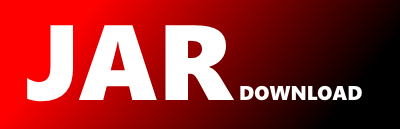
com.hedera.hashgraph.sdk.proto.TokenTransferList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk-jdk7 Show documentation
Show all versions of sdk-jdk7 Show documentation
Hedera™ Hashgraph SDK for Java
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: basic_types.proto
package com.hedera.hashgraph.sdk.proto;
/**
*
**
* A list of token IDs and amounts representing the transferred out (negative) or into (positive)
* amounts, represented in the lowest denomination of the token
*
*
* Protobuf type {@code proto.TokenTransferList}
*/
public final class TokenTransferList extends
com.google.protobuf.GeneratedMessageLite<
TokenTransferList, TokenTransferList.Builder> implements
// @@protoc_insertion_point(message_implements:proto.TokenTransferList)
TokenTransferListOrBuilder {
private TokenTransferList() {
transfers_ = emptyProtobufList();
nftTransfers_ = emptyProtobufList();
}
public static final int TOKEN_FIELD_NUMBER = 1;
private com.hedera.hashgraph.sdk.proto.TokenID token_;
/**
*
**
* The ID of the token
*
*
* .proto.TokenID token = 1;
*/
@java.lang.Override
public boolean hasToken() {
return token_ != null;
}
/**
*
**
* The ID of the token
*
*
* .proto.TokenID token = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.TokenID getToken() {
return token_ == null ? com.hedera.hashgraph.sdk.proto.TokenID.getDefaultInstance() : token_;
}
/**
*
**
* The ID of the token
*
*
* .proto.TokenID token = 1;
*/
private void setToken(com.hedera.hashgraph.sdk.proto.TokenID value) {
value.getClass();
token_ = value;
}
/**
*
**
* The ID of the token
*
*
* .proto.TokenID token = 1;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeToken(com.hedera.hashgraph.sdk.proto.TokenID value) {
value.getClass();
if (token_ != null &&
token_ != com.hedera.hashgraph.sdk.proto.TokenID.getDefaultInstance()) {
token_ =
com.hedera.hashgraph.sdk.proto.TokenID.newBuilder(token_).mergeFrom(value).buildPartial();
} else {
token_ = value;
}
}
/**
*
**
* The ID of the token
*
*
* .proto.TokenID token = 1;
*/
private void clearToken() { token_ = null;
}
public static final int TRANSFERS_FIELD_NUMBER = 2;
private com.google.protobuf.Internal.ProtobufList transfers_;
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
@java.lang.Override
public java.util.List getTransfersList() {
return transfers_;
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
public java.util.List extends com.hedera.hashgraph.sdk.proto.AccountAmountOrBuilder>
getTransfersOrBuilderList() {
return transfers_;
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
@java.lang.Override
public int getTransfersCount() {
return transfers_.size();
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountAmount getTransfers(int index) {
return transfers_.get(index);
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
public com.hedera.hashgraph.sdk.proto.AccountAmountOrBuilder getTransfersOrBuilder(
int index) {
return transfers_.get(index);
}
private void ensureTransfersIsMutable() {
com.google.protobuf.Internal.ProtobufList tmp = transfers_;
if (!tmp.isModifiable()) {
transfers_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(tmp);
}
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
private void setTransfers(
int index, com.hedera.hashgraph.sdk.proto.AccountAmount value) {
value.getClass();
ensureTransfersIsMutable();
transfers_.set(index, value);
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
private void addTransfers(com.hedera.hashgraph.sdk.proto.AccountAmount value) {
value.getClass();
ensureTransfersIsMutable();
transfers_.add(value);
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
private void addTransfers(
int index, com.hedera.hashgraph.sdk.proto.AccountAmount value) {
value.getClass();
ensureTransfersIsMutable();
transfers_.add(index, value);
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
private void addAllTransfers(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.AccountAmount> values) {
ensureTransfersIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, transfers_);
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
private void clearTransfers() {
transfers_ = emptyProtobufList();
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
private void removeTransfers(int index) {
ensureTransfersIsMutable();
transfers_.remove(index);
}
public static final int NFTTRANSFERS_FIELD_NUMBER = 3;
private com.google.protobuf.Internal.ProtobufList nftTransfers_;
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
@java.lang.Override
public java.util.List getNftTransfersList() {
return nftTransfers_;
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
public java.util.List extends com.hedera.hashgraph.sdk.proto.NftTransferOrBuilder>
getNftTransfersOrBuilderList() {
return nftTransfers_;
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
@java.lang.Override
public int getNftTransfersCount() {
return nftTransfers_.size();
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.NftTransfer getNftTransfers(int index) {
return nftTransfers_.get(index);
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
public com.hedera.hashgraph.sdk.proto.NftTransferOrBuilder getNftTransfersOrBuilder(
int index) {
return nftTransfers_.get(index);
}
private void ensureNftTransfersIsMutable() {
com.google.protobuf.Internal.ProtobufList tmp = nftTransfers_;
if (!tmp.isModifiable()) {
nftTransfers_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(tmp);
}
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
private void setNftTransfers(
int index, com.hedera.hashgraph.sdk.proto.NftTransfer value) {
value.getClass();
ensureNftTransfersIsMutable();
nftTransfers_.set(index, value);
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
private void addNftTransfers(com.hedera.hashgraph.sdk.proto.NftTransfer value) {
value.getClass();
ensureNftTransfersIsMutable();
nftTransfers_.add(value);
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
private void addNftTransfers(
int index, com.hedera.hashgraph.sdk.proto.NftTransfer value) {
value.getClass();
ensureNftTransfersIsMutable();
nftTransfers_.add(index, value);
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
private void addAllNftTransfers(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.NftTransfer> values) {
ensureNftTransfersIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, nftTransfers_);
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
private void clearNftTransfers() {
nftTransfers_ = emptyProtobufList();
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
private void removeNftTransfers(int index) {
ensureNftTransfersIsMutable();
nftTransfers_.remove(index);
}
public static final int EXPECTED_DECIMALS_FIELD_NUMBER = 4;
private com.google.protobuf.UInt32Value expectedDecimals_;
/**
*
**
* If present, the number of decimals this fungible token type is expected to have. The transfer
* will fail with UNEXPECTED_TOKEN_DECIMALS if the actual decimals differ.
*
*
* .google.protobuf.UInt32Value expected_decimals = 4;
*/
@java.lang.Override
public boolean hasExpectedDecimals() {
return expectedDecimals_ != null;
}
/**
*
**
* If present, the number of decimals this fungible token type is expected to have. The transfer
* will fail with UNEXPECTED_TOKEN_DECIMALS if the actual decimals differ.
*
*
* .google.protobuf.UInt32Value expected_decimals = 4;
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getExpectedDecimals() {
return expectedDecimals_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : expectedDecimals_;
}
/**
*
**
* If present, the number of decimals this fungible token type is expected to have. The transfer
* will fail with UNEXPECTED_TOKEN_DECIMALS if the actual decimals differ.
*
*
* .google.protobuf.UInt32Value expected_decimals = 4;
*/
private void setExpectedDecimals(com.google.protobuf.UInt32Value value) {
value.getClass();
expectedDecimals_ = value;
}
/**
*
**
* If present, the number of decimals this fungible token type is expected to have. The transfer
* will fail with UNEXPECTED_TOKEN_DECIMALS if the actual decimals differ.
*
*
* .google.protobuf.UInt32Value expected_decimals = 4;
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeExpectedDecimals(com.google.protobuf.UInt32Value value) {
value.getClass();
if (expectedDecimals_ != null &&
expectedDecimals_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
expectedDecimals_ =
com.google.protobuf.UInt32Value.newBuilder(expectedDecimals_).mergeFrom(value).buildPartial();
} else {
expectedDecimals_ = value;
}
}
/**
*
**
* If present, the number of decimals this fungible token type is expected to have. The transfer
* will fail with UNEXPECTED_TOKEN_DECIMALS if the actual decimals differ.
*
*
* .google.protobuf.UInt32Value expected_decimals = 4;
*/
private void clearExpectedDecimals() { expectedDecimals_ = null;
}
public static com.hedera.hashgraph.sdk.proto.TokenTransferList parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.TokenTransferList parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.TokenTransferList parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.TokenTransferList parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.TokenTransferList parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.TokenTransferList parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.TokenTransferList parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.TokenTransferList parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.TokenTransferList parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.TokenTransferList parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.TokenTransferList parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.TokenTransferList parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(com.hedera.hashgraph.sdk.proto.TokenTransferList prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
*
**
* A list of token IDs and amounts representing the transferred out (negative) or into (positive)
* amounts, represented in the lowest denomination of the token
*
*
* Protobuf type {@code proto.TokenTransferList}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
com.hedera.hashgraph.sdk.proto.TokenTransferList, Builder> implements
// @@protoc_insertion_point(builder_implements:proto.TokenTransferList)
com.hedera.hashgraph.sdk.proto.TokenTransferListOrBuilder {
// Construct using com.hedera.hashgraph.sdk.proto.TokenTransferList.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
*
**
* The ID of the token
*
*
* .proto.TokenID token = 1;
*/
@java.lang.Override
public boolean hasToken() {
return instance.hasToken();
}
/**
*
**
* The ID of the token
*
*
* .proto.TokenID token = 1;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.TokenID getToken() {
return instance.getToken();
}
/**
*
**
* The ID of the token
*
*
* .proto.TokenID token = 1;
*/
public Builder setToken(com.hedera.hashgraph.sdk.proto.TokenID value) {
copyOnWrite();
instance.setToken(value);
return this;
}
/**
*
**
* The ID of the token
*
*
* .proto.TokenID token = 1;
*/
public Builder setToken(
com.hedera.hashgraph.sdk.proto.TokenID.Builder builderForValue) {
copyOnWrite();
instance.setToken(builderForValue.build());
return this;
}
/**
*
**
* The ID of the token
*
*
* .proto.TokenID token = 1;
*/
public Builder mergeToken(com.hedera.hashgraph.sdk.proto.TokenID value) {
copyOnWrite();
instance.mergeToken(value);
return this;
}
/**
*
**
* The ID of the token
*
*
* .proto.TokenID token = 1;
*/
public Builder clearToken() { copyOnWrite();
instance.clearToken();
return this;
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
@java.lang.Override
public java.util.List getTransfersList() {
return java.util.Collections.unmodifiableList(
instance.getTransfersList());
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
@java.lang.Override
public int getTransfersCount() {
return instance.getTransfersCount();
}/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.AccountAmount getTransfers(int index) {
return instance.getTransfers(index);
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
public Builder setTransfers(
int index, com.hedera.hashgraph.sdk.proto.AccountAmount value) {
copyOnWrite();
instance.setTransfers(index, value);
return this;
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
public Builder setTransfers(
int index, com.hedera.hashgraph.sdk.proto.AccountAmount.Builder builderForValue) {
copyOnWrite();
instance.setTransfers(index,
builderForValue.build());
return this;
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
public Builder addTransfers(com.hedera.hashgraph.sdk.proto.AccountAmount value) {
copyOnWrite();
instance.addTransfers(value);
return this;
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
public Builder addTransfers(
int index, com.hedera.hashgraph.sdk.proto.AccountAmount value) {
copyOnWrite();
instance.addTransfers(index, value);
return this;
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
public Builder addTransfers(
com.hedera.hashgraph.sdk.proto.AccountAmount.Builder builderForValue) {
copyOnWrite();
instance.addTransfers(builderForValue.build());
return this;
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
public Builder addTransfers(
int index, com.hedera.hashgraph.sdk.proto.AccountAmount.Builder builderForValue) {
copyOnWrite();
instance.addTransfers(index,
builderForValue.build());
return this;
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
public Builder addAllTransfers(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.AccountAmount> values) {
copyOnWrite();
instance.addAllTransfers(values);
return this;
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
public Builder clearTransfers() {
copyOnWrite();
instance.clearTransfers();
return this;
}
/**
*
**
* Applicable to tokens of type FUNGIBLE_COMMON. Multiple list of AccountAmounts, each of which
* has an account and amount
*
*
* repeated .proto.AccountAmount transfers = 2;
*/
public Builder removeTransfers(int index) {
copyOnWrite();
instance.removeTransfers(index);
return this;
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
@java.lang.Override
public java.util.List getNftTransfersList() {
return java.util.Collections.unmodifiableList(
instance.getNftTransfersList());
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
@java.lang.Override
public int getNftTransfersCount() {
return instance.getNftTransfersCount();
}/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
@java.lang.Override
public com.hedera.hashgraph.sdk.proto.NftTransfer getNftTransfers(int index) {
return instance.getNftTransfers(index);
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
public Builder setNftTransfers(
int index, com.hedera.hashgraph.sdk.proto.NftTransfer value) {
copyOnWrite();
instance.setNftTransfers(index, value);
return this;
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
public Builder setNftTransfers(
int index, com.hedera.hashgraph.sdk.proto.NftTransfer.Builder builderForValue) {
copyOnWrite();
instance.setNftTransfers(index,
builderForValue.build());
return this;
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
public Builder addNftTransfers(com.hedera.hashgraph.sdk.proto.NftTransfer value) {
copyOnWrite();
instance.addNftTransfers(value);
return this;
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
public Builder addNftTransfers(
int index, com.hedera.hashgraph.sdk.proto.NftTransfer value) {
copyOnWrite();
instance.addNftTransfers(index, value);
return this;
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
public Builder addNftTransfers(
com.hedera.hashgraph.sdk.proto.NftTransfer.Builder builderForValue) {
copyOnWrite();
instance.addNftTransfers(builderForValue.build());
return this;
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
public Builder addNftTransfers(
int index, com.hedera.hashgraph.sdk.proto.NftTransfer.Builder builderForValue) {
copyOnWrite();
instance.addNftTransfers(index,
builderForValue.build());
return this;
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
public Builder addAllNftTransfers(
java.lang.Iterable extends com.hedera.hashgraph.sdk.proto.NftTransfer> values) {
copyOnWrite();
instance.addAllNftTransfers(values);
return this;
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
public Builder clearNftTransfers() {
copyOnWrite();
instance.clearNftTransfers();
return this;
}
/**
*
**
* Applicable to tokens of type NON_FUNGIBLE_UNIQUE. Multiple list of NftTransfers, each of
* which has a sender and receiver account, including the serial number of the NFT
*
*
* repeated .proto.NftTransfer nftTransfers = 3;
*/
public Builder removeNftTransfers(int index) {
copyOnWrite();
instance.removeNftTransfers(index);
return this;
}
/**
*
**
* If present, the number of decimals this fungible token type is expected to have. The transfer
* will fail with UNEXPECTED_TOKEN_DECIMALS if the actual decimals differ.
*
*
* .google.protobuf.UInt32Value expected_decimals = 4;
*/
@java.lang.Override
public boolean hasExpectedDecimals() {
return instance.hasExpectedDecimals();
}
/**
*
**
* If present, the number of decimals this fungible token type is expected to have. The transfer
* will fail with UNEXPECTED_TOKEN_DECIMALS if the actual decimals differ.
*
*
* .google.protobuf.UInt32Value expected_decimals = 4;
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getExpectedDecimals() {
return instance.getExpectedDecimals();
}
/**
*
**
* If present, the number of decimals this fungible token type is expected to have. The transfer
* will fail with UNEXPECTED_TOKEN_DECIMALS if the actual decimals differ.
*
*
* .google.protobuf.UInt32Value expected_decimals = 4;
*/
public Builder setExpectedDecimals(com.google.protobuf.UInt32Value value) {
copyOnWrite();
instance.setExpectedDecimals(value);
return this;
}
/**
*
**
* If present, the number of decimals this fungible token type is expected to have. The transfer
* will fail with UNEXPECTED_TOKEN_DECIMALS if the actual decimals differ.
*
*
* .google.protobuf.UInt32Value expected_decimals = 4;
*/
public Builder setExpectedDecimals(
com.google.protobuf.UInt32Value.Builder builderForValue) {
copyOnWrite();
instance.setExpectedDecimals(builderForValue.build());
return this;
}
/**
*
**
* If present, the number of decimals this fungible token type is expected to have. The transfer
* will fail with UNEXPECTED_TOKEN_DECIMALS if the actual decimals differ.
*
*
* .google.protobuf.UInt32Value expected_decimals = 4;
*/
public Builder mergeExpectedDecimals(com.google.protobuf.UInt32Value value) {
copyOnWrite();
instance.mergeExpectedDecimals(value);
return this;
}
/**
*
**
* If present, the number of decimals this fungible token type is expected to have. The transfer
* will fail with UNEXPECTED_TOKEN_DECIMALS if the actual decimals differ.
*
*
* .google.protobuf.UInt32Value expected_decimals = 4;
*/
public Builder clearExpectedDecimals() { copyOnWrite();
instance.clearExpectedDecimals();
return this;
}
// @@protoc_insertion_point(builder_scope:proto.TokenTransferList)
}
@java.lang.Override
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new com.hedera.hashgraph.sdk.proto.TokenTransferList();
}
case NEW_BUILDER: {
return new Builder();
}
case BUILD_MESSAGE_INFO: {
java.lang.Object[] objects = new java.lang.Object[] {
"token_",
"transfers_",
com.hedera.hashgraph.sdk.proto.AccountAmount.class,
"nftTransfers_",
com.hedera.hashgraph.sdk.proto.NftTransfer.class,
"expectedDecimals_",
};
java.lang.String info =
"\u0000\u0004\u0000\u0000\u0001\u0004\u0004\u0000\u0002\u0000\u0001\t\u0002\u001b" +
"\u0003\u001b\u0004\t";
return newMessageInfo(DEFAULT_INSTANCE, info, objects);
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
com.google.protobuf.Parser parser = PARSER;
if (parser == null) {
synchronized (com.hedera.hashgraph.sdk.proto.TokenTransferList.class) {
parser = PARSER;
if (parser == null) {
parser =
new DefaultInstanceBasedParser(
DEFAULT_INSTANCE);
PARSER = parser;
}
}
}
return parser;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:proto.TokenTransferList)
private static final com.hedera.hashgraph.sdk.proto.TokenTransferList DEFAULT_INSTANCE;
static {
TokenTransferList defaultInstance = new TokenTransferList();
// New instances are implicitly immutable so no need to make
// immutable.
DEFAULT_INSTANCE = defaultInstance;
com.google.protobuf.GeneratedMessageLite.registerDefaultInstance(
TokenTransferList.class, defaultInstance);
}
public static com.hedera.hashgraph.sdk.proto.TokenTransferList getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy