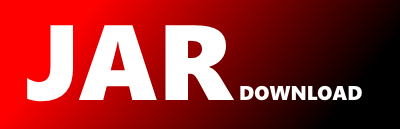
com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk-jdk7 Show documentation
Show all versions of sdk-jdk7 Show documentation
Hedera™ Hashgraph SDK for Java
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: contract_get_info.proto
package com.hedera.hashgraph.sdk.proto;
/**
*
**
* Response when the client sends the node ContractGetInfoQuery
*
*
* Protobuf type {@code proto.ContractGetInfoResponse}
*/
public final class ContractGetInfoResponse extends
com.google.protobuf.GeneratedMessageLite<
ContractGetInfoResponse, ContractGetInfoResponse.Builder> implements
// @@protoc_insertion_point(message_implements:proto.ContractGetInfoResponse)
ContractGetInfoResponseOrBuilder {
private ContractGetInfoResponse() {
}
public interface ContractInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:proto.ContractGetInfoResponse.ContractInfo)
com.google.protobuf.MessageLiteOrBuilder {
/**
*
**
* ID of the contract instance, in the format used in transactions
*
*
* .proto.ContractID contractID = 1;
* @return Whether the contractID field is set.
*/
boolean hasContractID();
/**
*
**
* ID of the contract instance, in the format used in transactions
*
*
* .proto.ContractID contractID = 1;
* @return The contractID.
*/
com.hedera.hashgraph.sdk.proto.ContractID getContractID();
/**
*
**
* ID of the cryptocurrency account owned by the contract instance, in the format used in
* transactions
*
*
* .proto.AccountID accountID = 2;
* @return Whether the accountID field is set.
*/
boolean hasAccountID();
/**
*
**
* ID of the cryptocurrency account owned by the contract instance, in the format used in
* transactions
*
*
* .proto.AccountID accountID = 2;
* @return The accountID.
*/
com.hedera.hashgraph.sdk.proto.AccountID getAccountID();
/**
*
**
* ID of both the contract instance and the cryptocurrency account owned by the contract
* instance, in the format used by Solidity
*
*
* string contractAccountID = 3;
* @return The contractAccountID.
*/
java.lang.String getContractAccountID();
/**
*
**
* ID of both the contract instance and the cryptocurrency account owned by the contract
* instance, in the format used by Solidity
*
*
* string contractAccountID = 3;
* @return The bytes for contractAccountID.
*/
com.google.protobuf.ByteString
getContractAccountIDBytes();
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator
* to authorize changing the admin keys, so there can never be any admin keys for that
* instance.
*
*
* .proto.Key adminKey = 4;
* @return Whether the adminKey field is set.
*/
boolean hasAdminKey();
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator
* to authorize changing the admin keys, so there can never be any admin keys for that
* instance.
*
*
* .proto.Key adminKey = 4;
* @return The adminKey.
*/
com.hedera.hashgraph.sdk.proto.Key getAdminKey();
/**
*
**
* the current time at which this contract instance (and its account) is set to expire
*
*
* .proto.Timestamp expirationTime = 5;
* @return Whether the expirationTime field is set.
*/
boolean hasExpirationTime();
/**
*
**
* the current time at which this contract instance (and its account) is set to expire
*
*
* .proto.Timestamp expirationTime = 5;
* @return The expirationTime.
*/
com.hedera.hashgraph.sdk.proto.Timestamp getExpirationTime();
/**
*
**
* the expiration time will extend every this many seconds. If there are insufficient funds,
* then it extends as long as possible. If the account is empty when it expires, then it is
* deleted.
*
*
* .proto.Duration autoRenewPeriod = 6;
* @return Whether the autoRenewPeriod field is set.
*/
boolean hasAutoRenewPeriod();
/**
*
**
* the expiration time will extend every this many seconds. If there are insufficient funds,
* then it extends as long as possible. If the account is empty when it expires, then it is
* deleted.
*
*
* .proto.Duration autoRenewPeriod = 6;
* @return The autoRenewPeriod.
*/
com.hedera.hashgraph.sdk.proto.Duration getAutoRenewPeriod();
/**
*
**
* number of bytes of storage being used by this instance (which affects the cost to extend
* the expiration time)
*
*
* int64 storage = 7;
* @return The storage.
*/
long getStorage();
/**
*
**
* the memo associated with the contract (max 100 bytes)
*
*
* string memo = 8;
* @return The memo.
*/
java.lang.String getMemo();
/**
*
**
* the memo associated with the contract (max 100 bytes)
*
*
* string memo = 8;
* @return The bytes for memo.
*/
com.google.protobuf.ByteString
getMemoBytes();
/**
*
**
* The current balance, in tinybars
*
*
* uint64 balance = 9;
* @return The balance.
*/
long getBalance();
/**
*
**
* Whether the contract has been deleted
*
*
* bool deleted = 10;
* @return The deleted.
*/
boolean getDeleted();
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Deprecated java.util.List
getTokenRelationshipsList();
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Deprecated com.hedera.hashgraph.sdk.proto.TokenRelationship getTokenRelationships(int index);
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Deprecated int getTokenRelationshipsCount();
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 12;
* @return The ledgerId.
*/
com.google.protobuf.ByteString getLedgerId();
/**
*
**
* ID of the an account to charge for auto-renewal of this contract. If not set, or set to an account with zero hbar
* balance, the contract's own hbar balance will be used to cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 13;
* @return Whether the autoRenewAccountId field is set.
*/
boolean hasAutoRenewAccountId();
/**
*
**
* ID of the an account to charge for auto-renewal of this contract. If not set, or set to an account with zero hbar
* balance, the contract's own hbar balance will be used to cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 13;
* @return The autoRenewAccountId.
*/
com.hedera.hashgraph.sdk.proto.AccountID getAutoRenewAccountId();
/**
*
**
* The maximum number of tokens that a contract can be implicitly associated with.
*
*
* int32 max_automatic_token_associations = 14;
* @return The maxAutomaticTokenAssociations.
*/
int getMaxAutomaticTokenAssociations();
/**
*
**
* Staking metadata for this contract.
*
*
* .proto.StakingInfo staking_info = 15;
* @return Whether the stakingInfo field is set.
*/
boolean hasStakingInfo();
/**
*
**
* Staking metadata for this contract.
*
*
* .proto.StakingInfo staking_info = 15;
* @return The stakingInfo.
*/
com.hedera.hashgraph.sdk.proto.StakingInfo getStakingInfo();
}
/**
* Protobuf type {@code proto.ContractGetInfoResponse.ContractInfo}
*/
public static final class ContractInfo extends
com.google.protobuf.GeneratedMessageLite<
ContractInfo, ContractInfo.Builder> implements
// @@protoc_insertion_point(message_implements:proto.ContractGetInfoResponse.ContractInfo)
ContractInfoOrBuilder {
private ContractInfo() {
contractAccountID_ = "";
memo_ = "";
tokenRelationships_ = emptyProtobufList();
ledgerId_ = com.google.protobuf.ByteString.EMPTY;
}
public static final int CONTRACTID_FIELD_NUMBER = 1;
private com.hedera.hashgraph.sdk.proto.ContractID contractID_;
/**
*
**
* ID of the contract instance, in the format used in transactions
*
*
* .proto.ContractID contractID = 1;
*/
@java.lang.Override
public boolean hasContractID() {
return contractID_ != null;
}
/**
*
**
* ID of the contract instance, in the format used in transactions
*
*
* .proto.ContractID contractID = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ContractID getContractID() {
return contractID_ == null ? com.hedera.hashgraph.sdk.proto.ContractID.getDefaultInstance() : contractID_;
}
/**
*
**
* ID of the contract instance, in the format used in transactions
*
*
* .proto.ContractID contractID = 1;
*/
private void setContractID(com.hedera.hashgraph.sdk.proto.ContractID value) {
value.getClass();
contractID_ = value;
}
/**
*
**
* ID of the contract instance, in the format used in transactions
*
*
* .proto.ContractID contractID = 1;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeContractID(com.hedera.hashgraph.sdk.proto.ContractID value) {
value.getClass();
if (contractID_ != null &&
contractID_ != com.hedera.hashgraph.sdk.proto.ContractID.getDefaultInstance()) {
contractID_ =
com.hedera.hashgraph.sdk.proto.ContractID.newBuilder(contractID_).mergeFrom(value).buildPartial();
} else {
contractID_ = value;
}
}
/**
*
**
* ID of the contract instance, in the format used in transactions
*
*
* .proto.ContractID contractID = 1;
*/
private void clearContractID() { contractID_ = null;
}
public static final int ACCOUNTID_FIELD_NUMBER = 2;
private com.hedera.hashgraph.sdk.proto.AccountID accountID_;
/**
*
**
* ID of the cryptocurrency account owned by the contract instance, in the format used in
* transactions
*
*
* .proto.AccountID accountID = 2;
*/
@java.lang.Override
public boolean hasAccountID() {
return accountID_ != null;
}
/**
*
**
* ID of the cryptocurrency account owned by the contract instance, in the format used in
* transactions
*
*
* .proto.AccountID accountID = 2;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getAccountID() {
return accountID_ == null ? com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance() : accountID_;
}
/**
*
**
* ID of the cryptocurrency account owned by the contract instance, in the format used in
* transactions
*
*
* .proto.AccountID accountID = 2;
*/
private void setAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
accountID_ = value;
}
/**
*
**
* ID of the cryptocurrency account owned by the contract instance, in the format used in
* transactions
*
*
* .proto.AccountID accountID = 2;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
if (accountID_ != null &&
accountID_ != com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance()) {
accountID_ =
com.hedera.hashgraph.sdk.proto.AccountID.newBuilder(accountID_).mergeFrom(value).buildPartial();
} else {
accountID_ = value;
}
}
/**
*
**
* ID of the cryptocurrency account owned by the contract instance, in the format used in
* transactions
*
*
* .proto.AccountID accountID = 2;
*/
private void clearAccountID() { accountID_ = null;
}
public static final int CONTRACTACCOUNTID_FIELD_NUMBER = 3;
private java.lang.String contractAccountID_;
/**
*
**
* ID of both the contract instance and the cryptocurrency account owned by the contract
* instance, in the format used by Solidity
*
*
* string contractAccountID = 3;
* @return The contractAccountID.
*/
@java.lang.Override
public java.lang.String getContractAccountID() {
return contractAccountID_;
}
/**
*
**
* ID of both the contract instance and the cryptocurrency account owned by the contract
* instance, in the format used by Solidity
*
*
* string contractAccountID = 3;
* @return The bytes for contractAccountID.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getContractAccountIDBytes() {
return com.google.protobuf.ByteString.copyFromUtf8(contractAccountID_);
}
/**
*
**
* ID of both the contract instance and the cryptocurrency account owned by the contract
* instance, in the format used by Solidity
*
*
* string contractAccountID = 3;
* @param value The contractAccountID to set.
*/
private void setContractAccountID(
java.lang.String value) {
java.lang.Class> valueClass = value.getClass();
contractAccountID_ = value;
}
/**
*
**
* ID of both the contract instance and the cryptocurrency account owned by the contract
* instance, in the format used by Solidity
*
*
* string contractAccountID = 3;
*/
private void clearContractAccountID() {
contractAccountID_ = getDefaultInstance().getContractAccountID();
}
/**
*
**
* ID of both the contract instance and the cryptocurrency account owned by the contract
* instance, in the format used by Solidity
*
*
* string contractAccountID = 3;
* @param value The bytes for contractAccountID to set.
*/
private void setContractAccountIDBytes(
com.google.protobuf.ByteString value) {
checkByteStringIsUtf8(value);
contractAccountID_ = value.toStringUtf8();
}
public static final int ADMINKEY_FIELD_NUMBER = 4;
private com.hedera.hashgraph.sdk.proto.Key adminKey_;
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator
* to authorize changing the admin keys, so there can never be any admin keys for that
* instance.
*
*
* .proto.Key adminKey = 4;
*/
@java.lang.Override
public boolean hasAdminKey() {
return adminKey_ != null;
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator
* to authorize changing the admin keys, so there can never be any admin keys for that
* instance.
*
*
* .proto.Key adminKey = 4;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Key getAdminKey() {
return adminKey_ == null ? com.hedera.hashgraph.sdk.proto.Key.getDefaultInstance() : adminKey_;
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator
* to authorize changing the admin keys, so there can never be any admin keys for that
* instance.
*
*
* .proto.Key adminKey = 4;
*/
private void setAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
value.getClass();
adminKey_ = value;
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator
* to authorize changing the admin keys, so there can never be any admin keys for that
* instance.
*
*
* .proto.Key adminKey = 4;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
value.getClass();
if (adminKey_ != null &&
adminKey_ != com.hedera.hashgraph.sdk.proto.Key.getDefaultInstance()) {
adminKey_ =
com.hedera.hashgraph.sdk.proto.Key.newBuilder(adminKey_).mergeFrom(value).buildPartial();
} else {
adminKey_ = value;
}
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator
* to authorize changing the admin keys, so there can never be any admin keys for that
* instance.
*
*
* .proto.Key adminKey = 4;
*/
private void clearAdminKey() { adminKey_ = null;
}
public static final int EXPIRATIONTIME_FIELD_NUMBER = 5;
private com.hedera.hashgraph.sdk.proto.Timestamp expirationTime_;
/**
*
**
* the current time at which this contract instance (and its account) is set to expire
*
*
* .proto.Timestamp expirationTime = 5;
*/
@java.lang.Override
public boolean hasExpirationTime() {
return expirationTime_ != null;
}
/**
*
**
* the current time at which this contract instance (and its account) is set to expire
*
*
* .proto.Timestamp expirationTime = 5;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Timestamp getExpirationTime() {
return expirationTime_ == null ? com.hedera.hashgraph.sdk.proto.Timestamp.getDefaultInstance() : expirationTime_;
}
/**
*
**
* the current time at which this contract instance (and its account) is set to expire
*
*
* .proto.Timestamp expirationTime = 5;
*/
private void setExpirationTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
value.getClass();
expirationTime_ = value;
}
/**
*
**
* the current time at which this contract instance (and its account) is set to expire
*
*
* .proto.Timestamp expirationTime = 5;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeExpirationTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
value.getClass();
if (expirationTime_ != null &&
expirationTime_ != com.hedera.hashgraph.sdk.proto.Timestamp.getDefaultInstance()) {
expirationTime_ =
com.hedera.hashgraph.sdk.proto.Timestamp.newBuilder(expirationTime_).mergeFrom(value).buildPartial();
} else {
expirationTime_ = value;
}
}
/**
*
**
* the current time at which this contract instance (and its account) is set to expire
*
*
* .proto.Timestamp expirationTime = 5;
*/
private void clearExpirationTime() { expirationTime_ = null;
}
public static final int AUTORENEWPERIOD_FIELD_NUMBER = 6;
private com.hedera.hashgraph.sdk.proto.Duration autoRenewPeriod_;
/**
*
**
* the expiration time will extend every this many seconds. If there are insufficient funds,
* then it extends as long as possible. If the account is empty when it expires, then it is
* deleted.
*
*
* .proto.Duration autoRenewPeriod = 6;
*/
@java.lang.Override
public boolean hasAutoRenewPeriod() {
return autoRenewPeriod_ != null;
}
/**
*
**
* the expiration time will extend every this many seconds. If there are insufficient funds,
* then it extends as long as possible. If the account is empty when it expires, then it is
* deleted.
*
*
* .proto.Duration autoRenewPeriod = 6;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Duration getAutoRenewPeriod() {
return autoRenewPeriod_ == null ? com.hedera.hashgraph.sdk.proto.Duration.getDefaultInstance() : autoRenewPeriod_;
}
/**
*
**
* the expiration time will extend every this many seconds. If there are insufficient funds,
* then it extends as long as possible. If the account is empty when it expires, then it is
* deleted.
*
*
* .proto.Duration autoRenewPeriod = 6;
*/
private void setAutoRenewPeriod(com.hedera.hashgraph.sdk.proto.Duration value) {
value.getClass();
autoRenewPeriod_ = value;
}
/**
*
**
* the expiration time will extend every this many seconds. If there are insufficient funds,
* then it extends as long as possible. If the account is empty when it expires, then it is
* deleted.
*
*
* .proto.Duration autoRenewPeriod = 6;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeAutoRenewPeriod(com.hedera.hashgraph.sdk.proto.Duration value) {
value.getClass();
if (autoRenewPeriod_ != null &&
autoRenewPeriod_ != com.hedera.hashgraph.sdk.proto.Duration.getDefaultInstance()) {
autoRenewPeriod_ =
com.hedera.hashgraph.sdk.proto.Duration.newBuilder(autoRenewPeriod_).mergeFrom(value).buildPartial();
} else {
autoRenewPeriod_ = value;
}
}
/**
*
**
* the expiration time will extend every this many seconds. If there are insufficient funds,
* then it extends as long as possible. If the account is empty when it expires, then it is
* deleted.
*
*
* .proto.Duration autoRenewPeriod = 6;
*/
private void clearAutoRenewPeriod() { autoRenewPeriod_ = null;
}
public static final int STORAGE_FIELD_NUMBER = 7;
private long storage_;
/**
*
**
* number of bytes of storage being used by this instance (which affects the cost to extend
* the expiration time)
*
*
* int64 storage = 7;
* @return The storage.
*/
@java.lang.Override
public long getStorage() {
return storage_;
}
/**
*
**
* number of bytes of storage being used by this instance (which affects the cost to extend
* the expiration time)
*
*
* int64 storage = 7;
* @param value The storage to set.
*/
private void setStorage(long value) {
storage_ = value;
}
/**
*
**
* number of bytes of storage being used by this instance (which affects the cost to extend
* the expiration time)
*
*
* int64 storage = 7;
*/
private void clearStorage() {
storage_ = 0L;
}
public static final int MEMO_FIELD_NUMBER = 8;
private java.lang.String memo_;
/**
*
**
* the memo associated with the contract (max 100 bytes)
*
*
* string memo = 8;
* @return The memo.
*/
@java.lang.Override
public java.lang.String getMemo() {
return memo_;
}
/**
*
**
* the memo associated with the contract (max 100 bytes)
*
*
* string memo = 8;
* @return The bytes for memo.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMemoBytes() {
return com.google.protobuf.ByteString.copyFromUtf8(memo_);
}
/**
*
**
* the memo associated with the contract (max 100 bytes)
*
*
* string memo = 8;
* @param value The memo to set.
*/
private void setMemo(
java.lang.String value) {
java.lang.Class> valueClass = value.getClass();
memo_ = value;
}
/**
*
**
* the memo associated with the contract (max 100 bytes)
*
*
* string memo = 8;
*/
private void clearMemo() {
memo_ = getDefaultInstance().getMemo();
}
/**
*
**
* the memo associated with the contract (max 100 bytes)
*
*
* string memo = 8;
* @param value The bytes for memo to set.
*/
private void setMemoBytes(
com.google.protobuf.ByteString value) {
checkByteStringIsUtf8(value);
memo_ = value.toStringUtf8();
}
public static final int BALANCE_FIELD_NUMBER = 9;
private long balance_;
/**
*
**
* The current balance, in tinybars
*
*
* uint64 balance = 9;
* @return The balance.
*/
@java.lang.Override
public long getBalance() {
return balance_;
}
/**
*
**
* The current balance, in tinybars
*
*
* uint64 balance = 9;
* @param value The balance to set.
*/
private void setBalance(long value) {
balance_ = value;
}
/**
*
**
* The current balance, in tinybars
*
*
* uint64 balance = 9;
*/
private void clearBalance() {
balance_ = 0L;
}
public static final int DELETED_FIELD_NUMBER = 10;
private boolean deleted_;
/**
*
**
* Whether the contract has been deleted
*
*
* bool deleted = 10;
* @return The deleted.
*/
@java.lang.Override
public boolean getDeleted() {
return deleted_;
}
/**
*
**
* Whether the contract has been deleted
*
*
* bool deleted = 10;
* @param value The deleted to set.
*/
private void setDeleted(boolean value) {
deleted_ = value;
}
/**
*
**
* Whether the contract has been deleted
*
*
* bool deleted = 10;
*/
private void clearDeleted() {
deleted_ = false;
}
public static final int TOKENRELATIONSHIPS_FIELD_NUMBER = 11;
private com.google.protobuf.Internal.ProtobufList tokenRelationships_;
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public java.util.List getTokenRelationshipsList() {
return tokenRelationships_;
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Deprecated public java.util.List extends com.hedera.hashgraph.sdk.proto.TokenRelationshipOrBuilder>
getTokenRelationshipsOrBuilderList() {
return tokenRelationships_;
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public int getTokenRelationshipsCount() {
return tokenRelationships_.size();
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.TokenRelationship getTokenRelationships(int index) {
return tokenRelationships_.get(index);
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.TokenRelationshipOrBuilder getTokenRelationshipsOrBuilder(
int index) {
return tokenRelationships_.get(index);
}
private void ensureTokenRelationshipsIsMutable() {
com.google.protobuf.Internal.ProtobufList tmp = tokenRelationships_;
if (!tmp.isModifiable()) {
tokenRelationships_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(tmp);
}
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
private void setTokenRelationships(
int index, com.hedera.hashgraph.sdk.proto.TokenRelationship value) {
value.getClass();
ensureTokenRelationshipsIsMutable();
tokenRelationships_.set(index, value);
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
private void addTokenRelationships(com.hedera.hashgraph.sdk.proto.TokenRelationship value) {
value.getClass();
ensureTokenRelationshipsIsMutable();
tokenRelationships_.add(value);
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
private void addTokenRelationships(
int index, com.hedera.hashgraph.sdk.proto.TokenRelationship value) {
value.getClass();
ensureTokenRelationshipsIsMutable();
tokenRelationships_.add(index, value);
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
private void addAllTokenRelationships(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.TokenRelationship> values) {
ensureTokenRelationshipsIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, tokenRelationships_);
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
private void clearTokenRelationships() {
tokenRelationships_ = emptyProtobufList();
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
private void removeTokenRelationships(int index) {
ensureTokenRelationshipsIsMutable();
tokenRelationships_.remove(index);
}
public static final int LEDGER_ID_FIELD_NUMBER = 12;
private com.google.protobuf.ByteString ledgerId_;
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 12;
* @return The ledgerId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLedgerId() {
return ledgerId_;
}
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 12;
* @param value The ledgerId to set.
*/
private void setLedgerId(com.google.protobuf.ByteString value) {
java.lang.Class> valueClass = value.getClass();
ledgerId_ = value;
}
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 12;
*/
private void clearLedgerId() {
ledgerId_ = getDefaultInstance().getLedgerId();
}
public static final int AUTO_RENEW_ACCOUNT_ID_FIELD_NUMBER = 13;
private com.hedera.hashgraph.sdk.proto.AccountID autoRenewAccountId_;
/**
*
**
* ID of the an account to charge for auto-renewal of this contract. If not set, or set to an account with zero hbar
* balance, the contract's own hbar balance will be used to cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 13;
*/
@java.lang.Override
public boolean hasAutoRenewAccountId() {
return autoRenewAccountId_ != null;
}
/**
*
**
* ID of the an account to charge for auto-renewal of this contract. If not set, or set to an account with zero hbar
* balance, the contract's own hbar balance will be used to cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 13;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getAutoRenewAccountId() {
return autoRenewAccountId_ == null ? com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance() : autoRenewAccountId_;
}
/**
*
**
* ID of the an account to charge for auto-renewal of this contract. If not set, or set to an account with zero hbar
* balance, the contract's own hbar balance will be used to cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 13;
*/
private void setAutoRenewAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
autoRenewAccountId_ = value;
}
/**
*
**
* ID of the an account to charge for auto-renewal of this contract. If not set, or set to an account with zero hbar
* balance, the contract's own hbar balance will be used to cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 13;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeAutoRenewAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
if (autoRenewAccountId_ != null &&
autoRenewAccountId_ != com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance()) {
autoRenewAccountId_ =
com.hedera.hashgraph.sdk.proto.AccountID.newBuilder(autoRenewAccountId_).mergeFrom(value).buildPartial();
} else {
autoRenewAccountId_ = value;
}
}
/**
*
**
* ID of the an account to charge for auto-renewal of this contract. If not set, or set to an account with zero hbar
* balance, the contract's own hbar balance will be used to cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 13;
*/
private void clearAutoRenewAccountId() { autoRenewAccountId_ = null;
}
public static final int MAX_AUTOMATIC_TOKEN_ASSOCIATIONS_FIELD_NUMBER = 14;
private int maxAutomaticTokenAssociations_;
/**
*
**
* The maximum number of tokens that a contract can be implicitly associated with.
*
*
* int32 max_automatic_token_associations = 14;
* @return The maxAutomaticTokenAssociations.
*/
@java.lang.Override
public int getMaxAutomaticTokenAssociations() {
return maxAutomaticTokenAssociations_;
}
/**
*
**
* The maximum number of tokens that a contract can be implicitly associated with.
*
*
* int32 max_automatic_token_associations = 14;
* @param value The maxAutomaticTokenAssociations to set.
*/
private void setMaxAutomaticTokenAssociations(int value) {
maxAutomaticTokenAssociations_ = value;
}
/**
*
**
* The maximum number of tokens that a contract can be implicitly associated with.
*
*
* int32 max_automatic_token_associations = 14;
*/
private void clearMaxAutomaticTokenAssociations() {
maxAutomaticTokenAssociations_ = 0;
}
public static final int STAKING_INFO_FIELD_NUMBER = 15;
private com.hedera.hashgraph.sdk.proto.StakingInfo stakingInfo_;
/**
*
**
* Staking metadata for this contract.
*
*
* .proto.StakingInfo staking_info = 15;
*/
@java.lang.Override
public boolean hasStakingInfo() {
return stakingInfo_ != null;
}
/**
*
**
* Staking metadata for this contract.
*
*
* .proto.StakingInfo staking_info = 15;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.StakingInfo getStakingInfo() {
return stakingInfo_ == null ? com.hedera.hashgraph.sdk.proto.StakingInfo.getDefaultInstance() : stakingInfo_;
}
/**
*
**
* Staking metadata for this contract.
*
*
* .proto.StakingInfo staking_info = 15;
*/
private void setStakingInfo(com.hedera.hashgraph.sdk.proto.StakingInfo value) {
value.getClass();
stakingInfo_ = value;
}
/**
*
**
* Staking metadata for this contract.
*
*
* .proto.StakingInfo staking_info = 15;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeStakingInfo(com.hedera.hashgraph.sdk.proto.StakingInfo value) {
value.getClass();
if (stakingInfo_ != null &&
stakingInfo_ != com.hedera.hashgraph.sdk.proto.StakingInfo.getDefaultInstance()) {
stakingInfo_ =
com.hedera.hashgraph.sdk.proto.StakingInfo.newBuilder(stakingInfo_).mergeFrom(value).buildPartial();
} else {
stakingInfo_ = value;
}
}
/**
*
**
* Staking metadata for this contract.
*
*
* .proto.StakingInfo staking_info = 15;
*/
private void clearStakingInfo() { stakingInfo_ = null;
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
* Protobuf type {@code proto.ContractGetInfoResponse.ContractInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo, Builder> implements
// @@protoc_insertion_point(builder_implements:proto.ContractGetInfoResponse.ContractInfo)
com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfoOrBuilder {
// Construct using com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
*
**
* ID of the contract instance, in the format used in transactions
*
*
* .proto.ContractID contractID = 1;
*/
@java.lang.Override
public boolean hasContractID() {
return instance.hasContractID();
}
/**
*
**
* ID of the contract instance, in the format used in transactions
*
*
* .proto.ContractID contractID = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ContractID getContractID() {
return instance.getContractID();
}
/**
*
**
* ID of the contract instance, in the format used in transactions
*
*
* .proto.ContractID contractID = 1;
*/
public Builder setContractID(com.hedera.hashgraph.sdk.proto.ContractID value) {
copyOnWrite();
instance.setContractID(value);
return this;
}
/**
*
**
* ID of the contract instance, in the format used in transactions
*
*
* .proto.ContractID contractID = 1;
*/
public Builder setContractID(
com.hedera.hashgraph.sdk.proto.ContractID.Builder builderForValue) {
copyOnWrite();
instance.setContractID(builderForValue.build());
return this;
}
/**
*
**
* ID of the contract instance, in the format used in transactions
*
*
* .proto.ContractID contractID = 1;
*/
public Builder mergeContractID(com.hedera.hashgraph.sdk.proto.ContractID value) {
copyOnWrite();
instance.mergeContractID(value);
return this;
}
/**
*
**
* ID of the contract instance, in the format used in transactions
*
*
* .proto.ContractID contractID = 1;
*/
public Builder clearContractID() { copyOnWrite();
instance.clearContractID();
return this;
}
/**
*
**
* ID of the cryptocurrency account owned by the contract instance, in the format used in
* transactions
*
*
* .proto.AccountID accountID = 2;
*/
@java.lang.Override
public boolean hasAccountID() {
return instance.hasAccountID();
}
/**
*
**
* ID of the cryptocurrency account owned by the contract instance, in the format used in
* transactions
*
*
* .proto.AccountID accountID = 2;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getAccountID() {
return instance.getAccountID();
}
/**
*
**
* ID of the cryptocurrency account owned by the contract instance, in the format used in
* transactions
*
*
* .proto.AccountID accountID = 2;
*/
public Builder setAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.setAccountID(value);
return this;
}
/**
*
**
* ID of the cryptocurrency account owned by the contract instance, in the format used in
* transactions
*
*
* .proto.AccountID accountID = 2;
*/
public Builder setAccountID(
com.hedera.hashgraph.sdk.proto.AccountID.Builder builderForValue) {
copyOnWrite();
instance.setAccountID(builderForValue.build());
return this;
}
/**
*
**
* ID of the cryptocurrency account owned by the contract instance, in the format used in
* transactions
*
*
* .proto.AccountID accountID = 2;
*/
public Builder mergeAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.mergeAccountID(value);
return this;
}
/**
*
**
* ID of the cryptocurrency account owned by the contract instance, in the format used in
* transactions
*
*
* .proto.AccountID accountID = 2;
*/
public Builder clearAccountID() { copyOnWrite();
instance.clearAccountID();
return this;
}
/**
*
**
* ID of both the contract instance and the cryptocurrency account owned by the contract
* instance, in the format used by Solidity
*
*
* string contractAccountID = 3;
* @return The contractAccountID.
*/
@java.lang.Override
public java.lang.String getContractAccountID() {
return instance.getContractAccountID();
}
/**
*
**
* ID of both the contract instance and the cryptocurrency account owned by the contract
* instance, in the format used by Solidity
*
*
* string contractAccountID = 3;
* @return The bytes for contractAccountID.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getContractAccountIDBytes() {
return instance.getContractAccountIDBytes();
}
/**
*
**
* ID of both the contract instance and the cryptocurrency account owned by the contract
* instance, in the format used by Solidity
*
*
* string contractAccountID = 3;
* @param value The contractAccountID to set.
* @return This builder for chaining.
*/
public Builder setContractAccountID(
java.lang.String value) {
copyOnWrite();
instance.setContractAccountID(value);
return this;
}
/**
*
**
* ID of both the contract instance and the cryptocurrency account owned by the contract
* instance, in the format used by Solidity
*
*
* string contractAccountID = 3;
* @return This builder for chaining.
*/
public Builder clearContractAccountID() {
copyOnWrite();
instance.clearContractAccountID();
return this;
}
/**
*
**
* ID of both the contract instance and the cryptocurrency account owned by the contract
* instance, in the format used by Solidity
*
*
* string contractAccountID = 3;
* @param value The bytes for contractAccountID to set.
* @return This builder for chaining.
*/
public Builder setContractAccountIDBytes(
com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setContractAccountIDBytes(value);
return this;
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator
* to authorize changing the admin keys, so there can never be any admin keys for that
* instance.
*
*
* .proto.Key adminKey = 4;
*/
@java.lang.Override
public boolean hasAdminKey() {
return instance.hasAdminKey();
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator
* to authorize changing the admin keys, so there can never be any admin keys for that
* instance.
*
*
* .proto.Key adminKey = 4;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Key getAdminKey() {
return instance.getAdminKey();
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator
* to authorize changing the admin keys, so there can never be any admin keys for that
* instance.
*
*
* .proto.Key adminKey = 4;
*/
public Builder setAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
copyOnWrite();
instance.setAdminKey(value);
return this;
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator
* to authorize changing the admin keys, so there can never be any admin keys for that
* instance.
*
*
* .proto.Key adminKey = 4;
*/
public Builder setAdminKey(
com.hedera.hashgraph.sdk.proto.Key.Builder builderForValue) {
copyOnWrite();
instance.setAdminKey(builderForValue.build());
return this;
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator
* to authorize changing the admin keys, so there can never be any admin keys for that
* instance.
*
*
* .proto.Key adminKey = 4;
*/
public Builder mergeAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
copyOnWrite();
instance.mergeAdminKey(value);
return this;
}
/**
*
**
* the state of the instance and its fields can be modified arbitrarily if this key signs a
* transaction to modify it. If this is null, then such modifications are not possible, and
* there is no administrator that can override the normal operation of this smart contract
* instance. Note that if it is created with no admin keys, then there is no administrator
* to authorize changing the admin keys, so there can never be any admin keys for that
* instance.
*
*
* .proto.Key adminKey = 4;
*/
public Builder clearAdminKey() { copyOnWrite();
instance.clearAdminKey();
return this;
}
/**
*
**
* the current time at which this contract instance (and its account) is set to expire
*
*
* .proto.Timestamp expirationTime = 5;
*/
@java.lang.Override
public boolean hasExpirationTime() {
return instance.hasExpirationTime();
}
/**
*
**
* the current time at which this contract instance (and its account) is set to expire
*
*
* .proto.Timestamp expirationTime = 5;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Timestamp getExpirationTime() {
return instance.getExpirationTime();
}
/**
*
**
* the current time at which this contract instance (and its account) is set to expire
*
*
* .proto.Timestamp expirationTime = 5;
*/
public Builder setExpirationTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
copyOnWrite();
instance.setExpirationTime(value);
return this;
}
/**
*
**
* the current time at which this contract instance (and its account) is set to expire
*
*
* .proto.Timestamp expirationTime = 5;
*/
public Builder setExpirationTime(
com.hedera.hashgraph.sdk.proto.Timestamp.Builder builderForValue) {
copyOnWrite();
instance.setExpirationTime(builderForValue.build());
return this;
}
/**
*
**
* the current time at which this contract instance (and its account) is set to expire
*
*
* .proto.Timestamp expirationTime = 5;
*/
public Builder mergeExpirationTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
copyOnWrite();
instance.mergeExpirationTime(value);
return this;
}
/**
*
**
* the current time at which this contract instance (and its account) is set to expire
*
*
* .proto.Timestamp expirationTime = 5;
*/
public Builder clearExpirationTime() { copyOnWrite();
instance.clearExpirationTime();
return this;
}
/**
*
**
* the expiration time will extend every this many seconds. If there are insufficient funds,
* then it extends as long as possible. If the account is empty when it expires, then it is
* deleted.
*
*
* .proto.Duration autoRenewPeriod = 6;
*/
@java.lang.Override
public boolean hasAutoRenewPeriod() {
return instance.hasAutoRenewPeriod();
}
/**
*
**
* the expiration time will extend every this many seconds. If there are insufficient funds,
* then it extends as long as possible. If the account is empty when it expires, then it is
* deleted.
*
*
* .proto.Duration autoRenewPeriod = 6;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Duration getAutoRenewPeriod() {
return instance.getAutoRenewPeriod();
}
/**
*
**
* the expiration time will extend every this many seconds. If there are insufficient funds,
* then it extends as long as possible. If the account is empty when it expires, then it is
* deleted.
*
*
* .proto.Duration autoRenewPeriod = 6;
*/
public Builder setAutoRenewPeriod(com.hedera.hashgraph.sdk.proto.Duration value) {
copyOnWrite();
instance.setAutoRenewPeriod(value);
return this;
}
/**
*
**
* the expiration time will extend every this many seconds. If there are insufficient funds,
* then it extends as long as possible. If the account is empty when it expires, then it is
* deleted.
*
*
* .proto.Duration autoRenewPeriod = 6;
*/
public Builder setAutoRenewPeriod(
com.hedera.hashgraph.sdk.proto.Duration.Builder builderForValue) {
copyOnWrite();
instance.setAutoRenewPeriod(builderForValue.build());
return this;
}
/**
*
**
* the expiration time will extend every this many seconds. If there are insufficient funds,
* then it extends as long as possible. If the account is empty when it expires, then it is
* deleted.
*
*
* .proto.Duration autoRenewPeriod = 6;
*/
public Builder mergeAutoRenewPeriod(com.hedera.hashgraph.sdk.proto.Duration value) {
copyOnWrite();
instance.mergeAutoRenewPeriod(value);
return this;
}
/**
*
**
* the expiration time will extend every this many seconds. If there are insufficient funds,
* then it extends as long as possible. If the account is empty when it expires, then it is
* deleted.
*
*
* .proto.Duration autoRenewPeriod = 6;
*/
public Builder clearAutoRenewPeriod() { copyOnWrite();
instance.clearAutoRenewPeriod();
return this;
}
/**
*
**
* number of bytes of storage being used by this instance (which affects the cost to extend
* the expiration time)
*
*
* int64 storage = 7;
* @return The storage.
*/
@java.lang.Override
public long getStorage() {
return instance.getStorage();
}
/**
*
**
* number of bytes of storage being used by this instance (which affects the cost to extend
* the expiration time)
*
*
* int64 storage = 7;
* @param value The storage to set.
* @return This builder for chaining.
*/
public Builder setStorage(long value) {
copyOnWrite();
instance.setStorage(value);
return this;
}
/**
*
**
* number of bytes of storage being used by this instance (which affects the cost to extend
* the expiration time)
*
*
* int64 storage = 7;
* @return This builder for chaining.
*/
public Builder clearStorage() {
copyOnWrite();
instance.clearStorage();
return this;
}
/**
*
**
* the memo associated with the contract (max 100 bytes)
*
*
* string memo = 8;
* @return The memo.
*/
@java.lang.Override
public java.lang.String getMemo() {
return instance.getMemo();
}
/**
*
**
* the memo associated with the contract (max 100 bytes)
*
*
* string memo = 8;
* @return The bytes for memo.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMemoBytes() {
return instance.getMemoBytes();
}
/**
*
**
* the memo associated with the contract (max 100 bytes)
*
*
* string memo = 8;
* @param value The memo to set.
* @return This builder for chaining.
*/
public Builder setMemo(
java.lang.String value) {
copyOnWrite();
instance.setMemo(value);
return this;
}
/**
*
**
* the memo associated with the contract (max 100 bytes)
*
*
* string memo = 8;
* @return This builder for chaining.
*/
public Builder clearMemo() {
copyOnWrite();
instance.clearMemo();
return this;
}
/**
*
**
* the memo associated with the contract (max 100 bytes)
*
*
* string memo = 8;
* @param value The bytes for memo to set.
* @return This builder for chaining.
*/
public Builder setMemoBytes(
com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setMemoBytes(value);
return this;
}
/**
*
**
* The current balance, in tinybars
*
*
* uint64 balance = 9;
* @return The balance.
*/
@java.lang.Override
public long getBalance() {
return instance.getBalance();
}
/**
*
**
* The current balance, in tinybars
*
*
* uint64 balance = 9;
* @param value The balance to set.
* @return This builder for chaining.
*/
public Builder setBalance(long value) {
copyOnWrite();
instance.setBalance(value);
return this;
}
/**
*
**
* The current balance, in tinybars
*
*
* uint64 balance = 9;
* @return This builder for chaining.
*/
public Builder clearBalance() {
copyOnWrite();
instance.clearBalance();
return this;
}
/**
*
**
* Whether the contract has been deleted
*
*
* bool deleted = 10;
* @return The deleted.
*/
@java.lang.Override
public boolean getDeleted() {
return instance.getDeleted();
}
/**
*
**
* Whether the contract has been deleted
*
*
* bool deleted = 10;
* @param value The deleted to set.
* @return This builder for chaining.
*/
public Builder setDeleted(boolean value) {
copyOnWrite();
instance.setDeleted(value);
return this;
}
/**
*
**
* Whether the contract has been deleted
*
*
* bool deleted = 10;
* @return This builder for chaining.
*/
public Builder clearDeleted() {
copyOnWrite();
instance.clearDeleted();
return this;
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public java.util.List getTokenRelationshipsList() {
return java.util.Collections.unmodifiableList(
instance.getTokenRelationshipsList());
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public int getTokenRelationshipsCount() {
return instance.getTokenRelationshipsCount();
}/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.TokenRelationship getTokenRelationships(int index) {
return instance.getTokenRelationships(index);
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Deprecated public Builder setTokenRelationships(
int index, com.hedera.hashgraph.sdk.proto.TokenRelationship value) {
copyOnWrite();
instance.setTokenRelationships(index, value);
return this;
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Deprecated public Builder setTokenRelationships(
int index, com.hedera.hashgraph.sdk.proto.TokenRelationship.Builder builderForValue) {
copyOnWrite();
instance.setTokenRelationships(index,
builderForValue.build());
return this;
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Deprecated public Builder addTokenRelationships(com.hedera.hashgraph.sdk.proto.TokenRelationship value) {
copyOnWrite();
instance.addTokenRelationships(value);
return this;
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Deprecated public Builder addTokenRelationships(
int index, com.hedera.hashgraph.sdk.proto.TokenRelationship value) {
copyOnWrite();
instance.addTokenRelationships(index, value);
return this;
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Deprecated public Builder addTokenRelationships(
com.hedera.hashgraph.sdk.proto.TokenRelationship.Builder builderForValue) {
copyOnWrite();
instance.addTokenRelationships(builderForValue.build());
return this;
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Deprecated public Builder addTokenRelationships(
int index, com.hedera.hashgraph.sdk.proto.TokenRelationship.Builder builderForValue) {
copyOnWrite();
instance.addTokenRelationships(index,
builderForValue.build());
return this;
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Deprecated public Builder addAllTokenRelationships(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.TokenRelationship> values) {
copyOnWrite();
instance.addAllTokenRelationships(values);
return this;
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearTokenRelationships() {
copyOnWrite();
instance.clearTokenRelationships();
return this;
}
/**
*
**
* [DEPRECATED] The metadata of the tokens associated to the contract. This field was
* deprecated by <a href="https://hips.hedera.com/hip/hip-367">HIP-367</a>, which allowed
* an account to be associated to an unlimited number of tokens. This scale makes it more
* efficient for users to consult mirror nodes to review their token associations.
*
*
* repeated .proto.TokenRelationship tokenRelationships = 11 [deprecated = true];
*/
@java.lang.Deprecated public Builder removeTokenRelationships(int index) {
copyOnWrite();
instance.removeTokenRelationships(index);
return this;
}
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 12;
* @return The ledgerId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLedgerId() {
return instance.getLedgerId();
}
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 12;
* @param value The ledgerId to set.
* @return This builder for chaining.
*/
public Builder setLedgerId(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setLedgerId(value);
return this;
}
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 12;
* @return This builder for chaining.
*/
public Builder clearLedgerId() {
copyOnWrite();
instance.clearLedgerId();
return this;
}
/**
*
**
* ID of the an account to charge for auto-renewal of this contract. If not set, or set to an account with zero hbar
* balance, the contract's own hbar balance will be used to cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 13;
*/
@java.lang.Override
public boolean hasAutoRenewAccountId() {
return instance.hasAutoRenewAccountId();
}
/**
*
**
* ID of the an account to charge for auto-renewal of this contract. If not set, or set to an account with zero hbar
* balance, the contract's own hbar balance will be used to cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 13;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getAutoRenewAccountId() {
return instance.getAutoRenewAccountId();
}
/**
*
**
* ID of the an account to charge for auto-renewal of this contract. If not set, or set to an account with zero hbar
* balance, the contract's own hbar balance will be used to cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 13;
*/
public Builder setAutoRenewAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.setAutoRenewAccountId(value);
return this;
}
/**
*
**
* ID of the an account to charge for auto-renewal of this contract. If not set, or set to an account with zero hbar
* balance, the contract's own hbar balance will be used to cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 13;
*/
public Builder setAutoRenewAccountId(
com.hedera.hashgraph.sdk.proto.AccountID.Builder builderForValue) {
copyOnWrite();
instance.setAutoRenewAccountId(builderForValue.build());
return this;
}
/**
*
**
* ID of the an account to charge for auto-renewal of this contract. If not set, or set to an account with zero hbar
* balance, the contract's own hbar balance will be used to cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 13;
*/
public Builder mergeAutoRenewAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.mergeAutoRenewAccountId(value);
return this;
}
/**
*
**
* ID of the an account to charge for auto-renewal of this contract. If not set, or set to an account with zero hbar
* balance, the contract's own hbar balance will be used to cover auto-renewal fees.
*
*
* .proto.AccountID auto_renew_account_id = 13;
*/
public Builder clearAutoRenewAccountId() { copyOnWrite();
instance.clearAutoRenewAccountId();
return this;
}
/**
*
**
* The maximum number of tokens that a contract can be implicitly associated with.
*
*
* int32 max_automatic_token_associations = 14;
* @return The maxAutomaticTokenAssociations.
*/
@java.lang.Override
public int getMaxAutomaticTokenAssociations() {
return instance.getMaxAutomaticTokenAssociations();
}
/**
*
**
* The maximum number of tokens that a contract can be implicitly associated with.
*
*
* int32 max_automatic_token_associations = 14;
* @param value The maxAutomaticTokenAssociations to set.
* @return This builder for chaining.
*/
public Builder setMaxAutomaticTokenAssociations(int value) {
copyOnWrite();
instance.setMaxAutomaticTokenAssociations(value);
return this;
}
/**
*
**
* The maximum number of tokens that a contract can be implicitly associated with.
*
*
* int32 max_automatic_token_associations = 14;
* @return This builder for chaining.
*/
public Builder clearMaxAutomaticTokenAssociations() {
copyOnWrite();
instance.clearMaxAutomaticTokenAssociations();
return this;
}
/**
*
**
* Staking metadata for this contract.
*
*
* .proto.StakingInfo staking_info = 15;
*/
@java.lang.Override
public boolean hasStakingInfo() {
return instance.hasStakingInfo();
}
/**
*
**
* Staking metadata for this contract.
*
*
* .proto.StakingInfo staking_info = 15;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.StakingInfo getStakingInfo() {
return instance.getStakingInfo();
}
/**
*
**
* Staking metadata for this contract.
*
*
* .proto.StakingInfo staking_info = 15;
*/
public Builder setStakingInfo(com.hedera.hashgraph.sdk.proto.StakingInfo value) {
copyOnWrite();
instance.setStakingInfo(value);
return this;
}
/**
*
**
* Staking metadata for this contract.
*
*
* .proto.StakingInfo staking_info = 15;
*/
public Builder setStakingInfo(
com.hedera.hashgraph.sdk.proto.StakingInfo.Builder builderForValue) {
copyOnWrite();
instance.setStakingInfo(builderForValue.build());
return this;
}
/**
*
**
* Staking metadata for this contract.
*
*
* .proto.StakingInfo staking_info = 15;
*/
public Builder mergeStakingInfo(com.hedera.hashgraph.sdk.proto.StakingInfo value) {
copyOnWrite();
instance.mergeStakingInfo(value);
return this;
}
/**
*
**
* Staking metadata for this contract.
*
*
* .proto.StakingInfo staking_info = 15;
*/
public Builder clearStakingInfo() { copyOnWrite();
instance.clearStakingInfo();
return this;
}
// @@protoc_insertion_point(builder_scope:proto.ContractGetInfoResponse.ContractInfo)
}
@java.lang.Override
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo();
}
case NEW_BUILDER: {
return new Builder();
}
case BUILD_MESSAGE_INFO: {
java.lang.Object[] objects = new java.lang.Object[] {
"contractID_",
"accountID_",
"contractAccountID_",
"adminKey_",
"expirationTime_",
"autoRenewPeriod_",
"storage_",
"memo_",
"balance_",
"deleted_",
"tokenRelationships_",
com.hedera.hashgraph.sdk.proto.TokenRelationship.class,
"ledgerId_",
"autoRenewAccountId_",
"maxAutomaticTokenAssociations_",
"stakingInfo_",
};
java.lang.String info =
"\u0000\u000f\u0000\u0000\u0001\u000f\u000f\u0000\u0001\u0000\u0001\t\u0002\t\u0003" +
"\u0208\u0004\t\u0005\t\u0006\t\u0007\u0002\b\u0208\t\u0003\n\u0007\u000b\u001b\f" +
"\n\r\t\u000e\u0004\u000f\t";
return newMessageInfo(DEFAULT_INSTANCE, info, objects);
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
com.google.protobuf.Parser parser = PARSER;
if (parser == null) {
synchronized (com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo.class) {
parser = PARSER;
if (parser == null) {
parser =
new DefaultInstanceBasedParser(
DEFAULT_INSTANCE);
PARSER = parser;
}
}
}
return parser;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:proto.ContractGetInfoResponse.ContractInfo)
private static final com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo DEFAULT_INSTANCE;
static {
ContractInfo defaultInstance = new ContractInfo();
// New instances are implicitly immutable so no need to make
// immutable.
DEFAULT_INSTANCE = defaultInstance;
com.google.protobuf.GeneratedMessageLite.registerDefaultInstance(
ContractInfo.class, defaultInstance);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
public static final int HEADER_FIELD_NUMBER = 1;
private com.hedera.hashgraph.sdk.proto.ResponseHeader header_;
/**
*
**
* standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
@java.lang.Override
public boolean hasHeader() {
return header_ != null;
}
/**
*
**
* standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ResponseHeader getHeader() {
return header_ == null ? com.hedera.hashgraph.sdk.proto.ResponseHeader.getDefaultInstance() : header_;
}
/**
*
**
* standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
private void setHeader(com.hedera.hashgraph.sdk.proto.ResponseHeader value) {
value.getClass();
header_ = value;
}
/**
*
**
* standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeHeader(com.hedera.hashgraph.sdk.proto.ResponseHeader value) {
value.getClass();
if (header_ != null &&
header_ != com.hedera.hashgraph.sdk.proto.ResponseHeader.getDefaultInstance()) {
header_ =
com.hedera.hashgraph.sdk.proto.ResponseHeader.newBuilder(header_).mergeFrom(value).buildPartial();
} else {
header_ = value;
}
}
/**
*
**
* standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
private void clearHeader() { header_ = null;
}
public static final int CONTRACTINFO_FIELD_NUMBER = 2;
private com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo contractInfo_;
/**
*
**
* the information about this contract instance (a state proof can be generated for this)
*
*
* .proto.ContractGetInfoResponse.ContractInfo contractInfo = 2;
*/
@java.lang.Override
public boolean hasContractInfo() {
return contractInfo_ != null;
}
/**
*
**
* the information about this contract instance (a state proof can be generated for this)
*
*
* .proto.ContractGetInfoResponse.ContractInfo contractInfo = 2;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo getContractInfo() {
return contractInfo_ == null ? com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo.getDefaultInstance() : contractInfo_;
}
/**
*
**
* the information about this contract instance (a state proof can be generated for this)
*
*
* .proto.ContractGetInfoResponse.ContractInfo contractInfo = 2;
*/
private void setContractInfo(com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo value) {
value.getClass();
contractInfo_ = value;
}
/**
*
**
* the information about this contract instance (a state proof can be generated for this)
*
*
* .proto.ContractGetInfoResponse.ContractInfo contractInfo = 2;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeContractInfo(com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo value) {
value.getClass();
if (contractInfo_ != null &&
contractInfo_ != com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo.getDefaultInstance()) {
contractInfo_ =
com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo.newBuilder(contractInfo_).mergeFrom(value).buildPartial();
} else {
contractInfo_ = value;
}
}
/**
*
**
* the information about this contract instance (a state proof can be generated for this)
*
*
* .proto.ContractGetInfoResponse.ContractInfo contractInfo = 2;
*/
private void clearContractInfo() { contractInfo_ = null;
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
*
**
* Response when the client sends the node ContractGetInfoQuery
*
*
* Protobuf type {@code proto.ContractGetInfoResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse, Builder> implements
// @@protoc_insertion_point(builder_implements:proto.ContractGetInfoResponse)
com.hedera.hashgraph.sdk.proto.ContractGetInfoResponseOrBuilder {
// Construct using com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
*
**
* standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
@java.lang.Override
public boolean hasHeader() {
return instance.hasHeader();
}
/**
*
**
* standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ResponseHeader getHeader() {
return instance.getHeader();
}
/**
*
**
* standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
public Builder setHeader(com.hedera.hashgraph.sdk.proto.ResponseHeader value) {
copyOnWrite();
instance.setHeader(value);
return this;
}
/**
*
**
* standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
public Builder setHeader(
com.hedera.hashgraph.sdk.proto.ResponseHeader.Builder builderForValue) {
copyOnWrite();
instance.setHeader(builderForValue.build());
return this;
}
/**
*
**
* standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
public Builder mergeHeader(com.hedera.hashgraph.sdk.proto.ResponseHeader value) {
copyOnWrite();
instance.mergeHeader(value);
return this;
}
/**
*
**
* standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
public Builder clearHeader() { copyOnWrite();
instance.clearHeader();
return this;
}
/**
*
**
* the information about this contract instance (a state proof can be generated for this)
*
*
* .proto.ContractGetInfoResponse.ContractInfo contractInfo = 2;
*/
@java.lang.Override
public boolean hasContractInfo() {
return instance.hasContractInfo();
}
/**
*
**
* the information about this contract instance (a state proof can be generated for this)
*
*
* .proto.ContractGetInfoResponse.ContractInfo contractInfo = 2;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo getContractInfo() {
return instance.getContractInfo();
}
/**
*
**
* the information about this contract instance (a state proof can be generated for this)
*
*
* .proto.ContractGetInfoResponse.ContractInfo contractInfo = 2;
*/
public Builder setContractInfo(com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo value) {
copyOnWrite();
instance.setContractInfo(value);
return this;
}
/**
*
**
* the information about this contract instance (a state proof can be generated for this)
*
*
* .proto.ContractGetInfoResponse.ContractInfo contractInfo = 2;
*/
public Builder setContractInfo(
com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo.Builder builderForValue) {
copyOnWrite();
instance.setContractInfo(builderForValue.build());
return this;
}
/**
*
**
* the information about this contract instance (a state proof can be generated for this)
*
*
* .proto.ContractGetInfoResponse.ContractInfo contractInfo = 2;
*/
public Builder mergeContractInfo(com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.ContractInfo value) {
copyOnWrite();
instance.mergeContractInfo(value);
return this;
}
/**
*
**
* the information about this contract instance (a state proof can be generated for this)
*
*
* .proto.ContractGetInfoResponse.ContractInfo contractInfo = 2;
*/
public Builder clearContractInfo() { copyOnWrite();
instance.clearContractInfo();
return this;
}
// @@protoc_insertion_point(builder_scope:proto.ContractGetInfoResponse)
}
@java.lang.Override
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse();
}
case NEW_BUILDER: {
return new Builder();
}
case BUILD_MESSAGE_INFO: {
java.lang.Object[] objects = new java.lang.Object[] {
"header_",
"contractInfo_",
};
java.lang.String info =
"\u0000\u0002\u0000\u0000\u0001\u0002\u0002\u0000\u0000\u0000\u0001\t\u0002\t";
return newMessageInfo(DEFAULT_INSTANCE, info, objects);
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
com.google.protobuf.Parser parser = PARSER;
if (parser == null) {
synchronized (com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse.class) {
parser = PARSER;
if (parser == null) {
parser =
new DefaultInstanceBasedParser(
DEFAULT_INSTANCE);
PARSER = parser;
}
}
}
return parser;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:proto.ContractGetInfoResponse)
private static final com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse DEFAULT_INSTANCE;
static {
ContractGetInfoResponse defaultInstance = new ContractGetInfoResponse();
// New instances are implicitly immutable so no need to make
// immutable.
DEFAULT_INSTANCE = defaultInstance;
com.google.protobuf.GeneratedMessageLite.registerDefaultInstance(
ContractGetInfoResponse.class, defaultInstance);
}
public static com.hedera.hashgraph.sdk.proto.ContractGetInfoResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy