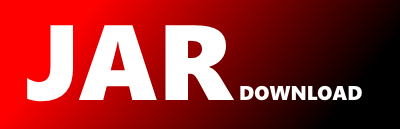
com.helger.ebinterface.v60.ObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-ebinterface Show documentation
Show all versions of ph-ebinterface Show documentation
ebInterface wrapper library to easily read and write ebInterface documents
package com.helger.ebinterface.v60;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.time.LocalDate;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
import com.helger.jaxb.adapter.AdapterLocalDate;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.helger.ebinterface.v60 package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _Invoice_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Invoice");
public final static QName _AccountingArea_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "AccountingArea");
public final static QName _AccountingCurrencyAmount_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "AccountingCurrencyAmount");
public final static QName _AdditionalInformation_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "AdditionalInformation");
public final static QName _Address_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Address");
public final static QName _AddressIdentifier_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "AddressIdentifier");
public final static QName _Amount_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Amount");
public final static QName _ArticleNumber_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "ArticleNumber");
public final static QName _BankAccountNr_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "BankAccountNr");
public final static QName _BankAccountOwner_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "BankAccountOwner");
public final static QName _BankCode_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "BankCode");
public final static QName _BankName_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "BankName");
public final static QName _BaseAmount_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "BaseAmount");
public final static QName _BeneficiaryAccount_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "BeneficiaryAccount");
public final static QName _BIC_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "BIC");
public final static QName _Biller_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Biller");
public final static QName _BillersInvoiceRecipientID_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "BillersInvoiceRecipientID");
public final static QName _BillersOrderingPartyID_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "BillersOrderingPartyID");
public final static QName _BillersOrderReference_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "BillersOrderReference");
public final static QName _CancelledOriginalDocument_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "CancelledOriginalDocument");
public final static QName _CardHolderName_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "CardHolderName");
public final static QName _Classification_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Classification");
public final static QName _Comment_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Comment");
public final static QName _ConsolidatorsBillerID_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "ConsolidatorsBillerID");
public final static QName _Contact_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Contact");
public final static QName _Country_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Country");
public final static QName _CreditorID_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "CreditorID");
public final static QName _Currency_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Currency");
public final static QName _CurrencyExchangeInformation_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "CurrencyExchangeInformation");
public final static QName _Custom_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Custom");
public final static QName _Date_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Date");
public final static QName _DebitCollectionDate_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "DebitCollectionDate");
public final static QName _Delivery_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Delivery");
public final static QName _DeliveryID_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "DeliveryID");
public final static QName _Description_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Description");
public final static QName _Details_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Details");
public final static QName _Discount_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Discount");
public final static QName _DocumentType_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "DocumentType");
public final static QName _DueDate_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "DueDate");
public final static QName _Email_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Email");
public final static QName _ExchangeRate_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "ExchangeRate");
public final static QName _ExchangeRateDate_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "ExchangeRateDate");
public final static QName _Extension_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Extension");
public final static QName _FooterDescription_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "FooterDescription");
public final static QName _FromDate_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "FromDate");
public final static QName _FurtherIdentification_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "FurtherIdentification");
public final static QName _HeaderDescription_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "HeaderDescription");
public final static QName _IBAN_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "IBAN");
public final static QName _InvoiceDate_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "InvoiceDate");
public final static QName _InvoiceNumber_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "InvoiceNumber");
public final static QName _InvoiceRecipient_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "InvoiceRecipient");
public final static QName _InvoiceRecipientsBillerID_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "InvoiceRecipientsBillerID");
public final static QName _InvoiceRecipientsOrderReference_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "InvoiceRecipientsOrderReference");
public final static QName _ItemList_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "ItemList");
public final static QName _LineItemAmount_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "LineItemAmount");
public final static QName _ListLineItem_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "ListLineItem");
public final static QName _MandateReference_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "MandateReference");
public final static QName _MinimumPayment_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "MinimumPayment");
public final static QName _Name_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Name");
public final static QName _NoPayment_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "NoPayment");
public final static QName _OrderID_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "OrderID");
public final static QName _OrderingParty_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "OrderingParty");
public final static QName _OrderPositionNumber_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "OrderPositionNumber");
public final static QName _OrderReference_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "OrderReference");
public final static QName _OtherPayment_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "OtherPayment");
public final static QName _OtherTax_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "OtherTax");
public final static QName _OtherVATableTax_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "OtherVATableTax");
public final static QName _OtherVATableTaxListLineItem_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "OtherVATableTaxListLineItem");
public final static QName _PayableAmount_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "PayableAmount");
public final static QName _PaymentCard_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "PaymentCard");
public final static QName _PaymentConditions_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "PaymentConditions");
public final static QName _PaymentDate_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "PaymentDate");
public final static QName _PaymentMethod_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "PaymentMethod");
public final static QName _PaymentReference_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "PaymentReference");
public final static QName _Percentage_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Percentage");
public final static QName _Period_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Period");
public final static QName _Phone_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Phone");
public final static QName _POBox_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "POBox");
public final static QName _PositionNumber_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "PositionNumber");
public final static QName _PrepaidAmount_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "PrepaidAmount");
public final static QName _PrimaryAccountNumber_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "PrimaryAccountNumber");
public final static QName _Quantity_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Quantity");
public final static QName _Reduction_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Reduction");
public final static QName _ReductionAndSurchargeDetails_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "ReductionAndSurchargeDetails");
public final static QName _ReductionAndSurchargeListLineItemDetails_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "ReductionAndSurchargeListLineItemDetails");
public final static QName _ReductionListLineItem_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "ReductionListLineItem");
public final static QName _ReferenceDate_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "ReferenceDate");
public final static QName _RelatedDocument_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "RelatedDocument");
public final static QName _RoundingAmount_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "RoundingAmount");
public final static QName _Salutation_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Salutation");
public final static QName _SEPADirectDebit_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "SEPADirectDebit");
public final static QName _Street_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Street");
public final static QName _SubOrganizationID_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "SubOrganizationID");
public final static QName _Surcharge_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Surcharge");
public final static QName _SurchargeListLineItem_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "SurchargeListLineItem");
public final static QName _Tax_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Tax");
public final static QName _TaxableAmount_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "TaxableAmount");
public final static QName _TaxAmount_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "TaxAmount");
public final static QName _TaxID_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "TaxID");
public final static QName _TaxItem_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "TaxItem");
public final static QName _TaxPercent_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "TaxPercent");
public final static QName _ToDate_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "ToDate");
public final static QName _TotalGrossAmount_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "TotalGrossAmount");
public final static QName _Town_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Town");
public final static QName _TradingName_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "TradingName");
public final static QName _Type_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "Type");
public final static QName _UnitPrice_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "UnitPrice");
public final static QName _UniversalBankTransaction_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "UniversalBankTransaction");
public final static QName _VATIdentificationNumber_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "VATIdentificationNumber");
public final static QName _ZIP_QNAME = new QName("http://www.ebinterface.at/schema/6p0/", "ZIP");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.helger.ebinterface.v60
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link Ebi60InvoiceType }
*
* @return
* The created Ebi60InvoiceType object and never null
.
*/
@Nonnull
public Ebi60InvoiceType createEbi60InvoiceType() {
return new Ebi60InvoiceType();
}
/**
* Create an instance of {@link Ebi60AccountingCurrencyAmountType }
*
* @return
* The created Ebi60AccountingCurrencyAmountType object and never null
.
*/
@Nonnull
public Ebi60AccountingCurrencyAmountType createEbi60AccountingCurrencyAmountType() {
return new Ebi60AccountingCurrencyAmountType();
}
/**
* Create an instance of {@link Ebi60AdditionalInformationType }
*
* @return
* The created Ebi60AdditionalInformationType object and never null
.
*/
@Nonnull
public Ebi60AdditionalInformationType createEbi60AdditionalInformationType() {
return new Ebi60AdditionalInformationType();
}
/**
* Create an instance of {@link Ebi60AddressType }
*
* @return
* The created Ebi60AddressType object and never null
.
*/
@Nonnull
public Ebi60AddressType createEbi60AddressType() {
return new Ebi60AddressType();
}
/**
* Create an instance of {@link Ebi60AddressIdentifierType }
*
* @return
* The created Ebi60AddressIdentifierType object and never null
.
*/
@Nonnull
public Ebi60AddressIdentifierType createEbi60AddressIdentifierType() {
return new Ebi60AddressIdentifierType();
}
/**
* Create an instance of {@link Ebi60ArticleNumberType }
*
* @return
* The created Ebi60ArticleNumberType object and never null
.
*/
@Nonnull
public Ebi60ArticleNumberType createEbi60ArticleNumberType() {
return new Ebi60ArticleNumberType();
}
/**
* Create an instance of {@link Ebi60BankCodeType }
*
* @return
* The created Ebi60BankCodeType object and never null
.
*/
@Nonnull
public Ebi60BankCodeType createEbi60BankCodeType() {
return new Ebi60BankCodeType();
}
/**
* Create an instance of {@link Ebi60AccountType }
*
* @return
* The created Ebi60AccountType object and never null
.
*/
@Nonnull
public Ebi60AccountType createEbi60AccountType() {
return new Ebi60AccountType();
}
/**
* Create an instance of {@link Ebi60BillerType }
*
* @return
* The created Ebi60BillerType object and never null
.
*/
@Nonnull
public Ebi60BillerType createEbi60BillerType() {
return new Ebi60BillerType();
}
/**
* Create an instance of {@link Ebi60OrderReferenceDetailType }
*
* @return
* The created Ebi60OrderReferenceDetailType object and never null
.
*/
@Nonnull
public Ebi60OrderReferenceDetailType createEbi60OrderReferenceDetailType() {
return new Ebi60OrderReferenceDetailType();
}
/**
* Create an instance of {@link Ebi60CancelledOriginalDocumentType }
*
* @return
* The created Ebi60CancelledOriginalDocumentType object and never null
.
*/
@Nonnull
public Ebi60CancelledOriginalDocumentType createEbi60CancelledOriginalDocumentType() {
return new Ebi60CancelledOriginalDocumentType();
}
/**
* Create an instance of {@link Ebi60ClassificationType }
*
* @return
* The created Ebi60ClassificationType object and never null
.
*/
@Nonnull
public Ebi60ClassificationType createEbi60ClassificationType() {
return new Ebi60ClassificationType();
}
/**
* Create an instance of {@link Ebi60ContactType }
*
* @return
* The created Ebi60ContactType object and never null
.
*/
@Nonnull
public Ebi60ContactType createEbi60ContactType() {
return new Ebi60ContactType();
}
/**
* Create an instance of {@link Ebi60CountryType }
*
* @return
* The created Ebi60CountryType object and never null
.
*/
@Nonnull
public Ebi60CountryType createEbi60CountryType() {
return new Ebi60CountryType();
}
/**
* Create an instance of {@link Ebi60CurrencyExchangeInformationType }
*
* @return
* The created Ebi60CurrencyExchangeInformationType object and never null
.
*/
@Nonnull
public Ebi60CurrencyExchangeInformationType createEbi60CurrencyExchangeInformationType() {
return new Ebi60CurrencyExchangeInformationType();
}
/**
* Create an instance of {@link Ebi60CustomType }
*
* @return
* The created Ebi60CustomType object and never null
.
*/
@Nonnull
public Ebi60CustomType createEbi60CustomType() {
return new Ebi60CustomType();
}
/**
* Create an instance of {@link Ebi60DeliveryType }
*
* @return
* The created Ebi60DeliveryType object and never null
.
*/
@Nonnull
public Ebi60DeliveryType createEbi60DeliveryType() {
return new Ebi60DeliveryType();
}
/**
* Create an instance of {@link Ebi60DetailsType }
*
* @return
* The created Ebi60DetailsType object and never null
.
*/
@Nonnull
public Ebi60DetailsType createEbi60DetailsType() {
return new Ebi60DetailsType();
}
/**
* Create an instance of {@link Ebi60DiscountType }
*
* @return
* The created Ebi60DiscountType object and never null
.
*/
@Nonnull
public Ebi60DiscountType createEbi60DiscountType() {
return new Ebi60DiscountType();
}
/**
* Create an instance of {@link Ebi60ExtensionType }
*
* @return
* The created Ebi60ExtensionType object and never null
.
*/
@Nonnull
public Ebi60ExtensionType createEbi60ExtensionType() {
return new Ebi60ExtensionType();
}
/**
* Create an instance of {@link Ebi60FurtherIdentificationType }
*
* @return
* The created Ebi60FurtherIdentificationType object and never null
.
*/
@Nonnull
public Ebi60FurtherIdentificationType createEbi60FurtherIdentificationType() {
return new Ebi60FurtherIdentificationType();
}
/**
* Create an instance of {@link Ebi60InvoiceRecipientType }
*
* @return
* The created Ebi60InvoiceRecipientType object and never null
.
*/
@Nonnull
public Ebi60InvoiceRecipientType createEbi60InvoiceRecipientType() {
return new Ebi60InvoiceRecipientType();
}
/**
* Create an instance of {@link Ebi60ItemListType }
*
* @return
* The created Ebi60ItemListType object and never null
.
*/
@Nonnull
public Ebi60ItemListType createEbi60ItemListType() {
return new Ebi60ItemListType();
}
/**
* Create an instance of {@link Ebi60ListLineItemType }
*
* @return
* The created Ebi60ListLineItemType object and never null
.
*/
@Nonnull
public Ebi60ListLineItemType createEbi60ListLineItemType() {
return new Ebi60ListLineItemType();
}
/**
* Create an instance of {@link Ebi60NoPaymentType }
*
* @return
* The created Ebi60NoPaymentType object and never null
.
*/
@Nonnull
public Ebi60NoPaymentType createEbi60NoPaymentType() {
return new Ebi60NoPaymentType();
}
/**
* Create an instance of {@link Ebi60OrderingPartyType }
*
* @return
* The created Ebi60OrderingPartyType object and never null
.
*/
@Nonnull
public Ebi60OrderingPartyType createEbi60OrderingPartyType() {
return new Ebi60OrderingPartyType();
}
/**
* Create an instance of {@link Ebi60OrderReferenceType }
*
* @return
* The created Ebi60OrderReferenceType object and never null
.
*/
@Nonnull
public Ebi60OrderReferenceType createEbi60OrderReferenceType() {
return new Ebi60OrderReferenceType();
}
/**
* Create an instance of {@link Ebi60OtherPaymentType }
*
* @return
* The created Ebi60OtherPaymentType object and never null
.
*/
@Nonnull
public Ebi60OtherPaymentType createEbi60OtherPaymentType() {
return new Ebi60OtherPaymentType();
}
/**
* Create an instance of {@link Ebi60OtherTaxType }
*
* @return
* The created Ebi60OtherTaxType object and never null
.
*/
@Nonnull
public Ebi60OtherTaxType createEbi60OtherTaxType() {
return new Ebi60OtherTaxType();
}
/**
* Create an instance of {@link Ebi60OtherVATableTaxType }
*
* @return
* The created Ebi60OtherVATableTaxType object and never null
.
*/
@Nonnull
public Ebi60OtherVATableTaxType createEbi60OtherVATableTaxType() {
return new Ebi60OtherVATableTaxType();
}
/**
* Create an instance of {@link Ebi60PaymentCardType }
*
* @return
* The created Ebi60PaymentCardType object and never null
.
*/
@Nonnull
public Ebi60PaymentCardType createEbi60PaymentCardType() {
return new Ebi60PaymentCardType();
}
/**
* Create an instance of {@link Ebi60PaymentConditionsType }
*
* @return
* The created Ebi60PaymentConditionsType object and never null
.
*/
@Nonnull
public Ebi60PaymentConditionsType createEbi60PaymentConditionsType() {
return new Ebi60PaymentConditionsType();
}
/**
* Create an instance of {@link Ebi60PaymentMethodType }
*
* @return
* The created Ebi60PaymentMethodType object and never null
.
*/
@Nonnull
public Ebi60PaymentMethodType createEbi60PaymentMethodType() {
return new Ebi60PaymentMethodType();
}
/**
* Create an instance of {@link Ebi60PaymentReferenceType }
*
* @return
* The created Ebi60PaymentReferenceType object and never null
.
*/
@Nonnull
public Ebi60PaymentReferenceType createEbi60PaymentReferenceType() {
return new Ebi60PaymentReferenceType();
}
/**
* Create an instance of {@link Ebi60PeriodType }
*
* @return
* The created Ebi60PeriodType object and never null
.
*/
@Nonnull
public Ebi60PeriodType createEbi60PeriodType() {
return new Ebi60PeriodType();
}
/**
* Create an instance of {@link Ebi60UnitType }
*
* @return
* The created Ebi60UnitType object and never null
.
*/
@Nonnull
public Ebi60UnitType createEbi60UnitType() {
return new Ebi60UnitType();
}
/**
* Create an instance of {@link Ebi60ReductionAndSurchargeType }
*
* @return
* The created Ebi60ReductionAndSurchargeType object and never null
.
*/
@Nonnull
public Ebi60ReductionAndSurchargeType createEbi60ReductionAndSurchargeType() {
return new Ebi60ReductionAndSurchargeType();
}
/**
* Create an instance of {@link Ebi60ReductionAndSurchargeDetailsType }
*
* @return
* The created Ebi60ReductionAndSurchargeDetailsType object and never null
.
*/
@Nonnull
public Ebi60ReductionAndSurchargeDetailsType createEbi60ReductionAndSurchargeDetailsType() {
return new Ebi60ReductionAndSurchargeDetailsType();
}
/**
* Create an instance of {@link Ebi60ReductionAndSurchargeListLineItemDetailsType }
*
* @return
* The created Ebi60ReductionAndSurchargeListLineItemDetailsType object and never null
.
*/
@Nonnull
public Ebi60ReductionAndSurchargeListLineItemDetailsType createEbi60ReductionAndSurchargeListLineItemDetailsType() {
return new Ebi60ReductionAndSurchargeListLineItemDetailsType();
}
/**
* Create an instance of {@link Ebi60ReductionAndSurchargeBaseType }
*
* @return
* The created Ebi60ReductionAndSurchargeBaseType object and never null
.
*/
@Nonnull
public Ebi60ReductionAndSurchargeBaseType createEbi60ReductionAndSurchargeBaseType() {
return new Ebi60ReductionAndSurchargeBaseType();
}
/**
* Create an instance of {@link Ebi60RelatedDocumentType }
*
* @return
* The created Ebi60RelatedDocumentType object and never null
.
*/
@Nonnull
public Ebi60RelatedDocumentType createEbi60RelatedDocumentType() {
return new Ebi60RelatedDocumentType();
}
/**
* Create an instance of {@link Ebi60SEPADirectDebitType }
*
* @return
* The created Ebi60SEPADirectDebitType object and never null
.
*/
@Nonnull
public Ebi60SEPADirectDebitType createEbi60SEPADirectDebitType() {
return new Ebi60SEPADirectDebitType();
}
/**
* Create an instance of {@link Ebi60TaxType }
*
* @return
* The created Ebi60TaxType object and never null
.
*/
@Nonnull
public Ebi60TaxType createEbi60TaxType() {
return new Ebi60TaxType();
}
/**
* Create an instance of {@link Ebi60TaxItemType }
*
* @return
* The created Ebi60TaxItemType object and never null
.
*/
@Nonnull
public Ebi60TaxItemType createEbi60TaxItemType() {
return new Ebi60TaxItemType();
}
/**
* Create an instance of {@link Ebi60TaxPercentType }
*
* @return
* The created Ebi60TaxPercentType object and never null
.
*/
@Nonnull
public Ebi60TaxPercentType createEbi60TaxPercentType() {
return new Ebi60TaxPercentType();
}
/**
* Create an instance of {@link Ebi60UnitPriceType }
*
* @return
* The created Ebi60UnitPriceType object and never null
.
*/
@Nonnull
public Ebi60UnitPriceType createEbi60UnitPriceType() {
return new Ebi60UnitPriceType();
}
/**
* Create an instance of {@link Ebi60UniversalBankTransactionType }
*
* @return
* The created Ebi60UniversalBankTransactionType object and never null
.
*/
@Nonnull
public Ebi60UniversalBankTransactionType createEbi60UniversalBankTransactionType() {
return new Ebi60UniversalBankTransactionType();
}
/**
* Create an instance of {@link Ebi60AbstractPartyType }
*
* @return
* The created Ebi60AbstractPartyType object and never null
.
*/
@Nonnull
public Ebi60AbstractPartyType createEbi60AbstractPartyType() {
return new Ebi60AbstractPartyType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60InvoiceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60InvoiceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Invoice")
@Nonnull
public JAXBElement createInvoice(
@Nullable
final Ebi60InvoiceType value) {
return new JAXBElement(_Invoice_QNAME, Ebi60InvoiceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "AccountingArea")
@Nonnull
public JAXBElement createAccountingArea(
@Nullable
final String value) {
return new JAXBElement(_AccountingArea_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60AccountingCurrencyAmountType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60AccountingCurrencyAmountType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "AccountingCurrencyAmount")
@Nonnull
public JAXBElement createAccountingCurrencyAmount(
@Nullable
final Ebi60AccountingCurrencyAmountType value) {
return new JAXBElement(_AccountingCurrencyAmount_QNAME, Ebi60AccountingCurrencyAmountType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60AdditionalInformationType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60AdditionalInformationType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "AdditionalInformation")
@Nonnull
public JAXBElement createAdditionalInformation(
@Nullable
final Ebi60AdditionalInformationType value) {
return new JAXBElement(_AdditionalInformation_QNAME, Ebi60AdditionalInformationType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60AddressType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60AddressType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Address")
@Nonnull
public JAXBElement createAddress(
@Nullable
final Ebi60AddressType value) {
return new JAXBElement(_Address_QNAME, Ebi60AddressType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60AddressIdentifierType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60AddressIdentifierType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "AddressIdentifier")
@Nonnull
public JAXBElement createAddressIdentifier(
@Nullable
final Ebi60AddressIdentifierType value) {
return new JAXBElement(_AddressIdentifier_QNAME, Ebi60AddressIdentifierType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Amount")
@Nonnull
public JAXBElement createAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_Amount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60ArticleNumberType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60ArticleNumberType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "ArticleNumber")
@Nonnull
public JAXBElement createArticleNumber(
@Nullable
final Ebi60ArticleNumberType value) {
return new JAXBElement(_ArticleNumber_QNAME, Ebi60ArticleNumberType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "BankAccountNr")
@Nonnull
public JAXBElement createBankAccountNr(
@Nullable
final String value) {
return new JAXBElement(_BankAccountNr_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "BankAccountOwner")
@Nonnull
public JAXBElement createBankAccountOwner(
@Nullable
final String value) {
return new JAXBElement(_BankAccountOwner_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60BankCodeType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60BankCodeType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "BankCode")
@Nonnull
public JAXBElement createBankCode(
@Nullable
final Ebi60BankCodeType value) {
return new JAXBElement(_BankCode_QNAME, Ebi60BankCodeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "BankName")
@Nonnull
public JAXBElement createBankName(
@Nullable
final String value) {
return new JAXBElement(_BankName_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "BaseAmount")
@Nonnull
public JAXBElement createBaseAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_BaseAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60AccountType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60AccountType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "BeneficiaryAccount")
@Nonnull
public JAXBElement createBeneficiaryAccount(
@Nullable
final Ebi60AccountType value) {
return new JAXBElement(_BeneficiaryAccount_QNAME, Ebi60AccountType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "BIC")
@Nonnull
public JAXBElement createBIC(
@Nullable
final String value) {
return new JAXBElement(_BIC_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60BillerType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60BillerType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Biller")
@Nonnull
public JAXBElement createBiller(
@Nullable
final Ebi60BillerType value) {
return new JAXBElement(_Biller_QNAME, Ebi60BillerType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "BillersInvoiceRecipientID")
@Nonnull
public JAXBElement createBillersInvoiceRecipientID(
@Nullable
final String value) {
return new JAXBElement(_BillersInvoiceRecipientID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "BillersOrderingPartyID")
@Nonnull
public JAXBElement createBillersOrderingPartyID(
@Nullable
final String value) {
return new JAXBElement(_BillersOrderingPartyID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60OrderReferenceDetailType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60OrderReferenceDetailType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "BillersOrderReference")
@Nonnull
public JAXBElement createBillersOrderReference(
@Nullable
final Ebi60OrderReferenceDetailType value) {
return new JAXBElement(_BillersOrderReference_QNAME, Ebi60OrderReferenceDetailType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60CancelledOriginalDocumentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60CancelledOriginalDocumentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "CancelledOriginalDocument")
@Nonnull
public JAXBElement createCancelledOriginalDocument(
@Nullable
final Ebi60CancelledOriginalDocumentType value) {
return new JAXBElement(_CancelledOriginalDocument_QNAME, Ebi60CancelledOriginalDocumentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "CardHolderName")
@Nonnull
public JAXBElement createCardHolderName(
@Nullable
final String value) {
return new JAXBElement(_CardHolderName_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60ClassificationType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60ClassificationType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Classification")
@Nonnull
public JAXBElement createClassification(
@Nullable
final Ebi60ClassificationType value) {
return new JAXBElement(_Classification_QNAME, Ebi60ClassificationType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Comment")
@Nonnull
public JAXBElement createComment(
@Nullable
final String value) {
return new JAXBElement(_Comment_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "ConsolidatorsBillerID")
@Nonnull
public JAXBElement createConsolidatorsBillerID(
@Nullable
final String value) {
return new JAXBElement(_ConsolidatorsBillerID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60ContactType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60ContactType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Contact")
@Nonnull
public JAXBElement createContact(
@Nullable
final Ebi60ContactType value) {
return new JAXBElement(_Contact_QNAME, Ebi60ContactType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60CountryType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60CountryType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Country")
@Nonnull
public JAXBElement createCountry(
@Nullable
final Ebi60CountryType value) {
return new JAXBElement(_Country_QNAME, Ebi60CountryType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "CreditorID")
@Nonnull
public JAXBElement createCreditorID(
@Nullable
final String value) {
return new JAXBElement(_CreditorID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Currency")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@Nonnull
public JAXBElement createCurrency(
@Nullable
final String value) {
return new JAXBElement(_Currency_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60CurrencyExchangeInformationType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60CurrencyExchangeInformationType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "CurrencyExchangeInformation")
@Nonnull
public JAXBElement createCurrencyExchangeInformation(
@Nullable
final Ebi60CurrencyExchangeInformationType value) {
return new JAXBElement(_CurrencyExchangeInformation_QNAME, Ebi60CurrencyExchangeInformationType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60CustomType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60CustomType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Custom")
@Nonnull
public JAXBElement createCustom(
@Nullable
final Ebi60CustomType value) {
return new JAXBElement(_Custom_QNAME, Ebi60CustomType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Date")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_Date_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "DebitCollectionDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createDebitCollectionDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_DebitCollectionDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60DeliveryType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60DeliveryType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Delivery")
@Nonnull
public JAXBElement createDelivery(
@Nullable
final Ebi60DeliveryType value) {
return new JAXBElement(_Delivery_QNAME, Ebi60DeliveryType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "DeliveryID")
@Nonnull
public JAXBElement createDeliveryID(
@Nullable
final String value) {
return new JAXBElement(_DeliveryID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Description")
@Nonnull
public JAXBElement createDescription(
@Nullable
final String value) {
return new JAXBElement(_Description_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60DetailsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60DetailsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Details")
@Nonnull
public JAXBElement createDetails(
@Nullable
final Ebi60DetailsType value) {
return new JAXBElement(_Details_QNAME, Ebi60DetailsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60DiscountType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60DiscountType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Discount")
@Nonnull
public JAXBElement createDiscount(
@Nullable
final Ebi60DiscountType value) {
return new JAXBElement(_Discount_QNAME, Ebi60DiscountType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60DocumentTypeType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60DocumentTypeType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "DocumentType")
@Nonnull
public JAXBElement createDocumentType(
@Nullable
final Ebi60DocumentTypeType value) {
return new JAXBElement(_DocumentType_QNAME, Ebi60DocumentTypeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "DueDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createDueDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_DueDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Email")
@Nonnull
public JAXBElement createEmail(
@Nullable
final String value) {
return new JAXBElement(_Email_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "ExchangeRate")
@Nonnull
public JAXBElement createExchangeRate(
@Nullable
final BigDecimal value) {
return new JAXBElement(_ExchangeRate_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "ExchangeRateDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createExchangeRateDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_ExchangeRateDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60ExtensionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60ExtensionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Extension")
@Nonnull
public JAXBElement createExtension(
@Nullable
final Ebi60ExtensionType value) {
return new JAXBElement(_Extension_QNAME, Ebi60ExtensionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "FooterDescription")
@Nonnull
public JAXBElement createFooterDescription(
@Nullable
final String value) {
return new JAXBElement(_FooterDescription_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "FromDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createFromDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_FromDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60FurtherIdentificationType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60FurtherIdentificationType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "FurtherIdentification")
@Nonnull
public JAXBElement createFurtherIdentification(
@Nullable
final Ebi60FurtherIdentificationType value) {
return new JAXBElement(_FurtherIdentification_QNAME, Ebi60FurtherIdentificationType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "HeaderDescription")
@Nonnull
public JAXBElement createHeaderDescription(
@Nullable
final String value) {
return new JAXBElement(_HeaderDescription_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "IBAN")
@Nonnull
public JAXBElement createIBAN(
@Nullable
final String value) {
return new JAXBElement(_IBAN_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "InvoiceDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createInvoiceDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_InvoiceDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "InvoiceNumber")
@Nonnull
public JAXBElement createInvoiceNumber(
@Nullable
final String value) {
return new JAXBElement(_InvoiceNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60InvoiceRecipientType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60InvoiceRecipientType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "InvoiceRecipient")
@Nonnull
public JAXBElement createInvoiceRecipient(
@Nullable
final Ebi60InvoiceRecipientType value) {
return new JAXBElement(_InvoiceRecipient_QNAME, Ebi60InvoiceRecipientType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "InvoiceRecipientsBillerID")
@Nonnull
public JAXBElement createInvoiceRecipientsBillerID(
@Nullable
final String value) {
return new JAXBElement(_InvoiceRecipientsBillerID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60OrderReferenceDetailType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60OrderReferenceDetailType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "InvoiceRecipientsOrderReference")
@Nonnull
public JAXBElement createInvoiceRecipientsOrderReference(
@Nullable
final Ebi60OrderReferenceDetailType value) {
return new JAXBElement(_InvoiceRecipientsOrderReference_QNAME, Ebi60OrderReferenceDetailType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60ItemListType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60ItemListType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "ItemList")
@Nonnull
public JAXBElement createItemList(
@Nullable
final Ebi60ItemListType value) {
return new JAXBElement(_ItemList_QNAME, Ebi60ItemListType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "LineItemAmount")
@Nonnull
public JAXBElement createLineItemAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_LineItemAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60ListLineItemType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60ListLineItemType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "ListLineItem")
@Nonnull
public JAXBElement createListLineItem(
@Nullable
final Ebi60ListLineItemType value) {
return new JAXBElement(_ListLineItem_QNAME, Ebi60ListLineItemType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "MandateReference")
@Nonnull
public JAXBElement createMandateReference(
@Nullable
final String value) {
return new JAXBElement(_MandateReference_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "MinimumPayment")
@Nonnull
public JAXBElement createMinimumPayment(
@Nullable
final BigDecimal value) {
return new JAXBElement(_MinimumPayment_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Name")
@Nonnull
public JAXBElement createName(
@Nullable
final String value) {
return new JAXBElement(_Name_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60NoPaymentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60NoPaymentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "NoPayment")
@Nonnull
public JAXBElement createNoPayment(
@Nullable
final Ebi60NoPaymentType value) {
return new JAXBElement(_NoPayment_QNAME, Ebi60NoPaymentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "OrderID")
@Nonnull
public JAXBElement createOrderID(
@Nullable
final String value) {
return new JAXBElement(_OrderID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60OrderingPartyType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60OrderingPartyType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "OrderingParty")
@Nonnull
public JAXBElement createOrderingParty(
@Nullable
final Ebi60OrderingPartyType value) {
return new JAXBElement(_OrderingParty_QNAME, Ebi60OrderingPartyType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "OrderPositionNumber")
@Nonnull
public JAXBElement createOrderPositionNumber(
@Nullable
final String value) {
return new JAXBElement(_OrderPositionNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60OrderReferenceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60OrderReferenceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "OrderReference")
@Nonnull
public JAXBElement createOrderReference(
@Nullable
final Ebi60OrderReferenceType value) {
return new JAXBElement(_OrderReference_QNAME, Ebi60OrderReferenceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60OtherPaymentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60OtherPaymentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "OtherPayment")
@Nonnull
public JAXBElement createOtherPayment(
@Nullable
final Ebi60OtherPaymentType value) {
return new JAXBElement(_OtherPayment_QNAME, Ebi60OtherPaymentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60OtherTaxType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60OtherTaxType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "OtherTax")
@Nonnull
public JAXBElement createOtherTax(
@Nullable
final Ebi60OtherTaxType value) {
return new JAXBElement(_OtherTax_QNAME, Ebi60OtherTaxType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60OtherVATableTaxType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60OtherVATableTaxType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "OtherVATableTax")
@Nonnull
public JAXBElement createOtherVATableTax(
@Nullable
final Ebi60OtherVATableTaxType value) {
return new JAXBElement(_OtherVATableTax_QNAME, Ebi60OtherVATableTaxType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60OtherVATableTaxType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60OtherVATableTaxType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "OtherVATableTaxListLineItem")
@Nonnull
public JAXBElement createOtherVATableTaxListLineItem(
@Nullable
final Ebi60OtherVATableTaxType value) {
return new JAXBElement(_OtherVATableTaxListLineItem_QNAME, Ebi60OtherVATableTaxType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "PayableAmount")
@Nonnull
public JAXBElement createPayableAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_PayableAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60PaymentCardType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60PaymentCardType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "PaymentCard")
@Nonnull
public JAXBElement createPaymentCard(
@Nullable
final Ebi60PaymentCardType value) {
return new JAXBElement(_PaymentCard_QNAME, Ebi60PaymentCardType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60PaymentConditionsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60PaymentConditionsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "PaymentConditions")
@Nonnull
public JAXBElement createPaymentConditions(
@Nullable
final Ebi60PaymentConditionsType value) {
return new JAXBElement(_PaymentConditions_QNAME, Ebi60PaymentConditionsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "PaymentDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createPaymentDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_PaymentDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60PaymentMethodType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60PaymentMethodType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "PaymentMethod")
@Nonnull
public JAXBElement createPaymentMethod(
@Nullable
final Ebi60PaymentMethodType value) {
return new JAXBElement(_PaymentMethod_QNAME, Ebi60PaymentMethodType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60PaymentReferenceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60PaymentReferenceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "PaymentReference")
@Nonnull
public JAXBElement createPaymentReference(
@Nullable
final Ebi60PaymentReferenceType value) {
return new JAXBElement(_PaymentReference_QNAME, Ebi60PaymentReferenceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Percentage")
@Nonnull
public JAXBElement createPercentage(
@Nullable
final BigDecimal value) {
return new JAXBElement(_Percentage_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60PeriodType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60PeriodType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Period")
@Nonnull
public JAXBElement createPeriod(
@Nullable
final Ebi60PeriodType value) {
return new JAXBElement(_Period_QNAME, Ebi60PeriodType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Phone")
@Nonnull
public JAXBElement createPhone(
@Nullable
final String value) {
return new JAXBElement(_Phone_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "POBox")
@Nonnull
public JAXBElement createPOBox(
@Nullable
final String value) {
return new JAXBElement(_POBox_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigInteger }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "PositionNumber")
@Nonnull
public JAXBElement createPositionNumber(
@Nullable
final BigInteger value) {
return new JAXBElement(_PositionNumber_QNAME, BigInteger.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "PrepaidAmount")
@Nonnull
public JAXBElement createPrepaidAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_PrepaidAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "PrimaryAccountNumber")
@Nonnull
public JAXBElement createPrimaryAccountNumber(
@Nullable
final String value) {
return new JAXBElement(_PrimaryAccountNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60UnitType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60UnitType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Quantity")
@Nonnull
public JAXBElement createQuantity(
@Nullable
final Ebi60UnitType value) {
return new JAXBElement(_Quantity_QNAME, Ebi60UnitType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60ReductionAndSurchargeType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60ReductionAndSurchargeType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Reduction")
@Nonnull
public JAXBElement createReduction(
@Nullable
final Ebi60ReductionAndSurchargeType value) {
return new JAXBElement(_Reduction_QNAME, Ebi60ReductionAndSurchargeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60ReductionAndSurchargeDetailsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60ReductionAndSurchargeDetailsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "ReductionAndSurchargeDetails")
@Nonnull
public JAXBElement createReductionAndSurchargeDetails(
@Nullable
final Ebi60ReductionAndSurchargeDetailsType value) {
return new JAXBElement(_ReductionAndSurchargeDetails_QNAME, Ebi60ReductionAndSurchargeDetailsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60ReductionAndSurchargeListLineItemDetailsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60ReductionAndSurchargeListLineItemDetailsType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "ReductionAndSurchargeListLineItemDetails")
@Nonnull
public JAXBElement createReductionAndSurchargeListLineItemDetails(
@Nullable
final Ebi60ReductionAndSurchargeListLineItemDetailsType value) {
return new JAXBElement(_ReductionAndSurchargeListLineItemDetails_QNAME, Ebi60ReductionAndSurchargeListLineItemDetailsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60ReductionAndSurchargeBaseType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60ReductionAndSurchargeBaseType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "ReductionListLineItem")
@Nonnull
public JAXBElement createReductionListLineItem(
@Nullable
final Ebi60ReductionAndSurchargeBaseType value) {
return new JAXBElement(_ReductionListLineItem_QNAME, Ebi60ReductionAndSurchargeBaseType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "ReferenceDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createReferenceDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_ReferenceDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60RelatedDocumentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60RelatedDocumentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "RelatedDocument")
@Nonnull
public JAXBElement createRelatedDocument(
@Nullable
final Ebi60RelatedDocumentType value) {
return new JAXBElement(_RelatedDocument_QNAME, Ebi60RelatedDocumentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "RoundingAmount")
@Nonnull
public JAXBElement createRoundingAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_RoundingAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Salutation")
@Nonnull
public JAXBElement createSalutation(
@Nullable
final String value) {
return new JAXBElement(_Salutation_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60SEPADirectDebitType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60SEPADirectDebitType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "SEPADirectDebit")
@Nonnull
public JAXBElement createSEPADirectDebit(
@Nullable
final Ebi60SEPADirectDebitType value) {
return new JAXBElement(_SEPADirectDebit_QNAME, Ebi60SEPADirectDebitType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Street")
@Nonnull
public JAXBElement createStreet(
@Nullable
final String value) {
return new JAXBElement(_Street_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "SubOrganizationID")
@Nonnull
public JAXBElement createSubOrganizationID(
@Nullable
final String value) {
return new JAXBElement(_SubOrganizationID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60ReductionAndSurchargeType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60ReductionAndSurchargeType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Surcharge")
@Nonnull
public JAXBElement createSurcharge(
@Nullable
final Ebi60ReductionAndSurchargeType value) {
return new JAXBElement(_Surcharge_QNAME, Ebi60ReductionAndSurchargeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60ReductionAndSurchargeBaseType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60ReductionAndSurchargeBaseType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "SurchargeListLineItem")
@Nonnull
public JAXBElement createSurchargeListLineItem(
@Nullable
final Ebi60ReductionAndSurchargeBaseType value) {
return new JAXBElement(_SurchargeListLineItem_QNAME, Ebi60ReductionAndSurchargeBaseType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60TaxType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60TaxType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Tax")
@Nonnull
public JAXBElement createTax(
@Nullable
final Ebi60TaxType value) {
return new JAXBElement(_Tax_QNAME, Ebi60TaxType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "TaxableAmount")
@Nonnull
public JAXBElement createTaxableAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_TaxableAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "TaxAmount")
@Nonnull
public JAXBElement createTaxAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_TaxAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "TaxID")
@Nonnull
public JAXBElement createTaxID(
@Nullable
final String value) {
return new JAXBElement(_TaxID_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60TaxItemType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60TaxItemType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "TaxItem")
@Nonnull
public JAXBElement createTaxItem(
@Nullable
final Ebi60TaxItemType value) {
return new JAXBElement(_TaxItem_QNAME, Ebi60TaxItemType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60TaxPercentType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60TaxPercentType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "TaxPercent")
@Nonnull
public JAXBElement createTaxPercent(
@Nullable
final Ebi60TaxPercentType value) {
return new JAXBElement(_TaxPercent_QNAME, Ebi60TaxPercentType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LocalDate }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "ToDate")
@XmlJavaTypeAdapter(AdapterLocalDate.class)
@Nonnull
public JAXBElement createToDate(
@Nullable
final LocalDate value) {
return new JAXBElement(_ToDate_QNAME, LocalDate.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BigDecimal }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "TotalGrossAmount")
@Nonnull
public JAXBElement createTotalGrossAmount(
@Nullable
final BigDecimal value) {
return new JAXBElement(_TotalGrossAmount_QNAME, BigDecimal.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Town")
@Nonnull
public JAXBElement createTown(
@Nullable
final String value) {
return new JAXBElement(_Town_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "TradingName")
@Nonnull
public JAXBElement createTradingName(
@Nullable
final String value) {
return new JAXBElement(_TradingName_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60SEPADirectDebitTypeType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60SEPADirectDebitTypeType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "Type")
@Nonnull
public JAXBElement createType(
@Nullable
final Ebi60SEPADirectDebitTypeType value) {
return new JAXBElement(_Type_QNAME, Ebi60SEPADirectDebitTypeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60UnitPriceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60UnitPriceType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "UnitPrice")
@Nonnull
public JAXBElement createUnitPrice(
@Nullable
final Ebi60UnitPriceType value) {
return new JAXBElement(_UnitPrice_QNAME, Ebi60UnitPriceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Ebi60UniversalBankTransactionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Ebi60UniversalBankTransactionType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "UniversalBankTransaction")
@Nonnull
public JAXBElement createUniversalBankTransaction(
@Nullable
final Ebi60UniversalBankTransactionType value) {
return new JAXBElement(_UniversalBankTransaction_QNAME, Ebi60UniversalBankTransactionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "VATIdentificationNumber")
@Nonnull
public JAXBElement createVATIdentificationNumber(
@Nullable
final String value) {
return new JAXBElement(_VATIdentificationNumber_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://www.ebinterface.at/schema/6p0/", name = "ZIP")
@Nonnull
public JAXBElement createZIP(
@Nullable
final String value) {
return new JAXBElement(_ZIP_QNAME, String.class, null, value);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy