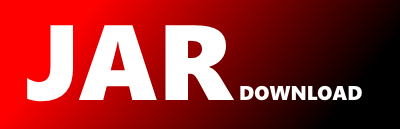
com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk-jdk7 Show documentation
Show all versions of sdk-jdk7 Show documentation
Hedera™ Hashgraph SDK for Java
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: node_stake_update.proto
package com.hedera.hashgraph.sdk.proto;
/**
*
**
* Updates the staking info at the end of staking period to indicate new staking period has started.
*
*
* Protobuf type {@code proto.NodeStakeUpdateTransactionBody}
*/
public final class NodeStakeUpdateTransactionBody extends
com.google.protobuf.GeneratedMessageLite<
NodeStakeUpdateTransactionBody, NodeStakeUpdateTransactionBody.Builder> implements
// @@protoc_insertion_point(message_implements:proto.NodeStakeUpdateTransactionBody)
NodeStakeUpdateTransactionBodyOrBuilder {
private NodeStakeUpdateTransactionBody() {
nodeStake_ = emptyProtobufList();
}
public static final int END_OF_STAKING_PERIOD_FIELD_NUMBER = 1;
private com.hedera.hashgraph.sdk.proto.Timestamp endOfStakingPeriod_;
/**
*
**
* Time and date of the end of the staking period that is ending
*
*
* .proto.Timestamp end_of_staking_period = 1;
*/
@java.lang.Override
public boolean hasEndOfStakingPeriod() {
return endOfStakingPeriod_ != null;
}
/**
*
**
* Time and date of the end of the staking period that is ending
*
*
* .proto.Timestamp end_of_staking_period = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Timestamp getEndOfStakingPeriod() {
return endOfStakingPeriod_ == null ? com.hedera.hashgraph.sdk.proto.Timestamp.getDefaultInstance() : endOfStakingPeriod_;
}
/**
*
**
* Time and date of the end of the staking period that is ending
*
*
* .proto.Timestamp end_of_staking_period = 1;
*/
private void setEndOfStakingPeriod(com.hedera.hashgraph.sdk.proto.Timestamp value) {
value.getClass();
endOfStakingPeriod_ = value;
}
/**
*
**
* Time and date of the end of the staking period that is ending
*
*
* .proto.Timestamp end_of_staking_period = 1;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeEndOfStakingPeriod(com.hedera.hashgraph.sdk.proto.Timestamp value) {
value.getClass();
if (endOfStakingPeriod_ != null &&
endOfStakingPeriod_ != com.hedera.hashgraph.sdk.proto.Timestamp.getDefaultInstance()) {
endOfStakingPeriod_ =
com.hedera.hashgraph.sdk.proto.Timestamp.newBuilder(endOfStakingPeriod_).mergeFrom(value).buildPartial();
} else {
endOfStakingPeriod_ = value;
}
}
/**
*
**
* Time and date of the end of the staking period that is ending
*
*
* .proto.Timestamp end_of_staking_period = 1;
*/
private void clearEndOfStakingPeriod() { endOfStakingPeriod_ = null;
}
public static final int NODE_STAKE_FIELD_NUMBER = 2;
private com.google.protobuf.Internal.ProtobufList nodeStake_;
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
@java.lang.Override
public java.util.List getNodeStakeList() {
return nodeStake_;
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
public java.util.List extends com.hedera.hashgraph.sdk.proto.NodeStakeOrBuilder>
getNodeStakeOrBuilderList() {
return nodeStake_;
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
@java.lang.Override
public int getNodeStakeCount() {
return nodeStake_.size();
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.NodeStake getNodeStake(int index) {
return nodeStake_.get(index);
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
public com.hedera.hashgraph.sdk.proto.NodeStakeOrBuilder getNodeStakeOrBuilder(
int index) {
return nodeStake_.get(index);
}
private void ensureNodeStakeIsMutable() {
com.google.protobuf.Internal.ProtobufList tmp = nodeStake_;
if (!tmp.isModifiable()) {
nodeStake_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(tmp);
}
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
private void setNodeStake(
int index, com.hedera.hashgraph.sdk.proto.NodeStake value) {
value.getClass();
ensureNodeStakeIsMutable();
nodeStake_.set(index, value);
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
private void addNodeStake(com.hedera.hashgraph.sdk.proto.NodeStake value) {
value.getClass();
ensureNodeStakeIsMutable();
nodeStake_.add(value);
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
private void addNodeStake(
int index, com.hedera.hashgraph.sdk.proto.NodeStake value) {
value.getClass();
ensureNodeStakeIsMutable();
nodeStake_.add(index, value);
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
private void addAllNodeStake(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.NodeStake> values) {
ensureNodeStakeIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, nodeStake_);
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
private void clearNodeStake() {
nodeStake_ = emptyProtobufList();
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
private void removeNodeStake(int index) {
ensureNodeStakeIsMutable();
nodeStake_.remove(index);
}
public static final int MAX_STAKING_REWARD_RATE_PER_HBAR_FIELD_NUMBER = 3;
private long maxStakingRewardRatePerHbar_;
/**
*
**
* The maximum reward rate, in tinybars per whole hbar, that any account can receive in a day.
*
*
* int64 max_staking_reward_rate_per_hbar = 3;
* @return The maxStakingRewardRatePerHbar.
*/
@java.lang.Override
public long getMaxStakingRewardRatePerHbar() {
return maxStakingRewardRatePerHbar_;
}
/**
*
**
* The maximum reward rate, in tinybars per whole hbar, that any account can receive in a day.
*
*
* int64 max_staking_reward_rate_per_hbar = 3;
* @param value The maxStakingRewardRatePerHbar to set.
*/
private void setMaxStakingRewardRatePerHbar(long value) {
maxStakingRewardRatePerHbar_ = value;
}
/**
*
**
* The maximum reward rate, in tinybars per whole hbar, that any account can receive in a day.
*
*
* int64 max_staking_reward_rate_per_hbar = 3;
*/
private void clearMaxStakingRewardRatePerHbar() {
maxStakingRewardRatePerHbar_ = 0L;
}
public static final int NODE_REWARD_FEE_FRACTION_FIELD_NUMBER = 4;
private com.hedera.hashgraph.sdk.proto.Fraction nodeRewardFeeFraction_;
/**
*
**
* The fraction of the network and service fees paid to the node reward account 0.0.801.
*
*
* .proto.Fraction node_reward_fee_fraction = 4;
*/
@java.lang.Override
public boolean hasNodeRewardFeeFraction() {
return nodeRewardFeeFraction_ != null;
}
/**
*
**
* The fraction of the network and service fees paid to the node reward account 0.0.801.
*
*
* .proto.Fraction node_reward_fee_fraction = 4;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Fraction getNodeRewardFeeFraction() {
return nodeRewardFeeFraction_ == null ? com.hedera.hashgraph.sdk.proto.Fraction.getDefaultInstance() : nodeRewardFeeFraction_;
}
/**
*
**
* The fraction of the network and service fees paid to the node reward account 0.0.801.
*
*
* .proto.Fraction node_reward_fee_fraction = 4;
*/
private void setNodeRewardFeeFraction(com.hedera.hashgraph.sdk.proto.Fraction value) {
value.getClass();
nodeRewardFeeFraction_ = value;
}
/**
*
**
* The fraction of the network and service fees paid to the node reward account 0.0.801.
*
*
* .proto.Fraction node_reward_fee_fraction = 4;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeNodeRewardFeeFraction(com.hedera.hashgraph.sdk.proto.Fraction value) {
value.getClass();
if (nodeRewardFeeFraction_ != null &&
nodeRewardFeeFraction_ != com.hedera.hashgraph.sdk.proto.Fraction.getDefaultInstance()) {
nodeRewardFeeFraction_ =
com.hedera.hashgraph.sdk.proto.Fraction.newBuilder(nodeRewardFeeFraction_).mergeFrom(value).buildPartial();
} else {
nodeRewardFeeFraction_ = value;
}
}
/**
*
**
* The fraction of the network and service fees paid to the node reward account 0.0.801.
*
*
* .proto.Fraction node_reward_fee_fraction = 4;
*/
private void clearNodeRewardFeeFraction() { nodeRewardFeeFraction_ = null;
}
public static final int STAKING_PERIODS_STORED_FIELD_NUMBER = 5;
private long stakingPeriodsStored_;
/**
*
**
* The maximum number of trailing periods for which a user can collect rewards. For example, if this
* is 365 with a UTC calendar day period, then users must collect rewards at least once per calendar
* year to avoid missing any value.
*
*
* int64 staking_periods_stored = 5;
* @return The stakingPeriodsStored.
*/
@java.lang.Override
public long getStakingPeriodsStored() {
return stakingPeriodsStored_;
}
/**
*
**
* The maximum number of trailing periods for which a user can collect rewards. For example, if this
* is 365 with a UTC calendar day period, then users must collect rewards at least once per calendar
* year to avoid missing any value.
*
*
* int64 staking_periods_stored = 5;
* @param value The stakingPeriodsStored to set.
*/
private void setStakingPeriodsStored(long value) {
stakingPeriodsStored_ = value;
}
/**
*
**
* The maximum number of trailing periods for which a user can collect rewards. For example, if this
* is 365 with a UTC calendar day period, then users must collect rewards at least once per calendar
* year to avoid missing any value.
*
*
* int64 staking_periods_stored = 5;
*/
private void clearStakingPeriodsStored() {
stakingPeriodsStored_ = 0L;
}
public static final int STAKING_PERIOD_FIELD_NUMBER = 6;
private long stakingPeriod_;
/**
*
**
* The number of minutes in a staking period. Note for the special case of 1440 minutes, periods are
* treated as UTC calendar days, rather than repeating 1440 minute periods left-aligned at the epoch.
*
*
* int64 staking_period = 6;
* @return The stakingPeriod.
*/
@java.lang.Override
public long getStakingPeriod() {
return stakingPeriod_;
}
/**
*
**
* The number of minutes in a staking period. Note for the special case of 1440 minutes, periods are
* treated as UTC calendar days, rather than repeating 1440 minute periods left-aligned at the epoch.
*
*
* int64 staking_period = 6;
* @param value The stakingPeriod to set.
*/
private void setStakingPeriod(long value) {
stakingPeriod_ = value;
}
/**
*
**
* The number of minutes in a staking period. Note for the special case of 1440 minutes, periods are
* treated as UTC calendar days, rather than repeating 1440 minute periods left-aligned at the epoch.
*
*
* int64 staking_period = 6;
*/
private void clearStakingPeriod() {
stakingPeriod_ = 0L;
}
public static final int STAKING_REWARD_FEE_FRACTION_FIELD_NUMBER = 7;
private com.hedera.hashgraph.sdk.proto.Fraction stakingRewardFeeFraction_;
/**
*
**
* The fraction of the network and service fees paid to the staking reward account 0.0.800.
*
*
* .proto.Fraction staking_reward_fee_fraction = 7;
*/
@java.lang.Override
public boolean hasStakingRewardFeeFraction() {
return stakingRewardFeeFraction_ != null;
}
/**
*
**
* The fraction of the network and service fees paid to the staking reward account 0.0.800.
*
*
* .proto.Fraction staking_reward_fee_fraction = 7;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Fraction getStakingRewardFeeFraction() {
return stakingRewardFeeFraction_ == null ? com.hedera.hashgraph.sdk.proto.Fraction.getDefaultInstance() : stakingRewardFeeFraction_;
}
/**
*
**
* The fraction of the network and service fees paid to the staking reward account 0.0.800.
*
*
* .proto.Fraction staking_reward_fee_fraction = 7;
*/
private void setStakingRewardFeeFraction(com.hedera.hashgraph.sdk.proto.Fraction value) {
value.getClass();
stakingRewardFeeFraction_ = value;
}
/**
*
**
* The fraction of the network and service fees paid to the staking reward account 0.0.800.
*
*
* .proto.Fraction staking_reward_fee_fraction = 7;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeStakingRewardFeeFraction(com.hedera.hashgraph.sdk.proto.Fraction value) {
value.getClass();
if (stakingRewardFeeFraction_ != null &&
stakingRewardFeeFraction_ != com.hedera.hashgraph.sdk.proto.Fraction.getDefaultInstance()) {
stakingRewardFeeFraction_ =
com.hedera.hashgraph.sdk.proto.Fraction.newBuilder(stakingRewardFeeFraction_).mergeFrom(value).buildPartial();
} else {
stakingRewardFeeFraction_ = value;
}
}
/**
*
**
* The fraction of the network and service fees paid to the staking reward account 0.0.800.
*
*
* .proto.Fraction staking_reward_fee_fraction = 7;
*/
private void clearStakingRewardFeeFraction() { stakingRewardFeeFraction_ = null;
}
public static final int STAKING_START_THRESHOLD_FIELD_NUMBER = 8;
private long stakingStartThreshold_;
/**
*
**
* The minimum balance of staking reward account 0.0.800 required to active rewards.
*
*
* int64 staking_start_threshold = 8;
* @return The stakingStartThreshold.
*/
@java.lang.Override
public long getStakingStartThreshold() {
return stakingStartThreshold_;
}
/**
*
**
* The minimum balance of staking reward account 0.0.800 required to active rewards.
*
*
* int64 staking_start_threshold = 8;
* @param value The stakingStartThreshold to set.
*/
private void setStakingStartThreshold(long value) {
stakingStartThreshold_ = value;
}
/**
*
**
* The minimum balance of staking reward account 0.0.800 required to active rewards.
*
*
* int64 staking_start_threshold = 8;
*/
private void clearStakingStartThreshold() {
stakingStartThreshold_ = 0L;
}
public static final int STAKING_REWARD_RATE_FIELD_NUMBER = 9;
private long stakingRewardRate_;
/**
*
**
* The total number of tinybars to be distributed as staking rewards each period.
*
*
* int64 staking_reward_rate = 9;
* @return The stakingRewardRate.
*/
@java.lang.Override
public long getStakingRewardRate() {
return stakingRewardRate_;
}
/**
*
**
* The total number of tinybars to be distributed as staking rewards each period.
*
*
* int64 staking_reward_rate = 9;
* @param value The stakingRewardRate to set.
*/
private void setStakingRewardRate(long value) {
stakingRewardRate_ = value;
}
/**
*
**
* The total number of tinybars to be distributed as staking rewards each period.
*
*
* int64 staking_reward_rate = 9;
*/
private void clearStakingRewardRate() {
stakingRewardRate_ = 0L;
}
public static com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
*
**
* Updates the staking info at the end of staking period to indicate new staking period has started.
*
*
* Protobuf type {@code proto.NodeStakeUpdateTransactionBody}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody, Builder> implements
// @@protoc_insertion_point(builder_implements:proto.NodeStakeUpdateTransactionBody)
com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBodyOrBuilder {
// Construct using com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
*
**
* Time and date of the end of the staking period that is ending
*
*
* .proto.Timestamp end_of_staking_period = 1;
*/
@java.lang.Override
public boolean hasEndOfStakingPeriod() {
return instance.hasEndOfStakingPeriod();
}
/**
*
**
* Time and date of the end of the staking period that is ending
*
*
* .proto.Timestamp end_of_staking_period = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Timestamp getEndOfStakingPeriod() {
return instance.getEndOfStakingPeriod();
}
/**
*
**
* Time and date of the end of the staking period that is ending
*
*
* .proto.Timestamp end_of_staking_period = 1;
*/
public Builder setEndOfStakingPeriod(com.hedera.hashgraph.sdk.proto.Timestamp value) {
copyOnWrite();
instance.setEndOfStakingPeriod(value);
return this;
}
/**
*
**
* Time and date of the end of the staking period that is ending
*
*
* .proto.Timestamp end_of_staking_period = 1;
*/
public Builder setEndOfStakingPeriod(
com.hedera.hashgraph.sdk.proto.Timestamp.Builder builderForValue) {
copyOnWrite();
instance.setEndOfStakingPeriod(builderForValue.build());
return this;
}
/**
*
**
* Time and date of the end of the staking period that is ending
*
*
* .proto.Timestamp end_of_staking_period = 1;
*/
public Builder mergeEndOfStakingPeriod(com.hedera.hashgraph.sdk.proto.Timestamp value) {
copyOnWrite();
instance.mergeEndOfStakingPeriod(value);
return this;
}
/**
*
**
* Time and date of the end of the staking period that is ending
*
*
* .proto.Timestamp end_of_staking_period = 1;
*/
public Builder clearEndOfStakingPeriod() { copyOnWrite();
instance.clearEndOfStakingPeriod();
return this;
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
@java.lang.Override
public java.util.List getNodeStakeList() {
return java.util.Collections.unmodifiableList(
instance.getNodeStakeList());
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
@java.lang.Override
public int getNodeStakeCount() {
return instance.getNodeStakeCount();
}/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.NodeStake getNodeStake(int index) {
return instance.getNodeStake(index);
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
public Builder setNodeStake(
int index, com.hedera.hashgraph.sdk.proto.NodeStake value) {
copyOnWrite();
instance.setNodeStake(index, value);
return this;
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
public Builder setNodeStake(
int index, com.hedera.hashgraph.sdk.proto.NodeStake.Builder builderForValue) {
copyOnWrite();
instance.setNodeStake(index,
builderForValue.build());
return this;
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
public Builder addNodeStake(com.hedera.hashgraph.sdk.proto.NodeStake value) {
copyOnWrite();
instance.addNodeStake(value);
return this;
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
public Builder addNodeStake(
int index, com.hedera.hashgraph.sdk.proto.NodeStake value) {
copyOnWrite();
instance.addNodeStake(index, value);
return this;
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
public Builder addNodeStake(
com.hedera.hashgraph.sdk.proto.NodeStake.Builder builderForValue) {
copyOnWrite();
instance.addNodeStake(builderForValue.build());
return this;
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
public Builder addNodeStake(
int index, com.hedera.hashgraph.sdk.proto.NodeStake.Builder builderForValue) {
copyOnWrite();
instance.addNodeStake(index,
builderForValue.build());
return this;
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
public Builder addAllNodeStake(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.NodeStake> values) {
copyOnWrite();
instance.addAllNodeStake(values);
return this;
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
public Builder clearNodeStake() {
copyOnWrite();
instance.clearNodeStake();
return this;
}
/**
*
**
* Staking info of each node at the beginning of the new staking period
*
*
* repeated .proto.NodeStake node_stake = 2;
*/
public Builder removeNodeStake(int index) {
copyOnWrite();
instance.removeNodeStake(index);
return this;
}
/**
*
**
* The maximum reward rate, in tinybars per whole hbar, that any account can receive in a day.
*
*
* int64 max_staking_reward_rate_per_hbar = 3;
* @return The maxStakingRewardRatePerHbar.
*/
@java.lang.Override
public long getMaxStakingRewardRatePerHbar() {
return instance.getMaxStakingRewardRatePerHbar();
}
/**
*
**
* The maximum reward rate, in tinybars per whole hbar, that any account can receive in a day.
*
*
* int64 max_staking_reward_rate_per_hbar = 3;
* @param value The maxStakingRewardRatePerHbar to set.
* @return This builder for chaining.
*/
public Builder setMaxStakingRewardRatePerHbar(long value) {
copyOnWrite();
instance.setMaxStakingRewardRatePerHbar(value);
return this;
}
/**
*
**
* The maximum reward rate, in tinybars per whole hbar, that any account can receive in a day.
*
*
* int64 max_staking_reward_rate_per_hbar = 3;
* @return This builder for chaining.
*/
public Builder clearMaxStakingRewardRatePerHbar() {
copyOnWrite();
instance.clearMaxStakingRewardRatePerHbar();
return this;
}
/**
*
**
* The fraction of the network and service fees paid to the node reward account 0.0.801.
*
*
* .proto.Fraction node_reward_fee_fraction = 4;
*/
@java.lang.Override
public boolean hasNodeRewardFeeFraction() {
return instance.hasNodeRewardFeeFraction();
}
/**
*
**
* The fraction of the network and service fees paid to the node reward account 0.0.801.
*
*
* .proto.Fraction node_reward_fee_fraction = 4;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Fraction getNodeRewardFeeFraction() {
return instance.getNodeRewardFeeFraction();
}
/**
*
**
* The fraction of the network and service fees paid to the node reward account 0.0.801.
*
*
* .proto.Fraction node_reward_fee_fraction = 4;
*/
public Builder setNodeRewardFeeFraction(com.hedera.hashgraph.sdk.proto.Fraction value) {
copyOnWrite();
instance.setNodeRewardFeeFraction(value);
return this;
}
/**
*
**
* The fraction of the network and service fees paid to the node reward account 0.0.801.
*
*
* .proto.Fraction node_reward_fee_fraction = 4;
*/
public Builder setNodeRewardFeeFraction(
com.hedera.hashgraph.sdk.proto.Fraction.Builder builderForValue) {
copyOnWrite();
instance.setNodeRewardFeeFraction(builderForValue.build());
return this;
}
/**
*
**
* The fraction of the network and service fees paid to the node reward account 0.0.801.
*
*
* .proto.Fraction node_reward_fee_fraction = 4;
*/
public Builder mergeNodeRewardFeeFraction(com.hedera.hashgraph.sdk.proto.Fraction value) {
copyOnWrite();
instance.mergeNodeRewardFeeFraction(value);
return this;
}
/**
*
**
* The fraction of the network and service fees paid to the node reward account 0.0.801.
*
*
* .proto.Fraction node_reward_fee_fraction = 4;
*/
public Builder clearNodeRewardFeeFraction() { copyOnWrite();
instance.clearNodeRewardFeeFraction();
return this;
}
/**
*
**
* The maximum number of trailing periods for which a user can collect rewards. For example, if this
* is 365 with a UTC calendar day period, then users must collect rewards at least once per calendar
* year to avoid missing any value.
*
*
* int64 staking_periods_stored = 5;
* @return The stakingPeriodsStored.
*/
@java.lang.Override
public long getStakingPeriodsStored() {
return instance.getStakingPeriodsStored();
}
/**
*
**
* The maximum number of trailing periods for which a user can collect rewards. For example, if this
* is 365 with a UTC calendar day period, then users must collect rewards at least once per calendar
* year to avoid missing any value.
*
*
* int64 staking_periods_stored = 5;
* @param value The stakingPeriodsStored to set.
* @return This builder for chaining.
*/
public Builder setStakingPeriodsStored(long value) {
copyOnWrite();
instance.setStakingPeriodsStored(value);
return this;
}
/**
*
**
* The maximum number of trailing periods for which a user can collect rewards. For example, if this
* is 365 with a UTC calendar day period, then users must collect rewards at least once per calendar
* year to avoid missing any value.
*
*
* int64 staking_periods_stored = 5;
* @return This builder for chaining.
*/
public Builder clearStakingPeriodsStored() {
copyOnWrite();
instance.clearStakingPeriodsStored();
return this;
}
/**
*
**
* The number of minutes in a staking period. Note for the special case of 1440 minutes, periods are
* treated as UTC calendar days, rather than repeating 1440 minute periods left-aligned at the epoch.
*
*
* int64 staking_period = 6;
* @return The stakingPeriod.
*/
@java.lang.Override
public long getStakingPeriod() {
return instance.getStakingPeriod();
}
/**
*
**
* The number of minutes in a staking period. Note for the special case of 1440 minutes, periods are
* treated as UTC calendar days, rather than repeating 1440 minute periods left-aligned at the epoch.
*
*
* int64 staking_period = 6;
* @param value The stakingPeriod to set.
* @return This builder for chaining.
*/
public Builder setStakingPeriod(long value) {
copyOnWrite();
instance.setStakingPeriod(value);
return this;
}
/**
*
**
* The number of minutes in a staking period. Note for the special case of 1440 minutes, periods are
* treated as UTC calendar days, rather than repeating 1440 minute periods left-aligned at the epoch.
*
*
* int64 staking_period = 6;
* @return This builder for chaining.
*/
public Builder clearStakingPeriod() {
copyOnWrite();
instance.clearStakingPeriod();
return this;
}
/**
*
**
* The fraction of the network and service fees paid to the staking reward account 0.0.800.
*
*
* .proto.Fraction staking_reward_fee_fraction = 7;
*/
@java.lang.Override
public boolean hasStakingRewardFeeFraction() {
return instance.hasStakingRewardFeeFraction();
}
/**
*
**
* The fraction of the network and service fees paid to the staking reward account 0.0.800.
*
*
* .proto.Fraction staking_reward_fee_fraction = 7;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Fraction getStakingRewardFeeFraction() {
return instance.getStakingRewardFeeFraction();
}
/**
*
**
* The fraction of the network and service fees paid to the staking reward account 0.0.800.
*
*
* .proto.Fraction staking_reward_fee_fraction = 7;
*/
public Builder setStakingRewardFeeFraction(com.hedera.hashgraph.sdk.proto.Fraction value) {
copyOnWrite();
instance.setStakingRewardFeeFraction(value);
return this;
}
/**
*
**
* The fraction of the network and service fees paid to the staking reward account 0.0.800.
*
*
* .proto.Fraction staking_reward_fee_fraction = 7;
*/
public Builder setStakingRewardFeeFraction(
com.hedera.hashgraph.sdk.proto.Fraction.Builder builderForValue) {
copyOnWrite();
instance.setStakingRewardFeeFraction(builderForValue.build());
return this;
}
/**
*
**
* The fraction of the network and service fees paid to the staking reward account 0.0.800.
*
*
* .proto.Fraction staking_reward_fee_fraction = 7;
*/
public Builder mergeStakingRewardFeeFraction(com.hedera.hashgraph.sdk.proto.Fraction value) {
copyOnWrite();
instance.mergeStakingRewardFeeFraction(value);
return this;
}
/**
*
**
* The fraction of the network and service fees paid to the staking reward account 0.0.800.
*
*
* .proto.Fraction staking_reward_fee_fraction = 7;
*/
public Builder clearStakingRewardFeeFraction() { copyOnWrite();
instance.clearStakingRewardFeeFraction();
return this;
}
/**
*
**
* The minimum balance of staking reward account 0.0.800 required to active rewards.
*
*
* int64 staking_start_threshold = 8;
* @return The stakingStartThreshold.
*/
@java.lang.Override
public long getStakingStartThreshold() {
return instance.getStakingStartThreshold();
}
/**
*
**
* The minimum balance of staking reward account 0.0.800 required to active rewards.
*
*
* int64 staking_start_threshold = 8;
* @param value The stakingStartThreshold to set.
* @return This builder for chaining.
*/
public Builder setStakingStartThreshold(long value) {
copyOnWrite();
instance.setStakingStartThreshold(value);
return this;
}
/**
*
**
* The minimum balance of staking reward account 0.0.800 required to active rewards.
*
*
* int64 staking_start_threshold = 8;
* @return This builder for chaining.
*/
public Builder clearStakingStartThreshold() {
copyOnWrite();
instance.clearStakingStartThreshold();
return this;
}
/**
*
**
* The total number of tinybars to be distributed as staking rewards each period.
*
*
* int64 staking_reward_rate = 9;
* @return The stakingRewardRate.
*/
@java.lang.Override
public long getStakingRewardRate() {
return instance.getStakingRewardRate();
}
/**
*
**
* The total number of tinybars to be distributed as staking rewards each period.
*
*
* int64 staking_reward_rate = 9;
* @param value The stakingRewardRate to set.
* @return This builder for chaining.
*/
public Builder setStakingRewardRate(long value) {
copyOnWrite();
instance.setStakingRewardRate(value);
return this;
}
/**
*
**
* The total number of tinybars to be distributed as staking rewards each period.
*
*
* int64 staking_reward_rate = 9;
* @return This builder for chaining.
*/
public Builder clearStakingRewardRate() {
copyOnWrite();
instance.clearStakingRewardRate();
return this;
}
// @@protoc_insertion_point(builder_scope:proto.NodeStakeUpdateTransactionBody)
}
@java.lang.Override
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody();
}
case NEW_BUILDER: {
return new Builder();
}
case BUILD_MESSAGE_INFO: {
java.lang.Object[] objects = new java.lang.Object[] {
"endOfStakingPeriod_",
"nodeStake_",
com.hedera.hashgraph.sdk.proto.NodeStake.class,
"maxStakingRewardRatePerHbar_",
"nodeRewardFeeFraction_",
"stakingPeriodsStored_",
"stakingPeriod_",
"stakingRewardFeeFraction_",
"stakingStartThreshold_",
"stakingRewardRate_",
};
java.lang.String info =
"\u0000\t\u0000\u0000\u0001\t\t\u0000\u0001\u0000\u0001\t\u0002\u001b\u0003\u0002" +
"\u0004\t\u0005\u0002\u0006\u0002\u0007\t\b\u0002\t\u0002";
return newMessageInfo(DEFAULT_INSTANCE, info, objects);
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
com.google.protobuf.Parser parser = PARSER;
if (parser == null) {
synchronized (com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody.class) {
parser = PARSER;
if (parser == null) {
parser =
new DefaultInstanceBasedParser(
DEFAULT_INSTANCE);
PARSER = parser;
}
}
}
return parser;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:proto.NodeStakeUpdateTransactionBody)
private static final com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody DEFAULT_INSTANCE;
static {
NodeStakeUpdateTransactionBody defaultInstance = new NodeStakeUpdateTransactionBody();
// New instances are implicitly immutable so no need to make
// immutable.
DEFAULT_INSTANCE = defaultInstance;
com.google.protobuf.GeneratedMessageLite.registerDefaultInstance(
NodeStakeUpdateTransactionBody.class, defaultInstance);
}
public static com.hedera.hashgraph.sdk.proto.NodeStakeUpdateTransactionBody getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy