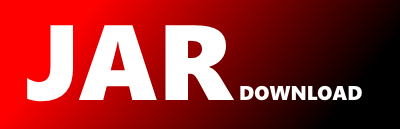
com.helger.ebinterface.v302.ObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-ebinterface Show documentation
Show all versions of ph-ebinterface Show documentation
ebInterface wrapper library to easily read and write ebInterface documents
package com.helger.ebinterface.v302;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.time.LocalDate;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
import com.helger.jaxb.adapter.AdapterLocalDate;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.helger.ebinterface.v302 package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _Invoice_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Invoice");
public final static QName _AdditionalInformation_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "AdditionalInformation");
public final static QName _Address_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Address");
public final static QName _AddressExtension_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "AddressExtension");
public final static QName _AlternativeQuantity_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "AlternativeQuantity");
public final static QName _Amount_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Amount");
public final static QName _BankAccountNr_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "BankAccountNr");
public final static QName _BankAccountOwner_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "BankAccountOwner");
public final static QName _BankCode_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "BankCode");
public final static QName _BankName_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "BankName");
public final static QName _BaseAmount_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "BaseAmount");
public final static QName _BeneficiaryAccount_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "BeneficiaryAccount");
public final static QName _BIC_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "BIC");
public final static QName _Biller_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Biller");
public final static QName _BillersOrderReference_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "BillersOrderReference");
public final static QName _BillersArticleNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "BillersArticleNumber");
public final static QName _BillersInvoiceRecipientID_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "BillersInvoiceRecipientID");
public final static QName _BillersOrderingPartyID_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "BillersOrderingPartyID");
public final static QName _Boxes_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Boxes");
public final static QName _ChargeNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "ChargeNumber");
public final static QName _Classification_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Classification");
public final static QName _Color_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Color");
public final static QName _Comment_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Comment");
public final static QName _ConsolidatorsBillerID_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "ConsolidatorsBillerID");
public final static QName _Contact_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Contact");
public final static QName _Country_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Country");
public final static QName _Custom_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Custom");
public final static QName _Date_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Date");
public final static QName _Delivery_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Delivery");
public final static QName _DeliveryID_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "DeliveryID");
public final static QName _Description_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Description");
public final static QName _Details_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Details");
public final static QName _DirectDebit_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "DirectDebit");
public final static QName _DiscountFlag_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "DiscountFlag");
public final static QName _Discount_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Discount");
public final static QName _DueDate_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "DueDate");
public final static QName _Email_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Email");
public final static QName _FooterDescription_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "FooterDescription");
public final static QName _FromDate_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "FromDate");
public final static QName _FurtherIdentification_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "FurtherIdentification");
public final static QName _HeaderDescription_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "HeaderDescription");
public final static QName _IBAN_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "IBAN");
public final static QName _OrderID_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "OrderID");
public final static QName _InvoiceDate_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "InvoiceDate");
public final static QName _InvoiceNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "InvoiceNumber");
public final static QName _InvoiceRecipient_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "InvoiceRecipient");
public final static QName _InvoiceRecipientsArticleNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "InvoiceRecipientsArticleNumber");
public final static QName _InvoiceRecipientsBillerID_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "InvoiceRecipientsBillerID");
public final static QName _InvoiceRecipientsOrderReference_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "InvoiceRecipientsOrderReference");
public final static QName _Item_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Item");
public final static QName _ItemList_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "ItemList");
public final static QName _LayoutID_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "LayoutID");
public final static QName _LineItemAmount_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "LineItemAmount");
public final static QName _ListLineItem_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "ListLineItem");
public final static QName _LogoURL_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "LogoURL");
public final static QName _MinimumPayment_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "MinimumPayment");
public final static QName _Name_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Name");
public final static QName _NoPayment_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "NoPayment");
public final static QName _OrderingParty_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "OrderingParty");
public final static QName _OrderPositionNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "OrderPositionNumber");
public final static QName _OrderReference_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "OrderReference");
public final static QName _OtherTax_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "OtherTax");
public final static QName _PaymentConditions_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "PaymentConditions");
public final static QName _PaymentDate_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "PaymentDate");
public final static QName _PaymentMethod_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "PaymentMethod");
public final static QName _PaymentReference_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "PaymentReference");
public final static QName _Percentage_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Percentage");
public final static QName _Period_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Period");
public final static QName _Phone_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Phone");
public final static QName _POBox_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "POBox");
public final static QName _PositionNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "PositionNumber");
public final static QName _PresentationDetails_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "PresentationDetails");
public final static QName _Quantity_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Quantity");
public final static QName _Reduction_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Reduction");
public final static QName _ReductionDetails_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "ReductionDetails");
public final static QName _ReductionRate_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "ReductionRate");
public final static QName _ReferenceDate_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "ReferenceDate");
public final static QName _Salutation_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Salutation");
public final static QName _SerialNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "SerialNumber");
public final static QName _Size_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Size");
public final static QName _Street_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Street");
public final static QName _SuppressZero_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "SuppressZero");
public final static QName _Tax_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Tax");
public final static QName _TaxExemption_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "TaxExemption");
public final static QName _TaxedAmount_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "TaxedAmount");
public final static QName _TaxRate_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "TaxRate");
public final static QName _ToDate_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "ToDate");
public final static QName _TotalGrossAmount_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "TotalGrossAmount");
public final static QName _Town_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Town");
public final static QName _UnitPrice_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "UnitPrice");
public final static QName _URL_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "URL");
public final static QName _UniversalBankTransaction_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "UniversalBankTransaction");
public final static QName _VAT_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "VAT");
public final static QName _VATIdentificationNumber_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "VATIdentificationNumber");
public final static QName _Weight_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "Weight");
public final static QName _ZIP_QNAME = new QName("http://www.ebinterface.at/schema/3p02/", "ZIP");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.helger.ebinterface.v302
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link Ebi302InvoiceType }
*
* @return
* The created Ebi302InvoiceType object and never null
.
*/
@Nonnull
public Ebi302InvoiceType createEbi302InvoiceType() {
return new Ebi302InvoiceType();
}
/**
* Create an instance of {@link Ebi302AdditionalInformationType }
*
* @return
* The created Ebi302AdditionalInformationType object and never null
.
*/
@Nonnull
public Ebi302AdditionalInformationType createEbi302AdditionalInformationType() {
return new Ebi302AdditionalInformationType();
}
/**
* Create an instance of {@link Ebi302AddressType }
*
* @return
* The created Ebi302AddressType object and never null
.
*/
@Nonnull
public Ebi302AddressType createEbi302AddressType() {
return new Ebi302AddressType();
}
/**
* Create an instance of {@link Ebi302UnitType }
*
* @return
* The created Ebi302UnitType object and never null
.
*/
@Nonnull
public Ebi302UnitType createEbi302UnitType() {
return new Ebi302UnitType();
}
/**
* Create an instance of {@link Ebi302BankCodeCType }
*
* @return
* The created Ebi302BankCodeCType object and never null
.
*/
@Nonnull
public Ebi302BankCodeCType createEbi302BankCodeCType() {
return new Ebi302BankCodeCType();
}
/**
* Create an instance of {@link Ebi302AccountType }
*
* @return
* The created Ebi302AccountType object and never null
.
*/
@Nonnull
public Ebi302AccountType createEbi302AccountType() {
return new Ebi302AccountType();
}
/**
* Create an instance of {@link Ebi302BillerType }
*
* @return
* The created Ebi302BillerType object and never null
.
*/
@Nonnull
public Ebi302BillerType createEbi302BillerType() {
return new Ebi302BillerType();
}
/**
* Create an instance of {@link Ebi302OrderReferenceDetailType }
*
* @return
* The created Ebi302OrderReferenceDetailType object and never null
.
*/
@Nonnull
public Ebi302OrderReferenceDetailType createEbi302OrderReferenceDetailType() {
return new Ebi302OrderReferenceDetailType();
}
/**
* Create an instance of {@link Ebi302ClassificationType }
*
* @return
* The created Ebi302ClassificationType object and never null
.
*/
@Nonnull
public Ebi302ClassificationType createEbi302ClassificationType() {
return new Ebi302ClassificationType();
}
/**
* Create an instance of {@link Ebi302CustomType }
*
* @return
* The created Ebi302CustomType object and never null
.
*/
@Nonnull
public Ebi302CustomType createEbi302CustomType() {
return new Ebi302CustomType();
}
/**
* Create an instance of {@link Ebi302DeliveryType }
*
* @return
* The created Ebi302DeliveryType object and never null
.
*/
@Nonnull
public Ebi302DeliveryType createEbi302DeliveryType() {
return new Ebi302DeliveryType();
}
/**
* Create an instance of {@link Ebi302DetailsType }
*
* @return
* The created Ebi302DetailsType object and never null
.
*/
@Nonnull
public Ebi302DetailsType createEbi302DetailsType() {
return new Ebi302DetailsType();
}
/**
* Create an instance of {@link Ebi302DirectDebitType }
*
* @return
* The created Ebi302DirectDebitType object and never null
.
*/
@Nonnull
public Ebi302DirectDebitType createEbi302DirectDebitType() {
return new Ebi302DirectDebitType();
}
/**
* Create an instance of {@link Ebi302DiscountType }
*
* @return
* The created Ebi302DiscountType object and never null
.
*/
@Nonnull
public Ebi302DiscountType createEbi302DiscountType() {
return new Ebi302DiscountType();
}
/**
* Create an instance of {@link Ebi302FurtherIdentificationType }
*
* @return
* The created Ebi302FurtherIdentificationType object and never null
.
*/
@Nonnull
public Ebi302FurtherIdentificationType createEbi302FurtherIdentificationType() {
return new Ebi302FurtherIdentificationType();
}
/**
* Create an instance of {@link Ebi302InvoiceRecipientType }
*
* @return
* The created Ebi302InvoiceRecipientType object and never null
.
*/
@Nonnull
public Ebi302InvoiceRecipientType createEbi302InvoiceRecipientType() {
return new Ebi302InvoiceRecipientType();
}
/**
* Create an instance of {@link Ebi302ItemType }
*
* @return
* The created Ebi302ItemType object and never null
.
*/
@Nonnull
public Ebi302ItemType createEbi302ItemType() {
return new Ebi302ItemType();
}
/**
* Create an instance of {@link Ebi302ItemListType }
*
* @return
* The created Ebi302ItemListType object and never null
.
*/
@Nonnull
public Ebi302ItemListType createEbi302ItemListType() {
return new Ebi302ItemListType();
}
/**
* Create an instance of {@link Ebi302ListLineItemType }
*
* @return
* The created Ebi302ListLineItemType object and never null
.
*/
@Nonnull
public Ebi302ListLineItemType createEbi302ListLineItemType() {
return new Ebi302ListLineItemType();
}
/**
* Create an instance of {@link Ebi302NoPaymentType }
*
* @return
* The created Ebi302NoPaymentType object and never null
.
*/
@Nonnull
public Ebi302NoPaymentType createEbi302NoPaymentType() {
return new Ebi302NoPaymentType();
}
/**
* Create an instance of {@link Ebi302OrderingPartyType }
*
* @return
* The created Ebi302OrderingPartyType object and never null
.
*/
@Nonnull
public Ebi302OrderingPartyType createEbi302OrderingPartyType() {
return new Ebi302OrderingPartyType();
}
/**
* Create an instance of {@link Ebi302OrderReferenceType }
*
* @return
* The created Ebi302OrderReferenceType object and never null
.
*/
@Nonnull
public Ebi302OrderReferenceType createEbi302OrderReferenceType() {
return new Ebi302OrderReferenceType();
}
/**
* Create an instance of {@link Ebi302OtherTaxType }
*
* @return
* The created Ebi302OtherTaxType object and never null
.
*/
@Nonnull
public Ebi302OtherTaxType createEbi302OtherTaxType() {
return new Ebi302OtherTaxType();
}
/**
* Create an instance of {@link Ebi302PaymentConditionsType }
*
* @return
* The created Ebi302PaymentConditionsType object and never null
.
*/
@Nonnull
public Ebi302PaymentConditionsType createEbi302PaymentConditionsType() {
return new Ebi302PaymentConditionsType();
}
/**
* Create an instance of {@link Ebi302PaymentMethodType }
*
* @return
* The created Ebi302PaymentMethodType object and never null
.
*/
@Nonnull
public Ebi302PaymentMethodType createEbi302PaymentMethodType() {
return new Ebi302PaymentMethodType();
}
/**
* Create an instance of {@link Ebi302PeriodType }
*
* @return
* The created Ebi302PeriodType object and never null
.
*/
@Nonnull
public Ebi302PeriodType createEbi302PeriodType() {
return new Ebi302PeriodType();
}
/**
* Create an instance of {@link Ebi302PresentationDetailsType }
*
* @return
* The created Ebi302PresentationDetailsType object and never null
.
*/
@Nonnull
public Ebi302PresentationDetailsType createEbi302PresentationDetailsType() {
return new Ebi302PresentationDetailsType();
}
/**
* Create an instance of {@link Ebi302ReductionType }
*
* @return
* The created Ebi302ReductionType object and never null
.
*/
@Nonnull
public Ebi302ReductionType createEbi302ReductionType() {
return new Ebi302ReductionType();
}
/**
* Create an instance of {@link Ebi302ReductionDetailsType }
*
* @return
* The created Ebi302ReductionDetailsType object and never null
.
*/
@Nonnull
public Ebi302ReductionDetailsType createEbi302ReductionDetailsType() {
return new Ebi302ReductionDetailsType();
}
/**
* Create an instance of {@link Ebi302TaxType }
*
* @return
* The created Ebi302TaxType object and never null
.
*/
@Nonnull
public Ebi302TaxType createEbi302TaxType() {
return new Ebi302TaxType();
}
/**
* Create an instance of {@link Ebi302TaxRateType }
*
* @return
* The created Ebi302TaxRateType object and never null
.
*/
@Nonnull
public Ebi302TaxRateType createEbi302TaxRateType() {
return new Ebi302TaxRateType();
}
/**
* Create an instance of {@link Ebi302UniversalBankTransactionType }
*
* @return
* The created Ebi302UniversalBankTransactionType object and never null
.
*/
@Nonnull
public Ebi302UniversalBankTransactionType createEbi302UniversalBankTransactionType() {
return new Ebi302UniversalBankTransactionType();
}
/**
* Create an instance of {@link Ebi302VATType }
*
* @return
* The created Ebi302VATType object and never null
.
*/
@Nonnull
public Ebi302VATType createEbi302VATType() {
return new Ebi302VATType();
}
/**
* Create an instance of {@link Ebi302EmptyType }
*
* @return
* The created Ebi302EmptyType object and never null
.
*/
@Nonnull
public Ebi302EmptyType createEbi302EmptyType() {
return new Ebi302EmptyType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302InvoiceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302InvoiceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Invoice")
@Nonnull
public JAXBElement createInvoice(
@Nullable
final Ebi302InvoiceType value) {
return new JAXBElement(_Invoice_QNAME, Ebi302InvoiceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302AdditionalInformationType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302AdditionalInformationType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "AdditionalInformation")
@Nonnull
public JAXBElement createAdditionalInformation(
@Nullable
final Ebi302AdditionalInformationType value) {
return new JAXBElement(_AdditionalInformation_QNAME, Ebi302AdditionalInformationType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302AddressType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302AddressType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Address")
@Nonnull
public JAXBElement createAddress(
@Nullable
final Ebi302AddressType value) {
return new JAXBElement(_Address_QNAME, Ebi302AddressType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "AddressExtension")
@Nonnull
public JAXBElement createAddressExtension(
@Nullable
final String value) {
return new JAXBElement(_AddressExtension_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302UnitType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302UnitType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "AlternativeQuantity")
@Nonnull
public JAXBElement createAlternativeQuantity(
@Nullable
final Ebi302UnitType value) {
return new JAXBElement(_AlternativeQuantity_QNAME, Ebi302UnitType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Amount")
@Nonnull
public JAXBElement createAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_Amount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "BankAccountNr")
@Nonnull
public JAXBElement createBankAccountNr(
@Nullable
final String value) {
return new JAXBElement(_BankAccountNr_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "BankAccountOwner")
@Nonnull
public JAXBElement createBankAccountOwner(
@Nullable
final String value) {
return new JAXBElement(_BankAccountOwner_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302BankCodeCType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302BankCodeCType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "BankCode")
@Nonnull
public JAXBElement createBankCode(
@Nullable
final Ebi302BankCodeCType value) {
return new JAXBElement(_BankCode_QNAME, Ebi302BankCodeCType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "BankName")
@Nonnull
public JAXBElement createBankName(
@Nullable
final String value) {
return new JAXBElement(_BankName_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "BaseAmount")
@Nonnull
public JAXBElement createBaseAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_BaseAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302AccountType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302AccountType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "BeneficiaryAccount")
@Nonnull
public JAXBElement createBeneficiaryAccount(
@Nullable
final Ebi302AccountType value) {
return new JAXBElement(_BeneficiaryAccount_QNAME, Ebi302AccountType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "BIC")
@Nonnull
public JAXBElement createBIC(
@Nullable
final String value) {
return new JAXBElement(_BIC_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302BillerType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302BillerType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Biller")
@Nonnull
public JAXBElement createBiller(
@Nullable
final Ebi302BillerType value) {
return new JAXBElement(_Biller_QNAME, Ebi302BillerType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302OrderReferenceDetailType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302OrderReferenceDetailType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "BillersOrderReference")
@Nonnull
public JAXBElement createBillersOrderReference(
@Nullable
final Ebi302OrderReferenceDetailType value) {
return new JAXBElement(_BillersOrderReference_QNAME, Ebi302OrderReferenceDetailType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "BillersArticleNumber")
@Nonnull
public JAXBElement createBillersArticleNumber(
@Nullable
final String value) {
return new JAXBElement(_BillersArticleNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "BillersInvoiceRecipientID")
@Nonnull
public JAXBElement createBillersInvoiceRecipientID(
@Nullable
final String value) {
return new JAXBElement(_BillersInvoiceRecipientID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "BillersOrderingPartyID")
@Nonnull
public JAXBElement createBillersOrderingPartyID(
@Nullable
final String value) {
return new JAXBElement(_BillersOrderingPartyID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Boxes")
@Nonnull
public JAXBElement createBoxes(
@Nullable
final BigInteger value) {
return new JAXBElement(_Boxes_QNAME, BigInteger.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "ChargeNumber")
@Nonnull
public JAXBElement createChargeNumber(
@Nullable
final String value) {
return new JAXBElement(_ChargeNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302ClassificationType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302ClassificationType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Classification")
@Nonnull
public JAXBElement createClassification(
@Nullable
final Ebi302ClassificationType value) {
return new JAXBElement(_Classification_QNAME, Ebi302ClassificationType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Color")
@Nonnull
public JAXBElement createColor(
@Nullable
final String value) {
return new JAXBElement(_Color_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Comment")
@Nonnull
public JAXBElement createComment(
@Nullable
final String value) {
return new JAXBElement(_Comment_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "ConsolidatorsBillerID")
@Nonnull
public JAXBElement createConsolidatorsBillerID(
@Nullable
final String value) {
return new JAXBElement(_ConsolidatorsBillerID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Contact")
@Nonnull
public JAXBElement createContact(
@Nullable
final String value) {
return new JAXBElement(_Contact_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Country")
@Nonnull
public JAXBElement createCountry(
@Nullable
final String value) {
return new JAXBElement(_Country_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302CustomType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302CustomType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Custom")
@Nonnull
public JAXBElement createCustom(
@Nullable
final Ebi302CustomType value) {
return new JAXBElement(_Custom_QNAME, Ebi302CustomType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Date")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_Date_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302DeliveryType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302DeliveryType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Delivery")
@Nonnull
public JAXBElement createDelivery(
@Nullable
final Ebi302DeliveryType value) {
return new JAXBElement(_Delivery_QNAME, Ebi302DeliveryType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "DeliveryID")
@Nonnull
public JAXBElement createDeliveryID(
@Nullable
final String value) {
return new JAXBElement(_DeliveryID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Description")
@Nonnull
public JAXBElement createDescription(
@Nullable
final String value) {
return new JAXBElement(_Description_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302DetailsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302DetailsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Details")
@Nonnull
public JAXBElement createDetails(
@Nullable
final Ebi302DetailsType value) {
return new JAXBElement(_Details_QNAME, Ebi302DetailsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302DirectDebitType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302DirectDebitType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "DirectDebit")
@Nonnull
public JAXBElement createDirectDebit(
@Nullable
final Ebi302DirectDebitType value) {
return new JAXBElement(_DirectDebit_QNAME, Ebi302DirectDebitType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Boolean }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Boolean }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "DiscountFlag")
@Nonnull
public JAXBElement createDiscountFlag(
@Nullable
final Boolean value) {
return new JAXBElement(_DiscountFlag_QNAME, Boolean.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302DiscountType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302DiscountType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Discount")
@Nonnull
public JAXBElement createDiscount(
@Nullable
final Ebi302DiscountType value) {
return new JAXBElement(_Discount_QNAME, Ebi302DiscountType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "DueDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createDueDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_DueDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Email")
@Nonnull
public JAXBElement createEmail(
@Nullable
final String value) {
return new JAXBElement(_Email_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "FooterDescription")
@Nonnull
public JAXBElement createFooterDescription(
@Nullable
final String value) {
return new JAXBElement(_FooterDescription_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "FromDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createFromDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_FromDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302FurtherIdentificationType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302FurtherIdentificationType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "FurtherIdentification")
@Nonnull
public JAXBElement createFurtherIdentification(
@Nullable
final Ebi302FurtherIdentificationType value) {
return new JAXBElement(_FurtherIdentification_QNAME, Ebi302FurtherIdentificationType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "HeaderDescription")
@Nonnull
public JAXBElement createHeaderDescription(
@Nullable
final String value) {
return new JAXBElement(_HeaderDescription_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "IBAN")
@Nonnull
public JAXBElement createIBAN(
@Nullable
final String value) {
return new JAXBElement(_IBAN_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "OrderID")
@Nonnull
public JAXBElement createOrderID(
@Nullable
final String value) {
return new JAXBElement(_OrderID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "InvoiceDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createInvoiceDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_InvoiceDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "InvoiceNumber")
@Nonnull
public JAXBElement createInvoiceNumber(
@Nullable
final String value) {
return new JAXBElement(_InvoiceNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302InvoiceRecipientType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302InvoiceRecipientType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "InvoiceRecipient")
@Nonnull
public JAXBElement createInvoiceRecipient(
@Nullable
final Ebi302InvoiceRecipientType value) {
return new JAXBElement(_InvoiceRecipient_QNAME, Ebi302InvoiceRecipientType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "InvoiceRecipientsArticleNumber")
@Nonnull
public JAXBElement createInvoiceRecipientsArticleNumber(
@Nullable
final String value) {
return new JAXBElement(_InvoiceRecipientsArticleNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "InvoiceRecipientsBillerID")
@Nonnull
public JAXBElement createInvoiceRecipientsBillerID(
@Nullable
final String value) {
return new JAXBElement(_InvoiceRecipientsBillerID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302OrderReferenceDetailType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302OrderReferenceDetailType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "InvoiceRecipientsOrderReference")
@Nonnull
public JAXBElement createInvoiceRecipientsOrderReference(
@Nullable
final Ebi302OrderReferenceDetailType value) {
return new JAXBElement(_InvoiceRecipientsOrderReference_QNAME, Ebi302OrderReferenceDetailType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302ItemType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302ItemType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Item")
@Nonnull
public JAXBElement createItem(
@Nullable
final Ebi302ItemType value) {
return new JAXBElement(_Item_QNAME, Ebi302ItemType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302ItemListType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302ItemListType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "ItemList")
@Nonnull
public JAXBElement createItemList(
@Nullable
final Ebi302ItemListType value) {
return new JAXBElement(_ItemList_QNAME, Ebi302ItemListType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "LayoutID")
@Nonnull
public JAXBElement createLayoutID(
@Nullable
final String value) {
return new JAXBElement(_LayoutID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "LineItemAmount")
@Nonnull
public JAXBElement createLineItemAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_LineItemAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302ListLineItemType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302ListLineItemType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "ListLineItem")
@Nonnull
public JAXBElement createListLineItem(
@Nullable
final Ebi302ListLineItemType value) {
return new JAXBElement(_ListLineItem_QNAME, Ebi302ListLineItemType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "LogoURL")
@Nonnull
public JAXBElement createLogoURL(
@Nullable
final String value) {
return new JAXBElement(_LogoURL_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "MinimumPayment")
@Nonnull
public JAXBElement createMinimumPayment(
@Nullable
final BigDecimal value) {
return new JAXBElement(_MinimumPayment_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Name")
@Nonnull
public JAXBElement createName(
@Nullable
final String value) {
return new JAXBElement(_Name_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302NoPaymentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302NoPaymentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "NoPayment")
@Nonnull
public JAXBElement createNoPayment(
@Nullable
final Ebi302NoPaymentType value) {
return new JAXBElement(_NoPayment_QNAME, Ebi302NoPaymentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302OrderingPartyType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302OrderingPartyType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "OrderingParty")
@Nonnull
public JAXBElement createOrderingParty(
@Nullable
final Ebi302OrderingPartyType value) {
return new JAXBElement(_OrderingParty_QNAME, Ebi302OrderingPartyType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "OrderPositionNumber")
@Nonnull
public JAXBElement createOrderPositionNumber(
@Nullable
final String value) {
return new JAXBElement(_OrderPositionNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302OrderReferenceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302OrderReferenceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "OrderReference")
@Nonnull
public JAXBElement createOrderReference(
@Nullable
final Ebi302OrderReferenceType value) {
return new JAXBElement(_OrderReference_QNAME, Ebi302OrderReferenceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302OtherTaxType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302OtherTaxType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "OtherTax")
@Nonnull
public JAXBElement createOtherTax(
@Nullable
final Ebi302OtherTaxType value) {
return new JAXBElement(_OtherTax_QNAME, Ebi302OtherTaxType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302PaymentConditionsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302PaymentConditionsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "PaymentConditions")
@Nonnull
public JAXBElement createPaymentConditions(
@Nullable
final Ebi302PaymentConditionsType value) {
return new JAXBElement(_PaymentConditions_QNAME, Ebi302PaymentConditionsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "PaymentDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createPaymentDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_PaymentDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302PaymentMethodType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302PaymentMethodType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "PaymentMethod")
@Nonnull
public JAXBElement createPaymentMethod(
@Nullable
final Ebi302PaymentMethodType value) {
return new JAXBElement(_PaymentMethod_QNAME, Ebi302PaymentMethodType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "PaymentReference")
@Nonnull
public JAXBElement createPaymentReference(
@Nullable
final BigInteger value) {
return new JAXBElement(_PaymentReference_QNAME, BigInteger.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Percentage")
@Nonnull
public JAXBElement createPercentage(
@Nullable
final BigDecimal value) {
return new JAXBElement(_Percentage_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302PeriodType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302PeriodType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Period")
@Nonnull
public JAXBElement createPeriod(
@Nullable
final Ebi302PeriodType value) {
return new JAXBElement(_Period_QNAME, Ebi302PeriodType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Phone")
@Nonnull
public JAXBElement createPhone(
@Nullable
final String value) {
return new JAXBElement(_Phone_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "POBox")
@Nonnull
public JAXBElement createPOBox(
@Nullable
final String value) {
return new JAXBElement(_POBox_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "PositionNumber")
@Nonnull
public JAXBElement createPositionNumber(
@Nullable
final BigInteger value) {
return new JAXBElement(_PositionNumber_QNAME, BigInteger.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302PresentationDetailsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302PresentationDetailsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "PresentationDetails")
@Nonnull
public JAXBElement createPresentationDetails(
@Nullable
final Ebi302PresentationDetailsType value) {
return new JAXBElement(_PresentationDetails_QNAME, Ebi302PresentationDetailsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302UnitType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302UnitType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Quantity")
@Nonnull
public JAXBElement createQuantity(
@Nullable
final Ebi302UnitType value) {
return new JAXBElement(_Quantity_QNAME, Ebi302UnitType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302ReductionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302ReductionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Reduction")
@Nonnull
public JAXBElement createReduction(
@Nullable
final Ebi302ReductionType value) {
return new JAXBElement(_Reduction_QNAME, Ebi302ReductionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302ReductionDetailsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302ReductionDetailsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "ReductionDetails")
@Nonnull
public JAXBElement createReductionDetails(
@Nullable
final Ebi302ReductionDetailsType value) {
return new JAXBElement(_ReductionDetails_QNAME, Ebi302ReductionDetailsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "ReductionRate")
@Nonnull
public JAXBElement createReductionRate(
@Nullable
final BigDecimal value) {
return new JAXBElement(_ReductionRate_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "ReferenceDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createReferenceDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_ReferenceDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Salutation")
@Nonnull
public JAXBElement createSalutation(
@Nullable
final String value) {
return new JAXBElement(_Salutation_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "SerialNumber")
@Nonnull
public JAXBElement createSerialNumber(
@Nullable
final String value) {
return new JAXBElement(_SerialNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Size")
@Nonnull
public JAXBElement createSize(
@Nullable
final String value) {
return new JAXBElement(_Size_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Street")
@Nonnull
public JAXBElement createStreet(
@Nullable
final String value) {
return new JAXBElement(_Street_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Boolean }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Boolean }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "SuppressZero")
@Nonnull
public JAXBElement createSuppressZero(
@Nullable
final Boolean value) {
return new JAXBElement(_SuppressZero_QNAME, Boolean.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302TaxType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302TaxType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Tax")
@Nonnull
public JAXBElement createTax(
@Nullable
final Ebi302TaxType value) {
return new JAXBElement(_Tax_QNAME, Ebi302TaxType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "TaxExemption")
@Nonnull
public JAXBElement createTaxExemption(
@Nullable
final String value) {
return new JAXBElement(_TaxExemption_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "TaxedAmount")
@Nonnull
public JAXBElement createTaxedAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_TaxedAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302TaxRateType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302TaxRateType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "TaxRate")
@Nonnull
public JAXBElement createTaxRate(
@Nullable
final Ebi302TaxRateType value) {
return new JAXBElement(_TaxRate_QNAME, Ebi302TaxRateType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "ToDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createToDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_ToDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "TotalGrossAmount")
@Nonnull
public JAXBElement createTotalGrossAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_TotalGrossAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Town")
@Nonnull
public JAXBElement createTown(
@Nullable
final String value) {
return new JAXBElement(_Town_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "UnitPrice")
@Nonnull
public JAXBElement createUnitPrice(
@Nullable
final BigDecimal value) {
return new JAXBElement(_UnitPrice_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "URL")
@Nonnull
public JAXBElement createURL(
@Nullable
final String value) {
return new JAXBElement(_URL_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302UniversalBankTransactionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302UniversalBankTransactionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "UniversalBankTransaction")
@Nonnull
public JAXBElement createUniversalBankTransaction(
@Nullable
final Ebi302UniversalBankTransactionType value) {
return new JAXBElement(_UniversalBankTransaction_QNAME, Ebi302UniversalBankTransactionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302VATType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302VATType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "VAT")
@Nonnull
public JAXBElement createVAT(
@Nullable
final Ebi302VATType value) {
return new JAXBElement(_VAT_QNAME, Ebi302VATType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "VATIdentificationNumber")
@Nonnull
public JAXBElement createVATIdentificationNumber(
@Nullable
final String value) {
return new JAXBElement(_VATIdentificationNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi302UnitType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi302UnitType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "Weight")
@Nonnull
public JAXBElement createWeight(
@Nullable
final Ebi302UnitType value) {
return new JAXBElement(_Weight_QNAME, Ebi302UnitType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/3p02/", name = "ZIP")
@Nonnull
public JAXBElement createZIP(
@Nullable
final String value) {
return new JAXBElement(_ZIP_QNAME, String.class, null, value);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy