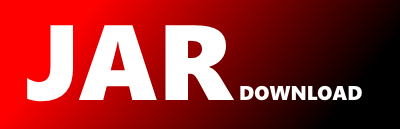
com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk-jdk7 Show documentation
Show all versions of sdk-jdk7 Show documentation
Hedera™ Hashgraph SDK for Java
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: get_account_details.proto
package com.hedera.hashgraph.sdk.proto;
/**
*
**
* Response when the client sends the node GetAccountDetailsQuery
*
*
* Protobuf type {@code proto.GetAccountDetailsResponse}
*/
public final class GetAccountDetailsResponse extends
com.google.protobuf.GeneratedMessageLite<
GetAccountDetailsResponse, GetAccountDetailsResponse.Builder> implements
// @@protoc_insertion_point(message_implements:proto.GetAccountDetailsResponse)
GetAccountDetailsResponseOrBuilder {
private GetAccountDetailsResponse() {
}
public interface AccountDetailsOrBuilder extends
// @@protoc_insertion_point(interface_extends:proto.GetAccountDetailsResponse.AccountDetails)
com.google.protobuf.MessageLiteOrBuilder {
/**
*
**
* The account ID for which this information applies
*
*
* .proto.AccountID account_id = 1;
* @return Whether the accountId field is set.
*/
boolean hasAccountId();
/**
*
**
* The account ID for which this information applies
*
*
* .proto.AccountID account_id = 1;
* @return The accountId.
*/
com.hedera.hashgraph.sdk.proto.AccountID getAccountId();
/**
*
**
* The Contract Account ID comprising of both the contract instance and the cryptocurrency
* account owned by the contract instance, in the format used by Solidity
*
*
* string contract_account_id = 2;
* @return The contractAccountId.
*/
java.lang.String getContractAccountId();
/**
*
**
* The Contract Account ID comprising of both the contract instance and the cryptocurrency
* account owned by the contract instance, in the format used by Solidity
*
*
* string contract_account_id = 2;
* @return The bytes for contractAccountId.
*/
com.google.protobuf.ByteString
getContractAccountIdBytes();
/**
*
**
* If true, then this account has been deleted, it will disappear when it expires, and all
* transactions for it will fail except the transaction to extend its expiration date
*
*
* bool deleted = 3;
* @return The deleted.
*/
boolean getDeleted();
/**
*
**
* [Deprecated] The Account ID of the account to which this is proxy staked. If proxyAccountID is null,
* or is an invalid account, or is an account that isn't a node, then this account is
* automatically proxy staked to a node chosen by the network, but without earning payments.
* If the proxyAccountID account refuses to accept proxy staking , or if it is not currently
* running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxy_account_id = 4 [deprecated = true];
* @deprecated proto.GetAccountDetailsResponse.AccountDetails.proxy_account_id is deprecated.
* See get_account_details.proto;l=88
* @return Whether the proxyAccountId field is set.
*/
@java.lang.Deprecated boolean hasProxyAccountId();
/**
*
**
* [Deprecated] The Account ID of the account to which this is proxy staked. If proxyAccountID is null,
* or is an invalid account, or is an account that isn't a node, then this account is
* automatically proxy staked to a node chosen by the network, but without earning payments.
* If the proxyAccountID account refuses to accept proxy staking , or if it is not currently
* running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxy_account_id = 4 [deprecated = true];
* @deprecated proto.GetAccountDetailsResponse.AccountDetails.proxy_account_id is deprecated.
* See get_account_details.proto;l=88
* @return The proxyAccountId.
*/
@java.lang.Deprecated com.hedera.hashgraph.sdk.proto.AccountID getProxyAccountId();
/**
*
**
* The total number of tinybars proxy staked to this account
*
*
* int64 proxy_received = 5;
* @return The proxyReceived.
*/
long getProxyReceived();
/**
*
**
* The key for the account, which must sign in order to transfer out, or to modify the
* account in any way other than extending its expiration date.
*
*
* .proto.Key key = 6;
* @return Whether the key field is set.
*/
boolean hasKey();
/**
*
**
* The key for the account, which must sign in order to transfer out, or to modify the
* account in any way other than extending its expiration date.
*
*
* .proto.Key key = 6;
* @return The key.
*/
com.hedera.hashgraph.sdk.proto.Key getKey();
/**
*
**
* The current balance of account in tinybars
*
*
* uint64 balance = 7;
* @return The balance.
*/
long getBalance();
/**
*
**
* If true, no transaction can transfer to this account unless signed by this account's key
*
*
* bool receiver_sig_required = 8;
* @return The receiverSigRequired.
*/
boolean getReceiverSigRequired();
/**
*
**
* The TimeStamp time at which this account is set to expire
*
*
* .proto.Timestamp expiration_time = 9;
* @return Whether the expirationTime field is set.
*/
boolean hasExpirationTime();
/**
*
**
* The TimeStamp time at which this account is set to expire
*
*
* .proto.Timestamp expiration_time = 9;
* @return The expirationTime.
*/
com.hedera.hashgraph.sdk.proto.Timestamp getExpirationTime();
/**
*
**
* The duration for expiration time will extend every this many seconds. If there are
* insufficient funds, then it extends as long as possible. If it is empty when it expires,
* then it is deleted.
*
*
* .proto.Duration auto_renew_period = 10;
* @return Whether the autoRenewPeriod field is set.
*/
boolean hasAutoRenewPeriod();
/**
*
**
* The duration for expiration time will extend every this many seconds. If there are
* insufficient funds, then it extends as long as possible. If it is empty when it expires,
* then it is deleted.
*
*
* .proto.Duration auto_renew_period = 10;
* @return The autoRenewPeriod.
*/
com.hedera.hashgraph.sdk.proto.Duration getAutoRenewPeriod();
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
java.util.List
getTokenRelationshipsList();
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
com.hedera.hashgraph.sdk.proto.TokenRelationship getTokenRelationships(int index);
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
int getTokenRelationshipsCount();
/**
*
**
* The memo associated with the account
*
*
* string memo = 12;
* @return The memo.
*/
java.lang.String getMemo();
/**
*
**
* The memo associated with the account
*
*
* string memo = 12;
* @return The bytes for memo.
*/
com.google.protobuf.ByteString
getMemoBytes();
/**
*
**
* The number of NFTs owned by this account
*
*
* int64 owned_nfts = 13;
* @return The ownedNfts.
*/
long getOwnedNfts();
/**
*
**
* The maximum number of tokens that an Account can be implicitly associated with.
*
*
* int32 max_automatic_token_associations = 14;
* @return The maxAutomaticTokenAssociations.
*/
int getMaxAutomaticTokenAssociations();
/**
*
**
* The alias of this account
*
*
* bytes alias = 15;
* @return The alias.
*/
com.google.protobuf.ByteString getAlias();
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 16;
* @return The ledgerId.
*/
com.google.protobuf.ByteString getLedgerId();
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
java.util.List
getGrantedCryptoAllowancesList();
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowance getGrantedCryptoAllowances(int index);
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
int getGrantedCryptoAllowancesCount();
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
java.util.List
getGrantedNftAllowancesList();
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
com.hedera.hashgraph.sdk.proto.GrantedNftAllowance getGrantedNftAllowances(int index);
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
int getGrantedNftAllowancesCount();
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
java.util.List
getGrantedTokenAllowancesList();
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
com.hedera.hashgraph.sdk.proto.GrantedTokenAllowance getGrantedTokenAllowances(int index);
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
int getGrantedTokenAllowancesCount();
}
/**
* Protobuf type {@code proto.GetAccountDetailsResponse.AccountDetails}
*/
public static final class AccountDetails extends
com.google.protobuf.GeneratedMessageLite<
AccountDetails, AccountDetails.Builder> implements
// @@protoc_insertion_point(message_implements:proto.GetAccountDetailsResponse.AccountDetails)
AccountDetailsOrBuilder {
private AccountDetails() {
contractAccountId_ = "";
tokenRelationships_ = emptyProtobufList();
memo_ = "";
alias_ = com.google.protobuf.ByteString.EMPTY;
ledgerId_ = com.google.protobuf.ByteString.EMPTY;
grantedCryptoAllowances_ = emptyProtobufList();
grantedNftAllowances_ = emptyProtobufList();
grantedTokenAllowances_ = emptyProtobufList();
}
public static final int ACCOUNT_ID_FIELD_NUMBER = 1;
private com.hedera.hashgraph.sdk.proto.AccountID accountId_;
/**
*
**
* The account ID for which this information applies
*
*
* .proto.AccountID account_id = 1;
*/
@java.lang.Override
public boolean hasAccountId() {
return accountId_ != null;
}
/**
*
**
* The account ID for which this information applies
*
*
* .proto.AccountID account_id = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getAccountId() {
return accountId_ == null ? com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance() : accountId_;
}
/**
*
**
* The account ID for which this information applies
*
*
* .proto.AccountID account_id = 1;
*/
private void setAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
accountId_ = value;
}
/**
*
**
* The account ID for which this information applies
*
*
* .proto.AccountID account_id = 1;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
if (accountId_ != null &&
accountId_ != com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance()) {
accountId_ =
com.hedera.hashgraph.sdk.proto.AccountID.newBuilder(accountId_).mergeFrom(value).buildPartial();
} else {
accountId_ = value;
}
}
/**
*
**
* The account ID for which this information applies
*
*
* .proto.AccountID account_id = 1;
*/
private void clearAccountId() { accountId_ = null;
}
public static final int CONTRACT_ACCOUNT_ID_FIELD_NUMBER = 2;
private java.lang.String contractAccountId_;
/**
*
**
* The Contract Account ID comprising of both the contract instance and the cryptocurrency
* account owned by the contract instance, in the format used by Solidity
*
*
* string contract_account_id = 2;
* @return The contractAccountId.
*/
@java.lang.Override
public java.lang.String getContractAccountId() {
return contractAccountId_;
}
/**
*
**
* The Contract Account ID comprising of both the contract instance and the cryptocurrency
* account owned by the contract instance, in the format used by Solidity
*
*
* string contract_account_id = 2;
* @return The bytes for contractAccountId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getContractAccountIdBytes() {
return com.google.protobuf.ByteString.copyFromUtf8(contractAccountId_);
}
/**
*
**
* The Contract Account ID comprising of both the contract instance and the cryptocurrency
* account owned by the contract instance, in the format used by Solidity
*
*
* string contract_account_id = 2;
* @param value The contractAccountId to set.
*/
private void setContractAccountId(
java.lang.String value) {
java.lang.Class> valueClass = value.getClass();
contractAccountId_ = value;
}
/**
*
**
* The Contract Account ID comprising of both the contract instance and the cryptocurrency
* account owned by the contract instance, in the format used by Solidity
*
*
* string contract_account_id = 2;
*/
private void clearContractAccountId() {
contractAccountId_ = getDefaultInstance().getContractAccountId();
}
/**
*
**
* The Contract Account ID comprising of both the contract instance and the cryptocurrency
* account owned by the contract instance, in the format used by Solidity
*
*
* string contract_account_id = 2;
* @param value The bytes for contractAccountId to set.
*/
private void setContractAccountIdBytes(
com.google.protobuf.ByteString value) {
checkByteStringIsUtf8(value);
contractAccountId_ = value.toStringUtf8();
}
public static final int DELETED_FIELD_NUMBER = 3;
private boolean deleted_;
/**
*
**
* If true, then this account has been deleted, it will disappear when it expires, and all
* transactions for it will fail except the transaction to extend its expiration date
*
*
* bool deleted = 3;
* @return The deleted.
*/
@java.lang.Override
public boolean getDeleted() {
return deleted_;
}
/**
*
**
* If true, then this account has been deleted, it will disappear when it expires, and all
* transactions for it will fail except the transaction to extend its expiration date
*
*
* bool deleted = 3;
* @param value The deleted to set.
*/
private void setDeleted(boolean value) {
deleted_ = value;
}
/**
*
**
* If true, then this account has been deleted, it will disappear when it expires, and all
* transactions for it will fail except the transaction to extend its expiration date
*
*
* bool deleted = 3;
*/
private void clearDeleted() {
deleted_ = false;
}
public static final int PROXY_ACCOUNT_ID_FIELD_NUMBER = 4;
private com.hedera.hashgraph.sdk.proto.AccountID proxyAccountId_;
/**
*
**
* [Deprecated] The Account ID of the account to which this is proxy staked. If proxyAccountID is null,
* or is an invalid account, or is an account that isn't a node, then this account is
* automatically proxy staked to a node chosen by the network, but without earning payments.
* If the proxyAccountID account refuses to accept proxy staking , or if it is not currently
* running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxy_account_id = 4 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasProxyAccountId() {
return proxyAccountId_ != null;
}
/**
*
**
* [Deprecated] The Account ID of the account to which this is proxy staked. If proxyAccountID is null,
* or is an invalid account, or is an account that isn't a node, then this account is
* automatically proxy staked to a node chosen by the network, but without earning payments.
* If the proxyAccountID account refuses to accept proxy staking , or if it is not currently
* running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxy_account_id = 4 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.AccountID getProxyAccountId() {
return proxyAccountId_ == null ? com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance() : proxyAccountId_;
}
/**
*
**
* [Deprecated] The Account ID of the account to which this is proxy staked. If proxyAccountID is null,
* or is an invalid account, or is an account that isn't a node, then this account is
* automatically proxy staked to a node chosen by the network, but without earning payments.
* If the proxyAccountID account refuses to accept proxy staking , or if it is not currently
* running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxy_account_id = 4 [deprecated = true];
*/
private void setProxyAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
proxyAccountId_ = value;
}
/**
*
**
* [Deprecated] The Account ID of the account to which this is proxy staked. If proxyAccountID is null,
* or is an invalid account, or is an account that isn't a node, then this account is
* automatically proxy staked to a node chosen by the network, but without earning payments.
* If the proxyAccountID account refuses to accept proxy staking , or if it is not currently
* running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxy_account_id = 4 [deprecated = true];
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeProxyAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
if (proxyAccountId_ != null &&
proxyAccountId_ != com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance()) {
proxyAccountId_ =
com.hedera.hashgraph.sdk.proto.AccountID.newBuilder(proxyAccountId_).mergeFrom(value).buildPartial();
} else {
proxyAccountId_ = value;
}
}
/**
*
**
* [Deprecated] The Account ID of the account to which this is proxy staked. If proxyAccountID is null,
* or is an invalid account, or is an account that isn't a node, then this account is
* automatically proxy staked to a node chosen by the network, but without earning payments.
* If the proxyAccountID account refuses to accept proxy staking , or if it is not currently
* running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxy_account_id = 4 [deprecated = true];
*/
private void clearProxyAccountId() { proxyAccountId_ = null;
}
public static final int PROXY_RECEIVED_FIELD_NUMBER = 5;
private long proxyReceived_;
/**
*
**
* The total number of tinybars proxy staked to this account
*
*
* int64 proxy_received = 5;
* @return The proxyReceived.
*/
@java.lang.Override
public long getProxyReceived() {
return proxyReceived_;
}
/**
*
**
* The total number of tinybars proxy staked to this account
*
*
* int64 proxy_received = 5;
* @param value The proxyReceived to set.
*/
private void setProxyReceived(long value) {
proxyReceived_ = value;
}
/**
*
**
* The total number of tinybars proxy staked to this account
*
*
* int64 proxy_received = 5;
*/
private void clearProxyReceived() {
proxyReceived_ = 0L;
}
public static final int KEY_FIELD_NUMBER = 6;
private com.hedera.hashgraph.sdk.proto.Key key_;
/**
*
**
* The key for the account, which must sign in order to transfer out, or to modify the
* account in any way other than extending its expiration date.
*
*
* .proto.Key key = 6;
*/
@java.lang.Override
public boolean hasKey() {
return key_ != null;
}
/**
*
**
* The key for the account, which must sign in order to transfer out, or to modify the
* account in any way other than extending its expiration date.
*
*
* .proto.Key key = 6;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Key getKey() {
return key_ == null ? com.hedera.hashgraph.sdk.proto.Key.getDefaultInstance() : key_;
}
/**
*
**
* The key for the account, which must sign in order to transfer out, or to modify the
* account in any way other than extending its expiration date.
*
*
* .proto.Key key = 6;
*/
private void setKey(com.hedera.hashgraph.sdk.proto.Key value) {
value.getClass();
key_ = value;
}
/**
*
**
* The key for the account, which must sign in order to transfer out, or to modify the
* account in any way other than extending its expiration date.
*
*
* .proto.Key key = 6;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeKey(com.hedera.hashgraph.sdk.proto.Key value) {
value.getClass();
if (key_ != null &&
key_ != com.hedera.hashgraph.sdk.proto.Key.getDefaultInstance()) {
key_ =
com.hedera.hashgraph.sdk.proto.Key.newBuilder(key_).mergeFrom(value).buildPartial();
} else {
key_ = value;
}
}
/**
*
**
* The key for the account, which must sign in order to transfer out, or to modify the
* account in any way other than extending its expiration date.
*
*
* .proto.Key key = 6;
*/
private void clearKey() { key_ = null;
}
public static final int BALANCE_FIELD_NUMBER = 7;
private long balance_;
/**
*
**
* The current balance of account in tinybars
*
*
* uint64 balance = 7;
* @return The balance.
*/
@java.lang.Override
public long getBalance() {
return balance_;
}
/**
*
**
* The current balance of account in tinybars
*
*
* uint64 balance = 7;
* @param value The balance to set.
*/
private void setBalance(long value) {
balance_ = value;
}
/**
*
**
* The current balance of account in tinybars
*
*
* uint64 balance = 7;
*/
private void clearBalance() {
balance_ = 0L;
}
public static final int RECEIVER_SIG_REQUIRED_FIELD_NUMBER = 8;
private boolean receiverSigRequired_;
/**
*
**
* If true, no transaction can transfer to this account unless signed by this account's key
*
*
* bool receiver_sig_required = 8;
* @return The receiverSigRequired.
*/
@java.lang.Override
public boolean getReceiverSigRequired() {
return receiverSigRequired_;
}
/**
*
**
* If true, no transaction can transfer to this account unless signed by this account's key
*
*
* bool receiver_sig_required = 8;
* @param value The receiverSigRequired to set.
*/
private void setReceiverSigRequired(boolean value) {
receiverSigRequired_ = value;
}
/**
*
**
* If true, no transaction can transfer to this account unless signed by this account's key
*
*
* bool receiver_sig_required = 8;
*/
private void clearReceiverSigRequired() {
receiverSigRequired_ = false;
}
public static final int EXPIRATION_TIME_FIELD_NUMBER = 9;
private com.hedera.hashgraph.sdk.proto.Timestamp expirationTime_;
/**
*
**
* The TimeStamp time at which this account is set to expire
*
*
* .proto.Timestamp expiration_time = 9;
*/
@java.lang.Override
public boolean hasExpirationTime() {
return expirationTime_ != null;
}
/**
*
**
* The TimeStamp time at which this account is set to expire
*
*
* .proto.Timestamp expiration_time = 9;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Timestamp getExpirationTime() {
return expirationTime_ == null ? com.hedera.hashgraph.sdk.proto.Timestamp.getDefaultInstance() : expirationTime_;
}
/**
*
**
* The TimeStamp time at which this account is set to expire
*
*
* .proto.Timestamp expiration_time = 9;
*/
private void setExpirationTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
value.getClass();
expirationTime_ = value;
}
/**
*
**
* The TimeStamp time at which this account is set to expire
*
*
* .proto.Timestamp expiration_time = 9;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeExpirationTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
value.getClass();
if (expirationTime_ != null &&
expirationTime_ != com.hedera.hashgraph.sdk.proto.Timestamp.getDefaultInstance()) {
expirationTime_ =
com.hedera.hashgraph.sdk.proto.Timestamp.newBuilder(expirationTime_).mergeFrom(value).buildPartial();
} else {
expirationTime_ = value;
}
}
/**
*
**
* The TimeStamp time at which this account is set to expire
*
*
* .proto.Timestamp expiration_time = 9;
*/
private void clearExpirationTime() { expirationTime_ = null;
}
public static final int AUTO_RENEW_PERIOD_FIELD_NUMBER = 10;
private com.hedera.hashgraph.sdk.proto.Duration autoRenewPeriod_;
/**
*
**
* The duration for expiration time will extend every this many seconds. If there are
* insufficient funds, then it extends as long as possible. If it is empty when it expires,
* then it is deleted.
*
*
* .proto.Duration auto_renew_period = 10;
*/
@java.lang.Override
public boolean hasAutoRenewPeriod() {
return autoRenewPeriod_ != null;
}
/**
*
**
* The duration for expiration time will extend every this many seconds. If there are
* insufficient funds, then it extends as long as possible. If it is empty when it expires,
* then it is deleted.
*
*
* .proto.Duration auto_renew_period = 10;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Duration getAutoRenewPeriod() {
return autoRenewPeriod_ == null ? com.hedera.hashgraph.sdk.proto.Duration.getDefaultInstance() : autoRenewPeriod_;
}
/**
*
**
* The duration for expiration time will extend every this many seconds. If there are
* insufficient funds, then it extends as long as possible. If it is empty when it expires,
* then it is deleted.
*
*
* .proto.Duration auto_renew_period = 10;
*/
private void setAutoRenewPeriod(com.hedera.hashgraph.sdk.proto.Duration value) {
value.getClass();
autoRenewPeriod_ = value;
}
/**
*
**
* The duration for expiration time will extend every this many seconds. If there are
* insufficient funds, then it extends as long as possible. If it is empty when it expires,
* then it is deleted.
*
*
* .proto.Duration auto_renew_period = 10;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeAutoRenewPeriod(com.hedera.hashgraph.sdk.proto.Duration value) {
value.getClass();
if (autoRenewPeriod_ != null &&
autoRenewPeriod_ != com.hedera.hashgraph.sdk.proto.Duration.getDefaultInstance()) {
autoRenewPeriod_ =
com.hedera.hashgraph.sdk.proto.Duration.newBuilder(autoRenewPeriod_).mergeFrom(value).buildPartial();
} else {
autoRenewPeriod_ = value;
}
}
/**
*
**
* The duration for expiration time will extend every this many seconds. If there are
* insufficient funds, then it extends as long as possible. If it is empty when it expires,
* then it is deleted.
*
*
* .proto.Duration auto_renew_period = 10;
*/
private void clearAutoRenewPeriod() { autoRenewPeriod_ = null;
}
public static final int TOKEN_RELATIONSHIPS_FIELD_NUMBER = 11;
private com.google.protobuf.Internal.ProtobufList tokenRelationships_;
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
@java.lang.Override
public java.util.List getTokenRelationshipsList() {
return tokenRelationships_;
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
public java.util.List extends com.hedera.hashgraph.sdk.proto.TokenRelationshipOrBuilder>
getTokenRelationshipsOrBuilderList() {
return tokenRelationships_;
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
@java.lang.Override
public int getTokenRelationshipsCount() {
return tokenRelationships_.size();
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.TokenRelationship getTokenRelationships(int index) {
return tokenRelationships_.get(index);
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
public com.hedera.hashgraph.sdk.proto.TokenRelationshipOrBuilder getTokenRelationshipsOrBuilder(
int index) {
return tokenRelationships_.get(index);
}
private void ensureTokenRelationshipsIsMutable() {
com.google.protobuf.Internal.ProtobufList tmp = tokenRelationships_;
if (!tmp.isModifiable()) {
tokenRelationships_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(tmp);
}
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
private void setTokenRelationships(
int index, com.hedera.hashgraph.sdk.proto.TokenRelationship value) {
value.getClass();
ensureTokenRelationshipsIsMutable();
tokenRelationships_.set(index, value);
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
private void addTokenRelationships(com.hedera.hashgraph.sdk.proto.TokenRelationship value) {
value.getClass();
ensureTokenRelationshipsIsMutable();
tokenRelationships_.add(value);
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
private void addTokenRelationships(
int index, com.hedera.hashgraph.sdk.proto.TokenRelationship value) {
value.getClass();
ensureTokenRelationshipsIsMutable();
tokenRelationships_.add(index, value);
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
private void addAllTokenRelationships(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.TokenRelationship> values) {
ensureTokenRelationshipsIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, tokenRelationships_);
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
private void clearTokenRelationships() {
tokenRelationships_ = emptyProtobufList();
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
private void removeTokenRelationships(int index) {
ensureTokenRelationshipsIsMutable();
tokenRelationships_.remove(index);
}
public static final int MEMO_FIELD_NUMBER = 12;
private java.lang.String memo_;
/**
*
**
* The memo associated with the account
*
*
* string memo = 12;
* @return The memo.
*/
@java.lang.Override
public java.lang.String getMemo() {
return memo_;
}
/**
*
**
* The memo associated with the account
*
*
* string memo = 12;
* @return The bytes for memo.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMemoBytes() {
return com.google.protobuf.ByteString.copyFromUtf8(memo_);
}
/**
*
**
* The memo associated with the account
*
*
* string memo = 12;
* @param value The memo to set.
*/
private void setMemo(
java.lang.String value) {
java.lang.Class> valueClass = value.getClass();
memo_ = value;
}
/**
*
**
* The memo associated with the account
*
*
* string memo = 12;
*/
private void clearMemo() {
memo_ = getDefaultInstance().getMemo();
}
/**
*
**
* The memo associated with the account
*
*
* string memo = 12;
* @param value The bytes for memo to set.
*/
private void setMemoBytes(
com.google.protobuf.ByteString value) {
checkByteStringIsUtf8(value);
memo_ = value.toStringUtf8();
}
public static final int OWNED_NFTS_FIELD_NUMBER = 13;
private long ownedNfts_;
/**
*
**
* The number of NFTs owned by this account
*
*
* int64 owned_nfts = 13;
* @return The ownedNfts.
*/
@java.lang.Override
public long getOwnedNfts() {
return ownedNfts_;
}
/**
*
**
* The number of NFTs owned by this account
*
*
* int64 owned_nfts = 13;
* @param value The ownedNfts to set.
*/
private void setOwnedNfts(long value) {
ownedNfts_ = value;
}
/**
*
**
* The number of NFTs owned by this account
*
*
* int64 owned_nfts = 13;
*/
private void clearOwnedNfts() {
ownedNfts_ = 0L;
}
public static final int MAX_AUTOMATIC_TOKEN_ASSOCIATIONS_FIELD_NUMBER = 14;
private int maxAutomaticTokenAssociations_;
/**
*
**
* The maximum number of tokens that an Account can be implicitly associated with.
*
*
* int32 max_automatic_token_associations = 14;
* @return The maxAutomaticTokenAssociations.
*/
@java.lang.Override
public int getMaxAutomaticTokenAssociations() {
return maxAutomaticTokenAssociations_;
}
/**
*
**
* The maximum number of tokens that an Account can be implicitly associated with.
*
*
* int32 max_automatic_token_associations = 14;
* @param value The maxAutomaticTokenAssociations to set.
*/
private void setMaxAutomaticTokenAssociations(int value) {
maxAutomaticTokenAssociations_ = value;
}
/**
*
**
* The maximum number of tokens that an Account can be implicitly associated with.
*
*
* int32 max_automatic_token_associations = 14;
*/
private void clearMaxAutomaticTokenAssociations() {
maxAutomaticTokenAssociations_ = 0;
}
public static final int ALIAS_FIELD_NUMBER = 15;
private com.google.protobuf.ByteString alias_;
/**
*
**
* The alias of this account
*
*
* bytes alias = 15;
* @return The alias.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAlias() {
return alias_;
}
/**
*
**
* The alias of this account
*
*
* bytes alias = 15;
* @param value The alias to set.
*/
private void setAlias(com.google.protobuf.ByteString value) {
java.lang.Class> valueClass = value.getClass();
alias_ = value;
}
/**
*
**
* The alias of this account
*
*
* bytes alias = 15;
*/
private void clearAlias() {
alias_ = getDefaultInstance().getAlias();
}
public static final int LEDGER_ID_FIELD_NUMBER = 16;
private com.google.protobuf.ByteString ledgerId_;
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 16;
* @return The ledgerId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLedgerId() {
return ledgerId_;
}
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 16;
* @param value The ledgerId to set.
*/
private void setLedgerId(com.google.protobuf.ByteString value) {
java.lang.Class> valueClass = value.getClass();
ledgerId_ = value;
}
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 16;
*/
private void clearLedgerId() {
ledgerId_ = getDefaultInstance().getLedgerId();
}
public static final int GRANTED_CRYPTO_ALLOWANCES_FIELD_NUMBER = 17;
private com.google.protobuf.Internal.ProtobufList grantedCryptoAllowances_;
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
@java.lang.Override
public java.util.List getGrantedCryptoAllowancesList() {
return grantedCryptoAllowances_;
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
public java.util.List extends com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowanceOrBuilder>
getGrantedCryptoAllowancesOrBuilderList() {
return grantedCryptoAllowances_;
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
@java.lang.Override
public int getGrantedCryptoAllowancesCount() {
return grantedCryptoAllowances_.size();
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowance getGrantedCryptoAllowances(int index) {
return grantedCryptoAllowances_.get(index);
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
public com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowanceOrBuilder getGrantedCryptoAllowancesOrBuilder(
int index) {
return grantedCryptoAllowances_.get(index);
}
private void ensureGrantedCryptoAllowancesIsMutable() {
com.google.protobuf.Internal.ProtobufList tmp = grantedCryptoAllowances_;
if (!tmp.isModifiable()) {
grantedCryptoAllowances_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(tmp);
}
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
private void setGrantedCryptoAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowance value) {
value.getClass();
ensureGrantedCryptoAllowancesIsMutable();
grantedCryptoAllowances_.set(index, value);
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
private void addGrantedCryptoAllowances(com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowance value) {
value.getClass();
ensureGrantedCryptoAllowancesIsMutable();
grantedCryptoAllowances_.add(value);
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
private void addGrantedCryptoAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowance value) {
value.getClass();
ensureGrantedCryptoAllowancesIsMutable();
grantedCryptoAllowances_.add(index, value);
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
private void addAllGrantedCryptoAllowances(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowance> values) {
ensureGrantedCryptoAllowancesIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, grantedCryptoAllowances_);
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
private void clearGrantedCryptoAllowances() {
grantedCryptoAllowances_ = emptyProtobufList();
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
private void removeGrantedCryptoAllowances(int index) {
ensureGrantedCryptoAllowancesIsMutable();
grantedCryptoAllowances_.remove(index);
}
public static final int GRANTED_NFT_ALLOWANCES_FIELD_NUMBER = 18;
private com.google.protobuf.Internal.ProtobufList grantedNftAllowances_;
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
@java.lang.Override
public java.util.List getGrantedNftAllowancesList() {
return grantedNftAllowances_;
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
public java.util.List extends com.hedera.hashgraph.sdk.proto.GrantedNftAllowanceOrBuilder>
getGrantedNftAllowancesOrBuilderList() {
return grantedNftAllowances_;
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
@java.lang.Override
public int getGrantedNftAllowancesCount() {
return grantedNftAllowances_.size();
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.GrantedNftAllowance getGrantedNftAllowances(int index) {
return grantedNftAllowances_.get(index);
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
public com.hedera.hashgraph.sdk.proto.GrantedNftAllowanceOrBuilder getGrantedNftAllowancesOrBuilder(
int index) {
return grantedNftAllowances_.get(index);
}
private void ensureGrantedNftAllowancesIsMutable() {
com.google.protobuf.Internal.ProtobufList tmp = grantedNftAllowances_;
if (!tmp.isModifiable()) {
grantedNftAllowances_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(tmp);
}
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
private void setGrantedNftAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedNftAllowance value) {
value.getClass();
ensureGrantedNftAllowancesIsMutable();
grantedNftAllowances_.set(index, value);
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
private void addGrantedNftAllowances(com.hedera.hashgraph.sdk.proto.GrantedNftAllowance value) {
value.getClass();
ensureGrantedNftAllowancesIsMutable();
grantedNftAllowances_.add(value);
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
private void addGrantedNftAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedNftAllowance value) {
value.getClass();
ensureGrantedNftAllowancesIsMutable();
grantedNftAllowances_.add(index, value);
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
private void addAllGrantedNftAllowances(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.GrantedNftAllowance> values) {
ensureGrantedNftAllowancesIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, grantedNftAllowances_);
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
private void clearGrantedNftAllowances() {
grantedNftAllowances_ = emptyProtobufList();
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
private void removeGrantedNftAllowances(int index) {
ensureGrantedNftAllowancesIsMutable();
grantedNftAllowances_.remove(index);
}
public static final int GRANTED_TOKEN_ALLOWANCES_FIELD_NUMBER = 19;
private com.google.protobuf.Internal.ProtobufList grantedTokenAllowances_;
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
@java.lang.Override
public java.util.List getGrantedTokenAllowancesList() {
return grantedTokenAllowances_;
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
public java.util.List extends com.hedera.hashgraph.sdk.proto.GrantedTokenAllowanceOrBuilder>
getGrantedTokenAllowancesOrBuilderList() {
return grantedTokenAllowances_;
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
@java.lang.Override
public int getGrantedTokenAllowancesCount() {
return grantedTokenAllowances_.size();
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.GrantedTokenAllowance getGrantedTokenAllowances(int index) {
return grantedTokenAllowances_.get(index);
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
public com.hedera.hashgraph.sdk.proto.GrantedTokenAllowanceOrBuilder getGrantedTokenAllowancesOrBuilder(
int index) {
return grantedTokenAllowances_.get(index);
}
private void ensureGrantedTokenAllowancesIsMutable() {
com.google.protobuf.Internal.ProtobufList tmp = grantedTokenAllowances_;
if (!tmp.isModifiable()) {
grantedTokenAllowances_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(tmp);
}
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
private void setGrantedTokenAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedTokenAllowance value) {
value.getClass();
ensureGrantedTokenAllowancesIsMutable();
grantedTokenAllowances_.set(index, value);
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
private void addGrantedTokenAllowances(com.hedera.hashgraph.sdk.proto.GrantedTokenAllowance value) {
value.getClass();
ensureGrantedTokenAllowancesIsMutable();
grantedTokenAllowances_.add(value);
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
private void addGrantedTokenAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedTokenAllowance value) {
value.getClass();
ensureGrantedTokenAllowancesIsMutable();
grantedTokenAllowances_.add(index, value);
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
private void addAllGrantedTokenAllowances(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.GrantedTokenAllowance> values) {
ensureGrantedTokenAllowancesIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, grantedTokenAllowances_);
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
private void clearGrantedTokenAllowances() {
grantedTokenAllowances_ = emptyProtobufList();
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
private void removeGrantedTokenAllowances(int index) {
ensureGrantedTokenAllowancesIsMutable();
grantedTokenAllowances_.remove(index);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
* Protobuf type {@code proto.GetAccountDetailsResponse.AccountDetails}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails, Builder> implements
// @@protoc_insertion_point(builder_implements:proto.GetAccountDetailsResponse.AccountDetails)
com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetailsOrBuilder {
// Construct using com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
*
**
* The account ID for which this information applies
*
*
* .proto.AccountID account_id = 1;
*/
@java.lang.Override
public boolean hasAccountId() {
return instance.hasAccountId();
}
/**
*
**
* The account ID for which this information applies
*
*
* .proto.AccountID account_id = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getAccountId() {
return instance.getAccountId();
}
/**
*
**
* The account ID for which this information applies
*
*
* .proto.AccountID account_id = 1;
*/
public Builder setAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.setAccountId(value);
return this;
}
/**
*
**
* The account ID for which this information applies
*
*
* .proto.AccountID account_id = 1;
*/
public Builder setAccountId(
com.hedera.hashgraph.sdk.proto.AccountID.Builder builderForValue) {
copyOnWrite();
instance.setAccountId(builderForValue.build());
return this;
}
/**
*
**
* The account ID for which this information applies
*
*
* .proto.AccountID account_id = 1;
*/
public Builder mergeAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.mergeAccountId(value);
return this;
}
/**
*
**
* The account ID for which this information applies
*
*
* .proto.AccountID account_id = 1;
*/
public Builder clearAccountId() { copyOnWrite();
instance.clearAccountId();
return this;
}
/**
*
**
* The Contract Account ID comprising of both the contract instance and the cryptocurrency
* account owned by the contract instance, in the format used by Solidity
*
*
* string contract_account_id = 2;
* @return The contractAccountId.
*/
@java.lang.Override
public java.lang.String getContractAccountId() {
return instance.getContractAccountId();
}
/**
*
**
* The Contract Account ID comprising of both the contract instance and the cryptocurrency
* account owned by the contract instance, in the format used by Solidity
*
*
* string contract_account_id = 2;
* @return The bytes for contractAccountId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getContractAccountIdBytes() {
return instance.getContractAccountIdBytes();
}
/**
*
**
* The Contract Account ID comprising of both the contract instance and the cryptocurrency
* account owned by the contract instance, in the format used by Solidity
*
*
* string contract_account_id = 2;
* @param value The contractAccountId to set.
* @return This builder for chaining.
*/
public Builder setContractAccountId(
java.lang.String value) {
copyOnWrite();
instance.setContractAccountId(value);
return this;
}
/**
*
**
* The Contract Account ID comprising of both the contract instance and the cryptocurrency
* account owned by the contract instance, in the format used by Solidity
*
*
* string contract_account_id = 2;
* @return This builder for chaining.
*/
public Builder clearContractAccountId() {
copyOnWrite();
instance.clearContractAccountId();
return this;
}
/**
*
**
* The Contract Account ID comprising of both the contract instance and the cryptocurrency
* account owned by the contract instance, in the format used by Solidity
*
*
* string contract_account_id = 2;
* @param value The bytes for contractAccountId to set.
* @return This builder for chaining.
*/
public Builder setContractAccountIdBytes(
com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setContractAccountIdBytes(value);
return this;
}
/**
*
**
* If true, then this account has been deleted, it will disappear when it expires, and all
* transactions for it will fail except the transaction to extend its expiration date
*
*
* bool deleted = 3;
* @return The deleted.
*/
@java.lang.Override
public boolean getDeleted() {
return instance.getDeleted();
}
/**
*
**
* If true, then this account has been deleted, it will disappear when it expires, and all
* transactions for it will fail except the transaction to extend its expiration date
*
*
* bool deleted = 3;
* @param value The deleted to set.
* @return This builder for chaining.
*/
public Builder setDeleted(boolean value) {
copyOnWrite();
instance.setDeleted(value);
return this;
}
/**
*
**
* If true, then this account has been deleted, it will disappear when it expires, and all
* transactions for it will fail except the transaction to extend its expiration date
*
*
* bool deleted = 3;
* @return This builder for chaining.
*/
public Builder clearDeleted() {
copyOnWrite();
instance.clearDeleted();
return this;
}
/**
*
**
* [Deprecated] The Account ID of the account to which this is proxy staked. If proxyAccountID is null,
* or is an invalid account, or is an account that isn't a node, then this account is
* automatically proxy staked to a node chosen by the network, but without earning payments.
* If the proxyAccountID account refuses to accept proxy staking , or if it is not currently
* running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxy_account_id = 4 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasProxyAccountId() {
return instance.hasProxyAccountId();
}
/**
*
**
* [Deprecated] The Account ID of the account to which this is proxy staked. If proxyAccountID is null,
* or is an invalid account, or is an account that isn't a node, then this account is
* automatically proxy staked to a node chosen by the network, but without earning payments.
* If the proxyAccountID account refuses to accept proxy staking , or if it is not currently
* running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxy_account_id = 4 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.AccountID getProxyAccountId() {
return instance.getProxyAccountId();
}
/**
*
**
* [Deprecated] The Account ID of the account to which this is proxy staked. If proxyAccountID is null,
* or is an invalid account, or is an account that isn't a node, then this account is
* automatically proxy staked to a node chosen by the network, but without earning payments.
* If the proxyAccountID account refuses to accept proxy staking , or if it is not currently
* running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxy_account_id = 4 [deprecated = true];
*/
@java.lang.Deprecated public Builder setProxyAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.setProxyAccountId(value);
return this;
}
/**
*
**
* [Deprecated] The Account ID of the account to which this is proxy staked. If proxyAccountID is null,
* or is an invalid account, or is an account that isn't a node, then this account is
* automatically proxy staked to a node chosen by the network, but without earning payments.
* If the proxyAccountID account refuses to accept proxy staking , or if it is not currently
* running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxy_account_id = 4 [deprecated = true];
*/
@java.lang.Deprecated public Builder setProxyAccountId(
com.hedera.hashgraph.sdk.proto.AccountID.Builder builderForValue) {
copyOnWrite();
instance.setProxyAccountId(builderForValue.build());
return this;
}
/**
*
**
* [Deprecated] The Account ID of the account to which this is proxy staked. If proxyAccountID is null,
* or is an invalid account, or is an account that isn't a node, then this account is
* automatically proxy staked to a node chosen by the network, but without earning payments.
* If the proxyAccountID account refuses to accept proxy staking , or if it is not currently
* running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxy_account_id = 4 [deprecated = true];
*/
@java.lang.Deprecated public Builder mergeProxyAccountId(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.mergeProxyAccountId(value);
return this;
}
/**
*
**
* [Deprecated] The Account ID of the account to which this is proxy staked. If proxyAccountID is null,
* or is an invalid account, or is an account that isn't a node, then this account is
* automatically proxy staked to a node chosen by the network, but without earning payments.
* If the proxyAccountID account refuses to accept proxy staking , or if it is not currently
* running a node, then it will behave as if proxyAccountID was null.
*
*
* .proto.AccountID proxy_account_id = 4 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearProxyAccountId() { copyOnWrite();
instance.clearProxyAccountId();
return this;
}
/**
*
**
* The total number of tinybars proxy staked to this account
*
*
* int64 proxy_received = 5;
* @return The proxyReceived.
*/
@java.lang.Override
public long getProxyReceived() {
return instance.getProxyReceived();
}
/**
*
**
* The total number of tinybars proxy staked to this account
*
*
* int64 proxy_received = 5;
* @param value The proxyReceived to set.
* @return This builder for chaining.
*/
public Builder setProxyReceived(long value) {
copyOnWrite();
instance.setProxyReceived(value);
return this;
}
/**
*
**
* The total number of tinybars proxy staked to this account
*
*
* int64 proxy_received = 5;
* @return This builder for chaining.
*/
public Builder clearProxyReceived() {
copyOnWrite();
instance.clearProxyReceived();
return this;
}
/**
*
**
* The key for the account, which must sign in order to transfer out, or to modify the
* account in any way other than extending its expiration date.
*
*
* .proto.Key key = 6;
*/
@java.lang.Override
public boolean hasKey() {
return instance.hasKey();
}
/**
*
**
* The key for the account, which must sign in order to transfer out, or to modify the
* account in any way other than extending its expiration date.
*
*
* .proto.Key key = 6;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Key getKey() {
return instance.getKey();
}
/**
*
**
* The key for the account, which must sign in order to transfer out, or to modify the
* account in any way other than extending its expiration date.
*
*
* .proto.Key key = 6;
*/
public Builder setKey(com.hedera.hashgraph.sdk.proto.Key value) {
copyOnWrite();
instance.setKey(value);
return this;
}
/**
*
**
* The key for the account, which must sign in order to transfer out, or to modify the
* account in any way other than extending its expiration date.
*
*
* .proto.Key key = 6;
*/
public Builder setKey(
com.hedera.hashgraph.sdk.proto.Key.Builder builderForValue) {
copyOnWrite();
instance.setKey(builderForValue.build());
return this;
}
/**
*
**
* The key for the account, which must sign in order to transfer out, or to modify the
* account in any way other than extending its expiration date.
*
*
* .proto.Key key = 6;
*/
public Builder mergeKey(com.hedera.hashgraph.sdk.proto.Key value) {
copyOnWrite();
instance.mergeKey(value);
return this;
}
/**
*
**
* The key for the account, which must sign in order to transfer out, or to modify the
* account in any way other than extending its expiration date.
*
*
* .proto.Key key = 6;
*/
public Builder clearKey() { copyOnWrite();
instance.clearKey();
return this;
}
/**
*
**
* The current balance of account in tinybars
*
*
* uint64 balance = 7;
* @return The balance.
*/
@java.lang.Override
public long getBalance() {
return instance.getBalance();
}
/**
*
**
* The current balance of account in tinybars
*
*
* uint64 balance = 7;
* @param value The balance to set.
* @return This builder for chaining.
*/
public Builder setBalance(long value) {
copyOnWrite();
instance.setBalance(value);
return this;
}
/**
*
**
* The current balance of account in tinybars
*
*
* uint64 balance = 7;
* @return This builder for chaining.
*/
public Builder clearBalance() {
copyOnWrite();
instance.clearBalance();
return this;
}
/**
*
**
* If true, no transaction can transfer to this account unless signed by this account's key
*
*
* bool receiver_sig_required = 8;
* @return The receiverSigRequired.
*/
@java.lang.Override
public boolean getReceiverSigRequired() {
return instance.getReceiverSigRequired();
}
/**
*
**
* If true, no transaction can transfer to this account unless signed by this account's key
*
*
* bool receiver_sig_required = 8;
* @param value The receiverSigRequired to set.
* @return This builder for chaining.
*/
public Builder setReceiverSigRequired(boolean value) {
copyOnWrite();
instance.setReceiverSigRequired(value);
return this;
}
/**
*
**
* If true, no transaction can transfer to this account unless signed by this account's key
*
*
* bool receiver_sig_required = 8;
* @return This builder for chaining.
*/
public Builder clearReceiverSigRequired() {
copyOnWrite();
instance.clearReceiverSigRequired();
return this;
}
/**
*
**
* The TimeStamp time at which this account is set to expire
*
*
* .proto.Timestamp expiration_time = 9;
*/
@java.lang.Override
public boolean hasExpirationTime() {
return instance.hasExpirationTime();
}
/**
*
**
* The TimeStamp time at which this account is set to expire
*
*
* .proto.Timestamp expiration_time = 9;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Timestamp getExpirationTime() {
return instance.getExpirationTime();
}
/**
*
**
* The TimeStamp time at which this account is set to expire
*
*
* .proto.Timestamp expiration_time = 9;
*/
public Builder setExpirationTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
copyOnWrite();
instance.setExpirationTime(value);
return this;
}
/**
*
**
* The TimeStamp time at which this account is set to expire
*
*
* .proto.Timestamp expiration_time = 9;
*/
public Builder setExpirationTime(
com.hedera.hashgraph.sdk.proto.Timestamp.Builder builderForValue) {
copyOnWrite();
instance.setExpirationTime(builderForValue.build());
return this;
}
/**
*
**
* The TimeStamp time at which this account is set to expire
*
*
* .proto.Timestamp expiration_time = 9;
*/
public Builder mergeExpirationTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
copyOnWrite();
instance.mergeExpirationTime(value);
return this;
}
/**
*
**
* The TimeStamp time at which this account is set to expire
*
*
* .proto.Timestamp expiration_time = 9;
*/
public Builder clearExpirationTime() { copyOnWrite();
instance.clearExpirationTime();
return this;
}
/**
*
**
* The duration for expiration time will extend every this many seconds. If there are
* insufficient funds, then it extends as long as possible. If it is empty when it expires,
* then it is deleted.
*
*
* .proto.Duration auto_renew_period = 10;
*/
@java.lang.Override
public boolean hasAutoRenewPeriod() {
return instance.hasAutoRenewPeriod();
}
/**
*
**
* The duration for expiration time will extend every this many seconds. If there are
* insufficient funds, then it extends as long as possible. If it is empty when it expires,
* then it is deleted.
*
*
* .proto.Duration auto_renew_period = 10;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Duration getAutoRenewPeriod() {
return instance.getAutoRenewPeriod();
}
/**
*
**
* The duration for expiration time will extend every this many seconds. If there are
* insufficient funds, then it extends as long as possible. If it is empty when it expires,
* then it is deleted.
*
*
* .proto.Duration auto_renew_period = 10;
*/
public Builder setAutoRenewPeriod(com.hedera.hashgraph.sdk.proto.Duration value) {
copyOnWrite();
instance.setAutoRenewPeriod(value);
return this;
}
/**
*
**
* The duration for expiration time will extend every this many seconds. If there are
* insufficient funds, then it extends as long as possible. If it is empty when it expires,
* then it is deleted.
*
*
* .proto.Duration auto_renew_period = 10;
*/
public Builder setAutoRenewPeriod(
com.hedera.hashgraph.sdk.proto.Duration.Builder builderForValue) {
copyOnWrite();
instance.setAutoRenewPeriod(builderForValue.build());
return this;
}
/**
*
**
* The duration for expiration time will extend every this many seconds. If there are
* insufficient funds, then it extends as long as possible. If it is empty when it expires,
* then it is deleted.
*
*
* .proto.Duration auto_renew_period = 10;
*/
public Builder mergeAutoRenewPeriod(com.hedera.hashgraph.sdk.proto.Duration value) {
copyOnWrite();
instance.mergeAutoRenewPeriod(value);
return this;
}
/**
*
**
* The duration for expiration time will extend every this many seconds. If there are
* insufficient funds, then it extends as long as possible. If it is empty when it expires,
* then it is deleted.
*
*
* .proto.Duration auto_renew_period = 10;
*/
public Builder clearAutoRenewPeriod() { copyOnWrite();
instance.clearAutoRenewPeriod();
return this;
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
@java.lang.Override
public java.util.List getTokenRelationshipsList() {
return java.util.Collections.unmodifiableList(
instance.getTokenRelationshipsList());
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
@java.lang.Override
public int getTokenRelationshipsCount() {
return instance.getTokenRelationshipsCount();
}/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.TokenRelationship getTokenRelationships(int index) {
return instance.getTokenRelationships(index);
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
public Builder setTokenRelationships(
int index, com.hedera.hashgraph.sdk.proto.TokenRelationship value) {
copyOnWrite();
instance.setTokenRelationships(index, value);
return this;
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
public Builder setTokenRelationships(
int index, com.hedera.hashgraph.sdk.proto.TokenRelationship.Builder builderForValue) {
copyOnWrite();
instance.setTokenRelationships(index,
builderForValue.build());
return this;
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
public Builder addTokenRelationships(com.hedera.hashgraph.sdk.proto.TokenRelationship value) {
copyOnWrite();
instance.addTokenRelationships(value);
return this;
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
public Builder addTokenRelationships(
int index, com.hedera.hashgraph.sdk.proto.TokenRelationship value) {
copyOnWrite();
instance.addTokenRelationships(index, value);
return this;
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
public Builder addTokenRelationships(
com.hedera.hashgraph.sdk.proto.TokenRelationship.Builder builderForValue) {
copyOnWrite();
instance.addTokenRelationships(builderForValue.build());
return this;
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
public Builder addTokenRelationships(
int index, com.hedera.hashgraph.sdk.proto.TokenRelationship.Builder builderForValue) {
copyOnWrite();
instance.addTokenRelationships(index,
builderForValue.build());
return this;
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
public Builder addAllTokenRelationships(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.TokenRelationship> values) {
copyOnWrite();
instance.addAllTokenRelationships(values);
return this;
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
public Builder clearTokenRelationships() {
copyOnWrite();
instance.clearTokenRelationships();
return this;
}
/**
*
**
* All tokens related to this account
*
*
* repeated .proto.TokenRelationship token_relationships = 11;
*/
public Builder removeTokenRelationships(int index) {
copyOnWrite();
instance.removeTokenRelationships(index);
return this;
}
/**
*
**
* The memo associated with the account
*
*
* string memo = 12;
* @return The memo.
*/
@java.lang.Override
public java.lang.String getMemo() {
return instance.getMemo();
}
/**
*
**
* The memo associated with the account
*
*
* string memo = 12;
* @return The bytes for memo.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMemoBytes() {
return instance.getMemoBytes();
}
/**
*
**
* The memo associated with the account
*
*
* string memo = 12;
* @param value The memo to set.
* @return This builder for chaining.
*/
public Builder setMemo(
java.lang.String value) {
copyOnWrite();
instance.setMemo(value);
return this;
}
/**
*
**
* The memo associated with the account
*
*
* string memo = 12;
* @return This builder for chaining.
*/
public Builder clearMemo() {
copyOnWrite();
instance.clearMemo();
return this;
}
/**
*
**
* The memo associated with the account
*
*
* string memo = 12;
* @param value The bytes for memo to set.
* @return This builder for chaining.
*/
public Builder setMemoBytes(
com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setMemoBytes(value);
return this;
}
/**
*
**
* The number of NFTs owned by this account
*
*
* int64 owned_nfts = 13;
* @return The ownedNfts.
*/
@java.lang.Override
public long getOwnedNfts() {
return instance.getOwnedNfts();
}
/**
*
**
* The number of NFTs owned by this account
*
*
* int64 owned_nfts = 13;
* @param value The ownedNfts to set.
* @return This builder for chaining.
*/
public Builder setOwnedNfts(long value) {
copyOnWrite();
instance.setOwnedNfts(value);
return this;
}
/**
*
**
* The number of NFTs owned by this account
*
*
* int64 owned_nfts = 13;
* @return This builder for chaining.
*/
public Builder clearOwnedNfts() {
copyOnWrite();
instance.clearOwnedNfts();
return this;
}
/**
*
**
* The maximum number of tokens that an Account can be implicitly associated with.
*
*
* int32 max_automatic_token_associations = 14;
* @return The maxAutomaticTokenAssociations.
*/
@java.lang.Override
public int getMaxAutomaticTokenAssociations() {
return instance.getMaxAutomaticTokenAssociations();
}
/**
*
**
* The maximum number of tokens that an Account can be implicitly associated with.
*
*
* int32 max_automatic_token_associations = 14;
* @param value The maxAutomaticTokenAssociations to set.
* @return This builder for chaining.
*/
public Builder setMaxAutomaticTokenAssociations(int value) {
copyOnWrite();
instance.setMaxAutomaticTokenAssociations(value);
return this;
}
/**
*
**
* The maximum number of tokens that an Account can be implicitly associated with.
*
*
* int32 max_automatic_token_associations = 14;
* @return This builder for chaining.
*/
public Builder clearMaxAutomaticTokenAssociations() {
copyOnWrite();
instance.clearMaxAutomaticTokenAssociations();
return this;
}
/**
*
**
* The alias of this account
*
*
* bytes alias = 15;
* @return The alias.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAlias() {
return instance.getAlias();
}
/**
*
**
* The alias of this account
*
*
* bytes alias = 15;
* @param value The alias to set.
* @return This builder for chaining.
*/
public Builder setAlias(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setAlias(value);
return this;
}
/**
*
**
* The alias of this account
*
*
* bytes alias = 15;
* @return This builder for chaining.
*/
public Builder clearAlias() {
copyOnWrite();
instance.clearAlias();
return this;
}
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 16;
* @return The ledgerId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLedgerId() {
return instance.getLedgerId();
}
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 16;
* @param value The ledgerId to set.
* @return This builder for chaining.
*/
public Builder setLedgerId(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setLedgerId(value);
return this;
}
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 16;
* @return This builder for chaining.
*/
public Builder clearLedgerId() {
copyOnWrite();
instance.clearLedgerId();
return this;
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
@java.lang.Override
public java.util.List getGrantedCryptoAllowancesList() {
return java.util.Collections.unmodifiableList(
instance.getGrantedCryptoAllowancesList());
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
@java.lang.Override
public int getGrantedCryptoAllowancesCount() {
return instance.getGrantedCryptoAllowancesCount();
}/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowance getGrantedCryptoAllowances(int index) {
return instance.getGrantedCryptoAllowances(index);
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
public Builder setGrantedCryptoAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowance value) {
copyOnWrite();
instance.setGrantedCryptoAllowances(index, value);
return this;
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
public Builder setGrantedCryptoAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowance.Builder builderForValue) {
copyOnWrite();
instance.setGrantedCryptoAllowances(index,
builderForValue.build());
return this;
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
public Builder addGrantedCryptoAllowances(com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowance value) {
copyOnWrite();
instance.addGrantedCryptoAllowances(value);
return this;
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
public Builder addGrantedCryptoAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowance value) {
copyOnWrite();
instance.addGrantedCryptoAllowances(index, value);
return this;
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
public Builder addGrantedCryptoAllowances(
com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowance.Builder builderForValue) {
copyOnWrite();
instance.addGrantedCryptoAllowances(builderForValue.build());
return this;
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
public Builder addGrantedCryptoAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowance.Builder builderForValue) {
copyOnWrite();
instance.addGrantedCryptoAllowances(index,
builderForValue.build());
return this;
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
public Builder addAllGrantedCryptoAllowances(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowance> values) {
copyOnWrite();
instance.addAllGrantedCryptoAllowances(values);
return this;
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
public Builder clearGrantedCryptoAllowances() {
copyOnWrite();
instance.clearGrantedCryptoAllowances();
return this;
}
/**
*
**
* All of the hbar allowances approved by the account owner.
*
*
* repeated .proto.GrantedCryptoAllowance granted_crypto_allowances = 17;
*/
public Builder removeGrantedCryptoAllowances(int index) {
copyOnWrite();
instance.removeGrantedCryptoAllowances(index);
return this;
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
@java.lang.Override
public java.util.List getGrantedNftAllowancesList() {
return java.util.Collections.unmodifiableList(
instance.getGrantedNftAllowancesList());
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
@java.lang.Override
public int getGrantedNftAllowancesCount() {
return instance.getGrantedNftAllowancesCount();
}/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.GrantedNftAllowance getGrantedNftAllowances(int index) {
return instance.getGrantedNftAllowances(index);
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
public Builder setGrantedNftAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedNftAllowance value) {
copyOnWrite();
instance.setGrantedNftAllowances(index, value);
return this;
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
public Builder setGrantedNftAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedNftAllowance.Builder builderForValue) {
copyOnWrite();
instance.setGrantedNftAllowances(index,
builderForValue.build());
return this;
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
public Builder addGrantedNftAllowances(com.hedera.hashgraph.sdk.proto.GrantedNftAllowance value) {
copyOnWrite();
instance.addGrantedNftAllowances(value);
return this;
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
public Builder addGrantedNftAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedNftAllowance value) {
copyOnWrite();
instance.addGrantedNftAllowances(index, value);
return this;
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
public Builder addGrantedNftAllowances(
com.hedera.hashgraph.sdk.proto.GrantedNftAllowance.Builder builderForValue) {
copyOnWrite();
instance.addGrantedNftAllowances(builderForValue.build());
return this;
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
public Builder addGrantedNftAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedNftAllowance.Builder builderForValue) {
copyOnWrite();
instance.addGrantedNftAllowances(index,
builderForValue.build());
return this;
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
public Builder addAllGrantedNftAllowances(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.GrantedNftAllowance> values) {
copyOnWrite();
instance.addAllGrantedNftAllowances(values);
return this;
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
public Builder clearGrantedNftAllowances() {
copyOnWrite();
instance.clearGrantedNftAllowances();
return this;
}
/**
*
**
* All of the non-fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedNftAllowance granted_nft_allowances = 18;
*/
public Builder removeGrantedNftAllowances(int index) {
copyOnWrite();
instance.removeGrantedNftAllowances(index);
return this;
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
@java.lang.Override
public java.util.List getGrantedTokenAllowancesList() {
return java.util.Collections.unmodifiableList(
instance.getGrantedTokenAllowancesList());
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
@java.lang.Override
public int getGrantedTokenAllowancesCount() {
return instance.getGrantedTokenAllowancesCount();
}/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.GrantedTokenAllowance getGrantedTokenAllowances(int index) {
return instance.getGrantedTokenAllowances(index);
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
public Builder setGrantedTokenAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedTokenAllowance value) {
copyOnWrite();
instance.setGrantedTokenAllowances(index, value);
return this;
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
public Builder setGrantedTokenAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedTokenAllowance.Builder builderForValue) {
copyOnWrite();
instance.setGrantedTokenAllowances(index,
builderForValue.build());
return this;
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
public Builder addGrantedTokenAllowances(com.hedera.hashgraph.sdk.proto.GrantedTokenAllowance value) {
copyOnWrite();
instance.addGrantedTokenAllowances(value);
return this;
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
public Builder addGrantedTokenAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedTokenAllowance value) {
copyOnWrite();
instance.addGrantedTokenAllowances(index, value);
return this;
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
public Builder addGrantedTokenAllowances(
com.hedera.hashgraph.sdk.proto.GrantedTokenAllowance.Builder builderForValue) {
copyOnWrite();
instance.addGrantedTokenAllowances(builderForValue.build());
return this;
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
public Builder addGrantedTokenAllowances(
int index, com.hedera.hashgraph.sdk.proto.GrantedTokenAllowance.Builder builderForValue) {
copyOnWrite();
instance.addGrantedTokenAllowances(index,
builderForValue.build());
return this;
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
public Builder addAllGrantedTokenAllowances(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.GrantedTokenAllowance> values) {
copyOnWrite();
instance.addAllGrantedTokenAllowances(values);
return this;
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
public Builder clearGrantedTokenAllowances() {
copyOnWrite();
instance.clearGrantedTokenAllowances();
return this;
}
/**
*
**
* All of the fungible token allowances approved by the account owner.
*
*
* repeated .proto.GrantedTokenAllowance granted_token_allowances = 19;
*/
public Builder removeGrantedTokenAllowances(int index) {
copyOnWrite();
instance.removeGrantedTokenAllowances(index);
return this;
}
// @@protoc_insertion_point(builder_scope:proto.GetAccountDetailsResponse.AccountDetails)
}
@java.lang.Override
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails();
}
case NEW_BUILDER: {
return new Builder();
}
case BUILD_MESSAGE_INFO: {
java.lang.Object[] objects = new java.lang.Object[] {
"accountId_",
"contractAccountId_",
"deleted_",
"proxyAccountId_",
"proxyReceived_",
"key_",
"balance_",
"receiverSigRequired_",
"expirationTime_",
"autoRenewPeriod_",
"tokenRelationships_",
com.hedera.hashgraph.sdk.proto.TokenRelationship.class,
"memo_",
"ownedNfts_",
"maxAutomaticTokenAssociations_",
"alias_",
"ledgerId_",
"grantedCryptoAllowances_",
com.hedera.hashgraph.sdk.proto.GrantedCryptoAllowance.class,
"grantedNftAllowances_",
com.hedera.hashgraph.sdk.proto.GrantedNftAllowance.class,
"grantedTokenAllowances_",
com.hedera.hashgraph.sdk.proto.GrantedTokenAllowance.class,
};
java.lang.String info =
"\u0000\u0013\u0000\u0000\u0001\u0013\u0013\u0000\u0004\u0000\u0001\t\u0002\u0208" +
"\u0003\u0007\u0004\t\u0005\u0002\u0006\t\u0007\u0003\b\u0007\t\t\n\t\u000b\u001b" +
"\f\u0208\r\u0002\u000e\u0004\u000f\n\u0010\n\u0011\u001b\u0012\u001b\u0013\u001b" +
"";
return newMessageInfo(DEFAULT_INSTANCE, info, objects);
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
com.google.protobuf.Parser parser = PARSER;
if (parser == null) {
synchronized (com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails.class) {
parser = PARSER;
if (parser == null) {
parser =
new DefaultInstanceBasedParser(
DEFAULT_INSTANCE);
PARSER = parser;
}
}
}
return parser;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:proto.GetAccountDetailsResponse.AccountDetails)
private static final com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails DEFAULT_INSTANCE;
static {
AccountDetails defaultInstance = new AccountDetails();
// New instances are implicitly immutable so no need to make
// immutable.
DEFAULT_INSTANCE = defaultInstance;
com.google.protobuf.GeneratedMessageLite.registerDefaultInstance(
AccountDetails.class, defaultInstance);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
public static final int HEADER_FIELD_NUMBER = 1;
private com.hedera.hashgraph.sdk.proto.ResponseHeader header_;
/**
*
**
* Standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
@java.lang.Override
public boolean hasHeader() {
return header_ != null;
}
/**
*
**
* Standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ResponseHeader getHeader() {
return header_ == null ? com.hedera.hashgraph.sdk.proto.ResponseHeader.getDefaultInstance() : header_;
}
/**
*
**
* Standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
private void setHeader(com.hedera.hashgraph.sdk.proto.ResponseHeader value) {
value.getClass();
header_ = value;
}
/**
*
**
* Standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeHeader(com.hedera.hashgraph.sdk.proto.ResponseHeader value) {
value.getClass();
if (header_ != null &&
header_ != com.hedera.hashgraph.sdk.proto.ResponseHeader.getDefaultInstance()) {
header_ =
com.hedera.hashgraph.sdk.proto.ResponseHeader.newBuilder(header_).mergeFrom(value).buildPartial();
} else {
header_ = value;
}
}
/**
*
**
* Standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
private void clearHeader() { header_ = null;
}
public static final int ACCOUNT_DETAILS_FIELD_NUMBER = 2;
private com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails accountDetails_;
/**
*
**
* Details of the account (a state proof can be generated for this)
*
*
* .proto.GetAccountDetailsResponse.AccountDetails account_details = 2;
*/
@java.lang.Override
public boolean hasAccountDetails() {
return accountDetails_ != null;
}
/**
*
**
* Details of the account (a state proof can be generated for this)
*
*
* .proto.GetAccountDetailsResponse.AccountDetails account_details = 2;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails getAccountDetails() {
return accountDetails_ == null ? com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails.getDefaultInstance() : accountDetails_;
}
/**
*
**
* Details of the account (a state proof can be generated for this)
*
*
* .proto.GetAccountDetailsResponse.AccountDetails account_details = 2;
*/
private void setAccountDetails(com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails value) {
value.getClass();
accountDetails_ = value;
}
/**
*
**
* Details of the account (a state proof can be generated for this)
*
*
* .proto.GetAccountDetailsResponse.AccountDetails account_details = 2;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeAccountDetails(com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails value) {
value.getClass();
if (accountDetails_ != null &&
accountDetails_ != com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails.getDefaultInstance()) {
accountDetails_ =
com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails.newBuilder(accountDetails_).mergeFrom(value).buildPartial();
} else {
accountDetails_ = value;
}
}
/**
*
**
* Details of the account (a state proof can be generated for this)
*
*
* .proto.GetAccountDetailsResponse.AccountDetails account_details = 2;
*/
private void clearAccountDetails() { accountDetails_ = null;
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
*
**
* Response when the client sends the node GetAccountDetailsQuery
*
*
* Protobuf type {@code proto.GetAccountDetailsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse, Builder> implements
// @@protoc_insertion_point(builder_implements:proto.GetAccountDetailsResponse)
com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponseOrBuilder {
// Construct using com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
*
**
* Standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
@java.lang.Override
public boolean hasHeader() {
return instance.hasHeader();
}
/**
*
**
* Standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ResponseHeader getHeader() {
return instance.getHeader();
}
/**
*
**
* Standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
public Builder setHeader(com.hedera.hashgraph.sdk.proto.ResponseHeader value) {
copyOnWrite();
instance.setHeader(value);
return this;
}
/**
*
**
* Standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
public Builder setHeader(
com.hedera.hashgraph.sdk.proto.ResponseHeader.Builder builderForValue) {
copyOnWrite();
instance.setHeader(builderForValue.build());
return this;
}
/**
*
**
* Standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
public Builder mergeHeader(com.hedera.hashgraph.sdk.proto.ResponseHeader value) {
copyOnWrite();
instance.mergeHeader(value);
return this;
}
/**
*
**
* Standard response from node to client, including the requested fields: cost, or state proof,
* or both, or neither
*
*
* .proto.ResponseHeader header = 1;
*/
public Builder clearHeader() { copyOnWrite();
instance.clearHeader();
return this;
}
/**
*
**
* Details of the account (a state proof can be generated for this)
*
*
* .proto.GetAccountDetailsResponse.AccountDetails account_details = 2;
*/
@java.lang.Override
public boolean hasAccountDetails() {
return instance.hasAccountDetails();
}
/**
*
**
* Details of the account (a state proof can be generated for this)
*
*
* .proto.GetAccountDetailsResponse.AccountDetails account_details = 2;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails getAccountDetails() {
return instance.getAccountDetails();
}
/**
*
**
* Details of the account (a state proof can be generated for this)
*
*
* .proto.GetAccountDetailsResponse.AccountDetails account_details = 2;
*/
public Builder setAccountDetails(com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails value) {
copyOnWrite();
instance.setAccountDetails(value);
return this;
}
/**
*
**
* Details of the account (a state proof can be generated for this)
*
*
* .proto.GetAccountDetailsResponse.AccountDetails account_details = 2;
*/
public Builder setAccountDetails(
com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails.Builder builderForValue) {
copyOnWrite();
instance.setAccountDetails(builderForValue.build());
return this;
}
/**
*
**
* Details of the account (a state proof can be generated for this)
*
*
* .proto.GetAccountDetailsResponse.AccountDetails account_details = 2;
*/
public Builder mergeAccountDetails(com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.AccountDetails value) {
copyOnWrite();
instance.mergeAccountDetails(value);
return this;
}
/**
*
**
* Details of the account (a state proof can be generated for this)
*
*
* .proto.GetAccountDetailsResponse.AccountDetails account_details = 2;
*/
public Builder clearAccountDetails() { copyOnWrite();
instance.clearAccountDetails();
return this;
}
// @@protoc_insertion_point(builder_scope:proto.GetAccountDetailsResponse)
}
@java.lang.Override
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse();
}
case NEW_BUILDER: {
return new Builder();
}
case BUILD_MESSAGE_INFO: {
java.lang.Object[] objects = new java.lang.Object[] {
"header_",
"accountDetails_",
};
java.lang.String info =
"\u0000\u0002\u0000\u0000\u0001\u0002\u0002\u0000\u0000\u0000\u0001\t\u0002\t";
return newMessageInfo(DEFAULT_INSTANCE, info, objects);
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
com.google.protobuf.Parser parser = PARSER;
if (parser == null) {
synchronized (com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse.class) {
parser = PARSER;
if (parser == null) {
parser =
new DefaultInstanceBasedParser(
DEFAULT_INSTANCE);
PARSER = parser;
}
}
}
return parser;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:proto.GetAccountDetailsResponse)
private static final com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse DEFAULT_INSTANCE;
static {
GetAccountDetailsResponse defaultInstance = new GetAccountDetailsResponse();
// New instances are implicitly immutable so no need to make
// immutable.
DEFAULT_INSTANCE = defaultInstance;
com.google.protobuf.GeneratedMessageLite.registerDefaultInstance(
GetAccountDetailsResponse.class, defaultInstance);
}
public static com.hedera.hashgraph.sdk.proto.GetAccountDetailsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy