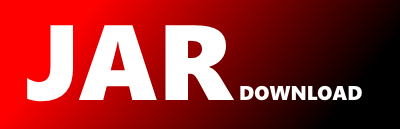
com.helger.bde.v11.cac.ObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-bde Show documentation
Show all versions of ph-bde Show documentation
Library for reading and writing OASIS BDE documents
package com.helger.bde.v11.cac;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.helger.bde.v11.cac package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _ExternalReference_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", "ExternalReference");
public final static QName _FromParty_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", "FromParty");
public final static QName _InstanceDecryptionInformationExternalReference_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", "InstanceDecryptionInformationExternalReference");
public final static QName _InstanceDecryptionKeyExternalReference_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", "InstanceDecryptionKeyExternalReference");
public final static QName _Party_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", "Party");
public final static QName _Payload_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", "Payload");
public final static QName _PayloadExternalReference_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", "PayloadExternalReference");
public final static QName _RelevantExternalReference_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", "RelevantExternalReference");
public final static QName _ToParty_QNAME = new QName("http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", "ToParty");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.helger.bde.v11.cac
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link ExternalReferenceType }
*
* @return
* The created ExternalReferenceType object and never null
.
*/
@Nonnull
public ExternalReferenceType createExternalReferenceType() {
return new ExternalReferenceType();
}
/**
* Create an instance of {@link PartyType }
*
* @return
* The created PartyType object and never null
.
*/
@Nonnull
public PartyType createPartyType() {
return new PartyType();
}
/**
* Create an instance of {@link PayloadType }
*
* @return
* The created PayloadType object and never null
.
*/
@Nonnull
public PayloadType createPayloadType() {
return new PayloadType();
}
/**
* Create an instance of {@link PayloadContentType }
*
* @return
* The created PayloadContentType object and never null
.
*/
@Nonnull
public PayloadContentType createPayloadContentType() {
return new PayloadContentType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExternalReferenceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ExternalReferenceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", name = "ExternalReference")
@Nonnull
public JAXBElement createExternalReference(
@Nullable
final ExternalReferenceType value) {
return new JAXBElement(_ExternalReference_QNAME, ExternalReferenceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PartyType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link PartyType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", name = "FromParty")
@Nonnull
public JAXBElement createFromParty(
@Nullable
final PartyType value) {
return new JAXBElement(_FromParty_QNAME, PartyType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExternalReferenceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ExternalReferenceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", name = "InstanceDecryptionInformationExternalReference")
@Nonnull
public JAXBElement createInstanceDecryptionInformationExternalReference(
@Nullable
final ExternalReferenceType value) {
return new JAXBElement(_InstanceDecryptionInformationExternalReference_QNAME, ExternalReferenceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExternalReferenceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ExternalReferenceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", name = "InstanceDecryptionKeyExternalReference")
@Nonnull
public JAXBElement createInstanceDecryptionKeyExternalReference(
@Nullable
final ExternalReferenceType value) {
return new JAXBElement(_InstanceDecryptionKeyExternalReference_QNAME, ExternalReferenceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PartyType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link PartyType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", name = "Party")
@Nonnull
public JAXBElement createParty(
@Nullable
final PartyType value) {
return new JAXBElement(_Party_QNAME, PartyType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PayloadType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link PayloadType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", name = "Payload")
@Nonnull
public JAXBElement createPayload(
@Nullable
final PayloadType value) {
return new JAXBElement(_Payload_QNAME, PayloadType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExternalReferenceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ExternalReferenceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", name = "PayloadExternalReference")
@Nonnull
public JAXBElement createPayloadExternalReference(
@Nullable
final ExternalReferenceType value) {
return new JAXBElement(_PayloadExternalReference_QNAME, ExternalReferenceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExternalReferenceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ExternalReferenceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", name = "RelevantExternalReference")
@Nonnull
public JAXBElement createRelevantExternalReference(
@Nullable
final ExternalReferenceType value) {
return new JAXBElement(_RelevantExternalReference_QNAME, ExternalReferenceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PartyType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link PartyType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/bdxr/ns/bde/1.0/AggregateComponents", name = "ToParty")
@Nonnull
public JAXBElement createToParty(
@Nullable
final PartyType value) {
return new JAXBElement(_ToParty_QNAME, PartyType.class, null, value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy