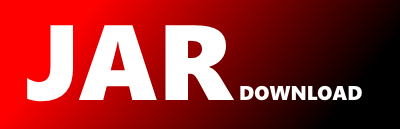
com.helger.holiday.jaxb.Holidays Maven / Gradle / Ivy
package com.helger.holiday.jaxb;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import com.helger.commons.annotation.CodingStyleguideUnaware;
import com.helger.commons.annotation.ReturnsMutableObject;
import com.helger.commons.equals.EqualsHelper;
import com.helger.commons.hashcode.HashCodeGenerator;
import com.helger.commons.string.ToStringGenerator;
/**
* Java class for Holidays complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Holidays">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Fixed" type="{http://www.example.org/Holiday}Fixed" maxOccurs="unbounded" minOccurs="0"/>
* <element name="RelativeToFixed" type="{http://www.example.org/Holiday}RelativeToFixed" maxOccurs="unbounded" minOccurs="0"/>
* <element name="RelativeToWeekdayInMonth" type="{http://www.example.org/Holiday}RelativeToWeekdayInMonth" maxOccurs="unbounded" minOccurs="0"/>
* <element name="FixedWeekday" type="{http://www.example.org/Holiday}FixedWeekdayInMonth" maxOccurs="unbounded" minOccurs="0"/>
* <element name="ChristianHoliday" type="{http://www.example.org/Holiday}ChristianHoliday" maxOccurs="unbounded" minOccurs="0"/>
* <element name="IslamicHoliday" type="{http://www.example.org/Holiday}IslamicHoliday" maxOccurs="unbounded" minOccurs="0"/>
* <element name="FixedWeekdayBetweenFixed" type="{http://www.example.org/Holiday}FixedWeekdayBetweenFixed" maxOccurs="unbounded" minOccurs="0"/>
* <element name="FixedWeekdayRelativeToFixed" type="{http://www.example.org/Holiday}FixedWeekdayRelativeToFixed" maxOccurs="unbounded" minOccurs="0"/>
* <element name="HinduHoliday" type="{http://www.example.org/Holiday}HinduHoliday" maxOccurs="unbounded" minOccurs="0"/>
* <element name="HebrewHoliday" type="{http://www.example.org/Holiday}HebrewHoliday" maxOccurs="unbounded" minOccurs="0"/>
* <element name="EthiopianOrthodoxHoliday" type="{http://www.example.org/Holiday}EthiopianOrthodoxHoliday" maxOccurs="unbounded" minOccurs="0"/>
* <element name="RelativeToEasterSunday" type="{http://www.example.org/Holiday}RelativeToEasterSunday" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
* This class was annotated by ph-jaxb22-plugin -Xph-annotate
* This class contains methods created by ph-jaxb22-plugin -Xph-equalshashcode
* This class contains methods created by ph-jaxb22-plugin -Xph-tostring
* This class contains methods created by ph-jaxb22-plugin -Xph-list-extension
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Holidays", propOrder = {
"fixed",
"relativeToFixed",
"relativeToWeekdayInMonth",
"fixedWeekday",
"christianHoliday",
"islamicHoliday",
"fixedWeekdayBetweenFixed",
"fixedWeekdayRelativeToFixed",
"hinduHoliday",
"hebrewHoliday",
"ethiopianOrthodoxHoliday",
"relativeToEasterSunday"
})
@CodingStyleguideUnaware
public class Holidays implements Serializable
{
@XmlElement(name = "Fixed")
private List fixed;
@XmlElement(name = "RelativeToFixed")
private List relativeToFixed;
@XmlElement(name = "RelativeToWeekdayInMonth")
private List relativeToWeekdayInMonth;
@XmlElement(name = "FixedWeekday")
private List fixedWeekday;
@XmlElement(name = "ChristianHoliday")
private List christianHoliday;
@XmlElement(name = "IslamicHoliday")
private List islamicHoliday;
@XmlElement(name = "FixedWeekdayBetweenFixed")
private List fixedWeekdayBetweenFixed;
@XmlElement(name = "FixedWeekdayRelativeToFixed")
private List fixedWeekdayRelativeToFixed;
@XmlElement(name = "HinduHoliday")
private List hinduHoliday;
@XmlElement(name = "HebrewHoliday")
private List hebrewHoliday;
@XmlElement(name = "EthiopianOrthodoxHoliday")
private List ethiopianOrthodoxHoliday;
@XmlElement(name = "RelativeToEasterSunday")
private List relativeToEasterSunday;
/**
* Gets the value of the fixed property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the fixed property.
*
*
* For example, to add a new item, do as follows:
*
* getFixed().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Fixed }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getFixed() {
if (fixed == null) {
fixed = new ArrayList();
}
return this.fixed;
}
/**
* Gets the value of the relativeToFixed property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the relativeToFixed property.
*
*
* For example, to add a new item, do as follows:
*
* getRelativeToFixed().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RelativeToFixed }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getRelativeToFixed() {
if (relativeToFixed == null) {
relativeToFixed = new ArrayList();
}
return this.relativeToFixed;
}
/**
* Gets the value of the relativeToWeekdayInMonth property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the relativeToWeekdayInMonth property.
*
*
* For example, to add a new item, do as follows:
*
* getRelativeToWeekdayInMonth().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RelativeToWeekdayInMonth }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getRelativeToWeekdayInMonth() {
if (relativeToWeekdayInMonth == null) {
relativeToWeekdayInMonth = new ArrayList();
}
return this.relativeToWeekdayInMonth;
}
/**
* Gets the value of the fixedWeekday property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the fixedWeekday property.
*
*
* For example, to add a new item, do as follows:
*
* getFixedWeekday().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FixedWeekdayInMonth }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getFixedWeekday() {
if (fixedWeekday == null) {
fixedWeekday = new ArrayList();
}
return this.fixedWeekday;
}
/**
* Gets the value of the christianHoliday property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the christianHoliday property.
*
*
* For example, to add a new item, do as follows:
*
* getChristianHoliday().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ChristianHoliday }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getChristianHoliday() {
if (christianHoliday == null) {
christianHoliday = new ArrayList();
}
return this.christianHoliday;
}
/**
* Gets the value of the islamicHoliday property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the islamicHoliday property.
*
*
* For example, to add a new item, do as follows:
*
* getIslamicHoliday().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link IslamicHoliday }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getIslamicHoliday() {
if (islamicHoliday == null) {
islamicHoliday = new ArrayList();
}
return this.islamicHoliday;
}
/**
* Gets the value of the fixedWeekdayBetweenFixed property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the fixedWeekdayBetweenFixed property.
*
*
* For example, to add a new item, do as follows:
*
* getFixedWeekdayBetweenFixed().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FixedWeekdayBetweenFixed }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getFixedWeekdayBetweenFixed() {
if (fixedWeekdayBetweenFixed == null) {
fixedWeekdayBetweenFixed = new ArrayList();
}
return this.fixedWeekdayBetweenFixed;
}
/**
* Gets the value of the fixedWeekdayRelativeToFixed property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the fixedWeekdayRelativeToFixed property.
*
*
* For example, to add a new item, do as follows:
*
* getFixedWeekdayRelativeToFixed().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FixedWeekdayRelativeToFixed }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getFixedWeekdayRelativeToFixed() {
if (fixedWeekdayRelativeToFixed == null) {
fixedWeekdayRelativeToFixed = new ArrayList();
}
return this.fixedWeekdayRelativeToFixed;
}
/**
* Gets the value of the hinduHoliday property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the hinduHoliday property.
*
*
* For example, to add a new item, do as follows:
*
* getHinduHoliday().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link HinduHoliday }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getHinduHoliday() {
if (hinduHoliday == null) {
hinduHoliday = new ArrayList();
}
return this.hinduHoliday;
}
/**
* Gets the value of the hebrewHoliday property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the hebrewHoliday property.
*
*
* For example, to add a new item, do as follows:
*
* getHebrewHoliday().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link HebrewHoliday }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getHebrewHoliday() {
if (hebrewHoliday == null) {
hebrewHoliday = new ArrayList();
}
return this.hebrewHoliday;
}
/**
* Gets the value of the ethiopianOrthodoxHoliday property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the ethiopianOrthodoxHoliday property.
*
*
* For example, to add a new item, do as follows:
*
* getEthiopianOrthodoxHoliday().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link EthiopianOrthodoxHoliday }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getEthiopianOrthodoxHoliday() {
if (ethiopianOrthodoxHoliday == null) {
ethiopianOrthodoxHoliday = new ArrayList();
}
return this.ethiopianOrthodoxHoliday;
}
/**
* Gets the value of the relativeToEasterSunday property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the relativeToEasterSunday property.
*
*
* For example, to add a new item, do as follows:
*
* getRelativeToEasterSunday().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RelativeToEasterSunday }
*
*
*/
@Nonnull
@ReturnsMutableObject("JAXB implementation style")
public List getRelativeToEasterSunday() {
if (relativeToEasterSunday == null) {
relativeToEasterSunday = new ArrayList();
}
return this.relativeToEasterSunday;
}
/**
* Created by ph-jaxb22-plugin -Xph-equalshashcode
*
*/
@Override
public boolean equals(final Object o) {
if (o == this) {
return true;
}
if ((o == null)||(!getClass().equals(o.getClass()))) {
return false;
}
final Holidays rhs = ((Holidays) o);
if (!EqualsHelper.equals(fixed, rhs.fixed)) {
return false;
}
if (!EqualsHelper.equals(relativeToFixed, rhs.relativeToFixed)) {
return false;
}
if (!EqualsHelper.equals(relativeToWeekdayInMonth, rhs.relativeToWeekdayInMonth)) {
return false;
}
if (!EqualsHelper.equals(fixedWeekday, rhs.fixedWeekday)) {
return false;
}
if (!EqualsHelper.equals(christianHoliday, rhs.christianHoliday)) {
return false;
}
if (!EqualsHelper.equals(islamicHoliday, rhs.islamicHoliday)) {
return false;
}
if (!EqualsHelper.equals(fixedWeekdayBetweenFixed, rhs.fixedWeekdayBetweenFixed)) {
return false;
}
if (!EqualsHelper.equals(fixedWeekdayRelativeToFixed, rhs.fixedWeekdayRelativeToFixed)) {
return false;
}
if (!EqualsHelper.equals(hinduHoliday, rhs.hinduHoliday)) {
return false;
}
if (!EqualsHelper.equals(hebrewHoliday, rhs.hebrewHoliday)) {
return false;
}
if (!EqualsHelper.equals(ethiopianOrthodoxHoliday, rhs.ethiopianOrthodoxHoliday)) {
return false;
}
if (!EqualsHelper.equals(relativeToEasterSunday, rhs.relativeToEasterSunday)) {
return false;
}
return true;
}
/**
* Created by ph-jaxb22-plugin -Xph-equalshashcode
*
*/
@Override
public int hashCode() {
return new HashCodeGenerator(this).append(fixed).append(relativeToFixed).append(relativeToWeekdayInMonth).append(fixedWeekday).append(christianHoliday).append(islamicHoliday).append(fixedWeekdayBetweenFixed).append(fixedWeekdayRelativeToFixed).append(hinduHoliday).append(hebrewHoliday).append(ethiopianOrthodoxHoliday).append(relativeToEasterSunday).getHashCode();
}
/**
* Created by ph-jaxb22-plugin -Xph-tostring
*
*/
@Override
public String toString() {
return new ToStringGenerator(this).append("fixed", fixed).append("relativeToFixed", relativeToFixed).append("relativeToWeekdayInMonth", relativeToWeekdayInMonth).append("fixedWeekday", fixedWeekday).append("christianHoliday", christianHoliday).append("islamicHoliday", islamicHoliday).append("fixedWeekdayBetweenFixed", fixedWeekdayBetweenFixed).append("fixedWeekdayRelativeToFixed", fixedWeekdayRelativeToFixed).append("hinduHoliday", hinduHoliday).append("hebrewHoliday", hebrewHoliday).append("ethiopianOrthodoxHoliday", ethiopianOrthodoxHoliday).append("relativeToEasterSunday", relativeToEasterSunday).getToString();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setFixed(
@Nullable
final List aList) {
fixed = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setRelativeToFixed(
@Nullable
final List aList) {
relativeToFixed = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setRelativeToWeekdayInMonth(
@Nullable
final List aList) {
relativeToWeekdayInMonth = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setFixedWeekday(
@Nullable
final List aList) {
fixedWeekday = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setChristianHoliday(
@Nullable
final List aList) {
christianHoliday = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setIslamicHoliday(
@Nullable
final List aList) {
islamicHoliday = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setFixedWeekdayBetweenFixed(
@Nullable
final List aList) {
fixedWeekdayBetweenFixed = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setFixedWeekdayRelativeToFixed(
@Nullable
final List aList) {
fixedWeekdayRelativeToFixed = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setHinduHoliday(
@Nullable
final List aList) {
hinduHoliday = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setHebrewHoliday(
@Nullable
final List aList) {
hebrewHoliday = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setEthiopianOrthodoxHoliday(
@Nullable
final List aList) {
ethiopianOrthodoxHoliday = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param aList
* The new list member to set. May be null
.
*/
public void setRelativeToEasterSunday(
@Nullable
final List aList) {
relativeToEasterSunday = aList;
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasFixedEntries() {
return (!getFixed().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoFixedEntries() {
return getFixed().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getFixedCount() {
return getFixed().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public Fixed getFixedAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getFixed().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addFixed(
@Nonnull
final Fixed elem) {
getFixed().add(elem);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasRelativeToFixedEntries() {
return (!getRelativeToFixed().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoRelativeToFixedEntries() {
return getRelativeToFixed().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getRelativeToFixedCount() {
return getRelativeToFixed().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public RelativeToFixed getRelativeToFixedAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getRelativeToFixed().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addRelativeToFixed(
@Nonnull
final RelativeToFixed elem) {
getRelativeToFixed().add(elem);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasRelativeToWeekdayInMonthEntries() {
return (!getRelativeToWeekdayInMonth().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoRelativeToWeekdayInMonthEntries() {
return getRelativeToWeekdayInMonth().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getRelativeToWeekdayInMonthCount() {
return getRelativeToWeekdayInMonth().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public RelativeToWeekdayInMonth getRelativeToWeekdayInMonthAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getRelativeToWeekdayInMonth().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addRelativeToWeekdayInMonth(
@Nonnull
final RelativeToWeekdayInMonth elem) {
getRelativeToWeekdayInMonth().add(elem);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasFixedWeekdayEntries() {
return (!getFixedWeekday().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoFixedWeekdayEntries() {
return getFixedWeekday().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getFixedWeekdayCount() {
return getFixedWeekday().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public FixedWeekdayInMonth getFixedWeekdayAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getFixedWeekday().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addFixedWeekday(
@Nonnull
final FixedWeekdayInMonth elem) {
getFixedWeekday().add(elem);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasChristianHolidayEntries() {
return (!getChristianHoliday().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoChristianHolidayEntries() {
return getChristianHoliday().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getChristianHolidayCount() {
return getChristianHoliday().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public ChristianHoliday getChristianHolidayAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getChristianHoliday().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addChristianHoliday(
@Nonnull
final ChristianHoliday elem) {
getChristianHoliday().add(elem);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasIslamicHolidayEntries() {
return (!getIslamicHoliday().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoIslamicHolidayEntries() {
return getIslamicHoliday().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getIslamicHolidayCount() {
return getIslamicHoliday().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public IslamicHoliday getIslamicHolidayAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getIslamicHoliday().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addIslamicHoliday(
@Nonnull
final IslamicHoliday elem) {
getIslamicHoliday().add(elem);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasFixedWeekdayBetweenFixedEntries() {
return (!getFixedWeekdayBetweenFixed().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoFixedWeekdayBetweenFixedEntries() {
return getFixedWeekdayBetweenFixed().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getFixedWeekdayBetweenFixedCount() {
return getFixedWeekdayBetweenFixed().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public FixedWeekdayBetweenFixed getFixedWeekdayBetweenFixedAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getFixedWeekdayBetweenFixed().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addFixedWeekdayBetweenFixed(
@Nonnull
final FixedWeekdayBetweenFixed elem) {
getFixedWeekdayBetweenFixed().add(elem);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasFixedWeekdayRelativeToFixedEntries() {
return (!getFixedWeekdayRelativeToFixed().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoFixedWeekdayRelativeToFixedEntries() {
return getFixedWeekdayRelativeToFixed().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getFixedWeekdayRelativeToFixedCount() {
return getFixedWeekdayRelativeToFixed().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public FixedWeekdayRelativeToFixed getFixedWeekdayRelativeToFixedAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getFixedWeekdayRelativeToFixed().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addFixedWeekdayRelativeToFixed(
@Nonnull
final FixedWeekdayRelativeToFixed elem) {
getFixedWeekdayRelativeToFixed().add(elem);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasHinduHolidayEntries() {
return (!getHinduHoliday().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoHinduHolidayEntries() {
return getHinduHoliday().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getHinduHolidayCount() {
return getHinduHoliday().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public HinduHoliday getHinduHolidayAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getHinduHoliday().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addHinduHoliday(
@Nonnull
final HinduHoliday elem) {
getHinduHoliday().add(elem);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasHebrewHolidayEntries() {
return (!getHebrewHoliday().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoHebrewHolidayEntries() {
return getHebrewHoliday().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getHebrewHolidayCount() {
return getHebrewHoliday().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public HebrewHoliday getHebrewHolidayAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getHebrewHoliday().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addHebrewHoliday(
@Nonnull
final HebrewHoliday elem) {
getHebrewHoliday().add(elem);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasEthiopianOrthodoxHolidayEntries() {
return (!getEthiopianOrthodoxHoliday().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoEthiopianOrthodoxHolidayEntries() {
return getEthiopianOrthodoxHoliday().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getEthiopianOrthodoxHolidayCount() {
return getEthiopianOrthodoxHoliday().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public EthiopianOrthodoxHoliday getEthiopianOrthodoxHolidayAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getEthiopianOrthodoxHoliday().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addEthiopianOrthodoxHoliday(
@Nonnull
final EthiopianOrthodoxHoliday elem) {
getEthiopianOrthodoxHoliday().add(elem);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if at least one item is contained, false
otherwise.
*/
public boolean hasRelativeToEasterSundayEntries() {
return (!getRelativeToEasterSunday().isEmpty());
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* true
if no item is contained, false
otherwise.
*/
public boolean hasNoRelativeToEasterSundayEntries() {
return getRelativeToEasterSunday().isEmpty();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @return
* The number of contained elements. Always ≥ 0.
*/
@Nonnegative
public int getRelativeToEasterSundayCount() {
return getRelativeToEasterSunday().size();
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param index
* The index to retrieve
* @return
* The element at the specified index. May be null
* @throws IndexOutOfBoundsException
* if the index is invalid!
*/
@Nullable
public RelativeToEasterSunday getRelativeToEasterSundayAtIndex(
@Nonnegative
final int index)
throws IndexOutOfBoundsException
{
return getRelativeToEasterSunday().get(index);
}
/**
* Created by ph-jaxb22-plugin -Xph-list-extension
*
* @param elem
* The element to be added. May not be null
.
*/
public void addRelativeToEasterSunday(
@Nonnull
final RelativeToEasterSunday elem) {
getRelativeToEasterSunday().add(elem);
}
}