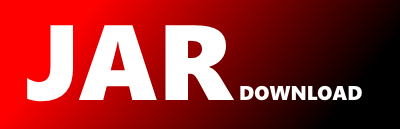
com.hedera.hashgraph.sdk.proto.ContractFunctionResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk-jdk7 Show documentation
Show all versions of sdk-jdk7 Show documentation
Hedera™ Hashgraph SDK for Java
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: contract_call_local.proto
package com.hedera.hashgraph.sdk.proto;
/**
*
**
* The result returned by a call to a smart contract function. This is part of the response to a
* ContractCallLocal query, and is in the record for a ContractCall or ContractCreateInstance
* transaction. The ContractCreateInstance transaction record has the results of the call to the
* constructor.
*
*
* Protobuf type {@code proto.ContractFunctionResult}
*/
public final class ContractFunctionResult extends
com.google.protobuf.GeneratedMessageLite<
ContractFunctionResult, ContractFunctionResult.Builder> implements
// @@protoc_insertion_point(message_implements:proto.ContractFunctionResult)
ContractFunctionResultOrBuilder {
private ContractFunctionResult() {
contractCallResult_ = com.google.protobuf.ByteString.EMPTY;
errorMessage_ = "";
bloom_ = com.google.protobuf.ByteString.EMPTY;
logInfo_ = emptyProtobufList();
createdContractIDs_ = emptyProtobufList();
functionParameters_ = com.google.protobuf.ByteString.EMPTY;
}
public static final int CONTRACTID_FIELD_NUMBER = 1;
private com.hedera.hashgraph.sdk.proto.ContractID contractID_;
/**
*
**
* the smart contract instance whose function was called
*
*
* .proto.ContractID contractID = 1;
*/
@java.lang.Override
public boolean hasContractID() {
return contractID_ != null;
}
/**
*
**
* the smart contract instance whose function was called
*
*
* .proto.ContractID contractID = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ContractID getContractID() {
return contractID_ == null ? com.hedera.hashgraph.sdk.proto.ContractID.getDefaultInstance() : contractID_;
}
/**
*
**
* the smart contract instance whose function was called
*
*
* .proto.ContractID contractID = 1;
*/
private void setContractID(com.hedera.hashgraph.sdk.proto.ContractID value) {
value.getClass();
contractID_ = value;
}
/**
*
**
* the smart contract instance whose function was called
*
*
* .proto.ContractID contractID = 1;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeContractID(com.hedera.hashgraph.sdk.proto.ContractID value) {
value.getClass();
if (contractID_ != null &&
contractID_ != com.hedera.hashgraph.sdk.proto.ContractID.getDefaultInstance()) {
contractID_ =
com.hedera.hashgraph.sdk.proto.ContractID.newBuilder(contractID_).mergeFrom(value).buildPartial();
} else {
contractID_ = value;
}
}
/**
*
**
* the smart contract instance whose function was called
*
*
* .proto.ContractID contractID = 1;
*/
private void clearContractID() { contractID_ = null;
}
public static final int CONTRACTCALLRESULT_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString contractCallResult_;
/**
*
**
* the result returned by the function
*
*
* bytes contractCallResult = 2;
* @return The contractCallResult.
*/
@java.lang.Override
public com.google.protobuf.ByteString getContractCallResult() {
return contractCallResult_;
}
/**
*
**
* the result returned by the function
*
*
* bytes contractCallResult = 2;
* @param value The contractCallResult to set.
*/
private void setContractCallResult(com.google.protobuf.ByteString value) {
java.lang.Class> valueClass = value.getClass();
contractCallResult_ = value;
}
/**
*
**
* the result returned by the function
*
*
* bytes contractCallResult = 2;
*/
private void clearContractCallResult() {
contractCallResult_ = getDefaultInstance().getContractCallResult();
}
public static final int ERRORMESSAGE_FIELD_NUMBER = 3;
private java.lang.String errorMessage_;
/**
*
**
* message In case there was an error during smart contract execution
*
*
* string errorMessage = 3;
* @return The errorMessage.
*/
@java.lang.Override
public java.lang.String getErrorMessage() {
return errorMessage_;
}
/**
*
**
* message In case there was an error during smart contract execution
*
*
* string errorMessage = 3;
* @return The bytes for errorMessage.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getErrorMessageBytes() {
return com.google.protobuf.ByteString.copyFromUtf8(errorMessage_);
}
/**
*
**
* message In case there was an error during smart contract execution
*
*
* string errorMessage = 3;
* @param value The errorMessage to set.
*/
private void setErrorMessage(
java.lang.String value) {
java.lang.Class> valueClass = value.getClass();
errorMessage_ = value;
}
/**
*
**
* message In case there was an error during smart contract execution
*
*
* string errorMessage = 3;
*/
private void clearErrorMessage() {
errorMessage_ = getDefaultInstance().getErrorMessage();
}
/**
*
**
* message In case there was an error during smart contract execution
*
*
* string errorMessage = 3;
* @param value The bytes for errorMessage to set.
*/
private void setErrorMessageBytes(
com.google.protobuf.ByteString value) {
checkByteStringIsUtf8(value);
errorMessage_ = value.toStringUtf8();
}
public static final int BLOOM_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString bloom_;
/**
*
**
* bloom filter for record
*
*
* bytes bloom = 4;
* @return The bloom.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBloom() {
return bloom_;
}
/**
*
**
* bloom filter for record
*
*
* bytes bloom = 4;
* @param value The bloom to set.
*/
private void setBloom(com.google.protobuf.ByteString value) {
java.lang.Class> valueClass = value.getClass();
bloom_ = value;
}
/**
*
**
* bloom filter for record
*
*
* bytes bloom = 4;
*/
private void clearBloom() {
bloom_ = getDefaultInstance().getBloom();
}
public static final int GASUSED_FIELD_NUMBER = 5;
private long gasUsed_;
/**
*
**
* units of gas used to execute contract
*
*
* uint64 gasUsed = 5;
* @return The gasUsed.
*/
@java.lang.Override
public long getGasUsed() {
return gasUsed_;
}
/**
*
**
* units of gas used to execute contract
*
*
* uint64 gasUsed = 5;
* @param value The gasUsed to set.
*/
private void setGasUsed(long value) {
gasUsed_ = value;
}
/**
*
**
* units of gas used to execute contract
*
*
* uint64 gasUsed = 5;
*/
private void clearGasUsed() {
gasUsed_ = 0L;
}
public static final int LOGINFO_FIELD_NUMBER = 6;
private com.google.protobuf.Internal.ProtobufList logInfo_;
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
@java.lang.Override
public java.util.List getLogInfoList() {
return logInfo_;
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
public java.util.List extends com.hedera.hashgraph.sdk.proto.ContractLoginfoOrBuilder>
getLogInfoOrBuilderList() {
return logInfo_;
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
@java.lang.Override
public int getLogInfoCount() {
return logInfo_.size();
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ContractLoginfo getLogInfo(int index) {
return logInfo_.get(index);
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
public com.hedera.hashgraph.sdk.proto.ContractLoginfoOrBuilder getLogInfoOrBuilder(
int index) {
return logInfo_.get(index);
}
private void ensureLogInfoIsMutable() {
com.google.protobuf.Internal.ProtobufList tmp = logInfo_;
if (!tmp.isModifiable()) {
logInfo_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(tmp);
}
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
private void setLogInfo(
int index, com.hedera.hashgraph.sdk.proto.ContractLoginfo value) {
value.getClass();
ensureLogInfoIsMutable();
logInfo_.set(index, value);
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
private void addLogInfo(com.hedera.hashgraph.sdk.proto.ContractLoginfo value) {
value.getClass();
ensureLogInfoIsMutable();
logInfo_.add(value);
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
private void addLogInfo(
int index, com.hedera.hashgraph.sdk.proto.ContractLoginfo value) {
value.getClass();
ensureLogInfoIsMutable();
logInfo_.add(index, value);
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
private void addAllLogInfo(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.ContractLoginfo> values) {
ensureLogInfoIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, logInfo_);
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
private void clearLogInfo() {
logInfo_ = emptyProtobufList();
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
private void removeLogInfo(int index) {
ensureLogInfoIsMutable();
logInfo_.remove(index);
}
public static final int CREATEDCONTRACTIDS_FIELD_NUMBER = 7;
private com.google.protobuf.Internal.ProtobufList createdContractIDs_;
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public java.util.List getCreatedContractIDsList() {
return createdContractIDs_;
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Deprecated public java.util.List extends com.hedera.hashgraph.sdk.proto.ContractIDOrBuilder>
getCreatedContractIDsOrBuilderList() {
return createdContractIDs_;
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public int getCreatedContractIDsCount() {
return createdContractIDs_.size();
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.ContractID getCreatedContractIDs(int index) {
return createdContractIDs_.get(index);
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.ContractIDOrBuilder getCreatedContractIDsOrBuilder(
int index) {
return createdContractIDs_.get(index);
}
private void ensureCreatedContractIDsIsMutable() {
com.google.protobuf.Internal.ProtobufList tmp = createdContractIDs_;
if (!tmp.isModifiable()) {
createdContractIDs_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(tmp);
}
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
private void setCreatedContractIDs(
int index, com.hedera.hashgraph.sdk.proto.ContractID value) {
value.getClass();
ensureCreatedContractIDsIsMutable();
createdContractIDs_.set(index, value);
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
private void addCreatedContractIDs(com.hedera.hashgraph.sdk.proto.ContractID value) {
value.getClass();
ensureCreatedContractIDsIsMutable();
createdContractIDs_.add(value);
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
private void addCreatedContractIDs(
int index, com.hedera.hashgraph.sdk.proto.ContractID value) {
value.getClass();
ensureCreatedContractIDsIsMutable();
createdContractIDs_.add(index, value);
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
private void addAllCreatedContractIDs(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.ContractID> values) {
ensureCreatedContractIDsIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, createdContractIDs_);
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
private void clearCreatedContractIDs() {
createdContractIDs_ = emptyProtobufList();
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
private void removeCreatedContractIDs(int index) {
ensureCreatedContractIDsIsMutable();
createdContractIDs_.remove(index);
}
public static final int EVM_ADDRESS_FIELD_NUMBER = 9;
private com.google.protobuf.BytesValue evmAddress_;
/**
*
**
* The new contract's 20-byte EVM address. Only populated after release 0.23,
* where each created contract will have its own record. (This is an important
* point--the field is not <tt>repeated</tt> because there will be a separate
* child record for each created contract.)
*
* Every contract has an EVM address determined by its <tt>shard.realm.num</tt> id.
* This address is as follows:
* <ol>
* <li>The first 4 bytes are the big-endian representation of the shard.</li>
* <li>The next 8 bytes are the big-endian representation of the realm.</li>
* <li>The final 8 bytes are the big-endian representation of the number.</li>
* </ol>
*
* Contracts created via CREATE2 have an <b>additional, primary address</b> that is
* derived from the <a href="https://eips.ethereum.org/EIPS/eip-1014">EIP-1014</a>
* specification, and does not have a simple relation to a <tt>shard.realm.num</tt> id.
*
* (Please do note that CREATE2 contracts can also be referenced by the three-part
* EVM address described above.)
*
*
* .google.protobuf.BytesValue evm_address = 9;
*/
@java.lang.Override
public boolean hasEvmAddress() {
return evmAddress_ != null;
}
/**
*
**
* The new contract's 20-byte EVM address. Only populated after release 0.23,
* where each created contract will have its own record. (This is an important
* point--the field is not <tt>repeated</tt> because there will be a separate
* child record for each created contract.)
*
* Every contract has an EVM address determined by its <tt>shard.realm.num</tt> id.
* This address is as follows:
* <ol>
* <li>The first 4 bytes are the big-endian representation of the shard.</li>
* <li>The next 8 bytes are the big-endian representation of the realm.</li>
* <li>The final 8 bytes are the big-endian representation of the number.</li>
* </ol>
*
* Contracts created via CREATE2 have an <b>additional, primary address</b> that is
* derived from the <a href="https://eips.ethereum.org/EIPS/eip-1014">EIP-1014</a>
* specification, and does not have a simple relation to a <tt>shard.realm.num</tt> id.
*
* (Please do note that CREATE2 contracts can also be referenced by the three-part
* EVM address described above.)
*
*
* .google.protobuf.BytesValue evm_address = 9;
*/
@java.lang.Override
public com.google.protobuf.BytesValue getEvmAddress() {
return evmAddress_ == null ? com.google.protobuf.BytesValue.getDefaultInstance() : evmAddress_;
}
/**
*
**
* The new contract's 20-byte EVM address. Only populated after release 0.23,
* where each created contract will have its own record. (This is an important
* point--the field is not <tt>repeated</tt> because there will be a separate
* child record for each created contract.)
*
* Every contract has an EVM address determined by its <tt>shard.realm.num</tt> id.
* This address is as follows:
* <ol>
* <li>The first 4 bytes are the big-endian representation of the shard.</li>
* <li>The next 8 bytes are the big-endian representation of the realm.</li>
* <li>The final 8 bytes are the big-endian representation of the number.</li>
* </ol>
*
* Contracts created via CREATE2 have an <b>additional, primary address</b> that is
* derived from the <a href="https://eips.ethereum.org/EIPS/eip-1014">EIP-1014</a>
* specification, and does not have a simple relation to a <tt>shard.realm.num</tt> id.
*
* (Please do note that CREATE2 contracts can also be referenced by the three-part
* EVM address described above.)
*
*
* .google.protobuf.BytesValue evm_address = 9;
*/
private void setEvmAddress(com.google.protobuf.BytesValue value) {
value.getClass();
evmAddress_ = value;
}
/**
*
**
* The new contract's 20-byte EVM address. Only populated after release 0.23,
* where each created contract will have its own record. (This is an important
* point--the field is not <tt>repeated</tt> because there will be a separate
* child record for each created contract.)
*
* Every contract has an EVM address determined by its <tt>shard.realm.num</tt> id.
* This address is as follows:
* <ol>
* <li>The first 4 bytes are the big-endian representation of the shard.</li>
* <li>The next 8 bytes are the big-endian representation of the realm.</li>
* <li>The final 8 bytes are the big-endian representation of the number.</li>
* </ol>
*
* Contracts created via CREATE2 have an <b>additional, primary address</b> that is
* derived from the <a href="https://eips.ethereum.org/EIPS/eip-1014">EIP-1014</a>
* specification, and does not have a simple relation to a <tt>shard.realm.num</tt> id.
*
* (Please do note that CREATE2 contracts can also be referenced by the three-part
* EVM address described above.)
*
*
* .google.protobuf.BytesValue evm_address = 9;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeEvmAddress(com.google.protobuf.BytesValue value) {
value.getClass();
if (evmAddress_ != null &&
evmAddress_ != com.google.protobuf.BytesValue.getDefaultInstance()) {
evmAddress_ =
com.google.protobuf.BytesValue.newBuilder(evmAddress_).mergeFrom(value).buildPartial();
} else {
evmAddress_ = value;
}
}
/**
*
**
* The new contract's 20-byte EVM address. Only populated after release 0.23,
* where each created contract will have its own record. (This is an important
* point--the field is not <tt>repeated</tt> because there will be a separate
* child record for each created contract.)
*
* Every contract has an EVM address determined by its <tt>shard.realm.num</tt> id.
* This address is as follows:
* <ol>
* <li>The first 4 bytes are the big-endian representation of the shard.</li>
* <li>The next 8 bytes are the big-endian representation of the realm.</li>
* <li>The final 8 bytes are the big-endian representation of the number.</li>
* </ol>
*
* Contracts created via CREATE2 have an <b>additional, primary address</b> that is
* derived from the <a href="https://eips.ethereum.org/EIPS/eip-1014">EIP-1014</a>
* specification, and does not have a simple relation to a <tt>shard.realm.num</tt> id.
*
* (Please do note that CREATE2 contracts can also be referenced by the three-part
* EVM address described above.)
*
*
* .google.protobuf.BytesValue evm_address = 9;
*/
private void clearEvmAddress() { evmAddress_ = null;
}
public static final int GAS_FIELD_NUMBER = 10;
private long gas_;
/**
*
**
* The amount of gas available for the call, aka the gasLimit.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* int64 gas = 10;
* @return The gas.
*/
@java.lang.Override
public long getGas() {
return gas_;
}
/**
*
**
* The amount of gas available for the call, aka the gasLimit.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* int64 gas = 10;
* @param value The gas to set.
*/
private void setGas(long value) {
gas_ = value;
}
/**
*
**
* The amount of gas available for the call, aka the gasLimit.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* int64 gas = 10;
*/
private void clearGas() {
gas_ = 0L;
}
public static final int AMOUNT_FIELD_NUMBER = 11;
private long amount_;
/**
*
**
* Number of tinybars sent (the function must be payable if this is nonzero).
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* int64 amount = 11;
* @return The amount.
*/
@java.lang.Override
public long getAmount() {
return amount_;
}
/**
*
**
* Number of tinybars sent (the function must be payable if this is nonzero).
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* int64 amount = 11;
* @param value The amount to set.
*/
private void setAmount(long value) {
amount_ = value;
}
/**
*
**
* Number of tinybars sent (the function must be payable if this is nonzero).
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* int64 amount = 11;
*/
private void clearAmount() {
amount_ = 0L;
}
public static final int FUNCTIONPARAMETERS_FIELD_NUMBER = 12;
private com.google.protobuf.ByteString functionParameters_;
/**
*
**
* The parameters passed into the contract call.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* bytes functionParameters = 12;
* @return The functionParameters.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFunctionParameters() {
return functionParameters_;
}
/**
*
**
* The parameters passed into the contract call.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* bytes functionParameters = 12;
* @param value The functionParameters to set.
*/
private void setFunctionParameters(com.google.protobuf.ByteString value) {
java.lang.Class> valueClass = value.getClass();
functionParameters_ = value;
}
/**
*
**
* The parameters passed into the contract call.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* bytes functionParameters = 12;
*/
private void clearFunctionParameters() {
functionParameters_ = getDefaultInstance().getFunctionParameters();
}
public static final int SENDER_ID_FIELD_NUMBER = 13;
private com.hedera.hashgraph.sdk.proto.AccountID senderId_;
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* .proto.AccountID sender_id = 13;
*/
@java.lang.Override
public boolean hasSenderId() {
return senderId_ != null;
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* .proto.AccountID sender_id = 13;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getSenderId() {
return senderId_ == null ? com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance() : senderId_;
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* .proto.AccountID sender_id = 13;
*/
private void setSenderId(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
senderId_ = value;
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* .proto.AccountID sender_id = 13;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeSenderId(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
if (senderId_ != null &&
senderId_ != com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance()) {
senderId_ =
com.hedera.hashgraph.sdk.proto.AccountID.newBuilder(senderId_).mergeFrom(value).buildPartial();
} else {
senderId_ = value;
}
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* .proto.AccountID sender_id = 13;
*/
private void clearSenderId() { senderId_ = null;
}
public static com.hedera.hashgraph.sdk.proto.ContractFunctionResult parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ContractFunctionResult parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractFunctionResult parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ContractFunctionResult parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractFunctionResult parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ContractFunctionResult parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractFunctionResult parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ContractFunctionResult parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractFunctionResult parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ContractFunctionResult parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractFunctionResult parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ContractFunctionResult parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(com.hedera.hashgraph.sdk.proto.ContractFunctionResult prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
*
**
* The result returned by a call to a smart contract function. This is part of the response to a
* ContractCallLocal query, and is in the record for a ContractCall or ContractCreateInstance
* transaction. The ContractCreateInstance transaction record has the results of the call to the
* constructor.
*
*
* Protobuf type {@code proto.ContractFunctionResult}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
com.hedera.hashgraph.sdk.proto.ContractFunctionResult, Builder> implements
// @@protoc_insertion_point(builder_implements:proto.ContractFunctionResult)
com.hedera.hashgraph.sdk.proto.ContractFunctionResultOrBuilder {
// Construct using com.hedera.hashgraph.sdk.proto.ContractFunctionResult.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
*
**
* the smart contract instance whose function was called
*
*
* .proto.ContractID contractID = 1;
*/
@java.lang.Override
public boolean hasContractID() {
return instance.hasContractID();
}
/**
*
**
* the smart contract instance whose function was called
*
*
* .proto.ContractID contractID = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ContractID getContractID() {
return instance.getContractID();
}
/**
*
**
* the smart contract instance whose function was called
*
*
* .proto.ContractID contractID = 1;
*/
public Builder setContractID(com.hedera.hashgraph.sdk.proto.ContractID value) {
copyOnWrite();
instance.setContractID(value);
return this;
}
/**
*
**
* the smart contract instance whose function was called
*
*
* .proto.ContractID contractID = 1;
*/
public Builder setContractID(
com.hedera.hashgraph.sdk.proto.ContractID.Builder builderForValue) {
copyOnWrite();
instance.setContractID(builderForValue.build());
return this;
}
/**
*
**
* the smart contract instance whose function was called
*
*
* .proto.ContractID contractID = 1;
*/
public Builder mergeContractID(com.hedera.hashgraph.sdk.proto.ContractID value) {
copyOnWrite();
instance.mergeContractID(value);
return this;
}
/**
*
**
* the smart contract instance whose function was called
*
*
* .proto.ContractID contractID = 1;
*/
public Builder clearContractID() { copyOnWrite();
instance.clearContractID();
return this;
}
/**
*
**
* the result returned by the function
*
*
* bytes contractCallResult = 2;
* @return The contractCallResult.
*/
@java.lang.Override
public com.google.protobuf.ByteString getContractCallResult() {
return instance.getContractCallResult();
}
/**
*
**
* the result returned by the function
*
*
* bytes contractCallResult = 2;
* @param value The contractCallResult to set.
* @return This builder for chaining.
*/
public Builder setContractCallResult(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setContractCallResult(value);
return this;
}
/**
*
**
* the result returned by the function
*
*
* bytes contractCallResult = 2;
* @return This builder for chaining.
*/
public Builder clearContractCallResult() {
copyOnWrite();
instance.clearContractCallResult();
return this;
}
/**
*
**
* message In case there was an error during smart contract execution
*
*
* string errorMessage = 3;
* @return The errorMessage.
*/
@java.lang.Override
public java.lang.String getErrorMessage() {
return instance.getErrorMessage();
}
/**
*
**
* message In case there was an error during smart contract execution
*
*
* string errorMessage = 3;
* @return The bytes for errorMessage.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getErrorMessageBytes() {
return instance.getErrorMessageBytes();
}
/**
*
**
* message In case there was an error during smart contract execution
*
*
* string errorMessage = 3;
* @param value The errorMessage to set.
* @return This builder for chaining.
*/
public Builder setErrorMessage(
java.lang.String value) {
copyOnWrite();
instance.setErrorMessage(value);
return this;
}
/**
*
**
* message In case there was an error during smart contract execution
*
*
* string errorMessage = 3;
* @return This builder for chaining.
*/
public Builder clearErrorMessage() {
copyOnWrite();
instance.clearErrorMessage();
return this;
}
/**
*
**
* message In case there was an error during smart contract execution
*
*
* string errorMessage = 3;
* @param value The bytes for errorMessage to set.
* @return This builder for chaining.
*/
public Builder setErrorMessageBytes(
com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setErrorMessageBytes(value);
return this;
}
/**
*
**
* bloom filter for record
*
*
* bytes bloom = 4;
* @return The bloom.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBloom() {
return instance.getBloom();
}
/**
*
**
* bloom filter for record
*
*
* bytes bloom = 4;
* @param value The bloom to set.
* @return This builder for chaining.
*/
public Builder setBloom(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setBloom(value);
return this;
}
/**
*
**
* bloom filter for record
*
*
* bytes bloom = 4;
* @return This builder for chaining.
*/
public Builder clearBloom() {
copyOnWrite();
instance.clearBloom();
return this;
}
/**
*
**
* units of gas used to execute contract
*
*
* uint64 gasUsed = 5;
* @return The gasUsed.
*/
@java.lang.Override
public long getGasUsed() {
return instance.getGasUsed();
}
/**
*
**
* units of gas used to execute contract
*
*
* uint64 gasUsed = 5;
* @param value The gasUsed to set.
* @return This builder for chaining.
*/
public Builder setGasUsed(long value) {
copyOnWrite();
instance.setGasUsed(value);
return this;
}
/**
*
**
* units of gas used to execute contract
*
*
* uint64 gasUsed = 5;
* @return This builder for chaining.
*/
public Builder clearGasUsed() {
copyOnWrite();
instance.clearGasUsed();
return this;
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
@java.lang.Override
public java.util.List getLogInfoList() {
return java.util.Collections.unmodifiableList(
instance.getLogInfoList());
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
@java.lang.Override
public int getLogInfoCount() {
return instance.getLogInfoCount();
}/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ContractLoginfo getLogInfo(int index) {
return instance.getLogInfo(index);
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
public Builder setLogInfo(
int index, com.hedera.hashgraph.sdk.proto.ContractLoginfo value) {
copyOnWrite();
instance.setLogInfo(index, value);
return this;
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
public Builder setLogInfo(
int index, com.hedera.hashgraph.sdk.proto.ContractLoginfo.Builder builderForValue) {
copyOnWrite();
instance.setLogInfo(index,
builderForValue.build());
return this;
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
public Builder addLogInfo(com.hedera.hashgraph.sdk.proto.ContractLoginfo value) {
copyOnWrite();
instance.addLogInfo(value);
return this;
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
public Builder addLogInfo(
int index, com.hedera.hashgraph.sdk.proto.ContractLoginfo value) {
copyOnWrite();
instance.addLogInfo(index, value);
return this;
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
public Builder addLogInfo(
com.hedera.hashgraph.sdk.proto.ContractLoginfo.Builder builderForValue) {
copyOnWrite();
instance.addLogInfo(builderForValue.build());
return this;
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
public Builder addLogInfo(
int index, com.hedera.hashgraph.sdk.proto.ContractLoginfo.Builder builderForValue) {
copyOnWrite();
instance.addLogInfo(index,
builderForValue.build());
return this;
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
public Builder addAllLogInfo(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.ContractLoginfo> values) {
copyOnWrite();
instance.addAllLogInfo(values);
return this;
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
public Builder clearLogInfo() {
copyOnWrite();
instance.clearLogInfo();
return this;
}
/**
*
**
* the log info for events returned by the function
*
*
* repeated .proto.ContractLoginfo logInfo = 6;
*/
public Builder removeLogInfo(int index) {
copyOnWrite();
instance.removeLogInfo(index);
return this;
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public java.util.List getCreatedContractIDsList() {
return java.util.Collections.unmodifiableList(
instance.getCreatedContractIDsList());
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public int getCreatedContractIDsCount() {
return instance.getCreatedContractIDsCount();
}/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.ContractID getCreatedContractIDs(int index) {
return instance.getCreatedContractIDs(index);
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Deprecated public Builder setCreatedContractIDs(
int index, com.hedera.hashgraph.sdk.proto.ContractID value) {
copyOnWrite();
instance.setCreatedContractIDs(index, value);
return this;
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Deprecated public Builder setCreatedContractIDs(
int index, com.hedera.hashgraph.sdk.proto.ContractID.Builder builderForValue) {
copyOnWrite();
instance.setCreatedContractIDs(index,
builderForValue.build());
return this;
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Deprecated public Builder addCreatedContractIDs(com.hedera.hashgraph.sdk.proto.ContractID value) {
copyOnWrite();
instance.addCreatedContractIDs(value);
return this;
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Deprecated public Builder addCreatedContractIDs(
int index, com.hedera.hashgraph.sdk.proto.ContractID value) {
copyOnWrite();
instance.addCreatedContractIDs(index, value);
return this;
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Deprecated public Builder addCreatedContractIDs(
com.hedera.hashgraph.sdk.proto.ContractID.Builder builderForValue) {
copyOnWrite();
instance.addCreatedContractIDs(builderForValue.build());
return this;
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Deprecated public Builder addCreatedContractIDs(
int index, com.hedera.hashgraph.sdk.proto.ContractID.Builder builderForValue) {
copyOnWrite();
instance.addCreatedContractIDs(index,
builderForValue.build());
return this;
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Deprecated public Builder addAllCreatedContractIDs(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.ContractID> values) {
copyOnWrite();
instance.addAllCreatedContractIDs(values);
return this;
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearCreatedContractIDs() {
copyOnWrite();
instance.clearCreatedContractIDs();
return this;
}
/**
*
**
* [DEPRECATED] the list of smart contracts that were created by the function call.
*
* The created ids will now _also_ be externalized through internal transaction
* records, where each record has its alias field populated with the new contract's
* EVM address. (This is needed for contracts created with CREATE2, since
* there is no longer a simple relationship between the new contract's 0.0.X id
* and its Solidity address.)
*
*
* repeated .proto.ContractID createdContractIDs = 7 [deprecated = true];
*/
@java.lang.Deprecated public Builder removeCreatedContractIDs(int index) {
copyOnWrite();
instance.removeCreatedContractIDs(index);
return this;
}
/**
*
**
* The new contract's 20-byte EVM address. Only populated after release 0.23,
* where each created contract will have its own record. (This is an important
* point--the field is not <tt>repeated</tt> because there will be a separate
* child record for each created contract.)
*
* Every contract has an EVM address determined by its <tt>shard.realm.num</tt> id.
* This address is as follows:
* <ol>
* <li>The first 4 bytes are the big-endian representation of the shard.</li>
* <li>The next 8 bytes are the big-endian representation of the realm.</li>
* <li>The final 8 bytes are the big-endian representation of the number.</li>
* </ol>
*
* Contracts created via CREATE2 have an <b>additional, primary address</b> that is
* derived from the <a href="https://eips.ethereum.org/EIPS/eip-1014">EIP-1014</a>
* specification, and does not have a simple relation to a <tt>shard.realm.num</tt> id.
*
* (Please do note that CREATE2 contracts can also be referenced by the three-part
* EVM address described above.)
*
*
* .google.protobuf.BytesValue evm_address = 9;
*/
@java.lang.Override
public boolean hasEvmAddress() {
return instance.hasEvmAddress();
}
/**
*
**
* The new contract's 20-byte EVM address. Only populated after release 0.23,
* where each created contract will have its own record. (This is an important
* point--the field is not <tt>repeated</tt> because there will be a separate
* child record for each created contract.)
*
* Every contract has an EVM address determined by its <tt>shard.realm.num</tt> id.
* This address is as follows:
* <ol>
* <li>The first 4 bytes are the big-endian representation of the shard.</li>
* <li>The next 8 bytes are the big-endian representation of the realm.</li>
* <li>The final 8 bytes are the big-endian representation of the number.</li>
* </ol>
*
* Contracts created via CREATE2 have an <b>additional, primary address</b> that is
* derived from the <a href="https://eips.ethereum.org/EIPS/eip-1014">EIP-1014</a>
* specification, and does not have a simple relation to a <tt>shard.realm.num</tt> id.
*
* (Please do note that CREATE2 contracts can also be referenced by the three-part
* EVM address described above.)
*
*
* .google.protobuf.BytesValue evm_address = 9;
*/
@java.lang.Override
public com.google.protobuf.BytesValue getEvmAddress() {
return instance.getEvmAddress();
}
/**
*
**
* The new contract's 20-byte EVM address. Only populated after release 0.23,
* where each created contract will have its own record. (This is an important
* point--the field is not <tt>repeated</tt> because there will be a separate
* child record for each created contract.)
*
* Every contract has an EVM address determined by its <tt>shard.realm.num</tt> id.
* This address is as follows:
* <ol>
* <li>The first 4 bytes are the big-endian representation of the shard.</li>
* <li>The next 8 bytes are the big-endian representation of the realm.</li>
* <li>The final 8 bytes are the big-endian representation of the number.</li>
* </ol>
*
* Contracts created via CREATE2 have an <b>additional, primary address</b> that is
* derived from the <a href="https://eips.ethereum.org/EIPS/eip-1014">EIP-1014</a>
* specification, and does not have a simple relation to a <tt>shard.realm.num</tt> id.
*
* (Please do note that CREATE2 contracts can also be referenced by the three-part
* EVM address described above.)
*
*
* .google.protobuf.BytesValue evm_address = 9;
*/
public Builder setEvmAddress(com.google.protobuf.BytesValue value) {
copyOnWrite();
instance.setEvmAddress(value);
return this;
}
/**
*
**
* The new contract's 20-byte EVM address. Only populated after release 0.23,
* where each created contract will have its own record. (This is an important
* point--the field is not <tt>repeated</tt> because there will be a separate
* child record for each created contract.)
*
* Every contract has an EVM address determined by its <tt>shard.realm.num</tt> id.
* This address is as follows:
* <ol>
* <li>The first 4 bytes are the big-endian representation of the shard.</li>
* <li>The next 8 bytes are the big-endian representation of the realm.</li>
* <li>The final 8 bytes are the big-endian representation of the number.</li>
* </ol>
*
* Contracts created via CREATE2 have an <b>additional, primary address</b> that is
* derived from the <a href="https://eips.ethereum.org/EIPS/eip-1014">EIP-1014</a>
* specification, and does not have a simple relation to a <tt>shard.realm.num</tt> id.
*
* (Please do note that CREATE2 contracts can also be referenced by the three-part
* EVM address described above.)
*
*
* .google.protobuf.BytesValue evm_address = 9;
*/
public Builder setEvmAddress(
com.google.protobuf.BytesValue.Builder builderForValue) {
copyOnWrite();
instance.setEvmAddress(builderForValue.build());
return this;
}
/**
*
**
* The new contract's 20-byte EVM address. Only populated after release 0.23,
* where each created contract will have its own record. (This is an important
* point--the field is not <tt>repeated</tt> because there will be a separate
* child record for each created contract.)
*
* Every contract has an EVM address determined by its <tt>shard.realm.num</tt> id.
* This address is as follows:
* <ol>
* <li>The first 4 bytes are the big-endian representation of the shard.</li>
* <li>The next 8 bytes are the big-endian representation of the realm.</li>
* <li>The final 8 bytes are the big-endian representation of the number.</li>
* </ol>
*
* Contracts created via CREATE2 have an <b>additional, primary address</b> that is
* derived from the <a href="https://eips.ethereum.org/EIPS/eip-1014">EIP-1014</a>
* specification, and does not have a simple relation to a <tt>shard.realm.num</tt> id.
*
* (Please do note that CREATE2 contracts can also be referenced by the three-part
* EVM address described above.)
*
*
* .google.protobuf.BytesValue evm_address = 9;
*/
public Builder mergeEvmAddress(com.google.protobuf.BytesValue value) {
copyOnWrite();
instance.mergeEvmAddress(value);
return this;
}
/**
*
**
* The new contract's 20-byte EVM address. Only populated after release 0.23,
* where each created contract will have its own record. (This is an important
* point--the field is not <tt>repeated</tt> because there will be a separate
* child record for each created contract.)
*
* Every contract has an EVM address determined by its <tt>shard.realm.num</tt> id.
* This address is as follows:
* <ol>
* <li>The first 4 bytes are the big-endian representation of the shard.</li>
* <li>The next 8 bytes are the big-endian representation of the realm.</li>
* <li>The final 8 bytes are the big-endian representation of the number.</li>
* </ol>
*
* Contracts created via CREATE2 have an <b>additional, primary address</b> that is
* derived from the <a href="https://eips.ethereum.org/EIPS/eip-1014">EIP-1014</a>
* specification, and does not have a simple relation to a <tt>shard.realm.num</tt> id.
*
* (Please do note that CREATE2 contracts can also be referenced by the three-part
* EVM address described above.)
*
*
* .google.protobuf.BytesValue evm_address = 9;
*/
public Builder clearEvmAddress() { copyOnWrite();
instance.clearEvmAddress();
return this;
}
/**
*
**
* The amount of gas available for the call, aka the gasLimit.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* int64 gas = 10;
* @return The gas.
*/
@java.lang.Override
public long getGas() {
return instance.getGas();
}
/**
*
**
* The amount of gas available for the call, aka the gasLimit.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* int64 gas = 10;
* @param value The gas to set.
* @return This builder for chaining.
*/
public Builder setGas(long value) {
copyOnWrite();
instance.setGas(value);
return this;
}
/**
*
**
* The amount of gas available for the call, aka the gasLimit.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* int64 gas = 10;
* @return This builder for chaining.
*/
public Builder clearGas() {
copyOnWrite();
instance.clearGas();
return this;
}
/**
*
**
* Number of tinybars sent (the function must be payable if this is nonzero).
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* int64 amount = 11;
* @return The amount.
*/
@java.lang.Override
public long getAmount() {
return instance.getAmount();
}
/**
*
**
* Number of tinybars sent (the function must be payable if this is nonzero).
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* int64 amount = 11;
* @param value The amount to set.
* @return This builder for chaining.
*/
public Builder setAmount(long value) {
copyOnWrite();
instance.setAmount(value);
return this;
}
/**
*
**
* Number of tinybars sent (the function must be payable if this is nonzero).
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* int64 amount = 11;
* @return This builder for chaining.
*/
public Builder clearAmount() {
copyOnWrite();
instance.clearAmount();
return this;
}
/**
*
**
* The parameters passed into the contract call.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* bytes functionParameters = 12;
* @return The functionParameters.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFunctionParameters() {
return instance.getFunctionParameters();
}
/**
*
**
* The parameters passed into the contract call.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* bytes functionParameters = 12;
* @param value The functionParameters to set.
* @return This builder for chaining.
*/
public Builder setFunctionParameters(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setFunctionParameters(value);
return this;
}
/**
*
**
* The parameters passed into the contract call.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* bytes functionParameters = 12;
* @return This builder for chaining.
*/
public Builder clearFunctionParameters() {
copyOnWrite();
instance.clearFunctionParameters();
return this;
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* .proto.AccountID sender_id = 13;
*/
@java.lang.Override
public boolean hasSenderId() {
return instance.hasSenderId();
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* .proto.AccountID sender_id = 13;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getSenderId() {
return instance.getSenderId();
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* .proto.AccountID sender_id = 13;
*/
public Builder setSenderId(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.setSenderId(value);
return this;
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* .proto.AccountID sender_id = 13;
*/
public Builder setSenderId(
com.hedera.hashgraph.sdk.proto.AccountID.Builder builderForValue) {
copyOnWrite();
instance.setSenderId(builderForValue.build());
return this;
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* .proto.AccountID sender_id = 13;
*/
public Builder mergeSenderId(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.mergeSenderId(value);
return this;
}
/**
*
**
* The account that is the "sender." If not present it is the accountId from the transactionId.
*
* This field should only be populated when the paired TransactionBody in the record stream is not a
* ContractCreateTransactionBody or a ContractCallTransactionBody.
*
*
* .proto.AccountID sender_id = 13;
*/
public Builder clearSenderId() { copyOnWrite();
instance.clearSenderId();
return this;
}
// @@protoc_insertion_point(builder_scope:proto.ContractFunctionResult)
}
@java.lang.Override
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new com.hedera.hashgraph.sdk.proto.ContractFunctionResult();
}
case NEW_BUILDER: {
return new Builder();
}
case BUILD_MESSAGE_INFO: {
java.lang.Object[] objects = new java.lang.Object[] {
"contractID_",
"contractCallResult_",
"errorMessage_",
"bloom_",
"gasUsed_",
"logInfo_",
com.hedera.hashgraph.sdk.proto.ContractLoginfo.class,
"createdContractIDs_",
com.hedera.hashgraph.sdk.proto.ContractID.class,
"evmAddress_",
"gas_",
"amount_",
"functionParameters_",
"senderId_",
};
java.lang.String info =
"\u0000\f\u0000\u0000\u0001\r\f\u0000\u0002\u0000\u0001\t\u0002\n\u0003\u0208\u0004" +
"\n\u0005\u0003\u0006\u001b\u0007\u001b\t\t\n\u0002\u000b\u0002\f\n\r\t";
return newMessageInfo(DEFAULT_INSTANCE, info, objects);
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
com.google.protobuf.Parser parser = PARSER;
if (parser == null) {
synchronized (com.hedera.hashgraph.sdk.proto.ContractFunctionResult.class) {
parser = PARSER;
if (parser == null) {
parser =
new DefaultInstanceBasedParser(
DEFAULT_INSTANCE);
PARSER = parser;
}
}
}
return parser;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:proto.ContractFunctionResult)
private static final com.hedera.hashgraph.sdk.proto.ContractFunctionResult DEFAULT_INSTANCE;
static {
ContractFunctionResult defaultInstance = new ContractFunctionResult();
// New instances are implicitly immutable so no need to make
// immutable.
DEFAULT_INSTANCE = defaultInstance;
com.google.protobuf.GeneratedMessageLite.registerDefaultInstance(
ContractFunctionResult.class, defaultInstance);
}
public static com.hedera.hashgraph.sdk.proto.ContractFunctionResult getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy