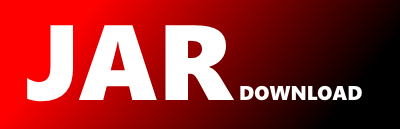
com.altova.text.edi.Writer Maven / Gradle / Ivy
////////////////////////////////////////////////////////////////////////
//
// Writer.java
//
// This file was generated by MapForce 2017sp2.
//
// YOU SHOULD NOT MODIFY THIS FILE, BECAUSE IT WILL BE
// OVERWRITTEN WHEN YOU RE-RUN CODE GENERATION.
//
// Refer to the MapForce Documentation for further details.
// http://www.altova.com/mapforce
//
////////////////////////////////////////////////////////////////////////
package com.altova.text.edi;
import java.io.IOException;
import java.util.TreeMap;
import com.altova.text.ITextNode;
public class Writer
{
java.io.Writer mOutput;
StringBuffer mPendingSeparators = new StringBuffer ();
boolean mNewlineAfterSegments = true;
EDISettings mSettings;
EDISemanticValidator mValidator;
Parser.Action[] mErrorSettings;
TreeMap mMessages;
long mLine;
long mPosition;
long mLineStart;
int mLineEnd = 0;
public ServiceChars getServiceChars() {
return mSettings.getServiceChars();
}
public boolean getNewlineAfterSegments() {
return mNewlineAfterSegments;
}
void setNewlineAfterSegments(boolean newline) {
mNewlineAfterSegments = newline;
}
public Writer (java.io.Writer output, TreeMap messages, EDISettings settings, Parser.Action[] errorSettings, int lineEnd) throws Exception {
mOutput = output;
mSettings = settings;
mValidator = new EDISemanticValidator( settings );
mValidator.setCurrentMessageType( messages.values().iterator().next().getMessageType() );
mErrorSettings = errorSettings;
mMessages = messages;
mLineEnd = lineEnd;
mLine = 0;
mLineStart = 0;
mPosition = 0;
if (EDISettings.EDIStandard.EDIFACT == getEDIKind()) {
EDIFactSettings edifactsettings = (EDIFactSettings) settings;
if (edifactsettings.getWriteUNA()) {
mPosition = settings.getServiceChars().serialize(output);
if (settings.getTerminateSegmentsWithLinefeed())
{
write(getLineEnd());
}
}
}
}
public void write (String s) throws IOException{
if (s.length() == 0)
return;
if( getEDIKind() != EDISettings.EDIStandard.EDIFixed )
{
String sepString = mPendingSeparators.toString();
mOutput.write (sepString);
mPosition += sepString.length();
checkNewLines(sepString);
}
mOutput.write (s);
mPosition += s.length();
checkNewLines(s);
mPendingSeparators.setLength(0);
}
public void writePendingSeparators () throws IOException{
String sepString = mPendingSeparators.toString();
mOutput.write (sepString);
mPosition += sepString.length();
checkNewLines( sepString);
mPendingSeparators.setLength(0);
}
private void checkNewLines( String s) {
for( int i = 0; i < s.length(); i++)
{
if (s.charAt(i) == '\n' || (s.charAt(i) == '\r' && SafeCharAt(s, i+1) != '\n'))
{
mLine++;
mLineStart = mPosition;
}
}
}
static private char SafeCharAt( String s, int index) {
if( index < s.length() )
return s.charAt(index);
else
return 0;
}
public EDISettings.EDIStandard getEDIKind( ) {
return mSettings.getStandard();
}
public void addSeparator (byte serviceChar) {
if ( serviceChar == ServiceChars.None)
return;
mPendingSeparators.append (getServiceChars().getSeparator(serviceChar));
}
public void clearPendingSeparators (byte separator) {
while (mPendingSeparators.length() > 0 && mPendingSeparators.charAt(mPendingSeparators.length()-1) == getServiceChars().getSeparator(separator))
mPendingSeparators.deleteCharAt(mPendingSeparators.length()-1); // gasp!
}
public void clearPendingSeparators () {
mPendingSeparators.setLength(0);
}
public EDISemanticValidator getValidator() {
return mValidator;
}
public void handleError (ITextNode node, Parser.ErrorType error, String message) {
String location = (node != null) ? node.getName() : "";
ITextNode parent = (node != null) ? node.getParent() : null;
while (parent != null && parent.getName() != null)
{
location = parent.getName() + " / " + location;
parent = parent.getParent();
}
String lineLoc = String.format(
"Line %d column %d (offset 0x%x): ",
getLine(),
getColumn(),
getPosition()
);
location = lineLoc + location;
switch ( mErrorSettings[error.ordinal()] )
{
case Stop:
{
throw new com.altova.AltovaException (location + ": " + message);
}
case ReportReject:
case ReportAccept:
{
System.err.println("Warning: " + location + ": " + message);
}
break;
case Ignore: break;
}
}
public void handleWarning (ITextNode node, String message) {
String location = (node != null) ? node.getName() : "";
ITextNode parent = (node != null) ? node.getParent() : null;
while (parent != null && parent.getName() != null)
{
location = parent.getName() + " / " + location;
parent = parent.getParent();
}
String lineLoc = String.format(
"Line %d column %d (offset 0x%x): ",
getLine(),
getColumn(),
getPosition()
);
location = lineLoc + location;
System.err.println("Warning: " + location + ": " + message);
}
public long getLine() {
return mLine + 1;
}
public long getColumn() {
return mPosition - mLineStart + mPendingSeparators.length() + 1;
}
public long getPosition() {
return mPosition + mPendingSeparators.length();
}
public String getLineEnd() {
switch(mLineEnd)
{
case 0: return java.lang.System.getProperty("line.separator");
case 1: return "\r\n";
case 2: return "\n";
case 3: return "\r";
default: return "\r\n";
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy