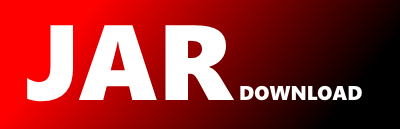
com.hedera.hashgraph.sdk.proto.ScheduleInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk-jdk7 Show documentation
Show all versions of sdk-jdk7 Show documentation
Hedera™ Hashgraph SDK for Java
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: schedule_get_info.proto
package com.hedera.hashgraph.sdk.proto;
/**
*
**
* Information summarizing schedule state
*
*
* Protobuf type {@code proto.ScheduleInfo}
*/
public final class ScheduleInfo extends
com.google.protobuf.GeneratedMessageLite<
ScheduleInfo, ScheduleInfo.Builder> implements
// @@protoc_insertion_point(message_implements:proto.ScheduleInfo)
ScheduleInfoOrBuilder {
private ScheduleInfo() {
memo_ = "";
ledgerId_ = com.google.protobuf.ByteString.EMPTY;
}
private int dataCase_ = 0;
private java.lang.Object data_;
public enum DataCase {
DELETION_TIME(2),
EXECUTION_TIME(3),
DATA_NOT_SET(0);
private final int value;
private DataCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DataCase valueOf(int value) {
return forNumber(value);
}
public static DataCase forNumber(int value) {
switch (value) {
case 2: return DELETION_TIME;
case 3: return EXECUTION_TIME;
case 0: return DATA_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
@java.lang.Override
public DataCase
getDataCase() {
return DataCase.forNumber(
dataCase_);
}
private void clearData() {
dataCase_ = 0;
data_ = null;
}
public static final int SCHEDULEID_FIELD_NUMBER = 1;
private com.hedera.hashgraph.sdk.proto.ScheduleID scheduleID_;
/**
*
**
* The id of the schedule
*
*
* .proto.ScheduleID scheduleID = 1;
*/
@java.lang.Override
public boolean hasScheduleID() {
return scheduleID_ != null;
}
/**
*
**
* The id of the schedule
*
*
* .proto.ScheduleID scheduleID = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ScheduleID getScheduleID() {
return scheduleID_ == null ? com.hedera.hashgraph.sdk.proto.ScheduleID.getDefaultInstance() : scheduleID_;
}
/**
*
**
* The id of the schedule
*
*
* .proto.ScheduleID scheduleID = 1;
*/
private void setScheduleID(com.hedera.hashgraph.sdk.proto.ScheduleID value) {
value.getClass();
scheduleID_ = value;
}
/**
*
**
* The id of the schedule
*
*
* .proto.ScheduleID scheduleID = 1;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeScheduleID(com.hedera.hashgraph.sdk.proto.ScheduleID value) {
value.getClass();
if (scheduleID_ != null &&
scheduleID_ != com.hedera.hashgraph.sdk.proto.ScheduleID.getDefaultInstance()) {
scheduleID_ =
com.hedera.hashgraph.sdk.proto.ScheduleID.newBuilder(scheduleID_).mergeFrom(value).buildPartial();
} else {
scheduleID_ = value;
}
}
/**
*
**
* The id of the schedule
*
*
* .proto.ScheduleID scheduleID = 1;
*/
private void clearScheduleID() { scheduleID_ = null;
}
public static final int DELETION_TIME_FIELD_NUMBER = 2;
/**
*
**
* If the schedule has been deleted, the consensus time when this occurred
*
*
* .proto.Timestamp deletion_time = 2;
*/
@java.lang.Override
public boolean hasDeletionTime() {
return dataCase_ == 2;
}
/**
*
**
* If the schedule has been deleted, the consensus time when this occurred
*
*
* .proto.Timestamp deletion_time = 2;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Timestamp getDeletionTime() {
if (dataCase_ == 2) {
return (com.hedera.hashgraph.sdk.proto.Timestamp) data_;
}
return com.hedera.hashgraph.sdk.proto.Timestamp.getDefaultInstance();
}
/**
*
**
* If the schedule has been deleted, the consensus time when this occurred
*
*
* .proto.Timestamp deletion_time = 2;
*/
private void setDeletionTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
value.getClass();
data_ = value;
dataCase_ = 2;
}
/**
*
**
* If the schedule has been deleted, the consensus time when this occurred
*
*
* .proto.Timestamp deletion_time = 2;
*/
private void mergeDeletionTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
value.getClass();
if (dataCase_ == 2 &&
data_ != com.hedera.hashgraph.sdk.proto.Timestamp.getDefaultInstance()) {
data_ = com.hedera.hashgraph.sdk.proto.Timestamp.newBuilder((com.hedera.hashgraph.sdk.proto.Timestamp) data_)
.mergeFrom(value).buildPartial();
} else {
data_ = value;
}
dataCase_ = 2;
}
/**
*
**
* If the schedule has been deleted, the consensus time when this occurred
*
*
* .proto.Timestamp deletion_time = 2;
*/
private void clearDeletionTime() {
if (dataCase_ == 2) {
dataCase_ = 0;
data_ = null;
}
}
public static final int EXECUTION_TIME_FIELD_NUMBER = 3;
/**
*
**
* If the schedule has been executed, the consensus time when this occurred
*
*
* .proto.Timestamp execution_time = 3;
*/
@java.lang.Override
public boolean hasExecutionTime() {
return dataCase_ == 3;
}
/**
*
**
* If the schedule has been executed, the consensus time when this occurred
*
*
* .proto.Timestamp execution_time = 3;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Timestamp getExecutionTime() {
if (dataCase_ == 3) {
return (com.hedera.hashgraph.sdk.proto.Timestamp) data_;
}
return com.hedera.hashgraph.sdk.proto.Timestamp.getDefaultInstance();
}
/**
*
**
* If the schedule has been executed, the consensus time when this occurred
*
*
* .proto.Timestamp execution_time = 3;
*/
private void setExecutionTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
value.getClass();
data_ = value;
dataCase_ = 3;
}
/**
*
**
* If the schedule has been executed, the consensus time when this occurred
*
*
* .proto.Timestamp execution_time = 3;
*/
private void mergeExecutionTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
value.getClass();
if (dataCase_ == 3 &&
data_ != com.hedera.hashgraph.sdk.proto.Timestamp.getDefaultInstance()) {
data_ = com.hedera.hashgraph.sdk.proto.Timestamp.newBuilder((com.hedera.hashgraph.sdk.proto.Timestamp) data_)
.mergeFrom(value).buildPartial();
} else {
data_ = value;
}
dataCase_ = 3;
}
/**
*
**
* If the schedule has been executed, the consensus time when this occurred
*
*
* .proto.Timestamp execution_time = 3;
*/
private void clearExecutionTime() {
if (dataCase_ == 3) {
dataCase_ = 0;
data_ = null;
}
}
public static final int EXPIRATIONTIME_FIELD_NUMBER = 4;
private com.hedera.hashgraph.sdk.proto.Timestamp expirationTime_;
/**
*
**
* The time at which the schedule will be evaluated for execution and then expire.
*
* Note: Before Long Term Scheduled Transactions are enabled, Scheduled Transactions will _never_ execute at expiration - they
* will _only_ execute during the initial ScheduleCreate transaction or via ScheduleSign transactions and will _always_
* expire at expirationTime.
*
*
* .proto.Timestamp expirationTime = 4;
*/
@java.lang.Override
public boolean hasExpirationTime() {
return expirationTime_ != null;
}
/**
*
**
* The time at which the schedule will be evaluated for execution and then expire.
*
* Note: Before Long Term Scheduled Transactions are enabled, Scheduled Transactions will _never_ execute at expiration - they
* will _only_ execute during the initial ScheduleCreate transaction or via ScheduleSign transactions and will _always_
* expire at expirationTime.
*
*
* .proto.Timestamp expirationTime = 4;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Timestamp getExpirationTime() {
return expirationTime_ == null ? com.hedera.hashgraph.sdk.proto.Timestamp.getDefaultInstance() : expirationTime_;
}
/**
*
**
* The time at which the schedule will be evaluated for execution and then expire.
*
* Note: Before Long Term Scheduled Transactions are enabled, Scheduled Transactions will _never_ execute at expiration - they
* will _only_ execute during the initial ScheduleCreate transaction or via ScheduleSign transactions and will _always_
* expire at expirationTime.
*
*
* .proto.Timestamp expirationTime = 4;
*/
private void setExpirationTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
value.getClass();
expirationTime_ = value;
}
/**
*
**
* The time at which the schedule will be evaluated for execution and then expire.
*
* Note: Before Long Term Scheduled Transactions are enabled, Scheduled Transactions will _never_ execute at expiration - they
* will _only_ execute during the initial ScheduleCreate transaction or via ScheduleSign transactions and will _always_
* expire at expirationTime.
*
*
* .proto.Timestamp expirationTime = 4;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeExpirationTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
value.getClass();
if (expirationTime_ != null &&
expirationTime_ != com.hedera.hashgraph.sdk.proto.Timestamp.getDefaultInstance()) {
expirationTime_ =
com.hedera.hashgraph.sdk.proto.Timestamp.newBuilder(expirationTime_).mergeFrom(value).buildPartial();
} else {
expirationTime_ = value;
}
}
/**
*
**
* The time at which the schedule will be evaluated for execution and then expire.
*
* Note: Before Long Term Scheduled Transactions are enabled, Scheduled Transactions will _never_ execute at expiration - they
* will _only_ execute during the initial ScheduleCreate transaction or via ScheduleSign transactions and will _always_
* expire at expirationTime.
*
*
* .proto.Timestamp expirationTime = 4;
*/
private void clearExpirationTime() { expirationTime_ = null;
}
public static final int SCHEDULEDTRANSACTIONBODY_FIELD_NUMBER = 5;
private com.hedera.hashgraph.sdk.proto.SchedulableTransactionBody scheduledTransactionBody_;
/**
*
**
* The scheduled transaction
*
*
* .proto.SchedulableTransactionBody scheduledTransactionBody = 5;
*/
@java.lang.Override
public boolean hasScheduledTransactionBody() {
return scheduledTransactionBody_ != null;
}
/**
*
**
* The scheduled transaction
*
*
* .proto.SchedulableTransactionBody scheduledTransactionBody = 5;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.SchedulableTransactionBody getScheduledTransactionBody() {
return scheduledTransactionBody_ == null ? com.hedera.hashgraph.sdk.proto.SchedulableTransactionBody.getDefaultInstance() : scheduledTransactionBody_;
}
/**
*
**
* The scheduled transaction
*
*
* .proto.SchedulableTransactionBody scheduledTransactionBody = 5;
*/
private void setScheduledTransactionBody(com.hedera.hashgraph.sdk.proto.SchedulableTransactionBody value) {
value.getClass();
scheduledTransactionBody_ = value;
}
/**
*
**
* The scheduled transaction
*
*
* .proto.SchedulableTransactionBody scheduledTransactionBody = 5;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeScheduledTransactionBody(com.hedera.hashgraph.sdk.proto.SchedulableTransactionBody value) {
value.getClass();
if (scheduledTransactionBody_ != null &&
scheduledTransactionBody_ != com.hedera.hashgraph.sdk.proto.SchedulableTransactionBody.getDefaultInstance()) {
scheduledTransactionBody_ =
com.hedera.hashgraph.sdk.proto.SchedulableTransactionBody.newBuilder(scheduledTransactionBody_).mergeFrom(value).buildPartial();
} else {
scheduledTransactionBody_ = value;
}
}
/**
*
**
* The scheduled transaction
*
*
* .proto.SchedulableTransactionBody scheduledTransactionBody = 5;
*/
private void clearScheduledTransactionBody() { scheduledTransactionBody_ = null;
}
public static final int MEMO_FIELD_NUMBER = 6;
private java.lang.String memo_;
/**
*
**
* The publicly visible memo of the schedule
*
*
* string memo = 6;
* @return The memo.
*/
@java.lang.Override
public java.lang.String getMemo() {
return memo_;
}
/**
*
**
* The publicly visible memo of the schedule
*
*
* string memo = 6;
* @return The bytes for memo.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMemoBytes() {
return com.google.protobuf.ByteString.copyFromUtf8(memo_);
}
/**
*
**
* The publicly visible memo of the schedule
*
*
* string memo = 6;
* @param value The memo to set.
*/
private void setMemo(
java.lang.String value) {
java.lang.Class> valueClass = value.getClass();
memo_ = value;
}
/**
*
**
* The publicly visible memo of the schedule
*
*
* string memo = 6;
*/
private void clearMemo() {
memo_ = getDefaultInstance().getMemo();
}
/**
*
**
* The publicly visible memo of the schedule
*
*
* string memo = 6;
* @param value The bytes for memo to set.
*/
private void setMemoBytes(
com.google.protobuf.ByteString value) {
checkByteStringIsUtf8(value);
memo_ = value.toStringUtf8();
}
public static final int ADMINKEY_FIELD_NUMBER = 7;
private com.hedera.hashgraph.sdk.proto.Key adminKey_;
/**
*
**
* The key used to delete the schedule from state
*
*
* .proto.Key adminKey = 7;
*/
@java.lang.Override
public boolean hasAdminKey() {
return adminKey_ != null;
}
/**
*
**
* The key used to delete the schedule from state
*
*
* .proto.Key adminKey = 7;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Key getAdminKey() {
return adminKey_ == null ? com.hedera.hashgraph.sdk.proto.Key.getDefaultInstance() : adminKey_;
}
/**
*
**
* The key used to delete the schedule from state
*
*
* .proto.Key adminKey = 7;
*/
private void setAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
value.getClass();
adminKey_ = value;
}
/**
*
**
* The key used to delete the schedule from state
*
*
* .proto.Key adminKey = 7;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
value.getClass();
if (adminKey_ != null &&
adminKey_ != com.hedera.hashgraph.sdk.proto.Key.getDefaultInstance()) {
adminKey_ =
com.hedera.hashgraph.sdk.proto.Key.newBuilder(adminKey_).mergeFrom(value).buildPartial();
} else {
adminKey_ = value;
}
}
/**
*
**
* The key used to delete the schedule from state
*
*
* .proto.Key adminKey = 7;
*/
private void clearAdminKey() { adminKey_ = null;
}
public static final int SIGNERS_FIELD_NUMBER = 8;
private com.hedera.hashgraph.sdk.proto.KeyList signers_;
/**
*
**
* The Ed25519 keys the network deems to have signed the scheduled transaction
*
*
* .proto.KeyList signers = 8;
*/
@java.lang.Override
public boolean hasSigners() {
return signers_ != null;
}
/**
*
**
* The Ed25519 keys the network deems to have signed the scheduled transaction
*
*
* .proto.KeyList signers = 8;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.KeyList getSigners() {
return signers_ == null ? com.hedera.hashgraph.sdk.proto.KeyList.getDefaultInstance() : signers_;
}
/**
*
**
* The Ed25519 keys the network deems to have signed the scheduled transaction
*
*
* .proto.KeyList signers = 8;
*/
private void setSigners(com.hedera.hashgraph.sdk.proto.KeyList value) {
value.getClass();
signers_ = value;
}
/**
*
**
* The Ed25519 keys the network deems to have signed the scheduled transaction
*
*
* .proto.KeyList signers = 8;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeSigners(com.hedera.hashgraph.sdk.proto.KeyList value) {
value.getClass();
if (signers_ != null &&
signers_ != com.hedera.hashgraph.sdk.proto.KeyList.getDefaultInstance()) {
signers_ =
com.hedera.hashgraph.sdk.proto.KeyList.newBuilder(signers_).mergeFrom(value).buildPartial();
} else {
signers_ = value;
}
}
/**
*
**
* The Ed25519 keys the network deems to have signed the scheduled transaction
*
*
* .proto.KeyList signers = 8;
*/
private void clearSigners() { signers_ = null;
}
public static final int CREATORACCOUNTID_FIELD_NUMBER = 9;
private com.hedera.hashgraph.sdk.proto.AccountID creatorAccountID_;
/**
*
**
* The id of the account that created the schedule
*
*
* .proto.AccountID creatorAccountID = 9;
*/
@java.lang.Override
public boolean hasCreatorAccountID() {
return creatorAccountID_ != null;
}
/**
*
**
* The id of the account that created the schedule
*
*
* .proto.AccountID creatorAccountID = 9;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getCreatorAccountID() {
return creatorAccountID_ == null ? com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance() : creatorAccountID_;
}
/**
*
**
* The id of the account that created the schedule
*
*
* .proto.AccountID creatorAccountID = 9;
*/
private void setCreatorAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
creatorAccountID_ = value;
}
/**
*
**
* The id of the account that created the schedule
*
*
* .proto.AccountID creatorAccountID = 9;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeCreatorAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
if (creatorAccountID_ != null &&
creatorAccountID_ != com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance()) {
creatorAccountID_ =
com.hedera.hashgraph.sdk.proto.AccountID.newBuilder(creatorAccountID_).mergeFrom(value).buildPartial();
} else {
creatorAccountID_ = value;
}
}
/**
*
**
* The id of the account that created the schedule
*
*
* .proto.AccountID creatorAccountID = 9;
*/
private void clearCreatorAccountID() { creatorAccountID_ = null;
}
public static final int PAYERACCOUNTID_FIELD_NUMBER = 10;
private com.hedera.hashgraph.sdk.proto.AccountID payerAccountID_;
/**
*
**
* The id of the account responsible for the service fee of the scheduled transaction
*
*
* .proto.AccountID payerAccountID = 10;
*/
@java.lang.Override
public boolean hasPayerAccountID() {
return payerAccountID_ != null;
}
/**
*
**
* The id of the account responsible for the service fee of the scheduled transaction
*
*
* .proto.AccountID payerAccountID = 10;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getPayerAccountID() {
return payerAccountID_ == null ? com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance() : payerAccountID_;
}
/**
*
**
* The id of the account responsible for the service fee of the scheduled transaction
*
*
* .proto.AccountID payerAccountID = 10;
*/
private void setPayerAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
payerAccountID_ = value;
}
/**
*
**
* The id of the account responsible for the service fee of the scheduled transaction
*
*
* .proto.AccountID payerAccountID = 10;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergePayerAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
value.getClass();
if (payerAccountID_ != null &&
payerAccountID_ != com.hedera.hashgraph.sdk.proto.AccountID.getDefaultInstance()) {
payerAccountID_ =
com.hedera.hashgraph.sdk.proto.AccountID.newBuilder(payerAccountID_).mergeFrom(value).buildPartial();
} else {
payerAccountID_ = value;
}
}
/**
*
**
* The id of the account responsible for the service fee of the scheduled transaction
*
*
* .proto.AccountID payerAccountID = 10;
*/
private void clearPayerAccountID() { payerAccountID_ = null;
}
public static final int SCHEDULEDTRANSACTIONID_FIELD_NUMBER = 11;
private com.hedera.hashgraph.sdk.proto.TransactionID scheduledTransactionID_;
/**
*
**
* The transaction id that will be used in the record of the scheduled transaction (if it
* executes)
*
*
* .proto.TransactionID scheduledTransactionID = 11;
*/
@java.lang.Override
public boolean hasScheduledTransactionID() {
return scheduledTransactionID_ != null;
}
/**
*
**
* The transaction id that will be used in the record of the scheduled transaction (if it
* executes)
*
*
* .proto.TransactionID scheduledTransactionID = 11;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.TransactionID getScheduledTransactionID() {
return scheduledTransactionID_ == null ? com.hedera.hashgraph.sdk.proto.TransactionID.getDefaultInstance() : scheduledTransactionID_;
}
/**
*
**
* The transaction id that will be used in the record of the scheduled transaction (if it
* executes)
*
*
* .proto.TransactionID scheduledTransactionID = 11;
*/
private void setScheduledTransactionID(com.hedera.hashgraph.sdk.proto.TransactionID value) {
value.getClass();
scheduledTransactionID_ = value;
}
/**
*
**
* The transaction id that will be used in the record of the scheduled transaction (if it
* executes)
*
*
* .proto.TransactionID scheduledTransactionID = 11;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeScheduledTransactionID(com.hedera.hashgraph.sdk.proto.TransactionID value) {
value.getClass();
if (scheduledTransactionID_ != null &&
scheduledTransactionID_ != com.hedera.hashgraph.sdk.proto.TransactionID.getDefaultInstance()) {
scheduledTransactionID_ =
com.hedera.hashgraph.sdk.proto.TransactionID.newBuilder(scheduledTransactionID_).mergeFrom(value).buildPartial();
} else {
scheduledTransactionID_ = value;
}
}
/**
*
**
* The transaction id that will be used in the record of the scheduled transaction (if it
* executes)
*
*
* .proto.TransactionID scheduledTransactionID = 11;
*/
private void clearScheduledTransactionID() { scheduledTransactionID_ = null;
}
public static final int LEDGER_ID_FIELD_NUMBER = 12;
private com.google.protobuf.ByteString ledgerId_;
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 12;
* @return The ledgerId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLedgerId() {
return ledgerId_;
}
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 12;
* @param value The ledgerId to set.
*/
private void setLedgerId(com.google.protobuf.ByteString value) {
java.lang.Class> valueClass = value.getClass();
ledgerId_ = value;
}
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 12;
*/
private void clearLedgerId() {
ledgerId_ = getDefaultInstance().getLedgerId();
}
public static final int WAIT_FOR_EXPIRY_FIELD_NUMBER = 13;
private boolean waitForExpiry_;
/**
*
**
* When set to true, the transaction will be evaluated for execution at expiration_time instead
* of when all required signatures are received.
* When set to false, the transaction will execute immediately after sufficient signatures are received
* to sign the contained transaction. During the initial ScheduleCreate transaction or via ScheduleSign transactions.
*
* Note: this field is unused until Long Term Scheduled Transactions are enabled.
*
*
* bool wait_for_expiry = 13;
* @return The waitForExpiry.
*/
@java.lang.Override
public boolean getWaitForExpiry() {
return waitForExpiry_;
}
/**
*
**
* When set to true, the transaction will be evaluated for execution at expiration_time instead
* of when all required signatures are received.
* When set to false, the transaction will execute immediately after sufficient signatures are received
* to sign the contained transaction. During the initial ScheduleCreate transaction or via ScheduleSign transactions.
*
* Note: this field is unused until Long Term Scheduled Transactions are enabled.
*
*
* bool wait_for_expiry = 13;
* @param value The waitForExpiry to set.
*/
private void setWaitForExpiry(boolean value) {
waitForExpiry_ = value;
}
/**
*
**
* When set to true, the transaction will be evaluated for execution at expiration_time instead
* of when all required signatures are received.
* When set to false, the transaction will execute immediately after sufficient signatures are received
* to sign the contained transaction. During the initial ScheduleCreate transaction or via ScheduleSign transactions.
*
* Note: this field is unused until Long Term Scheduled Transactions are enabled.
*
*
* bool wait_for_expiry = 13;
*/
private void clearWaitForExpiry() {
waitForExpiry_ = false;
}
public static com.hedera.hashgraph.sdk.proto.ScheduleInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ScheduleInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ScheduleInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ScheduleInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ScheduleInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.ScheduleInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ScheduleInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ScheduleInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ScheduleInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ScheduleInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.ScheduleInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.ScheduleInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(com.hedera.hashgraph.sdk.proto.ScheduleInfo prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
*
**
* Information summarizing schedule state
*
*
* Protobuf type {@code proto.ScheduleInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
com.hedera.hashgraph.sdk.proto.ScheduleInfo, Builder> implements
// @@protoc_insertion_point(builder_implements:proto.ScheduleInfo)
com.hedera.hashgraph.sdk.proto.ScheduleInfoOrBuilder {
// Construct using com.hedera.hashgraph.sdk.proto.ScheduleInfo.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
@java.lang.Override
public DataCase
getDataCase() {
return instance.getDataCase();
}
public Builder clearData() {
copyOnWrite();
instance.clearData();
return this;
}
/**
*
**
* The id of the schedule
*
*
* .proto.ScheduleID scheduleID = 1;
*/
@java.lang.Override
public boolean hasScheduleID() {
return instance.hasScheduleID();
}
/**
*
**
* The id of the schedule
*
*
* .proto.ScheduleID scheduleID = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.ScheduleID getScheduleID() {
return instance.getScheduleID();
}
/**
*
**
* The id of the schedule
*
*
* .proto.ScheduleID scheduleID = 1;
*/
public Builder setScheduleID(com.hedera.hashgraph.sdk.proto.ScheduleID value) {
copyOnWrite();
instance.setScheduleID(value);
return this;
}
/**
*
**
* The id of the schedule
*
*
* .proto.ScheduleID scheduleID = 1;
*/
public Builder setScheduleID(
com.hedera.hashgraph.sdk.proto.ScheduleID.Builder builderForValue) {
copyOnWrite();
instance.setScheduleID(builderForValue.build());
return this;
}
/**
*
**
* The id of the schedule
*
*
* .proto.ScheduleID scheduleID = 1;
*/
public Builder mergeScheduleID(com.hedera.hashgraph.sdk.proto.ScheduleID value) {
copyOnWrite();
instance.mergeScheduleID(value);
return this;
}
/**
*
**
* The id of the schedule
*
*
* .proto.ScheduleID scheduleID = 1;
*/
public Builder clearScheduleID() { copyOnWrite();
instance.clearScheduleID();
return this;
}
/**
*
**
* If the schedule has been deleted, the consensus time when this occurred
*
*
* .proto.Timestamp deletion_time = 2;
*/
@java.lang.Override
public boolean hasDeletionTime() {
return instance.hasDeletionTime();
}
/**
*
**
* If the schedule has been deleted, the consensus time when this occurred
*
*
* .proto.Timestamp deletion_time = 2;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Timestamp getDeletionTime() {
return instance.getDeletionTime();
}
/**
*
**
* If the schedule has been deleted, the consensus time when this occurred
*
*
* .proto.Timestamp deletion_time = 2;
*/
public Builder setDeletionTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
copyOnWrite();
instance.setDeletionTime(value);
return this;
}
/**
*
**
* If the schedule has been deleted, the consensus time when this occurred
*
*
* .proto.Timestamp deletion_time = 2;
*/
public Builder setDeletionTime(
com.hedera.hashgraph.sdk.proto.Timestamp.Builder builderForValue) {
copyOnWrite();
instance.setDeletionTime(builderForValue.build());
return this;
}
/**
*
**
* If the schedule has been deleted, the consensus time when this occurred
*
*
* .proto.Timestamp deletion_time = 2;
*/
public Builder mergeDeletionTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
copyOnWrite();
instance.mergeDeletionTime(value);
return this;
}
/**
*
**
* If the schedule has been deleted, the consensus time when this occurred
*
*
* .proto.Timestamp deletion_time = 2;
*/
public Builder clearDeletionTime() {
copyOnWrite();
instance.clearDeletionTime();
return this;
}
/**
*
**
* If the schedule has been executed, the consensus time when this occurred
*
*
* .proto.Timestamp execution_time = 3;
*/
@java.lang.Override
public boolean hasExecutionTime() {
return instance.hasExecutionTime();
}
/**
*
**
* If the schedule has been executed, the consensus time when this occurred
*
*
* .proto.Timestamp execution_time = 3;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Timestamp getExecutionTime() {
return instance.getExecutionTime();
}
/**
*
**
* If the schedule has been executed, the consensus time when this occurred
*
*
* .proto.Timestamp execution_time = 3;
*/
public Builder setExecutionTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
copyOnWrite();
instance.setExecutionTime(value);
return this;
}
/**
*
**
* If the schedule has been executed, the consensus time when this occurred
*
*
* .proto.Timestamp execution_time = 3;
*/
public Builder setExecutionTime(
com.hedera.hashgraph.sdk.proto.Timestamp.Builder builderForValue) {
copyOnWrite();
instance.setExecutionTime(builderForValue.build());
return this;
}
/**
*
**
* If the schedule has been executed, the consensus time when this occurred
*
*
* .proto.Timestamp execution_time = 3;
*/
public Builder mergeExecutionTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
copyOnWrite();
instance.mergeExecutionTime(value);
return this;
}
/**
*
**
* If the schedule has been executed, the consensus time when this occurred
*
*
* .proto.Timestamp execution_time = 3;
*/
public Builder clearExecutionTime() {
copyOnWrite();
instance.clearExecutionTime();
return this;
}
/**
*
**
* The time at which the schedule will be evaluated for execution and then expire.
*
* Note: Before Long Term Scheduled Transactions are enabled, Scheduled Transactions will _never_ execute at expiration - they
* will _only_ execute during the initial ScheduleCreate transaction or via ScheduleSign transactions and will _always_
* expire at expirationTime.
*
*
* .proto.Timestamp expirationTime = 4;
*/
@java.lang.Override
public boolean hasExpirationTime() {
return instance.hasExpirationTime();
}
/**
*
**
* The time at which the schedule will be evaluated for execution and then expire.
*
* Note: Before Long Term Scheduled Transactions are enabled, Scheduled Transactions will _never_ execute at expiration - they
* will _only_ execute during the initial ScheduleCreate transaction or via ScheduleSign transactions and will _always_
* expire at expirationTime.
*
*
* .proto.Timestamp expirationTime = 4;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Timestamp getExpirationTime() {
return instance.getExpirationTime();
}
/**
*
**
* The time at which the schedule will be evaluated for execution and then expire.
*
* Note: Before Long Term Scheduled Transactions are enabled, Scheduled Transactions will _never_ execute at expiration - they
* will _only_ execute during the initial ScheduleCreate transaction or via ScheduleSign transactions and will _always_
* expire at expirationTime.
*
*
* .proto.Timestamp expirationTime = 4;
*/
public Builder setExpirationTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
copyOnWrite();
instance.setExpirationTime(value);
return this;
}
/**
*
**
* The time at which the schedule will be evaluated for execution and then expire.
*
* Note: Before Long Term Scheduled Transactions are enabled, Scheduled Transactions will _never_ execute at expiration - they
* will _only_ execute during the initial ScheduleCreate transaction or via ScheduleSign transactions and will _always_
* expire at expirationTime.
*
*
* .proto.Timestamp expirationTime = 4;
*/
public Builder setExpirationTime(
com.hedera.hashgraph.sdk.proto.Timestamp.Builder builderForValue) {
copyOnWrite();
instance.setExpirationTime(builderForValue.build());
return this;
}
/**
*
**
* The time at which the schedule will be evaluated for execution and then expire.
*
* Note: Before Long Term Scheduled Transactions are enabled, Scheduled Transactions will _never_ execute at expiration - they
* will _only_ execute during the initial ScheduleCreate transaction or via ScheduleSign transactions and will _always_
* expire at expirationTime.
*
*
* .proto.Timestamp expirationTime = 4;
*/
public Builder mergeExpirationTime(com.hedera.hashgraph.sdk.proto.Timestamp value) {
copyOnWrite();
instance.mergeExpirationTime(value);
return this;
}
/**
*
**
* The time at which the schedule will be evaluated for execution and then expire.
*
* Note: Before Long Term Scheduled Transactions are enabled, Scheduled Transactions will _never_ execute at expiration - they
* will _only_ execute during the initial ScheduleCreate transaction or via ScheduleSign transactions and will _always_
* expire at expirationTime.
*
*
* .proto.Timestamp expirationTime = 4;
*/
public Builder clearExpirationTime() { copyOnWrite();
instance.clearExpirationTime();
return this;
}
/**
*
**
* The scheduled transaction
*
*
* .proto.SchedulableTransactionBody scheduledTransactionBody = 5;
*/
@java.lang.Override
public boolean hasScheduledTransactionBody() {
return instance.hasScheduledTransactionBody();
}
/**
*
**
* The scheduled transaction
*
*
* .proto.SchedulableTransactionBody scheduledTransactionBody = 5;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.SchedulableTransactionBody getScheduledTransactionBody() {
return instance.getScheduledTransactionBody();
}
/**
*
**
* The scheduled transaction
*
*
* .proto.SchedulableTransactionBody scheduledTransactionBody = 5;
*/
public Builder setScheduledTransactionBody(com.hedera.hashgraph.sdk.proto.SchedulableTransactionBody value) {
copyOnWrite();
instance.setScheduledTransactionBody(value);
return this;
}
/**
*
**
* The scheduled transaction
*
*
* .proto.SchedulableTransactionBody scheduledTransactionBody = 5;
*/
public Builder setScheduledTransactionBody(
com.hedera.hashgraph.sdk.proto.SchedulableTransactionBody.Builder builderForValue) {
copyOnWrite();
instance.setScheduledTransactionBody(builderForValue.build());
return this;
}
/**
*
**
* The scheduled transaction
*
*
* .proto.SchedulableTransactionBody scheduledTransactionBody = 5;
*/
public Builder mergeScheduledTransactionBody(com.hedera.hashgraph.sdk.proto.SchedulableTransactionBody value) {
copyOnWrite();
instance.mergeScheduledTransactionBody(value);
return this;
}
/**
*
**
* The scheduled transaction
*
*
* .proto.SchedulableTransactionBody scheduledTransactionBody = 5;
*/
public Builder clearScheduledTransactionBody() { copyOnWrite();
instance.clearScheduledTransactionBody();
return this;
}
/**
*
**
* The publicly visible memo of the schedule
*
*
* string memo = 6;
* @return The memo.
*/
@java.lang.Override
public java.lang.String getMemo() {
return instance.getMemo();
}
/**
*
**
* The publicly visible memo of the schedule
*
*
* string memo = 6;
* @return The bytes for memo.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMemoBytes() {
return instance.getMemoBytes();
}
/**
*
**
* The publicly visible memo of the schedule
*
*
* string memo = 6;
* @param value The memo to set.
* @return This builder for chaining.
*/
public Builder setMemo(
java.lang.String value) {
copyOnWrite();
instance.setMemo(value);
return this;
}
/**
*
**
* The publicly visible memo of the schedule
*
*
* string memo = 6;
* @return This builder for chaining.
*/
public Builder clearMemo() {
copyOnWrite();
instance.clearMemo();
return this;
}
/**
*
**
* The publicly visible memo of the schedule
*
*
* string memo = 6;
* @param value The bytes for memo to set.
* @return This builder for chaining.
*/
public Builder setMemoBytes(
com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setMemoBytes(value);
return this;
}
/**
*
**
* The key used to delete the schedule from state
*
*
* .proto.Key adminKey = 7;
*/
@java.lang.Override
public boolean hasAdminKey() {
return instance.hasAdminKey();
}
/**
*
**
* The key used to delete the schedule from state
*
*
* .proto.Key adminKey = 7;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.Key getAdminKey() {
return instance.getAdminKey();
}
/**
*
**
* The key used to delete the schedule from state
*
*
* .proto.Key adminKey = 7;
*/
public Builder setAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
copyOnWrite();
instance.setAdminKey(value);
return this;
}
/**
*
**
* The key used to delete the schedule from state
*
*
* .proto.Key adminKey = 7;
*/
public Builder setAdminKey(
com.hedera.hashgraph.sdk.proto.Key.Builder builderForValue) {
copyOnWrite();
instance.setAdminKey(builderForValue.build());
return this;
}
/**
*
**
* The key used to delete the schedule from state
*
*
* .proto.Key adminKey = 7;
*/
public Builder mergeAdminKey(com.hedera.hashgraph.sdk.proto.Key value) {
copyOnWrite();
instance.mergeAdminKey(value);
return this;
}
/**
*
**
* The key used to delete the schedule from state
*
*
* .proto.Key adminKey = 7;
*/
public Builder clearAdminKey() { copyOnWrite();
instance.clearAdminKey();
return this;
}
/**
*
**
* The Ed25519 keys the network deems to have signed the scheduled transaction
*
*
* .proto.KeyList signers = 8;
*/
@java.lang.Override
public boolean hasSigners() {
return instance.hasSigners();
}
/**
*
**
* The Ed25519 keys the network deems to have signed the scheduled transaction
*
*
* .proto.KeyList signers = 8;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.KeyList getSigners() {
return instance.getSigners();
}
/**
*
**
* The Ed25519 keys the network deems to have signed the scheduled transaction
*
*
* .proto.KeyList signers = 8;
*/
public Builder setSigners(com.hedera.hashgraph.sdk.proto.KeyList value) {
copyOnWrite();
instance.setSigners(value);
return this;
}
/**
*
**
* The Ed25519 keys the network deems to have signed the scheduled transaction
*
*
* .proto.KeyList signers = 8;
*/
public Builder setSigners(
com.hedera.hashgraph.sdk.proto.KeyList.Builder builderForValue) {
copyOnWrite();
instance.setSigners(builderForValue.build());
return this;
}
/**
*
**
* The Ed25519 keys the network deems to have signed the scheduled transaction
*
*
* .proto.KeyList signers = 8;
*/
public Builder mergeSigners(com.hedera.hashgraph.sdk.proto.KeyList value) {
copyOnWrite();
instance.mergeSigners(value);
return this;
}
/**
*
**
* The Ed25519 keys the network deems to have signed the scheduled transaction
*
*
* .proto.KeyList signers = 8;
*/
public Builder clearSigners() { copyOnWrite();
instance.clearSigners();
return this;
}
/**
*
**
* The id of the account that created the schedule
*
*
* .proto.AccountID creatorAccountID = 9;
*/
@java.lang.Override
public boolean hasCreatorAccountID() {
return instance.hasCreatorAccountID();
}
/**
*
**
* The id of the account that created the schedule
*
*
* .proto.AccountID creatorAccountID = 9;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getCreatorAccountID() {
return instance.getCreatorAccountID();
}
/**
*
**
* The id of the account that created the schedule
*
*
* .proto.AccountID creatorAccountID = 9;
*/
public Builder setCreatorAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.setCreatorAccountID(value);
return this;
}
/**
*
**
* The id of the account that created the schedule
*
*
* .proto.AccountID creatorAccountID = 9;
*/
public Builder setCreatorAccountID(
com.hedera.hashgraph.sdk.proto.AccountID.Builder builderForValue) {
copyOnWrite();
instance.setCreatorAccountID(builderForValue.build());
return this;
}
/**
*
**
* The id of the account that created the schedule
*
*
* .proto.AccountID creatorAccountID = 9;
*/
public Builder mergeCreatorAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.mergeCreatorAccountID(value);
return this;
}
/**
*
**
* The id of the account that created the schedule
*
*
* .proto.AccountID creatorAccountID = 9;
*/
public Builder clearCreatorAccountID() { copyOnWrite();
instance.clearCreatorAccountID();
return this;
}
/**
*
**
* The id of the account responsible for the service fee of the scheduled transaction
*
*
* .proto.AccountID payerAccountID = 10;
*/
@java.lang.Override
public boolean hasPayerAccountID() {
return instance.hasPayerAccountID();
}
/**
*
**
* The id of the account responsible for the service fee of the scheduled transaction
*
*
* .proto.AccountID payerAccountID = 10;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountID getPayerAccountID() {
return instance.getPayerAccountID();
}
/**
*
**
* The id of the account responsible for the service fee of the scheduled transaction
*
*
* .proto.AccountID payerAccountID = 10;
*/
public Builder setPayerAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.setPayerAccountID(value);
return this;
}
/**
*
**
* The id of the account responsible for the service fee of the scheduled transaction
*
*
* .proto.AccountID payerAccountID = 10;
*/
public Builder setPayerAccountID(
com.hedera.hashgraph.sdk.proto.AccountID.Builder builderForValue) {
copyOnWrite();
instance.setPayerAccountID(builderForValue.build());
return this;
}
/**
*
**
* The id of the account responsible for the service fee of the scheduled transaction
*
*
* .proto.AccountID payerAccountID = 10;
*/
public Builder mergePayerAccountID(com.hedera.hashgraph.sdk.proto.AccountID value) {
copyOnWrite();
instance.mergePayerAccountID(value);
return this;
}
/**
*
**
* The id of the account responsible for the service fee of the scheduled transaction
*
*
* .proto.AccountID payerAccountID = 10;
*/
public Builder clearPayerAccountID() { copyOnWrite();
instance.clearPayerAccountID();
return this;
}
/**
*
**
* The transaction id that will be used in the record of the scheduled transaction (if it
* executes)
*
*
* .proto.TransactionID scheduledTransactionID = 11;
*/
@java.lang.Override
public boolean hasScheduledTransactionID() {
return instance.hasScheduledTransactionID();
}
/**
*
**
* The transaction id that will be used in the record of the scheduled transaction (if it
* executes)
*
*
* .proto.TransactionID scheduledTransactionID = 11;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.TransactionID getScheduledTransactionID() {
return instance.getScheduledTransactionID();
}
/**
*
**
* The transaction id that will be used in the record of the scheduled transaction (if it
* executes)
*
*
* .proto.TransactionID scheduledTransactionID = 11;
*/
public Builder setScheduledTransactionID(com.hedera.hashgraph.sdk.proto.TransactionID value) {
copyOnWrite();
instance.setScheduledTransactionID(value);
return this;
}
/**
*
**
* The transaction id that will be used in the record of the scheduled transaction (if it
* executes)
*
*
* .proto.TransactionID scheduledTransactionID = 11;
*/
public Builder setScheduledTransactionID(
com.hedera.hashgraph.sdk.proto.TransactionID.Builder builderForValue) {
copyOnWrite();
instance.setScheduledTransactionID(builderForValue.build());
return this;
}
/**
*
**
* The transaction id that will be used in the record of the scheduled transaction (if it
* executes)
*
*
* .proto.TransactionID scheduledTransactionID = 11;
*/
public Builder mergeScheduledTransactionID(com.hedera.hashgraph.sdk.proto.TransactionID value) {
copyOnWrite();
instance.mergeScheduledTransactionID(value);
return this;
}
/**
*
**
* The transaction id that will be used in the record of the scheduled transaction (if it
* executes)
*
*
* .proto.TransactionID scheduledTransactionID = 11;
*/
public Builder clearScheduledTransactionID() { copyOnWrite();
instance.clearScheduledTransactionID();
return this;
}
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 12;
* @return The ledgerId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLedgerId() {
return instance.getLedgerId();
}
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 12;
* @param value The ledgerId to set.
* @return This builder for chaining.
*/
public Builder setLedgerId(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setLedgerId(value);
return this;
}
/**
*
**
* The ledger ID the response was returned from; please see <a href="https://github.com/hashgraph/hedera-improvement-proposal/blob/master/HIP/hip-198.md">HIP-198</a> for the network-specific IDs.
*
*
* bytes ledger_id = 12;
* @return This builder for chaining.
*/
public Builder clearLedgerId() {
copyOnWrite();
instance.clearLedgerId();
return this;
}
/**
*
**
* When set to true, the transaction will be evaluated for execution at expiration_time instead
* of when all required signatures are received.
* When set to false, the transaction will execute immediately after sufficient signatures are received
* to sign the contained transaction. During the initial ScheduleCreate transaction or via ScheduleSign transactions.
*
* Note: this field is unused until Long Term Scheduled Transactions are enabled.
*
*
* bool wait_for_expiry = 13;
* @return The waitForExpiry.
*/
@java.lang.Override
public boolean getWaitForExpiry() {
return instance.getWaitForExpiry();
}
/**
*
**
* When set to true, the transaction will be evaluated for execution at expiration_time instead
* of when all required signatures are received.
* When set to false, the transaction will execute immediately after sufficient signatures are received
* to sign the contained transaction. During the initial ScheduleCreate transaction or via ScheduleSign transactions.
*
* Note: this field is unused until Long Term Scheduled Transactions are enabled.
*
*
* bool wait_for_expiry = 13;
* @param value The waitForExpiry to set.
* @return This builder for chaining.
*/
public Builder setWaitForExpiry(boolean value) {
copyOnWrite();
instance.setWaitForExpiry(value);
return this;
}
/**
*
**
* When set to true, the transaction will be evaluated for execution at expiration_time instead
* of when all required signatures are received.
* When set to false, the transaction will execute immediately after sufficient signatures are received
* to sign the contained transaction. During the initial ScheduleCreate transaction or via ScheduleSign transactions.
*
* Note: this field is unused until Long Term Scheduled Transactions are enabled.
*
*
* bool wait_for_expiry = 13;
* @return This builder for chaining.
*/
public Builder clearWaitForExpiry() {
copyOnWrite();
instance.clearWaitForExpiry();
return this;
}
// @@protoc_insertion_point(builder_scope:proto.ScheduleInfo)
}
@java.lang.Override
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new com.hedera.hashgraph.sdk.proto.ScheduleInfo();
}
case NEW_BUILDER: {
return new Builder();
}
case BUILD_MESSAGE_INFO: {
java.lang.Object[] objects = new java.lang.Object[] {
"data_",
"dataCase_",
"scheduleID_",
com.hedera.hashgraph.sdk.proto.Timestamp.class,
com.hedera.hashgraph.sdk.proto.Timestamp.class,
"expirationTime_",
"scheduledTransactionBody_",
"memo_",
"adminKey_",
"signers_",
"creatorAccountID_",
"payerAccountID_",
"scheduledTransactionID_",
"ledgerId_",
"waitForExpiry_",
};
java.lang.String info =
"\u0000\r\u0001\u0000\u0001\r\r\u0000\u0000\u0000\u0001\t\u0002<\u0000\u0003<\u0000" +
"\u0004\t\u0005\t\u0006\u0208\u0007\t\b\t\t\t\n\t\u000b\t\f\n\r\u0007";
return newMessageInfo(DEFAULT_INSTANCE, info, objects);
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
com.google.protobuf.Parser parser = PARSER;
if (parser == null) {
synchronized (com.hedera.hashgraph.sdk.proto.ScheduleInfo.class) {
parser = PARSER;
if (parser == null) {
parser =
new DefaultInstanceBasedParser(
DEFAULT_INSTANCE);
PARSER = parser;
}
}
}
return parser;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:proto.ScheduleInfo)
private static final com.hedera.hashgraph.sdk.proto.ScheduleInfo DEFAULT_INSTANCE;
static {
ScheduleInfo defaultInstance = new ScheduleInfo();
// New instances are implicitly immutable so no need to make
// immutable.
DEFAULT_INSTANCE = defaultInstance;
com.google.protobuf.GeneratedMessageLite.registerDefaultInstance(
ScheduleInfo.class, defaultInstance);
}
public static com.hedera.hashgraph.sdk.proto.ScheduleInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy