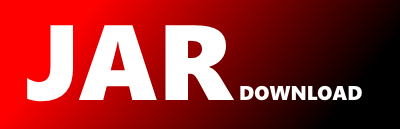
com.helger.ebinterface.v41.ObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-ebinterface Show documentation
Show all versions of ph-ebinterface Show documentation
ebInterface wrapper library to easily read and write ebInterface documents
package com.helger.ebinterface.v41;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.time.LocalDate;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
import com.helger.jaxb.adapter.AdapterLocalDate;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.helger.ebinterface.v41 package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _Invoice_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Invoice");
public final static QName _AccountingArea_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "AccountingArea");
public final static QName _AdditionalInformation_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "AdditionalInformation");
public final static QName _Address_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Address");
public final static QName _AddressExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "AddressExtension");
public final static QName _AddressIdentifier_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "AddressIdentifier");
public final static QName _AlternativeQuantity_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "AlternativeQuantity");
public final static QName _Amount_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Amount");
public final static QName _ArticleNumber_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "ArticleNumber");
public final static QName _BankAccountNr_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "BankAccountNr");
public final static QName _BankAccountOwner_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "BankAccountOwner");
public final static QName _BankCode_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "BankCode");
public final static QName _BankName_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "BankName");
public final static QName _BaseAmount_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "BaseAmount");
public final static QName _BelowTheLineItem_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "BelowTheLineItem");
public final static QName _BeneficiaryAccount_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "BeneficiaryAccount");
public final static QName _BIC_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "BIC");
public final static QName _Biller_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Biller");
public final static QName _BillersInvoiceRecipientID_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "BillersInvoiceRecipientID");
public final static QName _BillersOrderingPartyID_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "BillersOrderingPartyID");
public final static QName _BillersOrderReference_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "BillersOrderReference");
public final static QName _Boxes_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Boxes");
public final static QName _CancelledOriginalDocument_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "CancelledOriginalDocument");
public final static QName _ChargeNumber_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "ChargeNumber");
public final static QName _Classification_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Classification");
public final static QName _Color_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Color");
public final static QName _Comment_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Comment");
public final static QName _ConsolidatorsBillerID_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "ConsolidatorsBillerID");
public final static QName _Contact_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Contact");
public final static QName _Country_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Country");
public final static QName _CreditorID_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "CreditorID");
public final static QName _Date_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Date");
public final static QName _DebitCollectionDate_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "DebitCollectionDate");
public final static QName _Delivery_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Delivery");
public final static QName _DeliveryID_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "DeliveryID");
public final static QName _Description_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Description");
public final static QName _Details_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Details");
public final static QName _DirectDebit_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "DirectDebit");
public final static QName _Discount_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Discount");
public final static QName _DiscountFlag_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "DiscountFlag");
public final static QName _DocumentType_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "DocumentType");
public final static QName _DueDate_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "DueDate");
public final static QName _Email_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Email");
public final static QName _FooterDescription_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "FooterDescription");
public final static QName _FromDate_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "FromDate");
public final static QName _FurtherIdentification_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "FurtherIdentification");
public final static QName _HeaderDescription_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "HeaderDescription");
public final static QName _IBAN_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "IBAN");
public final static QName _InvoiceDate_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "InvoiceDate");
public final static QName _InvoiceNumber_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "InvoiceNumber");
public final static QName _InvoiceRecipient_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "InvoiceRecipient");
public final static QName _InvoiceRecipientsBillerID_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "InvoiceRecipientsBillerID");
public final static QName _InvoiceRecipientsOrderReference_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "InvoiceRecipientsOrderReference");
public final static QName _VATItem_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "VATItem");
public final static QName _ItemList_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "ItemList");
public final static QName _LayoutID_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "LayoutID");
public final static QName _LineItemAmount_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "LineItemAmount");
public final static QName _ListLineItem_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "ListLineItem");
public final static QName _LogoURL_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "LogoURL");
public final static QName _MandateReference_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "MandateReference");
public final static QName _MinimumPayment_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "MinimumPayment");
public final static QName _Name_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Name");
public final static QName _NoPayment_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "NoPayment");
public final static QName _OrderID_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "OrderID");
public final static QName _OrderingParty_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "OrderingParty");
public final static QName _OrderPositionNumber_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "OrderPositionNumber");
public final static QName _OrderReference_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "OrderReference");
public final static QName _OtherTax_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "OtherTax");
public final static QName _OtherVATableTax_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "OtherVATableTax");
public final static QName _OtherVATableTaxListLineItem_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "OtherVATableTaxListLineItem");
public final static QName _PayableAmount_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "PayableAmount");
public final static QName _PaymentConditions_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "PaymentConditions");
public final static QName _PaymentDate_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "PaymentDate");
public final static QName _PaymentMethod_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "PaymentMethod");
public final static QName _PaymentReference_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "PaymentReference");
public final static QName _Percentage_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Percentage");
public final static QName _Period_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Period");
public final static QName _Phone_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Phone");
public final static QName _POBox_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "POBox");
public final static QName _PositionNumber_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "PositionNumber");
public final static QName _PresentationDetails_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "PresentationDetails");
public final static QName _Quantity_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Quantity");
public final static QName _Reason_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Reason");
public final static QName _Reduction_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Reduction");
public final static QName _ReductionAndSurchargeDetails_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "ReductionAndSurchargeDetails");
public final static QName _ReductionAndSurchargeListLineItemDetails_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "ReductionAndSurchargeListLineItemDetails");
public final static QName _ReductionListLineItem_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "ReductionListLineItem");
public final static QName _ReferenceDate_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "ReferenceDate");
public final static QName _RelatedDocument_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "RelatedDocument");
public final static QName _Salutation_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Salutation");
public final static QName _SEPADirectDebit_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "SEPADirectDebit");
public final static QName _SerialNumber_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "SerialNumber");
public final static QName _Size_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Size");
public final static QName _Street_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Street");
public final static QName _SubOrganizationID_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "SubOrganizationID");
public final static QName _SuppressZero_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "SuppressZero");
public final static QName _Surcharge_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Surcharge");
public final static QName _SurchargeListLineItem_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "SurchargeListLineItem");
public final static QName _Tax_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Tax");
public final static QName _TaxedAmount_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "TaxedAmount");
public final static QName _TaxExemption_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "TaxExemption");
public final static QName _ToDate_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "ToDate");
public final static QName _TotalGrossAmount_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "TotalGrossAmount");
public final static QName _Town_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Town");
public final static QName _Type_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Type");
public final static QName _UnitPrice_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "UnitPrice");
public final static QName _UniversalBankTransaction_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "UniversalBankTransaction");
public final static QName _URL_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "URL");
public final static QName _VAT_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "VAT");
public final static QName _VATIdentificationNumber_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "VATIdentificationNumber");
public final static QName _VATRate_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "VATRate");
public final static QName _Weight_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "Weight");
public final static QName _ZIP_QNAME = new QName("http://www.ebinterface.at/schema/4p1/", "ZIP");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.helger.ebinterface.v41
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link Ebi41InvoiceType }
*
* @return
* The created Ebi41InvoiceType object and never null
.
*/
@Nonnull
public Ebi41InvoiceType createEbi41InvoiceType() {
return new Ebi41InvoiceType();
}
/**
* Create an instance of {@link Ebi41AdditionalInformationType }
*
* @return
* The created Ebi41AdditionalInformationType object and never null
.
*/
@Nonnull
public Ebi41AdditionalInformationType createEbi41AdditionalInformationType() {
return new Ebi41AdditionalInformationType();
}
/**
* Create an instance of {@link Ebi41AddressType }
*
* @return
* The created Ebi41AddressType object and never null
.
*/
@Nonnull
public Ebi41AddressType createEbi41AddressType() {
return new Ebi41AddressType();
}
/**
* Create an instance of {@link Ebi41AddressIdentifierType }
*
* @return
* The created Ebi41AddressIdentifierType object and never null
.
*/
@Nonnull
public Ebi41AddressIdentifierType createEbi41AddressIdentifierType() {
return new Ebi41AddressIdentifierType();
}
/**
* Create an instance of {@link Ebi41UnitType }
*
* @return
* The created Ebi41UnitType object and never null
.
*/
@Nonnull
public Ebi41UnitType createEbi41UnitType() {
return new Ebi41UnitType();
}
/**
* Create an instance of {@link Ebi41ArticleNumberType }
*
* @return
* The created Ebi41ArticleNumberType object and never null
.
*/
@Nonnull
public Ebi41ArticleNumberType createEbi41ArticleNumberType() {
return new Ebi41ArticleNumberType();
}
/**
* Create an instance of {@link Ebi41BankCodeType }
*
* @return
* The created Ebi41BankCodeType object and never null
.
*/
@Nonnull
public Ebi41BankCodeType createEbi41BankCodeType() {
return new Ebi41BankCodeType();
}
/**
* Create an instance of {@link Ebi41BelowTheLineItemType }
*
* @return
* The created Ebi41BelowTheLineItemType object and never null
.
*/
@Nonnull
public Ebi41BelowTheLineItemType createEbi41BelowTheLineItemType() {
return new Ebi41BelowTheLineItemType();
}
/**
* Create an instance of {@link Ebi41AccountType }
*
* @return
* The created Ebi41AccountType object and never null
.
*/
@Nonnull
public Ebi41AccountType createEbi41AccountType() {
return new Ebi41AccountType();
}
/**
* Create an instance of {@link Ebi41BillerType }
*
* @return
* The created Ebi41BillerType object and never null
.
*/
@Nonnull
public Ebi41BillerType createEbi41BillerType() {
return new Ebi41BillerType();
}
/**
* Create an instance of {@link Ebi41OrderReferenceDetailType }
*
* @return
* The created Ebi41OrderReferenceDetailType object and never null
.
*/
@Nonnull
public Ebi41OrderReferenceDetailType createEbi41OrderReferenceDetailType() {
return new Ebi41OrderReferenceDetailType();
}
/**
* Create an instance of {@link Ebi41CancelledOriginalDocumentType }
*
* @return
* The created Ebi41CancelledOriginalDocumentType object and never null
.
*/
@Nonnull
public Ebi41CancelledOriginalDocumentType createEbi41CancelledOriginalDocumentType() {
return new Ebi41CancelledOriginalDocumentType();
}
/**
* Create an instance of {@link Ebi41ClassificationType }
*
* @return
* The created Ebi41ClassificationType object and never null
.
*/
@Nonnull
public Ebi41ClassificationType createEbi41ClassificationType() {
return new Ebi41ClassificationType();
}
/**
* Create an instance of {@link Ebi41CountryType }
*
* @return
* The created Ebi41CountryType object and never null
.
*/
@Nonnull
public Ebi41CountryType createEbi41CountryType() {
return new Ebi41CountryType();
}
/**
* Create an instance of {@link Ebi41DeliveryType }
*
* @return
* The created Ebi41DeliveryType object and never null
.
*/
@Nonnull
public Ebi41DeliveryType createEbi41DeliveryType() {
return new Ebi41DeliveryType();
}
/**
* Create an instance of {@link Ebi41DetailsType }
*
* @return
* The created Ebi41DetailsType object and never null
.
*/
@Nonnull
public Ebi41DetailsType createEbi41DetailsType() {
return new Ebi41DetailsType();
}
/**
* Create an instance of {@link Ebi41DirectDebitType }
*
* @return
* The created Ebi41DirectDebitType object and never null
.
*/
@Nonnull
public Ebi41DirectDebitType createEbi41DirectDebitType() {
return new Ebi41DirectDebitType();
}
/**
* Create an instance of {@link Ebi41DiscountType }
*
* @return
* The created Ebi41DiscountType object and never null
.
*/
@Nonnull
public Ebi41DiscountType createEbi41DiscountType() {
return new Ebi41DiscountType();
}
/**
* Create an instance of {@link Ebi41FurtherIdentificationType }
*
* @return
* The created Ebi41FurtherIdentificationType object and never null
.
*/
@Nonnull
public Ebi41FurtherIdentificationType createEbi41FurtherIdentificationType() {
return new Ebi41FurtherIdentificationType();
}
/**
* Create an instance of {@link Ebi41InvoiceRecipientType }
*
* @return
* The created Ebi41InvoiceRecipientType object and never null
.
*/
@Nonnull
public Ebi41InvoiceRecipientType createEbi41InvoiceRecipientType() {
return new Ebi41InvoiceRecipientType();
}
/**
* Create an instance of {@link Ebi41VATItemType }
*
* @return
* The created Ebi41VATItemType object and never null
.
*/
@Nonnull
public Ebi41VATItemType createEbi41VATItemType() {
return new Ebi41VATItemType();
}
/**
* Create an instance of {@link Ebi41ItemListType }
*
* @return
* The created Ebi41ItemListType object and never null
.
*/
@Nonnull
public Ebi41ItemListType createEbi41ItemListType() {
return new Ebi41ItemListType();
}
/**
* Create an instance of {@link Ebi41ListLineItemType }
*
* @return
* The created Ebi41ListLineItemType object and never null
.
*/
@Nonnull
public Ebi41ListLineItemType createEbi41ListLineItemType() {
return new Ebi41ListLineItemType();
}
/**
* Create an instance of {@link Ebi41NoPaymentType }
*
* @return
* The created Ebi41NoPaymentType object and never null
.
*/
@Nonnull
public Ebi41NoPaymentType createEbi41NoPaymentType() {
return new Ebi41NoPaymentType();
}
/**
* Create an instance of {@link Ebi41OrderingPartyType }
*
* @return
* The created Ebi41OrderingPartyType object and never null
.
*/
@Nonnull
public Ebi41OrderingPartyType createEbi41OrderingPartyType() {
return new Ebi41OrderingPartyType();
}
/**
* Create an instance of {@link Ebi41OrderReferenceType }
*
* @return
* The created Ebi41OrderReferenceType object and never null
.
*/
@Nonnull
public Ebi41OrderReferenceType createEbi41OrderReferenceType() {
return new Ebi41OrderReferenceType();
}
/**
* Create an instance of {@link Ebi41OtherTaxType }
*
* @return
* The created Ebi41OtherTaxType object and never null
.
*/
@Nonnull
public Ebi41OtherTaxType createEbi41OtherTaxType() {
return new Ebi41OtherTaxType();
}
/**
* Create an instance of {@link Ebi41OtherVATableTaxType }
*
* @return
* The created Ebi41OtherVATableTaxType object and never null
.
*/
@Nonnull
public Ebi41OtherVATableTaxType createEbi41OtherVATableTaxType() {
return new Ebi41OtherVATableTaxType();
}
/**
* Create an instance of {@link Ebi41OtherVATableTaxBaseType }
*
* @return
* The created Ebi41OtherVATableTaxBaseType object and never null
.
*/
@Nonnull
public Ebi41OtherVATableTaxBaseType createEbi41OtherVATableTaxBaseType() {
return new Ebi41OtherVATableTaxBaseType();
}
/**
* Create an instance of {@link Ebi41PaymentConditionsType }
*
* @return
* The created Ebi41PaymentConditionsType object and never null
.
*/
@Nonnull
public Ebi41PaymentConditionsType createEbi41PaymentConditionsType() {
return new Ebi41PaymentConditionsType();
}
/**
* Create an instance of {@link Ebi41PaymentMethodType }
*
* @return
* The created Ebi41PaymentMethodType object and never null
.
*/
@Nonnull
public Ebi41PaymentMethodType createEbi41PaymentMethodType() {
return new Ebi41PaymentMethodType();
}
/**
* Create an instance of {@link Ebi41PaymentReferenceType }
*
* @return
* The created Ebi41PaymentReferenceType object and never null
.
*/
@Nonnull
public Ebi41PaymentReferenceType createEbi41PaymentReferenceType() {
return new Ebi41PaymentReferenceType();
}
/**
* Create an instance of {@link Ebi41PeriodType }
*
* @return
* The created Ebi41PeriodType object and never null
.
*/
@Nonnull
public Ebi41PeriodType createEbi41PeriodType() {
return new Ebi41PeriodType();
}
/**
* Create an instance of {@link Ebi41PresentationDetailsType }
*
* @return
* The created Ebi41PresentationDetailsType object and never null
.
*/
@Nonnull
public Ebi41PresentationDetailsType createEbi41PresentationDetailsType() {
return new Ebi41PresentationDetailsType();
}
/**
* Create an instance of {@link Ebi41ReasonType }
*
* @return
* The created Ebi41ReasonType object and never null
.
*/
@Nonnull
public Ebi41ReasonType createEbi41ReasonType() {
return new Ebi41ReasonType();
}
/**
* Create an instance of {@link Ebi41ReductionAndSurchargeType }
*
* @return
* The created Ebi41ReductionAndSurchargeType object and never null
.
*/
@Nonnull
public Ebi41ReductionAndSurchargeType createEbi41ReductionAndSurchargeType() {
return new Ebi41ReductionAndSurchargeType();
}
/**
* Create an instance of {@link Ebi41ReductionAndSurchargeDetailsType }
*
* @return
* The created Ebi41ReductionAndSurchargeDetailsType object and never null
.
*/
@Nonnull
public Ebi41ReductionAndSurchargeDetailsType createEbi41ReductionAndSurchargeDetailsType() {
return new Ebi41ReductionAndSurchargeDetailsType();
}
/**
* Create an instance of {@link Ebi41ReductionAndSurchargeListLineItemDetailsType }
*
* @return
* The created Ebi41ReductionAndSurchargeListLineItemDetailsType object and never null
.
*/
@Nonnull
public Ebi41ReductionAndSurchargeListLineItemDetailsType createEbi41ReductionAndSurchargeListLineItemDetailsType() {
return new Ebi41ReductionAndSurchargeListLineItemDetailsType();
}
/**
* Create an instance of {@link Ebi41ReductionAndSurchargeBaseType }
*
* @return
* The created Ebi41ReductionAndSurchargeBaseType object and never null
.
*/
@Nonnull
public Ebi41ReductionAndSurchargeBaseType createEbi41ReductionAndSurchargeBaseType() {
return new Ebi41ReductionAndSurchargeBaseType();
}
/**
* Create an instance of {@link Ebi41RelatedDocumentType }
*
* @return
* The created Ebi41RelatedDocumentType object and never null
.
*/
@Nonnull
public Ebi41RelatedDocumentType createEbi41RelatedDocumentType() {
return new Ebi41RelatedDocumentType();
}
/**
* Create an instance of {@link Ebi41SEPADirectDebitType }
*
* @return
* The created Ebi41SEPADirectDebitType object and never null
.
*/
@Nonnull
public Ebi41SEPADirectDebitType createEbi41SEPADirectDebitType() {
return new Ebi41SEPADirectDebitType();
}
/**
* Create an instance of {@link Ebi41TaxType }
*
* @return
* The created Ebi41TaxType object and never null
.
*/
@Nonnull
public Ebi41TaxType createEbi41TaxType() {
return new Ebi41TaxType();
}
/**
* Create an instance of {@link Ebi41TaxExemptionType }
*
* @return
* The created Ebi41TaxExemptionType object and never null
.
*/
@Nonnull
public Ebi41TaxExemptionType createEbi41TaxExemptionType() {
return new Ebi41TaxExemptionType();
}
/**
* Create an instance of {@link Ebi41UnitPriceType }
*
* @return
* The created Ebi41UnitPriceType object and never null
.
*/
@Nonnull
public Ebi41UnitPriceType createEbi41UnitPriceType() {
return new Ebi41UnitPriceType();
}
/**
* Create an instance of {@link Ebi41UniversalBankTransactionType }
*
* @return
* The created Ebi41UniversalBankTransactionType object and never null
.
*/
@Nonnull
public Ebi41UniversalBankTransactionType createEbi41UniversalBankTransactionType() {
return new Ebi41UniversalBankTransactionType();
}
/**
* Create an instance of {@link Ebi41VATType }
*
* @return
* The created Ebi41VATType object and never null
.
*/
@Nonnull
public Ebi41VATType createEbi41VATType() {
return new Ebi41VATType();
}
/**
* Create an instance of {@link Ebi41VATRateType }
*
* @return
* The created Ebi41VATRateType object and never null
.
*/
@Nonnull
public Ebi41VATRateType createEbi41VATRateType() {
return new Ebi41VATRateType();
}
/**
* Create an instance of {@link Ebi41AbstractPartyType }
*
* @return
* The created Ebi41AbstractPartyType object and never null
.
*/
@Nonnull
public Ebi41AbstractPartyType createEbi41AbstractPartyType() {
return new Ebi41AbstractPartyType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41InvoiceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41InvoiceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Invoice")
@Nonnull
public JAXBElement createInvoice(
@Nullable
final Ebi41InvoiceType value) {
return new JAXBElement(_Invoice_QNAME, Ebi41InvoiceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "AccountingArea")
@Nonnull
public JAXBElement createAccountingArea(
@Nullable
final String value) {
return new JAXBElement(_AccountingArea_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41AdditionalInformationType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41AdditionalInformationType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "AdditionalInformation")
@Nonnull
public JAXBElement createAdditionalInformation(
@Nullable
final Ebi41AdditionalInformationType value) {
return new JAXBElement(_AdditionalInformation_QNAME, Ebi41AdditionalInformationType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41AddressType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41AddressType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Address")
@Nonnull
public JAXBElement createAddress(
@Nullable
final Ebi41AddressType value) {
return new JAXBElement(_Address_QNAME, Ebi41AddressType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "AddressExtension")
@Nonnull
public JAXBElement createAddressExtension(
@Nullable
final String value) {
return new JAXBElement(_AddressExtension_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41AddressIdentifierType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41AddressIdentifierType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "AddressIdentifier")
@Nonnull
public JAXBElement createAddressIdentifier(
@Nullable
final Ebi41AddressIdentifierType value) {
return new JAXBElement(_AddressIdentifier_QNAME, Ebi41AddressIdentifierType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41UnitType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41UnitType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "AlternativeQuantity")
@Nonnull
public JAXBElement createAlternativeQuantity(
@Nullable
final Ebi41UnitType value) {
return new JAXBElement(_AlternativeQuantity_QNAME, Ebi41UnitType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Amount")
@Nonnull
public JAXBElement createAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_Amount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41ArticleNumberType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41ArticleNumberType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "ArticleNumber")
@Nonnull
public JAXBElement createArticleNumber(
@Nullable
final Ebi41ArticleNumberType value) {
return new JAXBElement(_ArticleNumber_QNAME, Ebi41ArticleNumberType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "BankAccountNr")
@Nonnull
public JAXBElement createBankAccountNr(
@Nullable
final String value) {
return new JAXBElement(_BankAccountNr_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "BankAccountOwner")
@Nonnull
public JAXBElement createBankAccountOwner(
@Nullable
final String value) {
return new JAXBElement(_BankAccountOwner_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41BankCodeType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41BankCodeType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "BankCode")
@Nonnull
public JAXBElement createBankCode(
@Nullable
final Ebi41BankCodeType value) {
return new JAXBElement(_BankCode_QNAME, Ebi41BankCodeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "BankName")
@Nonnull
public JAXBElement createBankName(
@Nullable
final String value) {
return new JAXBElement(_BankName_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "BaseAmount")
@Nonnull
public JAXBElement createBaseAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_BaseAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41BelowTheLineItemType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41BelowTheLineItemType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "BelowTheLineItem")
@Nonnull
public JAXBElement createBelowTheLineItem(
@Nullable
final Ebi41BelowTheLineItemType value) {
return new JAXBElement(_BelowTheLineItem_QNAME, Ebi41BelowTheLineItemType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41AccountType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41AccountType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "BeneficiaryAccount")
@Nonnull
public JAXBElement createBeneficiaryAccount(
@Nullable
final Ebi41AccountType value) {
return new JAXBElement(_BeneficiaryAccount_QNAME, Ebi41AccountType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "BIC")
@Nonnull
public JAXBElement createBIC(
@Nullable
final String value) {
return new JAXBElement(_BIC_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41BillerType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41BillerType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Biller")
@Nonnull
public JAXBElement createBiller(
@Nullable
final Ebi41BillerType value) {
return new JAXBElement(_Biller_QNAME, Ebi41BillerType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "BillersInvoiceRecipientID")
@Nonnull
public JAXBElement createBillersInvoiceRecipientID(
@Nullable
final String value) {
return new JAXBElement(_BillersInvoiceRecipientID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "BillersOrderingPartyID")
@Nonnull
public JAXBElement createBillersOrderingPartyID(
@Nullable
final String value) {
return new JAXBElement(_BillersOrderingPartyID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41OrderReferenceDetailType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41OrderReferenceDetailType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "BillersOrderReference")
@Nonnull
public JAXBElement createBillersOrderReference(
@Nullable
final Ebi41OrderReferenceDetailType value) {
return new JAXBElement(_BillersOrderReference_QNAME, Ebi41OrderReferenceDetailType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Boxes")
@Nonnull
public JAXBElement createBoxes(
@Nullable
final BigInteger value) {
return new JAXBElement(_Boxes_QNAME, BigInteger.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41CancelledOriginalDocumentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41CancelledOriginalDocumentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "CancelledOriginalDocument")
@Nonnull
public JAXBElement createCancelledOriginalDocument(
@Nullable
final Ebi41CancelledOriginalDocumentType value) {
return new JAXBElement(_CancelledOriginalDocument_QNAME, Ebi41CancelledOriginalDocumentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "ChargeNumber")
@Nonnull
public JAXBElement createChargeNumber(
@Nullable
final String value) {
return new JAXBElement(_ChargeNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41ClassificationType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41ClassificationType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Classification")
@Nonnull
public JAXBElement createClassification(
@Nullable
final Ebi41ClassificationType value) {
return new JAXBElement(_Classification_QNAME, Ebi41ClassificationType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Color")
@Nonnull
public JAXBElement createColor(
@Nullable
final String value) {
return new JAXBElement(_Color_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Comment")
@Nonnull
public JAXBElement createComment(
@Nullable
final String value) {
return new JAXBElement(_Comment_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "ConsolidatorsBillerID")
@Nonnull
public JAXBElement createConsolidatorsBillerID(
@Nullable
final String value) {
return new JAXBElement(_ConsolidatorsBillerID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Contact")
@Nonnull
public JAXBElement createContact(
@Nullable
final String value) {
return new JAXBElement(_Contact_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41CountryType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41CountryType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Country")
@Nonnull
public JAXBElement createCountry(
@Nullable
final Ebi41CountryType value) {
return new JAXBElement(_Country_QNAME, Ebi41CountryType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "CreditorID")
@Nonnull
public JAXBElement createCreditorID(
@Nullable
final String value) {
return new JAXBElement(_CreditorID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Date")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_Date_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "DebitCollectionDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createDebitCollectionDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_DebitCollectionDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41DeliveryType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41DeliveryType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Delivery")
@Nonnull
public JAXBElement createDelivery(
@Nullable
final Ebi41DeliveryType value) {
return new JAXBElement(_Delivery_QNAME, Ebi41DeliveryType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "DeliveryID")
@Nonnull
public JAXBElement createDeliveryID(
@Nullable
final String value) {
return new JAXBElement(_DeliveryID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Description")
@Nonnull
public JAXBElement createDescription(
@Nullable
final String value) {
return new JAXBElement(_Description_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41DetailsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41DetailsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Details")
@Nonnull
public JAXBElement createDetails(
@Nullable
final Ebi41DetailsType value) {
return new JAXBElement(_Details_QNAME, Ebi41DetailsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41DirectDebitType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41DirectDebitType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "DirectDebit")
@Nonnull
public JAXBElement createDirectDebit(
@Nullable
final Ebi41DirectDebitType value) {
return new JAXBElement(_DirectDebit_QNAME, Ebi41DirectDebitType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41DiscountType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41DiscountType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Discount")
@Nonnull
public JAXBElement createDiscount(
@Nullable
final Ebi41DiscountType value) {
return new JAXBElement(_Discount_QNAME, Ebi41DiscountType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Boolean }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Boolean }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "DiscountFlag")
@Nonnull
public JAXBElement createDiscountFlag(
@Nullable
final Boolean value) {
return new JAXBElement(_DiscountFlag_QNAME, Boolean.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41DocumentTypeType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41DocumentTypeType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "DocumentType")
@Nonnull
public JAXBElement createDocumentType(
@Nullable
final Ebi41DocumentTypeType value) {
return new JAXBElement(_DocumentType_QNAME, Ebi41DocumentTypeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "DueDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createDueDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_DueDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Email")
@Nonnull
public JAXBElement createEmail(
@Nullable
final String value) {
return new JAXBElement(_Email_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "FooterDescription")
@Nonnull
public JAXBElement createFooterDescription(
@Nullable
final String value) {
return new JAXBElement(_FooterDescription_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "FromDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createFromDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_FromDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41FurtherIdentificationType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41FurtherIdentificationType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "FurtherIdentification")
@Nonnull
public JAXBElement createFurtherIdentification(
@Nullable
final Ebi41FurtherIdentificationType value) {
return new JAXBElement(_FurtherIdentification_QNAME, Ebi41FurtherIdentificationType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "HeaderDescription")
@Nonnull
public JAXBElement createHeaderDescription(
@Nullable
final String value) {
return new JAXBElement(_HeaderDescription_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "IBAN")
@Nonnull
public JAXBElement createIBAN(
@Nullable
final String value) {
return new JAXBElement(_IBAN_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "InvoiceDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createInvoiceDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_InvoiceDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "InvoiceNumber")
@Nonnull
public JAXBElement createInvoiceNumber(
@Nullable
final String value) {
return new JAXBElement(_InvoiceNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41InvoiceRecipientType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41InvoiceRecipientType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "InvoiceRecipient")
@Nonnull
public JAXBElement createInvoiceRecipient(
@Nullable
final Ebi41InvoiceRecipientType value) {
return new JAXBElement(_InvoiceRecipient_QNAME, Ebi41InvoiceRecipientType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "InvoiceRecipientsBillerID")
@Nonnull
public JAXBElement createInvoiceRecipientsBillerID(
@Nullable
final String value) {
return new JAXBElement(_InvoiceRecipientsBillerID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41OrderReferenceDetailType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41OrderReferenceDetailType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "InvoiceRecipientsOrderReference")
@Nonnull
public JAXBElement createInvoiceRecipientsOrderReference(
@Nullable
final Ebi41OrderReferenceDetailType value) {
return new JAXBElement(_InvoiceRecipientsOrderReference_QNAME, Ebi41OrderReferenceDetailType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41VATItemType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41VATItemType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "VATItem")
@Nonnull
public JAXBElement createVATItem(
@Nullable
final Ebi41VATItemType value) {
return new JAXBElement(_VATItem_QNAME, Ebi41VATItemType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41ItemListType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41ItemListType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "ItemList")
@Nonnull
public JAXBElement createItemList(
@Nullable
final Ebi41ItemListType value) {
return new JAXBElement(_ItemList_QNAME, Ebi41ItemListType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "LayoutID")
@Nonnull
public JAXBElement createLayoutID(
@Nullable
final String value) {
return new JAXBElement(_LayoutID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "LineItemAmount")
@Nonnull
public JAXBElement createLineItemAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_LineItemAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41ListLineItemType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41ListLineItemType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "ListLineItem")
@Nonnull
public JAXBElement createListLineItem(
@Nullable
final Ebi41ListLineItemType value) {
return new JAXBElement(_ListLineItem_QNAME, Ebi41ListLineItemType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "LogoURL")
@Nonnull
public JAXBElement createLogoURL(
@Nullable
final String value) {
return new JAXBElement(_LogoURL_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "MandateReference")
@Nonnull
public JAXBElement createMandateReference(
@Nullable
final String value) {
return new JAXBElement(_MandateReference_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "MinimumPayment")
@Nonnull
public JAXBElement createMinimumPayment(
@Nullable
final BigDecimal value) {
return new JAXBElement(_MinimumPayment_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Name")
@Nonnull
public JAXBElement createName(
@Nullable
final String value) {
return new JAXBElement(_Name_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41NoPaymentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41NoPaymentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "NoPayment")
@Nonnull
public JAXBElement createNoPayment(
@Nullable
final Ebi41NoPaymentType value) {
return new JAXBElement(_NoPayment_QNAME, Ebi41NoPaymentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "OrderID")
@Nonnull
public JAXBElement createOrderID(
@Nullable
final String value) {
return new JAXBElement(_OrderID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41OrderingPartyType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41OrderingPartyType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "OrderingParty")
@Nonnull
public JAXBElement createOrderingParty(
@Nullable
final Ebi41OrderingPartyType value) {
return new JAXBElement(_OrderingParty_QNAME, Ebi41OrderingPartyType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "OrderPositionNumber")
@Nonnull
public JAXBElement createOrderPositionNumber(
@Nullable
final String value) {
return new JAXBElement(_OrderPositionNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41OrderReferenceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41OrderReferenceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "OrderReference")
@Nonnull
public JAXBElement createOrderReference(
@Nullable
final Ebi41OrderReferenceType value) {
return new JAXBElement(_OrderReference_QNAME, Ebi41OrderReferenceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41OtherTaxType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41OtherTaxType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "OtherTax")
@Nonnull
public JAXBElement createOtherTax(
@Nullable
final Ebi41OtherTaxType value) {
return new JAXBElement(_OtherTax_QNAME, Ebi41OtherTaxType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41OtherVATableTaxType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41OtherVATableTaxType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "OtherVATableTax")
@Nonnull
public JAXBElement createOtherVATableTax(
@Nullable
final Ebi41OtherVATableTaxType value) {
return new JAXBElement(_OtherVATableTax_QNAME, Ebi41OtherVATableTaxType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41OtherVATableTaxBaseType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41OtherVATableTaxBaseType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "OtherVATableTaxListLineItem")
@Nonnull
public JAXBElement createOtherVATableTaxListLineItem(
@Nullable
final Ebi41OtherVATableTaxBaseType value) {
return new JAXBElement(_OtherVATableTaxListLineItem_QNAME, Ebi41OtherVATableTaxBaseType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "PayableAmount")
@Nonnull
public JAXBElement createPayableAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_PayableAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41PaymentConditionsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41PaymentConditionsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "PaymentConditions")
@Nonnull
public JAXBElement createPaymentConditions(
@Nullable
final Ebi41PaymentConditionsType value) {
return new JAXBElement(_PaymentConditions_QNAME, Ebi41PaymentConditionsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "PaymentDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createPaymentDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_PaymentDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41PaymentMethodType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41PaymentMethodType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "PaymentMethod")
@Nonnull
public JAXBElement createPaymentMethod(
@Nullable
final Ebi41PaymentMethodType value) {
return new JAXBElement(_PaymentMethod_QNAME, Ebi41PaymentMethodType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41PaymentReferenceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41PaymentReferenceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "PaymentReference")
@Nonnull
public JAXBElement createPaymentReference(
@Nullable
final Ebi41PaymentReferenceType value) {
return new JAXBElement(_PaymentReference_QNAME, Ebi41PaymentReferenceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Percentage")
@Nonnull
public JAXBElement createPercentage(
@Nullable
final BigDecimal value) {
return new JAXBElement(_Percentage_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41PeriodType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41PeriodType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Period")
@Nonnull
public JAXBElement createPeriod(
@Nullable
final Ebi41PeriodType value) {
return new JAXBElement(_Period_QNAME, Ebi41PeriodType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Phone")
@Nonnull
public JAXBElement createPhone(
@Nullable
final String value) {
return new JAXBElement(_Phone_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "POBox")
@Nonnull
public JAXBElement createPOBox(
@Nullable
final String value) {
return new JAXBElement(_POBox_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "PositionNumber")
@Nonnull
public JAXBElement createPositionNumber(
@Nullable
final BigInteger value) {
return new JAXBElement(_PositionNumber_QNAME, BigInteger.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41PresentationDetailsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41PresentationDetailsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "PresentationDetails")
@Nonnull
public JAXBElement createPresentationDetails(
@Nullable
final Ebi41PresentationDetailsType value) {
return new JAXBElement(_PresentationDetails_QNAME, Ebi41PresentationDetailsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41UnitType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41UnitType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Quantity")
@Nonnull
public JAXBElement createQuantity(
@Nullable
final Ebi41UnitType value) {
return new JAXBElement(_Quantity_QNAME, Ebi41UnitType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41ReasonType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41ReasonType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Reason")
@Nonnull
public JAXBElement createReason(
@Nullable
final Ebi41ReasonType value) {
return new JAXBElement(_Reason_QNAME, Ebi41ReasonType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41ReductionAndSurchargeType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41ReductionAndSurchargeType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Reduction")
@Nonnull
public JAXBElement createReduction(
@Nullable
final Ebi41ReductionAndSurchargeType value) {
return new JAXBElement(_Reduction_QNAME, Ebi41ReductionAndSurchargeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41ReductionAndSurchargeDetailsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41ReductionAndSurchargeDetailsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "ReductionAndSurchargeDetails")
@Nonnull
public JAXBElement createReductionAndSurchargeDetails(
@Nullable
final Ebi41ReductionAndSurchargeDetailsType value) {
return new JAXBElement(_ReductionAndSurchargeDetails_QNAME, Ebi41ReductionAndSurchargeDetailsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41ReductionAndSurchargeListLineItemDetailsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41ReductionAndSurchargeListLineItemDetailsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "ReductionAndSurchargeListLineItemDetails")
@Nonnull
public JAXBElement createReductionAndSurchargeListLineItemDetails(
@Nullable
final Ebi41ReductionAndSurchargeListLineItemDetailsType value) {
return new JAXBElement(_ReductionAndSurchargeListLineItemDetails_QNAME, Ebi41ReductionAndSurchargeListLineItemDetailsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41ReductionAndSurchargeBaseType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41ReductionAndSurchargeBaseType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "ReductionListLineItem")
@Nonnull
public JAXBElement createReductionListLineItem(
@Nullable
final Ebi41ReductionAndSurchargeBaseType value) {
return new JAXBElement(_ReductionListLineItem_QNAME, Ebi41ReductionAndSurchargeBaseType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "ReferenceDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createReferenceDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_ReferenceDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41RelatedDocumentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41RelatedDocumentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "RelatedDocument")
@Nonnull
public JAXBElement createRelatedDocument(
@Nullable
final Ebi41RelatedDocumentType value) {
return new JAXBElement(_RelatedDocument_QNAME, Ebi41RelatedDocumentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Salutation")
@Nonnull
public JAXBElement createSalutation(
@Nullable
final String value) {
return new JAXBElement(_Salutation_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41SEPADirectDebitType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41SEPADirectDebitType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "SEPADirectDebit")
@Nonnull
public JAXBElement createSEPADirectDebit(
@Nullable
final Ebi41SEPADirectDebitType value) {
return new JAXBElement(_SEPADirectDebit_QNAME, Ebi41SEPADirectDebitType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "SerialNumber")
@Nonnull
public JAXBElement createSerialNumber(
@Nullable
final String value) {
return new JAXBElement(_SerialNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Size")
@Nonnull
public JAXBElement createSize(
@Nullable
final String value) {
return new JAXBElement(_Size_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Street")
@Nonnull
public JAXBElement createStreet(
@Nullable
final String value) {
return new JAXBElement(_Street_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "SubOrganizationID")
@Nonnull
public JAXBElement createSubOrganizationID(
@Nullable
final String value) {
return new JAXBElement(_SubOrganizationID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Boolean }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Boolean }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "SuppressZero")
@Nonnull
public JAXBElement createSuppressZero(
@Nullable
final Boolean value) {
return new JAXBElement(_SuppressZero_QNAME, Boolean.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41ReductionAndSurchargeType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41ReductionAndSurchargeType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Surcharge")
@Nonnull
public JAXBElement createSurcharge(
@Nullable
final Ebi41ReductionAndSurchargeType value) {
return new JAXBElement(_Surcharge_QNAME, Ebi41ReductionAndSurchargeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41ReductionAndSurchargeBaseType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41ReductionAndSurchargeBaseType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "SurchargeListLineItem")
@Nonnull
public JAXBElement createSurchargeListLineItem(
@Nullable
final Ebi41ReductionAndSurchargeBaseType value) {
return new JAXBElement(_SurchargeListLineItem_QNAME, Ebi41ReductionAndSurchargeBaseType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41TaxType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41TaxType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Tax")
@Nonnull
public JAXBElement createTax(
@Nullable
final Ebi41TaxType value) {
return new JAXBElement(_Tax_QNAME, Ebi41TaxType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "TaxedAmount")
@Nonnull
public JAXBElement createTaxedAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_TaxedAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41TaxExemptionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41TaxExemptionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "TaxExemption")
@Nonnull
public JAXBElement createTaxExemption(
@Nullable
final Ebi41TaxExemptionType value) {
return new JAXBElement(_TaxExemption_QNAME, Ebi41TaxExemptionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "ToDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createToDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_ToDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "TotalGrossAmount")
@Nonnull
public JAXBElement createTotalGrossAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_TotalGrossAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Town")
@Nonnull
public JAXBElement createTown(
@Nullable
final String value) {
return new JAXBElement(_Town_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41SEPADirectDebitTypeType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41SEPADirectDebitTypeType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Type")
@Nonnull
public JAXBElement createType(
@Nullable
final Ebi41SEPADirectDebitTypeType value) {
return new JAXBElement(_Type_QNAME, Ebi41SEPADirectDebitTypeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41UnitPriceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41UnitPriceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "UnitPrice")
@Nonnull
public JAXBElement createUnitPrice(
@Nullable
final Ebi41UnitPriceType value) {
return new JAXBElement(_UnitPrice_QNAME, Ebi41UnitPriceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41UniversalBankTransactionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41UniversalBankTransactionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "UniversalBankTransaction")
@Nonnull
public JAXBElement createUniversalBankTransaction(
@Nullable
final Ebi41UniversalBankTransactionType value) {
return new JAXBElement(_UniversalBankTransaction_QNAME, Ebi41UniversalBankTransactionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "URL")
@Nonnull
public JAXBElement createURL(
@Nullable
final String value) {
return new JAXBElement(_URL_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41VATType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41VATType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "VAT")
@Nonnull
public JAXBElement createVAT(
@Nullable
final Ebi41VATType value) {
return new JAXBElement(_VAT_QNAME, Ebi41VATType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "VATIdentificationNumber")
@Nonnull
public JAXBElement createVATIdentificationNumber(
@Nullable
final String value) {
return new JAXBElement(_VATIdentificationNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41VATRateType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41VATRateType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "VATRate")
@Nonnull
public JAXBElement createVATRate(
@Nullable
final Ebi41VATRateType value) {
return new JAXBElement(_VATRate_QNAME, Ebi41VATRateType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41UnitType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41UnitType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "Weight")
@Nonnull
public JAXBElement createWeight(
@Nullable
final Ebi41UnitType value) {
return new JAXBElement(_Weight_QNAME, Ebi41UnitType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/", name = "ZIP")
@Nonnull
public JAXBElement createZIP(
@Nullable
final String value) {
return new JAXBElement(_ZIP_QNAME, String.class, null, value);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy