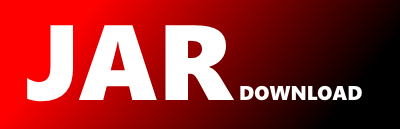
com.hedera.hashgraph.sdk.proto.Transaction Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: transaction.proto
package com.hedera.hashgraph.sdk.proto;
/**
*
**
* A single signed transaction, including all its signatures. The SignatureList will have a
* Signature for each Key in the transaction, either explicit or implicit, in the order that they
* appear in the transaction. For example, a CryptoTransfer will first have a Signature
* corresponding to the Key for the paying account, followed by a Signature corresponding to the Key
* for each account that is sending or receiving cryptocurrency in the transfer. Each Transaction
* should not have more than 50 levels.
* The SignatureList field is deprecated and succeeded by SignatureMap.
*
*
* Protobuf type {@code proto.Transaction}
*/
public final class Transaction extends
com.google.protobuf.GeneratedMessageLite<
Transaction, Transaction.Builder> implements
// @@protoc_insertion_point(message_implements:proto.Transaction)
TransactionOrBuilder {
private Transaction() {
bodyBytes_ = com.google.protobuf.ByteString.EMPTY;
signedTransactionBytes_ = com.google.protobuf.ByteString.EMPTY;
}
public static final int BODY_FIELD_NUMBER = 1;
private com.hedera.hashgraph.sdk.proto.TransactionBody body_;
/**
*
**
* the body of the transaction, which needs to be signed
*
*
* .proto.TransactionBody body = 1 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasBody() {
return body_ != null;
}
/**
*
**
* the body of the transaction, which needs to be signed
*
*
* .proto.TransactionBody body = 1 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.TransactionBody getBody() {
return body_ == null ? com.hedera.hashgraph.sdk.proto.TransactionBody.getDefaultInstance() : body_;
}
/**
*
**
* the body of the transaction, which needs to be signed
*
*
* .proto.TransactionBody body = 1 [deprecated = true];
*/
private void setBody(com.hedera.hashgraph.sdk.proto.TransactionBody value) {
value.getClass();
body_ = value;
}
/**
*
**
* the body of the transaction, which needs to be signed
*
*
* .proto.TransactionBody body = 1 [deprecated = true];
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeBody(com.hedera.hashgraph.sdk.proto.TransactionBody value) {
value.getClass();
if (body_ != null &&
body_ != com.hedera.hashgraph.sdk.proto.TransactionBody.getDefaultInstance()) {
body_ =
com.hedera.hashgraph.sdk.proto.TransactionBody.newBuilder(body_).mergeFrom(value).buildPartial();
} else {
body_ = value;
}
}
/**
*
**
* the body of the transaction, which needs to be signed
*
*
* .proto.TransactionBody body = 1 [deprecated = true];
*/
private void clearBody() { body_ = null;
}
public static final int SIGS_FIELD_NUMBER = 2;
private com.hedera.hashgraph.sdk.proto.SignatureList sigs_;
/**
*
**
* The signatures on the body, to authorize the transaction; deprecated and to be succeeded by
* SignatureMap field
*
*
* .proto.SignatureList sigs = 2 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasSigs() {
return sigs_ != null;
}
/**
*
**
* The signatures on the body, to authorize the transaction; deprecated and to be succeeded by
* SignatureMap field
*
*
* .proto.SignatureList sigs = 2 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.SignatureList getSigs() {
return sigs_ == null ? com.hedera.hashgraph.sdk.proto.SignatureList.getDefaultInstance() : sigs_;
}
/**
*
**
* The signatures on the body, to authorize the transaction; deprecated and to be succeeded by
* SignatureMap field
*
*
* .proto.SignatureList sigs = 2 [deprecated = true];
*/
private void setSigs(com.hedera.hashgraph.sdk.proto.SignatureList value) {
value.getClass();
sigs_ = value;
}
/**
*
**
* The signatures on the body, to authorize the transaction; deprecated and to be succeeded by
* SignatureMap field
*
*
* .proto.SignatureList sigs = 2 [deprecated = true];
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeSigs(com.hedera.hashgraph.sdk.proto.SignatureList value) {
value.getClass();
if (sigs_ != null &&
sigs_ != com.hedera.hashgraph.sdk.proto.SignatureList.getDefaultInstance()) {
sigs_ =
com.hedera.hashgraph.sdk.proto.SignatureList.newBuilder(sigs_).mergeFrom(value).buildPartial();
} else {
sigs_ = value;
}
}
/**
*
**
* The signatures on the body, to authorize the transaction; deprecated and to be succeeded by
* SignatureMap field
*
*
* .proto.SignatureList sigs = 2 [deprecated = true];
*/
private void clearSigs() { sigs_ = null;
}
public static final int SIGMAP_FIELD_NUMBER = 3;
private com.hedera.hashgraph.sdk.proto.SignatureMap sigMap_;
/**
*
**
* The signatures on the body with the new format, to authorize the transaction
*
*
* .proto.SignatureMap sigMap = 3 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasSigMap() {
return sigMap_ != null;
}
/**
*
**
* The signatures on the body with the new format, to authorize the transaction
*
*
* .proto.SignatureMap sigMap = 3 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.SignatureMap getSigMap() {
return sigMap_ == null ? com.hedera.hashgraph.sdk.proto.SignatureMap.getDefaultInstance() : sigMap_;
}
/**
*
**
* The signatures on the body with the new format, to authorize the transaction
*
*
* .proto.SignatureMap sigMap = 3 [deprecated = true];
*/
private void setSigMap(com.hedera.hashgraph.sdk.proto.SignatureMap value) {
value.getClass();
sigMap_ = value;
}
/**
*
**
* The signatures on the body with the new format, to authorize the transaction
*
*
* .proto.SignatureMap sigMap = 3 [deprecated = true];
*/
@java.lang.SuppressWarnings({"ReferenceEquality"})
private void mergeSigMap(com.hedera.hashgraph.sdk.proto.SignatureMap value) {
value.getClass();
if (sigMap_ != null &&
sigMap_ != com.hedera.hashgraph.sdk.proto.SignatureMap.getDefaultInstance()) {
sigMap_ =
com.hedera.hashgraph.sdk.proto.SignatureMap.newBuilder(sigMap_).mergeFrom(value).buildPartial();
} else {
sigMap_ = value;
}
}
/**
*
**
* The signatures on the body with the new format, to authorize the transaction
*
*
* .proto.SignatureMap sigMap = 3 [deprecated = true];
*/
private void clearSigMap() { sigMap_ = null;
}
public static final int BODYBYTES_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString bodyBytes_;
/**
*
**
* TransactionBody serialized into bytes, which needs to be signed
*
*
* bytes bodyBytes = 4 [deprecated = true];
* @deprecated proto.Transaction.bodyBytes is deprecated.
* See transaction.proto;l=62
* @return The bodyBytes.
*/
@java.lang.Override
@java.lang.Deprecated public com.google.protobuf.ByteString getBodyBytes() {
return bodyBytes_;
}
/**
*
**
* TransactionBody serialized into bytes, which needs to be signed
*
*
* bytes bodyBytes = 4 [deprecated = true];
* @deprecated proto.Transaction.bodyBytes is deprecated.
* See transaction.proto;l=62
* @param value The bodyBytes to set.
*/
private void setBodyBytes(com.google.protobuf.ByteString value) {
java.lang.Class> valueClass = value.getClass();
bodyBytes_ = value;
}
/**
*
**
* TransactionBody serialized into bytes, which needs to be signed
*
*
* bytes bodyBytes = 4 [deprecated = true];
* @deprecated proto.Transaction.bodyBytes is deprecated.
* See transaction.proto;l=62
*/
private void clearBodyBytes() {
bodyBytes_ = getDefaultInstance().getBodyBytes();
}
public static final int SIGNEDTRANSACTIONBYTES_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString signedTransactionBytes_;
/**
*
**
* SignedTransaction serialized into bytes
*
*
* bytes signedTransactionBytes = 5;
* @return The signedTransactionBytes.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSignedTransactionBytes() {
return signedTransactionBytes_;
}
/**
*
**
* SignedTransaction serialized into bytes
*
*
* bytes signedTransactionBytes = 5;
* @param value The signedTransactionBytes to set.
*/
private void setSignedTransactionBytes(com.google.protobuf.ByteString value) {
java.lang.Class> valueClass = value.getClass();
signedTransactionBytes_ = value;
}
/**
*
**
* SignedTransaction serialized into bytes
*
*
* bytes signedTransactionBytes = 5;
*/
private void clearSignedTransactionBytes() {
signedTransactionBytes_ = getDefaultInstance().getSignedTransactionBytes();
}
public static com.hedera.hashgraph.sdk.proto.Transaction parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.Transaction parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.Transaction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.Transaction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.Transaction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static com.hedera.hashgraph.sdk.proto.Transaction parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.Transaction parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.Transaction parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.Transaction parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.Transaction parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static com.hedera.hashgraph.sdk.proto.Transaction parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static com.hedera.hashgraph.sdk.proto.Transaction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(com.hedera.hashgraph.sdk.proto.Transaction prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
*
**
* A single signed transaction, including all its signatures. The SignatureList will have a
* Signature for each Key in the transaction, either explicit or implicit, in the order that they
* appear in the transaction. For example, a CryptoTransfer will first have a Signature
* corresponding to the Key for the paying account, followed by a Signature corresponding to the Key
* for each account that is sending or receiving cryptocurrency in the transfer. Each Transaction
* should not have more than 50 levels.
* The SignatureList field is deprecated and succeeded by SignatureMap.
*
*
* Protobuf type {@code proto.Transaction}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
com.hedera.hashgraph.sdk.proto.Transaction, Builder> implements
// @@protoc_insertion_point(builder_implements:proto.Transaction)
com.hedera.hashgraph.sdk.proto.TransactionOrBuilder {
// Construct using com.hedera.hashgraph.sdk.proto.Transaction.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
*
**
* the body of the transaction, which needs to be signed
*
*
* .proto.TransactionBody body = 1 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasBody() {
return instance.hasBody();
}
/**
*
**
* the body of the transaction, which needs to be signed
*
*
* .proto.TransactionBody body = 1 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.TransactionBody getBody() {
return instance.getBody();
}
/**
*
**
* the body of the transaction, which needs to be signed
*
*
* .proto.TransactionBody body = 1 [deprecated = true];
*/
@java.lang.Deprecated public Builder setBody(com.hedera.hashgraph.sdk.proto.TransactionBody value) {
copyOnWrite();
instance.setBody(value);
return this;
}
/**
*
**
* the body of the transaction, which needs to be signed
*
*
* .proto.TransactionBody body = 1 [deprecated = true];
*/
@java.lang.Deprecated public Builder setBody(
com.hedera.hashgraph.sdk.proto.TransactionBody.Builder builderForValue) {
copyOnWrite();
instance.setBody(builderForValue.build());
return this;
}
/**
*
**
* the body of the transaction, which needs to be signed
*
*
* .proto.TransactionBody body = 1 [deprecated = true];
*/
@java.lang.Deprecated public Builder mergeBody(com.hedera.hashgraph.sdk.proto.TransactionBody value) {
copyOnWrite();
instance.mergeBody(value);
return this;
}
/**
*
**
* the body of the transaction, which needs to be signed
*
*
* .proto.TransactionBody body = 1 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearBody() { copyOnWrite();
instance.clearBody();
return this;
}
/**
*
**
* The signatures on the body, to authorize the transaction; deprecated and to be succeeded by
* SignatureMap field
*
*
* .proto.SignatureList sigs = 2 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasSigs() {
return instance.hasSigs();
}
/**
*
**
* The signatures on the body, to authorize the transaction; deprecated and to be succeeded by
* SignatureMap field
*
*
* .proto.SignatureList sigs = 2 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.SignatureList getSigs() {
return instance.getSigs();
}
/**
*
**
* The signatures on the body, to authorize the transaction; deprecated and to be succeeded by
* SignatureMap field
*
*
* .proto.SignatureList sigs = 2 [deprecated = true];
*/
@java.lang.Deprecated public Builder setSigs(com.hedera.hashgraph.sdk.proto.SignatureList value) {
copyOnWrite();
instance.setSigs(value);
return this;
}
/**
*
**
* The signatures on the body, to authorize the transaction; deprecated and to be succeeded by
* SignatureMap field
*
*
* .proto.SignatureList sigs = 2 [deprecated = true];
*/
@java.lang.Deprecated public Builder setSigs(
com.hedera.hashgraph.sdk.proto.SignatureList.Builder builderForValue) {
copyOnWrite();
instance.setSigs(builderForValue.build());
return this;
}
/**
*
**
* The signatures on the body, to authorize the transaction; deprecated and to be succeeded by
* SignatureMap field
*
*
* .proto.SignatureList sigs = 2 [deprecated = true];
*/
@java.lang.Deprecated public Builder mergeSigs(com.hedera.hashgraph.sdk.proto.SignatureList value) {
copyOnWrite();
instance.mergeSigs(value);
return this;
}
/**
*
**
* The signatures on the body, to authorize the transaction; deprecated and to be succeeded by
* SignatureMap field
*
*
* .proto.SignatureList sigs = 2 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearSigs() { copyOnWrite();
instance.clearSigs();
return this;
}
/**
*
**
* The signatures on the body with the new format, to authorize the transaction
*
*
* .proto.SignatureMap sigMap = 3 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasSigMap() {
return instance.hasSigMap();
}
/**
*
**
* The signatures on the body with the new format, to authorize the transaction
*
*
* .proto.SignatureMap sigMap = 3 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public com.hedera.hashgraph.sdk.proto.SignatureMap getSigMap() {
return instance.getSigMap();
}
/**
*
**
* The signatures on the body with the new format, to authorize the transaction
*
*
* .proto.SignatureMap sigMap = 3 [deprecated = true];
*/
@java.lang.Deprecated public Builder setSigMap(com.hedera.hashgraph.sdk.proto.SignatureMap value) {
copyOnWrite();
instance.setSigMap(value);
return this;
}
/**
*
**
* The signatures on the body with the new format, to authorize the transaction
*
*
* .proto.SignatureMap sigMap = 3 [deprecated = true];
*/
@java.lang.Deprecated public Builder setSigMap(
com.hedera.hashgraph.sdk.proto.SignatureMap.Builder builderForValue) {
copyOnWrite();
instance.setSigMap(builderForValue.build());
return this;
}
/**
*
**
* The signatures on the body with the new format, to authorize the transaction
*
*
* .proto.SignatureMap sigMap = 3 [deprecated = true];
*/
@java.lang.Deprecated public Builder mergeSigMap(com.hedera.hashgraph.sdk.proto.SignatureMap value) {
copyOnWrite();
instance.mergeSigMap(value);
return this;
}
/**
*
**
* The signatures on the body with the new format, to authorize the transaction
*
*
* .proto.SignatureMap sigMap = 3 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearSigMap() { copyOnWrite();
instance.clearSigMap();
return this;
}
/**
*
**
* TransactionBody serialized into bytes, which needs to be signed
*
*
* bytes bodyBytes = 4 [deprecated = true];
* @deprecated proto.Transaction.bodyBytes is deprecated.
* See transaction.proto;l=62
* @return The bodyBytes.
*/
@java.lang.Override
@java.lang.Deprecated public com.google.protobuf.ByteString getBodyBytes() {
return instance.getBodyBytes();
}
/**
*
**
* TransactionBody serialized into bytes, which needs to be signed
*
*
* bytes bodyBytes = 4 [deprecated = true];
* @deprecated proto.Transaction.bodyBytes is deprecated.
* See transaction.proto;l=62
* @param value The bodyBytes to set.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder setBodyBytes(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setBodyBytes(value);
return this;
}
/**
*
**
* TransactionBody serialized into bytes, which needs to be signed
*
*
* bytes bodyBytes = 4 [deprecated = true];
* @deprecated proto.Transaction.bodyBytes is deprecated.
* See transaction.proto;l=62
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder clearBodyBytes() {
copyOnWrite();
instance.clearBodyBytes();
return this;
}
/**
*
**
* SignedTransaction serialized into bytes
*
*
* bytes signedTransactionBytes = 5;
* @return The signedTransactionBytes.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSignedTransactionBytes() {
return instance.getSignedTransactionBytes();
}
/**
*
**
* SignedTransaction serialized into bytes
*
*
* bytes signedTransactionBytes = 5;
* @param value The signedTransactionBytes to set.
* @return This builder for chaining.
*/
public Builder setSignedTransactionBytes(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setSignedTransactionBytes(value);
return this;
}
/**
*
**
* SignedTransaction serialized into bytes
*
*
* bytes signedTransactionBytes = 5;
* @return This builder for chaining.
*/
public Builder clearSignedTransactionBytes() {
copyOnWrite();
instance.clearSignedTransactionBytes();
return this;
}
// @@protoc_insertion_point(builder_scope:proto.Transaction)
}
@java.lang.Override
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new com.hedera.hashgraph.sdk.proto.Transaction();
}
case NEW_BUILDER: {
return new Builder();
}
case BUILD_MESSAGE_INFO: {
java.lang.Object[] objects = new java.lang.Object[] {
"body_",
"sigs_",
"sigMap_",
"bodyBytes_",
"signedTransactionBytes_",
};
java.lang.String info =
"\u0000\u0005\u0000\u0000\u0001\u0005\u0005\u0000\u0000\u0000\u0001\t\u0002\t\u0003" +
"\t\u0004\n\u0005\n";
return newMessageInfo(DEFAULT_INSTANCE, info, objects);
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
com.google.protobuf.Parser parser = PARSER;
if (parser == null) {
synchronized (com.hedera.hashgraph.sdk.proto.Transaction.class) {
parser = PARSER;
if (parser == null) {
parser =
new DefaultInstanceBasedParser(
DEFAULT_INSTANCE);
PARSER = parser;
}
}
}
return parser;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:proto.Transaction)
private static final com.hedera.hashgraph.sdk.proto.Transaction DEFAULT_INSTANCE;
static {
Transaction defaultInstance = new Transaction();
// New instances are implicitly immutable so no need to make
// immutable.
DEFAULT_INSTANCE = defaultInstance;
com.google.protobuf.GeneratedMessageLite.registerDefaultInstance(
Transaction.class, defaultInstance);
}
public static com.hedera.hashgraph.sdk.proto.Transaction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy