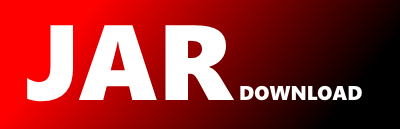
com.helger.ebinterface.v40.extensions.ObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-ebinterface Show documentation
Show all versions of ph-ebinterface Show documentation
ebInterface wrapper library to easily read and write ebInterface documents
package com.helger.ebinterface.v40.extensions;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.helger.ebinterface.v40.extensions package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _BillerExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p0/extensions/ext", "BillerExtension");
public final static QName _Custom_QNAME = new QName("http://www.ebinterface.at/schema/4p0/extensions/ext", "Custom");
public final static QName _DeliveryExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p0/extensions/ext", "DeliveryExtension");
public final static QName _DetailsExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p0/extensions/ext", "DetailsExtension");
public final static QName _InvoiceRecipientExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p0/extensions/ext", "InvoiceRecipientExtension");
public final static QName _InvoiceRootExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p0/extensions/ext", "InvoiceRootExtension");
public final static QName _ListLineItemExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p0/extensions/ext", "ListLineItemExtension");
public final static QName _OrderingPartyExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p0/extensions/ext", "OrderingPartyExtension");
public final static QName _PaymentConditionsExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p0/extensions/ext", "PaymentConditionsExtension");
public final static QName _PaymentMethodExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p0/extensions/ext", "PaymentMethodExtension");
public final static QName _PresentationDetailsExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p0/extensions/ext", "PresentationDetailsExtension");
public final static QName _ReductionAndSurchargeDetailsExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p0/extensions/ext", "ReductionAndSurchargeDetailsExtension");
public final static QName _TaxExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p0/extensions/ext", "TaxExtension");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.helger.ebinterface.v40.extensions
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link Ebi40BillerExtensionType }
*
* @return
* The created Ebi40BillerExtensionType object and never null
.
*/
@Nonnull
public Ebi40BillerExtensionType createEbi40BillerExtensionType() {
return new Ebi40BillerExtensionType();
}
/**
* Create an instance of {@link Ebi40CustomType }
*
* @return
* The created Ebi40CustomType object and never null
.
*/
@Nonnull
public Ebi40CustomType createEbi40CustomType() {
return new Ebi40CustomType();
}
/**
* Create an instance of {@link Ebi40DeliveryExtensionType }
*
* @return
* The created Ebi40DeliveryExtensionType object and never null
.
*/
@Nonnull
public Ebi40DeliveryExtensionType createEbi40DeliveryExtensionType() {
return new Ebi40DeliveryExtensionType();
}
/**
* Create an instance of {@link Ebi40DetailsExtensionType }
*
* @return
* The created Ebi40DetailsExtensionType object and never null
.
*/
@Nonnull
public Ebi40DetailsExtensionType createEbi40DetailsExtensionType() {
return new Ebi40DetailsExtensionType();
}
/**
* Create an instance of {@link Ebi40InvoiceRecipientExtensionType }
*
* @return
* The created Ebi40InvoiceRecipientExtensionType object and never null
.
*/
@Nonnull
public Ebi40InvoiceRecipientExtensionType createEbi40InvoiceRecipientExtensionType() {
return new Ebi40InvoiceRecipientExtensionType();
}
/**
* Create an instance of {@link Ebi40InvoiceRootExtensionType }
*
* @return
* The created Ebi40InvoiceRootExtensionType object and never null
.
*/
@Nonnull
public Ebi40InvoiceRootExtensionType createEbi40InvoiceRootExtensionType() {
return new Ebi40InvoiceRootExtensionType();
}
/**
* Create an instance of {@link Ebi40ListLineItemExtensionType }
*
* @return
* The created Ebi40ListLineItemExtensionType object and never null
.
*/
@Nonnull
public Ebi40ListLineItemExtensionType createEbi40ListLineItemExtensionType() {
return new Ebi40ListLineItemExtensionType();
}
/**
* Create an instance of {@link Ebi40OrderingPartyExtensionType }
*
* @return
* The created Ebi40OrderingPartyExtensionType object and never null
.
*/
@Nonnull
public Ebi40OrderingPartyExtensionType createEbi40OrderingPartyExtensionType() {
return new Ebi40OrderingPartyExtensionType();
}
/**
* Create an instance of {@link Ebi40PaymentConditionsExtensionType }
*
* @return
* The created Ebi40PaymentConditionsExtensionType object and never null
.
*/
@Nonnull
public Ebi40PaymentConditionsExtensionType createEbi40PaymentConditionsExtensionType() {
return new Ebi40PaymentConditionsExtensionType();
}
/**
* Create an instance of {@link Ebi40PaymentMethodExtensionType }
*
* @return
* The created Ebi40PaymentMethodExtensionType object and never null
.
*/
@Nonnull
public Ebi40PaymentMethodExtensionType createEbi40PaymentMethodExtensionType() {
return new Ebi40PaymentMethodExtensionType();
}
/**
* Create an instance of {@link Ebi40PresentationDetailsExtensionType }
*
* @return
* The created Ebi40PresentationDetailsExtensionType object and never null
.
*/
@Nonnull
public Ebi40PresentationDetailsExtensionType createEbi40PresentationDetailsExtensionType() {
return new Ebi40PresentationDetailsExtensionType();
}
/**
* Create an instance of {@link Ebi40ReductionAndSurchargeDetailsExtensionType }
*
* @return
* The created Ebi40ReductionAndSurchargeDetailsExtensionType object and never null
.
*/
@Nonnull
public Ebi40ReductionAndSurchargeDetailsExtensionType createEbi40ReductionAndSurchargeDetailsExtensionType() {
return new Ebi40ReductionAndSurchargeDetailsExtensionType();
}
/**
* Create an instance of {@link Ebi40TaxExtensionType }
*
* @return
* The created Ebi40TaxExtensionType object and never null
.
*/
@Nonnull
public Ebi40TaxExtensionType createEbi40TaxExtensionType() {
return new Ebi40TaxExtensionType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi40BillerExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi40BillerExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p0/extensions/ext", name = "BillerExtension")
@Nonnull
public JAXBElement createBillerExtension(
@Nullable
final Ebi40BillerExtensionType value) {
return new JAXBElement(_BillerExtension_QNAME, Ebi40BillerExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi40CustomType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi40CustomType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p0/extensions/ext", name = "Custom")
@Nonnull
public JAXBElement createCustom(
@Nullable
final Ebi40CustomType value) {
return new JAXBElement(_Custom_QNAME, Ebi40CustomType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi40DeliveryExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi40DeliveryExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p0/extensions/ext", name = "DeliveryExtension")
@Nonnull
public JAXBElement createDeliveryExtension(
@Nullable
final Ebi40DeliveryExtensionType value) {
return new JAXBElement(_DeliveryExtension_QNAME, Ebi40DeliveryExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi40DetailsExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi40DetailsExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p0/extensions/ext", name = "DetailsExtension")
@Nonnull
public JAXBElement createDetailsExtension(
@Nullable
final Ebi40DetailsExtensionType value) {
return new JAXBElement(_DetailsExtension_QNAME, Ebi40DetailsExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi40InvoiceRecipientExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi40InvoiceRecipientExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p0/extensions/ext", name = "InvoiceRecipientExtension")
@Nonnull
public JAXBElement createInvoiceRecipientExtension(
@Nullable
final Ebi40InvoiceRecipientExtensionType value) {
return new JAXBElement(_InvoiceRecipientExtension_QNAME, Ebi40InvoiceRecipientExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi40InvoiceRootExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi40InvoiceRootExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p0/extensions/ext", name = "InvoiceRootExtension")
@Nonnull
public JAXBElement createInvoiceRootExtension(
@Nullable
final Ebi40InvoiceRootExtensionType value) {
return new JAXBElement(_InvoiceRootExtension_QNAME, Ebi40InvoiceRootExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi40ListLineItemExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi40ListLineItemExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p0/extensions/ext", name = "ListLineItemExtension")
@Nonnull
public JAXBElement createListLineItemExtension(
@Nullable
final Ebi40ListLineItemExtensionType value) {
return new JAXBElement(_ListLineItemExtension_QNAME, Ebi40ListLineItemExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi40OrderingPartyExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi40OrderingPartyExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p0/extensions/ext", name = "OrderingPartyExtension")
@Nonnull
public JAXBElement createOrderingPartyExtension(
@Nullable
final Ebi40OrderingPartyExtensionType value) {
return new JAXBElement(_OrderingPartyExtension_QNAME, Ebi40OrderingPartyExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi40PaymentConditionsExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi40PaymentConditionsExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p0/extensions/ext", name = "PaymentConditionsExtension")
@Nonnull
public JAXBElement createPaymentConditionsExtension(
@Nullable
final Ebi40PaymentConditionsExtensionType value) {
return new JAXBElement(_PaymentConditionsExtension_QNAME, Ebi40PaymentConditionsExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi40PaymentMethodExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi40PaymentMethodExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p0/extensions/ext", name = "PaymentMethodExtension")
@Nonnull
public JAXBElement createPaymentMethodExtension(
@Nullable
final Ebi40PaymentMethodExtensionType value) {
return new JAXBElement(_PaymentMethodExtension_QNAME, Ebi40PaymentMethodExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi40PresentationDetailsExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi40PresentationDetailsExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p0/extensions/ext", name = "PresentationDetailsExtension")
@Nonnull
public JAXBElement createPresentationDetailsExtension(
@Nullable
final Ebi40PresentationDetailsExtensionType value) {
return new JAXBElement(_PresentationDetailsExtension_QNAME, Ebi40PresentationDetailsExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi40ReductionAndSurchargeDetailsExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi40ReductionAndSurchargeDetailsExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p0/extensions/ext", name = "ReductionAndSurchargeDetailsExtension")
@Nonnull
public JAXBElement createReductionAndSurchargeDetailsExtension(
@Nullable
final Ebi40ReductionAndSurchargeDetailsExtensionType value) {
return new JAXBElement(_ReductionAndSurchargeDetailsExtension_QNAME, Ebi40ReductionAndSurchargeDetailsExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi40TaxExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi40TaxExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p0/extensions/ext", name = "TaxExtension")
@Nonnull
public JAXBElement createTaxExtension(
@Nullable
final Ebi40TaxExtensionType value) {
return new JAXBElement(_TaxExtension_QNAME, Ebi40TaxExtensionType.class, null, value);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy