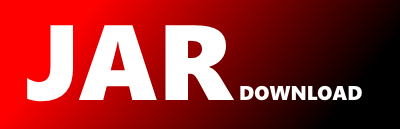
com.helger.ebinterface.v41.extensions.sv.ObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-ebinterface Show documentation
Show all versions of ph-ebinterface Show documentation
ebInterface wrapper library to easily read and write ebInterface documents
package com.helger.ebinterface.v41.extensions.sv;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.helger.ebinterface.v41.extensions.sv package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _BillerExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p1/extensions/sv", "BillerExtension");
public final static QName _DeliveryExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p1/extensions/sv", "DeliveryExtension");
public final static QName _DetailsExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p1/extensions/sv", "DetailsExtension");
public final static QName _InvoiceRecipientExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p1/extensions/sv", "InvoiceRecipientExtension");
public final static QName _InvoiceRootExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p1/extensions/sv", "InvoiceRootExtension");
public final static QName _ListLineItemExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p1/extensions/sv", "ListLineItemExtension");
public final static QName _OrderingPartyExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p1/extensions/sv", "OrderingPartyExtension");
public final static QName _PaymentConditionsExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p1/extensions/sv", "PaymentConditionsExtension");
public final static QName _PaymentMethodExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p1/extensions/sv", "PaymentMethodExtension");
public final static QName _ReductionAndSurchargeDetailsExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p1/extensions/sv", "ReductionAndSurchargeDetailsExtension");
public final static QName _PresentationDetailsExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p1/extensions/sv", "PresentationDetailsExtension");
public final static QName _TaxExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p1/extensions/sv", "TaxExtension");
public final static QName _BeneficiarySocialInsuranceNumber_QNAME = new QName("http://www.ebinterface.at/schema/4p1/extensions/sv", "BeneficiarySocialInsuranceNumber");
public final static QName _BillersContractPartnerNumber_QNAME = new QName("http://www.ebinterface.at/schema/4p1/extensions/sv", "BillersContractPartnerNumber");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.helger.ebinterface.v41.extensions.sv
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link Ebi41BillerExtensionType }
*
* @return
* The created Ebi41BillerExtensionType object and never null
.
*/
@Nonnull
public Ebi41BillerExtensionType createEbi41BillerExtensionType() {
return new Ebi41BillerExtensionType();
}
/**
* Create an instance of {@link Ebi41DeliveryExtensionType }
*
* @return
* The created Ebi41DeliveryExtensionType object and never null
.
*/
@Nonnull
public Ebi41DeliveryExtensionType createEbi41DeliveryExtensionType() {
return new Ebi41DeliveryExtensionType();
}
/**
* Create an instance of {@link Ebi41DetailsExtensionType }
*
* @return
* The created Ebi41DetailsExtensionType object and never null
.
*/
@Nonnull
public Ebi41DetailsExtensionType createEbi41DetailsExtensionType() {
return new Ebi41DetailsExtensionType();
}
/**
* Create an instance of {@link Ebi41InvoiceRecipientExtensionType }
*
* @return
* The created Ebi41InvoiceRecipientExtensionType object and never null
.
*/
@Nonnull
public Ebi41InvoiceRecipientExtensionType createEbi41InvoiceRecipientExtensionType() {
return new Ebi41InvoiceRecipientExtensionType();
}
/**
* Create an instance of {@link Ebi41InvoiceRootExtensionType }
*
* @return
* The created Ebi41InvoiceRootExtensionType object and never null
.
*/
@Nonnull
public Ebi41InvoiceRootExtensionType createEbi41InvoiceRootExtensionType() {
return new Ebi41InvoiceRootExtensionType();
}
/**
* Create an instance of {@link Ebi41ListLineItemExtensionType }
*
* @return
* The created Ebi41ListLineItemExtensionType object and never null
.
*/
@Nonnull
public Ebi41ListLineItemExtensionType createEbi41ListLineItemExtensionType() {
return new Ebi41ListLineItemExtensionType();
}
/**
* Create an instance of {@link Ebi41OrderingPartyExtensionType }
*
* @return
* The created Ebi41OrderingPartyExtensionType object and never null
.
*/
@Nonnull
public Ebi41OrderingPartyExtensionType createEbi41OrderingPartyExtensionType() {
return new Ebi41OrderingPartyExtensionType();
}
/**
* Create an instance of {@link Ebi41PaymentConditionsExtensionType }
*
* @return
* The created Ebi41PaymentConditionsExtensionType object and never null
.
*/
@Nonnull
public Ebi41PaymentConditionsExtensionType createEbi41PaymentConditionsExtensionType() {
return new Ebi41PaymentConditionsExtensionType();
}
/**
* Create an instance of {@link Ebi41PaymentMethodExtensionType }
*
* @return
* The created Ebi41PaymentMethodExtensionType object and never null
.
*/
@Nonnull
public Ebi41PaymentMethodExtensionType createEbi41PaymentMethodExtensionType() {
return new Ebi41PaymentMethodExtensionType();
}
/**
* Create an instance of {@link Ebi41ReductionAndSurchargeDetailsExtensionType }
*
* @return
* The created Ebi41ReductionAndSurchargeDetailsExtensionType object and never null
.
*/
@Nonnull
public Ebi41ReductionAndSurchargeDetailsExtensionType createEbi41ReductionAndSurchargeDetailsExtensionType() {
return new Ebi41ReductionAndSurchargeDetailsExtensionType();
}
/**
* Create an instance of {@link Ebi41PresentationDetailsExtensionType }
*
* @return
* The created Ebi41PresentationDetailsExtensionType object and never null
.
*/
@Nonnull
public Ebi41PresentationDetailsExtensionType createEbi41PresentationDetailsExtensionType() {
return new Ebi41PresentationDetailsExtensionType();
}
/**
* Create an instance of {@link Ebi41TaxExtensionType }
*
* @return
* The created Ebi41TaxExtensionType object and never null
.
*/
@Nonnull
public Ebi41TaxExtensionType createEbi41TaxExtensionType() {
return new Ebi41TaxExtensionType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41BillerExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41BillerExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/extensions/sv", name = "BillerExtension")
@Nonnull
public JAXBElement createBillerExtension(
@Nullable
final Ebi41BillerExtensionType value) {
return new JAXBElement(_BillerExtension_QNAME, Ebi41BillerExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41DeliveryExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41DeliveryExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/extensions/sv", name = "DeliveryExtension")
@Nonnull
public JAXBElement createDeliveryExtension(
@Nullable
final Ebi41DeliveryExtensionType value) {
return new JAXBElement(_DeliveryExtension_QNAME, Ebi41DeliveryExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41DetailsExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41DetailsExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/extensions/sv", name = "DetailsExtension")
@Nonnull
public JAXBElement createDetailsExtension(
@Nullable
final Ebi41DetailsExtensionType value) {
return new JAXBElement(_DetailsExtension_QNAME, Ebi41DetailsExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41InvoiceRecipientExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41InvoiceRecipientExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/extensions/sv", name = "InvoiceRecipientExtension")
@Nonnull
public JAXBElement createInvoiceRecipientExtension(
@Nullable
final Ebi41InvoiceRecipientExtensionType value) {
return new JAXBElement(_InvoiceRecipientExtension_QNAME, Ebi41InvoiceRecipientExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41InvoiceRootExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41InvoiceRootExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/extensions/sv", name = "InvoiceRootExtension")
@Nonnull
public JAXBElement createInvoiceRootExtension(
@Nullable
final Ebi41InvoiceRootExtensionType value) {
return new JAXBElement(_InvoiceRootExtension_QNAME, Ebi41InvoiceRootExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41ListLineItemExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41ListLineItemExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/extensions/sv", name = "ListLineItemExtension")
@Nonnull
public JAXBElement createListLineItemExtension(
@Nullable
final Ebi41ListLineItemExtensionType value) {
return new JAXBElement(_ListLineItemExtension_QNAME, Ebi41ListLineItemExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41OrderingPartyExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41OrderingPartyExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/extensions/sv", name = "OrderingPartyExtension")
@Nonnull
public JAXBElement createOrderingPartyExtension(
@Nullable
final Ebi41OrderingPartyExtensionType value) {
return new JAXBElement(_OrderingPartyExtension_QNAME, Ebi41OrderingPartyExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41PaymentConditionsExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41PaymentConditionsExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/extensions/sv", name = "PaymentConditionsExtension")
@Nonnull
public JAXBElement createPaymentConditionsExtension(
@Nullable
final Ebi41PaymentConditionsExtensionType value) {
return new JAXBElement(_PaymentConditionsExtension_QNAME, Ebi41PaymentConditionsExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41PaymentMethodExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41PaymentMethodExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/extensions/sv", name = "PaymentMethodExtension")
@Nonnull
public JAXBElement createPaymentMethodExtension(
@Nullable
final Ebi41PaymentMethodExtensionType value) {
return new JAXBElement(_PaymentMethodExtension_QNAME, Ebi41PaymentMethodExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41ReductionAndSurchargeDetailsExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41ReductionAndSurchargeDetailsExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/extensions/sv", name = "ReductionAndSurchargeDetailsExtension")
@Nonnull
public JAXBElement createReductionAndSurchargeDetailsExtension(
@Nullable
final Ebi41ReductionAndSurchargeDetailsExtensionType value) {
return new JAXBElement(_ReductionAndSurchargeDetailsExtension_QNAME, Ebi41ReductionAndSurchargeDetailsExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41PresentationDetailsExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41PresentationDetailsExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/extensions/sv", name = "PresentationDetailsExtension")
@Nonnull
public JAXBElement createPresentationDetailsExtension(
@Nullable
final Ebi41PresentationDetailsExtensionType value) {
return new JAXBElement(_PresentationDetailsExtension_QNAME, Ebi41PresentationDetailsExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi41TaxExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi41TaxExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/extensions/sv", name = "TaxExtension")
@Nonnull
public JAXBElement createTaxExtension(
@Nullable
final Ebi41TaxExtensionType value) {
return new JAXBElement(_TaxExtension_QNAME, Ebi41TaxExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/extensions/sv", name = "BeneficiarySocialInsuranceNumber")
@Nonnull
public JAXBElement createBeneficiarySocialInsuranceNumber(
@Nullable
final String value) {
return new JAXBElement(_BeneficiarySocialInsuranceNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p1/extensions/sv", name = "BillersContractPartnerNumber")
@Nonnull
public JAXBElement createBillersContractPartnerNumber(
@Nullable
final String value) {
return new JAXBElement(_BillersContractPartnerNumber_QNAME, String.class, null, value);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy