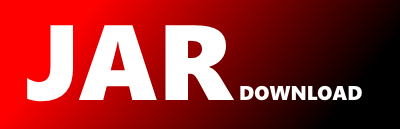
com.altova.text.edi.DataElement Maven / Gradle / Ivy
////////////////////////////////////////////////////////////////////////
//
// DataElement.java
//
// This file was generated by MapForce 2017sp2.
//
// YOU SHOULD NOT MODIFY THIS FILE, BECAUSE IT WILL BE
// OVERWRITTEN WHEN YOU RE-RUN CODE GENERATION.
//
// Refer to the MapForce Documentation for further details.
// http://www.altova.com/mapforce
//
////////////////////////////////////////////////////////////////////////
package com.altova.text.edi;
import com.altova.text.ITextNode;
import com.altova.text.edi.Scanner.State;
import java.io.IOException;
public class DataElement extends StructureItem {
DataTypeValidator mValidator;
public DataElement (String name, DataTypeValidator validator) {
super(name, ITextNode.DataElement);
mValidator = validator;
}
public boolean read (Parser.Context context) {
State beforeState = context.getScanner().getCurrentState();
if ( context.getParser().getEDIKind() == EDISettings.EDIStandard.EDIFixed )
{
Scanner scanner = context.getScanner();
StringBuffer sBuffer = new StringBuffer();
while( sBuffer.length() < mValidator.getMaxLength() && !scanner.isAtEnd() &&
scanner.getCurrentChar() != scanner.mServiceChars.getSegmentTerminator() )
{
char c = scanner.rawConsumeChar();
if( scanner.getCurrentChar() == '\n' )
{
if( c != '\r' )
sBuffer.append( c );
}
else
sBuffer.append( c );
}
mValidator.makeValidOnRead( sBuffer, context, beforeState);
if ( sBuffer.length() == 0)
return false; //data element is absent
context.getGenerator().insertElement (context.getParticle().getName(), sBuffer.toString(), mNodeClass);
return true;
}
//MSH-2 in HL7 MODE also needs special treatment
if( context.getParser().getEDIKind() == EDISettings.EDIStandard.EDIHL7
&& isHL7SpecialField(context.getParticle().getName(), "-2"))
{
Scanner scanner = context.getScanner();
String s = new String();
char c = scanner.rawConsumeChar();
scanner.mServiceChars.setComponentSeparator( c);
s += c;
s += c = scanner.rawConsumeChar();
scanner.mServiceChars.setRepetitionSeparator( c);
if( scanner.getCurrentChar() != scanner.mServiceChars.getDataElementSeparator())
{
s += c = scanner.rawConsumeChar();
scanner.mServiceChars.setReleaseCharacter( c);
}
if( scanner.getCurrentChar() != scanner.mServiceChars.getDataElementSeparator())
{
s += c = scanner.rawConsumeChar();
scanner.mServiceChars.setSubComponentSeparator( c);
}
while( scanner.getCurrentChar() != scanner.mServiceChars.getDataElementSeparator())
{
s += c = scanner.rawConsumeChar();
}
context.getGenerator().insertElement (context.getParticle().getName(), s, mNodeClass);
return true;
}
StringBuffer s = context.getScanner().consumeString(ServiceChars.ComponentSeparator, true);
if ( s.length() == 0 )
return false; // data element is absent
mValidator.makeValidOnRead(s, context, beforeState);
if( context.getParser().getEDIKind() == EDISettings.EDIStandard.EDIX12 )
{
if( context.getParticle().getName().equals( "F447") && context.getCurrentSegmentName().equals("LS") )
context.getParser().setF447( new String( s) );
}
// codelist validate
if (!mValidator.hasValue(s.toString()))
{
if (!mValidator.isIncomplete())
context.handleError(
Parser.ErrorType.CodeListValueWrong,
ErrorMessages.GetInvalidCodeListValueMessage(s.toString(), mValidator.getCodeListValues()),
new ErrorPosition( beforeState ),
s.toString()
);
else
context.handleWarning(
ErrorMessages.GetIncompleteCodeListValueMessage(s.toString(), mValidator.getCodeListValues()),
new ErrorPosition( beforeState ),
s.toString()
);
}
// embedded codelist validate
if (!embeddedCodeListValidate(s.toString(), context.getParticle()))
context.handleError(
Parser.ErrorType.CodeListValueWrong,
ErrorMessages.GetInvalidCodeListValueMessage(s.toString(), embeddedCodeListValueList(context.getParticle())),
new ErrorPosition( beforeState ),
s.toString()
);
// edi validate
String sError = context.getValidator().validate( context.getParticle().getName(), s.toString());
if( sError.length() > 0)
context.handleError(
Parser.ErrorType.SemanticWrong,
sError,
new ErrorPosition( beforeState ),
s.toString()
);
context.getGenerator().insertElement (context.getParticle().getName(), s.toString(), mNodeClass);
return true;
}
public void write (Writer writer, ITextNode node, Particle particle) throws IOException {
StringBuffer sbvalue = new StringBuffer();
String nodeName = new String();
if (node != null)
{
sbvalue.append(node.getValue());
nodeName = node.getName();
}
if (writer.getEDIKind() == EDISettings.EDIStandard.EDIFixed)
{
mValidator.makeValidOnWrite( sbvalue, node, writer, false);
writer.write( sbvalue.toString() );
return;
}
boolean escapeString = true;
if (writer.getEDIKind() == EDISettings.EDIStandard.EDIHL7
&& isHL7SpecialField(nodeName, "-2"))
{
sbvalue = new StringBuffer();
sbvalue.append( writer.getServiceChars().getComponentSeparator());
sbvalue.append( writer.getServiceChars().getRepetitionSeparator());
if( writer.getServiceChars().getReleaseCharacter() != 0 )
{
sbvalue.append( writer.getServiceChars().getReleaseCharacter());
if( writer.getServiceChars().getSubComponentSeparator() != 0 )
sbvalue.append( writer.getServiceChars().getSubComponentSeparator());
}
//don't escape separators
escapeString = false;
}
if (!mValidator.makeValidOnWrite (sbvalue, node, writer, escapeString))
return;
//codelist validate
String sValue = sbvalue.toString();
if (!mValidator.hasValue( sValue))
{
if (!mValidator.isIncomplete())
writer.handleError( node, Parser.ErrorType.CodeListValueWrong, ErrorMessages.GetInvalidCodeListValueMessage(sValue, mValidator.getCodeListValues()) );
else
writer.handleWarning( node, ErrorMessages.GetIncompleteCodeListValueMessage(sValue, mValidator.getCodeListValues()) );
}
// embedded codelist validate
if (!embeddedCodeListValidate(sValue, particle))
writer.handleError(
node,
Parser.ErrorType.CodeListValueWrong,
ErrorMessages.GetInvalidCodeListValueMessage(sValue, embeddedCodeListValueList(particle))
);
//edi validate
String sError = writer.getValidator().validate( nodeName, sValue);
if( sError.length() > 0)
writer.handleError( node, Parser.ErrorType.SemanticWrong, sError);
writer.write (sValue);
}
private boolean embeddedCodeListValidate(String value, Particle particle)
{
if (particle.getCodeValues().length == 0)
return true;
for(String s : particle.getCodeValues())
if (s.equals( value ))
return true;
return false;
}
private String embeddedCodeListValueList(Particle particle)
{
StringBuffer validList = new StringBuffer();
for(String s : particle.getCodeValues())
validList.append(", '" + s + "'");
return validList.toString().substring(2);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy