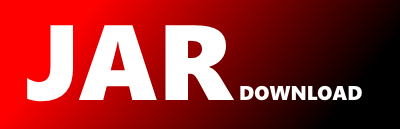
com.helger.photon.bootstrap4.form.IBootstrapFormGroupContainer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-oton-bootstrap4 Show documentation
Show all versions of ph-oton-bootstrap4 Show documentation
Library wrapping Bootstrap 4 controls as Java web application components
The newest version!
/*
* Copyright (C) 2018-2024 Philip Helger (www.helger.com)
* philip[at]helger[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.helger.photon.bootstrap4.form;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import com.helger.html.hc.html.IHCElement;
import com.helger.html.hc.html.IHCElementWithChildren;
import com.helger.photon.bootstrap4.grid.BootstrapGridSpec;
/**
* Base interface for a form group container.
*
* @author Philip Helger
* @param
* Implementation type
*/
public interface IBootstrapFormGroupContainer > extends IHCElement
{
/**
* @return The form type for aligning the form groups. Never null
* .
*/
@Nonnull
EBootstrapFormType getFormType ();
/**
* Set the left part of a horizontal form. This implicitly sets the correct
* right parts (= CBootstrap.GRID_SYSTEM_MAX - left).
*
* @param nLeftParts
* The left parts. Must be ≥ 1 and ≤ 12!
* @return this
*/
@Nonnull
default IMPLTYPE setLeft (@Nonnegative final int nLeftParts)
{
return setLeft (nLeftParts, nLeftParts, nLeftParts, nLeftParts, nLeftParts);
}
/**
* Set the left part of a horizontal form. This implicitly sets the correct
* right parts (= CBootstrap.GRID_SYSTEM_MAX - left).
*
* @param nLeftPartsXS
* The left parts XS. Must be ≥ 1 and ≤ 12!
* @param nLeftPartsSM
* The left parts SM. Must be ≥ 1 and ≤ 12!
* @param nLeftPartsMD
* The left parts MD. Must be ≥ 1 and ≤ 12!
* @param nLeftPartsLG
* The left parts LG. Must be ≥ 1 and ≤ 12!
* @param nLeftPartsXL
* The left parts XL. Must be ≥ 1 and ≤ 12!
* @return this
*/
@Nonnull
IMPLTYPE setLeft (@Nonnegative int nLeftPartsXS,
@Nonnegative int nLeftPartsSM,
@Nonnegative int nLeftPartsMD,
@Nonnegative int nLeftPartsLG,
@Nonnegative int nLeftPartsXL);
/**
* @return The left parts. Always ≥ 1 and ≤ CBootstrap.GRID_SYSTEM_MAX.
* Never null
.
*/
@Nonnull
BootstrapGridSpec getLeft ();
/**
* @return The right parts. Always
* CBootstrap.GRID_SYSTEM_MAX - getLeft ()
except left is
* CBootstrap.GRID_SYSTEM_MAX
than right is also
* CBootstrap.GRID_SYSTEM_MAX
. Never null
.
*/
@Nonnull
BootstrapGridSpec getRight ();
/**
* Set the left part of a horizontal form.
*
* @param aLeft
* The left parts. Must not be null
.
* @param aRight
* The right parts. Must not be null
.
* @return this
*/
@Nonnull
IMPLTYPE setSplitting (@Nonnull BootstrapGridSpec aLeft, @Nonnull BootstrapGridSpec aRight);
/**
* @return The renderer used to convert form groups into HC nodes. Never
* null
.
*/
@Nonnull
IBootstrapFormGroupRenderer getFormGroupRenderer ();
/**
* Set the form group renderer to be used.
*
* @param aFormGroupRenderer
* The from group renderer. May not be null
.
* @return this
*/
@Nonnull
IMPLTYPE setFormGroupRenderer (@Nonnull IBootstrapFormGroupRenderer aFormGroupRenderer);
/**
* Add a new form group at the end.
*
* @param aFormGroup
* The form group to be added. May not be null
.
* @return this
*/
@Nonnull
IMPLTYPE addFormGroup (@Nonnull BootstrapFormGroup aFormGroup);
/**
* Get the rendered form group based on the contained form group renderer. The
* form group is NOT added to this container!
*
* @param aFormGroup
* The form group to be rendered. May not be null
.
* @return The rendered form group and never null
.
*/
@Nonnull
IHCElementWithChildren > getRenderedFormGroup (@Nonnull BootstrapFormGroup aFormGroup);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy