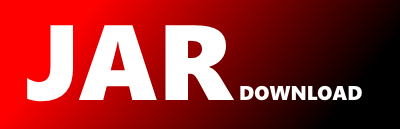
com.helger.graph.IBaseGraph Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-graph Show documentation
Show all versions of ph-graph Show documentation
Java library with basic graph implementations
/**
* Copyright (C) 2014-2016 Philip Helger (www.helger.com)
* philip[at]helger[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.helger.graph;
import java.util.function.Consumer;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.helger.commons.annotation.ReturnsMutableCopy;
import com.helger.commons.collection.ext.ICommonsList;
import com.helger.commons.collection.ext.ICommonsOrderedMap;
import com.helger.commons.collection.ext.ICommonsOrderedSet;
import com.helger.matrix.Matrix;
/**
* Base interface for a read-only graph.
*
* @author Philip Helger
* @param
* Node class
* @param
* Relation class
*/
public interface IBaseGraph , RELATIONTYPE extends IBaseGraphRelation >
extends IBaseGraphObject
{
/**
* @return The number of nodes currently in the graph. Always ≥ 0.
*/
@Nonnegative
int getNodeCount ();
/**
* Find the graph node with the specified ID.
*
* @param sID
* The ID to be searched. Maybe null
.
* @return null
if no such graph node exists in this graph.
*/
@Nullable
NODETYPE getNodeOfID (@Nullable String sID);
/**
* @return A non-null
collection of the nodes in this graph, in
* arbitrary order!
*/
@Nonnull
@ReturnsMutableCopy
ICommonsOrderedMap getAllNodes ();
/**
* @return A non-null
set of all the node IDs in this graph, in
* arbitrary order!
*/
@Nonnull
@ReturnsMutableCopy
ICommonsOrderedSet getAllNodeIDs ();
/**
* Iterate each node calling the provided consumer with the node object.
*
* @param aConsumer
* The consumer to be invoked. May not be null
. May only
* perform reading operations!
*/
void forEachNode (@Nonnull Consumer super NODETYPE> aConsumer);
/**
* @return A non-null
map of the relations in this graph, in
* arbitrary order!
*/
@Nonnull
@ReturnsMutableCopy
ICommonsOrderedMap getAllRelations ();
/**
* @return A non-null
list of the relations in this graph, in
* arbitrary order!
*/
@Nonnull
@ReturnsMutableCopy
ICommonsList getAllRelationObjs ();
/**
* @return A non-null
set of all the relation IDs in this graph,
* in arbitrary order!
*/
@Nonnull
@ReturnsMutableCopy
ICommonsOrderedSet getAllRelationIDs ();
/**
* Iterate each relation calling the provided consumer with the relation
* object.
*
* @param aConsumer
* The consumer to be invoked. May not be null
. May only
* perform reading operations!
*/
void forEachRelation (@Nonnull Consumer super RELATIONTYPE> aConsumer);
/**
* Check if this graph contains cycles. An example for a cycle is e.g. if
* NodeA
has an outgoing relation to NodeB
,
* NodeB
has an outgoing relation to NodeC
and
* finally NodeC
has an outgoing relation to NodeA
.
*
* @return true
if this graph contains at least one cycle,
* false
if this graph is cycle-free.
*/
boolean containsCycles ();
/**
* Check if this graph is completely self contained. As relations between
* nodes do not check whether both nodes belong to the same graph it is
* possible to link different graphs together with relations. This method
* returns true, if all nodes referenced from all relations link to objects
* inside this graph.
*
* @return true
if this graph is self contained,
* false
if not.
*/
boolean isSelfContained ();
/**
* @return A new incidence matrix (Symmetric matrix where 1/-1 is set if a
* relation is present, 0 if no relation is present; Number of rows
* and columns is equal to the number of nodes).
* @throws IllegalArgumentException
* If this graph contains no node
*/
@Nonnull
Matrix createIncidenceMatrix ();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy