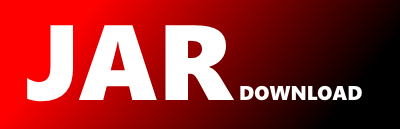
com.altova.text.TextNodeXMLSerializer Maven / Gradle / Ivy
////////////////////////////////////////////////////////////////////////
//
// TextNodeXMLSerializer.java
//
// This file was generated by MapForce 2017sp2.
//
// YOU SHOULD NOT MODIFY THIS FILE, BECAUSE IT WILL BE
// OVERWRITTEN WHEN YOU RE-RUN CODE GENERATION.
//
// Refer to the MapForce Documentation for further details.
// http://www.altova.com/mapforce
//
////////////////////////////////////////////////////////////////////////
package com.altova.text;
import java.io.IOException;
import java.io.Writer;
public class TextNodeXMLSerializer {
private Writer m_Stream;
private void writeStartTag(String name, byte nodeClass) throws IOException {
StringBuffer buffer = new StringBuffer();
buffer.append('<');
buffer.append(name);
buffer.append(" Class=\"");
switch (nodeClass) {
case ITextNode.DataElement:
buffer.append("DataElement");
break;
case ITextNode.Composite:
buffer.append("Composite");
break;
case ITextNode.Segment:
buffer.append("Segment");
break;
case ITextNode.Group:
buffer.append("Group");
break;
case ITextNode.SubComposite:
buffer.append("SubComposite");
break;
}
buffer.append("\">");
m_Stream.write(buffer.toString());
}
private void writeValue(String value) throws IOException {
m_Stream.write(value);
}
private void writeEndTag(String name) throws IOException {
m_Stream.write("" + name + ">");
}
public TextNodeXMLSerializer(Writer writer) throws IOException {
m_Stream = writer;
m_Stream.write("");
}
public void serialize(ITextNode node) throws Exception {
String name = node.getName();
if (null != name) {
this.writeStartTag(name, node.getNodeClass());
String value = node.getValue();
this.writeValue(value);
final ITextNodeList children = node.getChildren();
final int count = children.size();
for (int i = 0; i < count; ++i)
serialize(children.getAt(i));
this.writeEndTag(name);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy