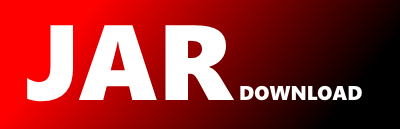
com.helger.html.jquery.IJQueryInvocation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ph-html-jquery Show documentation
Show all versions of ph-html-jquery Show documentation
Java library encapsulate the dynamic creation of JQuery based JS
/**
* Copyright (C) 2014-2015 Philip Helger (www.helger.com)
* philip[at]helger[dot]com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.helger.html.jquery;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.helger.commons.annotation.Nonempty;
import com.helger.html.css.ICSSClassProvider;
import com.helger.html.jscode.JSFieldRef;
/**
* This file is generated - do NOT edit!
* @author com.helger.html.jquery.supplementary.main.Main_IJQueryInvocation
* @param Implementation type
*/
public interface IJQueryInvocation >
{
/**
* Invoke an arbitrary function on this jQuery object.
*
* @param sMethod
* The method to be invoked. May neither be null
nor
* empty.
* @return A new jQuery invocation object. Never null
.
*/
@Nonnull
IMPLTYPE jqinvoke (@Nonnull @Nonempty String sMethod);
/**
* Adds a CSSClassProvider selector as a string argument.
*
* @param aArgument
* value to be added as an argument
* @return this
*/
@Nonnull
IMPLTYPE arg (@Nullable ICSSClassProvider aArgument);
/**
* Adds a JQuery selector as a string argument.
*
* @param aArgument
* value to be added as an argument
* @return this
*/
@Nonnull
IMPLTYPE arg (@Nullable IJQuerySelector aArgument);
/**
* Adds a JQuery selector list as a string argument.
*
* @param aArgument
* value to be added as an argument
* @return this
*/
@Nonnull
IMPLTYPE arg (@Nullable JQuerySelectorList aArgument);
// Properties of jQuery Object Instances
/**
* @return The invocation of the jQuery function context()
* @since jQuery 1.3
* @deprecated Deprecated since jQuery 1.10
*/
@Deprecated
@Nonnull
JSFieldRef context ();
/**
* @return The invocation of the jQuery field jquery
*/
@Nonnull
JSFieldRef jquery ();
/**
* @return The invocation of the jQuery field length()
*/
@Nonnull
JSFieldRef length ();
/**
* @return The invocation of the jQuery function add()
with return type jQuery
*/
@Nonnull
IMPLTYPE add ();
/**
* @return The invocation of the jQuery function addBack()
with return type jQuery
*/
@Nonnull
IMPLTYPE addBack ();
/**
* @return The invocation of the jQuery function addClass()
with return type jQuery
*/
@Nonnull
IMPLTYPE addClass ();
/**
* @return The invocation of the jQuery function after()
with return type jQuery
*/
@Nonnull
IMPLTYPE after ();
/**
* @return The invocation of the jQuery function ajaxComplete()
with return type jQuery
*/
@Nonnull
IMPLTYPE ajaxComplete ();
/**
* @return The invocation of the jQuery function ajaxError()
with return type jQuery
*/
@Nonnull
IMPLTYPE ajaxError ();
/**
* @return The invocation of the jQuery function ajaxSend()
with return type jQuery
*/
@Nonnull
IMPLTYPE ajaxSend ();
/**
* @return The invocation of the jQuery function ajaxStart()
with return type jQuery
*/
@Nonnull
IMPLTYPE ajaxStart ();
/**
* @return The invocation of the jQuery function ajaxStop()
with return type jQuery
*/
@Nonnull
IMPLTYPE ajaxStop ();
/**
* @return The invocation of the jQuery function ajaxSuccess()
with return type jQuery
*/
@Nonnull
IMPLTYPE ajaxSuccess ();
/**
* @return The invocation of the jQuery function andSelf()
with return type jQuery
* @deprecated Deprecated since jQuery 1.8
* @since jQuery 1.2
*/
@Deprecated
@Nonnull
IMPLTYPE andSelf ();
/**
* @return The invocation of the jQuery function animate()
with return type jQuery
*/
@Nonnull
IMPLTYPE animate ();
/**
* @return The invocation of the jQuery function append()
with return type jQuery
*/
@Nonnull
IMPLTYPE append ();
/**
* @return The invocation of the jQuery function appendTo()
with return type jQuery
*/
@Nonnull
IMPLTYPE appendTo ();
/**
* @return The invocation of the jQuery function attr()
with return type String or jQuery
*/
@Nonnull
IMPLTYPE attr ();
/**
* @return The invocation of the jQuery function before()
with return type jQuery
*/
@Nonnull
IMPLTYPE before ();
/**
* @return The invocation of the jQuery function bind()
with return type jQuery
*/
@Nonnull
IMPLTYPE bind ();
/**
* @return The invocation of the jQuery function blur()
with return type jQuery
*/
@Nonnull
IMPLTYPE blur ();
/**
* @return The invocation of the jQuery callbacks function add()
with return type Callbacks
* @since jQuery 1.7
*/
@Nonnull
IMPLTYPE callbacks_add ();
/**
* @return The invocation of the jQuery callbacks function disable()
with return type Callbacks
* @since jQuery 1.7
*/
@Nonnull
IMPLTYPE callbacks_disable ();
/**
* @return The invocation of the jQuery callbacks function disabled()
with return type Boolean
* @since jQuery 1.7
*/
@Nonnull
IMPLTYPE callbacks_disabled ();
/**
* @return The invocation of the jQuery callbacks function empty()
with return type Callbacks
* @since jQuery 1.7
*/
@Nonnull
IMPLTYPE callbacks_empty ();
/**
* @return The invocation of the jQuery callbacks function fire()
with return type Callbacks
* @since jQuery 1.7
*/
@Nonnull
IMPLTYPE callbacks_fire ();
/**
* @return The invocation of the jQuery callbacks function fireWith()
with return type Callbacks
*/
@Nonnull
IMPLTYPE callbacks_fireWith ();
/**
* @return The invocation of the jQuery callbacks function fired()
with return type Boolean
* @since jQuery 1.7
*/
@Nonnull
IMPLTYPE callbacks_fired ();
/**
* @return The invocation of the jQuery callbacks function has()
with return type Boolean
*/
@Nonnull
IMPLTYPE callbacks_has ();
/**
* @return The invocation of the jQuery callbacks function lock()
with return type Callbacks
* @since jQuery 1.7
*/
@Nonnull
IMPLTYPE callbacks_lock ();
/**
* @return The invocation of the jQuery callbacks function locked()
with return type Boolean
* @since jQuery 1.7
*/
@Nonnull
IMPLTYPE callbacks_locked ();
/**
* @return The invocation of the jQuery callbacks function remove()
with return type Callbacks
* @since jQuery 1.7
*/
@Nonnull
IMPLTYPE callbacks_remove ();
/**
* @return The invocation of the jQuery function change()
with return type jQuery
*/
@Nonnull
IMPLTYPE change ();
/**
* @return The invocation of the jQuery function children()
with return type jQuery
*/
@Nonnull
IMPLTYPE children ();
/**
* @return The invocation of the jQuery function clearQueue()
with return type jQuery
*/
@Nonnull
IMPLTYPE clearQueue ();
/**
* @return The invocation of the jQuery function click()
with return type jQuery
*/
@Nonnull
IMPLTYPE click ();
/**
* @return The invocation of the jQuery function clone()
with return type jQuery
*/
@Nonnull
IMPLTYPE _clone ();
/**
* Certain versions of this method are deprecated since jQuery 1.7
* @return The invocation of the jQuery function closest()
with return type jQuery or Array
*/
@Nonnull
IMPLTYPE closest ();
/**
* @return The invocation of the jQuery function contents()
with return type jQuery
* @since jQuery 1.2
*/
@Nonnull
IMPLTYPE contents ();
/**
* @return The invocation of the jQuery function css()
with return type String or jQuery
*/
@Nonnull
IMPLTYPE css ();
/**
* @return The invocation of the jQuery function data()
with return type jQuery or Object
*/
@Nonnull
IMPLTYPE data ();
/**
* @return The invocation of the jQuery function dblclick()
with return type jQuery
*/
@Nonnull
IMPLTYPE dblclick ();
/**
* @return The invocation of the jQuery deferred function always()
with return type Deferred
*/
@Nonnull
IMPLTYPE deferred_always ();
/**
* @return The invocation of the jQuery deferred function done()
with return type Deferred
*/
@Nonnull
IMPLTYPE deferred_done ();
/**
* @return The invocation of the jQuery deferred function fail()
with return type Deferred
*/
@Nonnull
IMPLTYPE deferred_fail ();
/**
* @return The invocation of the jQuery deferred function isRejected()
with return type Boolean
* @deprecated Deprecated since jQuery 1.7
* @since jQuery 1.5
*/
@Deprecated
@Nonnull
IMPLTYPE deferred_isRejected ();
/**
* @return The invocation of the jQuery deferred function isResolved()
with return type Boolean
* @deprecated Deprecated since jQuery 1.7
* @since jQuery 1.5
*/
@Deprecated
@Nonnull
IMPLTYPE deferred_isResolved ();
/**
* @return The invocation of the jQuery deferred function notify()
with return type Deferred
* @since jQuery 1.7
*/
@Nonnull
IMPLTYPE deferred_notify ();
/**
* @return The invocation of the jQuery deferred function notifyWith()
with return type Deferred
*/
@Nonnull
IMPLTYPE deferred_notifyWith ();
/**
* @return The invocation of the jQuery deferred function pipe()
with return type Promise
* @deprecated Deprecated since jQuery 1.8
*/
@Deprecated
@Nonnull
IMPLTYPE deferred_pipe ();
/**
* @return The invocation of the jQuery deferred function progress()
with return type Deferred
*/
@Nonnull
IMPLTYPE deferred_progress ();
/**
* @return The invocation of the jQuery deferred function promise()
with return type Promise
*/
@Nonnull
IMPLTYPE deferred_promise ();
/**
* @return The invocation of the jQuery deferred function reject()
with return type Deferred
*/
@Nonnull
IMPLTYPE deferred_reject ();
/**
* @return The invocation of the jQuery deferred function rejectWith()
with return type Deferred
*/
@Nonnull
IMPLTYPE deferred_rejectWith ();
/**
* @return The invocation of the jQuery deferred function resolve()
with return type Deferred
*/
@Nonnull
IMPLTYPE deferred_resolve ();
/**
* @return The invocation of the jQuery deferred function resolveWith()
with return type Deferred
*/
@Nonnull
IMPLTYPE deferred_resolveWith ();
/**
* @return The invocation of the jQuery deferred function state()
with return type String
* @since jQuery 1.7
*/
@Nonnull
IMPLTYPE deferred_state ();
/**
* @return The invocation of the jQuery deferred function then()
with return type Promise
*/
@Nonnull
IMPLTYPE deferred_then ();
/**
* @return The invocation of the jQuery function delay()
with return type jQuery
*/
@Nonnull
IMPLTYPE delay ();
/**
* @return The invocation of the jQuery function delegate()
with return type jQuery
*/
@Nonnull
IMPLTYPE delegate ();
/**
* @return The invocation of the jQuery function dequeue()
with return type jQuery
*/
@Nonnull
IMPLTYPE dequeue ();
/**
* @return The invocation of the jQuery function detach()
with return type jQuery
*/
@Nonnull
IMPLTYPE detach ();
/**
* @return The invocation of the jQuery function die()
with return type jQuery
* @deprecated Deprecated since jQuery 1.7
*/
@Deprecated
@Nonnull
IMPLTYPE die ();
/**
* @return The invocation of the jQuery function each()
with return type jQuery
*/
@Nonnull
IMPLTYPE each ();
/**
* @return The invocation of the jQuery function empty()
with return type jQuery
*/
@Nonnull
IMPLTYPE empty ();
/**
* @return The invocation of the jQuery function end()
with return type jQuery
*/
@Nonnull
IMPLTYPE end ();
/**
* @return The invocation of the jQuery function eq()
with return type jQuery
* @since jQuery 1.1.2
*/
@Nonnull
IMPLTYPE _eq ();
/**
* @return The invocation of the jQuery function error()
with return type jQuery
* @deprecated Deprecated since jQuery 1.8
*/
@Deprecated
@Nonnull
IMPLTYPE error ();
/**
* @return The invocation of the jQuery event function isDefaultPrevented()
with return type Boolean
* @since jQuery 1.3
*/
@Nonnull
IMPLTYPE event_isDefaultPrevented ();
/**
* @return The invocation of the jQuery event function isImmediatePropagationStopped()
with return type Boolean
* @since jQuery 1.3
*/
@Nonnull
IMPLTYPE event_isImmediatePropagationStopped ();
/**
* @return The invocation of the jQuery event function isPropagationStopped()
with return type Boolean
* @since jQuery 1.3
*/
@Nonnull
IMPLTYPE event_isPropagationStopped ();
/**
* @return The invocation of the jQuery event function preventDefault()
with return type undefined
*/
@Nonnull
IMPLTYPE event_preventDefault ();
/**
* @return The invocation of the jQuery event function stopImmediatePropagation()
with return type void
* @since jQuery 1.3
*/
@Nonnull
IMPLTYPE event_stopImmediatePropagation ();
/**
* @return The invocation of the jQuery event function stopPropagation()
with return type void
*/
@Nonnull
IMPLTYPE event_stopPropagation ();
/**
* @return The invocation of the jQuery function fadeIn()
with return type jQuery
*/
@Nonnull
IMPLTYPE fadeIn ();
/**
* @return The invocation of the jQuery function fadeOut()
with return type jQuery
*/
@Nonnull
IMPLTYPE fadeOut ();
/**
* @return The invocation of the jQuery function fadeTo()
with return type jQuery
*/
@Nonnull
IMPLTYPE fadeTo ();
/**
* @return The invocation of the jQuery function fadeToggle()
with return type jQuery
* @since jQuery 1.4.4
*/
@Nonnull
IMPLTYPE fadeToggle ();
/**
* @return The invocation of the jQuery function filter()
with return type jQuery
*/
@Nonnull
IMPLTYPE filter ();
/**
* @return The invocation of the jQuery function find()
with return type jQuery
*/
@Nonnull
IMPLTYPE find ();
/**
* @return The invocation of the jQuery function finish()
with return type jQuery
*/
@Nonnull
IMPLTYPE finish ();
/**
* @return The invocation of the jQuery function first()
with return type jQuery
* @since jQuery 1.4
*/
@Nonnull
IMPLTYPE first ();
/**
* @return The invocation of the jQuery function focus()
with return type jQuery
*/
@Nonnull
IMPLTYPE focus ();
/**
* @return The invocation of the jQuery function focusin()
with return type jQuery
*/
@Nonnull
IMPLTYPE focusin ();
/**
* @return The invocation of the jQuery function focusout()
with return type jQuery
*/
@Nonnull
IMPLTYPE focusout ();
/**
* @return The invocation of the jQuery function get()
with return type Element or Array
*/
@Nonnull
IMPLTYPE get ();
/**
* @return The invocation of the jQuery function has()
with return type jQuery
*/
@Nonnull
IMPLTYPE has ();
/**
* @return The invocation of the jQuery function hasClass()
with return type Boolean
* @since jQuery 1.2
*/
@Nonnull
IMPLTYPE hasClass ();
/**
* @return The invocation of the jQuery function height()
with return type Number or jQuery
*/
@Nonnull
IMPLTYPE height ();
/**
* @return The invocation of the jQuery function hide()
with return type jQuery
*/
@Nonnull
IMPLTYPE hide ();
/**
* @return The invocation of the jQuery function hover()
with return type jQuery
*/
@Nonnull
IMPLTYPE hover ();
/**
* @return The invocation of the jQuery function html()
with return type String or jQuery
*/
@Nonnull
IMPLTYPE html ();
/**
* @return The invocation of the jQuery function index()
with return type Number
*/
@Nonnull
IMPLTYPE index ();
/**
* @return The invocation of the jQuery function innerHeight()
with return type Number or jQuery
*/
@Nonnull
IMPLTYPE innerHeight ();
/**
* @return The invocation of the jQuery function innerWidth()
with return type Number or jQuery
*/
@Nonnull
IMPLTYPE innerWidth ();
/**
* @return The invocation of the jQuery function insertAfter()
with return type jQuery
*/
@Nonnull
IMPLTYPE insertAfter ();
/**
* @return The invocation of the jQuery function insertBefore()
with return type jQuery
*/
@Nonnull
IMPLTYPE insertBefore ();
/**
* @return The invocation of the jQuery function is()
with return type Boolean
*/
@Nonnull
IMPLTYPE is ();
/**
* @return The invocation of the jQuery function keydown()
with return type jQuery
*/
@Nonnull
IMPLTYPE keydown ();
/**
* @return The invocation of the jQuery function keypress()
with return type jQuery
*/
@Nonnull
IMPLTYPE keypress ();
/**
* @return The invocation of the jQuery function keyup()
with return type jQuery
*/
@Nonnull
IMPLTYPE keyup ();
/**
* @return The invocation of the jQuery function last()
with return type jQuery
* @since jQuery 1.4
*/
@Nonnull
IMPLTYPE last ();
/**
* @return The invocation of the jQuery function live()
with return type jQuery
* @deprecated Deprecated since jQuery 1.7
*/
@Deprecated
@Nonnull
IMPLTYPE live ();
/**
* Certain versions of this method are deprecated since jQuery 1.8
* @return The invocation of the jQuery function load()
with return type jQuery
*/
@Nonnull
IMPLTYPE load ();
/**
* @return The invocation of the jQuery function map()
with return type jQuery
* @since jQuery 1.2
*/
@Nonnull
IMPLTYPE map ();
/**
* @return The invocation of the jQuery function mousedown()
with return type jQuery
*/
@Nonnull
IMPLTYPE mousedown ();
/**
* @return The invocation of the jQuery function mouseenter()
with return type jQuery
*/
@Nonnull
IMPLTYPE mouseenter ();
/**
* @return The invocation of the jQuery function mouseleave()
with return type jQuery
*/
@Nonnull
IMPLTYPE mouseleave ();
/**
* @return The invocation of the jQuery function mousemove()
with return type jQuery
*/
@Nonnull
IMPLTYPE mousemove ();
/**
* @return The invocation of the jQuery function mouseout()
with return type jQuery
*/
@Nonnull
IMPLTYPE mouseout ();
/**
* @return The invocation of the jQuery function mouseover()
with return type jQuery
*/
@Nonnull
IMPLTYPE mouseover ();
/**
* @return The invocation of the jQuery function mouseup()
with return type jQuery
*/
@Nonnull
IMPLTYPE mouseup ();
/**
* @return The invocation of the jQuery function next()
with return type jQuery
*/
@Nonnull
IMPLTYPE next ();
/**
* @return The invocation of the jQuery function nextAll()
with return type jQuery
*/
@Nonnull
IMPLTYPE nextAll ();
/**
* @return The invocation of the jQuery function nextUntil()
with return type jQuery
*/
@Nonnull
IMPLTYPE nextUntil ();
/**
* @return The invocation of the jQuery function not()
with return type jQuery
*/
@Nonnull
IMPLTYPE _not ();
/**
* @return The invocation of the jQuery function off()
with return type jQuery
*/
@Nonnull
IMPLTYPE off ();
/**
* @return The invocation of the jQuery function offset()
with return type Object or jQuery
*/
@Nonnull
IMPLTYPE offset ();
/**
* @return The invocation of the jQuery function offsetParent()
with return type jQuery
* @since jQuery 1.2.6
*/
@Nonnull
IMPLTYPE offsetParent ();
/**
* @return The invocation of the jQuery function on()
with return type jQuery
*/
@Nonnull
IMPLTYPE on ();
/**
* @return The invocation of the jQuery function one()
with return type jQuery
*/
@Nonnull
IMPLTYPE one ();
/**
* @return The invocation of the jQuery function outerHeight()
with return type Number or jQuery
*/
@Nonnull
IMPLTYPE outerHeight ();
/**
* @return The invocation of the jQuery function outerWidth()
with return type Number or jQuery
*/
@Nonnull
IMPLTYPE outerWidth ();
/**
* @return The invocation of the jQuery function parent()
with return type jQuery
*/
@Nonnull
IMPLTYPE parent ();
/**
* @return The invocation of the jQuery function parents()
with return type jQuery
*/
@Nonnull
IMPLTYPE parents ();
/**
* @return The invocation of the jQuery function parentsUntil()
with return type jQuery
*/
@Nonnull
IMPLTYPE parentsUntil ();
/**
* @return The invocation of the jQuery function position()
with return type Object
* @since jQuery 1.2
*/
@Nonnull
IMPLTYPE position ();
/**
* @return The invocation of the jQuery function prepend()
with return type jQuery
*/
@Nonnull
IMPLTYPE prepend ();
/**
* @return The invocation of the jQuery function prependTo()
with return type jQuery
*/
@Nonnull
IMPLTYPE prependTo ();
/**
* @return The invocation of the jQuery function prev()
with return type jQuery
*/
@Nonnull
IMPLTYPE prev ();
/**
* @return The invocation of the jQuery function prevAll()
with return type jQuery
*/
@Nonnull
IMPLTYPE prevAll ();
/**
* @return The invocation of the jQuery function prevUntil()
with return type jQuery
*/
@Nonnull
IMPLTYPE prevUntil ();
/**
* @return The invocation of the jQuery function promise()
with return type Promise
*/
@Nonnull
IMPLTYPE promise ();
/**
* @return The invocation of the jQuery function prop()
with return type void or jQuery
*/
@Nonnull
IMPLTYPE prop ();
/**
* @return The invocation of the jQuery function pushStack()
with return type jQuery
*/
@Nonnull
IMPLTYPE pushStack ();
/**
* @return The invocation of the jQuery function queue()
with return type Array or jQuery
*/
@Nonnull
IMPLTYPE queue ();
/**
* @return The invocation of the jQuery function ready()
with return type jQuery
*/
@Nonnull
IMPLTYPE ready ();
/**
* @return The invocation of the jQuery function remove()
with return type jQuery
*/
@Nonnull
IMPLTYPE remove ();
/**
* @return The invocation of the jQuery function removeAttr()
with return type jQuery
*/
@Nonnull
IMPLTYPE removeAttr ();
/**
* @return The invocation of the jQuery function removeClass()
with return type jQuery
*/
@Nonnull
IMPLTYPE removeClass ();
/**
* @return The invocation of the jQuery function removeData()
with return type jQuery
*/
@Nonnull
IMPLTYPE removeData ();
/**
* @return The invocation of the jQuery function removeProp()
with return type jQuery
* @since jQuery 1.6
*/
@Nonnull
IMPLTYPE removeProp ();
/**
* @return The invocation of the jQuery function replaceAll()
with return type jQuery
* @since jQuery 1.2
*/
@Nonnull
IMPLTYPE replaceAll ();
/**
* @return The invocation of the jQuery function replaceWith()
with return type jQuery
*/
@Nonnull
IMPLTYPE replaceWith ();
/**
* @return The invocation of the jQuery function resize()
with return type jQuery
*/
@Nonnull
IMPLTYPE resize ();
/**
* @return The invocation of the jQuery function scroll()
with return type jQuery
*/
@Nonnull
IMPLTYPE scroll ();
/**
* @return The invocation of the jQuery function scrollLeft()
with return type Integer or jQuery
*/
@Nonnull
IMPLTYPE scrollLeft ();
/**
* @return The invocation of the jQuery function scrollTop()
with return type Integer or jQuery
*/
@Nonnull
IMPLTYPE scrollTop ();
/**
* @return The invocation of the jQuery function select()
with return type jQuery
*/
@Nonnull
IMPLTYPE select ();
/**
* @return The invocation of the jQuery function serialize()
with return type String
*/
@Nonnull
IMPLTYPE serialize ();
/**
* @return The invocation of the jQuery function serializeArray()
with return type Array
* @since jQuery 1.2
*/
@Nonnull
IMPLTYPE serializeArray ();
/**
* @return The invocation of the jQuery function show()
with return type jQuery
*/
@Nonnull
IMPLTYPE show ();
/**
* @return The invocation of the jQuery function siblings()
with return type jQuery
*/
@Nonnull
IMPLTYPE siblings ();
/**
* @return The invocation of the jQuery function size()
with return type Integer
* @deprecated Deprecated since jQuery 1.8
*/
@Deprecated
@Nonnull
IMPLTYPE size ();
/**
* @return The invocation of the jQuery function slice()
with return type jQuery
*/
@Nonnull
IMPLTYPE slice ();
/**
* @return The invocation of the jQuery function slideDown()
with return type jQuery
*/
@Nonnull
IMPLTYPE slideDown ();
/**
* @return The invocation of the jQuery function slideToggle()
with return type jQuery
*/
@Nonnull
IMPLTYPE slideToggle ();
/**
* @return The invocation of the jQuery function slideUp()
with return type jQuery
*/
@Nonnull
IMPLTYPE slideUp ();
/**
* @return The invocation of the jQuery function stop()
with return type jQuery
*/
@Nonnull
IMPLTYPE stop ();
/**
* @return The invocation of the jQuery function submit()
with return type jQuery
*/
@Nonnull
IMPLTYPE submit ();
/**
* @return The invocation of the jQuery function text()
with return type String or jQuery
*/
@Nonnull
IMPLTYPE text ();
/**
* @return The invocation of the jQuery function toArray()
with return type Array
* @since jQuery 1.4
*/
@Nonnull
IMPLTYPE toArray ();
/**
* Certain versions of this method are deprecated since jQuery 1.8
* @return The invocation of the jQuery function toggle()
with return type jQuery
*/
@Nonnull
IMPLTYPE toggle ();
/**
* @return The invocation of the jQuery function toggleClass()
with return type jQuery
*/
@Nonnull
IMPLTYPE toggleClass ();
/**
* @return The invocation of the jQuery function trigger()
with return type jQuery
*/
@Nonnull
IMPLTYPE trigger ();
/**
* @return The invocation of the jQuery function triggerHandler()
with return type Object
*/
@Nonnull
IMPLTYPE triggerHandler ();
/**
* @return The invocation of the jQuery function unbind()
with return type jQuery
*/
@Nonnull
IMPLTYPE unbind ();
/**
* @return The invocation of the jQuery function undelegate()
with return type jQuery
*/
@Nonnull
IMPLTYPE undelegate ();
/**
* @return The invocation of the jQuery function unload()
with return type jQuery
* @deprecated Deprecated since jQuery 1.8
*/
@Deprecated
@Nonnull
IMPLTYPE unload ();
/**
* @return The invocation of the jQuery function unwrap()
with return type jQuery
* @since jQuery 1.4
*/
@Nonnull
IMPLTYPE unwrap ();
/**
* @return The invocation of the jQuery function val()
with return type void or jQuery
*/
@Nonnull
IMPLTYPE val ();
/**
* @return The invocation of the jQuery function width()
with return type Number or jQuery
*/
@Nonnull
IMPLTYPE width ();
/**
* @return The invocation of the jQuery function wrap()
with return type jQuery
*/
@Nonnull
IMPLTYPE wrap ();
/**
* @return The invocation of the jQuery function wrapAll()
with return type jQuery
*/
@Nonnull
IMPLTYPE wrapAll ();
/**
* @return The invocation of the jQuery function wrapInner()
with return type jQuery
*/
@Nonnull
IMPLTYPE wrapInner ();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy