConstructorParameters(value);
return this;
}
/**
*
**
* parameters to pass to the constructor
*
*
* bytes constructorParameters = 9;
* @return This builder for chaining.
*/
public Builder clearConstructorParameters() {
copyOnWrite();
instance.clearConstructorParameters();
return this;
}
/**
*
**
* shard in which to create this
*
*
* .proto.ShardID shardID = 10;
*/
@java.lang.Override
public boolean hasShardID() {
return instance.hasShardID();
}
/**
*
**
* shard in which to create this
*
*
* .proto.ShardID shardID = 10;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ShardID getShardID() {
return instance.getShardID();
}
/**
*
**
* shard in which to create this
*
*
* .proto.ShardID shardID = 10;
*/
public Builder setShardID(com.hedera.hashgraph.sdk.proto.ShardID value) {
copyOnWrite();
instance.setShardID(value);
return this;
}
/**
*
**
* shard in which to create this
*
*
* .proto.ShardID shardID = 10;
*/
public Builder setShardID(
com.hedera.hashgraph.sdk.proto.ShardID.Builder builderForValue) {
copyOnWrite();
instance.setShardID(builderForValue.build());
return this;
}
/**
*
**
* shard in which to create this
*
*
* .proto.ShardID shardID = 10;
*/
public Builder mergeShardID(com.hedera.hashgraph.sdk.proto.ShardID value) {
copyOnWrite();
instance.mergeShardID(value);
return this;
}
/**
*
**
* shard in which to create this
*
*
* .proto.ShardID shardID = 10;
*/
public Builder clearShardID() { copyOnWrite();
instance.clearShardID();
return this;
}
/**
*
**
* realm in which to create this (leave this null to create a new realm)
*
*
* .proto.RealmID realmID = 11;
*/
@java.lang.Override
public boolean hasRealmID() {
return instance.hasRealmID();
}
/**
*
**
* realm in which to create this (leave this null to create a new realm)
*
*
* .proto.RealmID realmID = 11;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.RealmID getRealmID() {
return instance.getRealmID();
}
/**
*
**
* realm in which to create this (leave this null to create a new realm)
*
*
* .proto.RealmID realmID = 11;
*/
public Builder setRealmID(com.hedera.hashgraph.sdk.proto.RealmID value) {
copyOnWrite();
instance.setRealmID(value);
return this;
}
/**
*
**
* realm in which to create this (leave this null to create a new realm)
*
*
* .proto.RealmID realmID = 11;
*/
public Builder setRealmID(
com.hedera.hashgraph.sdk.proto.RealmID.Builder builderForValue) {
copyOnWrite();
instance.setRealmID(builderForValue.build());
return this;
}
/**
*
**
* realm in which to create this (leave this null to create a new realm)
*
*
* .proto.RealmID realmID = 11;
*/
public Builder mergeRealmID(com.hedera.hashgraph.sdk.proto.RealmID value) {
copyOnWrite();
instance.mergeRealmID(value);
return this;
}
/**
*
**
* realm in which to create this (leave this null to create a new realm)
*
*
* .proto.RealmID realmID = 11;
*/
public Builder clearRealmID() { copyOnWrite();
instance.clearRealmID();
return this;
}
/**
*
**
* if realmID is null, then this the admin key for the new realm that will be created
*
*
* .proto.Key newRealmAdminKey = 12;
*/
@java.lang.Override
public boolean hasNewRealmAdminKey() {
return instance.hasNewRealmAdminKey();
}
/**
*
**
* if realmID is null, then this the admin key for the new realm that will be created
*
*
* .proto.Key newRealmAdminKey = 12;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Key getNewRealmAdminKey() {
return instance.getNewRealmAdminKey();
}
/**
*
**
* if realmID is null, then this the admin key for the new realm that will be created
*
*
* .proto.Key newRealmAdminKey = 12;
*/
public Builder setNewRealmAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
copyOnWrite();
instance.setNewRealmAdminKey(value);
return this;
}
/**
*
**
* if realmID is null, then this the admin key for the new realm that will be created
*
*
* .proto.Key newRealmAdminKey = 12;
*/
public Builder setNewRealmAdminKey(
com.hedera.hashgraph.sdk.proto.Key.Builder builderForValue) {
copyOnWrite();
instance.setNewRealmAdminKey(builderForValue.build());
return this;
}
/**
*
**
* if realmID is null, then this the admin key for the new realm that will be created
*
*
* .proto.Key newRealmAdminKey = 12;
*/
public Builder mergeNewRealmAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
copyOnWrite();
instance.mergeNewRealmAdminKey(value);
return this;
}
/**
*
**
* if realmID is null, then this the admin key for the new realm that will be created
*
*
* .proto.Key newRealmAdminKey = 12;
*/
public Builder clearNewRealmAdminKey() { copyOnWrite();
instance.clearNewRealmAdminKey();
return this;
}
/**
*
**
* the memo that was submitted as part of the contract (max 100 bytes)
*
*
* string memo = 13;
* @return The memo.
*/
@java.lang.Override
public java.lang.String getMemo() {
return instance.getMemo();
}
/**
*
**
* the memo that was submitted as part of the contract (max 100 bytes)
*
*
* string memo = 13;
* @return The bytes for memo.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMemoBytes() {
return instance.getMemoBytes();
}
/**
*
**
* the memo that was submitted as part of the contract (max 100 bytes)
*
*
* string memo = 13;
* @param value The memo to set.
* @return This builder for chaining.
*/
public Builder setMemo(
java.lang.String value) {
copyOnWrite();
instance.setMemo(value);
return this;
}
/**
*
**
* the memo that was submitted as part of the contract (max 100 bytes)
*
*
* string memo = 13;
* @return This builder for chaining.
*/
public Builder clearMemo() {
copyOnWrite();
instance.clearMemo();
return this;
}
/**
*
**
* the memo that was submitted as part of the contract (max 100 bytes)
*
*
* string memo = 13;
* @param value The bytes for memo to set.
* @return This builder for chaining.
*/
public Builder setMemoBytes(
com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setMemoBytes(value);
return this;
}
/**
*
**
* The maximum number of tokens that this contract can be automatically associated
* with (i.e., receive air-drops from).
*
*
* int32 max_automatic_token_associations = 14;
* @return The maxAutomaticTokenAssociations.
*/
@java.lang.Override
public int getMaxAutomaticTokenAssociations() {
return instance.getMaxAutomaticTokenAssociations();
}
/**
*
**
* The maximum number of tokens that this contract can be automatically associated
* with (i.e., receive air-drops from).
*
*
* int32 max_automatic_token_associations = 14;
* @param value The maxAutomaticTokenAssociations to set.
* @return This builder for chaining.
*/
public Builder setMaxAutomaticTokenAssociations(int value) {
copyOnWrite();
instance.setMaxAutomaticTokenAssociations(value);
return this;
}
/**
*
**
* The maximum number of tokens that this contract can be automatically associated
* with (i.e., receive air-drops from).
*
*
* int32 max_automatic_token_associations = 14;
* @return This builder for chaining.
*/
public Builder clearMaxAutomaticTokenAssociations() {
copyOnWrite();
instance.clearMaxAutomaticTokenAssociations();
return this;
}
/**
*
**
* An account to charge for auto-renewal of this contract. If not set, or set to an
* account with zero hbar balance, the contract's own hbar balance will be used to
* cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 15;
*/
@java.lang.Override
public boolean hasAutoRenewAccountId() {
return instance.hasAutoRenewAccountId();
}
/**
*
**
* An account to charge for auto-renewal of this contract. If not set, or set to an
* account with zero hbar balance, the contract's own hbar balance will be used to
* cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 15;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getAutoRenewAccountId() {
return instance.getAutoRenewAccountId();
}
/**
*
**
* An account to charge for auto-renewal of this contract. If not set, or set to an
* account with zero hbar balance, the contract's own hbar balance will be used to
* cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 15;
*/
public Builder setAutoRenewAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.setAutoRenewAccountId(value);
return this;
}
/**
*
**
* An account to charge for auto-renewal of this contract. If not set, or set to an
* account with zero hbar balance, the contract's own hbar balance will be used to
* cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 15;
*/
public Builder setAutoRenewAccountId(
com.hedera.hashgraph.sdk.proto.AccountID.Builder builderForValue) {
copyOnWrite();
instance.setAutoRenewAccountId(builderForValue.build());
return this;
}
/**
*
**
* An account to charge for auto-renewal of this contract. If not set, or set to an
* account with zero hbar balance, the contract's own hbar balance will be used to
* cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 15;
*/
public Builder mergeAutoRenewAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.mergeAutoRenewAccountId(value);
return this;
}
/**
*
**
* An account to charge for auto-renewal of this contract. If not set, or set to an
* account with zero hbar balance, the contract's own hbar balance will be used to
* cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 15;
*/
public Builder clearAutoRenewAccountId() { copyOnWrite();
instance.clearAutoRenewAccountId();
return this;
}
/**
*
**
* ID of the account to which this contract is staking.
*
*
* .proto.AccountID staked_account_id = 17;
*/
@java.lang.Override
public boolean hasStakedAccountId() {
return instance.hasStakedAccountId();
}
/**
*
**
* ID of the account to which this contract is staking.
*
*
* .proto.AccountID staked_account_id = 17;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getStakedAccountId() {
return instance.getStakedAccountId();
}
/**
*
**
* ID of the account to which this contract is staking.
*
*
* .proto.AccountID staked_account_id = 17;
*/
public Builder setStakedAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.setStakedAccountId(value);
return this;
}
/**
*
**
* ID of the account to which this contract is staking.
*
*
* .proto.AccountID staked_account_id = 17;
*/
public Builder setStakedAccountId(
com.hedera.hashgraph.sdk.proto.AccountID.Builder builderForValue) {
copyOnWrite();
instance.setStakedAccountId(builderForValue.build());
return this;
}
/**
*
**
* ID of the account to which this contract is staking.
*
*
* .proto.AccountID staked_account_id = 17;
*/
public Builder mergeStakedAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.mergeStakedAccountId(value);
return this;
}
/**
*
**
* ID of the account to which this contract is staking.
*
*
* .proto.AccountID staked_account_id = 17;
*/
public Builder clearStakedAccountId() {
copyOnWrite();
instance.clearStakedAccountId();
return this;
}
/**
*
**
* ID of the node this contract is staked to.
*
*
* int64 staked_node_id = 18;
* @return Whether the stakedNodeId field is set.
*/
@java.lang.Override
public boolean hasStakedNodeId() {
return instance.hasStakedNodeId();
}
/**
*
**
* ID of the node this contract is staked to.
*
*
* int64 staked_node_id = 18;
* @return The stakedNodeId.
*/
@java.lang.Override
public long getStakedNodeId() {
return instance.getStakedNodeId();
}
/**
*
**
* ID of the node this contract is staked to.
*
*
* int64 staked_node_id = 18;
* @param value The stakedNodeId to set.
* @return This builder for chaining.
*/
public Builder setStakedNodeId(long value) {
copyOnWrite();
instance.setStakedNodeId(value);
return this;
}
/**
*
**
* ID of the node this contract is staked to.
*
*
* int64 staked_node_id = 18;
* @return This builder for chaining.
*/
public Builder clearStakedNodeId() {
copyOnWrite();
instance.clearStakedNodeId();
return this;
}
/**
*
**
* If true, the contract declines receiving a staking reward. The default value is false.
*
*
* bool decline_reward = 19;
* @return The declineReward.
*/
@java.lang.Override
public boolean getDeclineReward() {
return instance.getDeclineReward();
}
/**
*
**
* If true, the contract declines receiving a staking reward. The default value is false.
*
*
* bool decline_reward = 19;
* @param value The declineReward to set.
* @return This builder for chaining.
*/
public Builder setDeclineReward(boolean value) {
copyOnWrite();
instance.setDeclineReward(value);
return this;
}
/**
*
**
* If true, the contract declines receiving a staking reward. The default value is false.
*
*
* bool decline_reward = 19;
* @return This builder for chaining.
*/
public Builder clearDeclineReward() {
copyOnWrite();
instance.clearDeclineReward();
return this;
}
// @@protoc_insertion_point(builder_scope:proto.ContractCreateTransactionBody)
}
@java.lang.Override
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody();
}
case NEW_BUILDER: {
return new Builder();
}
case BUILD_MESSAGE_INFO: {
java.lang.Object[] objects = new java.lang.Object[] {
"initcodeSource_",
"initcodeSourceCase_",
"stakedId_",
"stakedIdCase_",
com.hedera.hashgraph.sdk.proto.FileID.class,
"adminKey_",
"gas_",
"initialBalance_",
"proxyAccountID_",
"autoRenewPeriod_",
"constructorParameters_",
"shardID_",
"realmID_",
"newRealmAdminKey_",
"memo_",
"maxAutomaticTokenAssociations_",
"autoRenewAccountId_",
com.hedera.hashgraph.sdk.proto.AccountID.class,
"declineReward_",
};
java.lang.String info =
"\u0000\u0011\u0002\u0000\u0001\u0013\u0011\u0000\u0000\u0000\u0001<\u0000\u0003\t" +
"\u0004\u0002\u0005\u0002\u0006\t\b\t\t\n\n\t\u000b\t\f\t\r\u0208\u000e\u0004\u000f" +
"\t\u0010=\u0000\u0011<\u0001\u00125\u0001\u0013\u0007";
return newMessageInfo(DEFAULT_INSTANCE, info, objects);
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
com.google.protobuf.Parser parser = PARSER;
if (parser == null) {
synchronized (com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody.class) {
parser = PARSER;
if (parser == null) {
parser =
new DefaultInstanceBasedParser(
DEFAULT_INSTANCE);
PARSER = parser;
}
}
}
return parser;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:proto.ContractCreateTransactionBody)
private static final com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody DEFAULT_INSTANCE;
static {
ContractCreateTransactionBody defaultInstance = new ContractCreateTransactionBody();
// New instances are implicitly immutable so no need to make
// immutable.
DEFAULT_INSTANCE = defaultInstance;
com.google.protobuf.GeneratedMessageLite.registerDefaultInstance(
ContractCreateTransactionBody.class, defaultInstance);
}
public static com.hedera.hashgraph.sdk.proto.ContractCreateTransactionBody getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy