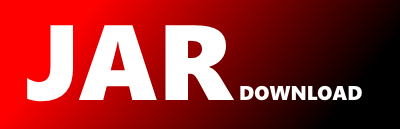
com.helger.fatturapa.v120.ObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-fatturapa Show documentation
Show all versions of ph-fatturapa Show documentation
Library for reading and writing fatturaPA documents
package com.helger.fatturapa.v120;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.helger.fatturapa.v120 package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _FatturaElettronica_QNAME = new QName("http://ivaservizi.agenziaentrate.gov.it/docs/xsd/fatture/v1.2", "FatturaElettronica");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.helger.fatturapa.v120
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link FPA120FatturaElettronicaType }
*
* @return
* The created FPA120FatturaElettronicaType object and never null
.
*/
@Nonnull
public FPA120FatturaElettronicaType createFPA120FatturaElettronicaType() {
return new FPA120FatturaElettronicaType();
}
/**
* Create an instance of {@link FPA120FatturaElettronicaHeaderType }
*
* @return
* The created FPA120FatturaElettronicaHeaderType object and never null
.
*/
@Nonnull
public FPA120FatturaElettronicaHeaderType createFPA120FatturaElettronicaHeaderType() {
return new FPA120FatturaElettronicaHeaderType();
}
/**
* Create an instance of {@link FPA120FatturaElettronicaBodyType }
*
* @return
* The created FPA120FatturaElettronicaBodyType object and never null
.
*/
@Nonnull
public FPA120FatturaElettronicaBodyType createFPA120FatturaElettronicaBodyType() {
return new FPA120FatturaElettronicaBodyType();
}
/**
* Create an instance of {@link FPA120DatiTrasmissioneType }
*
* @return
* The created FPA120DatiTrasmissioneType object and never null
.
*/
@Nonnull
public FPA120DatiTrasmissioneType createFPA120DatiTrasmissioneType() {
return new FPA120DatiTrasmissioneType();
}
/**
* Create an instance of {@link FPA120IdFiscaleType }
*
* @return
* The created FPA120IdFiscaleType object and never null
.
*/
@Nonnull
public FPA120IdFiscaleType createFPA120IdFiscaleType() {
return new FPA120IdFiscaleType();
}
/**
* Create an instance of {@link FPA120ContattiTrasmittenteType }
*
* @return
* The created FPA120ContattiTrasmittenteType object and never null
.
*/
@Nonnull
public FPA120ContattiTrasmittenteType createFPA120ContattiTrasmittenteType() {
return new FPA120ContattiTrasmittenteType();
}
/**
* Create an instance of {@link FPA120DatiGeneraliType }
*
* @return
* The created FPA120DatiGeneraliType object and never null
.
*/
@Nonnull
public FPA120DatiGeneraliType createFPA120DatiGeneraliType() {
return new FPA120DatiGeneraliType();
}
/**
* Create an instance of {@link FPA120DatiGeneraliDocumentoType }
*
* @return
* The created FPA120DatiGeneraliDocumentoType object and never null
.
*/
@Nonnull
public FPA120DatiGeneraliDocumentoType createFPA120DatiGeneraliDocumentoType() {
return new FPA120DatiGeneraliDocumentoType();
}
/**
* Create an instance of {@link FPA120DatiRitenutaType }
*
* @return
* The created FPA120DatiRitenutaType object and never null
.
*/
@Nonnull
public FPA120DatiRitenutaType createFPA120DatiRitenutaType() {
return new FPA120DatiRitenutaType();
}
/**
* Create an instance of {@link FPA120DatiBolloType }
*
* @return
* The created FPA120DatiBolloType object and never null
.
*/
@Nonnull
public FPA120DatiBolloType createFPA120DatiBolloType() {
return new FPA120DatiBolloType();
}
/**
* Create an instance of {@link FPA120DatiCassaPrevidenzialeType }
*
* @return
* The created FPA120DatiCassaPrevidenzialeType object and never null
.
*/
@Nonnull
public FPA120DatiCassaPrevidenzialeType createFPA120DatiCassaPrevidenzialeType() {
return new FPA120DatiCassaPrevidenzialeType();
}
/**
* Create an instance of {@link FPA120ScontoMaggiorazioneType }
*
* @return
* The created FPA120ScontoMaggiorazioneType object and never null
.
*/
@Nonnull
public FPA120ScontoMaggiorazioneType createFPA120ScontoMaggiorazioneType() {
return new FPA120ScontoMaggiorazioneType();
}
/**
* Create an instance of {@link FPA120DatiSALType }
*
* @return
* The created FPA120DatiSALType object and never null
.
*/
@Nonnull
public FPA120DatiSALType createFPA120DatiSALType() {
return new FPA120DatiSALType();
}
/**
* Create an instance of {@link FPA120DatiDocumentiCorrelatiType }
*
* @return
* The created FPA120DatiDocumentiCorrelatiType object and never null
.
*/
@Nonnull
public FPA120DatiDocumentiCorrelatiType createFPA120DatiDocumentiCorrelatiType() {
return new FPA120DatiDocumentiCorrelatiType();
}
/**
* Create an instance of {@link FPA120DatiDDTType }
*
* @return
* The created FPA120DatiDDTType object and never null
.
*/
@Nonnull
public FPA120DatiDDTType createFPA120DatiDDTType() {
return new FPA120DatiDDTType();
}
/**
* Create an instance of {@link FPA120DatiTrasportoType }
*
* @return
* The created FPA120DatiTrasportoType object and never null
.
*/
@Nonnull
public FPA120DatiTrasportoType createFPA120DatiTrasportoType() {
return new FPA120DatiTrasportoType();
}
/**
* Create an instance of {@link FPA120IndirizzoType }
*
* @return
* The created FPA120IndirizzoType object and never null
.
*/
@Nonnull
public FPA120IndirizzoType createFPA120IndirizzoType() {
return new FPA120IndirizzoType();
}
/**
* Create an instance of {@link FPA120FatturaPrincipaleType }
*
* @return
* The created FPA120FatturaPrincipaleType object and never null
.
*/
@Nonnull
public FPA120FatturaPrincipaleType createFPA120FatturaPrincipaleType() {
return new FPA120FatturaPrincipaleType();
}
/**
* Create an instance of {@link FPA120CedentePrestatoreType }
*
* @return
* The created FPA120CedentePrestatoreType object and never null
.
*/
@Nonnull
public FPA120CedentePrestatoreType createFPA120CedentePrestatoreType() {
return new FPA120CedentePrestatoreType();
}
/**
* Create an instance of {@link FPA120DatiAnagraficiCedenteType }
*
* @return
* The created FPA120DatiAnagraficiCedenteType object and never null
.
*/
@Nonnull
public FPA120DatiAnagraficiCedenteType createFPA120DatiAnagraficiCedenteType() {
return new FPA120DatiAnagraficiCedenteType();
}
/**
* Create an instance of {@link FPA120AnagraficaType }
*
* @return
* The created FPA120AnagraficaType object and never null
.
*/
@Nonnull
public FPA120AnagraficaType createFPA120AnagraficaType() {
return new FPA120AnagraficaType();
}
/**
* Create an instance of {@link FPA120DatiAnagraficiVettoreType }
*
* @return
* The created FPA120DatiAnagraficiVettoreType object and never null
.
*/
@Nonnull
public FPA120DatiAnagraficiVettoreType createFPA120DatiAnagraficiVettoreType() {
return new FPA120DatiAnagraficiVettoreType();
}
/**
* Create an instance of {@link FPA120IscrizioneREAType }
*
* @return
* The created FPA120IscrizioneREAType object and never null
.
*/
@Nonnull
public FPA120IscrizioneREAType createFPA120IscrizioneREAType() {
return new FPA120IscrizioneREAType();
}
/**
* Create an instance of {@link FPA120ContattiType }
*
* @return
* The created FPA120ContattiType object and never null
.
*/
@Nonnull
public FPA120ContattiType createFPA120ContattiType() {
return new FPA120ContattiType();
}
/**
* Create an instance of {@link FPA120RappresentanteFiscaleType }
*
* @return
* The created FPA120RappresentanteFiscaleType object and never null
.
*/
@Nonnull
public FPA120RappresentanteFiscaleType createFPA120RappresentanteFiscaleType() {
return new FPA120RappresentanteFiscaleType();
}
/**
* Create an instance of {@link FPA120DatiAnagraficiRappresentanteType }
*
* @return
* The created FPA120DatiAnagraficiRappresentanteType object and never null
.
*/
@Nonnull
public FPA120DatiAnagraficiRappresentanteType createFPA120DatiAnagraficiRappresentanteType() {
return new FPA120DatiAnagraficiRappresentanteType();
}
/**
* Create an instance of {@link FPA120CessionarioCommittenteType }
*
* @return
* The created FPA120CessionarioCommittenteType object and never null
.
*/
@Nonnull
public FPA120CessionarioCommittenteType createFPA120CessionarioCommittenteType() {
return new FPA120CessionarioCommittenteType();
}
/**
* Create an instance of {@link FPA120RappresentanteFiscaleCessionarioType }
*
* @return
* The created FPA120RappresentanteFiscaleCessionarioType object and never null
.
*/
@Nonnull
public FPA120RappresentanteFiscaleCessionarioType createFPA120RappresentanteFiscaleCessionarioType() {
return new FPA120RappresentanteFiscaleCessionarioType();
}
/**
* Create an instance of {@link FPA120DatiAnagraficiCessionarioType }
*
* @return
* The created FPA120DatiAnagraficiCessionarioType object and never null
.
*/
@Nonnull
public FPA120DatiAnagraficiCessionarioType createFPA120DatiAnagraficiCessionarioType() {
return new FPA120DatiAnagraficiCessionarioType();
}
/**
* Create an instance of {@link FPA120DatiBeniServiziType }
*
* @return
* The created FPA120DatiBeniServiziType object and never null
.
*/
@Nonnull
public FPA120DatiBeniServiziType createFPA120DatiBeniServiziType() {
return new FPA120DatiBeniServiziType();
}
/**
* Create an instance of {@link FPA120DatiVeicoliType }
*
* @return
* The created FPA120DatiVeicoliType object and never null
.
*/
@Nonnull
public FPA120DatiVeicoliType createFPA120DatiVeicoliType() {
return new FPA120DatiVeicoliType();
}
/**
* Create an instance of {@link FPA120DatiPagamentoType }
*
* @return
* The created FPA120DatiPagamentoType object and never null
.
*/
@Nonnull
public FPA120DatiPagamentoType createFPA120DatiPagamentoType() {
return new FPA120DatiPagamentoType();
}
/**
* Create an instance of {@link FPA120DettaglioPagamentoType }
*
* @return
* The created FPA120DettaglioPagamentoType object and never null
.
*/
@Nonnull
public FPA120DettaglioPagamentoType createFPA120DettaglioPagamentoType() {
return new FPA120DettaglioPagamentoType();
}
/**
* Create an instance of {@link FPA120TerzoIntermediarioSoggettoEmittenteType }
*
* @return
* The created FPA120TerzoIntermediarioSoggettoEmittenteType object and never null
.
*/
@Nonnull
public FPA120TerzoIntermediarioSoggettoEmittenteType createFPA120TerzoIntermediarioSoggettoEmittenteType() {
return new FPA120TerzoIntermediarioSoggettoEmittenteType();
}
/**
* Create an instance of {@link FPA120DatiAnagraficiTerzoIntermediarioType }
*
* @return
* The created FPA120DatiAnagraficiTerzoIntermediarioType object and never null
.
*/
@Nonnull
public FPA120DatiAnagraficiTerzoIntermediarioType createFPA120DatiAnagraficiTerzoIntermediarioType() {
return new FPA120DatiAnagraficiTerzoIntermediarioType();
}
/**
* Create an instance of {@link FPA120AllegatiType }
*
* @return
* The created FPA120AllegatiType object and never null
.
*/
@Nonnull
public FPA120AllegatiType createFPA120AllegatiType() {
return new FPA120AllegatiType();
}
/**
* Create an instance of {@link FPA120DettaglioLineeType }
*
* @return
* The created FPA120DettaglioLineeType object and never null
.
*/
@Nonnull
public FPA120DettaglioLineeType createFPA120DettaglioLineeType() {
return new FPA120DettaglioLineeType();
}
/**
* Create an instance of {@link FPA120CodiceArticoloType }
*
* @return
* The created FPA120CodiceArticoloType object and never null
.
*/
@Nonnull
public FPA120CodiceArticoloType createFPA120CodiceArticoloType() {
return new FPA120CodiceArticoloType();
}
/**
* Create an instance of {@link FPA120AltriDatiGestionaliType }
*
* @return
* The created FPA120AltriDatiGestionaliType object and never null
.
*/
@Nonnull
public FPA120AltriDatiGestionaliType createFPA120AltriDatiGestionaliType() {
return new FPA120AltriDatiGestionaliType();
}
/**
* Create an instance of {@link FPA120DatiRiepilogoType }
*
* @return
* The created FPA120DatiRiepilogoType object and never null
.
*/
@Nonnull
public FPA120DatiRiepilogoType createFPA120DatiRiepilogoType() {
return new FPA120DatiRiepilogoType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link FPA120FatturaElettronicaType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link FPA120FatturaElettronicaType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://ivaservizi.agenziaentrate.gov.it/docs/xsd/fatture/v1.2", name = "FatturaElettronica")
@Nonnull
public JAXBElement createFatturaElettronica(
@Nullable
final FPA120FatturaElettronicaType value) {
return new JAXBElement(_FatturaElettronica_QNAME, FPA120FatturaElettronicaType.class, null, value);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy