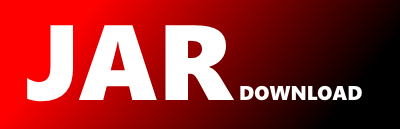
com.helger.ebinterface.v42.extensions.sv.ObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-ebinterface Show documentation
Show all versions of ph-ebinterface Show documentation
ebInterface wrapper library to easily read and write ebInterface documents
package com.helger.ebinterface.v42.extensions.sv;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.helger.ebinterface.v42.extensions.sv package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _BillerExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p2/extensions/sv", "BillerExtension");
public final static QName _DeliveryExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p2/extensions/sv", "DeliveryExtension");
public final static QName _DetailsExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p2/extensions/sv", "DetailsExtension");
public final static QName _InvoiceRecipientExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p2/extensions/sv", "InvoiceRecipientExtension");
public final static QName _InvoiceRootExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p2/extensions/sv", "InvoiceRootExtension");
public final static QName _ListLineItemExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p2/extensions/sv", "ListLineItemExtension");
public final static QName _OrderingPartyExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p2/extensions/sv", "OrderingPartyExtension");
public final static QName _PaymentConditionsExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p2/extensions/sv", "PaymentConditionsExtension");
public final static QName _PaymentMethodExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p2/extensions/sv", "PaymentMethodExtension");
public final static QName _ReductionAndSurchargeDetailsExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p2/extensions/sv", "ReductionAndSurchargeDetailsExtension");
public final static QName _PresentationDetailsExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p2/extensions/sv", "PresentationDetailsExtension");
public final static QName _TaxExtension_QNAME = new QName("http://www.ebinterface.at/schema/4p2/extensions/sv", "TaxExtension");
public final static QName _BeneficiarySocialInsuranceNumber_QNAME = new QName("http://www.ebinterface.at/schema/4p2/extensions/sv", "BeneficiarySocialInsuranceNumber");
public final static QName _BillersContractPartnerNumber_QNAME = new QName("http://www.ebinterface.at/schema/4p2/extensions/sv", "BillersContractPartnerNumber");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.helger.ebinterface.v42.extensions.sv
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link Ebi42BillerExtensionType }
*
* @return
* The created Ebi42BillerExtensionType object and never null
.
*/
@Nonnull
public Ebi42BillerExtensionType createEbi42BillerExtensionType() {
return new Ebi42BillerExtensionType();
}
/**
* Create an instance of {@link Ebi42DeliveryExtensionType }
*
* @return
* The created Ebi42DeliveryExtensionType object and never null
.
*/
@Nonnull
public Ebi42DeliveryExtensionType createEbi42DeliveryExtensionType() {
return new Ebi42DeliveryExtensionType();
}
/**
* Create an instance of {@link Ebi42DetailsExtensionType }
*
* @return
* The created Ebi42DetailsExtensionType object and never null
.
*/
@Nonnull
public Ebi42DetailsExtensionType createEbi42DetailsExtensionType() {
return new Ebi42DetailsExtensionType();
}
/**
* Create an instance of {@link Ebi42InvoiceRecipientExtensionType }
*
* @return
* The created Ebi42InvoiceRecipientExtensionType object and never null
.
*/
@Nonnull
public Ebi42InvoiceRecipientExtensionType createEbi42InvoiceRecipientExtensionType() {
return new Ebi42InvoiceRecipientExtensionType();
}
/**
* Create an instance of {@link Ebi42InvoiceRootExtensionType }
*
* @return
* The created Ebi42InvoiceRootExtensionType object and never null
.
*/
@Nonnull
public Ebi42InvoiceRootExtensionType createEbi42InvoiceRootExtensionType() {
return new Ebi42InvoiceRootExtensionType();
}
/**
* Create an instance of {@link Ebi42ListLineItemExtensionType }
*
* @return
* The created Ebi42ListLineItemExtensionType object and never null
.
*/
@Nonnull
public Ebi42ListLineItemExtensionType createEbi42ListLineItemExtensionType() {
return new Ebi42ListLineItemExtensionType();
}
/**
* Create an instance of {@link Ebi42OrderingPartyExtensionType }
*
* @return
* The created Ebi42OrderingPartyExtensionType object and never null
.
*/
@Nonnull
public Ebi42OrderingPartyExtensionType createEbi42OrderingPartyExtensionType() {
return new Ebi42OrderingPartyExtensionType();
}
/**
* Create an instance of {@link Ebi42PaymentConditionsExtensionType }
*
* @return
* The created Ebi42PaymentConditionsExtensionType object and never null
.
*/
@Nonnull
public Ebi42PaymentConditionsExtensionType createEbi42PaymentConditionsExtensionType() {
return new Ebi42PaymentConditionsExtensionType();
}
/**
* Create an instance of {@link Ebi42PaymentMethodExtensionType }
*
* @return
* The created Ebi42PaymentMethodExtensionType object and never null
.
*/
@Nonnull
public Ebi42PaymentMethodExtensionType createEbi42PaymentMethodExtensionType() {
return new Ebi42PaymentMethodExtensionType();
}
/**
* Create an instance of {@link Ebi42ReductionAndSurchargeDetailsExtensionType }
*
* @return
* The created Ebi42ReductionAndSurchargeDetailsExtensionType object and never null
.
*/
@Nonnull
public Ebi42ReductionAndSurchargeDetailsExtensionType createEbi42ReductionAndSurchargeDetailsExtensionType() {
return new Ebi42ReductionAndSurchargeDetailsExtensionType();
}
/**
* Create an instance of {@link Ebi42PresentationDetailsExtensionType }
*
* @return
* The created Ebi42PresentationDetailsExtensionType object and never null
.
*/
@Nonnull
public Ebi42PresentationDetailsExtensionType createEbi42PresentationDetailsExtensionType() {
return new Ebi42PresentationDetailsExtensionType();
}
/**
* Create an instance of {@link Ebi42TaxExtensionType }
*
* @return
* The created Ebi42TaxExtensionType object and never null
.
*/
@Nonnull
public Ebi42TaxExtensionType createEbi42TaxExtensionType() {
return new Ebi42TaxExtensionType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi42BillerExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi42BillerExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p2/extensions/sv", name = "BillerExtension")
@Nonnull
public JAXBElement createBillerExtension(
@Nullable
final Ebi42BillerExtensionType value) {
return new JAXBElement(_BillerExtension_QNAME, Ebi42BillerExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi42DeliveryExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi42DeliveryExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p2/extensions/sv", name = "DeliveryExtension")
@Nonnull
public JAXBElement createDeliveryExtension(
@Nullable
final Ebi42DeliveryExtensionType value) {
return new JAXBElement(_DeliveryExtension_QNAME, Ebi42DeliveryExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi42DetailsExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi42DetailsExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p2/extensions/sv", name = "DetailsExtension")
@Nonnull
public JAXBElement createDetailsExtension(
@Nullable
final Ebi42DetailsExtensionType value) {
return new JAXBElement(_DetailsExtension_QNAME, Ebi42DetailsExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi42InvoiceRecipientExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi42InvoiceRecipientExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p2/extensions/sv", name = "InvoiceRecipientExtension")
@Nonnull
public JAXBElement createInvoiceRecipientExtension(
@Nullable
final Ebi42InvoiceRecipientExtensionType value) {
return new JAXBElement(_InvoiceRecipientExtension_QNAME, Ebi42InvoiceRecipientExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi42InvoiceRootExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi42InvoiceRootExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p2/extensions/sv", name = "InvoiceRootExtension")
@Nonnull
public JAXBElement createInvoiceRootExtension(
@Nullable
final Ebi42InvoiceRootExtensionType value) {
return new JAXBElement(_InvoiceRootExtension_QNAME, Ebi42InvoiceRootExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi42ListLineItemExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi42ListLineItemExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p2/extensions/sv", name = "ListLineItemExtension")
@Nonnull
public JAXBElement createListLineItemExtension(
@Nullable
final Ebi42ListLineItemExtensionType value) {
return new JAXBElement(_ListLineItemExtension_QNAME, Ebi42ListLineItemExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi42OrderingPartyExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi42OrderingPartyExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p2/extensions/sv", name = "OrderingPartyExtension")
@Nonnull
public JAXBElement createOrderingPartyExtension(
@Nullable
final Ebi42OrderingPartyExtensionType value) {
return new JAXBElement(_OrderingPartyExtension_QNAME, Ebi42OrderingPartyExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi42PaymentConditionsExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi42PaymentConditionsExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p2/extensions/sv", name = "PaymentConditionsExtension")
@Nonnull
public JAXBElement createPaymentConditionsExtension(
@Nullable
final Ebi42PaymentConditionsExtensionType value) {
return new JAXBElement(_PaymentConditionsExtension_QNAME, Ebi42PaymentConditionsExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi42PaymentMethodExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi42PaymentMethodExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p2/extensions/sv", name = "PaymentMethodExtension")
@Nonnull
public JAXBElement createPaymentMethodExtension(
@Nullable
final Ebi42PaymentMethodExtensionType value) {
return new JAXBElement(_PaymentMethodExtension_QNAME, Ebi42PaymentMethodExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi42ReductionAndSurchargeDetailsExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi42ReductionAndSurchargeDetailsExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p2/extensions/sv", name = "ReductionAndSurchargeDetailsExtension")
@Nonnull
public JAXBElement createReductionAndSurchargeDetailsExtension(
@Nullable
final Ebi42ReductionAndSurchargeDetailsExtensionType value) {
return new JAXBElement(_ReductionAndSurchargeDetailsExtension_QNAME, Ebi42ReductionAndSurchargeDetailsExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi42PresentationDetailsExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi42PresentationDetailsExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p2/extensions/sv", name = "PresentationDetailsExtension")
@Nonnull
public JAXBElement createPresentationDetailsExtension(
@Nullable
final Ebi42PresentationDetailsExtensionType value) {
return new JAXBElement(_PresentationDetailsExtension_QNAME, Ebi42PresentationDetailsExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi42TaxExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi42TaxExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p2/extensions/sv", name = "TaxExtension")
@Nonnull
public JAXBElement createTaxExtension(
@Nullable
final Ebi42TaxExtensionType value) {
return new JAXBElement(_TaxExtension_QNAME, Ebi42TaxExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p2/extensions/sv", name = "BeneficiarySocialInsuranceNumber")
@Nonnull
public JAXBElement createBeneficiarySocialInsuranceNumber(
@Nullable
final String value) {
return new JAXBElement(_BeneficiarySocialInsuranceNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/4p2/extensions/sv", name = "BillersContractPartnerNumber")
@Nonnull
public JAXBElement createBillersContractPartnerNumber(
@Nullable
final String value) {
return new JAXBElement(_BillersContractPartnerNumber_QNAME, String.class, null, value);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy