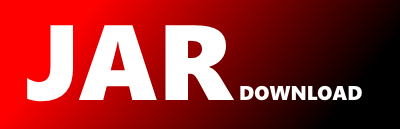
com.helger.ebinterface.v30.ObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-ebinterface Show documentation
Show all versions of ph-ebinterface Show documentation
ebInterface wrapper library to easily read and write ebInterface documents
package com.helger.ebinterface.v30;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.time.LocalDate;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
import com.helger.jaxb.adapter.AdapterLocalDate;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.helger.ebinterface.v30 package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _Invoice_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Invoice");
public final static QName _AdditionalInformation_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "AdditionalInformation");
public final static QName _Address_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Address");
public final static QName _AddressExtension_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "AddressExtension");
public final static QName _AlternativeQuantity_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "AlternativeQuantity");
public final static QName _Amount_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Amount");
public final static QName _BankAccountNr_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "BankAccountNr");
public final static QName _BankAccountOwner_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "BankAccountOwner");
public final static QName _BankCode_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "BankCode");
public final static QName _BankName_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "BankName");
public final static QName _BaseAmount_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "BaseAmount");
public final static QName _BeneficiaryAccount_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "BeneficiaryAccount");
public final static QName _BIC_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "BIC");
public final static QName _Biller_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Biller");
public final static QName _BillersOrderReference_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "BillersOrderReference");
public final static QName _BillersArticleNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "BillersArticleNumber");
public final static QName _BillersInvoiceRecipientID_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "BillersInvoiceRecipientID");
public final static QName _BillersOrderingPartyID_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "BillersOrderingPartyID");
public final static QName _Boxes_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Boxes");
public final static QName _ChargeNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "ChargeNumber");
public final static QName _Classification_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Classification");
public final static QName _Color_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Color");
public final static QName _Comment_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Comment");
public final static QName _ConsolidatorsBillerID_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "ConsolidatorsBillerID");
public final static QName _Contact_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Contact");
public final static QName _Country_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Country");
public final static QName _Custom_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Custom");
public final static QName _Date_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Date");
public final static QName _Delivery_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Delivery");
public final static QName _DeliveryID_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "DeliveryID");
public final static QName _Description_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Description");
public final static QName _Details_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Details");
public final static QName _DirectDebit_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "DirectDebit");
public final static QName _DiscountFlag_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "DiscountFlag");
public final static QName _Discount_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Discount");
public final static QName _DueDate_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "DueDate");
public final static QName _Email_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Email");
public final static QName _FooterDescription_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "FooterDescription");
public final static QName _FromDate_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "FromDate");
public final static QName _FurtherIdentification_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "FurtherIdentification");
public final static QName _HeaderDescription_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "HeaderDescription");
public final static QName _IBAN_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "IBAN");
public final static QName _OrderID_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "OrderID");
public final static QName _InvoiceDate_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "InvoiceDate");
public final static QName _InvoiceNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "InvoiceNumber");
public final static QName _InvoiceRecipient_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "InvoiceRecipient");
public final static QName _InvoiceRecipientsArticleNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "InvoiceRecipientsArticleNumber");
public final static QName _InvoiceRecipientsBillerID_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "InvoiceRecipientsBillerID");
public final static QName _InvoiceRecipientsOrderReference_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "InvoiceRecipientsOrderReference");
public final static QName _Item_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Item");
public final static QName _ItemList_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "ItemList");
public final static QName _LayoutID_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "LayoutID");
public final static QName _LineItemAmount_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "LineItemAmount");
public final static QName _ListLineItem_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "ListLineItem");
public final static QName _LogoURL_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "LogoURL");
public final static QName _MinimumPayment_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "MinimumPayment");
public final static QName _Name_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Name");
public final static QName _NoPayment_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "NoPayment");
public final static QName _OrderingParty_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "OrderingParty");
public final static QName _OrderPositionNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "OrderPositionNumber");
public final static QName _OrderReference_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "OrderReference");
public final static QName _OtherTax_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "OtherTax");
public final static QName _PaymentConditions_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "PaymentConditions");
public final static QName _PaymentDate_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "PaymentDate");
public final static QName _PaymentMethod_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "PaymentMethod");
public final static QName _PaymentReference_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "PaymentReference");
public final static QName _Percentage_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Percentage");
public final static QName _Period_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Period");
public final static QName _Phone_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Phone");
public final static QName _POBox_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "POBox");
public final static QName _PositionNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "PositionNumber");
public final static QName _PresentationDetails_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "PresentationDetails");
public final static QName _Quantity_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Quantity");
public final static QName _Reduction_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Reduction");
public final static QName _ReductionDetails_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "ReductionDetails");
public final static QName _ReductionRate_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "ReductionRate");
public final static QName _ReferenceDate_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "ReferenceDate");
public final static QName _Salutation_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Salutation");
public final static QName _SerialNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "SerialNumber");
public final static QName _Size_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Size");
public final static QName _Street_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Street");
public final static QName _SuppressZero_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "SuppressZero");
public final static QName _Tax_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Tax");
public final static QName _TaxExemption_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "TaxExemption");
public final static QName _TaxedAmount_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "TaxedAmount");
public final static QName _TaxRate_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "TaxRate");
public final static QName _ToDate_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "ToDate");
public final static QName _TotalGrossAmount_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "TotalGrossAmount");
public final static QName _Town_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Town");
public final static QName _UnitPrice_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "UnitPrice");
public final static QName _URL_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "URL");
public final static QName _UniversalBankTransaction_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "UniversalBankTransaction");
public final static QName _VAT_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "VAT");
public final static QName _VATIdentificationNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "VATIdentificationNumber");
public final static QName _Weight_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "Weight");
public final static QName _ZIP_QNAME = new QName("http://www.ebinterface.at/schema/3p0/", "ZIP");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.helger.ebinterface.v30
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link Ebi30InvoiceType }
*
* @return
* The created Ebi30InvoiceType object and never null
.
*/
@Nonnull
public Ebi30InvoiceType createEbi30InvoiceType() {
return new Ebi30InvoiceType();
}
/**
* Create an instance of {@link Ebi30AdditionalInformationType }
*
* @return
* The created Ebi30AdditionalInformationType object and never null
.
*/
@Nonnull
public Ebi30AdditionalInformationType createEbi30AdditionalInformationType() {
return new Ebi30AdditionalInformationType();
}
/**
* Create an instance of {@link Ebi30AddressType }
*
* @return
* The created Ebi30AddressType object and never null
.
*/
@Nonnull
public Ebi30AddressType createEbi30AddressType() {
return new Ebi30AddressType();
}
/**
* Create an instance of {@link Ebi30UnitType }
*
* @return
* The created Ebi30UnitType object and never null
.
*/
@Nonnull
public Ebi30UnitType createEbi30UnitType() {
return new Ebi30UnitType();
}
/**
* Create an instance of {@link Ebi30BankCodeCType }
*
* @return
* The created Ebi30BankCodeCType object and never null
.
*/
@Nonnull
public Ebi30BankCodeCType createEbi30BankCodeCType() {
return new Ebi30BankCodeCType();
}
/**
* Create an instance of {@link Ebi30AccountType }
*
* @return
* The created Ebi30AccountType object and never null
.
*/
@Nonnull
public Ebi30AccountType createEbi30AccountType() {
return new Ebi30AccountType();
}
/**
* Create an instance of {@link Ebi30BillerType }
*
* @return
* The created Ebi30BillerType object and never null
.
*/
@Nonnull
public Ebi30BillerType createEbi30BillerType() {
return new Ebi30BillerType();
}
/**
* Create an instance of {@link Ebi30OrderReferenceDetailType }
*
* @return
* The created Ebi30OrderReferenceDetailType object and never null
.
*/
@Nonnull
public Ebi30OrderReferenceDetailType createEbi30OrderReferenceDetailType() {
return new Ebi30OrderReferenceDetailType();
}
/**
* Create an instance of {@link Ebi30ClassificationType }
*
* @return
* The created Ebi30ClassificationType object and never null
.
*/
@Nonnull
public Ebi30ClassificationType createEbi30ClassificationType() {
return new Ebi30ClassificationType();
}
/**
* Create an instance of {@link Ebi30CustomType }
*
* @return
* The created Ebi30CustomType object and never null
.
*/
@Nonnull
public Ebi30CustomType createEbi30CustomType() {
return new Ebi30CustomType();
}
/**
* Create an instance of {@link Ebi30DeliveryType }
*
* @return
* The created Ebi30DeliveryType object and never null
.
*/
@Nonnull
public Ebi30DeliveryType createEbi30DeliveryType() {
return new Ebi30DeliveryType();
}
/**
* Create an instance of {@link Ebi30DetailsType }
*
* @return
* The created Ebi30DetailsType object and never null
.
*/
@Nonnull
public Ebi30DetailsType createEbi30DetailsType() {
return new Ebi30DetailsType();
}
/**
* Create an instance of {@link Ebi30DirectDebitType }
*
* @return
* The created Ebi30DirectDebitType object and never null
.
*/
@Nonnull
public Ebi30DirectDebitType createEbi30DirectDebitType() {
return new Ebi30DirectDebitType();
}
/**
* Create an instance of {@link Ebi30DiscountType }
*
* @return
* The created Ebi30DiscountType object and never null
.
*/
@Nonnull
public Ebi30DiscountType createEbi30DiscountType() {
return new Ebi30DiscountType();
}
/**
* Create an instance of {@link Ebi30FurtherIdentificationType }
*
* @return
* The created Ebi30FurtherIdentificationType object and never null
.
*/
@Nonnull
public Ebi30FurtherIdentificationType createEbi30FurtherIdentificationType() {
return new Ebi30FurtherIdentificationType();
}
/**
* Create an instance of {@link Ebi30InvoiceRecipientType }
*
* @return
* The created Ebi30InvoiceRecipientType object and never null
.
*/
@Nonnull
public Ebi30InvoiceRecipientType createEbi30InvoiceRecipientType() {
return new Ebi30InvoiceRecipientType();
}
/**
* Create an instance of {@link Ebi30ItemType }
*
* @return
* The created Ebi30ItemType object and never null
.
*/
@Nonnull
public Ebi30ItemType createEbi30ItemType() {
return new Ebi30ItemType();
}
/**
* Create an instance of {@link Ebi30ItemListType }
*
* @return
* The created Ebi30ItemListType object and never null
.
*/
@Nonnull
public Ebi30ItemListType createEbi30ItemListType() {
return new Ebi30ItemListType();
}
/**
* Create an instance of {@link Ebi30ListLineItemType }
*
* @return
* The created Ebi30ListLineItemType object and never null
.
*/
@Nonnull
public Ebi30ListLineItemType createEbi30ListLineItemType() {
return new Ebi30ListLineItemType();
}
/**
* Create an instance of {@link Ebi30NoPaymentType }
*
* @return
* The created Ebi30NoPaymentType object and never null
.
*/
@Nonnull
public Ebi30NoPaymentType createEbi30NoPaymentType() {
return new Ebi30NoPaymentType();
}
/**
* Create an instance of {@link Ebi30OrderingPartyType }
*
* @return
* The created Ebi30OrderingPartyType object and never null
.
*/
@Nonnull
public Ebi30OrderingPartyType createEbi30OrderingPartyType() {
return new Ebi30OrderingPartyType();
}
/**
* Create an instance of {@link Ebi30OrderReferenceType }
*
* @return
* The created Ebi30OrderReferenceType object and never null
.
*/
@Nonnull
public Ebi30OrderReferenceType createEbi30OrderReferenceType() {
return new Ebi30OrderReferenceType();
}
/**
* Create an instance of {@link Ebi30OtherTaxType }
*
* @return
* The created Ebi30OtherTaxType object and never null
.
*/
@Nonnull
public Ebi30OtherTaxType createEbi30OtherTaxType() {
return new Ebi30OtherTaxType();
}
/**
* Create an instance of {@link Ebi30PaymentConditionsType }
*
* @return
* The created Ebi30PaymentConditionsType object and never null
.
*/
@Nonnull
public Ebi30PaymentConditionsType createEbi30PaymentConditionsType() {
return new Ebi30PaymentConditionsType();
}
/**
* Create an instance of {@link Ebi30PaymentMethodType }
*
* @return
* The created Ebi30PaymentMethodType object and never null
.
*/
@Nonnull
public Ebi30PaymentMethodType createEbi30PaymentMethodType() {
return new Ebi30PaymentMethodType();
}
/**
* Create an instance of {@link Ebi30PeriodType }
*
* @return
* The created Ebi30PeriodType object and never null
.
*/
@Nonnull
public Ebi30PeriodType createEbi30PeriodType() {
return new Ebi30PeriodType();
}
/**
* Create an instance of {@link Ebi30PresentationDetailsType }
*
* @return
* The created Ebi30PresentationDetailsType object and never null
.
*/
@Nonnull
public Ebi30PresentationDetailsType createEbi30PresentationDetailsType() {
return new Ebi30PresentationDetailsType();
}
/**
* Create an instance of {@link Ebi30ReductionType }
*
* @return
* The created Ebi30ReductionType object and never null
.
*/
@Nonnull
public Ebi30ReductionType createEbi30ReductionType() {
return new Ebi30ReductionType();
}
/**
* Create an instance of {@link Ebi30ReductionDetailsType }
*
* @return
* The created Ebi30ReductionDetailsType object and never null
.
*/
@Nonnull
public Ebi30ReductionDetailsType createEbi30ReductionDetailsType() {
return new Ebi30ReductionDetailsType();
}
/**
* Create an instance of {@link Ebi30TaxType }
*
* @return
* The created Ebi30TaxType object and never null
.
*/
@Nonnull
public Ebi30TaxType createEbi30TaxType() {
return new Ebi30TaxType();
}
/**
* Create an instance of {@link Ebi30TaxRateType }
*
* @return
* The created Ebi30TaxRateType object and never null
.
*/
@Nonnull
public Ebi30TaxRateType createEbi30TaxRateType() {
return new Ebi30TaxRateType();
}
/**
* Create an instance of {@link Ebi30UniversalBankTransactionType }
*
* @return
* The created Ebi30UniversalBankTransactionType object and never null
.
*/
@Nonnull
public Ebi30UniversalBankTransactionType createEbi30UniversalBankTransactionType() {
return new Ebi30UniversalBankTransactionType();
}
/**
* Create an instance of {@link Ebi30VATType }
*
* @return
* The created Ebi30VATType object and never null
.
*/
@Nonnull
public Ebi30VATType createEbi30VATType() {
return new Ebi30VATType();
}
/**
* Create an instance of {@link Ebi30EmptyType }
*
* @return
* The created Ebi30EmptyType object and never null
.
*/
@Nonnull
public Ebi30EmptyType createEbi30EmptyType() {
return new Ebi30EmptyType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30InvoiceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30InvoiceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Invoice")
@Nonnull
public JAXBElement createInvoice(
@Nullable
final Ebi30InvoiceType value) {
return new JAXBElement(_Invoice_QNAME, Ebi30InvoiceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30AdditionalInformationType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30AdditionalInformationType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "AdditionalInformation")
@Nonnull
public JAXBElement createAdditionalInformation(
@Nullable
final Ebi30AdditionalInformationType value) {
return new JAXBElement(_AdditionalInformation_QNAME, Ebi30AdditionalInformationType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30AddressType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30AddressType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Address")
@Nonnull
public JAXBElement createAddress(
@Nullable
final Ebi30AddressType value) {
return new JAXBElement(_Address_QNAME, Ebi30AddressType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "AddressExtension")
@Nonnull
public JAXBElement createAddressExtension(
@Nullable
final String value) {
return new JAXBElement(_AddressExtension_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30UnitType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30UnitType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "AlternativeQuantity")
@Nonnull
public JAXBElement createAlternativeQuantity(
@Nullable
final Ebi30UnitType value) {
return new JAXBElement(_AlternativeQuantity_QNAME, Ebi30UnitType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Amount")
@Nonnull
public JAXBElement createAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_Amount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "BankAccountNr")
@Nonnull
public JAXBElement createBankAccountNr(
@Nullable
final String value) {
return new JAXBElement(_BankAccountNr_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "BankAccountOwner")
@Nonnull
public JAXBElement createBankAccountOwner(
@Nullable
final String value) {
return new JAXBElement(_BankAccountOwner_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30BankCodeCType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30BankCodeCType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "BankCode")
@Nonnull
public JAXBElement createBankCode(
@Nullable
final Ebi30BankCodeCType value) {
return new JAXBElement(_BankCode_QNAME, Ebi30BankCodeCType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "BankName")
@Nonnull
public JAXBElement createBankName(
@Nullable
final String value) {
return new JAXBElement(_BankName_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "BaseAmount")
@Nonnull
public JAXBElement createBaseAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_BaseAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30AccountType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30AccountType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "BeneficiaryAccount")
@Nonnull
public JAXBElement createBeneficiaryAccount(
@Nullable
final Ebi30AccountType value) {
return new JAXBElement(_BeneficiaryAccount_QNAME, Ebi30AccountType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "BIC")
@Nonnull
public JAXBElement createBIC(
@Nullable
final String value) {
return new JAXBElement(_BIC_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30BillerType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30BillerType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Biller")
@Nonnull
public JAXBElement createBiller(
@Nullable
final Ebi30BillerType value) {
return new JAXBElement(_Biller_QNAME, Ebi30BillerType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30OrderReferenceDetailType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30OrderReferenceDetailType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "BillersOrderReference")
@Nonnull
public JAXBElement createBillersOrderReference(
@Nullable
final Ebi30OrderReferenceDetailType value) {
return new JAXBElement(_BillersOrderReference_QNAME, Ebi30OrderReferenceDetailType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "BillersArticleNumber")
@Nonnull
public JAXBElement createBillersArticleNumber(
@Nullable
final String value) {
return new JAXBElement(_BillersArticleNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "BillersInvoiceRecipientID")
@Nonnull
public JAXBElement createBillersInvoiceRecipientID(
@Nullable
final String value) {
return new JAXBElement(_BillersInvoiceRecipientID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "BillersOrderingPartyID")
@Nonnull
public JAXBElement createBillersOrderingPartyID(
@Nullable
final String value) {
return new JAXBElement(_BillersOrderingPartyID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Boxes")
@Nonnull
public JAXBElement createBoxes(
@Nullable
final BigInteger value) {
return new JAXBElement(_Boxes_QNAME, BigInteger.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "ChargeNumber")
@Nonnull
public JAXBElement createChargeNumber(
@Nullable
final String value) {
return new JAXBElement(_ChargeNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30ClassificationType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30ClassificationType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Classification")
@Nonnull
public JAXBElement createClassification(
@Nullable
final Ebi30ClassificationType value) {
return new JAXBElement(_Classification_QNAME, Ebi30ClassificationType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Color")
@Nonnull
public JAXBElement createColor(
@Nullable
final String value) {
return new JAXBElement(_Color_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Comment")
@Nonnull
public JAXBElement createComment(
@Nullable
final String value) {
return new JAXBElement(_Comment_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "ConsolidatorsBillerID")
@Nonnull
public JAXBElement createConsolidatorsBillerID(
@Nullable
final String value) {
return new JAXBElement(_ConsolidatorsBillerID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Contact")
@Nonnull
public JAXBElement createContact(
@Nullable
final String value) {
return new JAXBElement(_Contact_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Country")
@Nonnull
public JAXBElement createCountry(
@Nullable
final String value) {
return new JAXBElement(_Country_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30CustomType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30CustomType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Custom")
@Nonnull
public JAXBElement createCustom(
@Nullable
final Ebi30CustomType value) {
return new JAXBElement(_Custom_QNAME, Ebi30CustomType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Date")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_Date_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30DeliveryType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30DeliveryType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Delivery")
@Nonnull
public JAXBElement createDelivery(
@Nullable
final Ebi30DeliveryType value) {
return new JAXBElement(_Delivery_QNAME, Ebi30DeliveryType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "DeliveryID")
@Nonnull
public JAXBElement createDeliveryID(
@Nullable
final String value) {
return new JAXBElement(_DeliveryID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Description")
@Nonnull
public JAXBElement createDescription(
@Nullable
final String value) {
return new JAXBElement(_Description_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30DetailsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30DetailsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Details")
@Nonnull
public JAXBElement createDetails(
@Nullable
final Ebi30DetailsType value) {
return new JAXBElement(_Details_QNAME, Ebi30DetailsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30DirectDebitType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30DirectDebitType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "DirectDebit")
@Nonnull
public JAXBElement createDirectDebit(
@Nullable
final Ebi30DirectDebitType value) {
return new JAXBElement(_DirectDebit_QNAME, Ebi30DirectDebitType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Boolean }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Boolean }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "DiscountFlag")
@Nonnull
public JAXBElement createDiscountFlag(
@Nullable
final Boolean value) {
return new JAXBElement(_DiscountFlag_QNAME, Boolean.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30DiscountType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30DiscountType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Discount")
@Nonnull
public JAXBElement createDiscount(
@Nullable
final Ebi30DiscountType value) {
return new JAXBElement(_Discount_QNAME, Ebi30DiscountType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "DueDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createDueDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_DueDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Email")
@Nonnull
public JAXBElement createEmail(
@Nullable
final String value) {
return new JAXBElement(_Email_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "FooterDescription")
@Nonnull
public JAXBElement createFooterDescription(
@Nullable
final String value) {
return new JAXBElement(_FooterDescription_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "FromDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createFromDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_FromDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30FurtherIdentificationType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30FurtherIdentificationType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "FurtherIdentification")
@Nonnull
public JAXBElement createFurtherIdentification(
@Nullable
final Ebi30FurtherIdentificationType value) {
return new JAXBElement(_FurtherIdentification_QNAME, Ebi30FurtherIdentificationType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "HeaderDescription")
@Nonnull
public JAXBElement createHeaderDescription(
@Nullable
final String value) {
return new JAXBElement(_HeaderDescription_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "IBAN")
@Nonnull
public JAXBElement createIBAN(
@Nullable
final String value) {
return new JAXBElement(_IBAN_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "OrderID")
@Nonnull
public JAXBElement createOrderID(
@Nullable
final String value) {
return new JAXBElement(_OrderID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "InvoiceDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createInvoiceDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_InvoiceDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "InvoiceNumber")
@Nonnull
public JAXBElement createInvoiceNumber(
@Nullable
final String value) {
return new JAXBElement(_InvoiceNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30InvoiceRecipientType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30InvoiceRecipientType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "InvoiceRecipient")
@Nonnull
public JAXBElement createInvoiceRecipient(
@Nullable
final Ebi30InvoiceRecipientType value) {
return new JAXBElement(_InvoiceRecipient_QNAME, Ebi30InvoiceRecipientType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "InvoiceRecipientsArticleNumber")
@Nonnull
public JAXBElement createInvoiceRecipientsArticleNumber(
@Nullable
final String value) {
return new JAXBElement(_InvoiceRecipientsArticleNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "InvoiceRecipientsBillerID")
@Nonnull
public JAXBElement createInvoiceRecipientsBillerID(
@Nullable
final String value) {
return new JAXBElement(_InvoiceRecipientsBillerID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30OrderReferenceDetailType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30OrderReferenceDetailType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "InvoiceRecipientsOrderReference")
@Nonnull
public JAXBElement createInvoiceRecipientsOrderReference(
@Nullable
final Ebi30OrderReferenceDetailType value) {
return new JAXBElement(_InvoiceRecipientsOrderReference_QNAME, Ebi30OrderReferenceDetailType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30ItemType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30ItemType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Item")
@Nonnull
public JAXBElement createItem(
@Nullable
final Ebi30ItemType value) {
return new JAXBElement(_Item_QNAME, Ebi30ItemType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30ItemListType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30ItemListType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "ItemList")
@Nonnull
public JAXBElement createItemList(
@Nullable
final Ebi30ItemListType value) {
return new JAXBElement(_ItemList_QNAME, Ebi30ItemListType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "LayoutID")
@Nonnull
public JAXBElement createLayoutID(
@Nullable
final String value) {
return new JAXBElement(_LayoutID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "LineItemAmount")
@Nonnull
public JAXBElement createLineItemAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_LineItemAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30ListLineItemType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30ListLineItemType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "ListLineItem")
@Nonnull
public JAXBElement createListLineItem(
@Nullable
final Ebi30ListLineItemType value) {
return new JAXBElement(_ListLineItem_QNAME, Ebi30ListLineItemType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "LogoURL")
@Nonnull
public JAXBElement createLogoURL(
@Nullable
final String value) {
return new JAXBElement(_LogoURL_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "MinimumPayment")
@Nonnull
public JAXBElement createMinimumPayment(
@Nullable
final BigDecimal value) {
return new JAXBElement(_MinimumPayment_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Name")
@Nonnull
public JAXBElement createName(
@Nullable
final String value) {
return new JAXBElement(_Name_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30NoPaymentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30NoPaymentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "NoPayment")
@Nonnull
public JAXBElement createNoPayment(
@Nullable
final Ebi30NoPaymentType value) {
return new JAXBElement(_NoPayment_QNAME, Ebi30NoPaymentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30OrderingPartyType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30OrderingPartyType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "OrderingParty")
@Nonnull
public JAXBElement createOrderingParty(
@Nullable
final Ebi30OrderingPartyType value) {
return new JAXBElement(_OrderingParty_QNAME, Ebi30OrderingPartyType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "OrderPositionNumber")
@Nonnull
public JAXBElement createOrderPositionNumber(
@Nullable
final String value) {
return new JAXBElement(_OrderPositionNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30OrderReferenceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30OrderReferenceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "OrderReference")
@Nonnull
public JAXBElement createOrderReference(
@Nullable
final Ebi30OrderReferenceType value) {
return new JAXBElement(_OrderReference_QNAME, Ebi30OrderReferenceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30OtherTaxType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30OtherTaxType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "OtherTax")
@Nonnull
public JAXBElement createOtherTax(
@Nullable
final Ebi30OtherTaxType value) {
return new JAXBElement(_OtherTax_QNAME, Ebi30OtherTaxType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30PaymentConditionsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30PaymentConditionsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "PaymentConditions")
@Nonnull
public JAXBElement createPaymentConditions(
@Nullable
final Ebi30PaymentConditionsType value) {
return new JAXBElement(_PaymentConditions_QNAME, Ebi30PaymentConditionsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "PaymentDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createPaymentDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_PaymentDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30PaymentMethodType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30PaymentMethodType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "PaymentMethod")
@Nonnull
public JAXBElement createPaymentMethod(
@Nullable
final Ebi30PaymentMethodType value) {
return new JAXBElement(_PaymentMethod_QNAME, Ebi30PaymentMethodType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "PaymentReference")
@Nonnull
public JAXBElement createPaymentReference(
@Nullable
final BigInteger value) {
return new JAXBElement(_PaymentReference_QNAME, BigInteger.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Percentage")
@Nonnull
public JAXBElement createPercentage(
@Nullable
final BigDecimal value) {
return new JAXBElement(_Percentage_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30PeriodType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30PeriodType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Period")
@Nonnull
public JAXBElement createPeriod(
@Nullable
final Ebi30PeriodType value) {
return new JAXBElement(_Period_QNAME, Ebi30PeriodType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Phone")
@Nonnull
public JAXBElement createPhone(
@Nullable
final String value) {
return new JAXBElement(_Phone_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "POBox")
@Nonnull
public JAXBElement createPOBox(
@Nullable
final String value) {
return new JAXBElement(_POBox_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "PositionNumber")
@Nonnull
public JAXBElement createPositionNumber(
@Nullable
final BigInteger value) {
return new JAXBElement(_PositionNumber_QNAME, BigInteger.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30PresentationDetailsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30PresentationDetailsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "PresentationDetails")
@Nonnull
public JAXBElement createPresentationDetails(
@Nullable
final Ebi30PresentationDetailsType value) {
return new JAXBElement(_PresentationDetails_QNAME, Ebi30PresentationDetailsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30UnitType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30UnitType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Quantity")
@Nonnull
public JAXBElement createQuantity(
@Nullable
final Ebi30UnitType value) {
return new JAXBElement(_Quantity_QNAME, Ebi30UnitType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30ReductionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30ReductionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Reduction")
@Nonnull
public JAXBElement createReduction(
@Nullable
final Ebi30ReductionType value) {
return new JAXBElement(_Reduction_QNAME, Ebi30ReductionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30ReductionDetailsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30ReductionDetailsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "ReductionDetails")
@Nonnull
public JAXBElement createReductionDetails(
@Nullable
final Ebi30ReductionDetailsType value) {
return new JAXBElement(_ReductionDetails_QNAME, Ebi30ReductionDetailsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "ReductionRate")
@Nonnull
public JAXBElement createReductionRate(
@Nullable
final BigDecimal value) {
return new JAXBElement(_ReductionRate_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "ReferenceDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createReferenceDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_ReferenceDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Salutation")
@Nonnull
public JAXBElement createSalutation(
@Nullable
final String value) {
return new JAXBElement(_Salutation_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "SerialNumber")
@Nonnull
public JAXBElement createSerialNumber(
@Nullable
final String value) {
return new JAXBElement(_SerialNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Size")
@Nonnull
public JAXBElement createSize(
@Nullable
final String value) {
return new JAXBElement(_Size_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Street")
@Nonnull
public JAXBElement createStreet(
@Nullable
final String value) {
return new JAXBElement(_Street_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Boolean }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Boolean }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "SuppressZero")
@Nonnull
public JAXBElement createSuppressZero(
@Nullable
final Boolean value) {
return new JAXBElement(_SuppressZero_QNAME, Boolean.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30TaxType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30TaxType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Tax")
@Nonnull
public JAXBElement createTax(
@Nullable
final Ebi30TaxType value) {
return new JAXBElement(_Tax_QNAME, Ebi30TaxType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "TaxExemption")
@Nonnull
public JAXBElement createTaxExemption(
@Nullable
final String value) {
return new JAXBElement(_TaxExemption_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "TaxedAmount")
@Nonnull
public JAXBElement createTaxedAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_TaxedAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30TaxRateType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30TaxRateType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "TaxRate")
@Nonnull
public JAXBElement createTaxRate(
@Nullable
final Ebi30TaxRateType value) {
return new JAXBElement(_TaxRate_QNAME, Ebi30TaxRateType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "ToDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createToDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_ToDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "TotalGrossAmount")
@Nonnull
public JAXBElement createTotalGrossAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_TotalGrossAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Town")
@Nonnull
public JAXBElement createTown(
@Nullable
final String value) {
return new JAXBElement(_Town_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "UnitPrice")
@Nonnull
public JAXBElement createUnitPrice(
@Nullable
final BigDecimal value) {
return new JAXBElement(_UnitPrice_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "URL")
@Nonnull
public JAXBElement createURL(
@Nullable
final String value) {
return new JAXBElement(_URL_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30UniversalBankTransactionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30UniversalBankTransactionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "UniversalBankTransaction")
@Nonnull
public JAXBElement createUniversalBankTransaction(
@Nullable
final Ebi30UniversalBankTransactionType value) {
return new JAXBElement(_UniversalBankTransaction_QNAME, Ebi30UniversalBankTransactionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30VATType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30VATType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "VAT")
@Nonnull
public JAXBElement createVAT(
@Nullable
final Ebi30VATType value) {
return new JAXBElement(_VAT_QNAME, Ebi30VATType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "VATIdentificationNumber")
@Nonnull
public JAXBElement createVATIdentificationNumber(
@Nullable
final String value) {
return new JAXBElement(_VATIdentificationNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi30UnitType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi30UnitType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "Weight")
@Nonnull
public JAXBElement createWeight(
@Nullable
final Ebi30UnitType value) {
return new JAXBElement(_Weight_QNAME, Ebi30UnitType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p0/", name = "ZIP")
@Nonnull
public JAXBElement createZIP(
@Nullable
final String value) {
return new JAXBElement(_ZIP_QNAME, String.class, null, value);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy