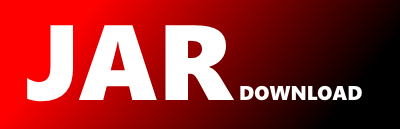
com.helger.ddd.model.DDDSyntax Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ddd Show documentation
Show all versions of ddd Show documentation
Document Details Determinator library
The newest version!
/*
* Copyright (C) 2023-2024 Philip Helger (www.helger.com)
* philip[at]helger[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.helger.ddd.model;
import java.util.Map;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.annotation.concurrent.Immutable;
import org.w3c.dom.Node;
import com.helger.commons.ValueEnforcer;
import com.helger.commons.annotation.Nonempty;
import com.helger.commons.annotation.ReturnsMutableCopy;
import com.helger.commons.collection.impl.CommonsArrayList;
import com.helger.commons.collection.impl.CommonsHashMap;
import com.helger.commons.collection.impl.ICommonsList;
import com.helger.commons.collection.impl.ICommonsMap;
import com.helger.commons.error.list.IErrorList;
import com.helger.commons.id.IHasID;
import com.helger.commons.name.IHasName;
import com.helger.commons.string.StringHelper;
import com.helger.commons.string.ToStringGenerator;
import com.helger.ddd.model.jaxb.syntax1.GetType;
import com.helger.ddd.model.jaxb.syntax1.SyntaxType;
/**
* Defines a single supported syntax model
*
* @author Philip Helger
*/
@Immutable
public class DDDSyntax implements IHasID , IHasName
{
private final String m_sID;
private final String m_sRootElementNamespaceURI;
private final String m_sRootElementLocalName;
private final String m_sName;
private final String m_sVersion;
private final ICommonsMap > m_aGetters;
public DDDSyntax (@Nonnull @Nonempty final String sID,
@Nonnull @Nonempty final String sRootElementNamespaceURI,
@Nonnull @Nonempty final String sRootElementLocalName,
@Nonnull @Nonempty final String sName,
@Nullable final String sVersion,
@Nonnull @Nonempty final ICommonsMap > aGetters)
{
ValueEnforcer.notEmpty (sID, "ID");
ValueEnforcer.notEmpty (sRootElementNamespaceURI, "RootElementNamespaceURI");
ValueEnforcer.notEmpty (sRootElementLocalName, "RootElementLocalName");
ValueEnforcer.notEmpty (sName, "Name");
ValueEnforcer.notEmptyNoNullValue (aGetters, "Getters");
m_sID = sID;
m_sRootElementNamespaceURI = sRootElementNamespaceURI;
m_sRootElementLocalName = sRootElementLocalName;
m_sName = sName;
m_sVersion = sVersion;
m_aGetters = aGetters;
}
/**
* @return The ID of the syntax. Neither null
nor empty.
*/
@Nonnull
@Nonempty
public final String getID ()
{
return m_sID;
}
@Nonnull
@Nonempty
public final String getRootElementNamespaceURI ()
{
return m_sRootElementNamespaceURI;
}
@Nonnull
@Nonempty
public final String getRootElementLocalName ()
{
return m_sRootElementLocalName;
}
public boolean matches (@Nullable final String sNamespaceURI, @Nullable final String sLocalName)
{
return m_sRootElementNamespaceURI.equals (sNamespaceURI) && m_sRootElementLocalName.equals (sLocalName);
}
@Nonnull
@Nonempty
public final String getName ()
{
return m_sName;
}
/**
* @return true
if a specific syntax version is used,
* false
if not.
*/
public final boolean hasVersion ()
{
return StringHelper.hasText (m_sVersion);
}
/**
* @return The syntax version to be used. May be null
.
*/
@Nullable
public final String getVersion ()
{
return m_sVersion;
}
@Nonnull
@Nonempty
@ReturnsMutableCopy
public final ICommonsMap > getAllGetters ()
{
// Deep clone
final ICommonsMap > ret = new CommonsHashMap <> ();
for (final Map.Entry > e : m_aGetters.entrySet ())
ret.put (e.getKey (), e.getValue ().getClone ());
return ret;
}
@Nullable
public String getValue (@Nonnull final EDDDSourceField eGetter,
@Nonnull final Node aSourceNode,
@Nonnull final IErrorList aErrorList)
{
ValueEnforcer.notNull (eGetter, "Getter");
ValueEnforcer.notNull (aSourceNode, "SourceNode");
ValueEnforcer.notNull (aErrorList, "ErrorList");
final ICommonsList aGetters = m_aGetters.get (eGetter);
if (aGetters != null)
{
// Apply them all in order. First result is used
for (final IDDDGetter aGetter : aGetters)
{
final String ret = aGetter.getValue (aSourceNode, aErrorList);
if (ret != null)
return ret;
}
}
return null;
}
@Override
public String toString ()
{
return new ToStringGenerator (null).append ("ID", m_sID)
.append ("RootElementNamespaceURI", m_sRootElementNamespaceURI)
.append ("RootElementLocalName", m_sRootElementLocalName)
.append ("Name", m_sName)
.append ("Version", m_sVersion)
.append ("Getters", m_aGetters)
.getToString ();
}
@Nonnull
public static DDDSyntax createFromJaxb (@Nonnull final SyntaxType aSyntax)
{
final String sSyntaxID = aSyntax.getId ();
final String sLogPrefix = "Syntax with ID '" + sSyntaxID + "': ";
// Name
final String sName = aSyntax.getName ().trim ();
if (StringHelper.hasNoText (sName))
throw new IllegalArgumentException (sLogPrefix + "Element 'name' may not be empty");
// Version (optional)
final String sVersion = aSyntax.getVersion ();
// Getters
final ICommonsMap > aGetters = new CommonsHashMap <> ();
for (final GetType aGet : aSyntax.getGet ())
{
// Resolve source field
final String sFieldID = aGet.getId ();
final EDDDSourceField eGetter = EDDDSourceField.getFromIDOrNull (sFieldID);
if (eGetter == null)
throw new IllegalArgumentException (sLogPrefix + "The getter ID field '" + sFieldID + "' is invalid");
// Build XPath getters
final ICommonsList aGetterList = new CommonsArrayList <> ();
if (aGet.getXpath () != null)
aGetterList.add (new DDDGetterXPath (aGet.getXpath ()));
if (aGetterList.isEmpty ())
throw new IllegalArgumentException (sLogPrefix + "The getter '" + sFieldID + "' contains no actual getter");
aGetters.put (eGetter, aGetterList);
}
// Check if all mandatory getters are present
for (final EDDDSourceField eGetter : EDDDSourceField.values ())
if (eGetter.isSyntaxDefinitionMandatory ())
if (!aGetters.containsKey (eGetter))
throw new IllegalArgumentException (sLogPrefix +
"The mandatory getter '" +
eGetter.getID () +
"' is missing");
return new DDDSyntax (sSyntaxID,
aSyntax.getNsuri ().trim (),
aSyntax.getRoot ().trim (),
sName,
sVersion == null ? null : sVersion.trim (),
aGetters);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy