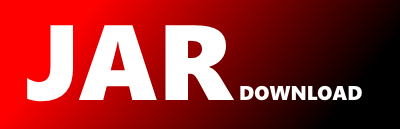
com.helger.fatturapa.v121.ObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-fatturapa Show documentation
Show all versions of ph-fatturapa Show documentation
Library for reading and writing fatturaPA documents
package com.helger.fatturapa.v121;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
import com.helger.commons.annotation.CodingStyleguideUnaware;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.helger.fatturapa.v121 package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
This class was annotated by ph-jaxb22-plugin -Xph-annotate
*
*
*/
@XmlRegistry
@CodingStyleguideUnaware
public class ObjectFactory {
public final static QName _FatturaElettronica_QNAME = new QName("http://ivaservizi.agenziaentrate.gov.it/docs/xsd/fatture/v1.2", "FatturaElettronica");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.helger.fatturapa.v121
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link FPA121FatturaElettronicaType }
*
* @return
* The created FPA121FatturaElettronicaType object and never null
.
*/
@Nonnull
public FPA121FatturaElettronicaType createFPA121FatturaElettronicaType() {
return new FPA121FatturaElettronicaType();
}
/**
* Create an instance of {@link FPA121FatturaElettronicaHeaderType }
*
* @return
* The created FPA121FatturaElettronicaHeaderType object and never null
.
*/
@Nonnull
public FPA121FatturaElettronicaHeaderType createFPA121FatturaElettronicaHeaderType() {
return new FPA121FatturaElettronicaHeaderType();
}
/**
* Create an instance of {@link FPA121FatturaElettronicaBodyType }
*
* @return
* The created FPA121FatturaElettronicaBodyType object and never null
.
*/
@Nonnull
public FPA121FatturaElettronicaBodyType createFPA121FatturaElettronicaBodyType() {
return new FPA121FatturaElettronicaBodyType();
}
/**
* Create an instance of {@link FPA121DatiTrasmissioneType }
*
* @return
* The created FPA121DatiTrasmissioneType object and never null
.
*/
@Nonnull
public FPA121DatiTrasmissioneType createFPA121DatiTrasmissioneType() {
return new FPA121DatiTrasmissioneType();
}
/**
* Create an instance of {@link FPA121IdFiscaleType }
*
* @return
* The created FPA121IdFiscaleType object and never null
.
*/
@Nonnull
public FPA121IdFiscaleType createFPA121IdFiscaleType() {
return new FPA121IdFiscaleType();
}
/**
* Create an instance of {@link FPA121ContattiTrasmittenteType }
*
* @return
* The created FPA121ContattiTrasmittenteType object and never null
.
*/
@Nonnull
public FPA121ContattiTrasmittenteType createFPA121ContattiTrasmittenteType() {
return new FPA121ContattiTrasmittenteType();
}
/**
* Create an instance of {@link FPA121DatiGeneraliType }
*
* @return
* The created FPA121DatiGeneraliType object and never null
.
*/
@Nonnull
public FPA121DatiGeneraliType createFPA121DatiGeneraliType() {
return new FPA121DatiGeneraliType();
}
/**
* Create an instance of {@link FPA121DatiGeneraliDocumentoType }
*
* @return
* The created FPA121DatiGeneraliDocumentoType object and never null
.
*/
@Nonnull
public FPA121DatiGeneraliDocumentoType createFPA121DatiGeneraliDocumentoType() {
return new FPA121DatiGeneraliDocumentoType();
}
/**
* Create an instance of {@link FPA121DatiRitenutaType }
*
* @return
* The created FPA121DatiRitenutaType object and never null
.
*/
@Nonnull
public FPA121DatiRitenutaType createFPA121DatiRitenutaType() {
return new FPA121DatiRitenutaType();
}
/**
* Create an instance of {@link FPA121DatiBolloType }
*
* @return
* The created FPA121DatiBolloType object and never null
.
*/
@Nonnull
public FPA121DatiBolloType createFPA121DatiBolloType() {
return new FPA121DatiBolloType();
}
/**
* Create an instance of {@link FPA121DatiCassaPrevidenzialeType }
*
* @return
* The created FPA121DatiCassaPrevidenzialeType object and never null
.
*/
@Nonnull
public FPA121DatiCassaPrevidenzialeType createFPA121DatiCassaPrevidenzialeType() {
return new FPA121DatiCassaPrevidenzialeType();
}
/**
* Create an instance of {@link FPA121ScontoMaggiorazioneType }
*
* @return
* The created FPA121ScontoMaggiorazioneType object and never null
.
*/
@Nonnull
public FPA121ScontoMaggiorazioneType createFPA121ScontoMaggiorazioneType() {
return new FPA121ScontoMaggiorazioneType();
}
/**
* Create an instance of {@link FPA121DatiSALType }
*
* @return
* The created FPA121DatiSALType object and never null
.
*/
@Nonnull
public FPA121DatiSALType createFPA121DatiSALType() {
return new FPA121DatiSALType();
}
/**
* Create an instance of {@link FPA121DatiDocumentiCorrelatiType }
*
* @return
* The created FPA121DatiDocumentiCorrelatiType object and never null
.
*/
@Nonnull
public FPA121DatiDocumentiCorrelatiType createFPA121DatiDocumentiCorrelatiType() {
return new FPA121DatiDocumentiCorrelatiType();
}
/**
* Create an instance of {@link FPA121DatiDDTType }
*
* @return
* The created FPA121DatiDDTType object and never null
.
*/
@Nonnull
public FPA121DatiDDTType createFPA121DatiDDTType() {
return new FPA121DatiDDTType();
}
/**
* Create an instance of {@link FPA121DatiTrasportoType }
*
* @return
* The created FPA121DatiTrasportoType object and never null
.
*/
@Nonnull
public FPA121DatiTrasportoType createFPA121DatiTrasportoType() {
return new FPA121DatiTrasportoType();
}
/**
* Create an instance of {@link FPA121IndirizzoType }
*
* @return
* The created FPA121IndirizzoType object and never null
.
*/
@Nonnull
public FPA121IndirizzoType createFPA121IndirizzoType() {
return new FPA121IndirizzoType();
}
/**
* Create an instance of {@link FPA121FatturaPrincipaleType }
*
* @return
* The created FPA121FatturaPrincipaleType object and never null
.
*/
@Nonnull
public FPA121FatturaPrincipaleType createFPA121FatturaPrincipaleType() {
return new FPA121FatturaPrincipaleType();
}
/**
* Create an instance of {@link FPA121CedentePrestatoreType }
*
* @return
* The created FPA121CedentePrestatoreType object and never null
.
*/
@Nonnull
public FPA121CedentePrestatoreType createFPA121CedentePrestatoreType() {
return new FPA121CedentePrestatoreType();
}
/**
* Create an instance of {@link FPA121DatiAnagraficiCedenteType }
*
* @return
* The created FPA121DatiAnagraficiCedenteType object and never null
.
*/
@Nonnull
public FPA121DatiAnagraficiCedenteType createFPA121DatiAnagraficiCedenteType() {
return new FPA121DatiAnagraficiCedenteType();
}
/**
* Create an instance of {@link FPA121AnagraficaType }
*
* @return
* The created FPA121AnagraficaType object and never null
.
*/
@Nonnull
public FPA121AnagraficaType createFPA121AnagraficaType() {
return new FPA121AnagraficaType();
}
/**
* Create an instance of {@link FPA121DatiAnagraficiVettoreType }
*
* @return
* The created FPA121DatiAnagraficiVettoreType object and never null
.
*/
@Nonnull
public FPA121DatiAnagraficiVettoreType createFPA121DatiAnagraficiVettoreType() {
return new FPA121DatiAnagraficiVettoreType();
}
/**
* Create an instance of {@link FPA121IscrizioneREAType }
*
* @return
* The created FPA121IscrizioneREAType object and never null
.
*/
@Nonnull
public FPA121IscrizioneREAType createFPA121IscrizioneREAType() {
return new FPA121IscrizioneREAType();
}
/**
* Create an instance of {@link FPA121ContattiType }
*
* @return
* The created FPA121ContattiType object and never null
.
*/
@Nonnull
public FPA121ContattiType createFPA121ContattiType() {
return new FPA121ContattiType();
}
/**
* Create an instance of {@link FPA121RappresentanteFiscaleType }
*
* @return
* The created FPA121RappresentanteFiscaleType object and never null
.
*/
@Nonnull
public FPA121RappresentanteFiscaleType createFPA121RappresentanteFiscaleType() {
return new FPA121RappresentanteFiscaleType();
}
/**
* Create an instance of {@link FPA121DatiAnagraficiRappresentanteType }
*
* @return
* The created FPA121DatiAnagraficiRappresentanteType object and never null
.
*/
@Nonnull
public FPA121DatiAnagraficiRappresentanteType createFPA121DatiAnagraficiRappresentanteType() {
return new FPA121DatiAnagraficiRappresentanteType();
}
/**
* Create an instance of {@link FPA121CessionarioCommittenteType }
*
* @return
* The created FPA121CessionarioCommittenteType object and never null
.
*/
@Nonnull
public FPA121CessionarioCommittenteType createFPA121CessionarioCommittenteType() {
return new FPA121CessionarioCommittenteType();
}
/**
* Create an instance of {@link FPA121RappresentanteFiscaleCessionarioType }
*
* @return
* The created FPA121RappresentanteFiscaleCessionarioType object and never null
.
*/
@Nonnull
public FPA121RappresentanteFiscaleCessionarioType createFPA121RappresentanteFiscaleCessionarioType() {
return new FPA121RappresentanteFiscaleCessionarioType();
}
/**
* Create an instance of {@link FPA121DatiAnagraficiCessionarioType }
*
* @return
* The created FPA121DatiAnagraficiCessionarioType object and never null
.
*/
@Nonnull
public FPA121DatiAnagraficiCessionarioType createFPA121DatiAnagraficiCessionarioType() {
return new FPA121DatiAnagraficiCessionarioType();
}
/**
* Create an instance of {@link FPA121DatiBeniServiziType }
*
* @return
* The created FPA121DatiBeniServiziType object and never null
.
*/
@Nonnull
public FPA121DatiBeniServiziType createFPA121DatiBeniServiziType() {
return new FPA121DatiBeniServiziType();
}
/**
* Create an instance of {@link FPA121DatiVeicoliType }
*
* @return
* The created FPA121DatiVeicoliType object and never null
.
*/
@Nonnull
public FPA121DatiVeicoliType createFPA121DatiVeicoliType() {
return new FPA121DatiVeicoliType();
}
/**
* Create an instance of {@link FPA121DatiPagamentoType }
*
* @return
* The created FPA121DatiPagamentoType object and never null
.
*/
@Nonnull
public FPA121DatiPagamentoType createFPA121DatiPagamentoType() {
return new FPA121DatiPagamentoType();
}
/**
* Create an instance of {@link FPA121DettaglioPagamentoType }
*
* @return
* The created FPA121DettaglioPagamentoType object and never null
.
*/
@Nonnull
public FPA121DettaglioPagamentoType createFPA121DettaglioPagamentoType() {
return new FPA121DettaglioPagamentoType();
}
/**
* Create an instance of {@link FPA121TerzoIntermediarioSoggettoEmittenteType }
*
* @return
* The created FPA121TerzoIntermediarioSoggettoEmittenteType object and never null
.
*/
@Nonnull
public FPA121TerzoIntermediarioSoggettoEmittenteType createFPA121TerzoIntermediarioSoggettoEmittenteType() {
return new FPA121TerzoIntermediarioSoggettoEmittenteType();
}
/**
* Create an instance of {@link FPA121DatiAnagraficiTerzoIntermediarioType }
*
* @return
* The created FPA121DatiAnagraficiTerzoIntermediarioType object and never null
.
*/
@Nonnull
public FPA121DatiAnagraficiTerzoIntermediarioType createFPA121DatiAnagraficiTerzoIntermediarioType() {
return new FPA121DatiAnagraficiTerzoIntermediarioType();
}
/**
* Create an instance of {@link FPA121AllegatiType }
*
* @return
* The created FPA121AllegatiType object and never null
.
*/
@Nonnull
public FPA121AllegatiType createFPA121AllegatiType() {
return new FPA121AllegatiType();
}
/**
* Create an instance of {@link FPA121DettaglioLineeType }
*
* @return
* The created FPA121DettaglioLineeType object and never null
.
*/
@Nonnull
public FPA121DettaglioLineeType createFPA121DettaglioLineeType() {
return new FPA121DettaglioLineeType();
}
/**
* Create an instance of {@link FPA121CodiceArticoloType }
*
* @return
* The created FPA121CodiceArticoloType object and never null
.
*/
@Nonnull
public FPA121CodiceArticoloType createFPA121CodiceArticoloType() {
return new FPA121CodiceArticoloType();
}
/**
* Create an instance of {@link FPA121AltriDatiGestionaliType }
*
* @return
* The created FPA121AltriDatiGestionaliType object and never null
.
*/
@Nonnull
public FPA121AltriDatiGestionaliType createFPA121AltriDatiGestionaliType() {
return new FPA121AltriDatiGestionaliType();
}
/**
* Create an instance of {@link FPA121DatiRiepilogoType }
*
* @return
* The created FPA121DatiRiepilogoType object and never null
.
*/
@Nonnull
public FPA121DatiRiepilogoType createFPA121DatiRiepilogoType() {
return new FPA121DatiRiepilogoType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link FPA121FatturaElettronicaType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link FPA121FatturaElettronicaType }{@code >} The created JAXBElement and never null
.
*/
@XmlElementDecl(namespace = "http://ivaservizi.agenziaentrate.gov.it/docs/xsd/fatture/v1.2", name = "FatturaElettronica")
@Nonnull
public JAXBElement createFatturaElettronica(
@Nullable
final FPA121FatturaElettronicaType value) {
return new JAXBElement(_FatturaElettronica_QNAME, FPA121FatturaElettronicaType.class, null, value);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy